Articles tagged javascript
Secure Your Express App with OAuth 2.0, OIDC, and PKCE
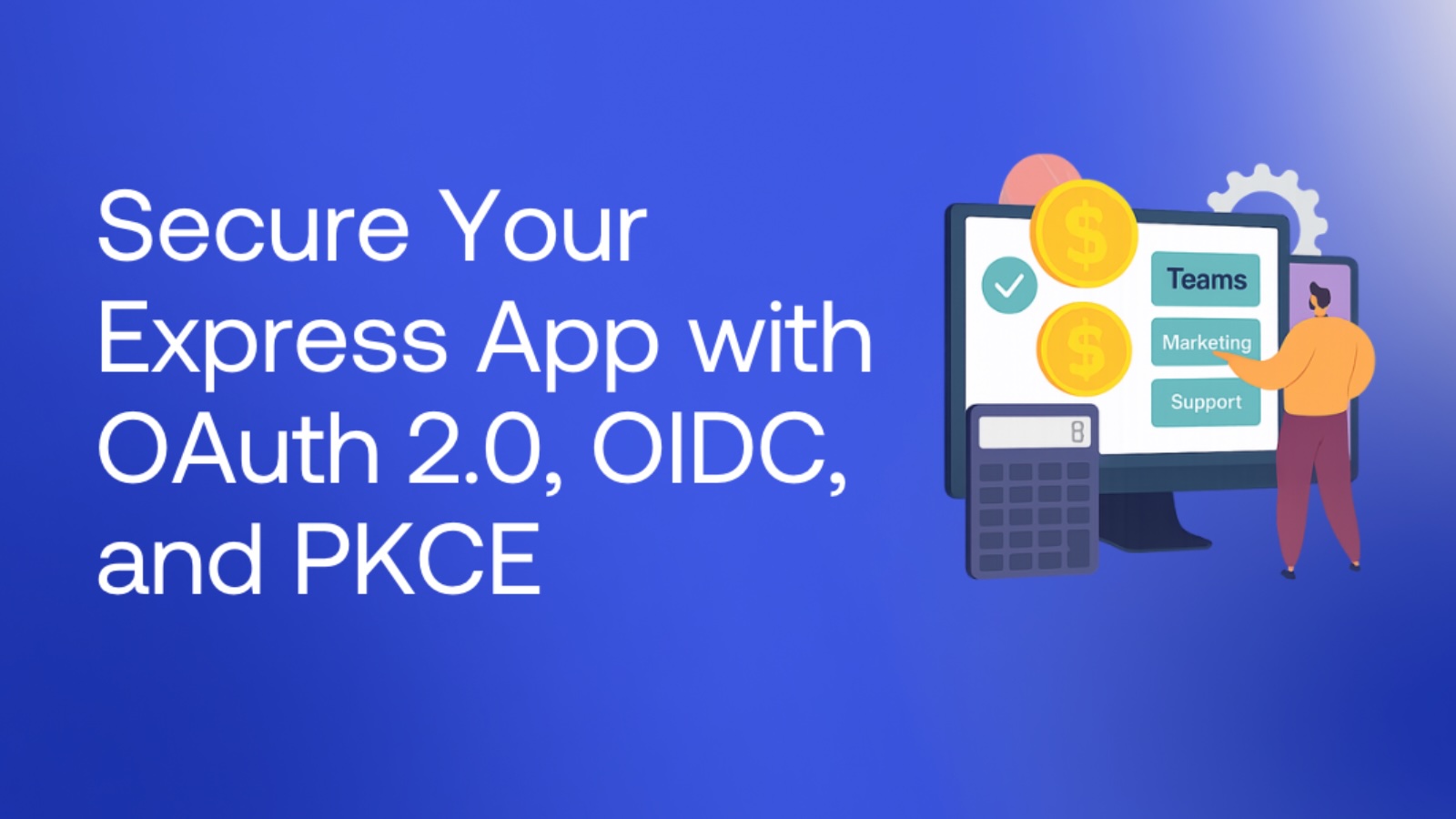
Every web application needs authentication, but building it yourself is risky and time-consuming. Instead of starting from scratch, you can integrate Okta to manage user identity and pair Passport with the openid-client library in Express to simplify and secure the login flow. In this tutorial, you’ll build a secure, role-based expense dashboard where users can view their expenses tailored to their team. Check out the complete source code on GitHub and get started without setting...
Superheroes, Startups, and Security: Sohail's Path to Developer Advocacy at Okta
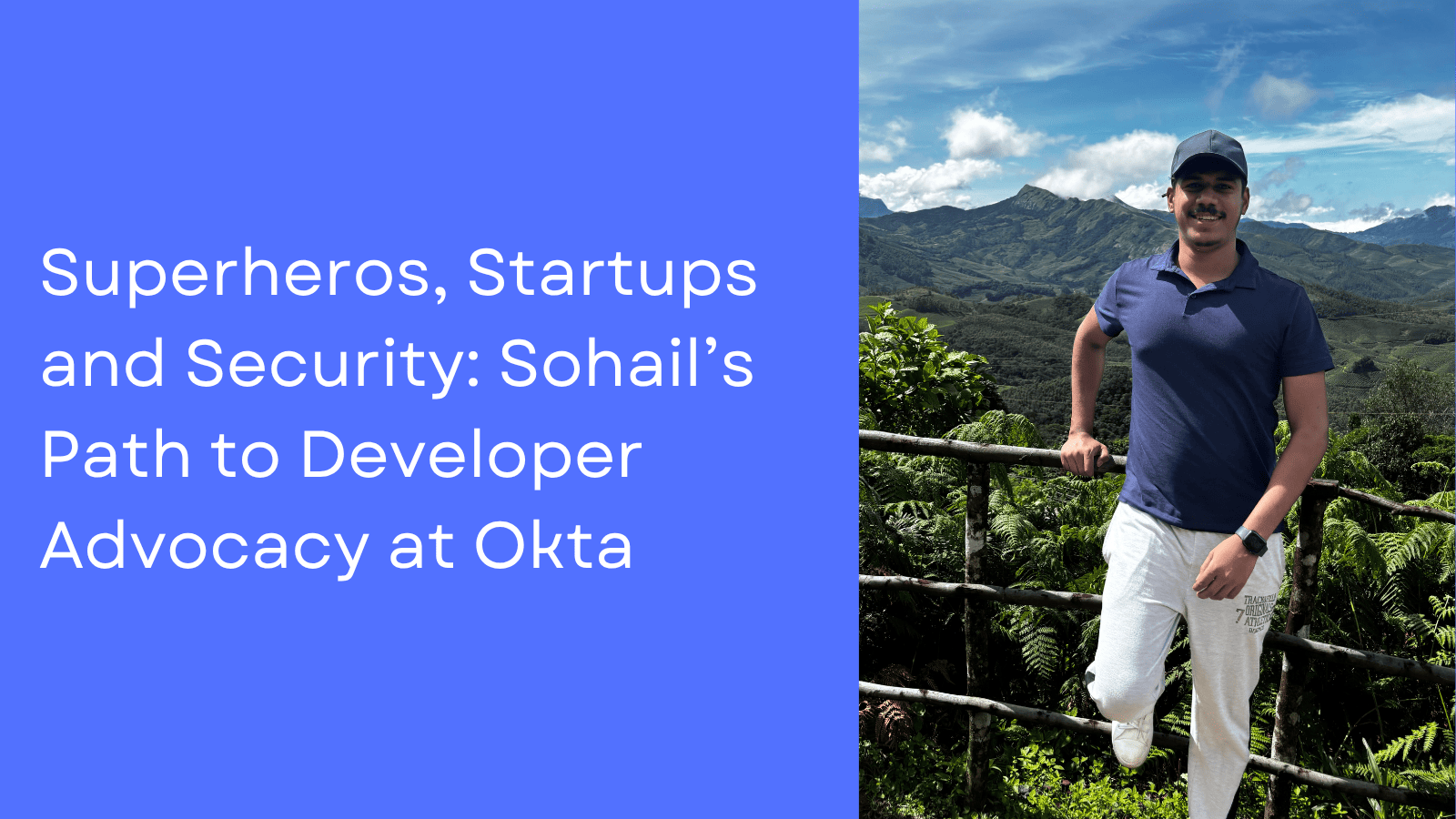
“Sometimes, all it takes is a spark of curiosity to ignite a lifelong journey.” - Unknown Hello OktaDev community 👋! Let me tell you a story – a story of dreams, passion, and the continuous pursuit of curiosity. My name is Sohail Pathan, and I’m thrilled to join Okta as a Senior Developer Advocate. It all started in Nagpur, a quaint city nestled in central India. As a child, I eagerly peered through my window,...
Changes Are Coming to the Okta Developer Edition Organizations
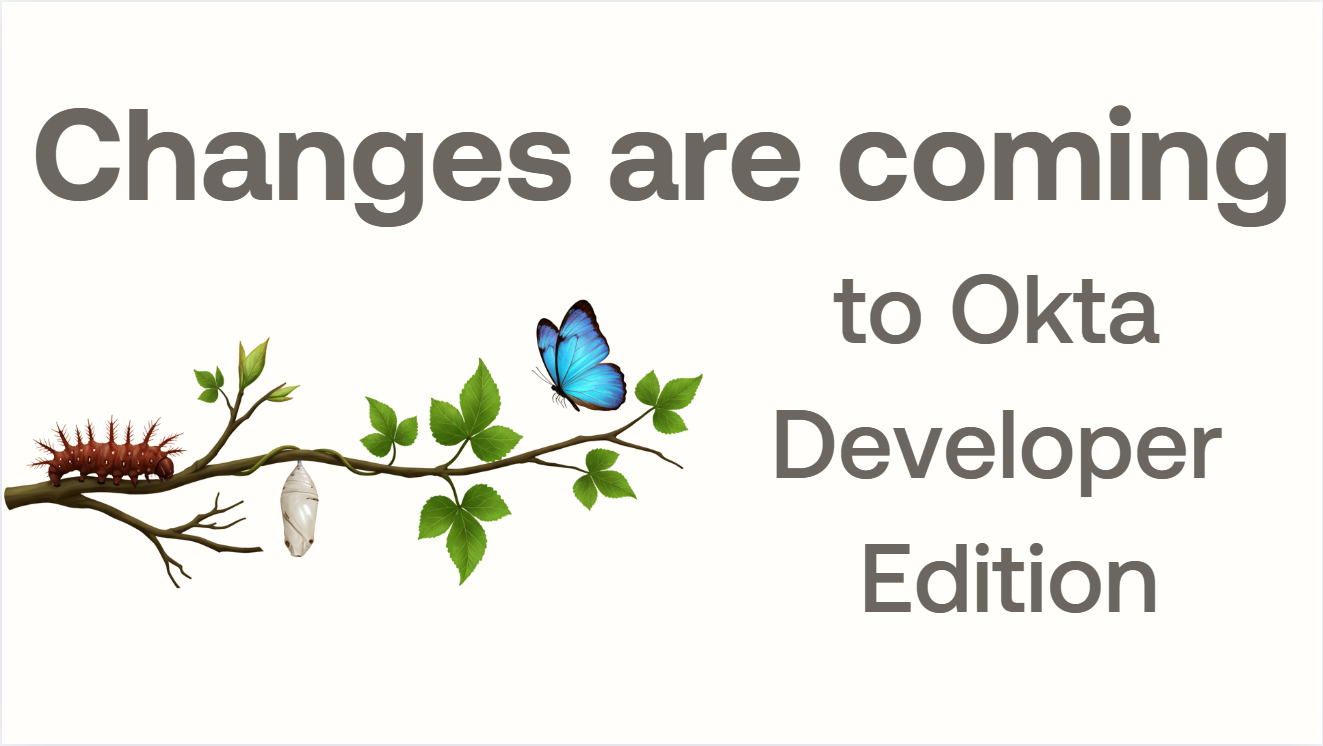
As part of Okta’s Secure Identity Commitment (OSIC) to lead the industry in the fight against identity attacks, we are making changes to improve our architecture related to developer organizations. On May 22, 2025, our new Integrator Free Plan will become the default organization type when you sign up on developer.okta.com. If you are actively using an Okta Developer Edition org, please create an Integrator organization and migrate to it. The Okta Developer Edition terms...
Astronomy Geek to Oktanaut: Landing as a Dev Advocate at Okta
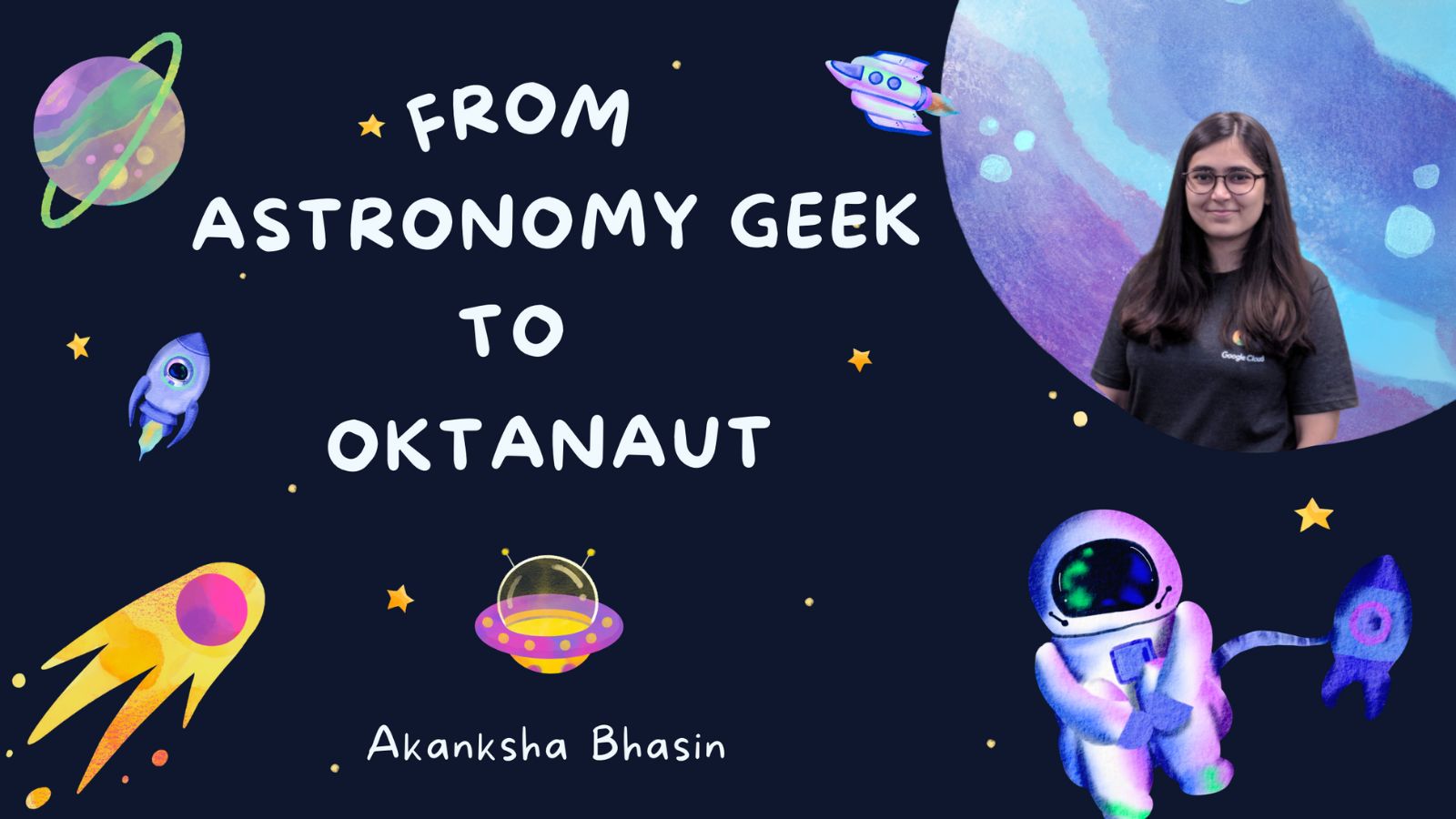
I’m Akanksha Bhasin, and I’m all about building community for developers. I come from a developer background, and for over six years, I’ve been building and growing communities for developers. On top of that, I’ve spent the last four years diving into Developer Relations. I’ve had the chance to work with some fast-growing companies, helping them build their communities worldwide. That means getting the word out about emerging tech to developers, organizing significant events and...
Supporting Devs Through Advocacy
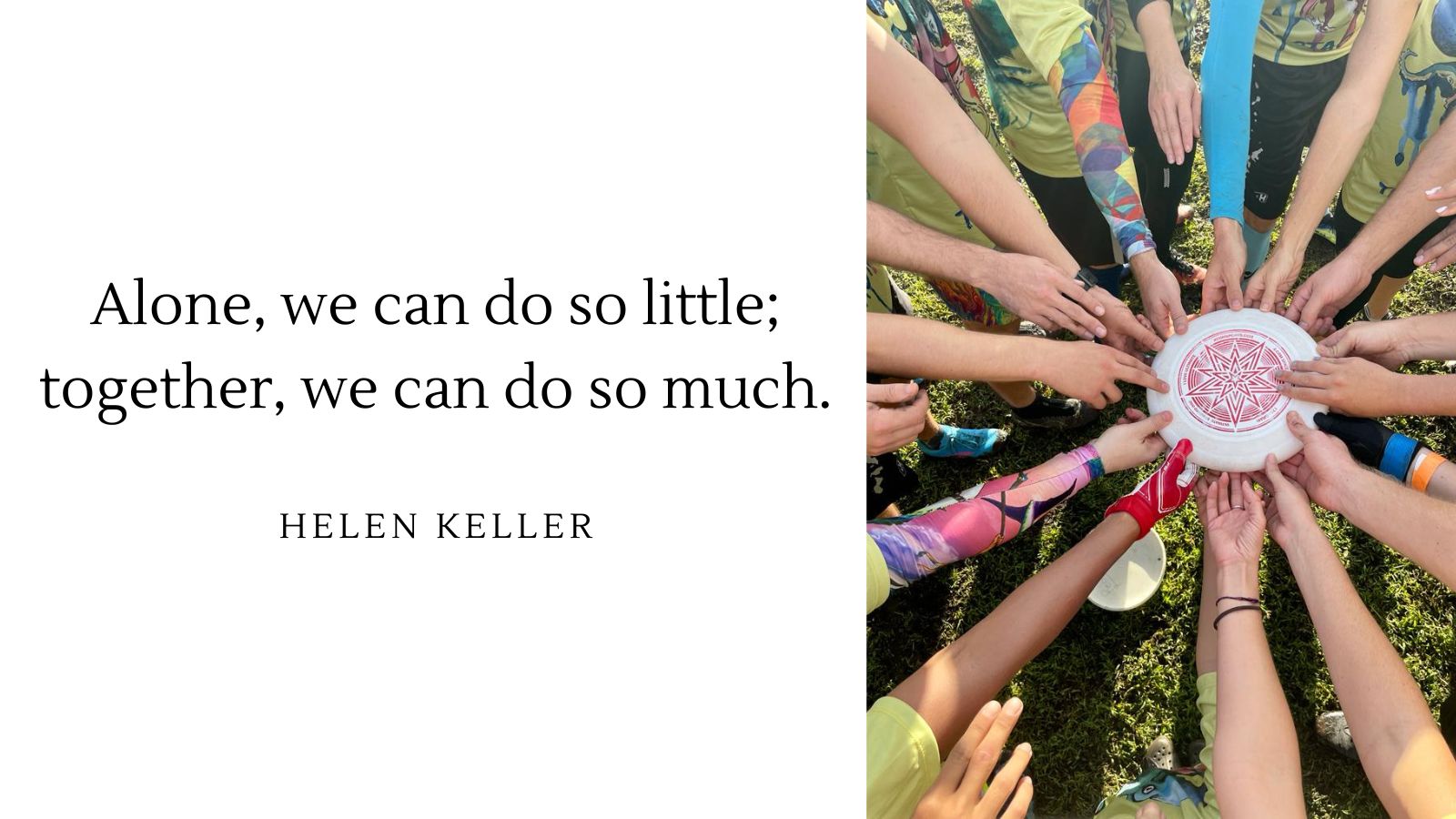
Supporting developers is my modus operandi. I’ve been with Okta for three years, formerly as a Developer Support Engineer and now as a Developer Advocate. Before joining Okta, I graduated from Hackbright Academy, an all-women boot camp based in San Francisco. I learned to think like a programmer through coding in Python and Javascript. My full-stack capstone project was a web application that tracked sugar intake, inspired by the community health service volunteer work I...
How to Build a Secure React and Fastify API App
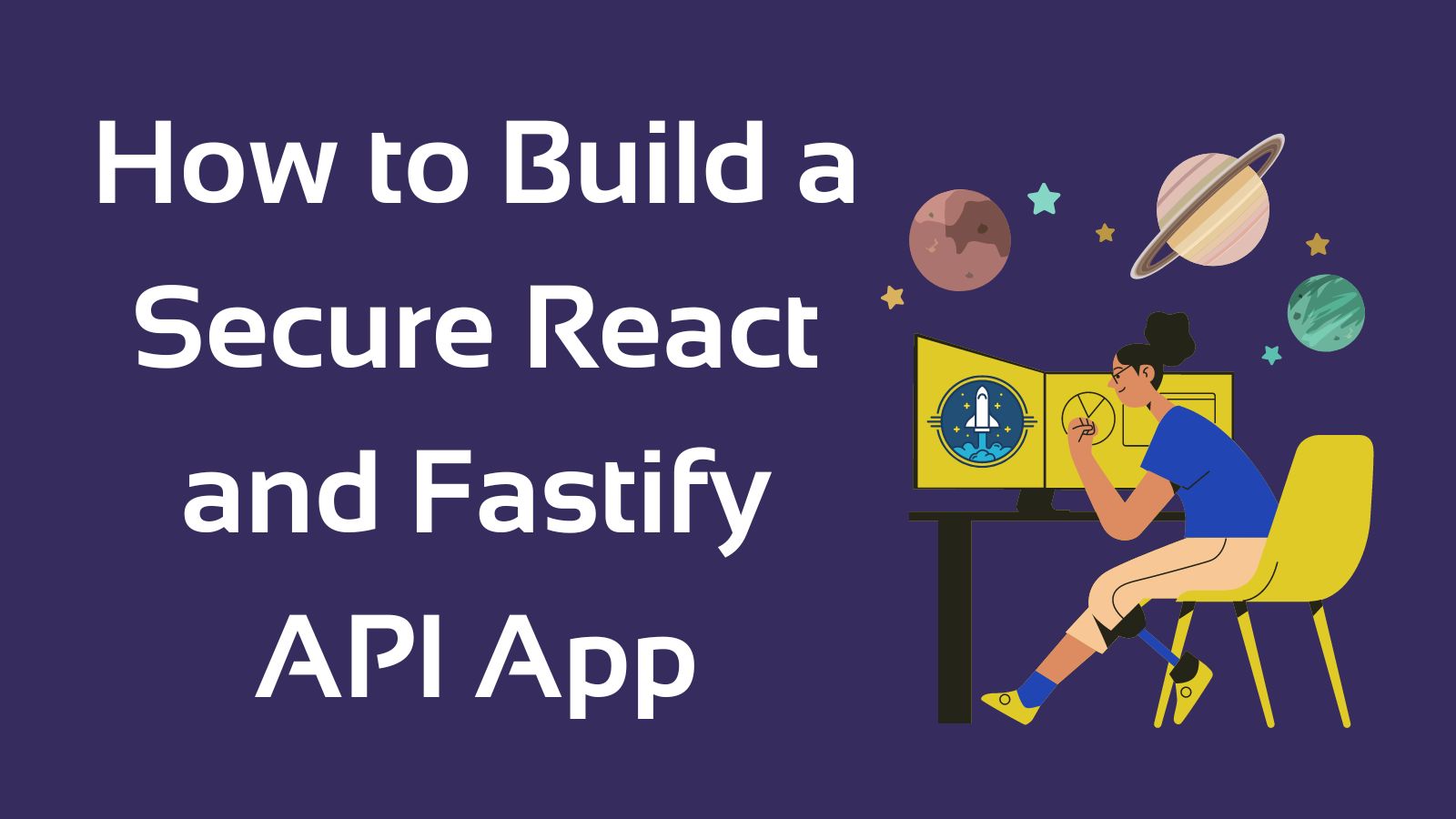
The National Aeronautics and Space Administration (NASA) is an independent agency of the US federal government, responsible for space exploration and research, with field facilities across the United States. In this tutorial, we’ll set up an app to keep track of what NASA facilities we’ve visited and which ones we still want to check out. Our app will be a monorepo with Okta authentication, using React for the frontend and Fastify for the backend. Fastify...
Quick JavaScript Authentication with OktaDev Schematics
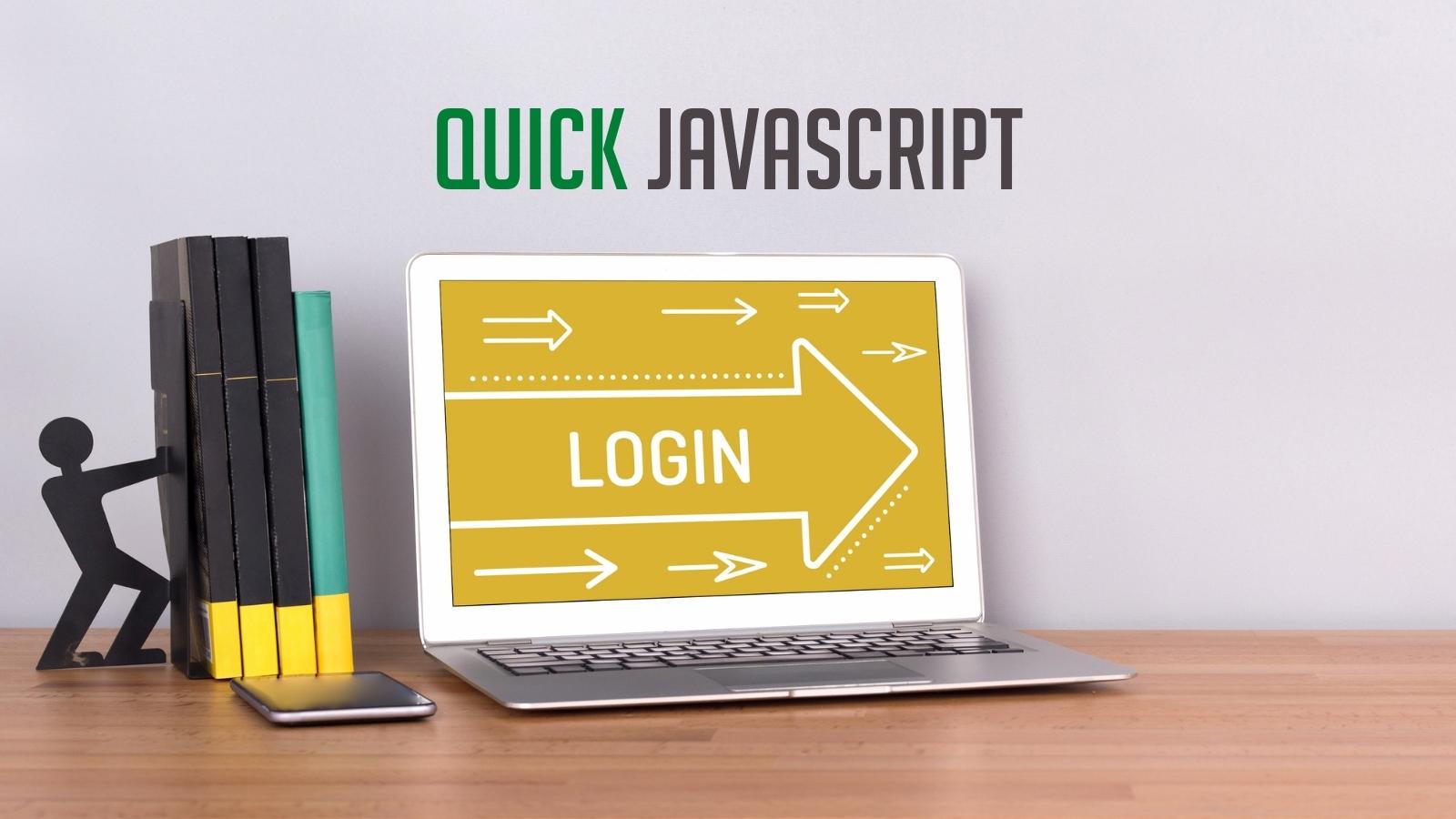
Hello, My name is Matt Raible, and I am a developer advocate at Okta. In early 2019, I created OktaDev Schematics to simplify my life when doing Angular demos. But let me step back a bit first. I’ve worked at Okta for over five years; before that, I was an independent consultant for 20 years, mainly doing Java and web development for clients. I’ve learned a lot about OpenID Connect (OIDC) and Okta’s JavaScript SDKs...
Practical Uses of Dependency Injection in Angular
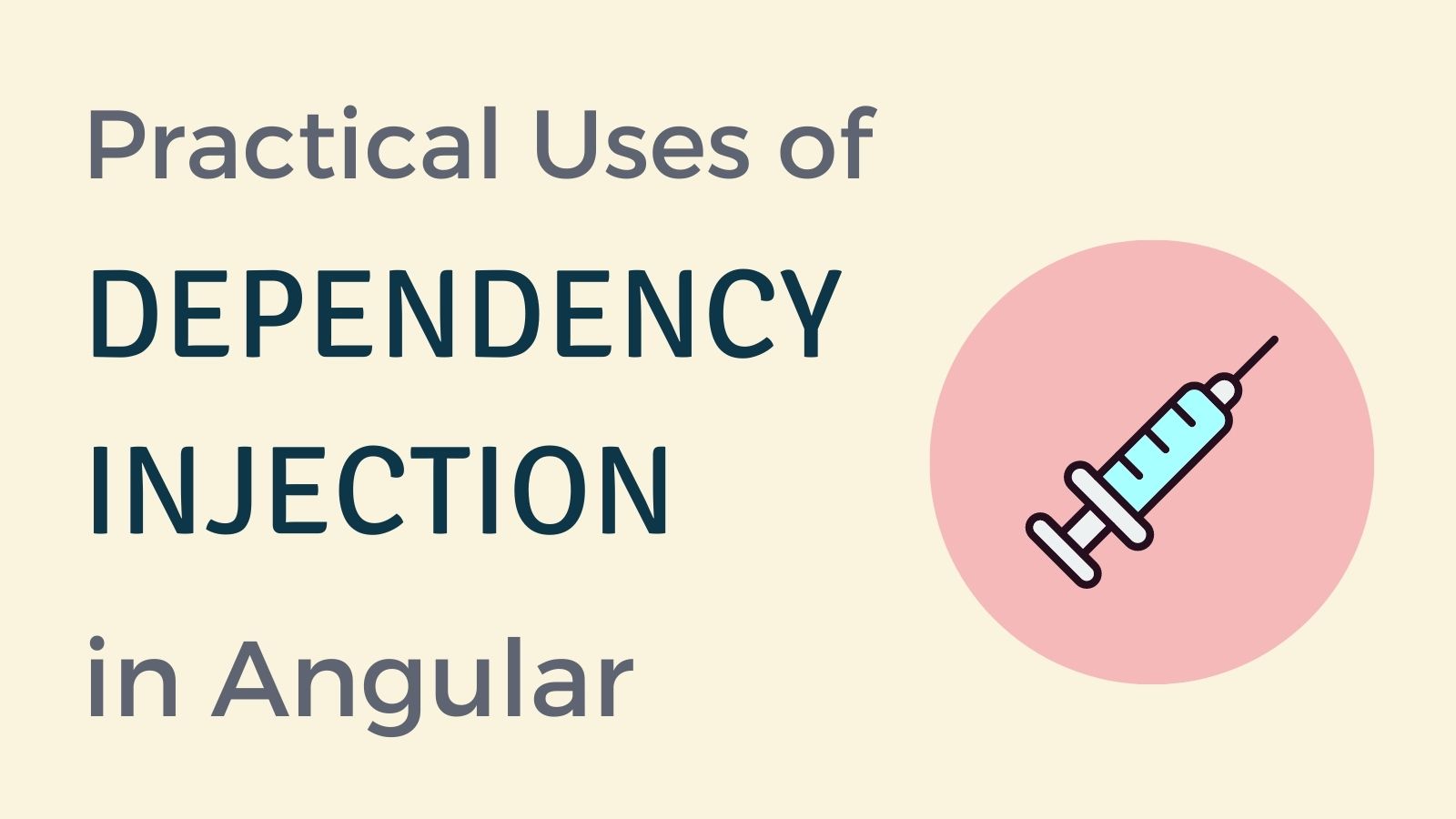
Angular has an extensive system that uses *providers” to add and configure dependencies to the application you’re building. To create providers, you use the built-in Dependency Injection (DI) system. This post will cover Angular’s powerful DI system at a high level and demonstrate a few practical use cases and strategies for configuring your dependencies. Let’s get practical! Table of Contents Quick overview of Dependency Injection Angular’s Dependency Injection system Injection tokens in Angular Configuring providers...
Use Redux to Manage Authenticated State in a React App
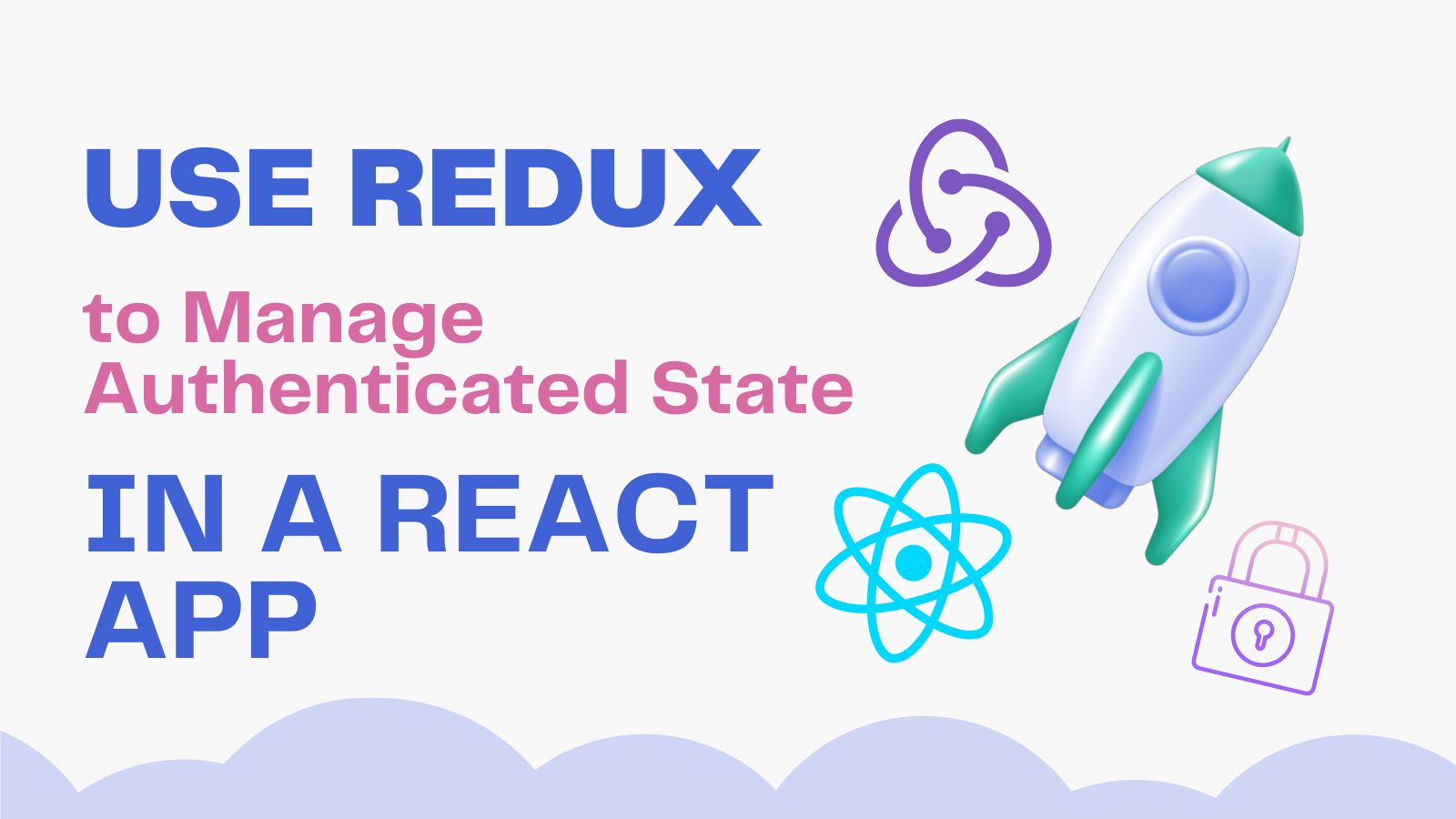
There are a myriad of state management options available for React. React provides the option of using the built-in Context for when you have a nested tree of components that share a state. There is also a built-in useState hook that will allow you to set local state for a component. For more complex scenarios where you need a single source of truth that changes frequently and is shared across large sections of your application,...
Build a Simple CRUD App with Spring Boot and Vue.js
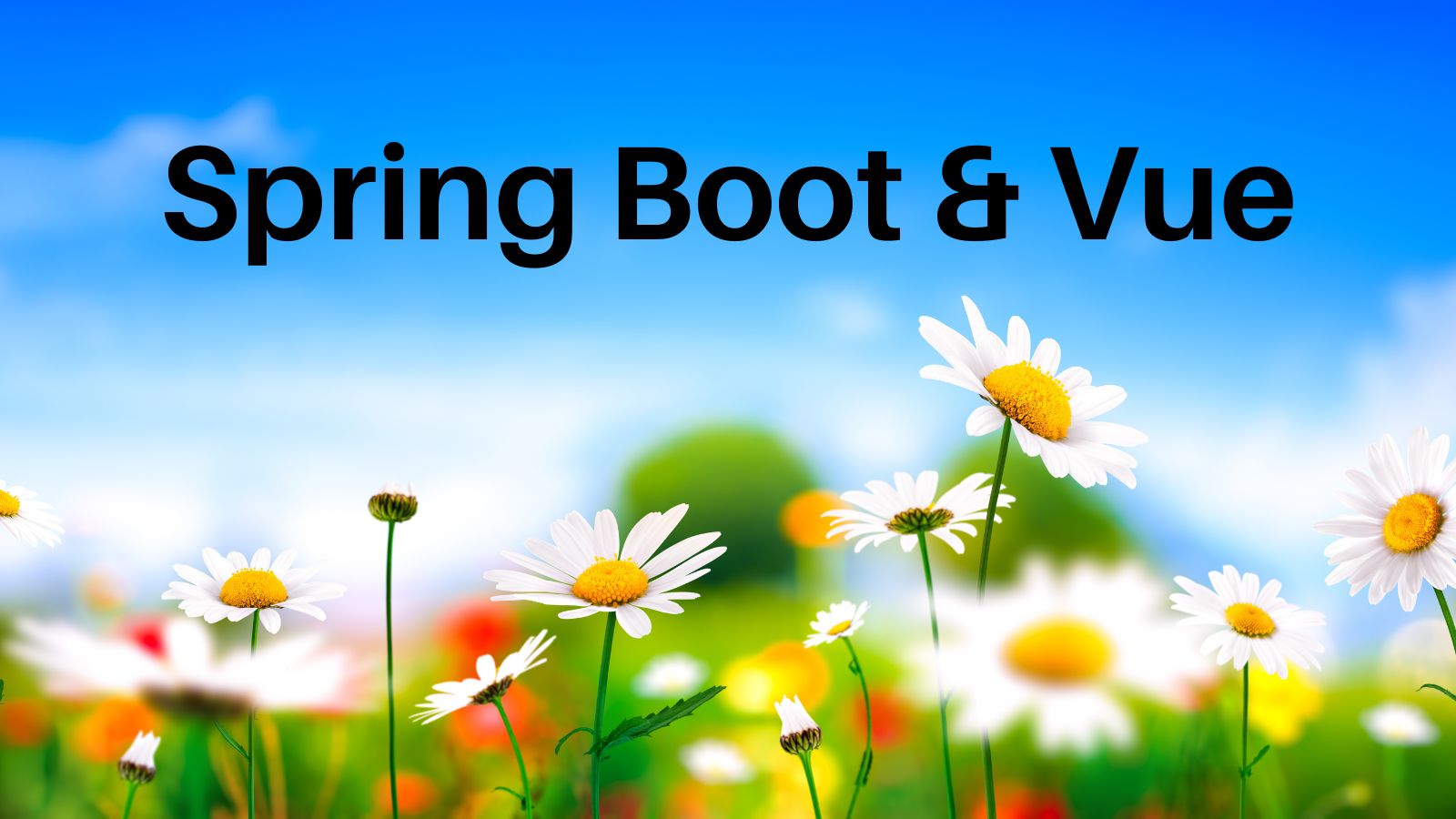
You will use Vue and Spring Boot to build a todo list web application. The application will include CRUD abilities, meaning that you can create, read, update, and delete the todo items on the Spring Boot API via the client. The Vue frontend client will use the Quasar framework for the presentation. OAuth 2.0 and OpenID Connect (OIDC) will secure the Spring Boot API and the Vue client, initially by using Okta as the security...
