Articles tagged dotnet
How to Use Okta's PowerShell Module to Manage Your Okta Org