How to Build and Deploy a Serverless React App on Azure
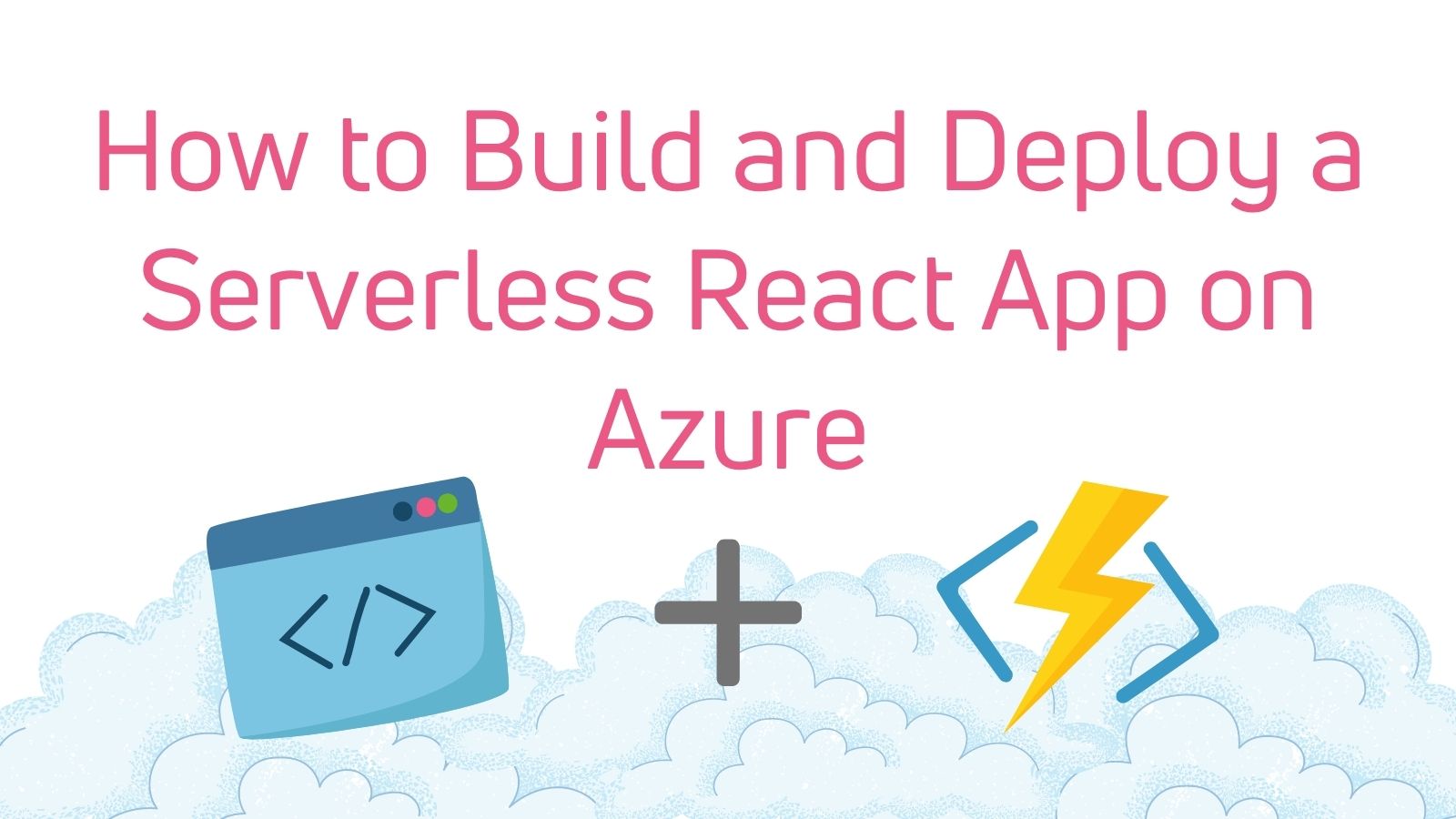
Microsoft’s Azure platform has as many high-tech products as anyone could ever want, including the Azure Static Web Apps service. As the name suggests, the platform hosts static web apps that don’t require a back end. Azure supports React, Angular, Vue, Gatsby, and many more, out of the box. However, you may run into situations where you want some back-end support, such as when you need the backend to run one or two API calls....
Creating a TypeScript React Application with Vite
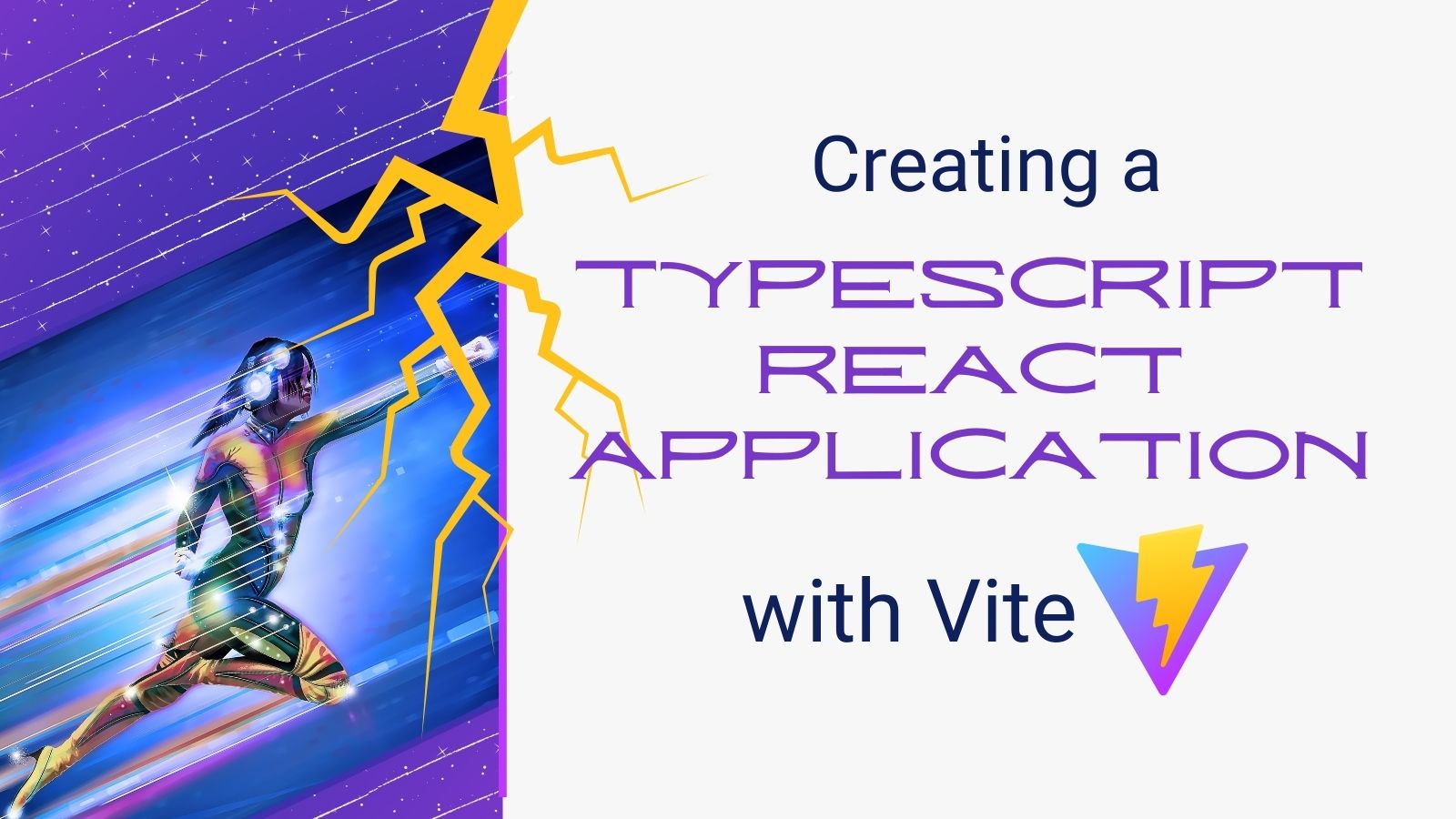
Front-end applications are becoming ever bigger and more complex. It is not uncommon for a React app to have hundreds or even thousands of components. As the project size increases, build times become increasingly important. In large projects, you may have to wait up to a minute for the code to be translated and bundled into a production package run in the browser. The compile and load times for the development server are also a...
Build and Deploy a Node.js App to Heroku
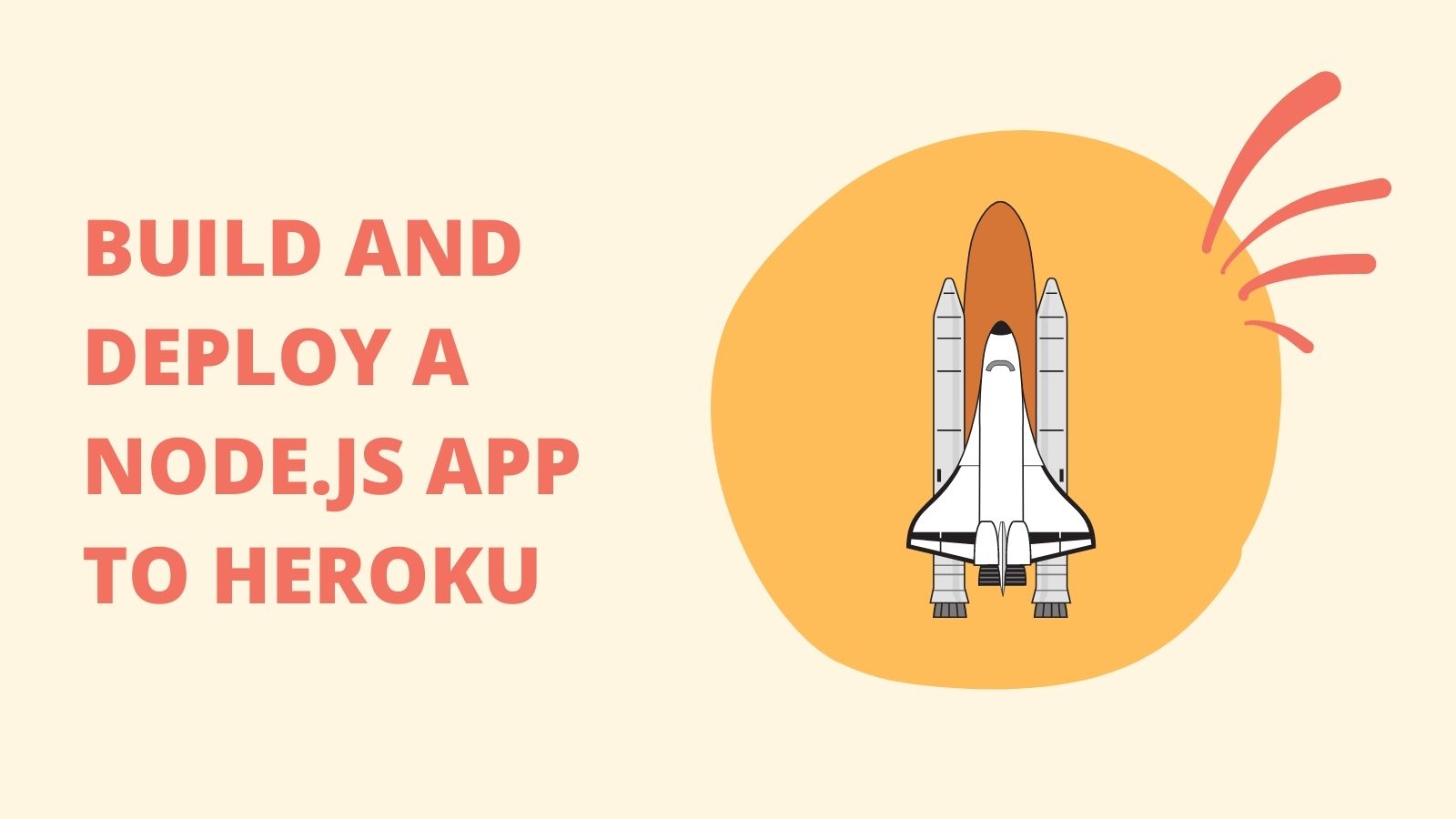
Heroku is a platform as a service (PaaS) that supports many languages. Initially, it supported only Ruby sites but now supports various languages, including JavaScript with Node.js. Heroku also has Docker support so that you can deploy just about anything to it. This tutorial will teach you how to build a small application using the Express framework for Node.js. You will then secure that application using Okta by integrating the Okta OIDC middleware with your...
Three Ways to Configure Modules in Your Angular App
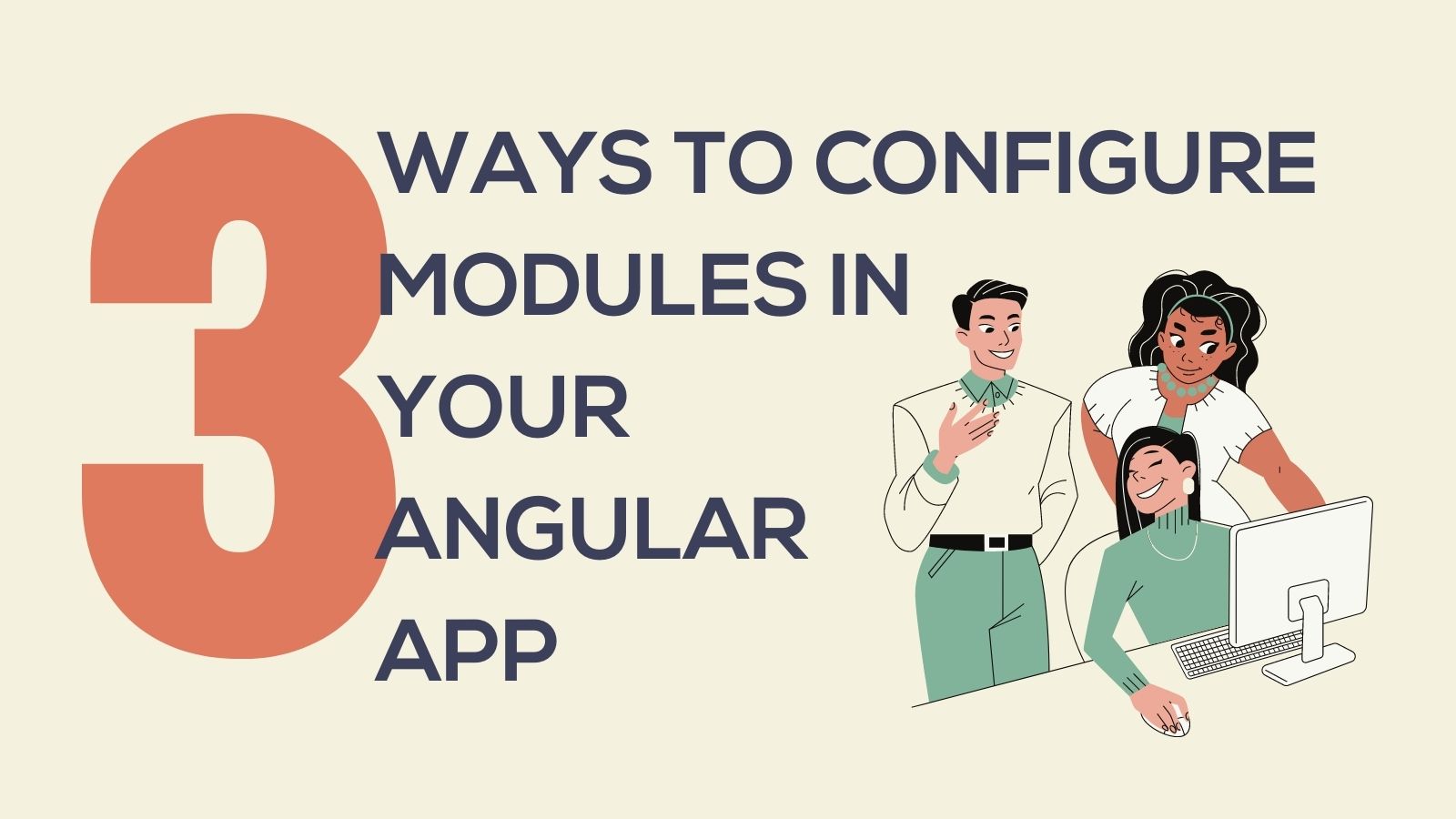
Configurations are part of a developer’s life. Configuration data is information your app needs to run and may include tokens for third-party systems or settings you pass into libraries. There are different ways to load configuration data as part of application initialization in Angular. Your requirements for configuration data might change based on needs. For example, you may have one unchanging configuration for your app, or you may need a different configuration based on the...
Create a Secure Serverless Application with FaunaDB
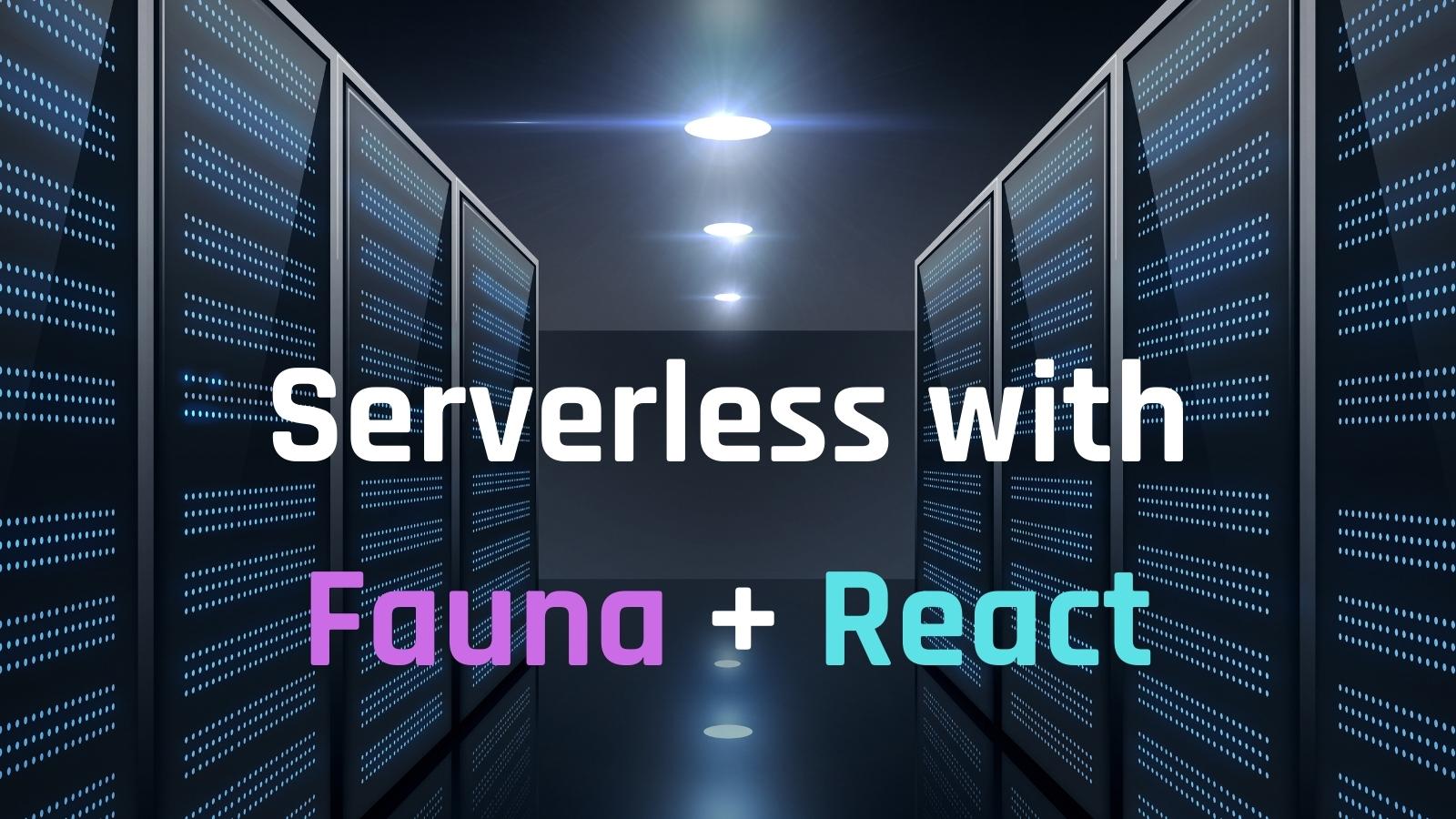
Fauna is a transactional database that is delivered to your application as a secure cloud API. Fauna hosts your database and then allows your application to make calls against it. Typically, to secure such an API, you would establish a relationship between your users and the database in your code. This would mean signing in your users using your authentication provider, in this case, Okta. Then you would need to convert that user to an...
Add OpenID Connect to Angular Apps Quickly
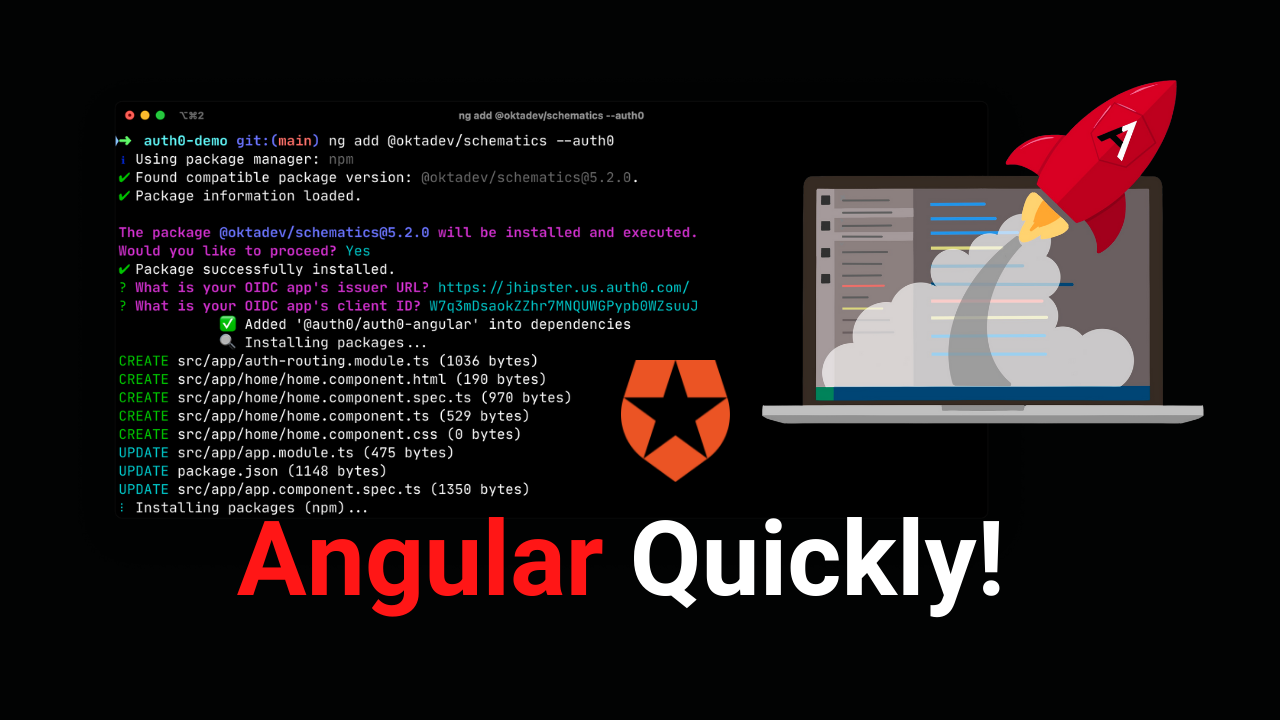
AngularJS 1.0 was released in October 2010. At the time, it was considered one of the most revolutionary and popular web frameworks ever to see the light of day. Developers loved it, and created many apps with it. However, as a pioneer in the JS framework space, AngularJS had some growing pains and significant issues. The team went back to the drawing board for a major breaking release with Angular 2. It took two years...
A Comparison of Cookies and Tokens for Secure Authentication
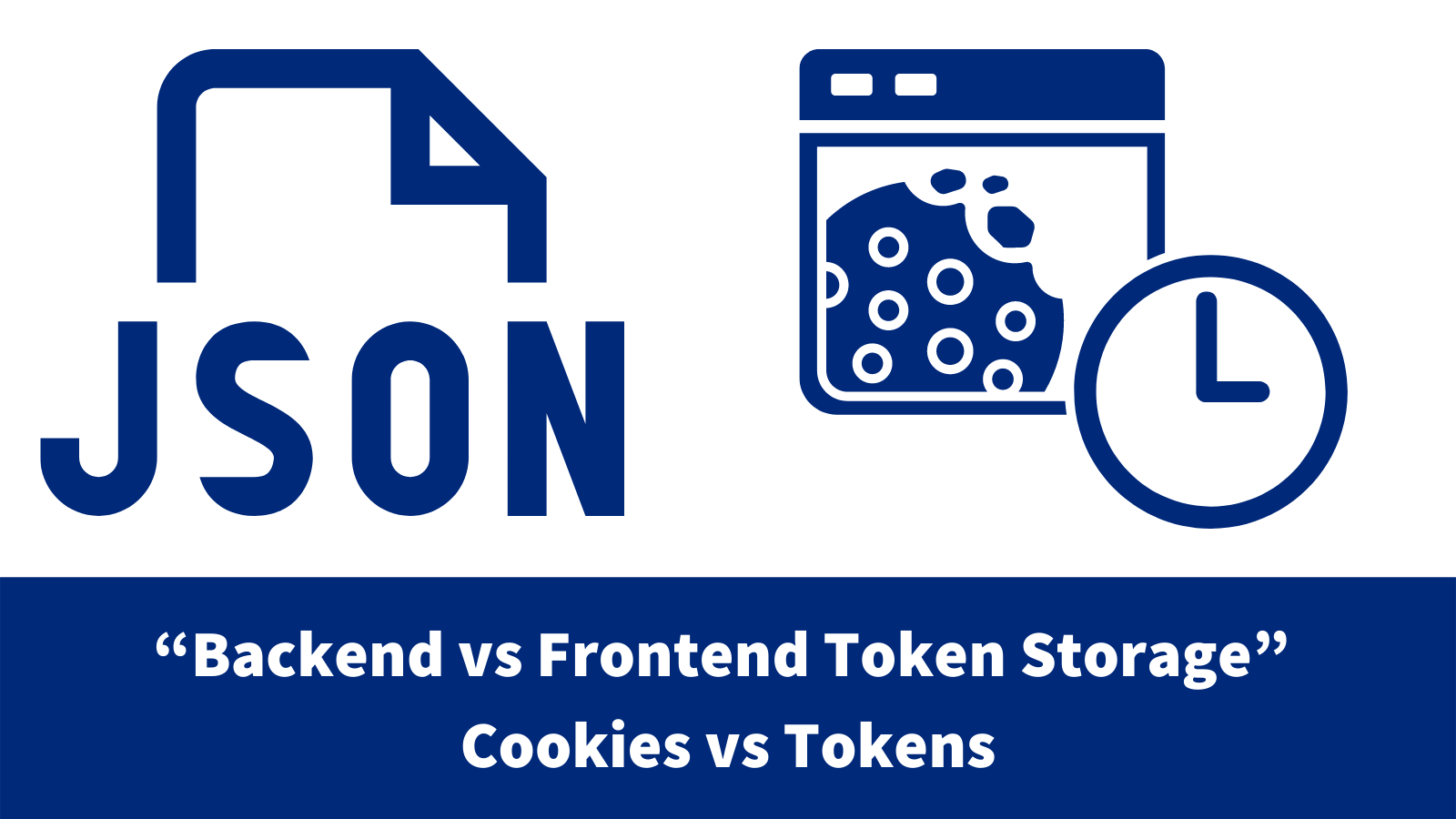
Access control in websites and web applications is a top priority for security, but how you set up access depends on how you store the data to be authenticated. This, in turn, enables user authorization. Cookies and tokens are two common ways of setting up authentication. Cookies are chunks of data created by the server and sent to the client for communication purposes. Tokens, usually referring to JSON Web Tokens (JWTs), are signed credentials encoded...
How to Build a Website With Eleventy
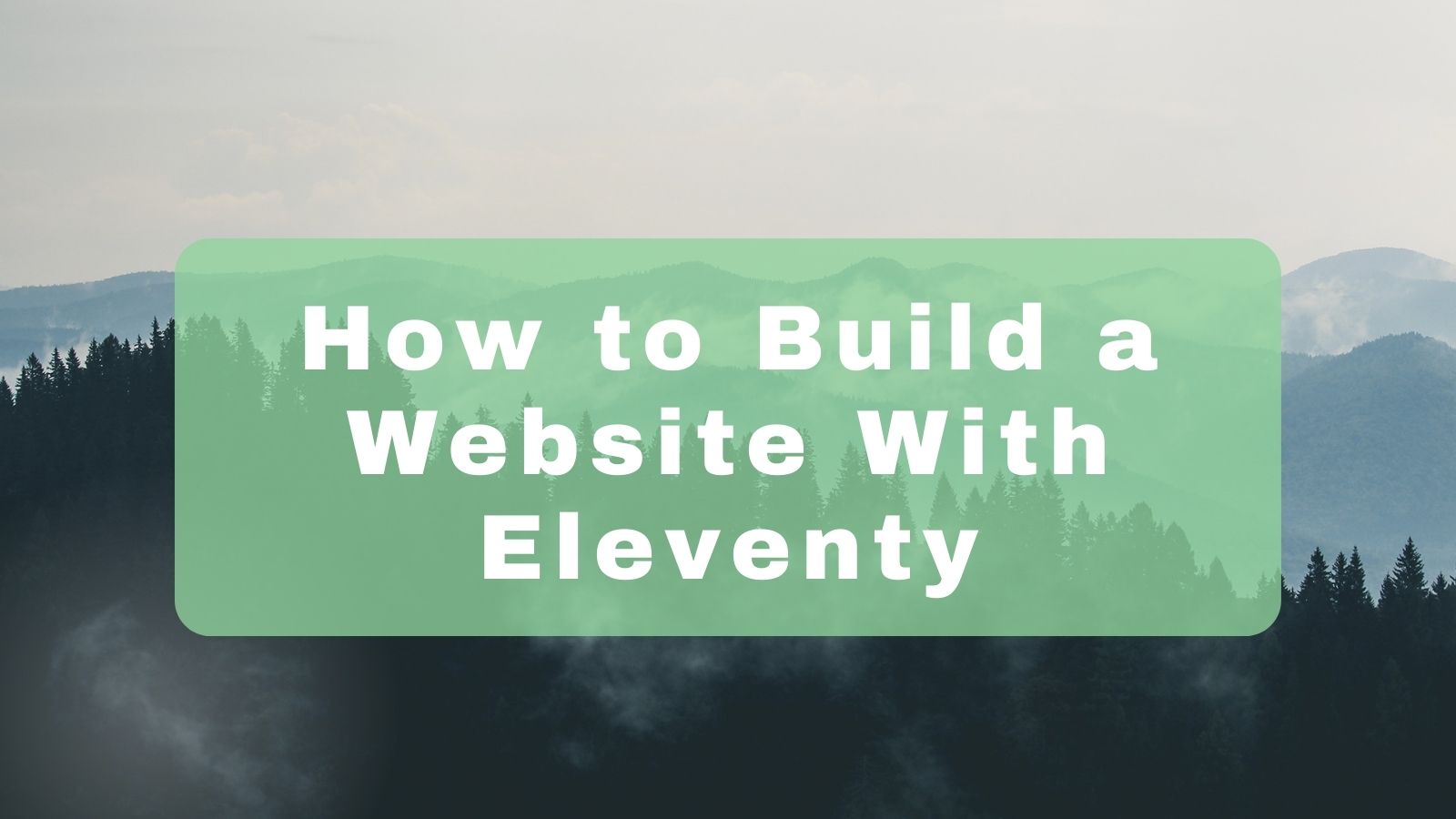
11ty is a fantastic tool for quickly creating static sites using a variety of templating languages. 11ty makes designing and developing static sites simple. It supports HTML, Markdown, JavaScript, Nunjucks, Handlebars, and many other template styles, along with built-in support for layouts, pagination, and slugify. 11ty aims to compete against other frameworks such as Jekyll, Hugo, Hexo, Gatsby, and Nuxt. The framework is indeed as simple and powerful as its creators claim. This tutorial will...
Simplify Building Vue Applications with NuxtJS
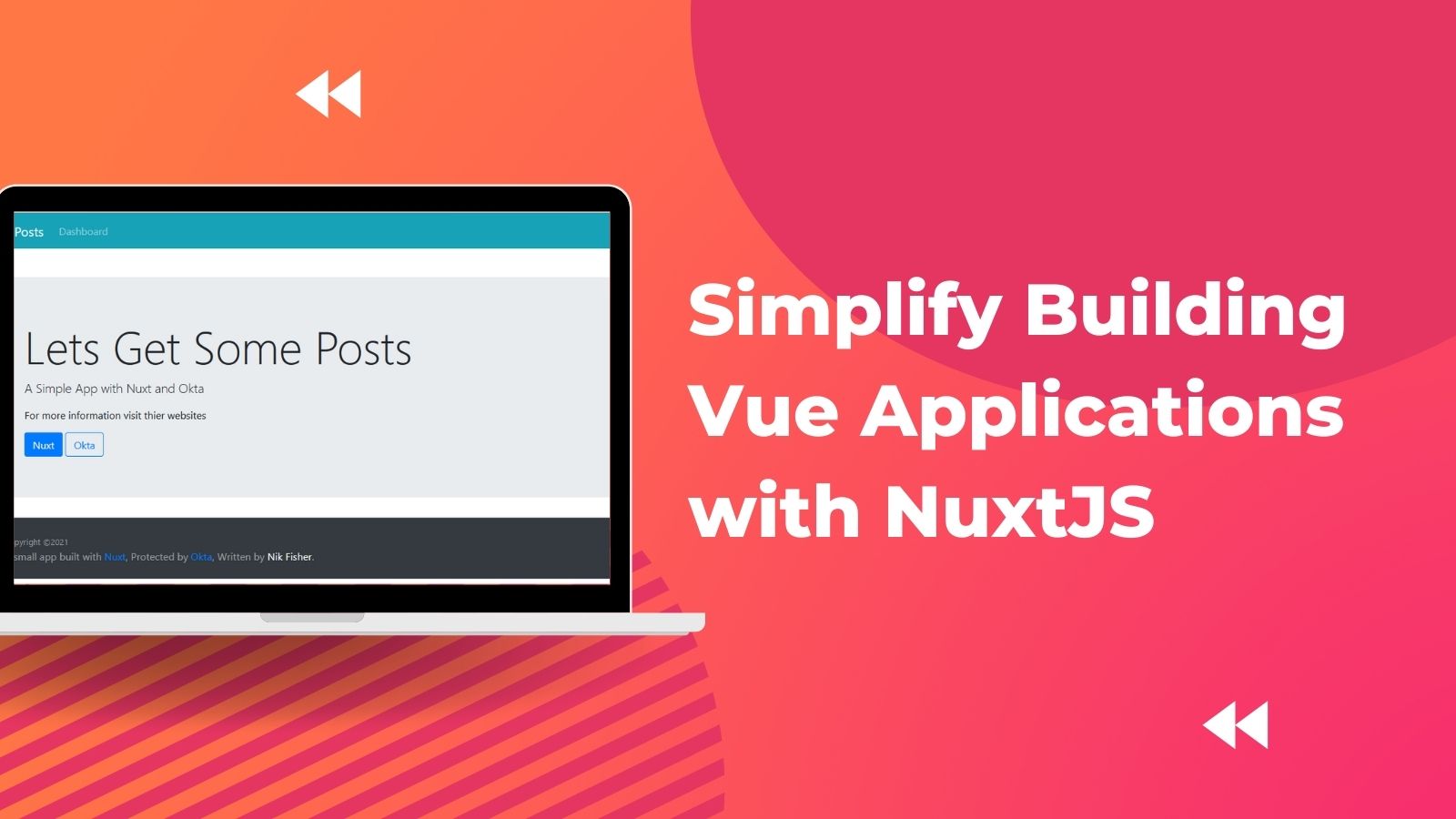
Nuxt calls itself the intuitive Vue framework. It aims to make a developer-friendly experience while not sacrificing performance or degrading the integrity of your architecture. It has been exciting to see the community and tooling around VueJS grow and evolve — there’s no better time to get started in this ecosystem than now. In this tutorial, you will build a small web application that retrieves some posts from an API and displays them for authenticated...
How to Create a React App with Storybook
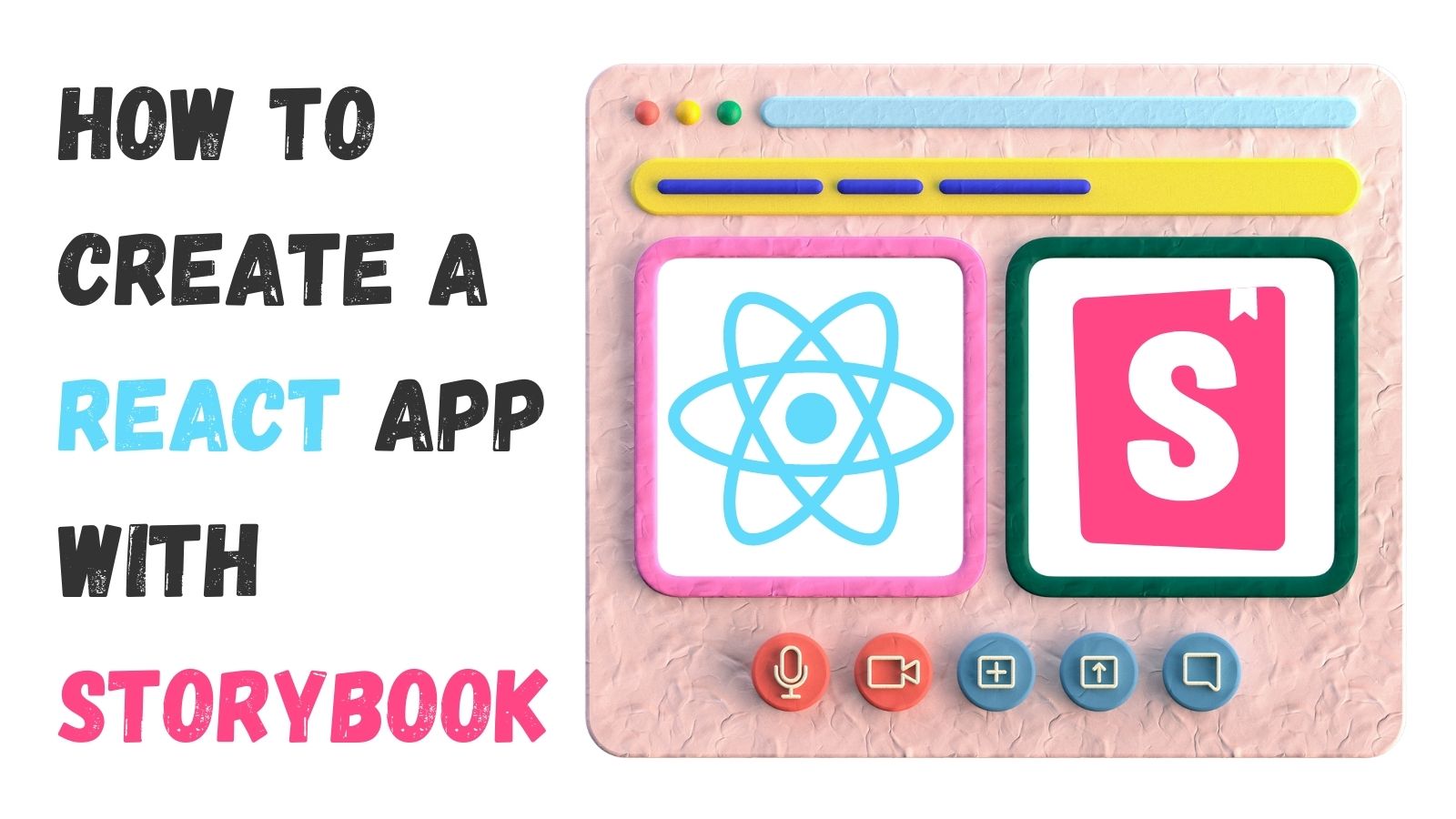
UI designers and front-end developers are tasked with creating clean and consistent user interfaces. At the same time, testing is a cornerstone of software development. Each part of a software project is tested individually and isolated from the other elements in unit tests. This practice has been challenging to achieve in the context of user interfaces. Now Storybook provides an open-source framework that lets you test UI components in isolation from the rest of the...
Loading Components Dynamically in an Angular App
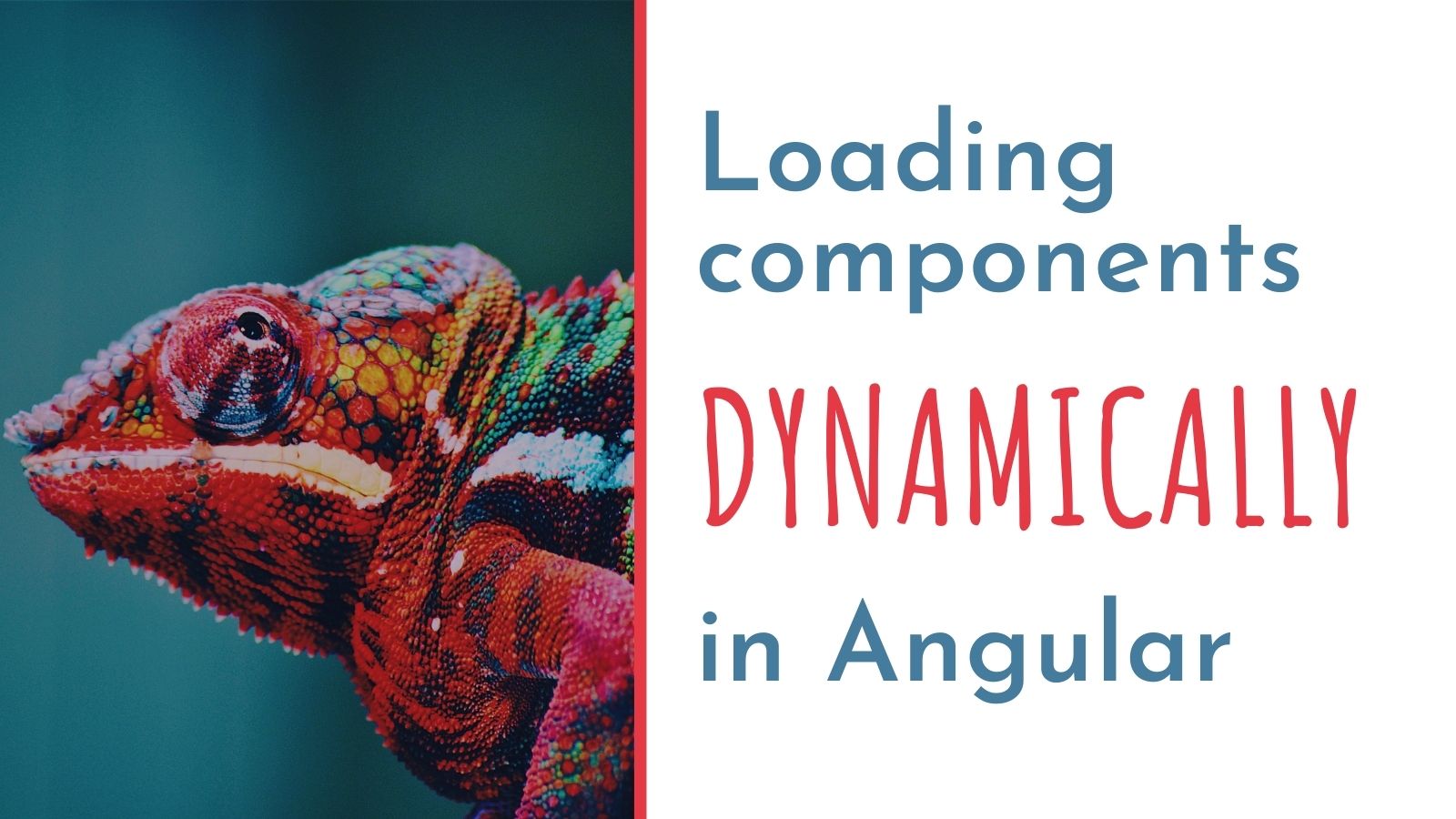
Businesses have unique and complex needs. In addition to the user or organization-specific data to show, there might be a need to display different views and content conditionally. The conditions might include the user’s role or which department they belong to. The information about a user might be part of the authenticated user’s ID token as a profile claim. In Angular, you can show different components or even parts of templates conditionally using built-in directives...
Build an Electron App with JavaScript and Authentication
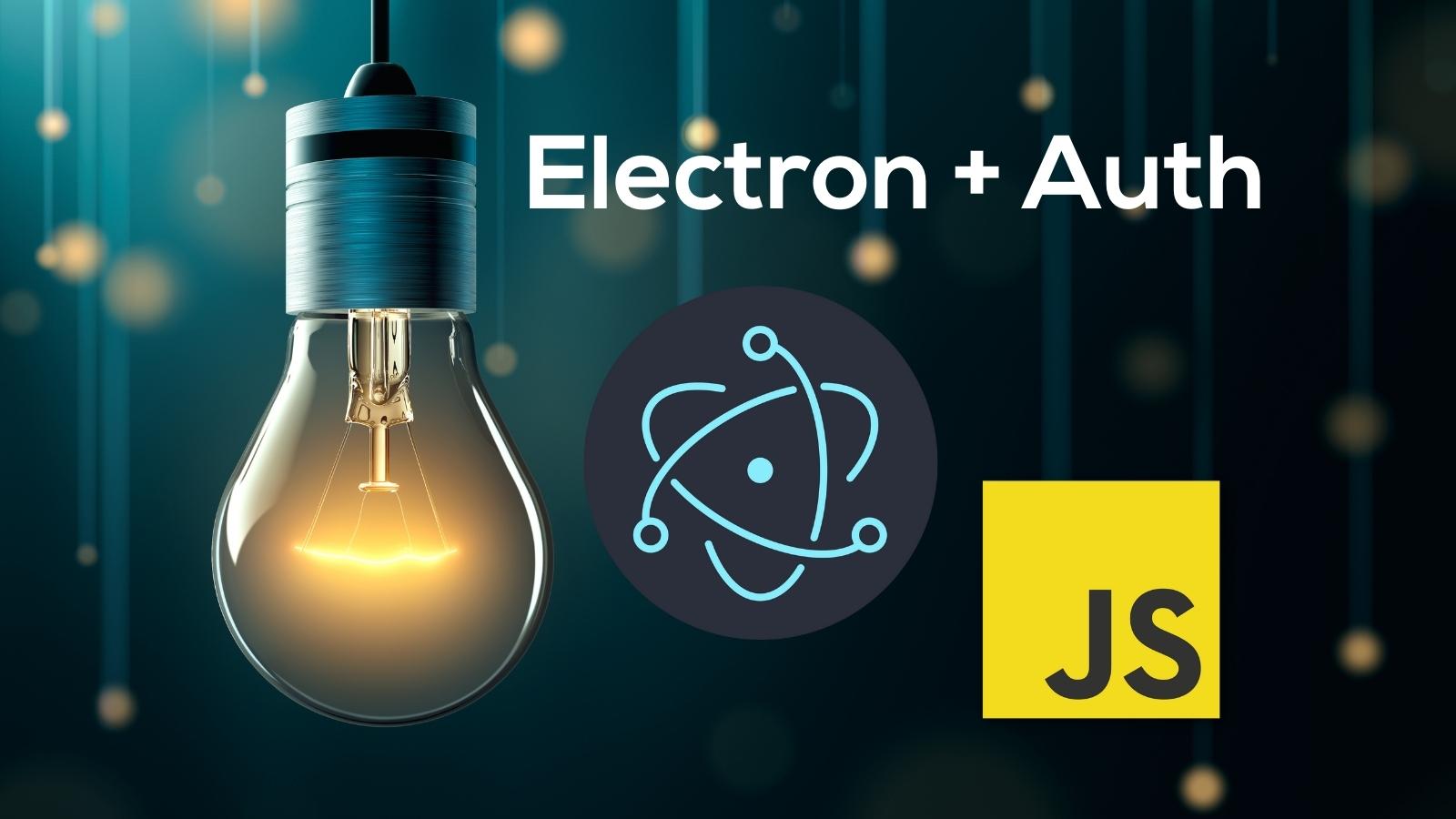
Electron is one of the most popular desktop frameworks today. Electron uses HTML, JavaScript, and CSS for its front end and Node.js for its backend. This design enables developers to quickly write and easily maintain cross-platform applications between their desktop and web applications. Since Electron uses Node on the backend, it also has access to the entire npm ecosystem that Node developers have come to rely on. Even though it is built on Node, Electron...
What You Need to Know about Angular v13
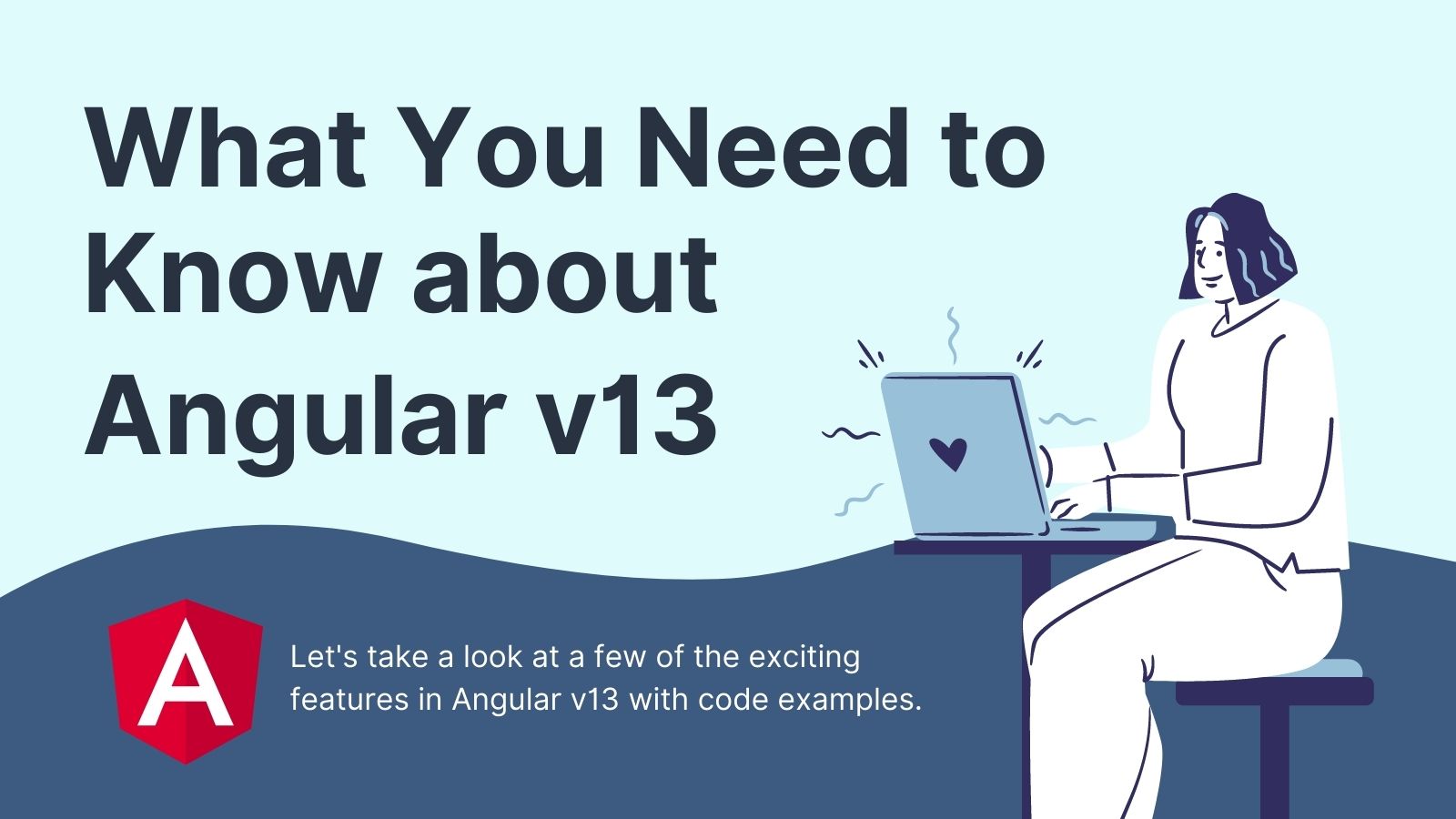
Angular v13 has arrived! And with it come a lot of exciting new features and updates. Angular continues to improve runtime performance, decrease compilation time, promote good software development practices, enhance developer experience, and keep up to date with dependencies such as TypeScript and RxJS. Is anyone else excited about RxJS v7?! 🤩 Let’s take a look at a few of the many new exciting features in Angular v13 with some code examples using authentication....
Kubernetes To The Cloud With AWS: Deploying a Node.js App to EKS
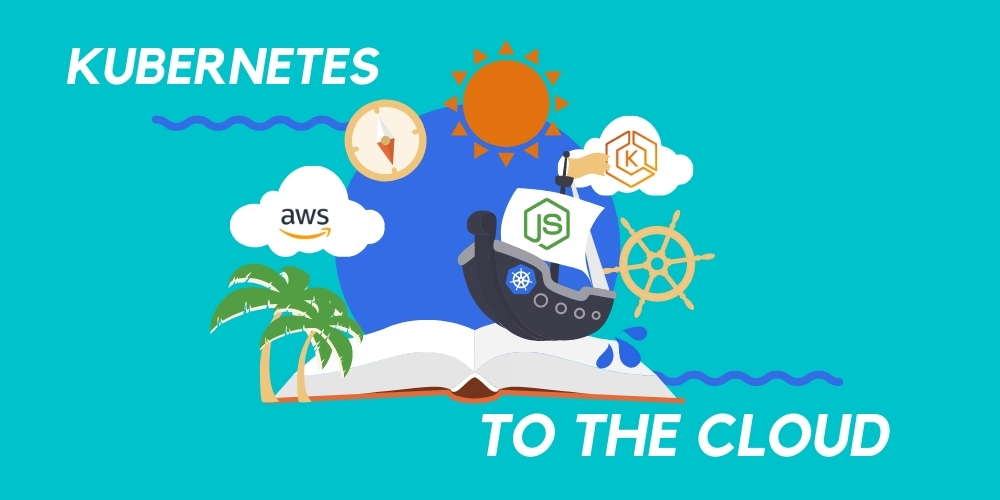
Since 2013 when it was released to the public, Docker has become an industry-standard tool for development teams to package applications into small executable containers. A year later, Kubernetes was released by Google to manage large amounts of containers, and provide features for high availability (HA) and auto-scaling. While Kubernetes adds many benefits to your container management, you might find the process of setting up Kubernetes within your on-premise infrastructure quite challenging, especially on the...
Build a Secure SPA with React Routing
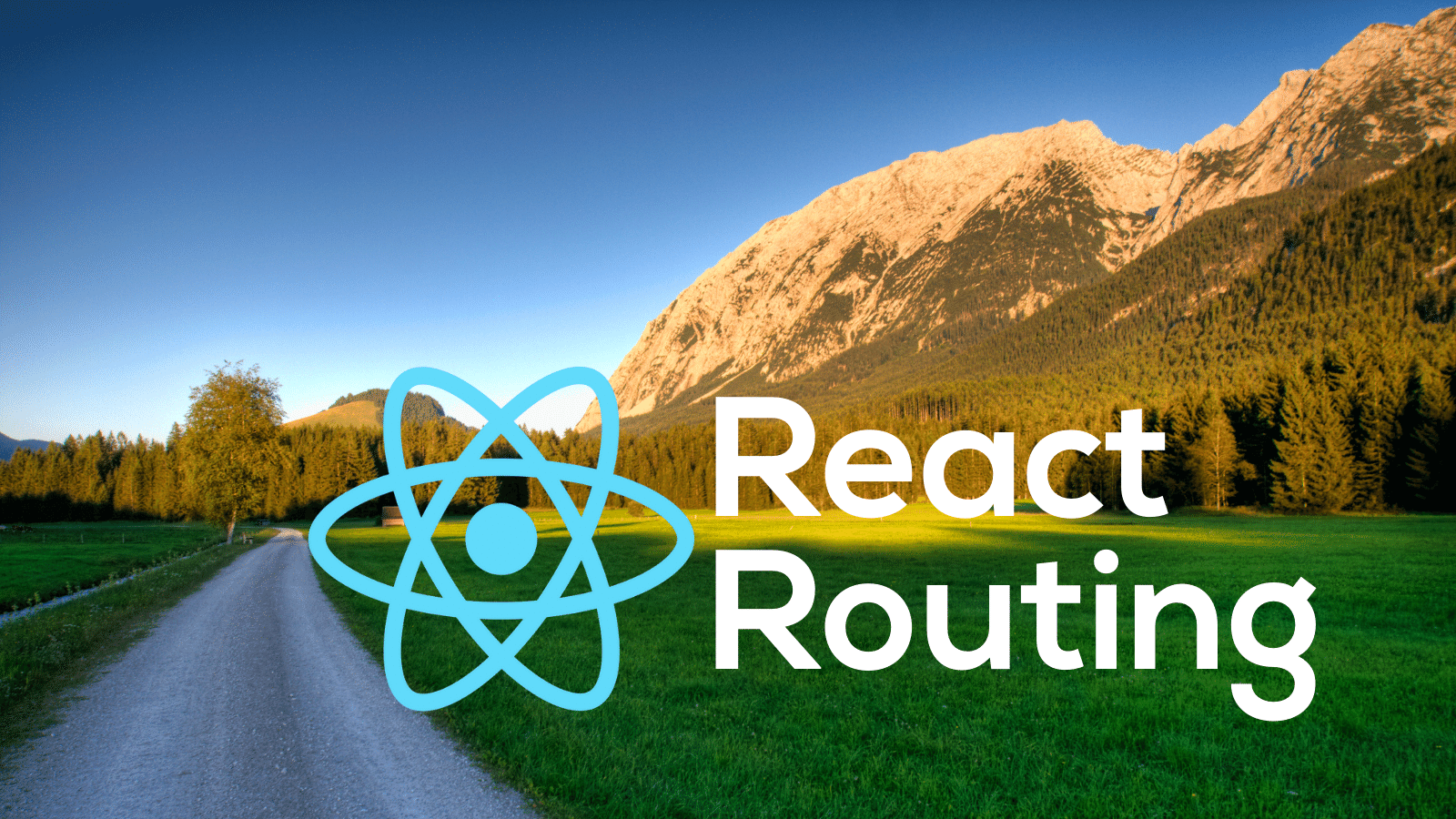
When building an SPA (single page application) with React, routing is one of the fundamental processes a developer must handle. React routing is the process of building routes, determining the content at the route, and securing it under authentication and authorization. There are many tools available to manage and secure your routes in React. The most commonly used one is react-router. However, many developers are not in a situation where they can use the react-router...
Oktanaut Tanay, Reporting for Duty
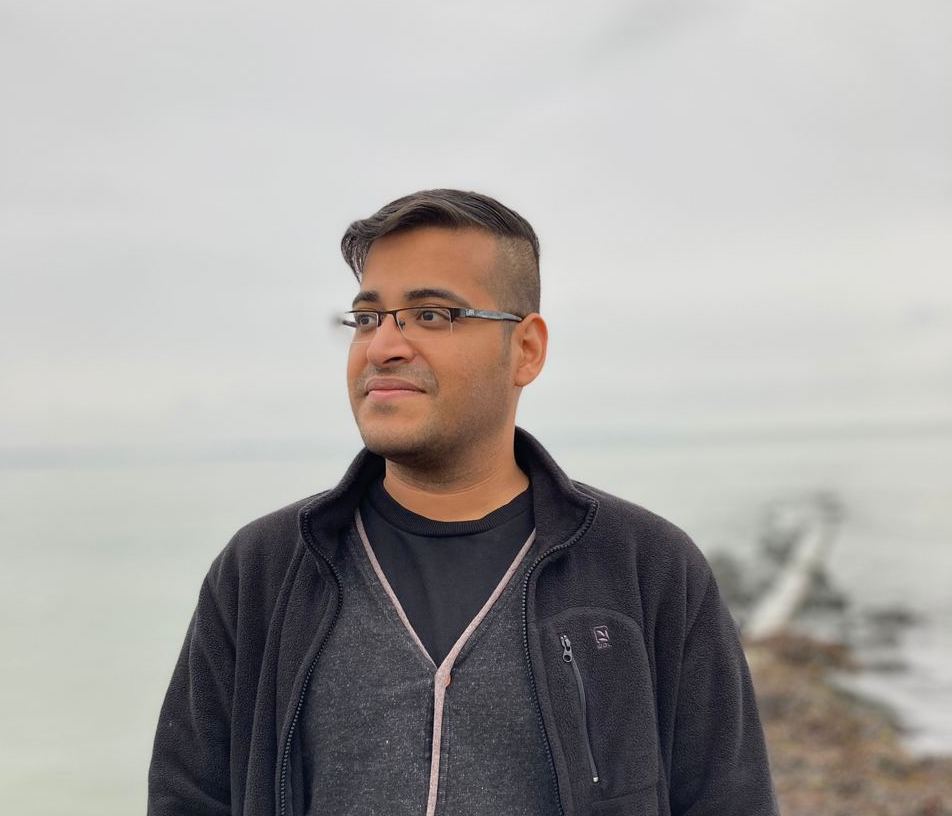
Greetings Oktaverse! I’m Tanay 🖖 I have been following Okta for many years, and I am very excited to finally join you all and get a chance to serve this wonderful community. First things first, here’s a picture of me so that you can recognize me and say hi the next time we meet. 👇 Who I am and what I’ve done so far I started my journey into the world of tech communities and...
A Quick Guide to Angular and GraphQL
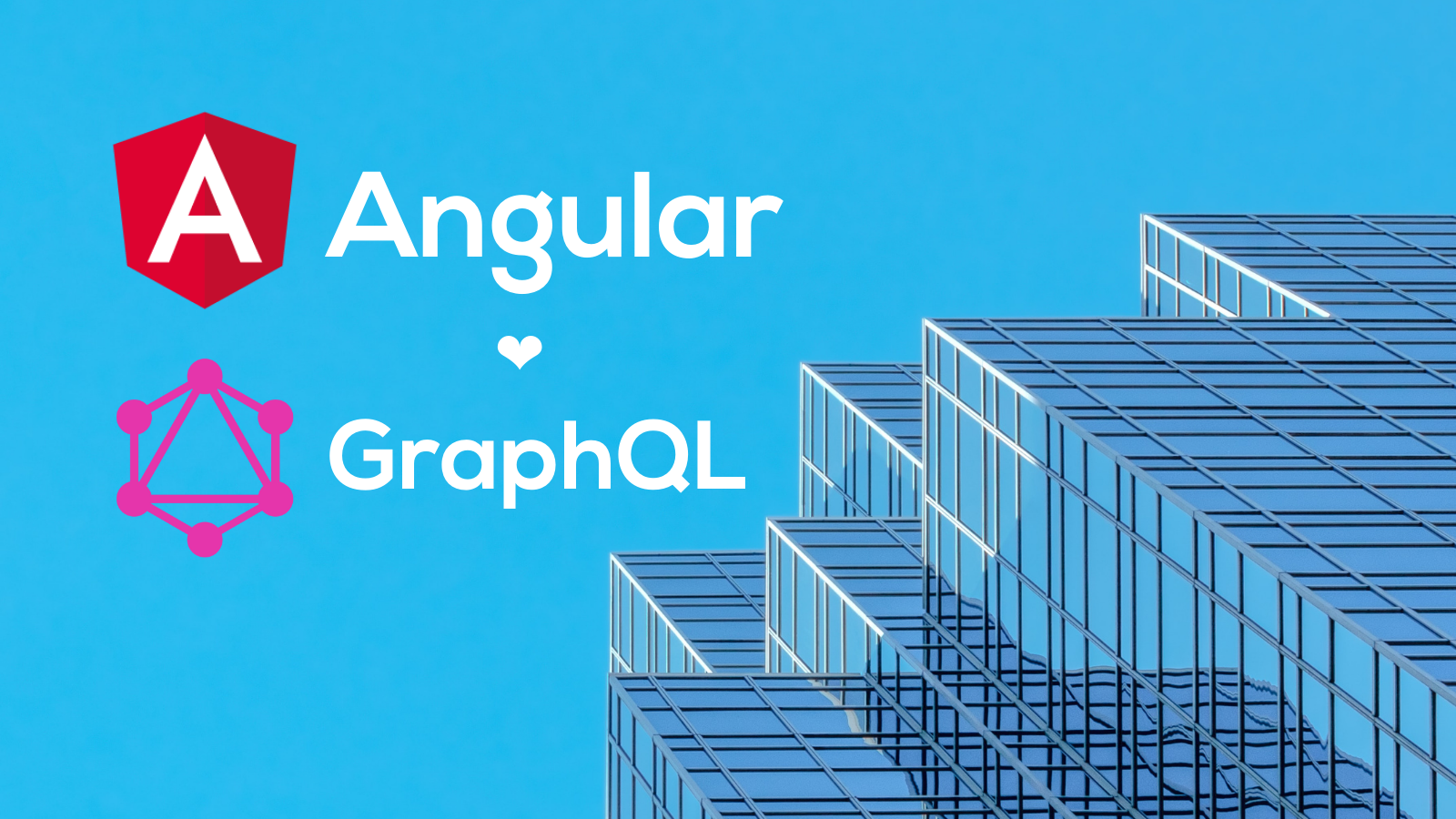
Over the past five years, GraphQL has established itself as the most popular alternative to REST APIs. GraphQL has several advantages over traditional REST-based services. First of all, GraphQL makes the query schema available to the clients. A client that reads the schema immediately knows what services are available on the server. On top of that, the client is able to perform a query on a subset of the data. Both of these features make...
Flying Into Okta
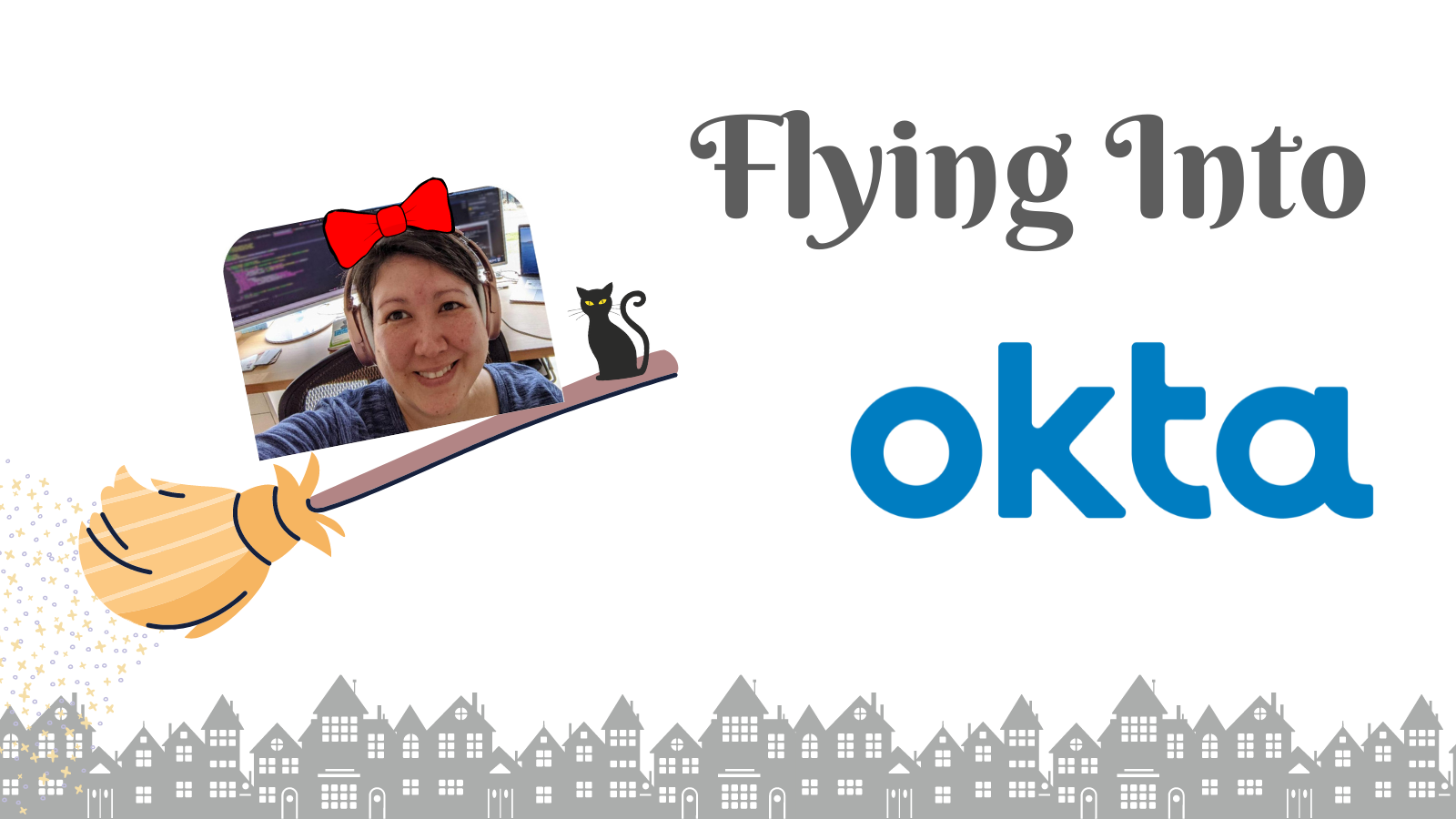
“Just follow your heart and keep smiling.” – Kiki’s Delivery Service I’m embarking on a new adventure and entering the wide world of Developer Advocacy at Okta! Much like Kiki setting out on her journey, I’m full of enthusiasm and curiosity and am ready to fly. I’m thrilled to be here at Okta and looking forward to everything. Now, I just need to get a talking cat… “Smile. We have to make a good first...
Spreading Some Okta Love to the DevOps World
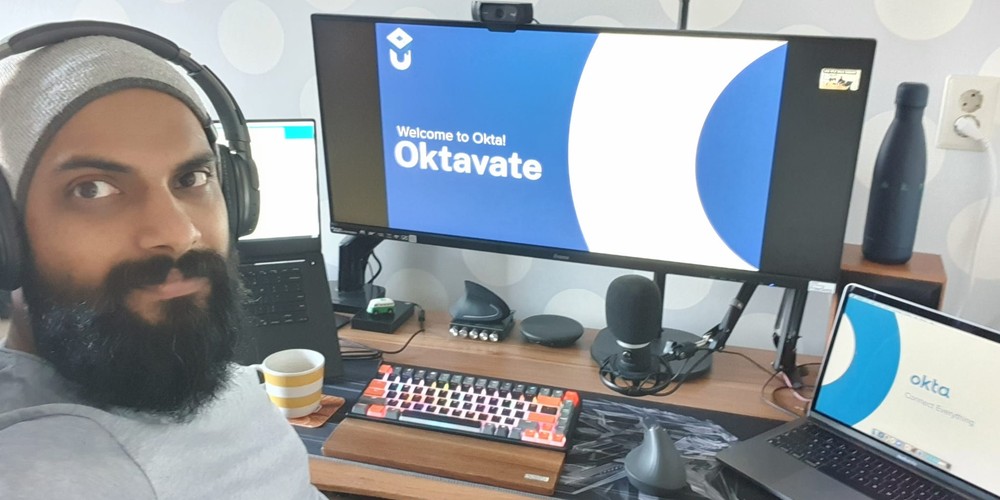
Hello Oktaverse! So finally, I have landed at Okta on my second attempt 😉. I’m so excited about this new chapter in my career journey and can’t wait to see what’s in store. But first, introductions. Who am I I’m from the south of India, a village in Kerala to be specific, but I grew up in Chennai since my parents moved there looking for work when I was 12. I like to call myself...
Learn How to Build a Single-Page App with Vue and Spring Boot
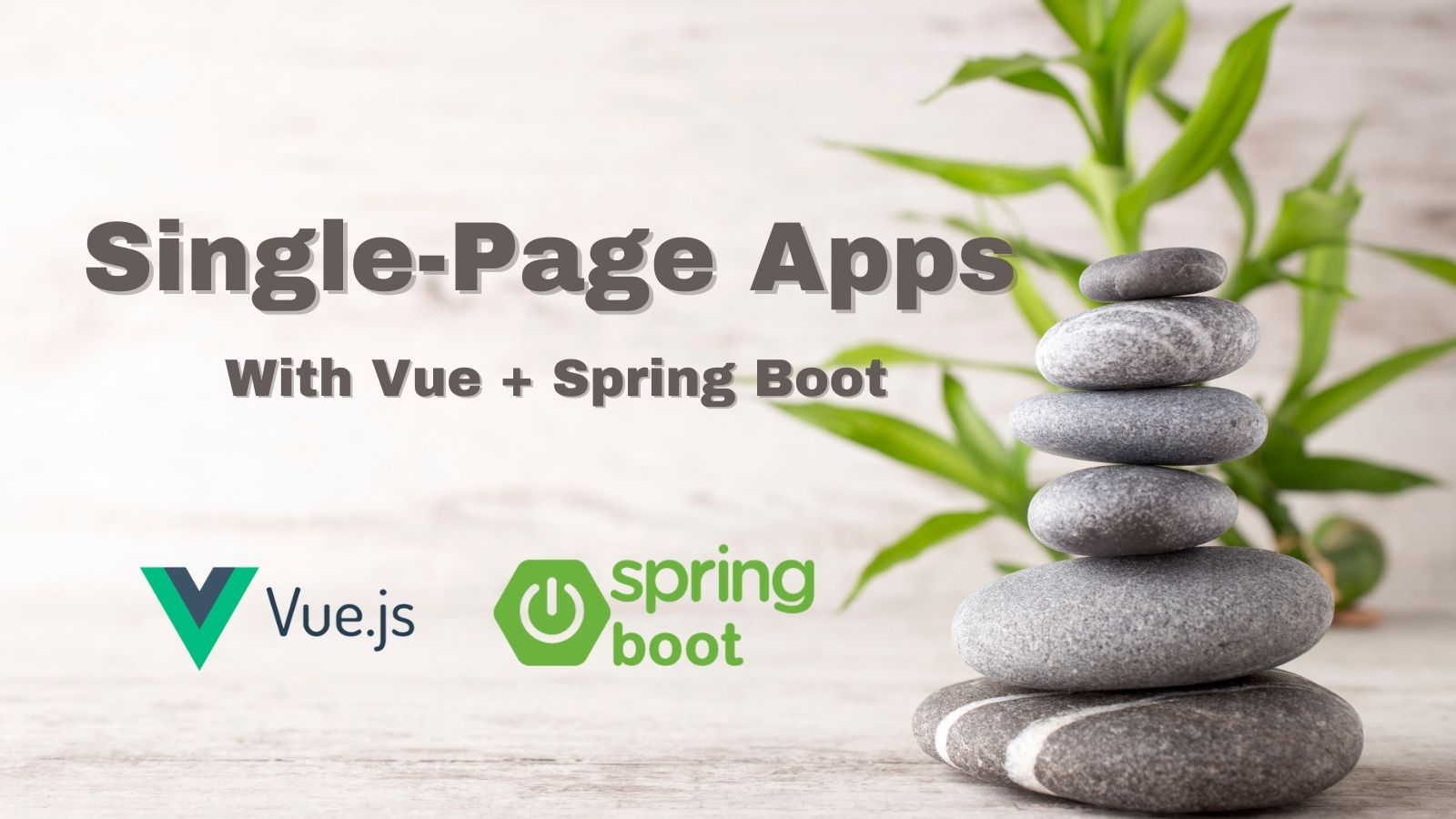
In this tutorial, you are going to create a single-page application (SPA) that uses a Spring Boot resource server and a Vue front-end client. You’ll see how to configure Spring Boot to use JSON Web Tokens (JWT) for authentication and authorization, with Okta as an OAuth 2.0 and OpenID Connect (OIDC) provider. You’ll also see how to bootstrap a Vue client app with the Vue CLI and how to secure it using the Okta Sign-In...
Fixing Common Problems with CORS and JavaScript
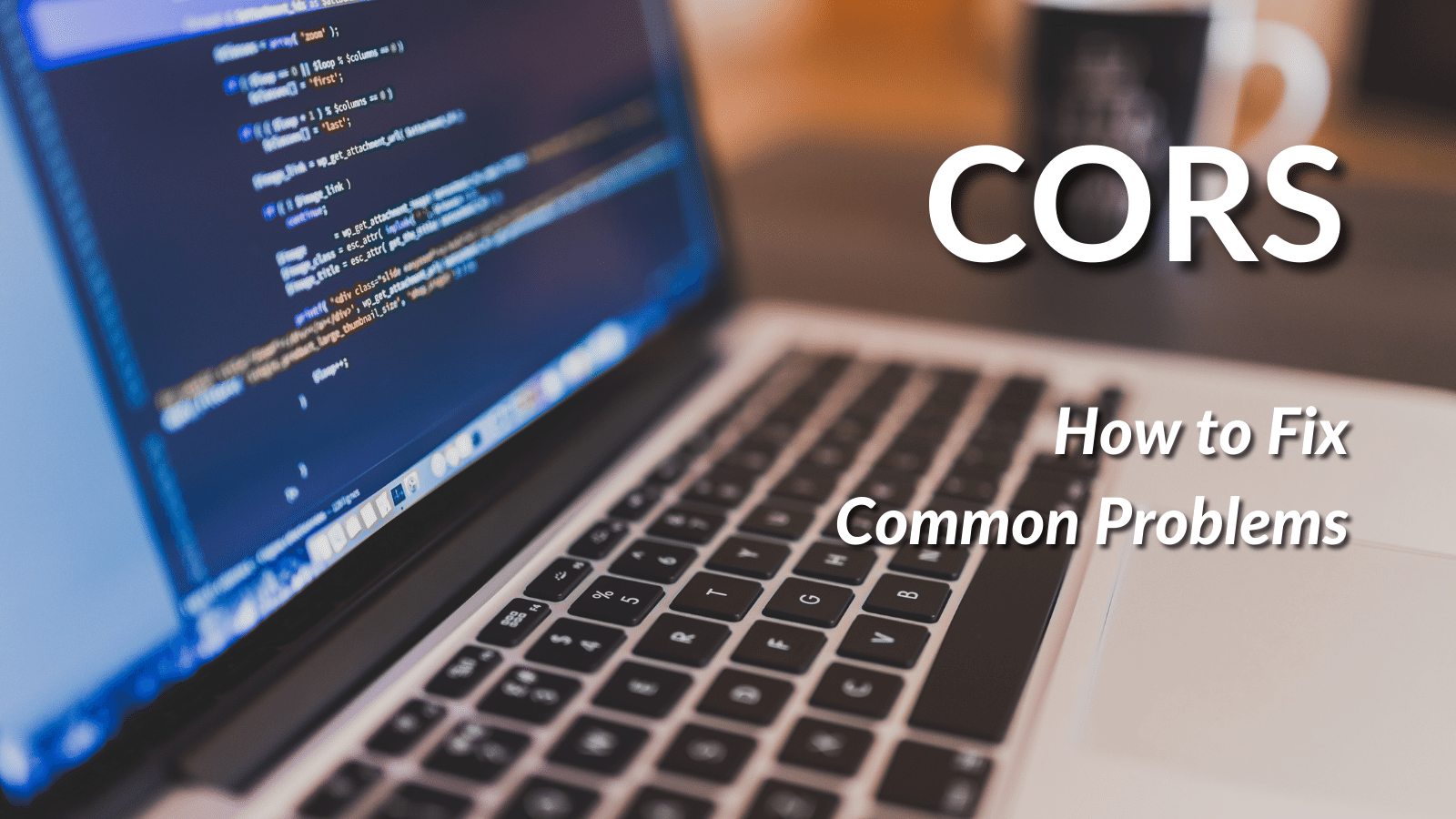
Many websites have JavaScript functions that make network requests to a server, such as a REST API. The web pages and APIs are often in different domains. This introduces security issues in that any website can request data from an API. Cross-Origin Resource Sharing (CORS) provides a solution to these issues. It became a W3C recommendation in 2014. It makes it the responsibility of the web browser to prevent unauthorized access to APIs. All modern...
Create a Secure Chat Application with Socket.IO and React
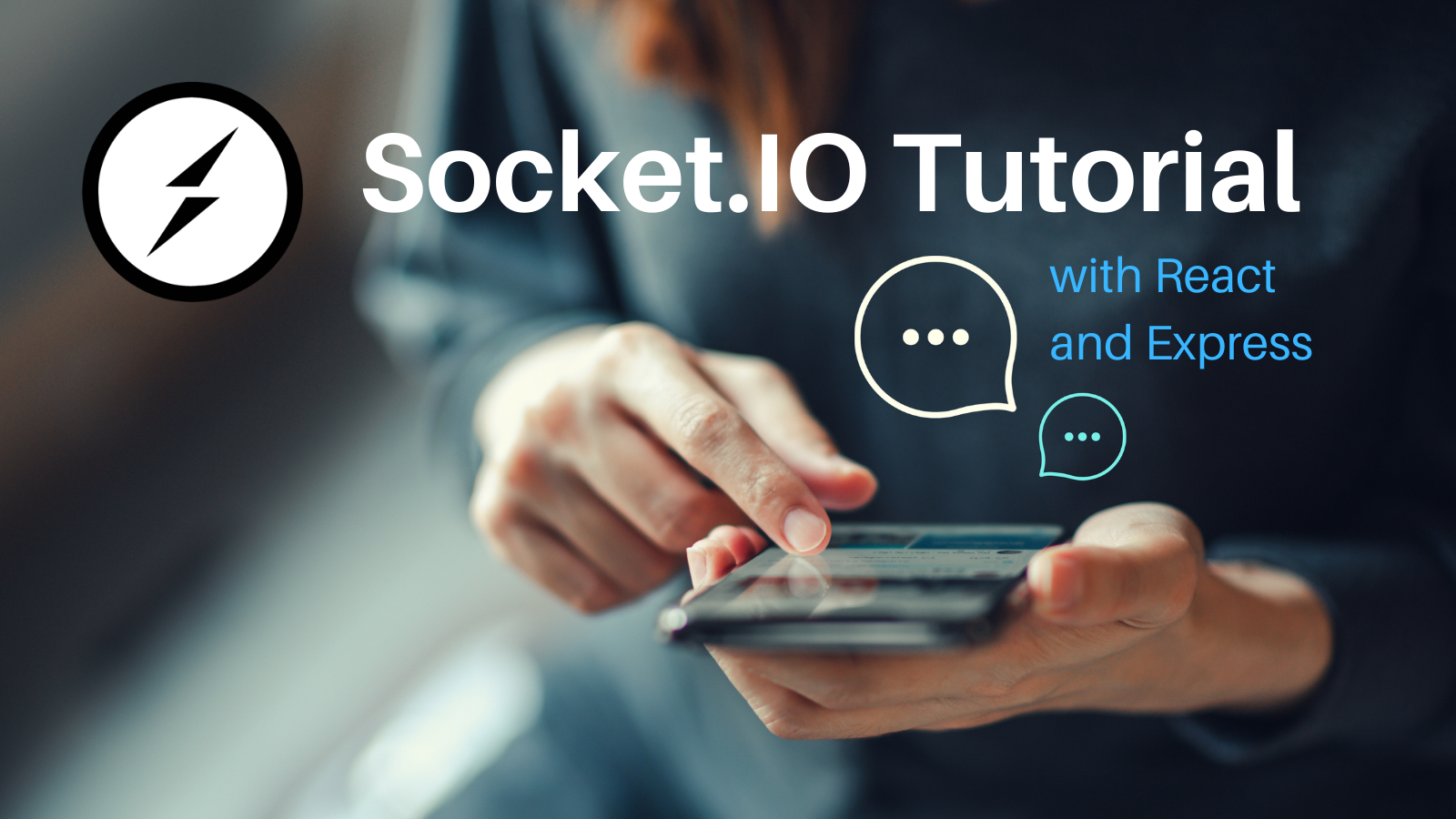
The HTTP protocol powers the web. Traditionally, HTTP is a request-response protocol. This means that a client requests data from a server, and the server responds to that request. In this model, a server will never send data to a client without having been queried first. This approach is suitable for many use cases that the web is used for. It allows loose coupling between clients and servers without the need to keep a persistent...
Build a Secure NestJS Back End for Your React Application
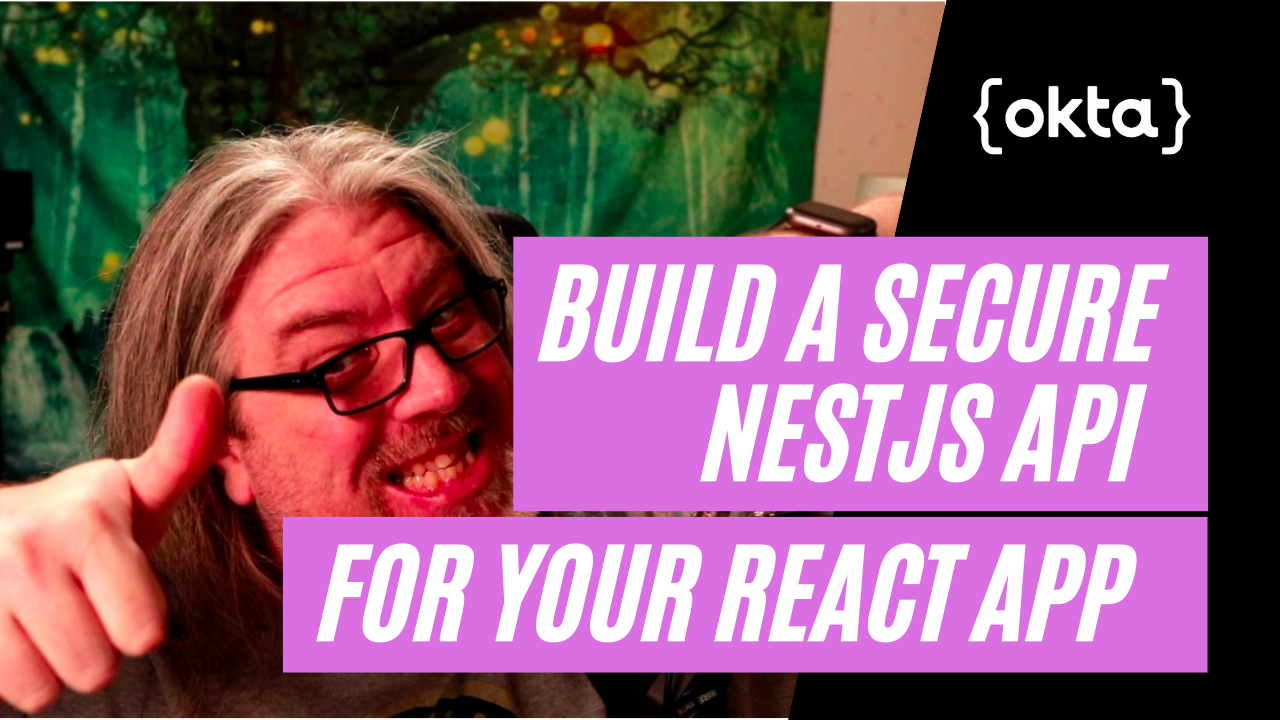
NestJS is a node.js framework that boasts the ability to build efficient, reliable, and scalable applications. A NestJS application can serve as the backend for your SPA. In this tutorial, you will use React, one of the most popular javascript front-end libraries available to build your SPA, and then use NestJS for your server. To secure everything, you will level Okta’s simple and powerful single sign-on provider. You will learn how to authenticate a user...
Build Your First NestJS Application
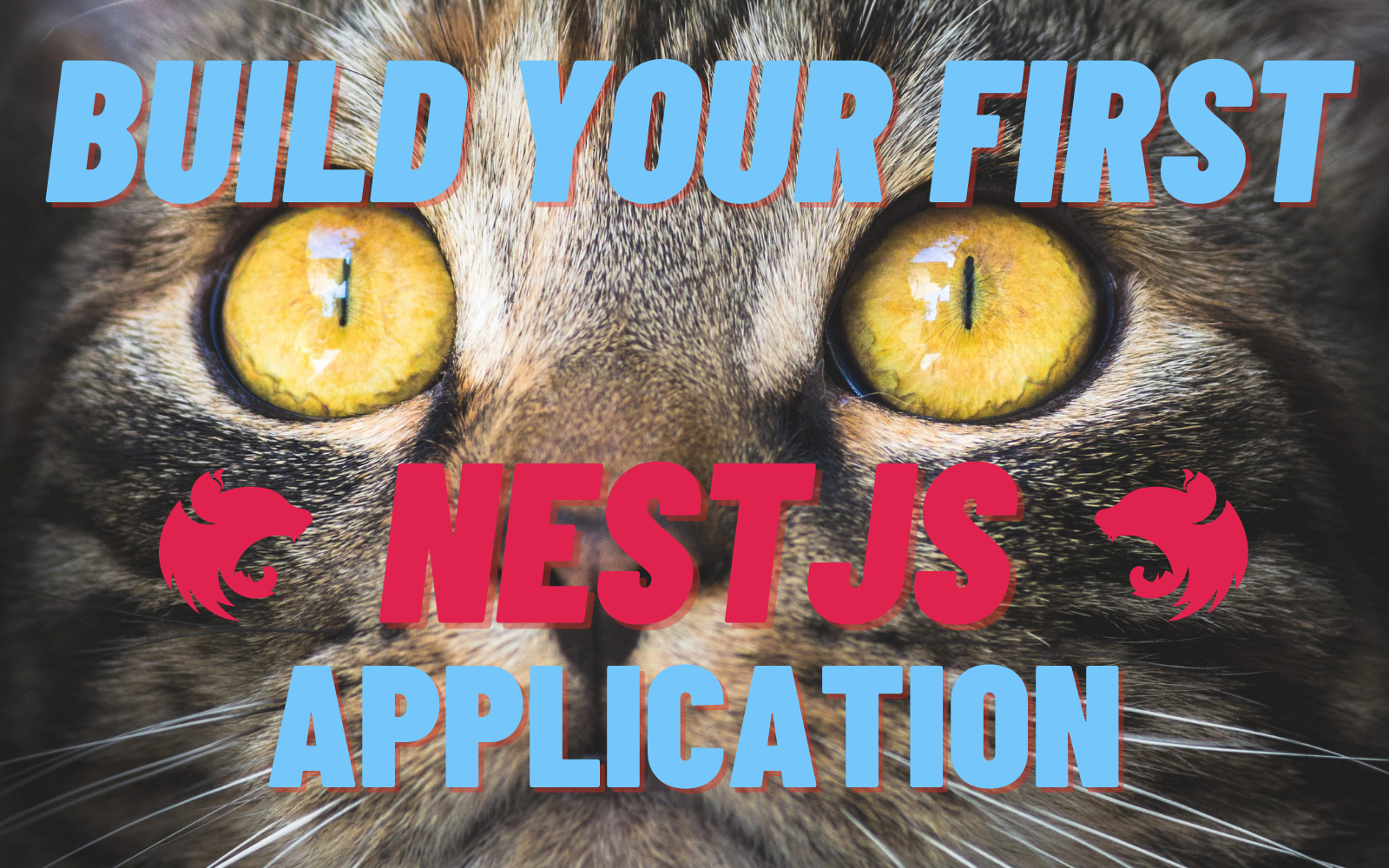
NestJs is a popular Node.js framework that is built with typescript and makes use of object-oriented programming, functional programming, and functional reactive programming. NestJs boasts that it provides a framework for building scalable server-side applications. NestJs integrates nicely with Okta’s single sign-on provider. Okta makes securing a web service, such as the one you will build, quick and easy. Note: In May 2025, the Okta Integrator Free Plan replaced Okta Developer Edition Accounts, and the...
Building and Securing a Go and Gin Web Application
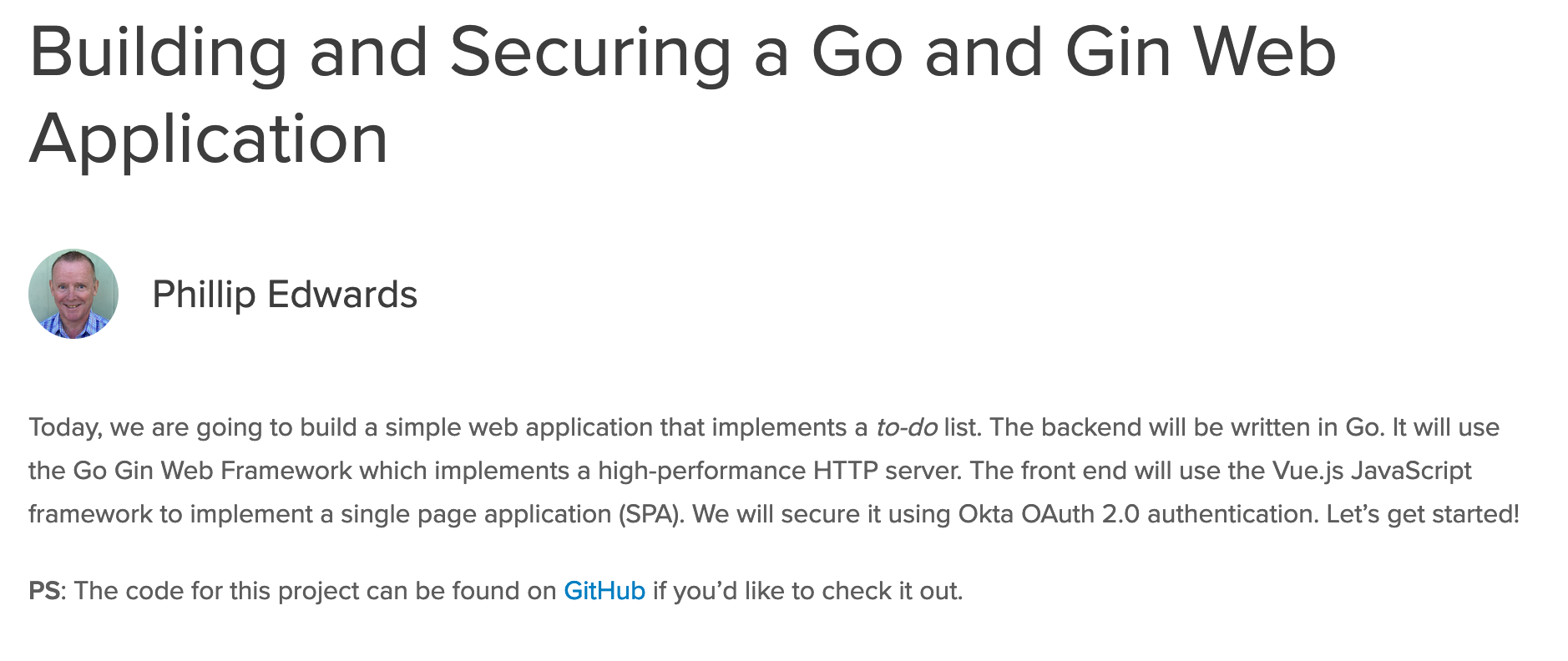
Today, we are going to build a simple web application that implements a to-do list. The backend will be written in Go. It will use the Go Gin Web Framework which implements a high-performance HTTP server. The front end will use the Vue.js JavaScript framework to implement a single page application (SPA). We will secure it using Okta OAuth 2.0 authentication. Let’s get started! PS: The code for this project can be found on GitHub...
A Quick Guide to React Login Options
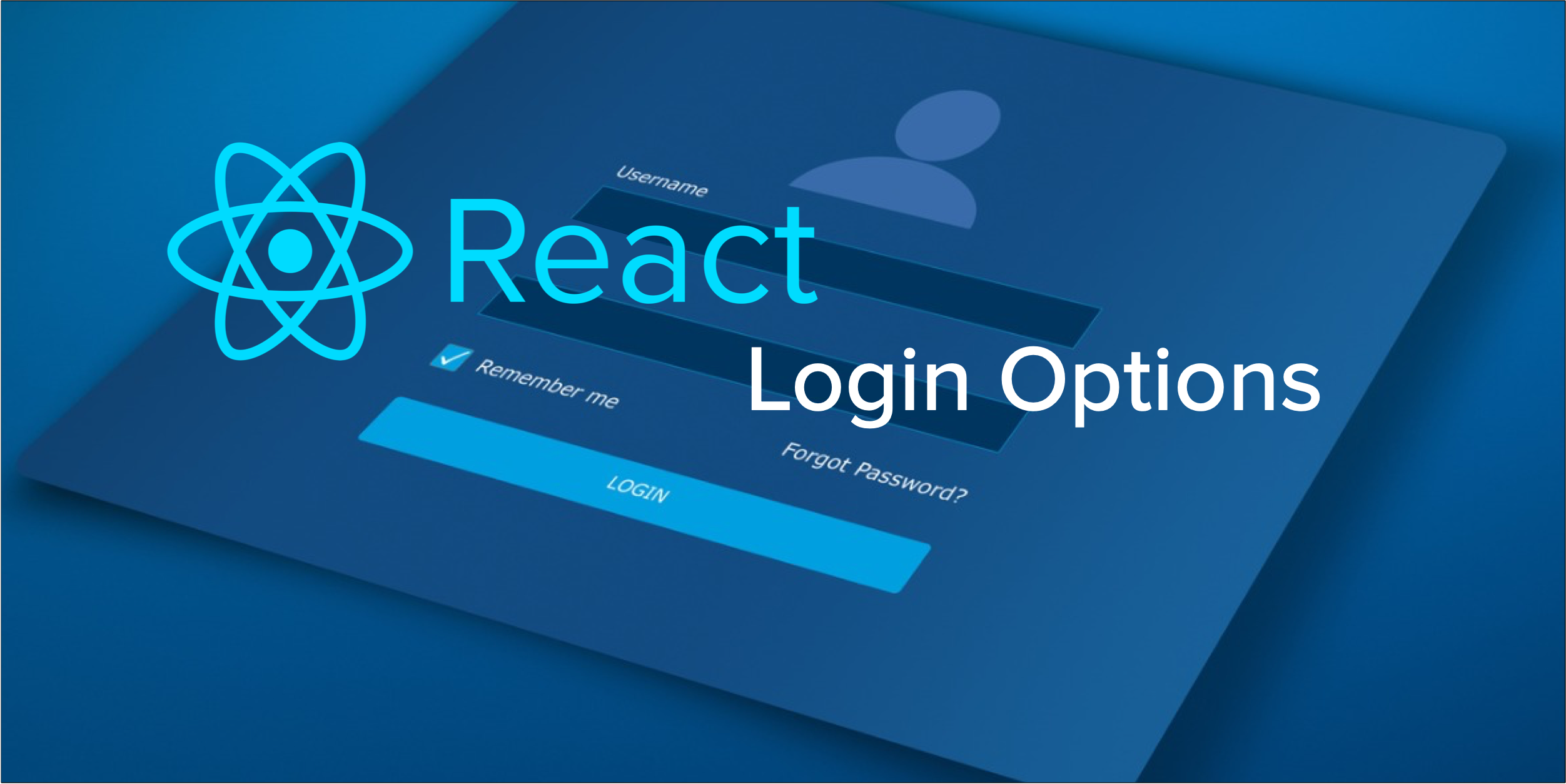
Almost any web app needs some sort of access control, usually implemented by user login. Choosing how user authentication is implemented depends on the type of application and its audience. In this post, I want to show you a few different ways of creating a login feature in a single-page React application using Okta. I will start with a login redirect. This is the easiest option to implement and is a good choice for some...
25 Years of JavaScript and Java! 🎉
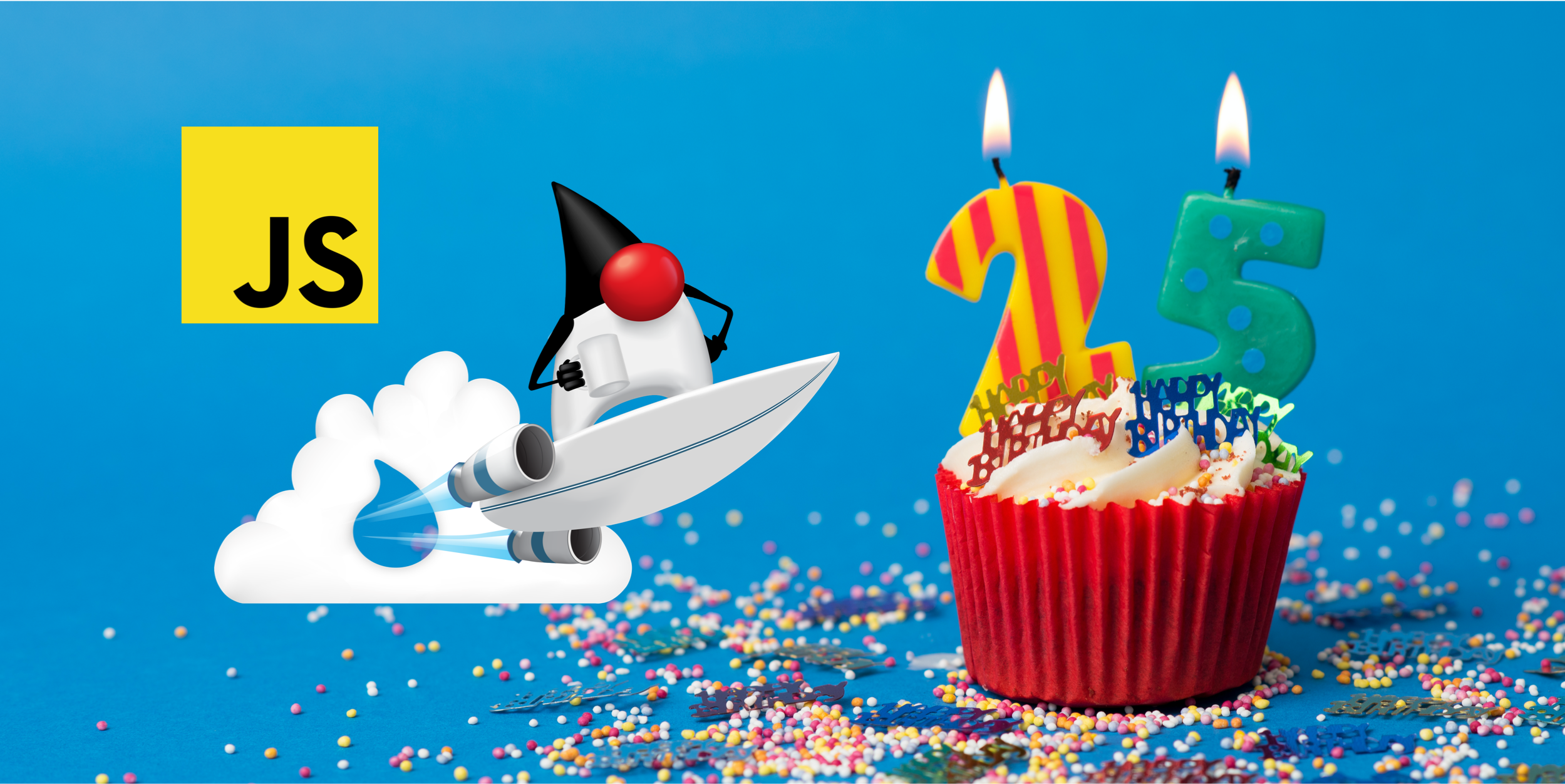
The year is 1995… Java is born in May. So is JavaScript (but it’s called Mocha). Microsoft releases Windows 95 in August. JavaScript, as it’s known today, first appeared on this day, December 4th. Wow! It’s so crazy to look back and see so many influential software releases happen in such a short period. Congrats to both JavaScript and Java for doing so well over the last 25 years! Fun fact from the @JavaScriptDaily 👇...
Use the Okta CLI to Quickly Build Secure Angular Apps
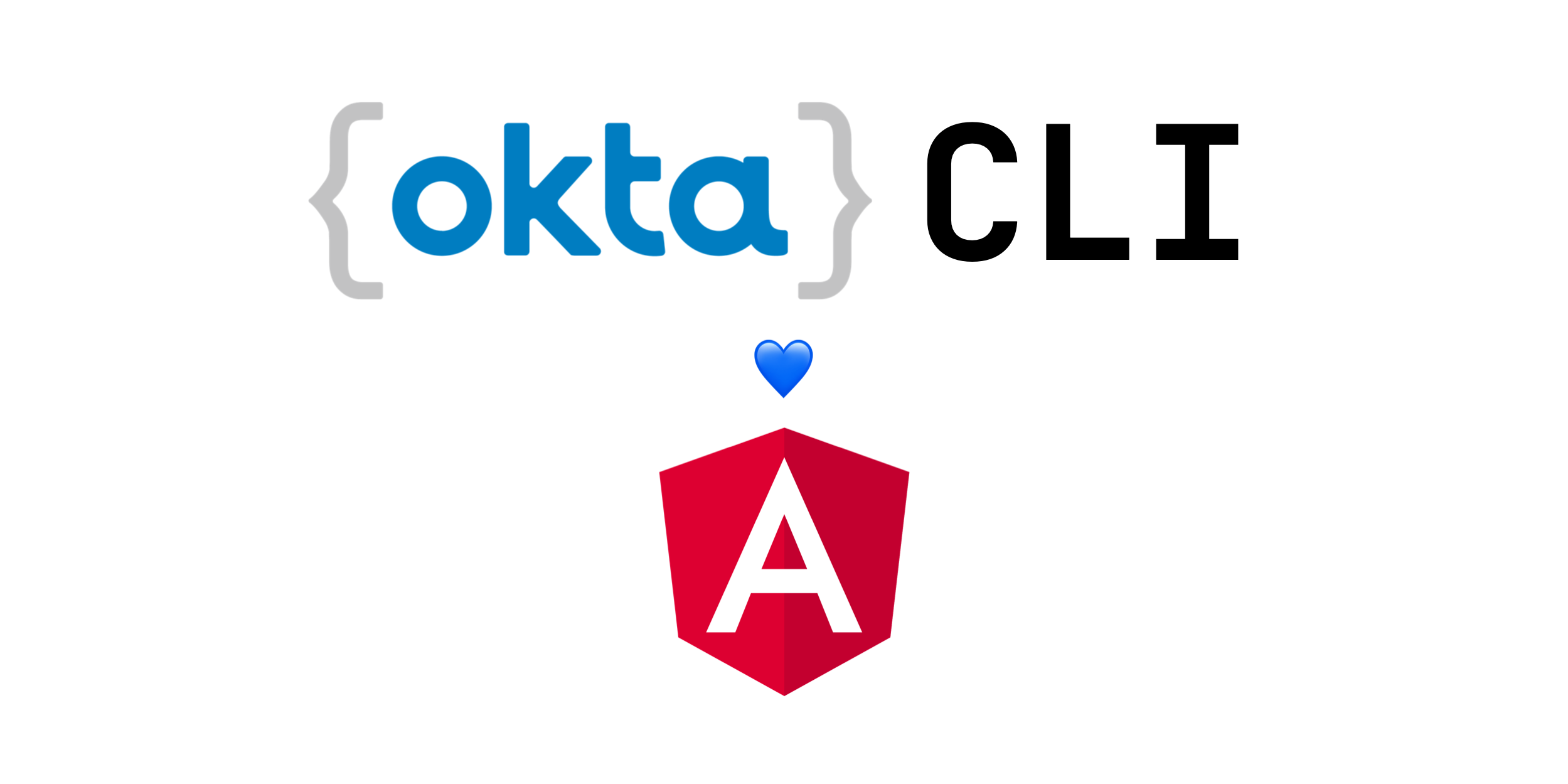
The Okta CLI is a new tool we’ve created here at Okta. It’s designed to streamline creating new Okta accounts, registering apps, and getting started. Wwwhhaaattt, you might say?! That’s right, it’s super awesome! To show you how easy it is, I created a screencast that shows you how to use it with Angular. To create the same app as the one shown in this video, you’ll need to run okta start angular --branch widget....
Develop Secure Apps with WebSockets and Node.js
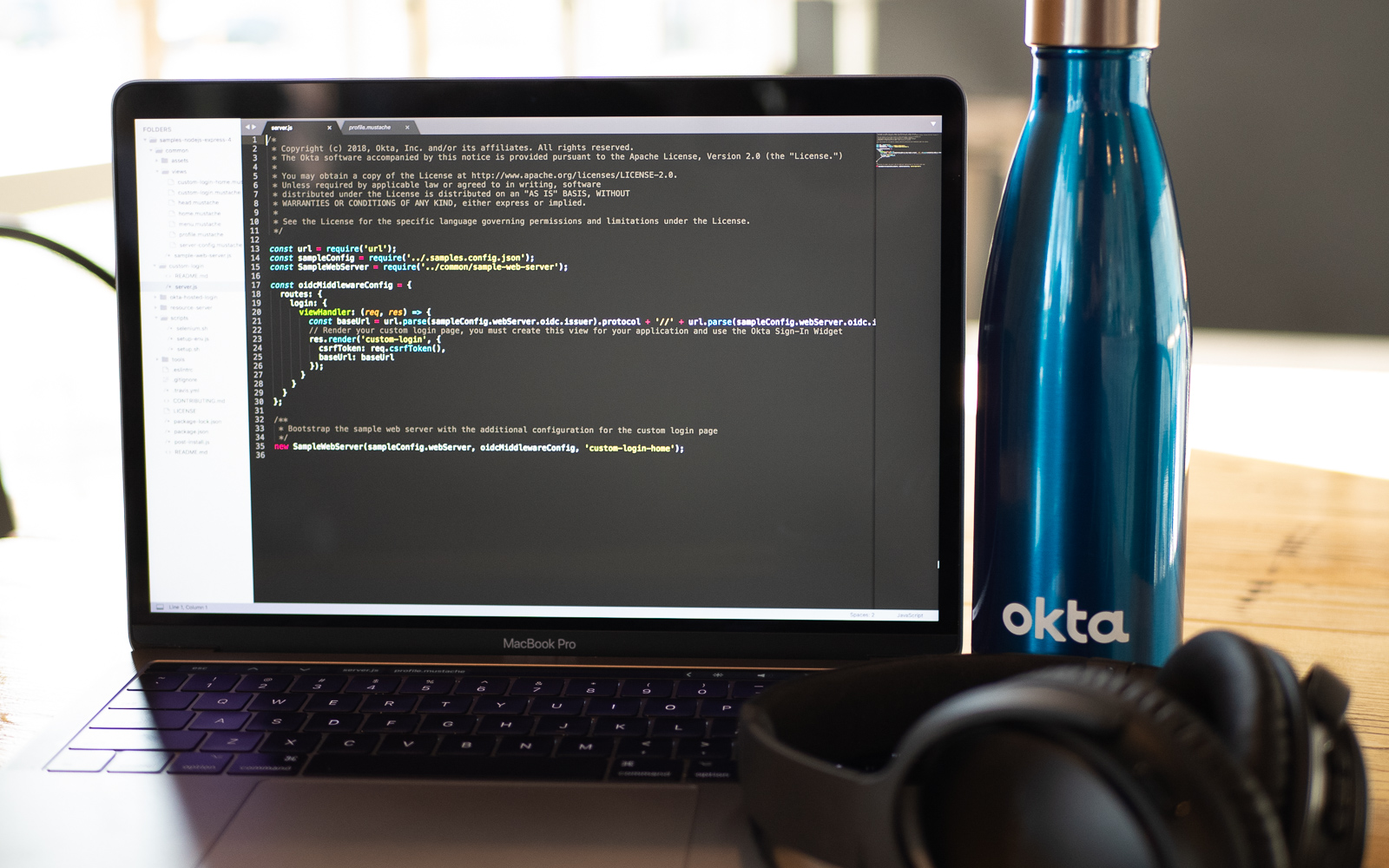
WebSockets is a technology for communicating between the client and the server in a web application, where an open socket creates a persistent connection between the client and the server. This method of communication works outside of the HTTP request/response paradigm that has existed since the earliest days of the internet. Since sockets don’t use HTTP they can eliminate the overhead that comes with HTTP for low latency communications. In this tutorial, you will learn...
Build a Video Chat Service with JavaScript, WebRTC, and Okta
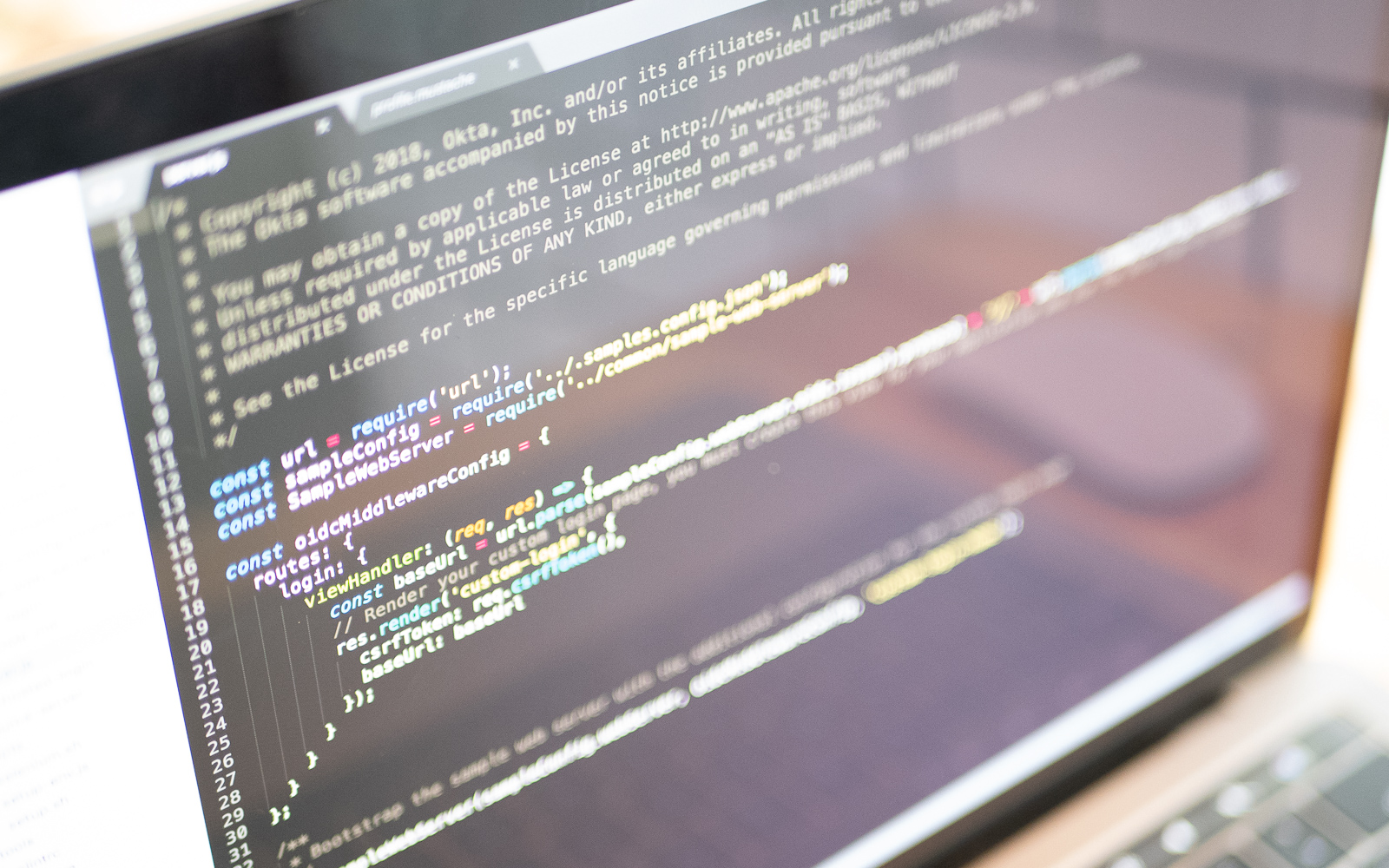
If you are familiar with any sort of real-time communications over the internet such as GoToMeeting, Google Meet, or Discord then chances are you have used WebRTC. WebRTC is an open framework for handling real-time communications. It supports video, voice, or any data between peers. WebRTC is supported by Google, Apple, Microsoft, Mozilla, and many others. In this tutorial, you will learn how to build a web application that allows a user to broadcast their...
Build a Modern API using Fastify and Node.js
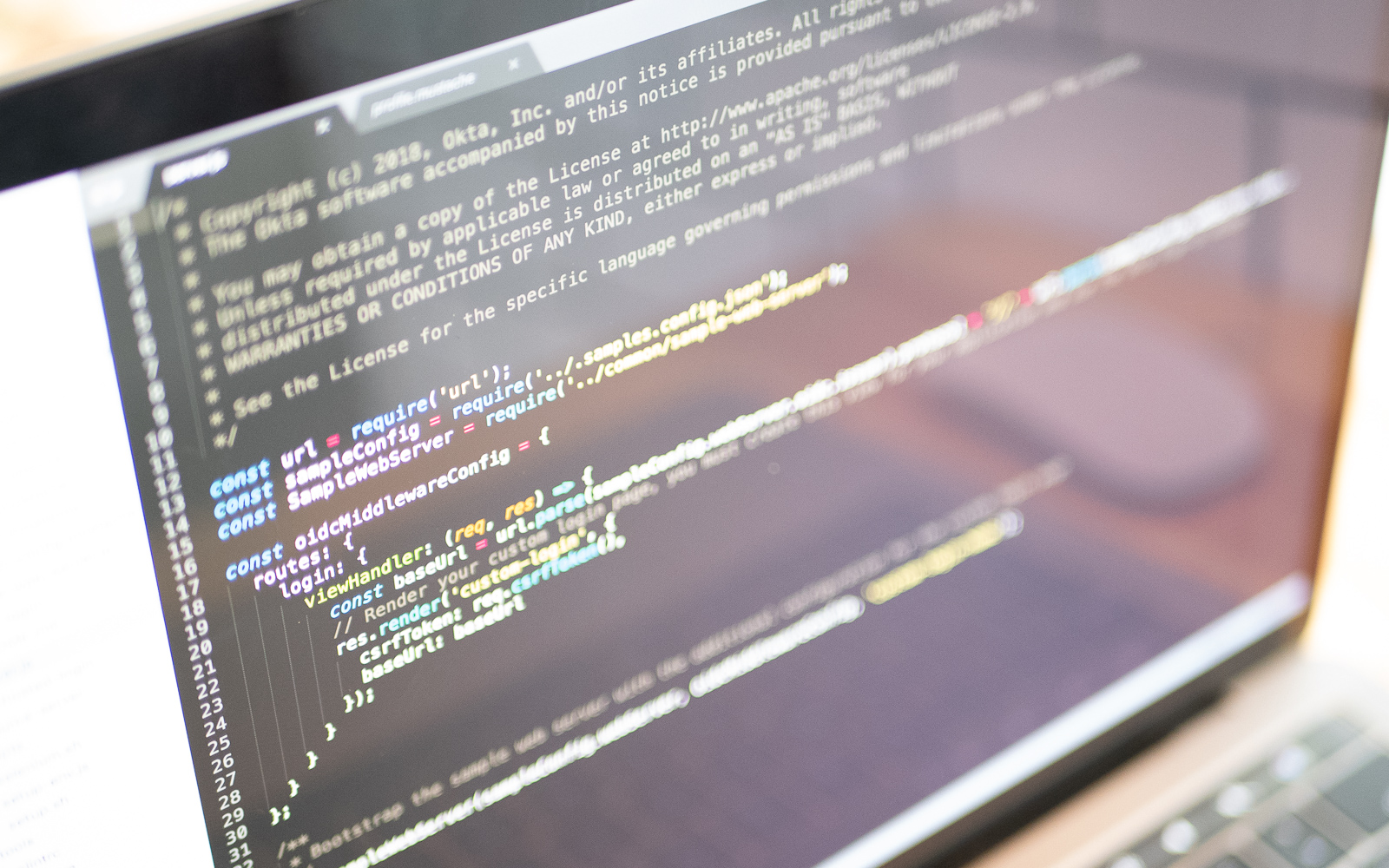
Fastify is just as the name implies, fast. Not just in terms of development speed—its low overhead means the server is fast as well. When writing APIs, speed on both sides is paramount. Fastify is a web framework for Node.js that was designed for efficiency. Fastify is fully extensible with hooks, plugins, and decorators. It is schema-based, meaning you can define your response and request objects in your routes and have Fastify do the data...
Migrate User Passwords with Okta's Password Hook
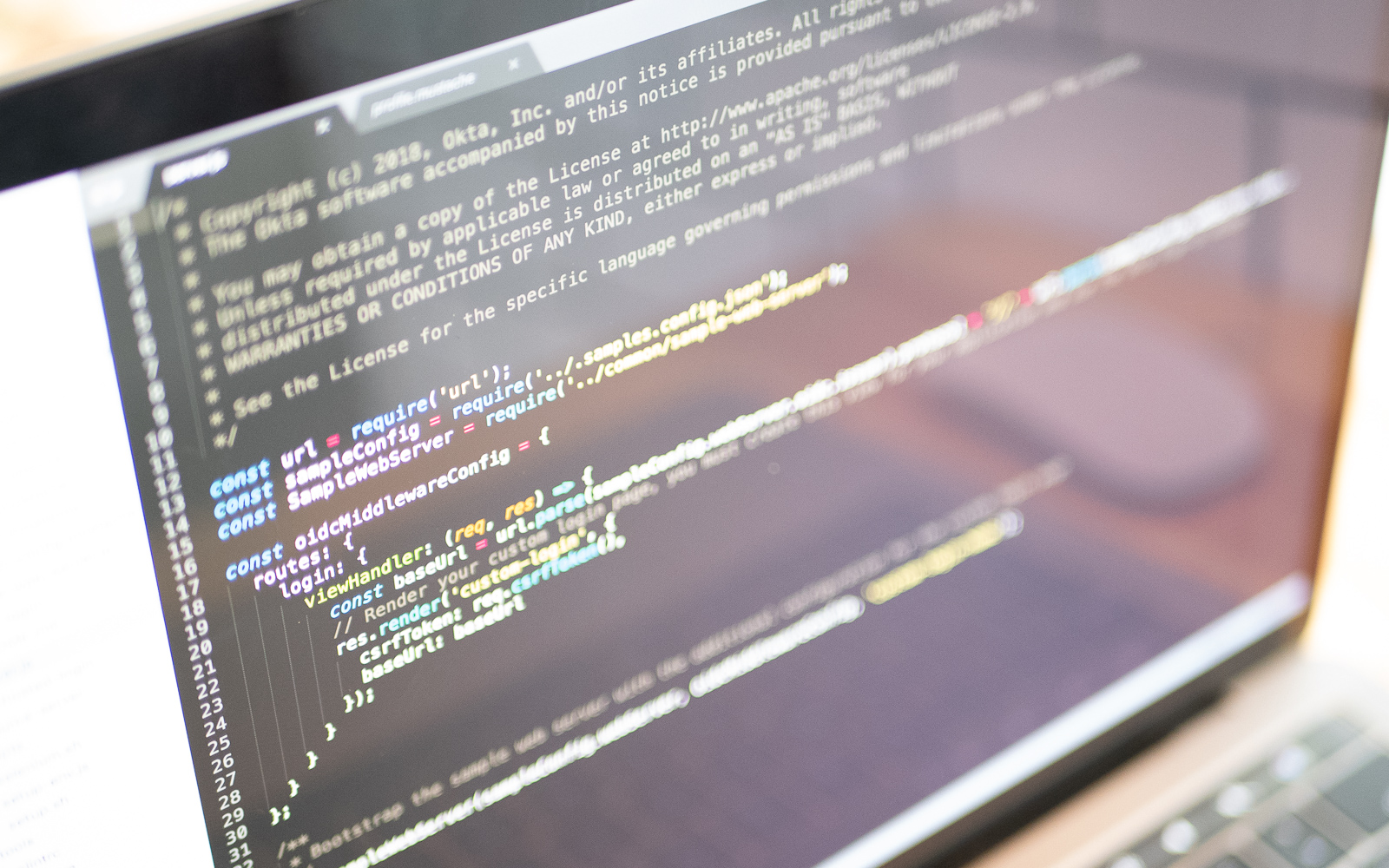
Okta is an identity platform focused on making authentication easy to build with minimal code. Our goal at Okta is to build a solution so flexible and easy to use, that you’ll never have to build authentication again. And while Okta can provide a lot of new functionality to your application, including multi-factor authentication (MFA) based on contextual policies, self-service password resets, and federation to enterprise identity providers and social media accounts, we’ve found that...
Build a React App with ANT Design Principles
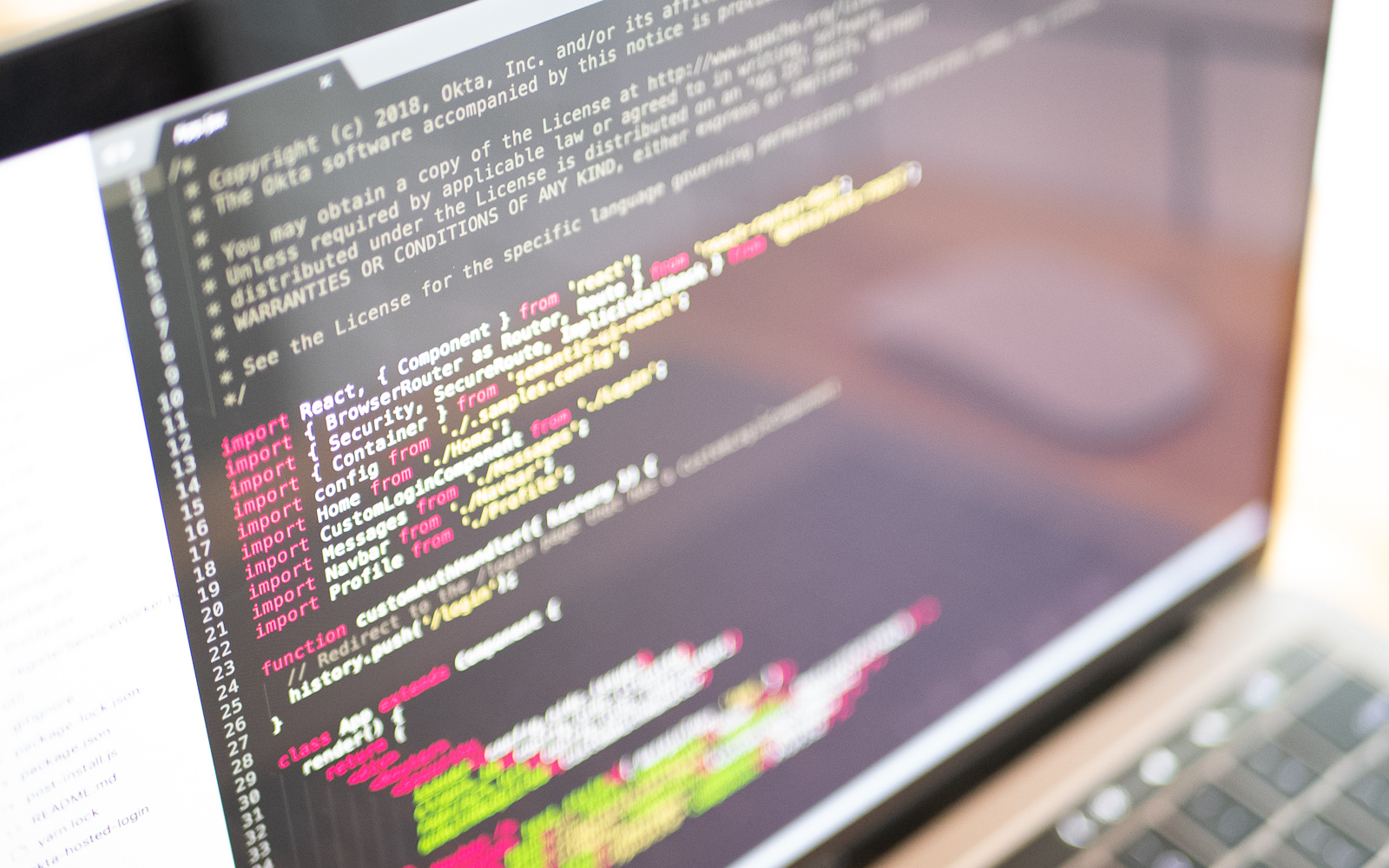
For years the go-to HTML/CSS framework of choice for developers was Bootstrap. A new contender has appeared in the form of Ant Design. Ant should feel familiar to veteran developers but it’s built on new principles. Their site spends a good amount of effort distinguishing between good and bad design. There is an emphasis on clarity and meaning. Ant Design is heavily based on psychological principles to anticipate—and be customized for—user behavior. Ant Design is...
Build Your First Deno App with Authentication
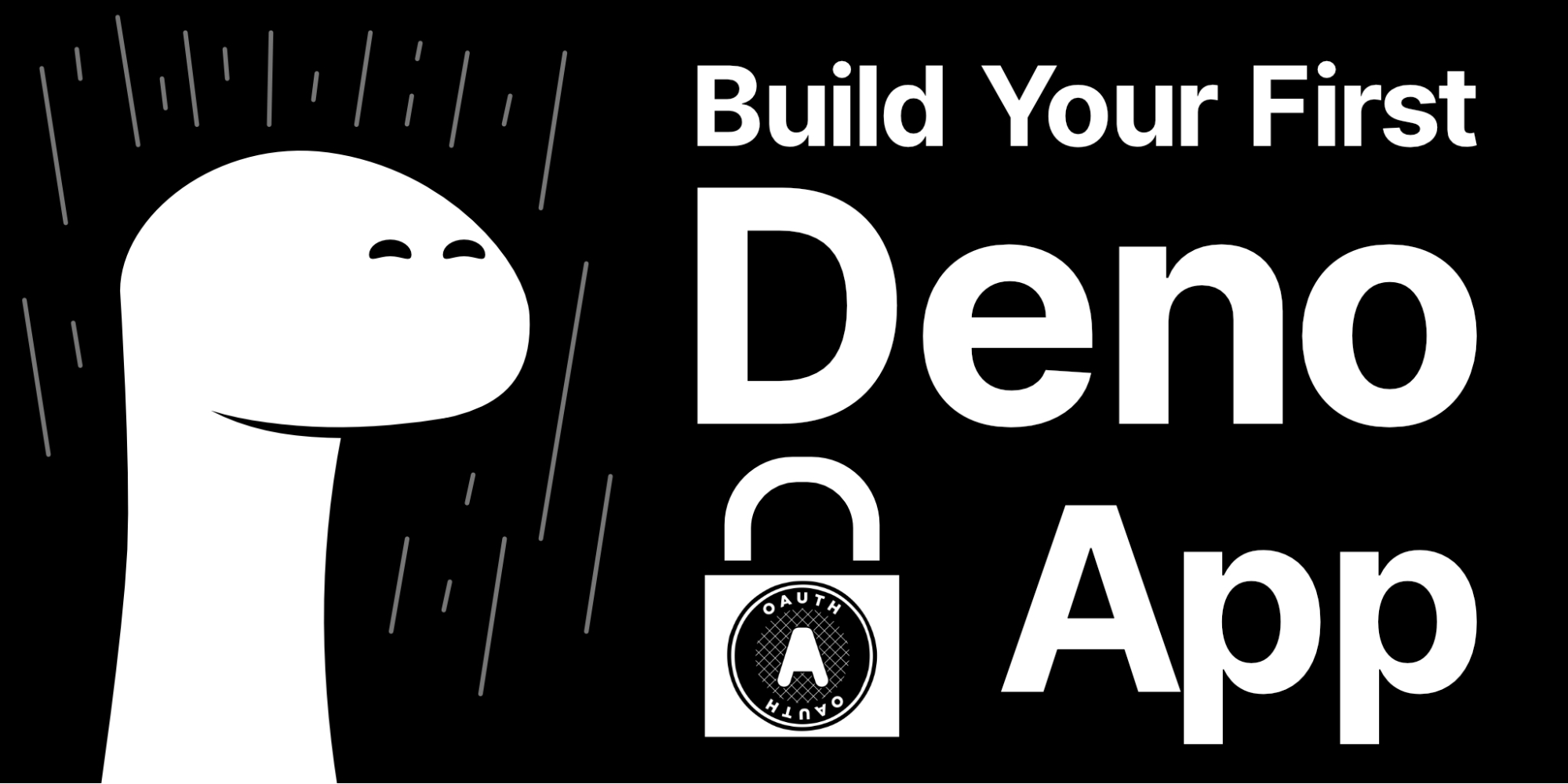
The creator of Node.js, Ryan Dahl, has authored a new framework for designing web applications. He went back and fixed some mistakes he made in hindsight, taking advantage of new technologies that were not available at the time he originally wrote Node. The result is Deno (pronounced DEH-no), a framework for writing “Node-like” web applications in TypeScript. Here, I will walk you through creating a basic web application with authentication. You can find almost all...
Build a Simple React Application Using Hooks
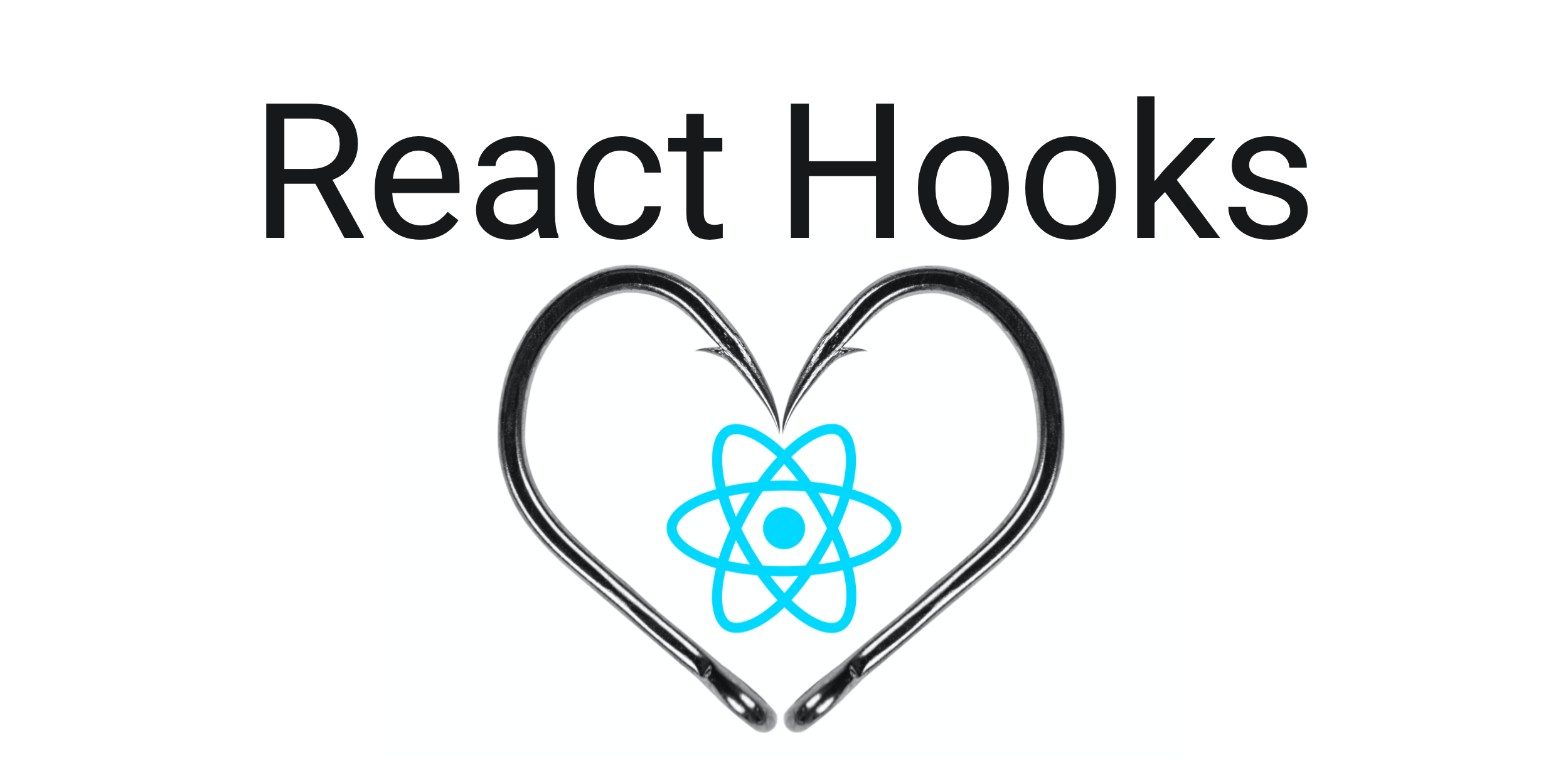
If you have been developing React applications, then you probably know that there are two ways of creating React components. You can create a component class that extends from React.Component. You then have to implement specific methods such as render() that renders the component. The alternative is to create a functional component. This type of component is simply a JavaScript function that returns a rendered element. Functional components are much shorter, they contain less boilerplate...
Build and Deploy Secure Serverless Functions with Netlify
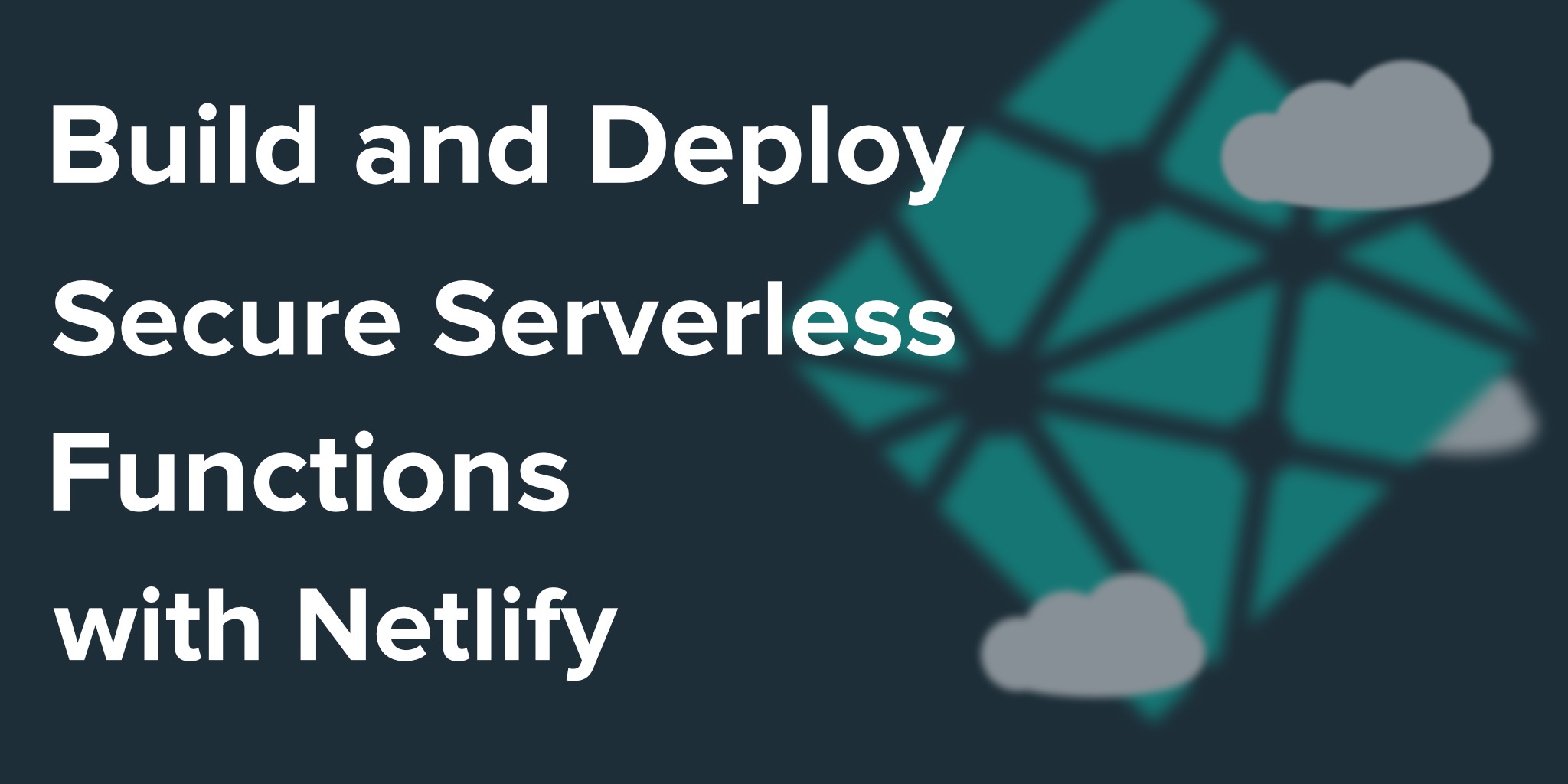
Serverless computing, sometimes referred to as “functions as a service” (FaaS), is an on-demand approach to providing backend application services. The serverless architecture is an excellent solution for many use cases where an application needs backend services occasionally, periodically (e.g., once a day), or dynamically scaled to meet demand. In this tutorial, you will learn to build serverless functions with the JavaScript language, deploy them to Netlify, and secure them using Okta. Netlify is a...
Quickly Build Node.js Apps with Sails.js
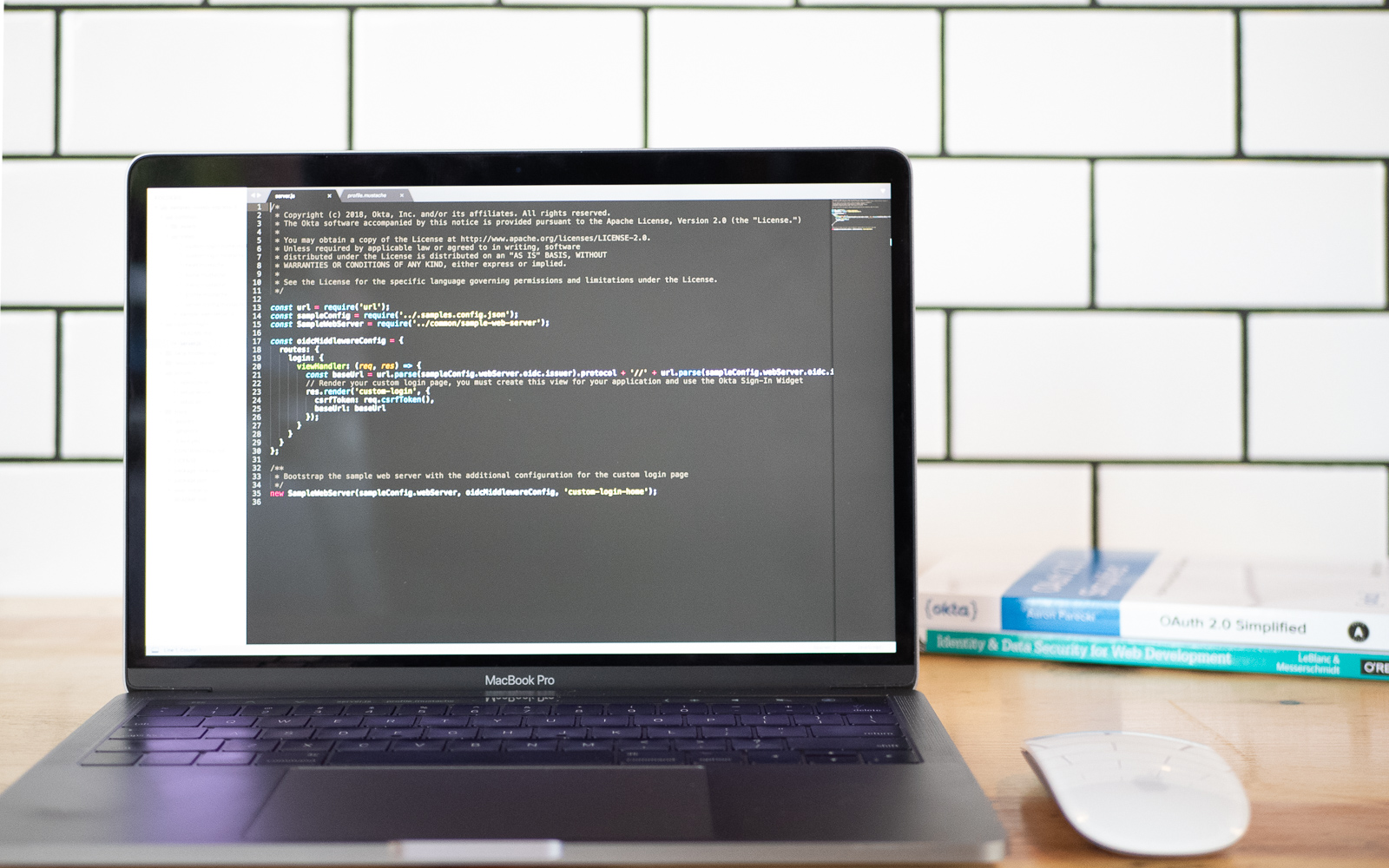
Sails.js is an exciting MVC framework for Node.js. Recently the team released version 1.0 and today you’re going to learn how to use the framework to rapidly build a new website. The hype around Sails.js is real. As a veteran MVC developer, I was impressed with the extensibility, organization, flow, and speed Sails.js provides. The team at Sails. js has done an excellent job of abstracting the MVC portion of the codebase away from the...
Node.js Login with Express and OIDC
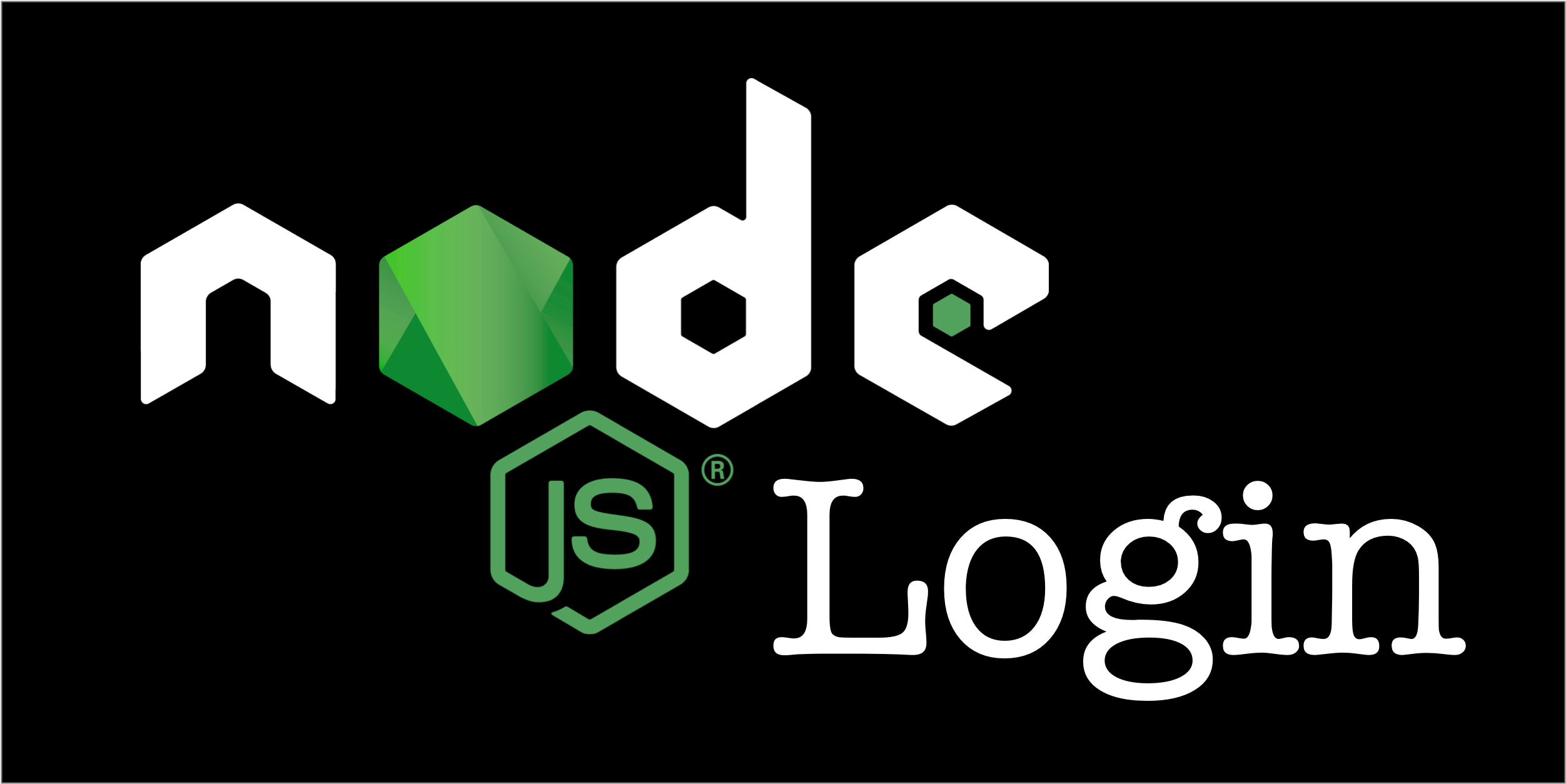
Node.js just celebrated its 11th birthday on May 27! With the state of things nows, it’s pretty crazy to think back to the massive JavaScript Renaissance boost of 2009. In case you’re not aware, the JavaScript Renaissance began in around 2004 with Ajax, increased exponentially with jQuery in the mid-2000s, and then really took off with Node.js and a plethora of early JavaScript web frameworks; including Backbone.js, Ember.js, and AngularJS. Today, I’d like to show...
Vue Login and Access Control the Easy Way
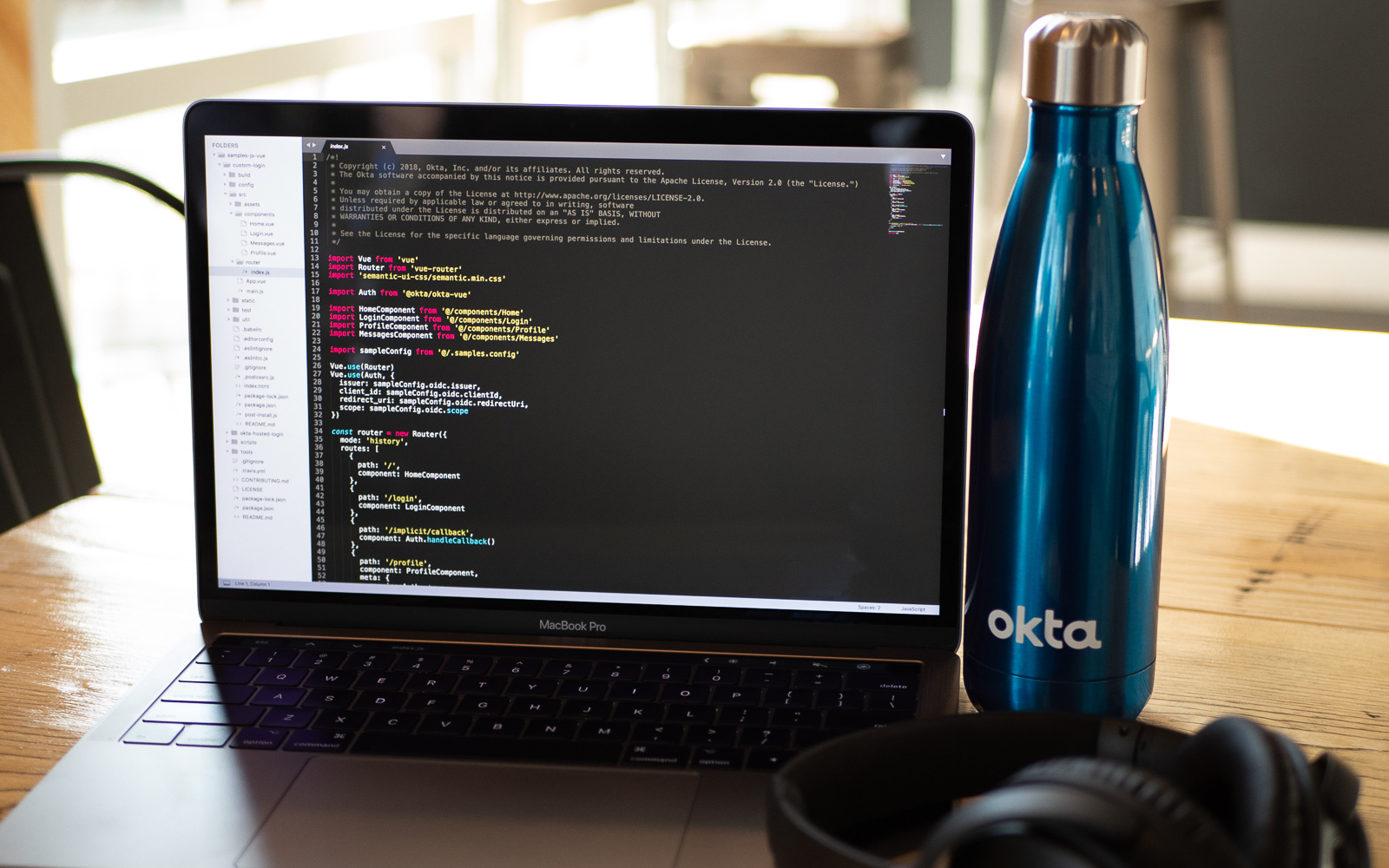
Vue lets you create complex and high-performance web applications. As a front-end JavaScript framework, it’s becoming more popular than ever. Vue Router is the official router for Vue. It facilitates routing in single-page applications, and provides a way to process user authentications and restrict page access. Combined with Okta, implementing secure login in a Vue app can be accomplished in a matter of minutes. User management is an important aspect of nearly all web applications,...
How to Build a Secure AWS Lambda API with Node.js and React
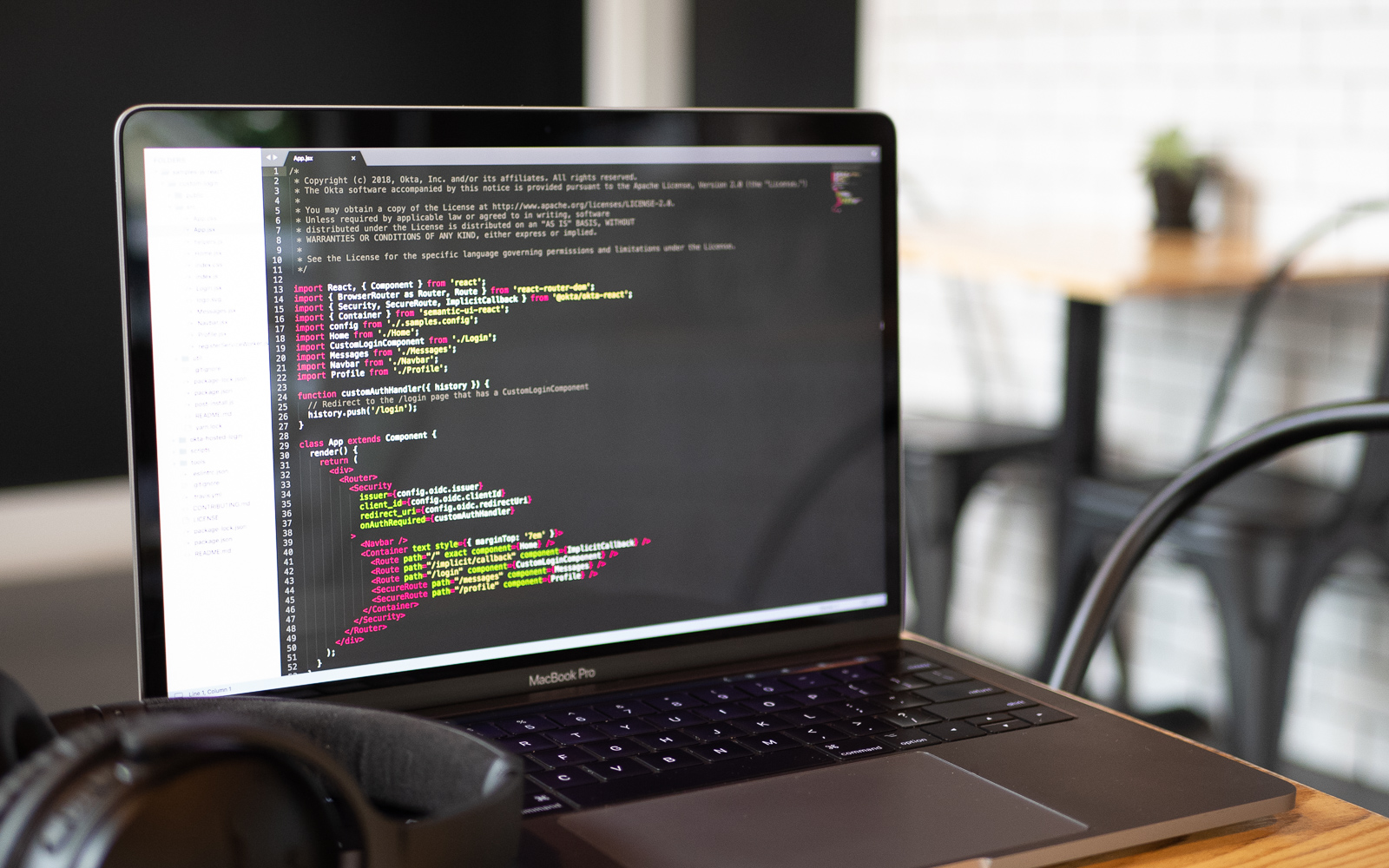
Serverless architecture with AWS Lambdas is quickly becoming a popular option for companies looking to deploy applications without the overhead of maintaining servers. AWS Lambda functions are event-driven and serverless—triggered to process a piece of code and return a result. AWS Lambdas can be written in most common languages today on a variety of platforms including .NET Core, Java, Go and, in the case of this post, Node.js. The example in this post uses Node.js...
Build a React App with Styled Components
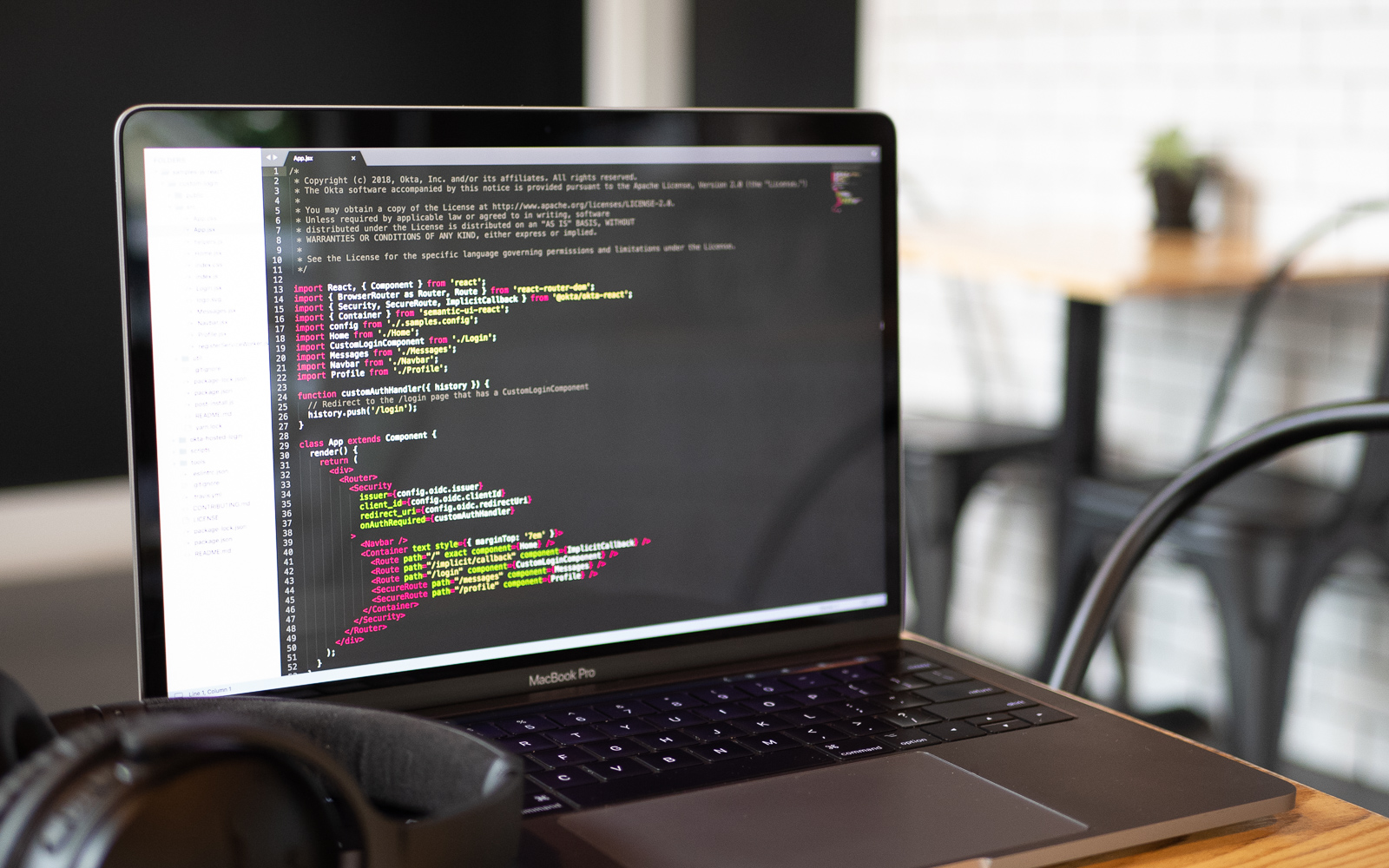
When you create React applications, at some point you have to make a decision on how to organize your CSS styles. For larger applications, you’ll have to modularize the stylesheets. Tools such as Sass and Less let you divide up your styles into separate files and provide lots of other features that make writing CSS files more productive. But some problems remain. The tools separate your styles from your components, and keeping the styles up-to-date...
Stop Writing Server-Based Web Apps
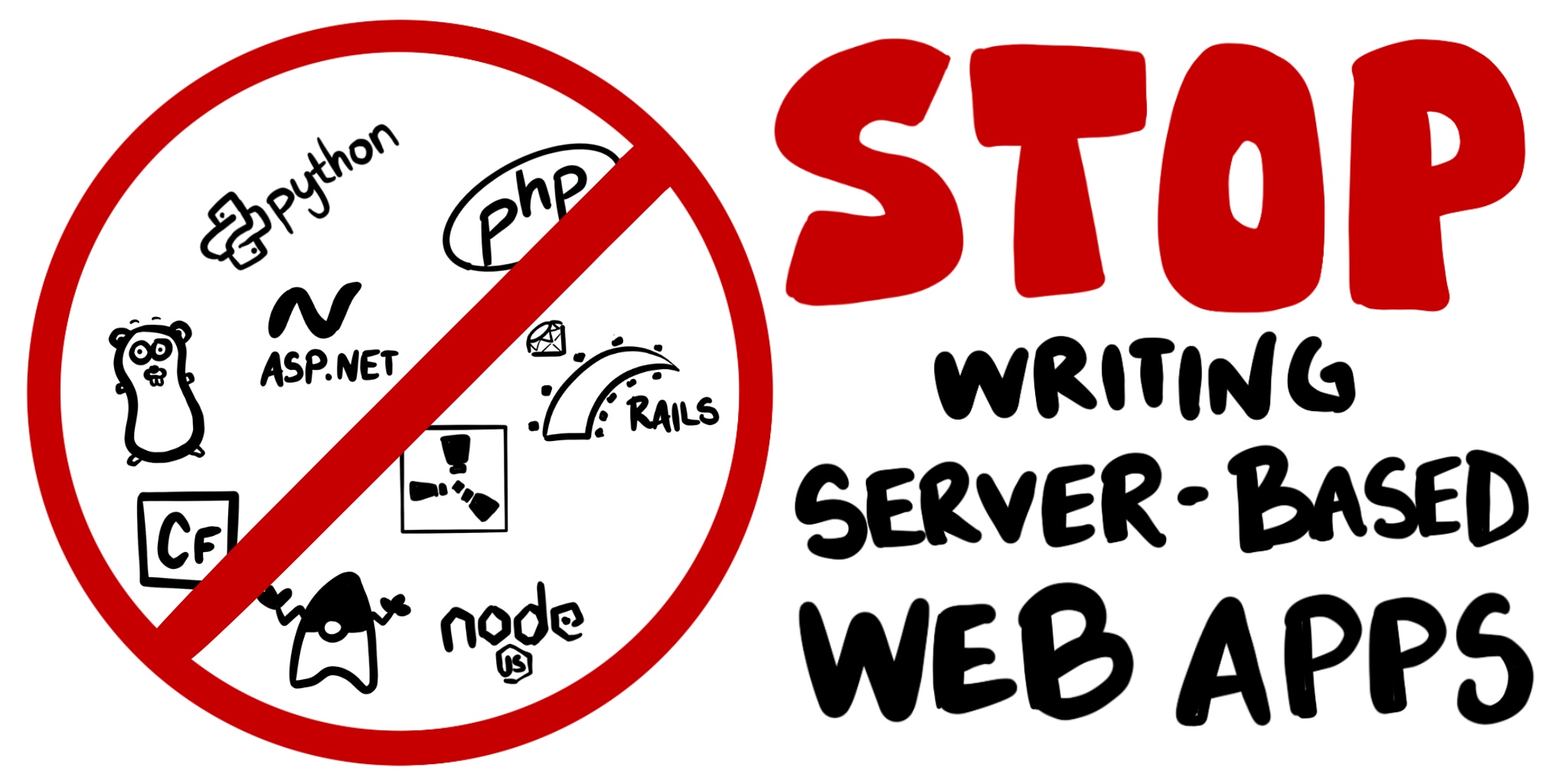
The World-Wide Web, as we know it, started around 1993 by serving static HTML files with links to other HTML files. It didn’t take long for developers to find ways of making websites more “dynamic” using technologies like Common Gateway Interface (CGI), Perl, and Python. Since the ’90s, I have built web applications using a variety of languages, platforms, and frameworks. I’ve written application frameworks, content management systems, a blog engine, and a social media...
Build a Secure NestJS API with Postgres
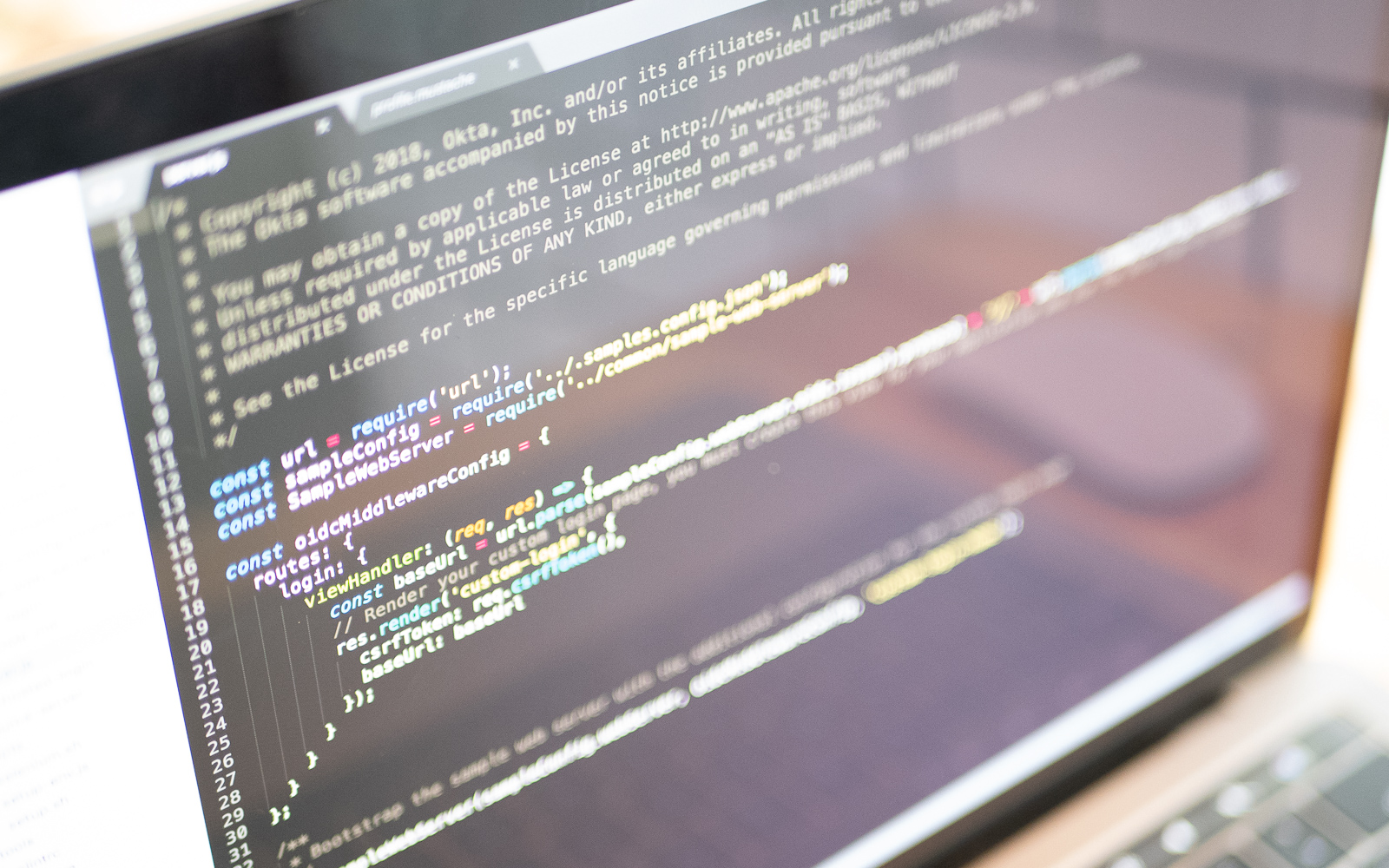
NestJS is a modern, progressive framework for building Node.js applications and APIs. NestJS is built on TypeScript, and is designed to use solid programming metaphors such as controllers and modules. Having automatic Swagger API documentation built-in is also a great feature. Postgres (or PostgreSQL), much like other relational databases, provides a way to persist and query data. It’s a powerful, open-source, object-relational database system with over 30 years of active development that has earned it...
Build Reusable React Components
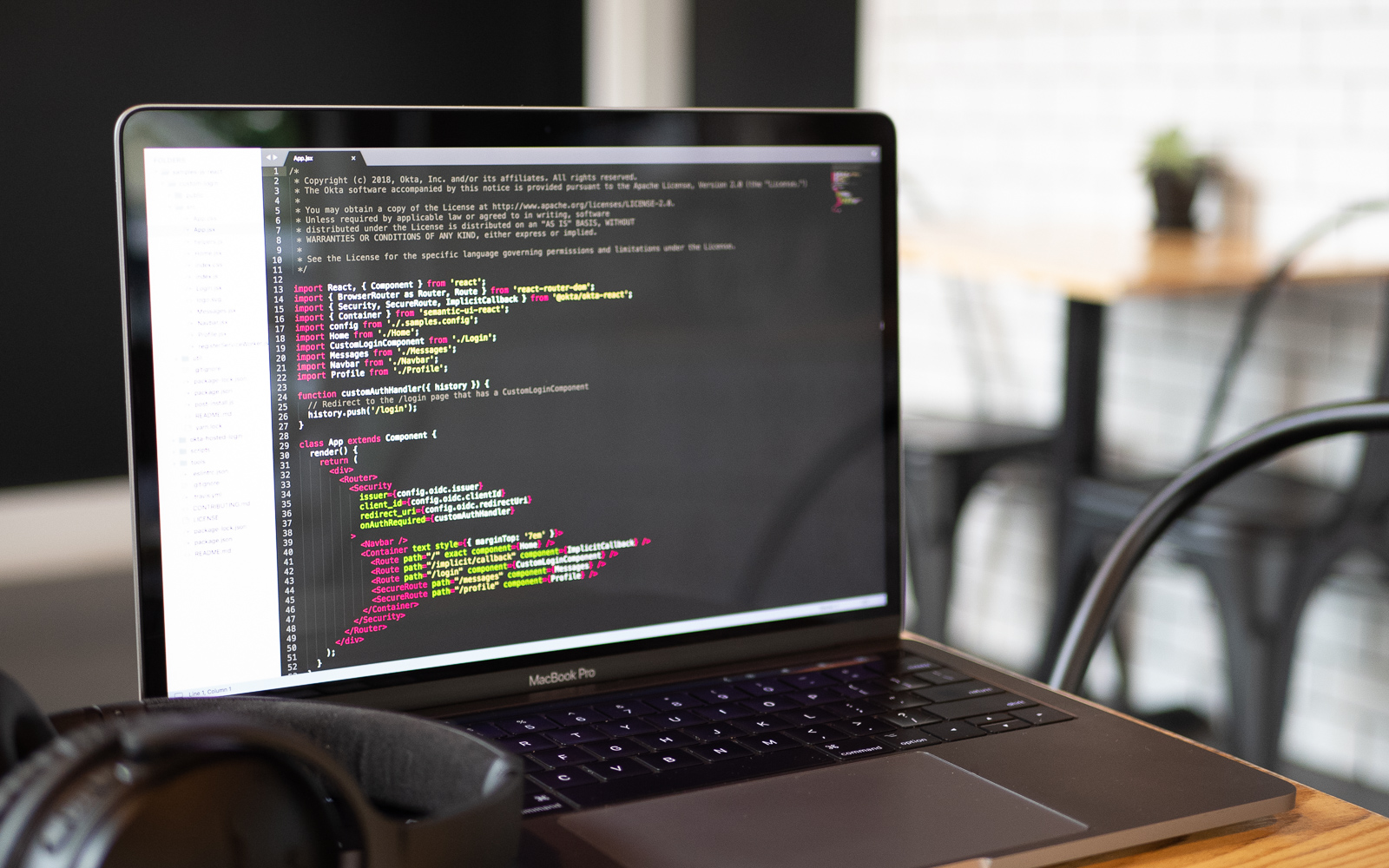
Who doesn’t love beer? When you drink a great beer you want to tell someone. You definitely want to be able to remind yourself of the great beers you’ve had. Enter Brewstr, a beer rating application that allows you to enter a beer you’re drinking and give it a rating. This way, you know what to get next time since there’s no way you’ll remember it later. React gives the ability to create a component...
Build Components in JavaScript Without a Framework
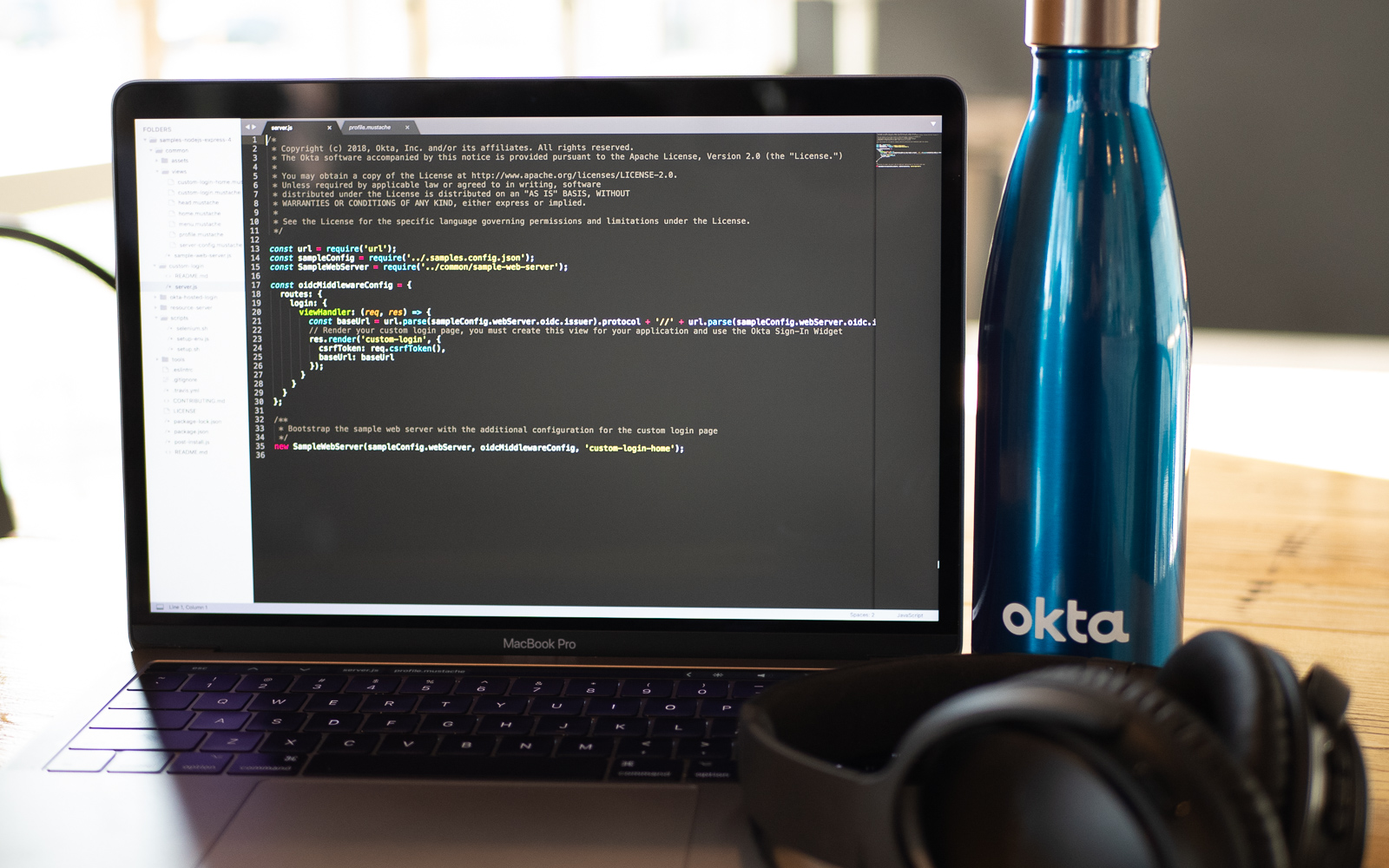
Everyone has their favorite framework, and most developers aren’t shy about sharing those opinions. I guarantee you right now two developers are arguing about their favorite frameworks. Personally, I’ve been using JavaScript frameworks since JQuery was introduced. I’ve written applications for clients using Knockout.js, Angular 1+, React (since before v15), and have made some small learning apps using Stencil and Vue. One of the great things that all of these frameworks bring to the table...
Use Sass with React to Build Beautiful Apps
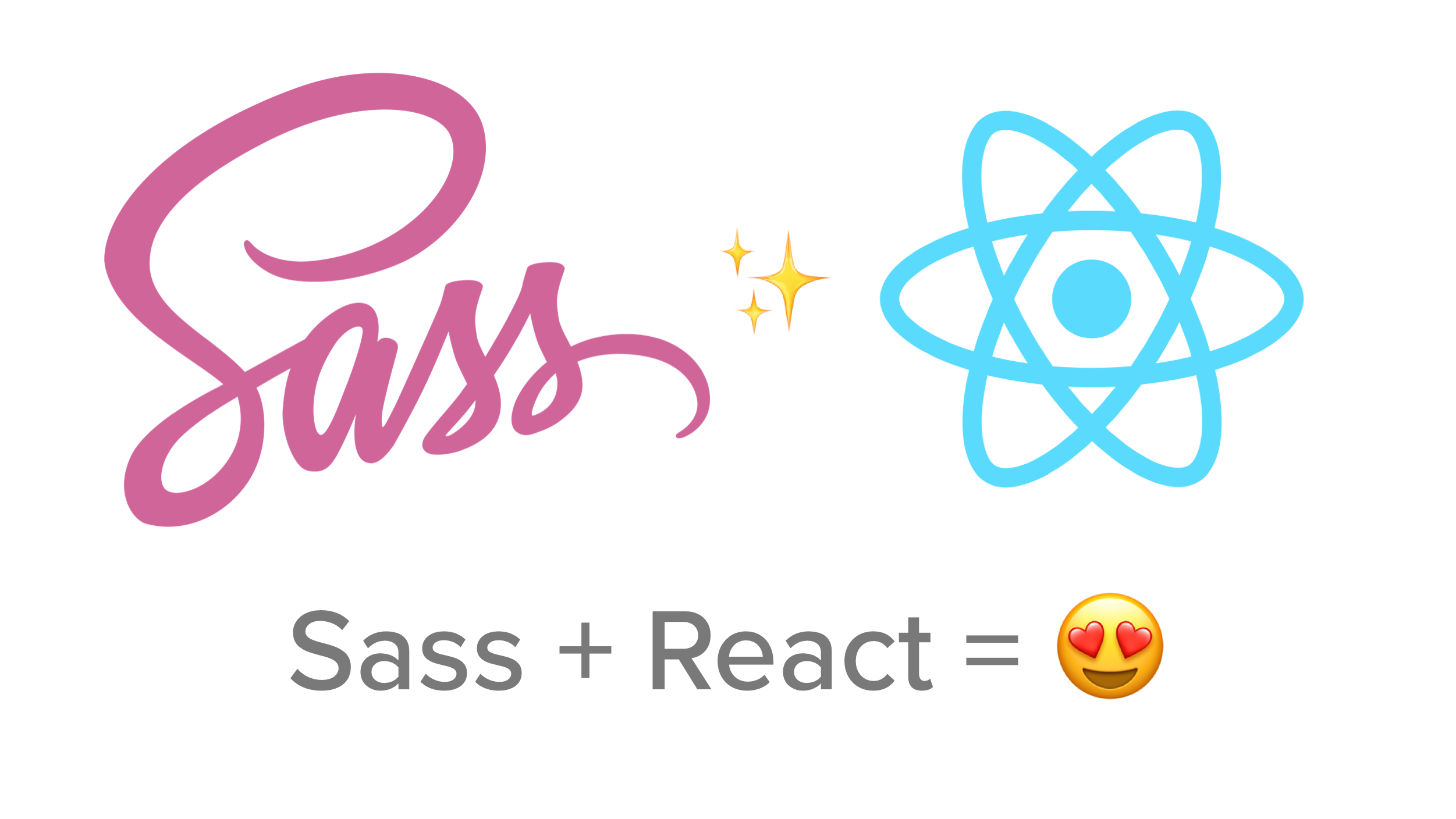
When you are developing web applications with React, you know that writing the JavaScript code is only half of the story. The other half is implementing the design using style sheets. When your application becomes larger, using plain CSS style sheets can become tedious and unmaintainable. Sass is one of the most popular alternatives to CSS. It extends the CSS language with variables, mixins, and many other features. It also lets you divide up the...
How to Customize Your Angular Build With Webpack
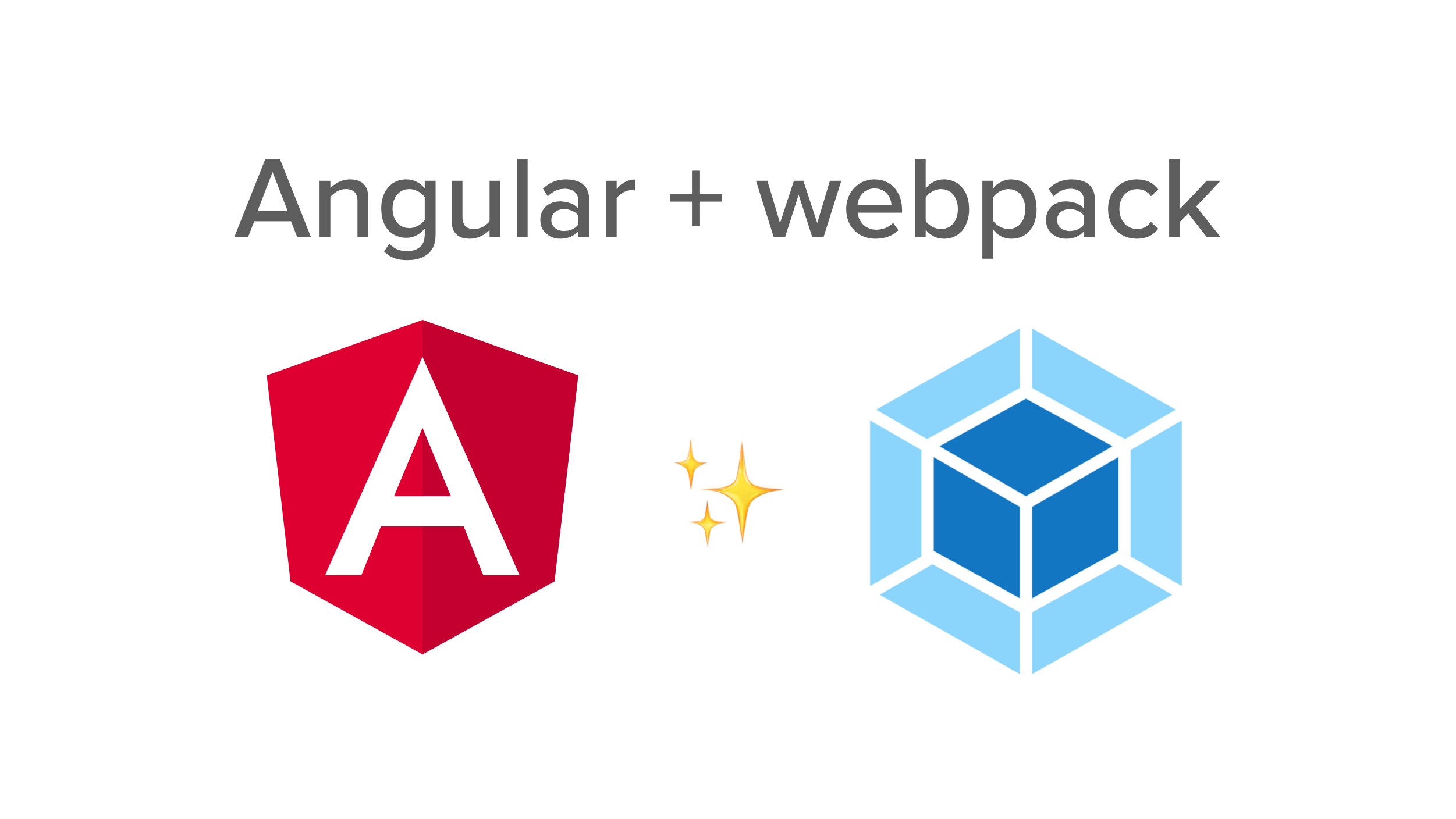
If you’re a frontend dev in the world today you’ve probably heard of (and possibly even used) webpack. The Angular build process uses webpack behind the scenes to transpile TypeScript to JavaScript, transform Sass files to CSS, and many other tasks. To understand the importance of this build tool, it helps to understand why it exists. Browsers have very limited support for JavaScript modules. In practice, any JavaScript application loaded into the browser should be...
A Quick Guide to Integrating React and GraphQL
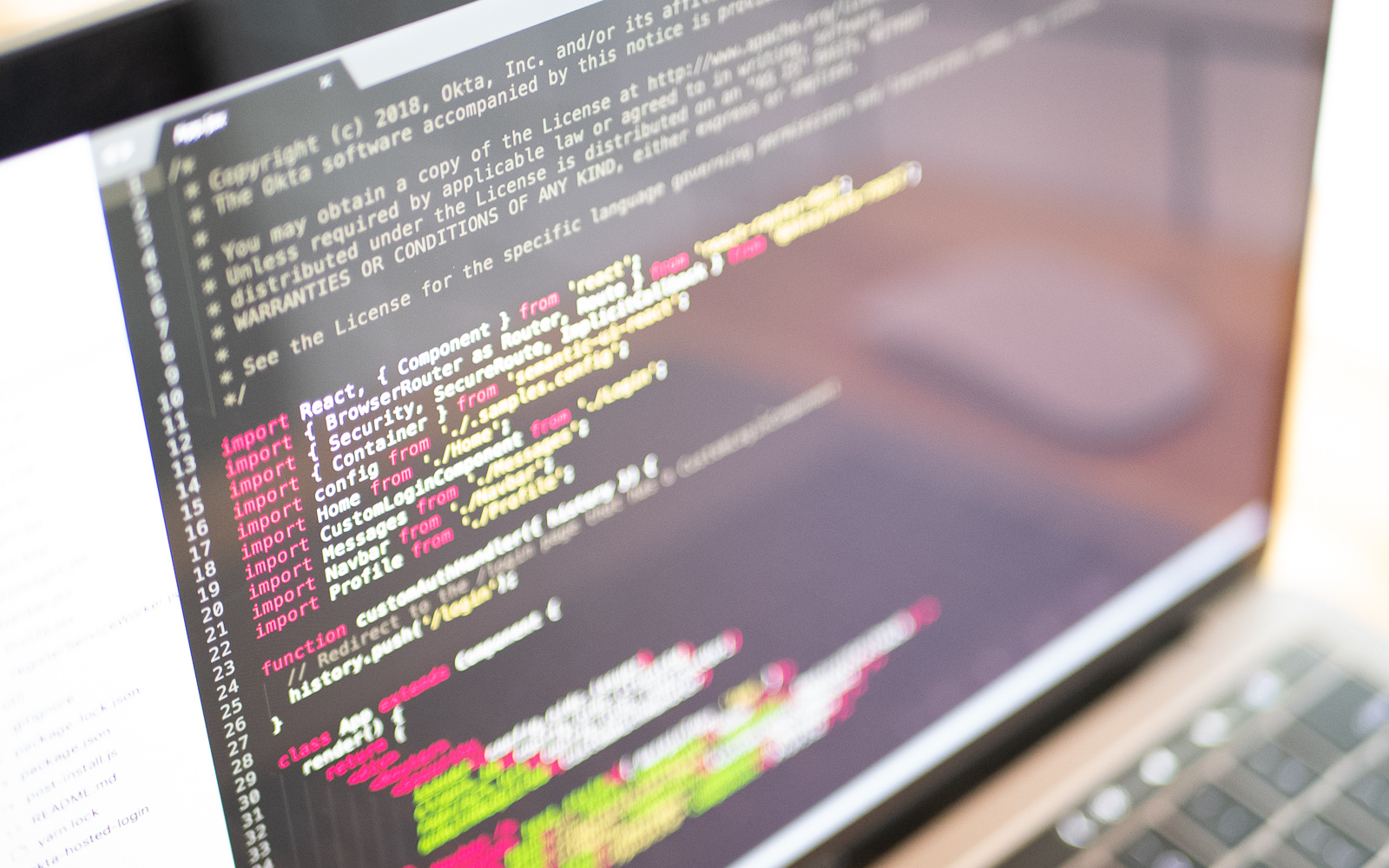
If your application consumes a ReST API from React, the default setup will give you ALL the data for a resource. But if you want to specify what data you need, GraphQL can help! Specifying exactly the data you want can reduce the amount of data sent over the wire, and the React applications you write can have less code filtering out useless data from data you need. There are a lot of GraphQL clients...
What's New for Node.js in 2020
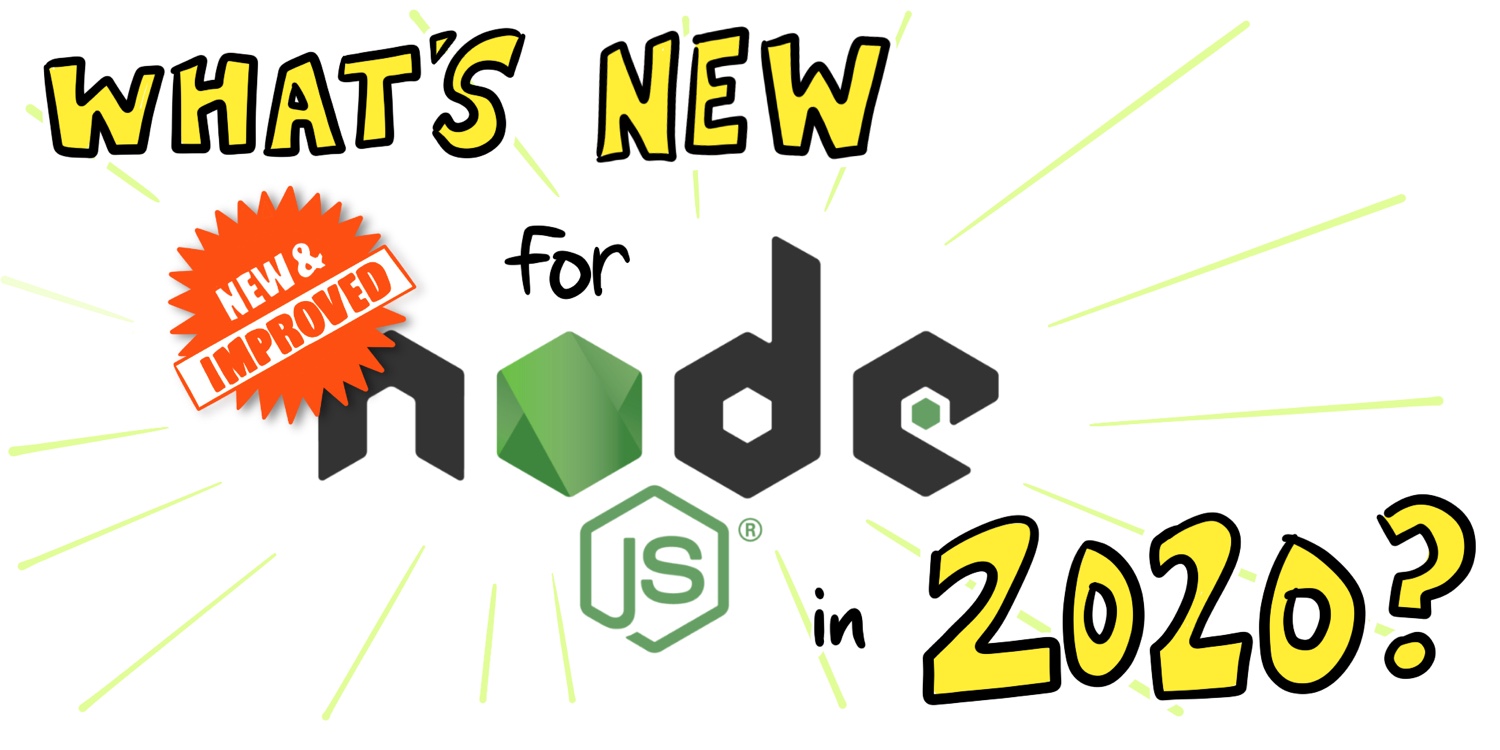
In 2019, Node.js turned 10 years old, and the number of packages available on npm crossed one million. Downloads for Node.js itself continues to rise, growing 40% year over year. Another significant milestone is Node.js recently joined the OpenJS Foundation, which promises to improve project health and sustainability, as well as improve collaboration with the JavaScript community at large. As you can see, a lot has happened in a relatively short amount of time! Every...
Create a React Native App with Login in 10 Minutes
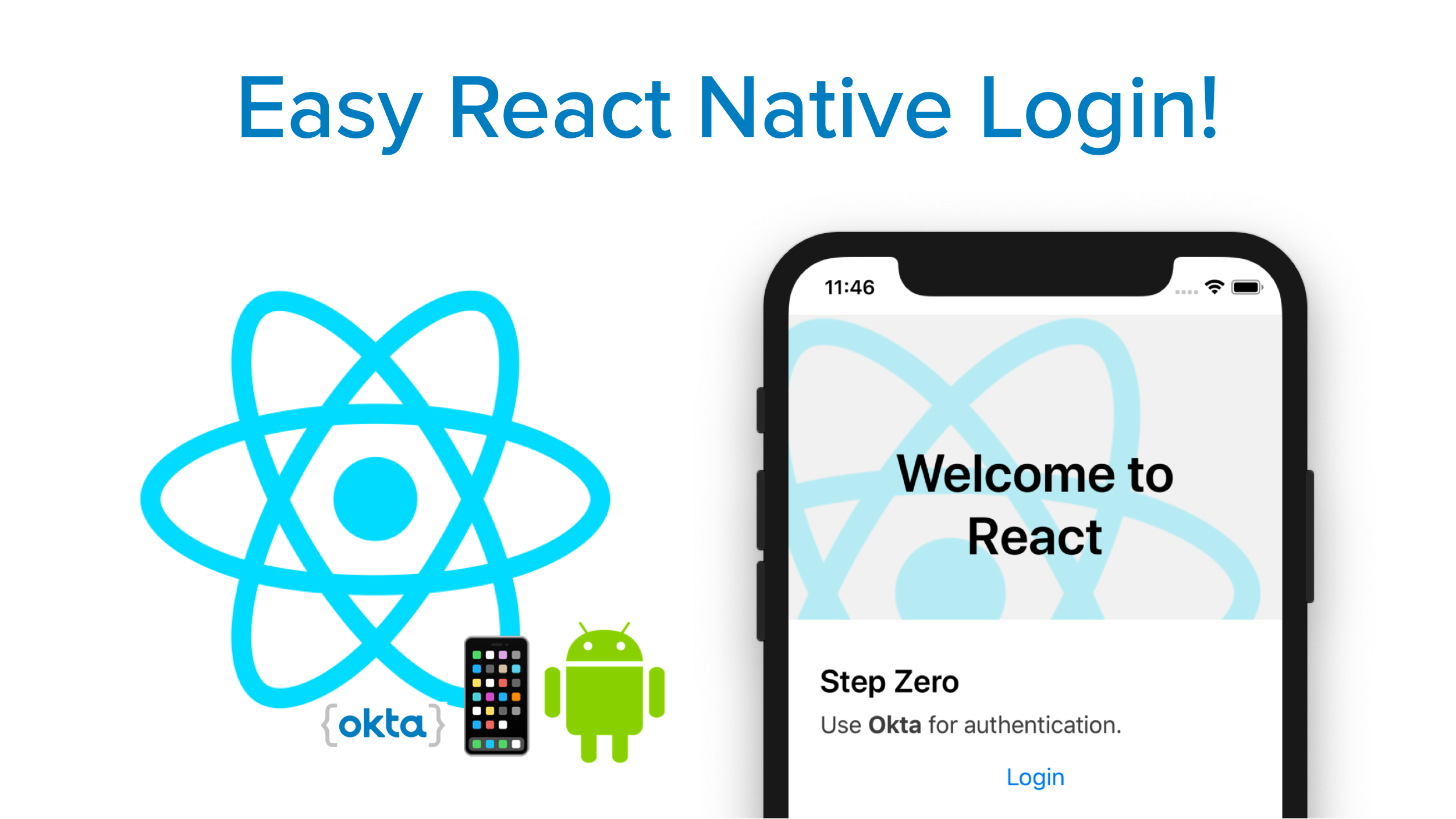
React Native is a mobile app development framework that allows you to use React to build native iOS and Android mobile apps. Instead of using a web view and rendering HTML and JavaScript, it converts React components to native platform components. This means you can use React Native in your existing Android and iOS projects, or you can create a whole new app from scratch. In this post, I’ll show you how to add a...
Use Vue and GraphQL to Build a Secure App
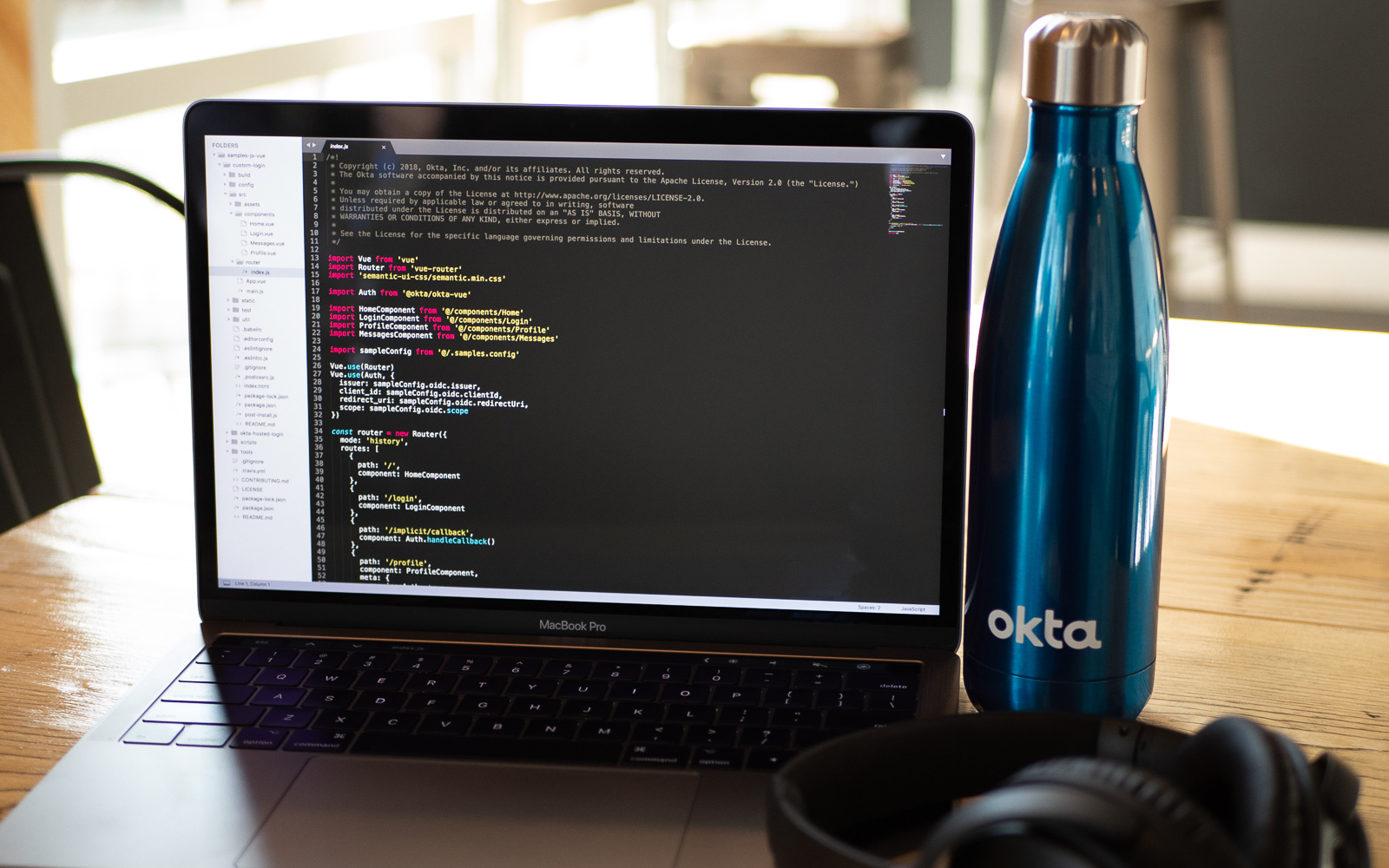
When you develop a new web application, you most likely split the application into two parts. The server-side provides access control and data persistence, while the client-side is mostly presentational. The two parts have to communicate, and your first idea may be to implement a REST API. However, a number of flaws have been identified with the RESTful pattern, mostly related to the flexibility and speed of the request-response pattern. At Facebook, these problems were...
Secure and Scalable: An Introduction to JAMStack
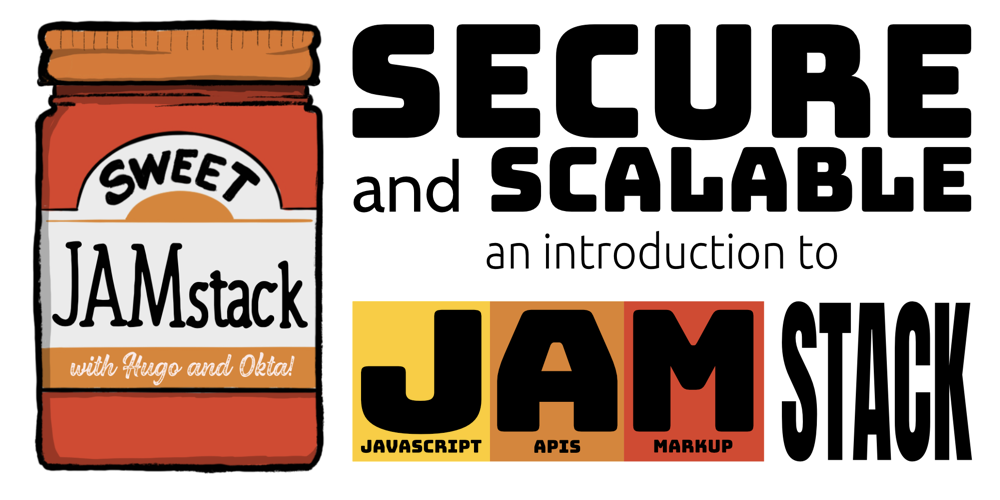
If you’re a web developer, chances are you have heard the term “JAMstack.” Curiously, JAMstack isn’t a solution to prevent clogged printers, something to eat on toast, or a way to make music. However, by the time you finish reading this tutorial, you’ll understand JAMstack and its benefits, and learn one approach to implementing JAMstack for yourself. Let’s get ready to JAM. The “JAM” in JAMstack stands for JavaScript, APIs, and Markup. JAMstack’s pattern of...
Painless Node.js Authentication
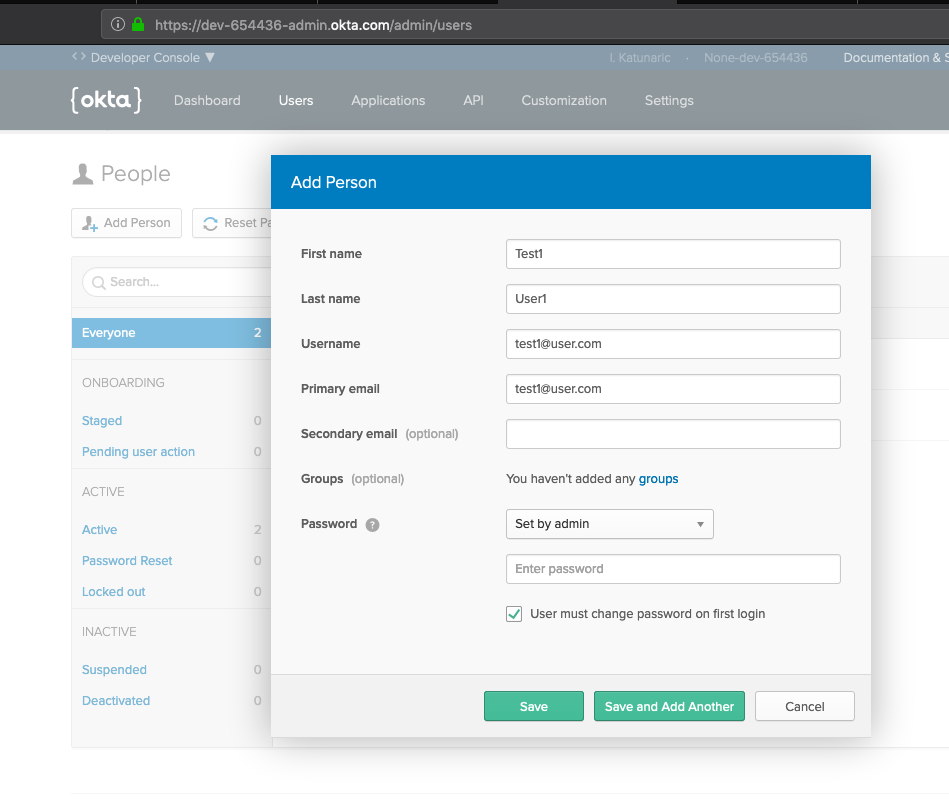
User authentication is a critical component of just about every web application. Unfortunately, while authentication is a core part of all websites, it can still be difficult to get right. Despite the Node.js community being around for a while, there still aren’t a lot of simple, foolproof ways to authenticate users in Node.js applications. In this article I’m going to explain how to build a Node.js application that authenticates users in a best practices way....
Build a NodeJS App with TypeScript
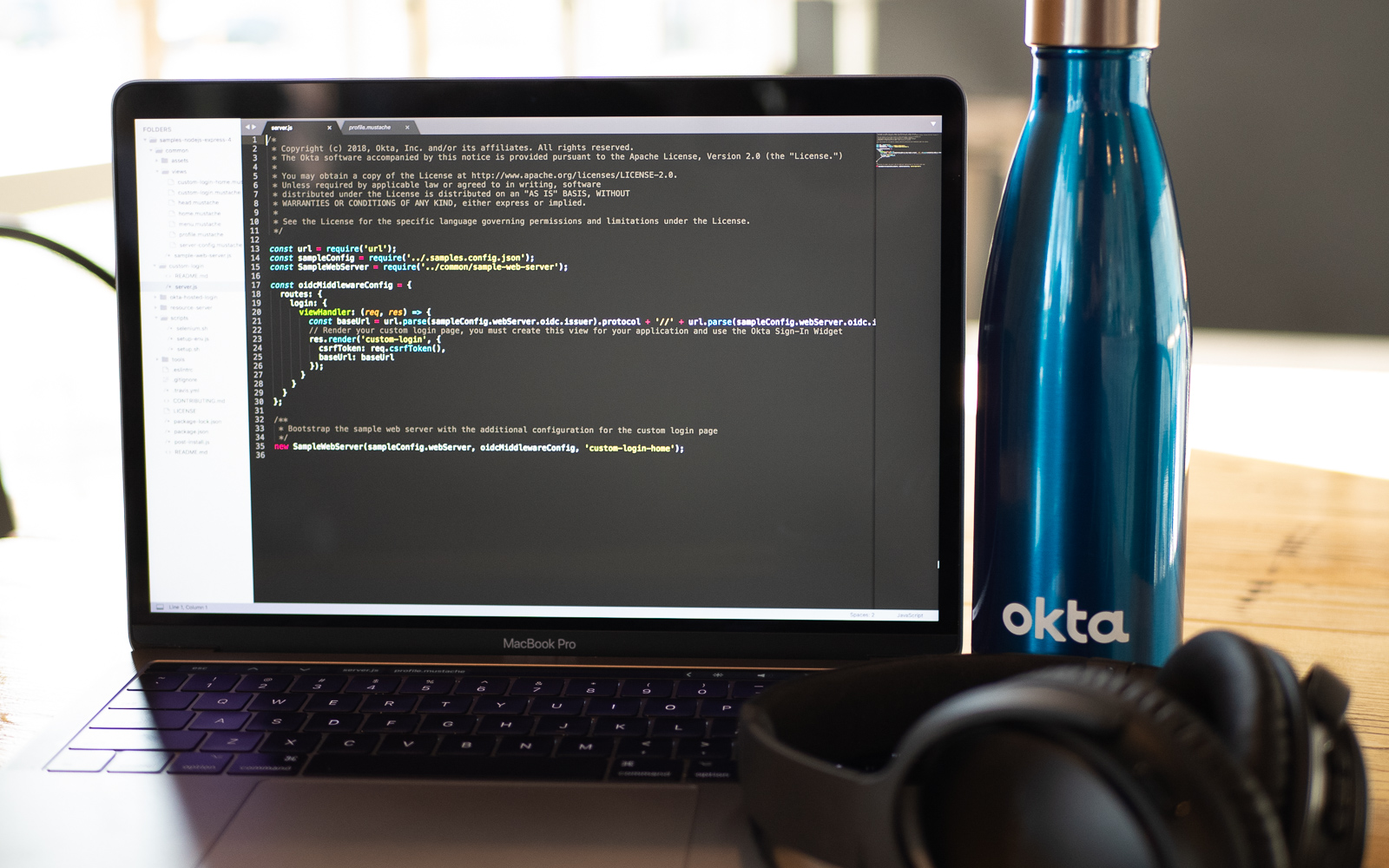
As dynamically typed languages became prominent during the last decade, typeless (or should I say lawless?) programming became the norm for the backend as well as the frontend. Many people believe the simplicity of “just writing” code is efficient for providing a proof of concept or prototyping applications. However, as those applications grow, the typeless code used to build them often becomes incredibly convoluted and more difficult (some would say impossible) to manage. In the...
How to Connect Angular and MongoDB to Build a Secure App
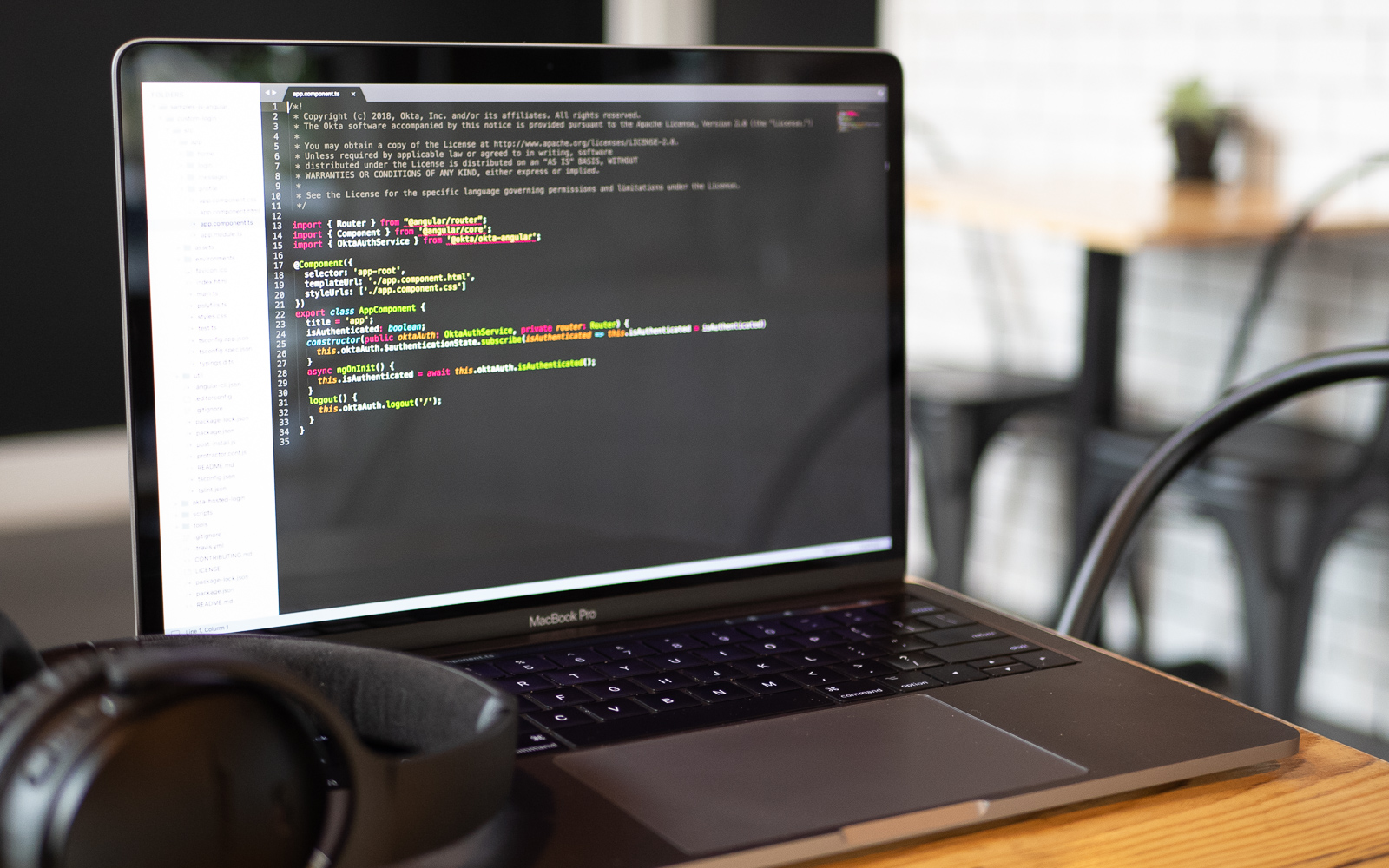
MongoDB is often the first suggestion when it comes time to select a NoSQL database. But what is a NoSQL database, and why would you want to use one in the first place? To answer this question, let’s step back and look at SQL databases and where they shine. SQL databases are a good choice if you have well-defined data that will not change much over time. They also allow you to define complex relationships...
Use Vue.js Data Binding Options for Reactive Applications
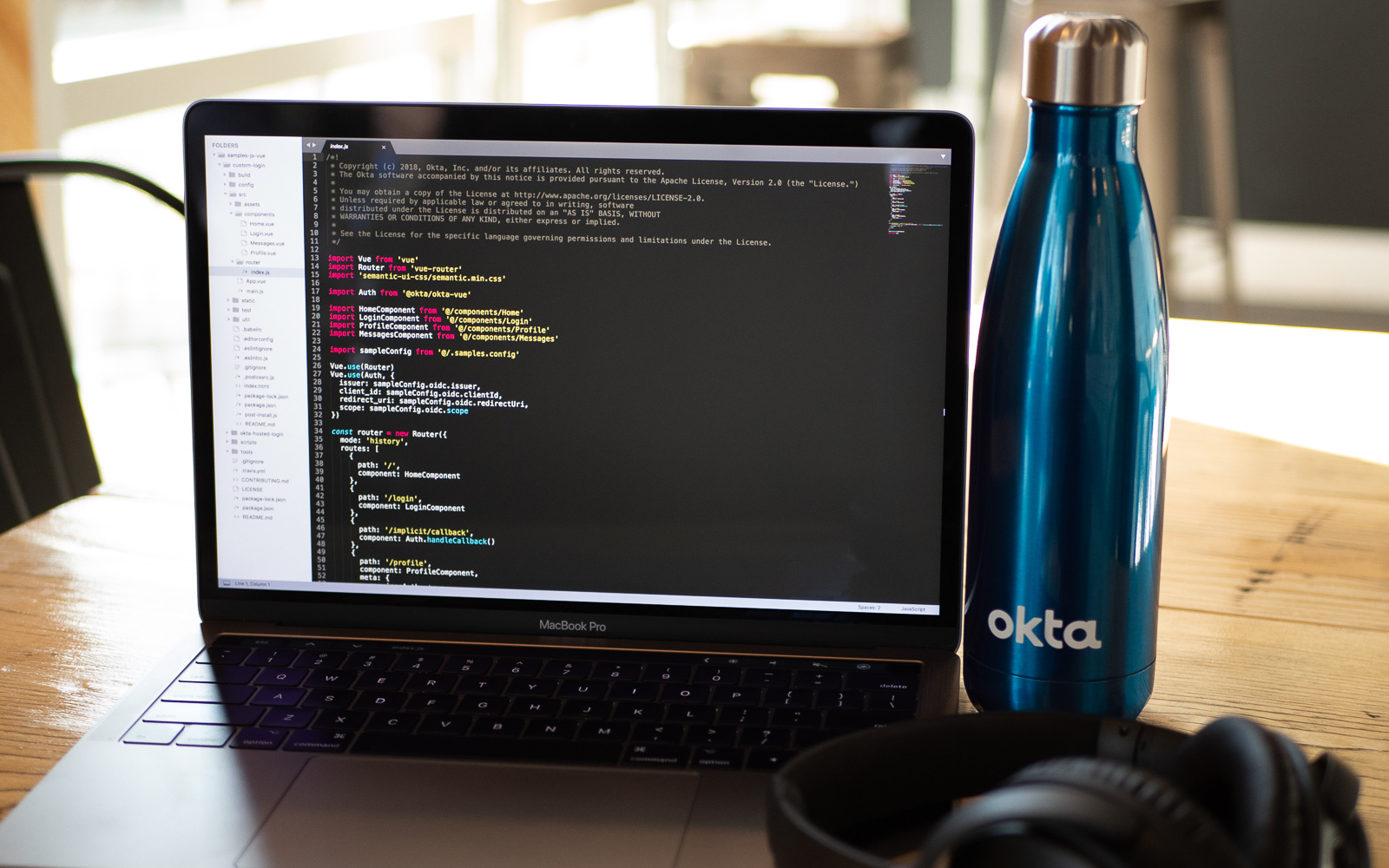
Vue.js is known as a “progressive framework for building user interfaces”. There’s a lot to unpack in this simple statement. It’s easy to get started with Vue.js, with a minimal feature set, and then layer in more of the framework as you need it. Unike React, it has full support for the MVC (Model View Controller) pattern out-of-the-box. It’s easier to use and grow with than Angular. And, if you couldn’t tell, I’m a little...
Add Authentication and Personalization to VuePress
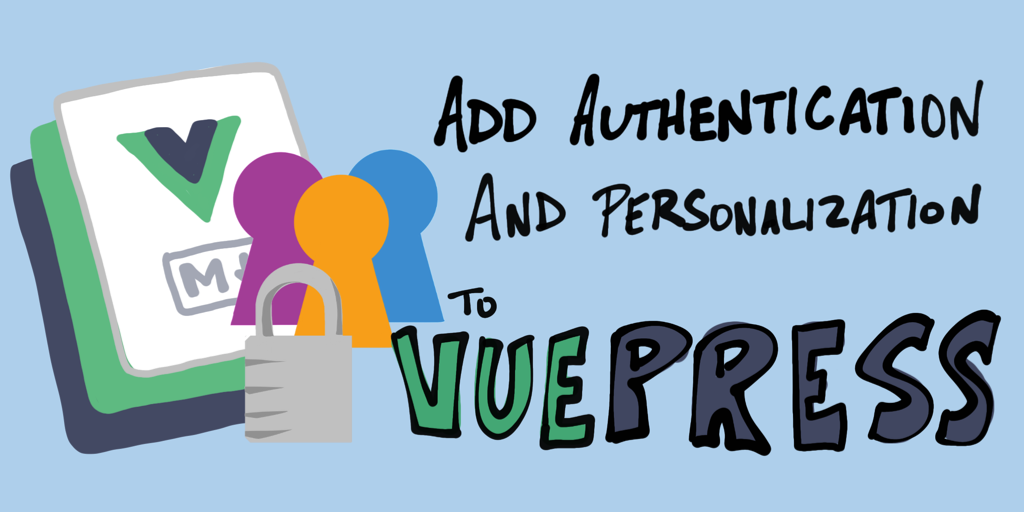
There are several advantages to using a static site generator such as VuePress. With VuePress, you can focus on writing content using markdown, and the VuePress application generates static HTML files. VuePress also turns your content into a single-page application (SPA), so transitions between pages seem instant and seamless. The generated static files can be cached and distributed across a content delivery network (CDN) for even more performance. For the reader, VuePress creates a great...
Build a Phone System for Your Company With Twilio, Okta, and JavaScript
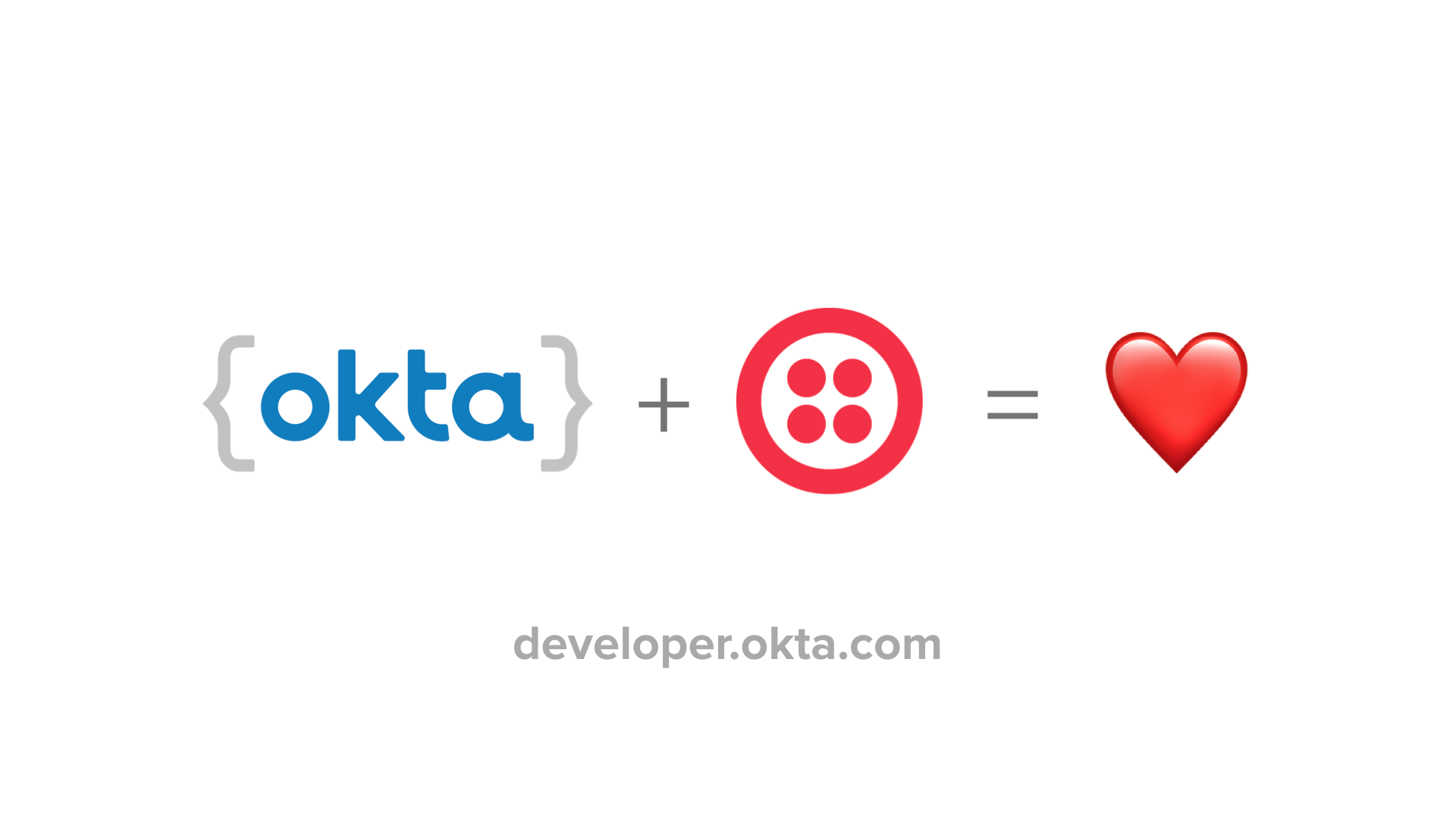
If you’ve ever worked for a company with more than a few employees, you’ve probably seen some interesting phone systems. When I used to work at Cisco, everyone was given a dedicated Cisco desk phone that hooked up to a server somewhere in the company and each employee was assigned a unique phone number and extension. I never really liked that setup. It annoyed me that I had this big, clunky desk phone taking up...
Angular Authentication with JWT
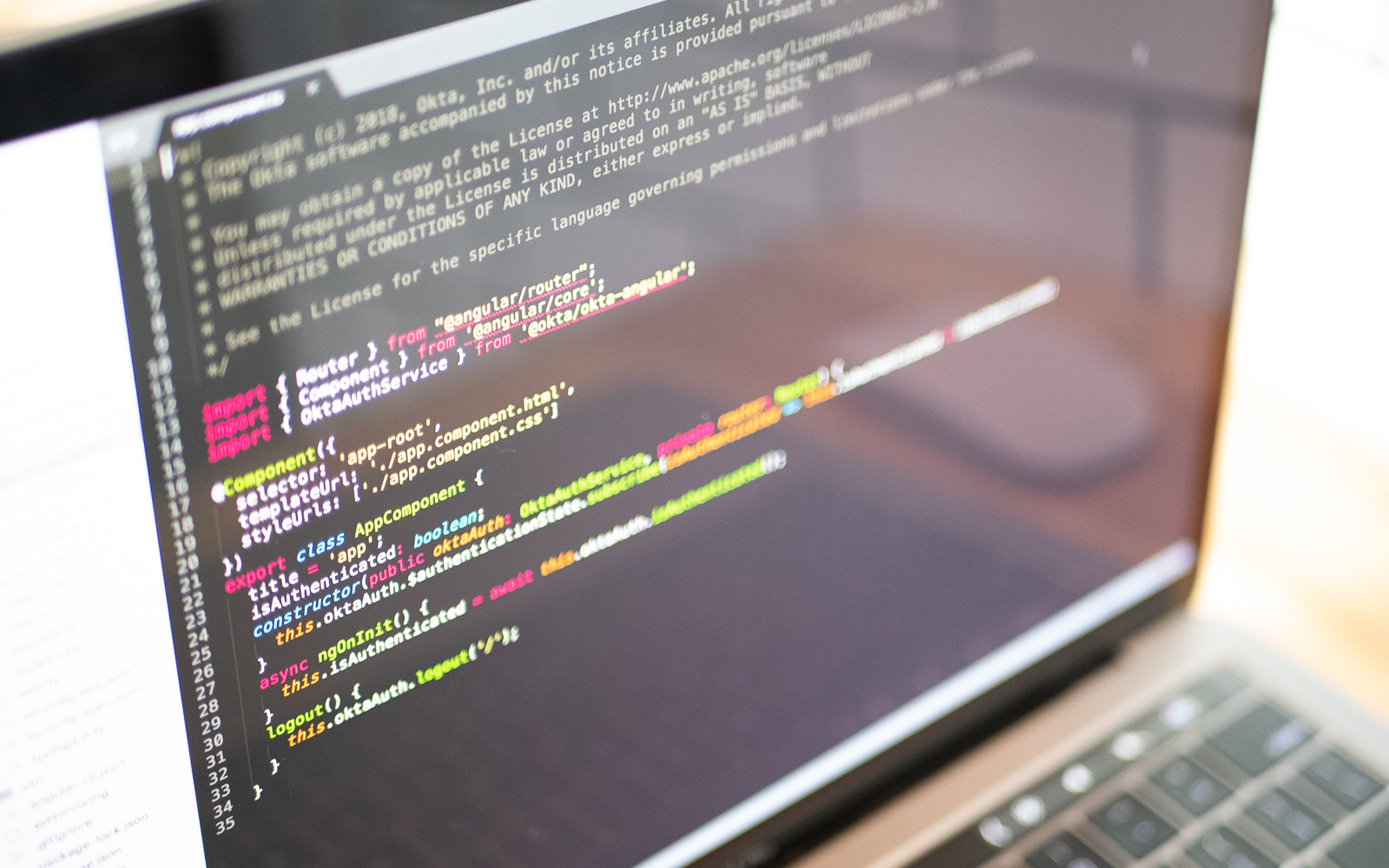
User registration and authentication are one of the features that almost no web application can do without. Authentication usually consists of a user entering using a username and a password and then being granted access to various resources or services. Authentication, by its very nature, relies on keeping the state of the user. This seems to contradict a fundamental property of HTTP, which is a stateless protocol. JSON Web Tokens (JWTs) provide one way to...
Top 10 Visual Studio Code Extensions for Node.js
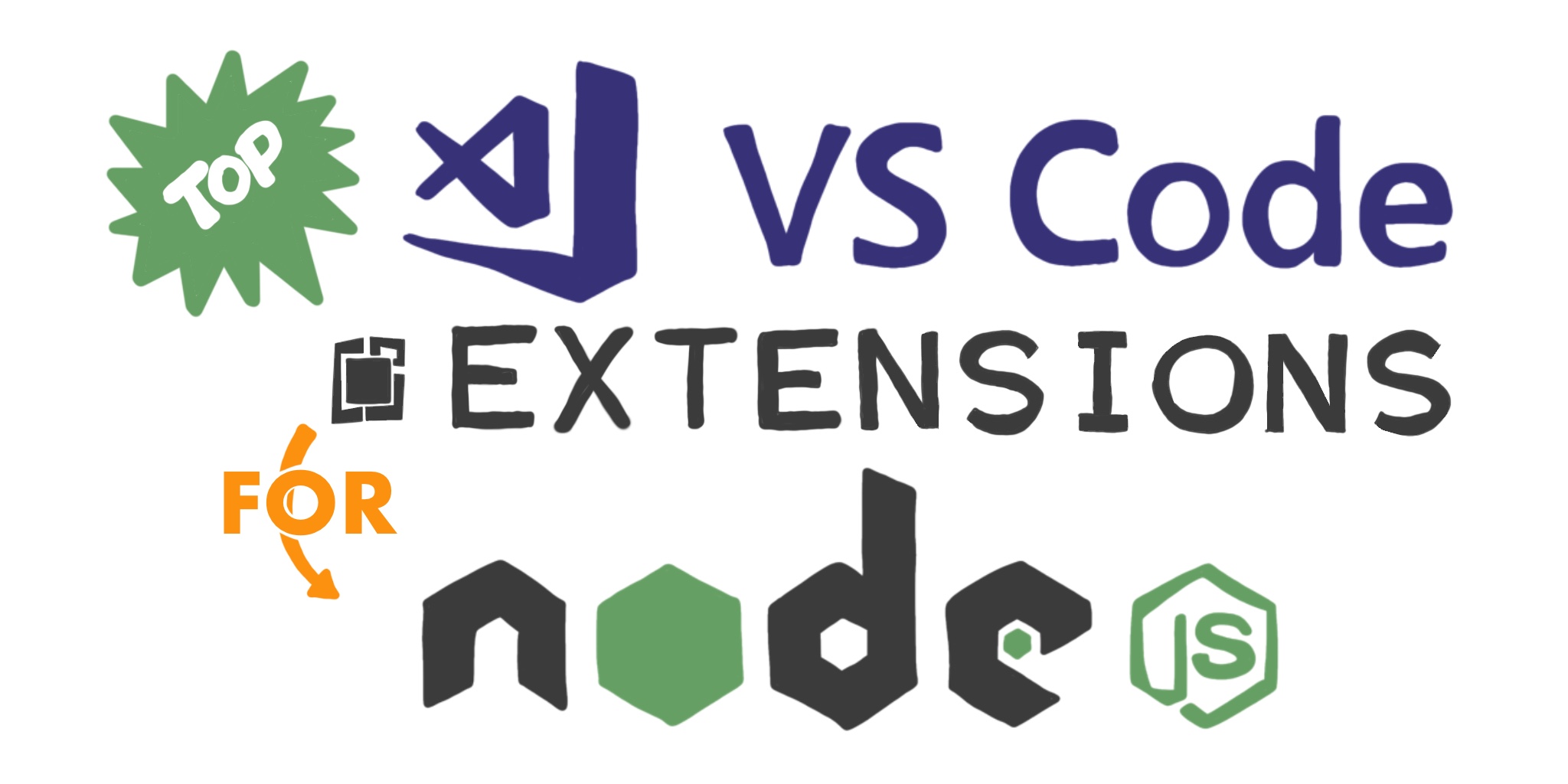
I am amazed at the adoption of Visual Studio Code by developers from all platforms and languages. According to the 2019 Stack Overflow Developer Survey, VS Code is dominating. The primary reasons I use VS Code are its great support for debugging JavaScript and Node.js code, and how easy it is to customize with free extensions available in Visual Studio Marketplace. However, there are thousands of extensions available! How do you know which ones are...
Is the OAuth 2.0 Implicit Flow Dead?
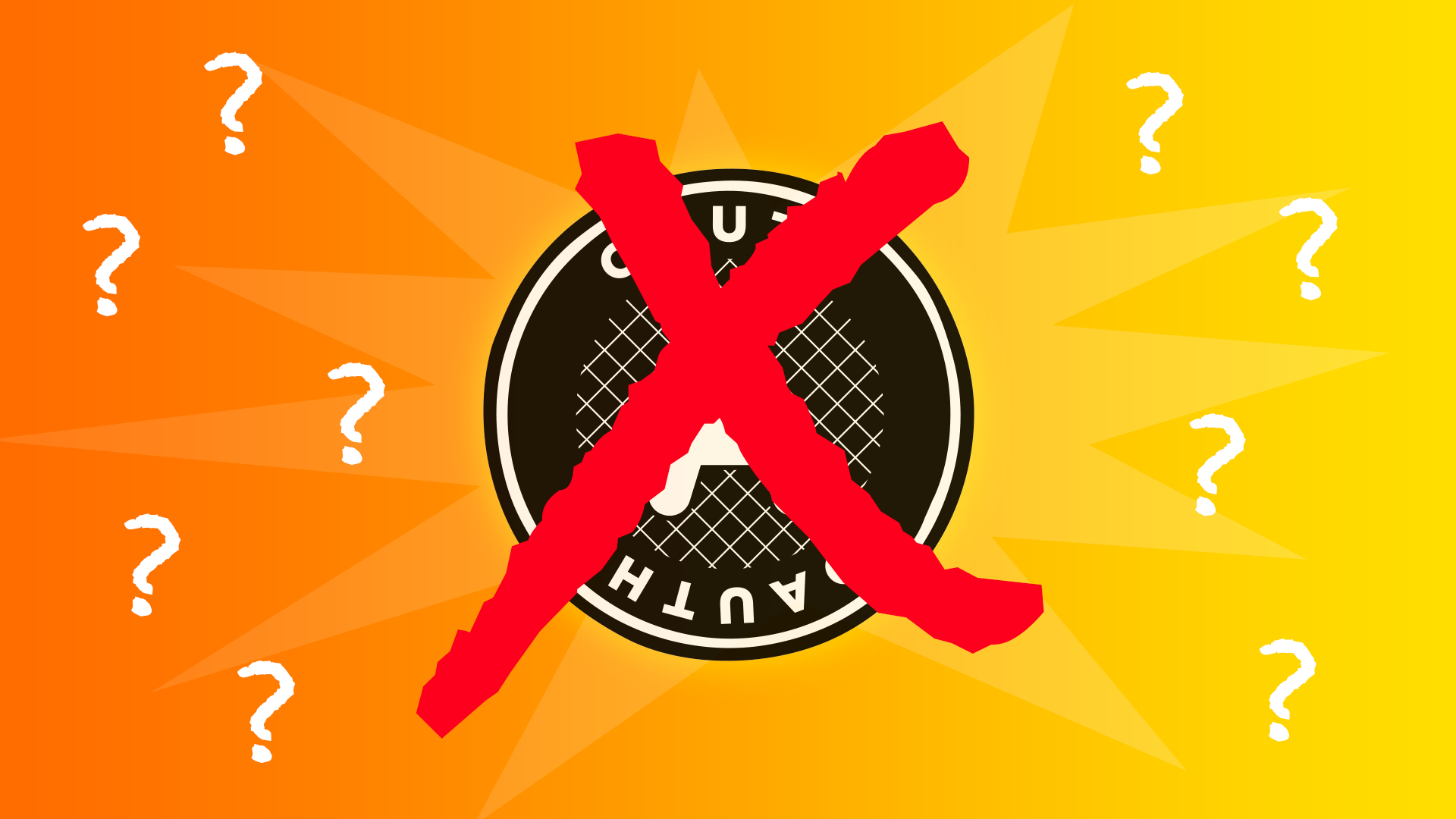
You may have heard some buzz recently about the OAuth 2.0 Implicit flow. The OAuth Working Group has published some new guidance around the Implicit flow and JavaScript-based apps, specifically that the Implicit flow should no longer be used. In this post we’ll look at what’s changing with the Implicit flow and why. Note: In May 2025, the Okta Integrator Free Plan replaced Okta Developer Edition Accounts, and the Okta CLI was deprecated. We preserved...
Angular MVC - A Primer
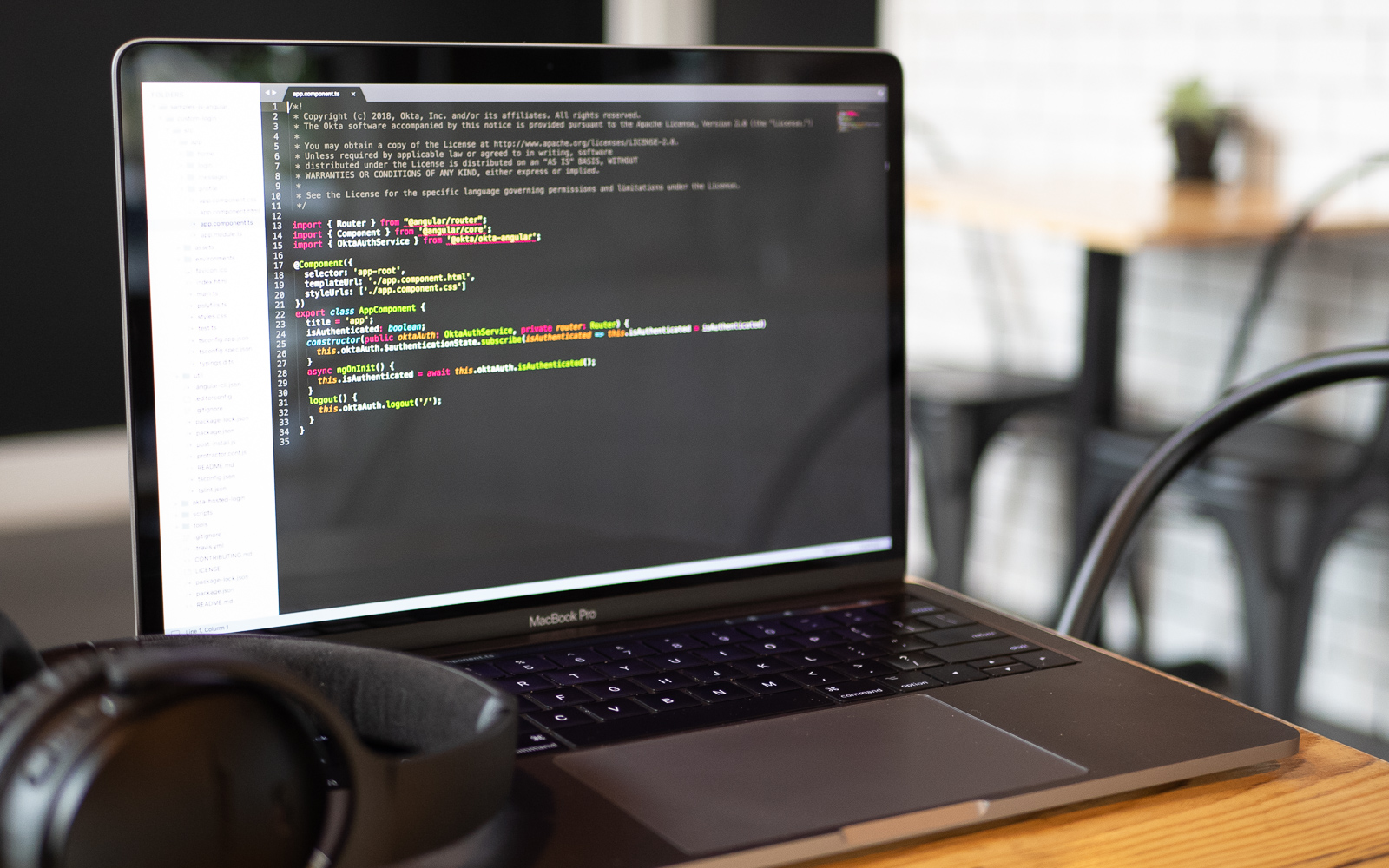
When designing software with a user interface, it is important to structure the code in a way that makes it easy to extend and maintain. Over time, there have been a few approaches in separating out responsibilities of the different components of an application. Although there is plenty of literature on these design patterns around, it can be very confusing for a beginner to understand the features of limitations of the different patterns and the...
Build a Desktop Application with Angular and Electron
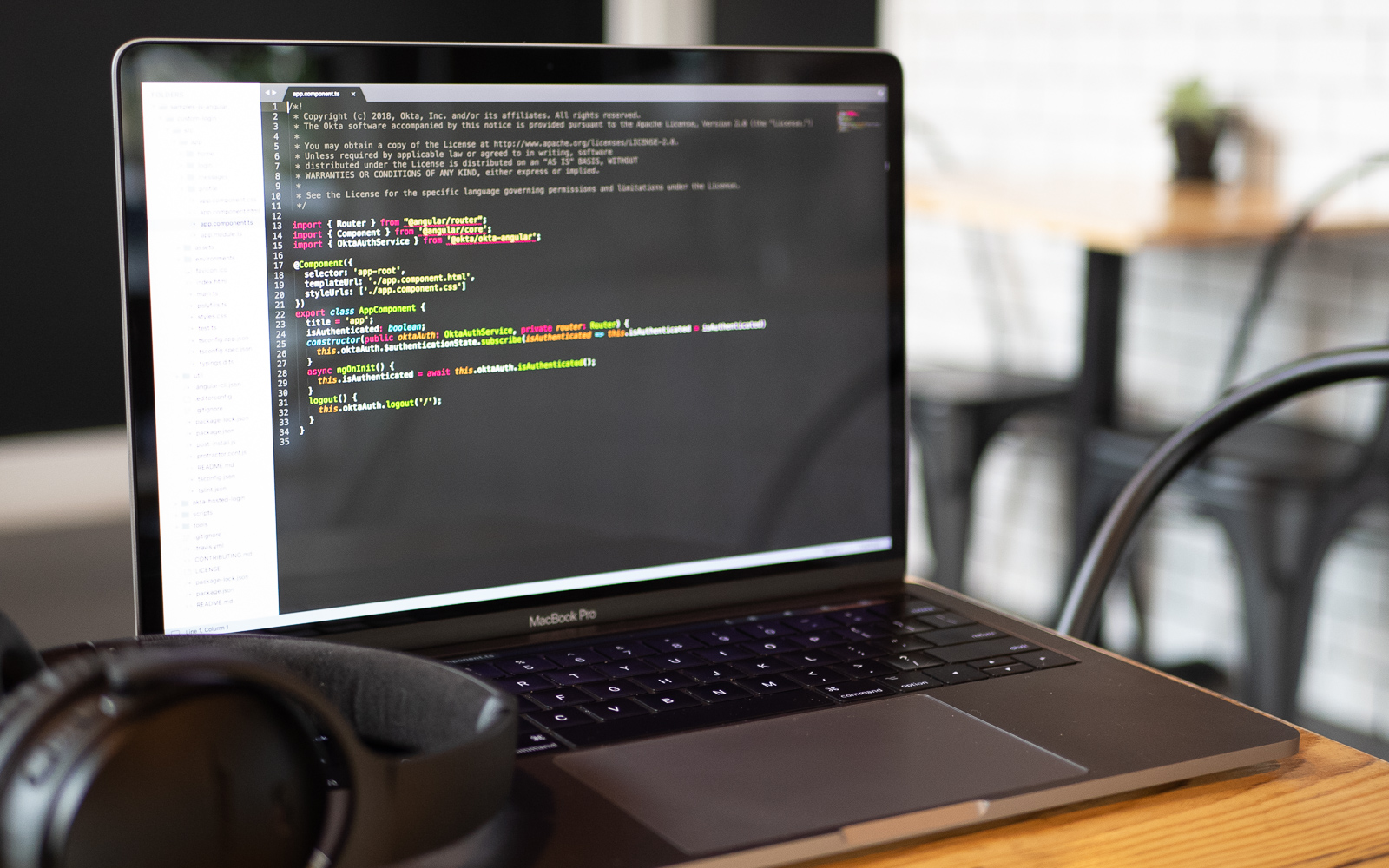
So, you have been learning all about web technologies including JavaScript, HTML, and CSS. The advantage of web technologies is, of course, that the same software can be used on many different platforms. But this advantage comes with a number of problems. Web applications have to be run inside a browser and the interoperability with the operating system is limited. Direct access to features of the operating system is usually the domain for desktop applications....
Build a Secure Node.js App with SQL Server
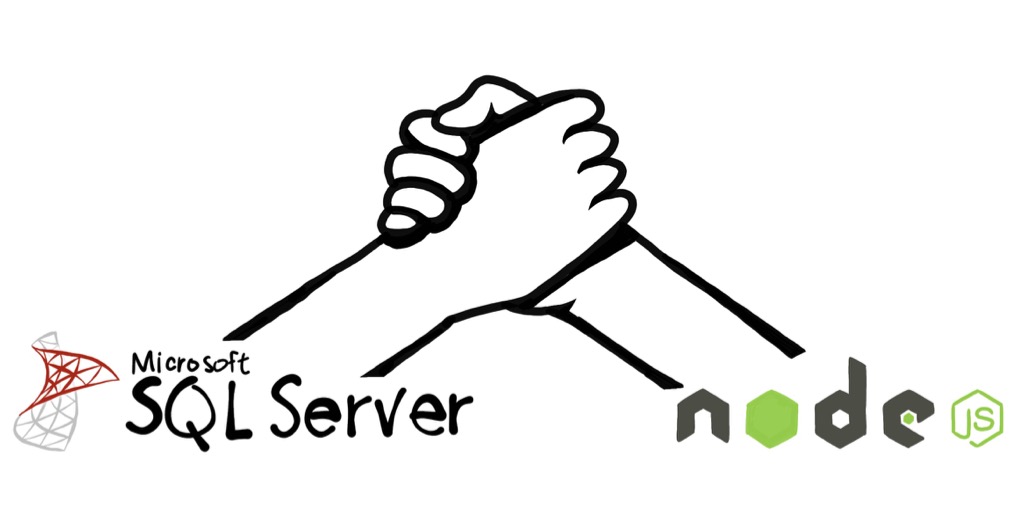
I am a long-time relational database nerd, specifically SQL Server. At times in my career, I’ve focused on database design, deployments, migrations, administration, query optimization, and carefully crafting stored procedures, triggers, and views. I’ve written applications on top of SQL Server using Visual Basic, “Classic” ASP, ASP.NET, and, in recent years, Node.js. Yes, it’s true. You can build Node.js applications with SQL Server! In this tutorial, you will learn the basics of creating a Node.js...
Build a CRUD App with Angular and Firebase
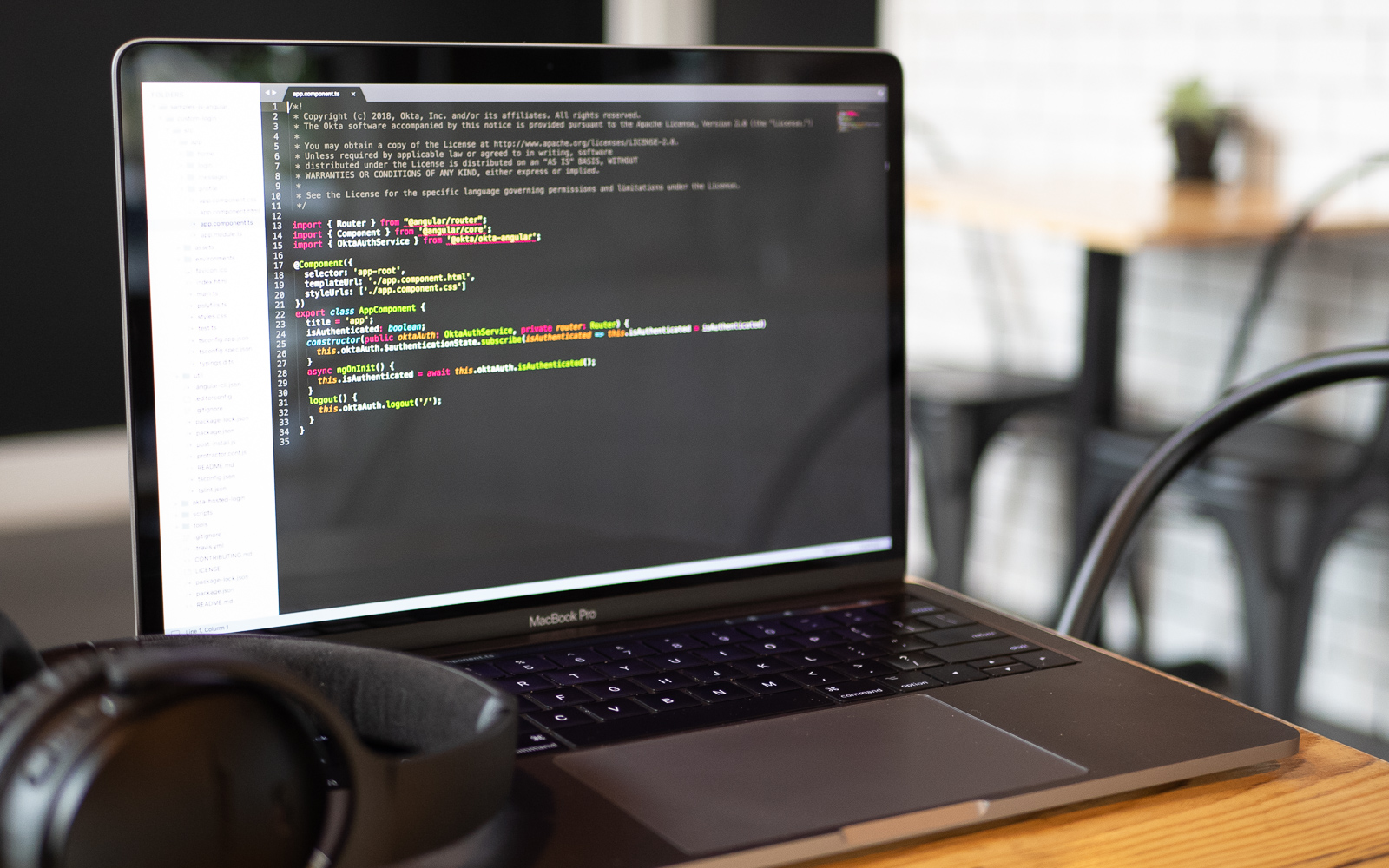
Storage as a Service (SaaS) is becoming ever more popular with many businesses. The advantages are clear. Instead of maintaining your own backend server you can outsource the service to a different provider. This can result in a significant increase in productivity, as well as a reduction in development and maintenance costs. In addition, the worry about server security is offloaded to the storage provider. SaaS is an option whenever the server part of your...
Devnexus 2019: Join the <dev/>olution
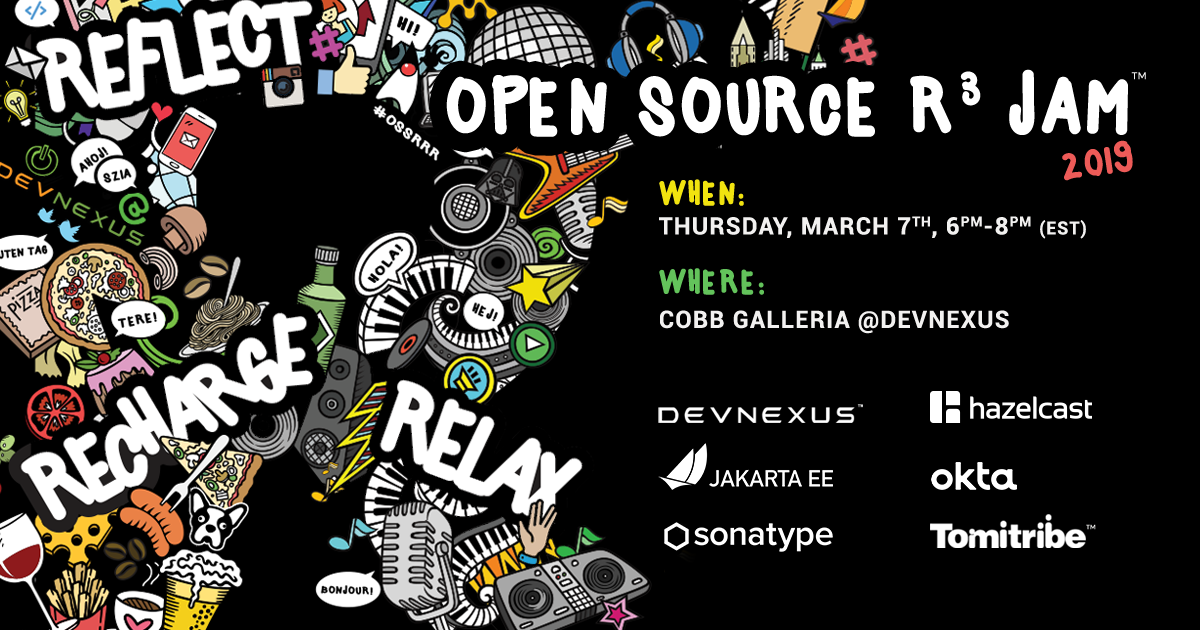
Hello, Developers! Have you ever been to the wonderful conference known as Devnexus? I attended for the first time two years ago and had a blast! It’s well organized, affordable, and has a diverse and fun crowd. This year, Okta is sponsoring Devnexus and we have a number of speakers sharing their wisdom. I thought it’d be fun to write a blog post that highlights my team members and what they’ll be talking about. If...
The Basics of JavaScript Generators
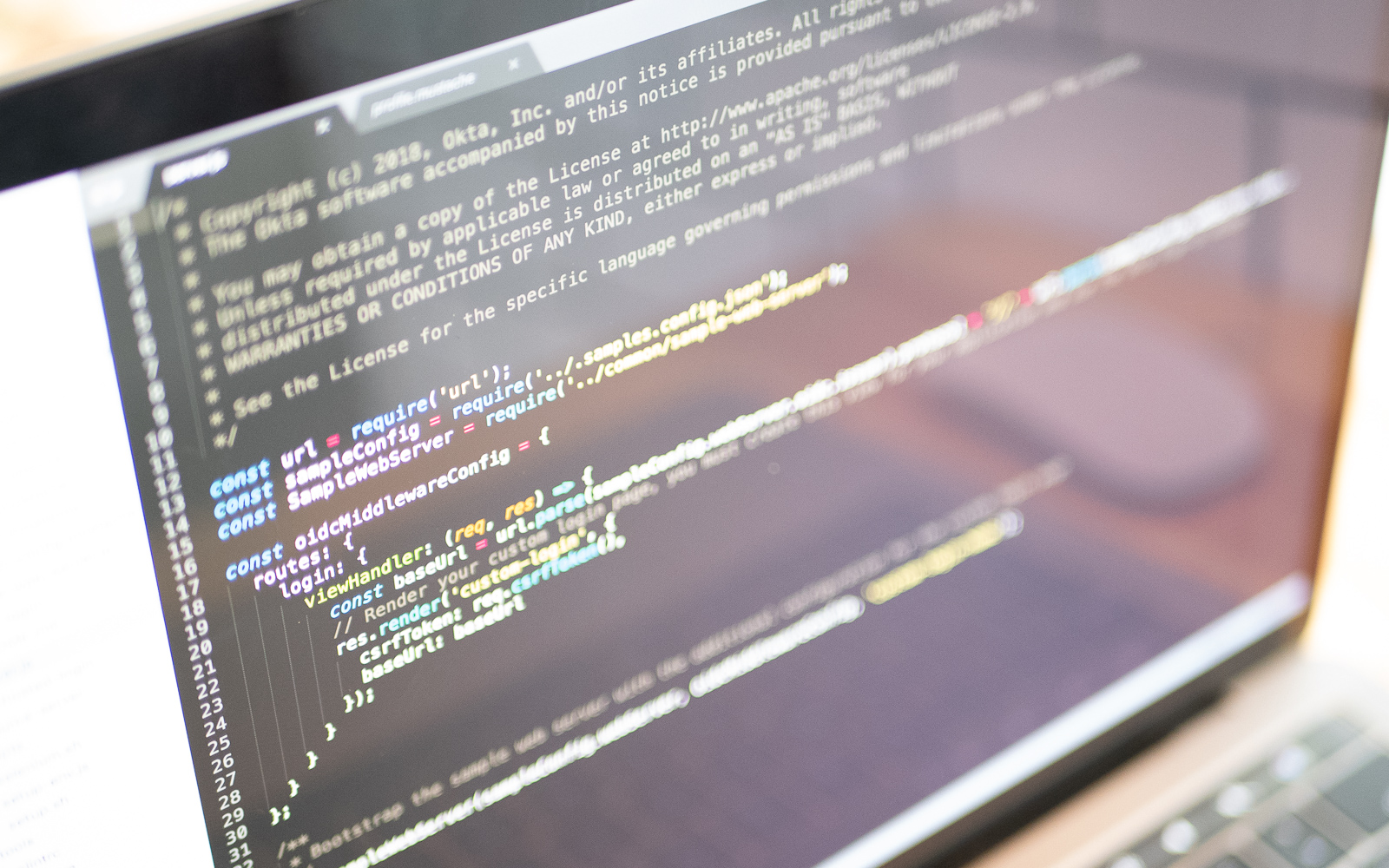
JavaScript does a pretty good job of iterating over collections. But what if you don’t know what the collection is, or how big it will be? What if the thing you want to iterate over doesn’t have an iterator to use? JavaScript generators can help! What Are JavaScript Generators? JavaScript generators are just ways to make iterators. They use the yield keyword to yield execution control back to the calling function and can then resume...
Modern Token Authentication in Node with Express
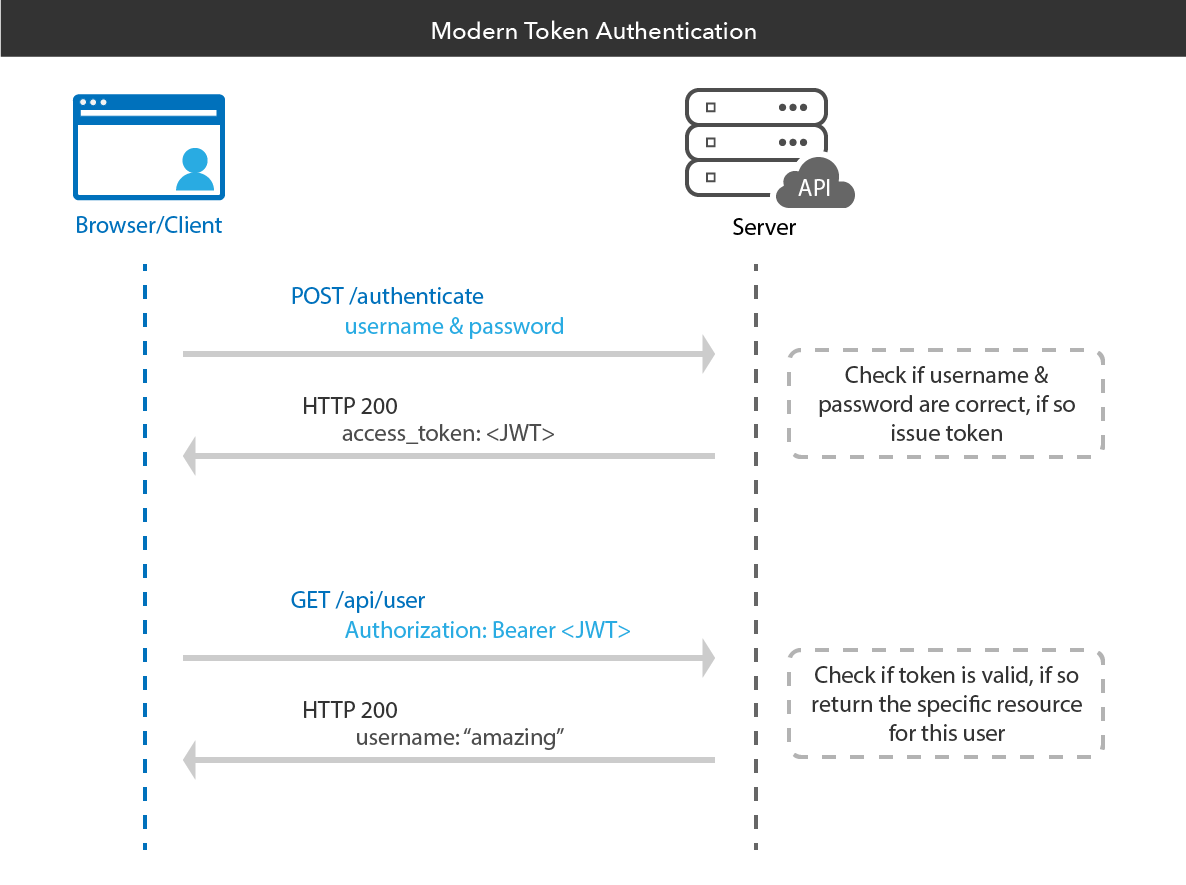
Token authentication is the hottest way to authenticate users to your web applications nowadays. There’s a lot of interest in token authentication because it can be faster than traditional session-based authentication in some scenarios, and also allows you some additional flexibility. In this post, I’m going to teach you all about token authentication: what it is, how it works, why you should use it, and how you can use it in your Node applications. Let’s...
If It Ain't TypeScript It Ain't Sexy
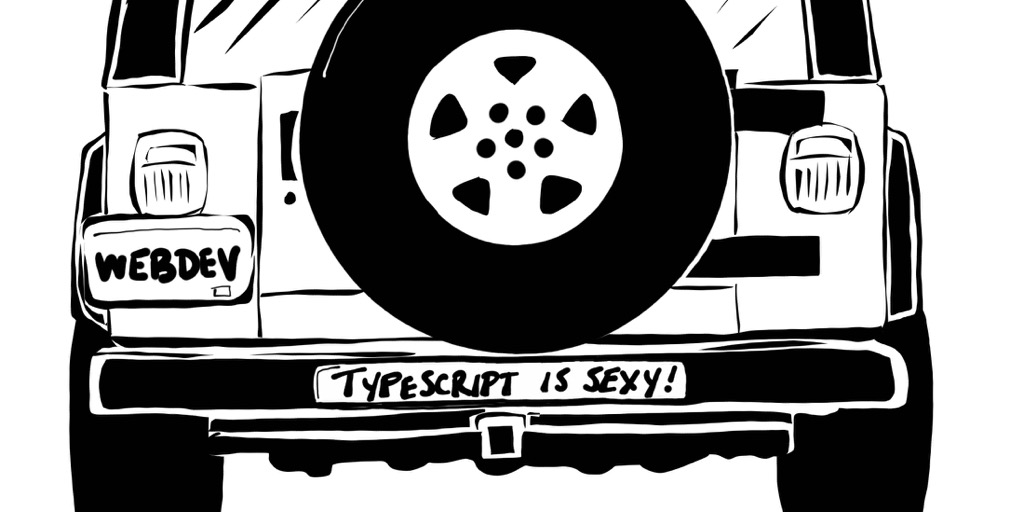
A few years ago I got “Jeep fever.” I began daydreaming about owning a Jeep, driving around with the top down, and going on trips into the mountains. That’s when it happened. Everywhere I went, I saw Jeeps. I passed countless Jeeps on the road. There were Jeeps in every parking lot. Practically everyone had a Jeep but me. Where did all these Jeeps come from?! Logically, I had to assume there was relatively the...
What's New in JavaScript for 2019
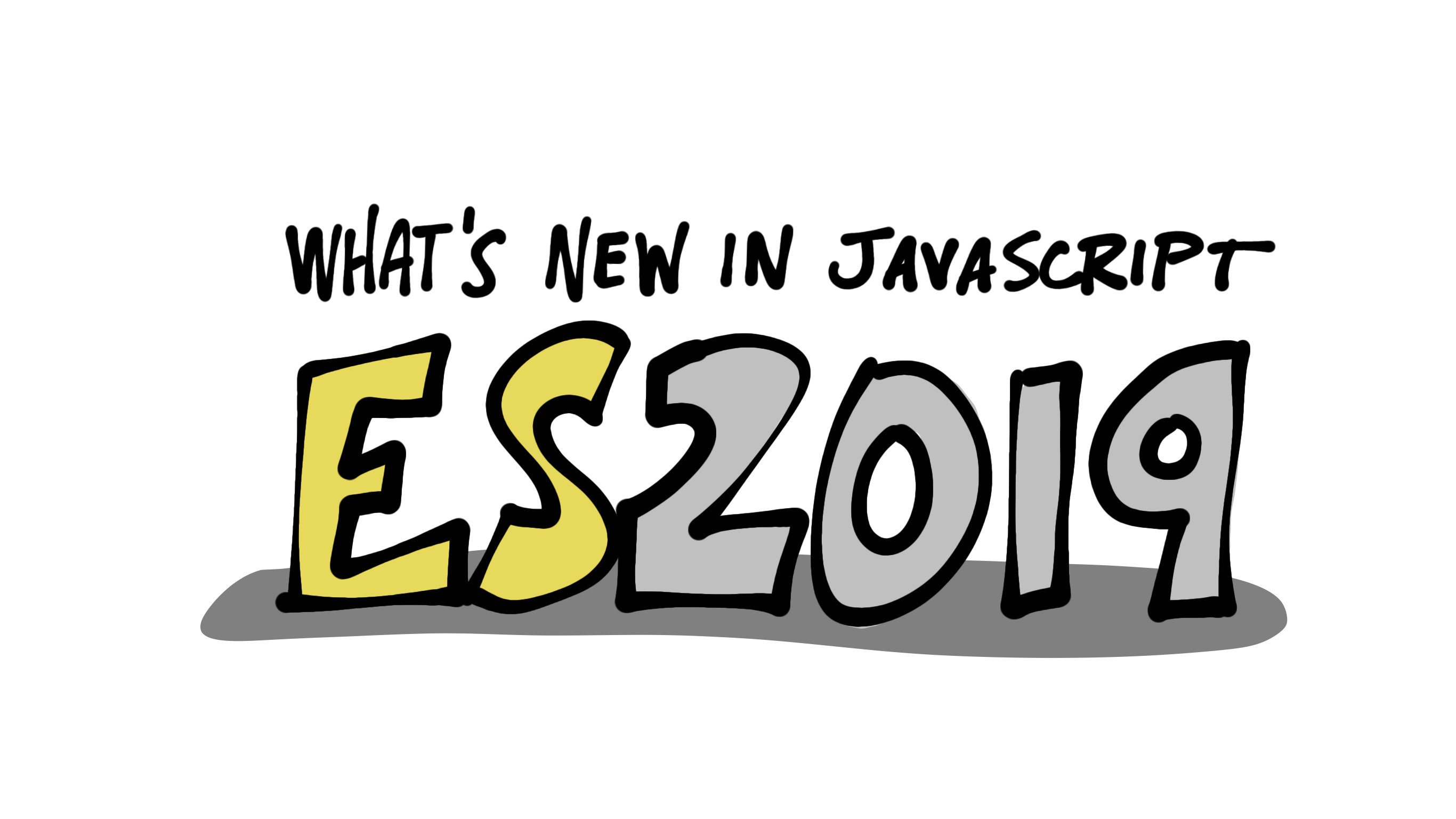
For the last several years, JavaScript has been evolving on a steady cadence with new language features. If you’re curious to see what’s in store for the next version of JavaScript, this post is for you! Before we talk about the latest features, it’s important to understand how new ideas become part of the JavaScript language. The Process for New JavaScript Language Features In a nutshell, the language specification that drives JavaScript is called ECMAScript....
The History (and Future) of Asynchronous JavaScript
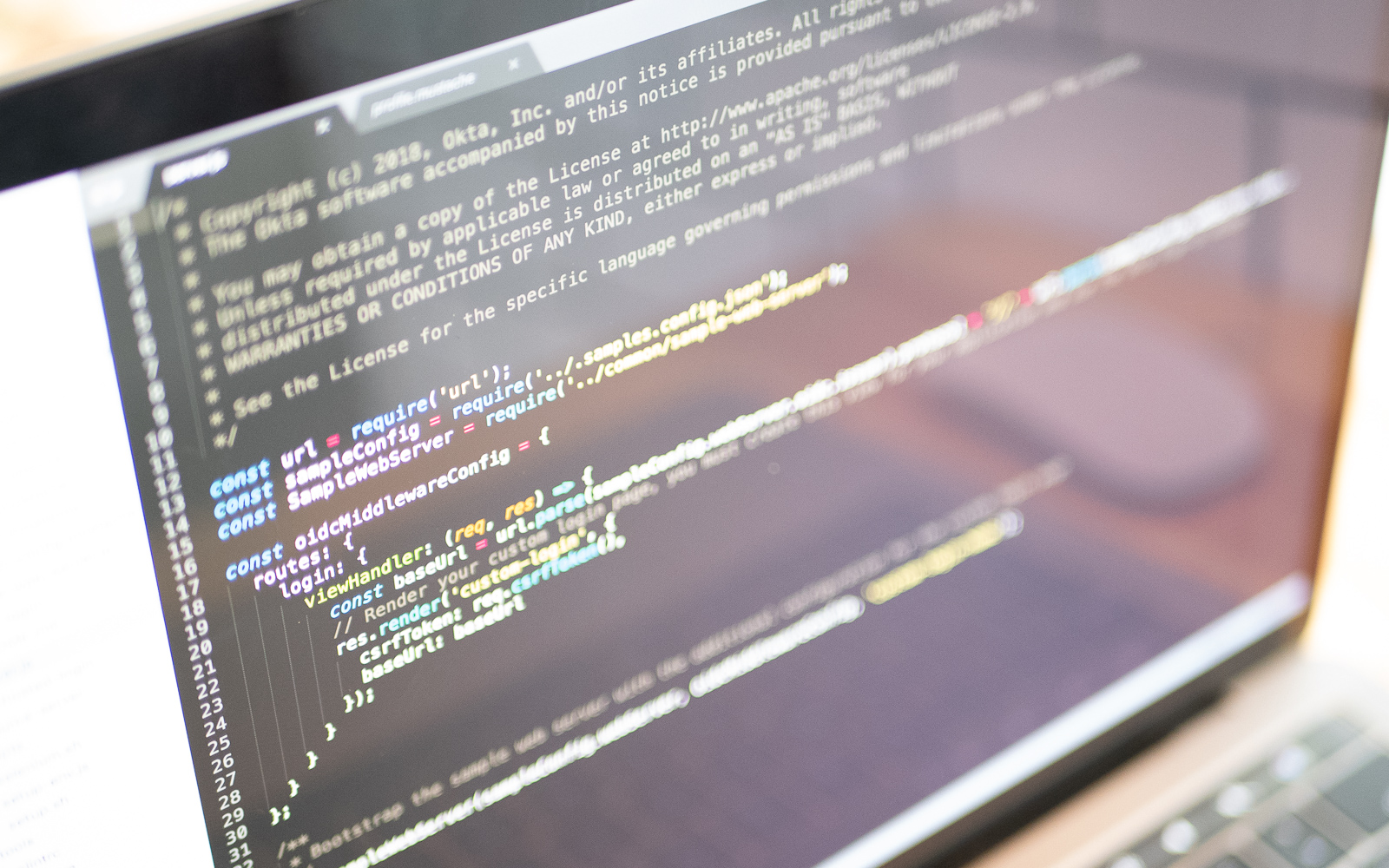
Everyone knows that JavaScript is eating the world. The problem is, there are lots of developers responsible for building software with JavaScript without a particularly deep knowledge of the language. It is easy to learn the basics and be productive, but newer programmers have a tendency to misuse some of JavaScript. Asynchronous JavaScript is particularly misunderstood. In this post, I will show you the most common ways to make asynchronous JavaScript calls and when to...
Learn JavaScript in 2019
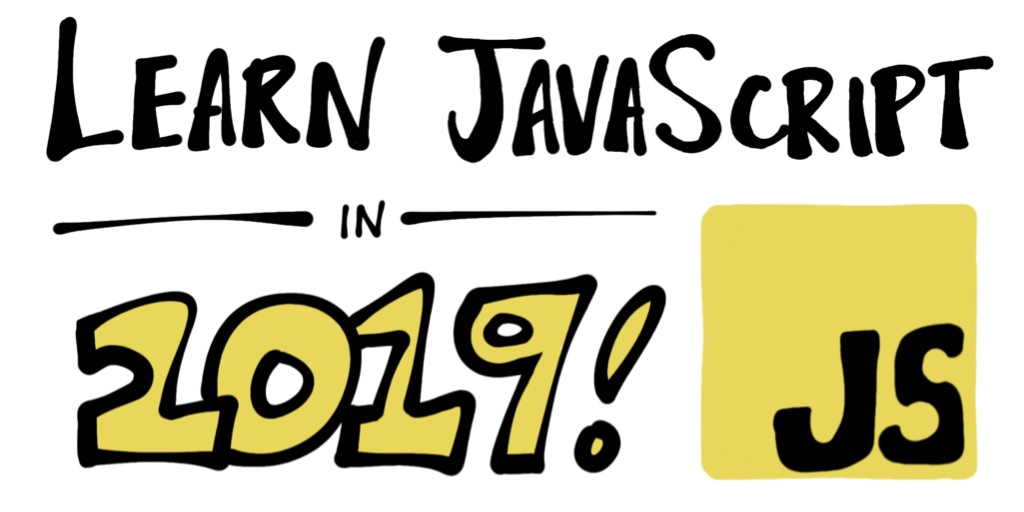
There’s never been a better time to be a programmer. Technology isn’t slowing down. Neither will the demand for innovative solutions to solve new challenges or take advantage of new opportunities. The key differentiation in the marketplace is often the one with the better technology. And companies are willing to pay big bucks for it. Not only are there new challenges and opportunities, but there are also better systems today to support programmers. Languages, code...
Build a Mobile App with React Native and Spring Boot
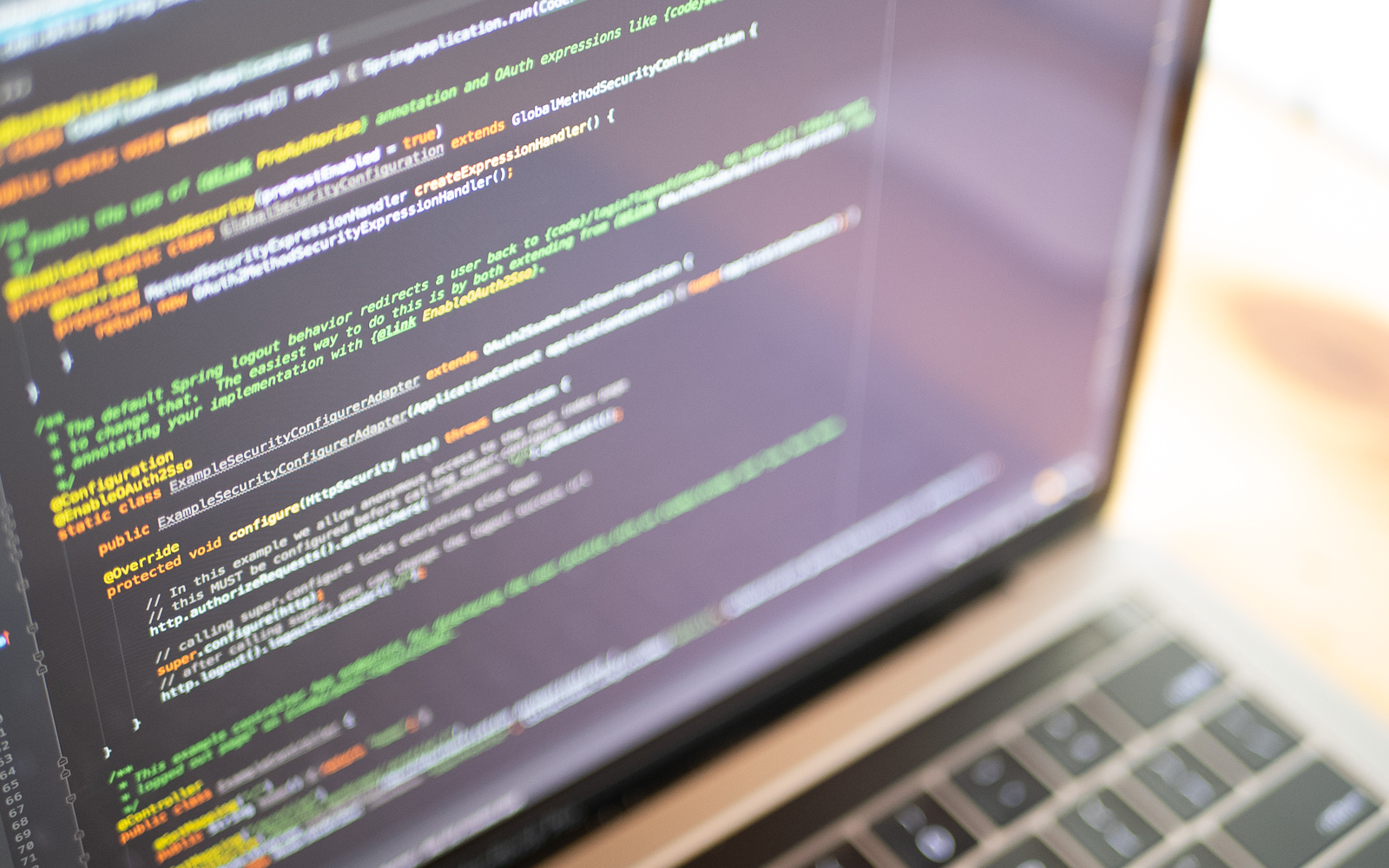
React Native is a framework for building mobile applications with React. React allows you to use a declarative style of programming to describe how your UI should look. It uses embedded HTML (called JSX) to render buttons, lists, scrollable views, and many other components. I’m a seasoned Java and JavaScript developer that loves Spring and TypeScript. Some might call me a Java hipster because I like JavaScript. In this post, I’m going to show you...
Build a Desktop App with Electron and Authentication
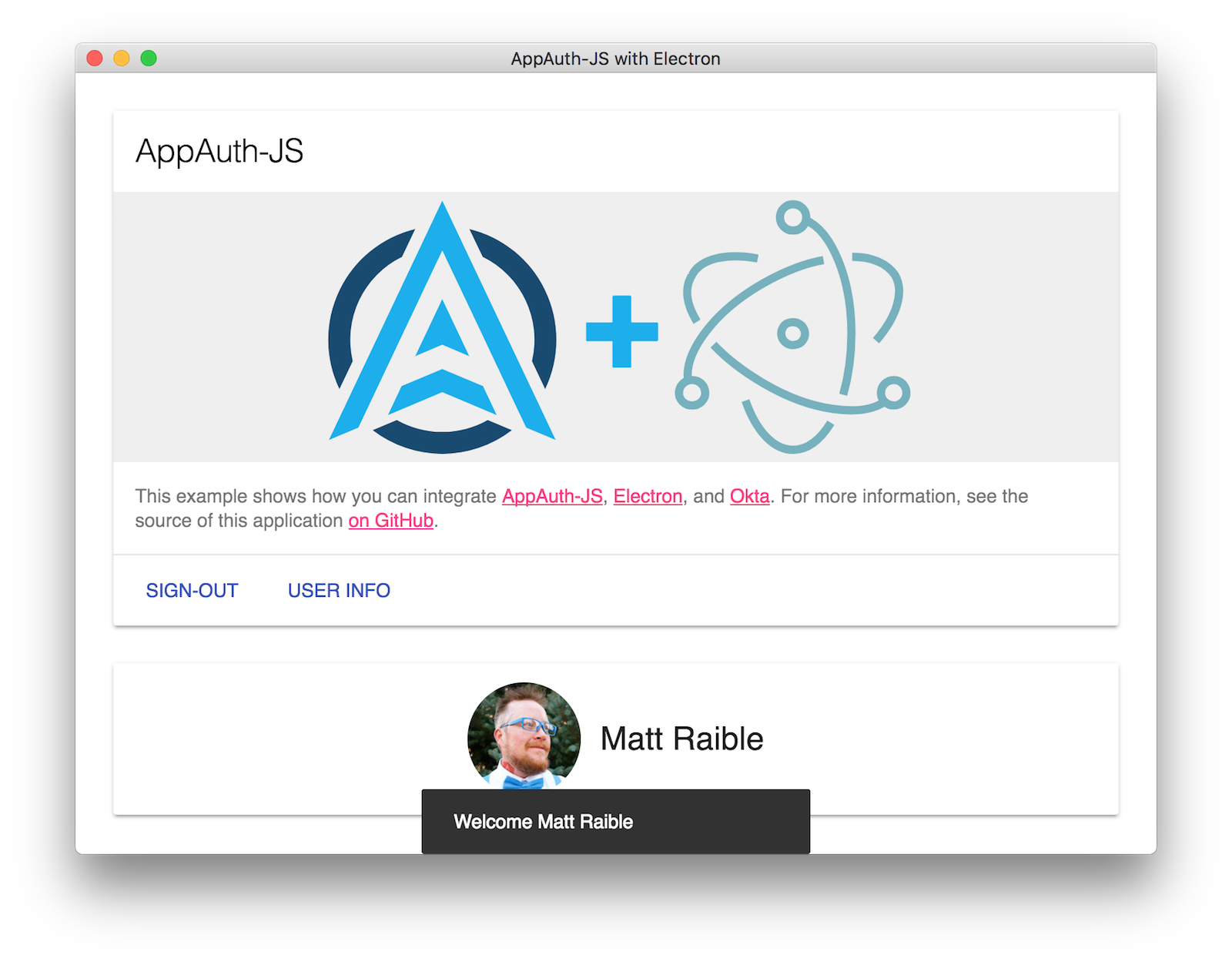
Electron is a framework for building cross-platform desktop applications with web technologies like JavaScript, HTML, and CSS. It was created for GitHub’s Atom editor and has achieved widespread adoption since. Electron powers several apps that I use on a daily basis: Slack, Kitematic, and Visual Studio Code to name a few. Electron 2.0 was released in early May 2018, along with changes to the project to adhere to strict semantic versioning. This is good news...
Hey, Okta! Where's the Bacon?
I am so excited to announce I have joined Okta’s developer relations team as a Senior Developer Advocate! My focus is on all the JavaScripts: Node.js, Vue, React, and a bazillion other things! My Origin Story My first taste of programming was BASIC on the TRS-80. In the 90’s, I wrote a lot of DOS and Windows applications using Pascal and Visual Basic. Around 1998, I made the jump to building Web applications using “classic”...
Build a Health Tracking App with React, GraphQL, and User Authentication
I think you’ll like the story I’m about to tell you. I’m going to show you how to build a GraphQL API with Vesper framework, TypeORM, and MySQL. These are Node frameworks, and I’ll use TypeScript for the language. For the client, I’ll use React, reactstrap, and Apollo Client to talk to the API. Once you have this environment working, and you add secure user authentication, I believe you’ll love the experience! Why focus on...
How to Prevent Your Users from Using Breached Passwords
Not too long ago, the National Institute of Standards and Technology (NIST) officially recommended that user-provided passwords be checked against existing data breaches. Today I’m going to show you how you can easily add this functionality to any website you run using PassProtect, an open-source developer library we created specifically for this purpose. Why Check User Passwords? The new NIST recommendations mean that every time a user gives you a password, it’s your responsibility as...
Add Authentication to Any Web Page in 10 Minutes
× Heads up... this blog post is old! For updated instructions on adding authentication using the Okta Sign-in Widget, see Embedded Okta Sign-In Widget fundamentals. Adding authentication to web pages can be pretty annoying. While I’d like to say that over the course of my programming tenure I’ve learned to easily add authentication to any app I create, my attempts tend to devolve into me bickering with myself endlessly over a User schema and the...
Secure a Node API with OAuth 2.0 Client Credentials
Securing server-to-server API services can be tricky. OAuth 2.0 is an excellent way to offload user authentication to another service, but what if there is no user to authenticate? In this article, I’ll show you how you can use OAuth 2.0 outside the context of a user, in what is also known as the Client Credentials Flow. Instead of storing and managing API keys for your clients (other servers), you can use a third-party service...
Add Authentication to Your Vanilla JavaScript App in 20 Minutes
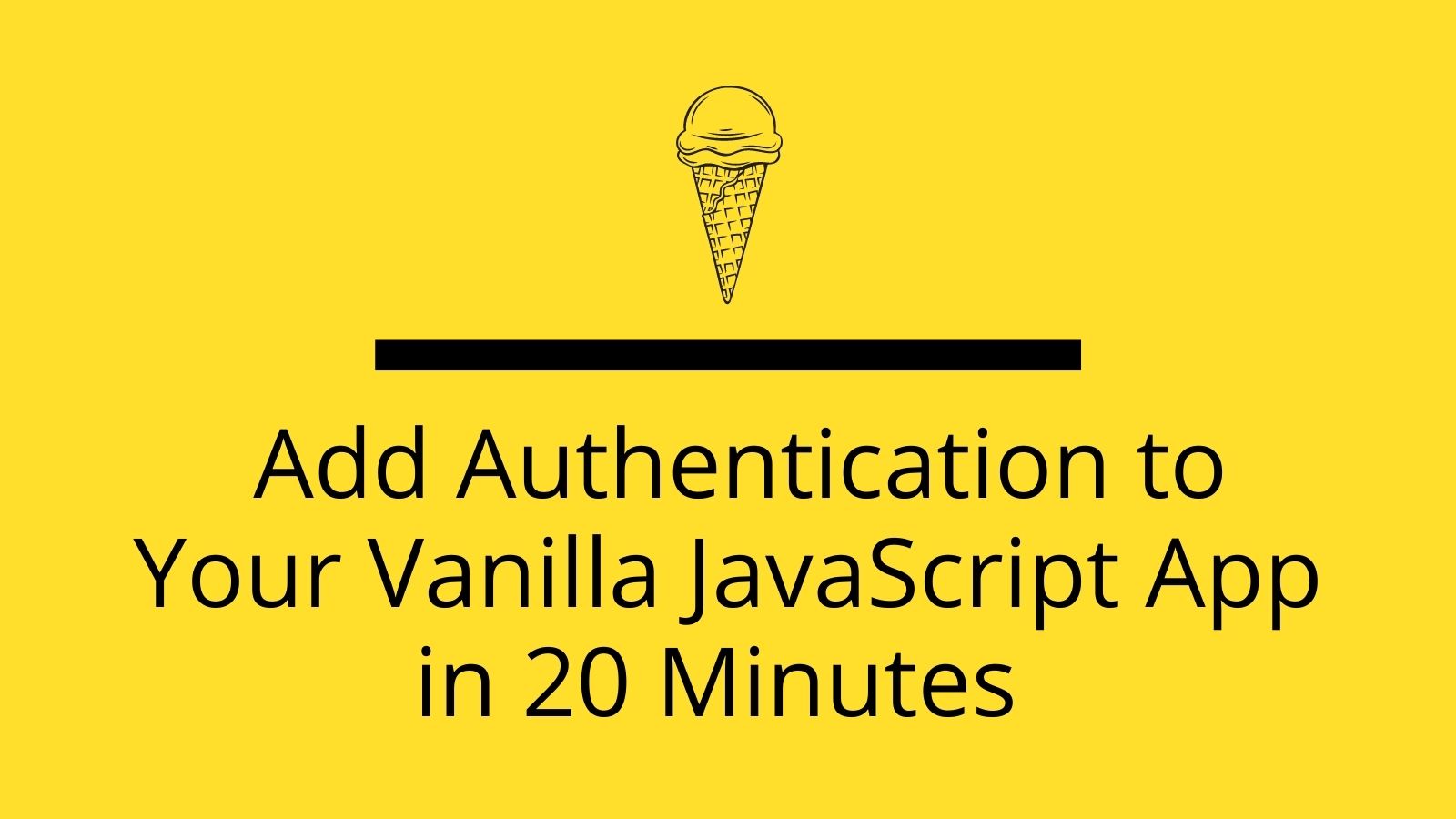
“Sometimes nothing is good enough” is a phrase that software engineers don’t speak or hear often. In the fast-changing world of web development, there is no shortage of bleeding-edge JavaScript frameworks promising to make your life easier or inch out its predecessors. You may ask yourself if it is even possible to build a modern web application without one of these frameworks, let alone add secure authentication. Well, it is! Vanilla JavaScript is frequently used...
Announcing PassProtect - Proactive Web Security
If you’re reading this article you probably care about web security. You probably use a password manager to manage your passwords, you’ve probably got multi-factor authentication setup for all of your services, and you’re probably already subscribed to Have I Been Pwned? so you’re alerted when one of your logins have been involved in a data breach. But you’re not most people. Most web users are completely disconnected from the incredible advancements that have been...
Build a Video Chat Service with JavaScript, WebRTC, and Okta
As recently as seven short years ago, building video applications on the web was a massive pain. Remember the days of using Flash and proprietary codecs (which often required licensing)? Yuck. In the last few years, video chat technology has dramatically improved and Flash is no longer required. Today, the video chat landscape is much simpler thanks to WebRTC: an open source project built and maintained by Google, Mozilla, Opera, and others. WebRTC allows you...
Simple Node Authentication
Authenticating users for Node.js/Express.js web apps can be difficult. You have to set up a database, define a user schema (or use something more flexible like NoSQL), write code to handle password hashing, etc. It’s annoying. Using Okta’s API service, however, you can easily register and log in users to your Node website using our OpenID Connect integration. Note: In May 2025, the Okta Integrator Free Plan replaced Okta Developer Edition Accounts, and the Okta...
Hello, Okta. It's me, Dave
Spread Serverless Holiday Cheer with Lambda and API Gateway
It’s that time of year again: Christmas music on the radio, festive decorations in the workplace, and a chill in the air. Except in the Southern Hemisphere, where it’s warm and balmy. And on the International Space Station, where the temperature is strictly regulated. But I digress. It’s a season for reconnecting with loved ones, exchanging gifts, and eating delicious food. And, if you’re like me, a little downtime to work on fun projects. Looking...
Use OpenID Connect to Build a Simple Node.js Website
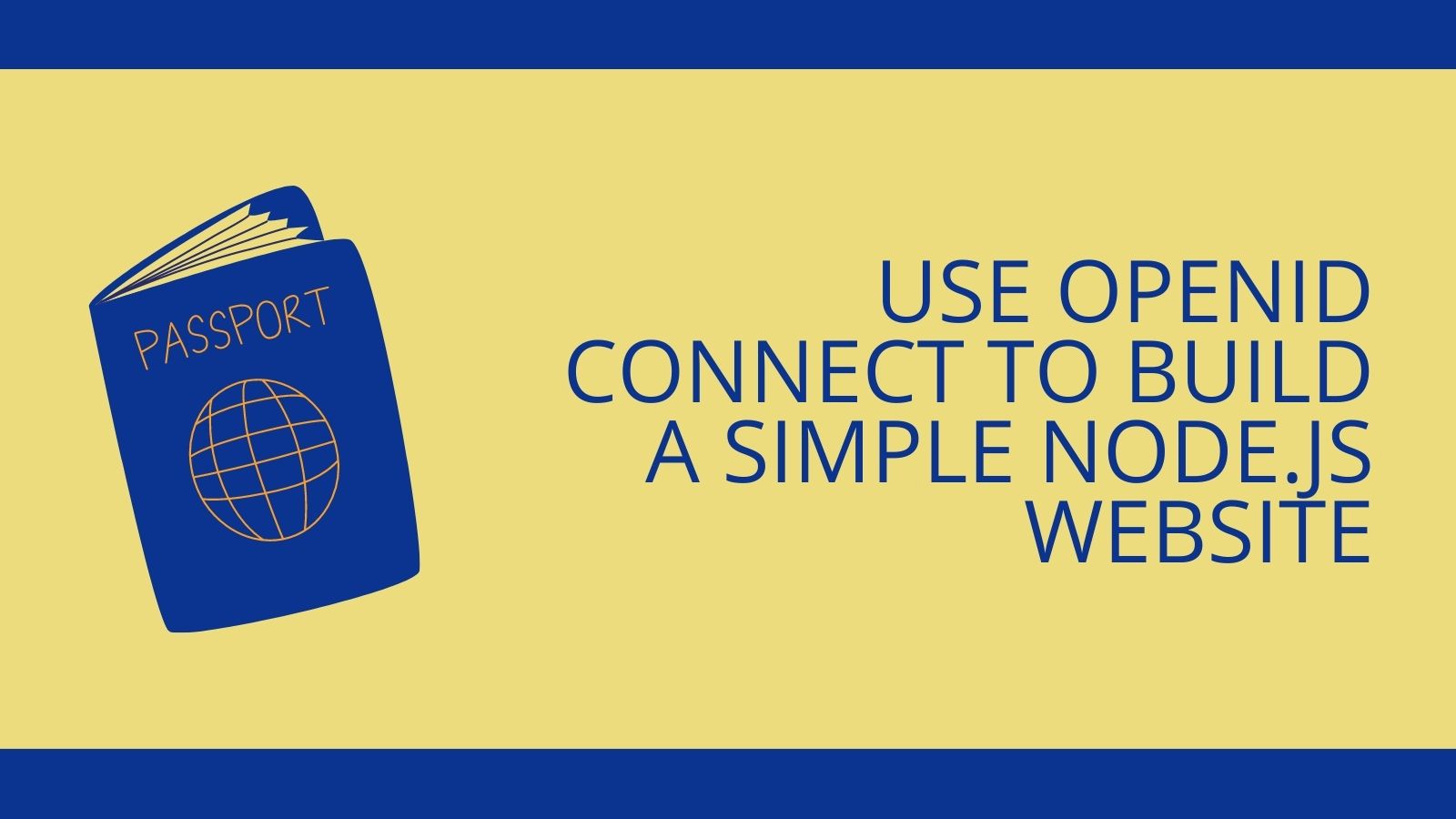
If you’ve ever spent time trying to figure out the best way to handle user authentication for your Node app and been confused: you’re not alone. Over the last few years, authentication practices have changed quite a bit. Today I’m going to show you how to use OpenID Connect to build an extremely simple Node.js website (using Express.js) that allows you to manage your users, log them in, and log them out. Websites used to...
Build a Preact App with Authentication
React is a fast, and lightweight library, which has led to fast adoption across the SPA (single-page app) ecosystem. Preact is an even lighter-and-faster alternative to React, weighing in at a measly 3kb! For less complex applications, it can be a great choice. In this tutorial, you’ll build a basic Preact application with a couple of pages and user authentication using the Okta Sign-In Widget. Bootstrap Your App With PreactCLI To get your project started,...
The Top 10 JavaOne 2017 Sessions for the Java Hipster
A “hipster” is defined as a person who is exceptionally aware of or interested in the latest trends and tastes. JHipster is an open source project whose name stands for “Java Hipster.” If you’re using JHipster, chances are you’re aware of and using the latest trends and techniques in Java development. Trendy things in server-side Java development include microservices, embedded app servers, deployment with containers, auto-configuration, and monitoring. JHipster supports all of these trends, embracing...
Build a Cryptocurrency Comparison Site with Vue.js
Vue.js is a simple JavaScript framework that lets you build dynamic front-end web applications. Lots of people compare it to React and Angular. As a back-end developer, and someone not incredibly experienced with frontend web applications, I’ve found Vue.js a lot simpler to learn, use, and be successful with vs. React and Angular. In this article, I’ll walk you through the basics of Vue.js, and in the process we’ll build a very simple single page...
Seven Awesome New Features In Visual Studio 2017
Microsoft developers have been using Visual Studio for their IDE since before .NET was even a thing. Visual Studio is twenty years old this year, and on March 7th, 2017 Microsoft released the latest version of its flagship developer product, Visual Studio. With this release are a bunch of new features, improvements, and exciting changes for the beloved Microsoft developer environment. Here are seven features in the new IDE that will excite developers using the...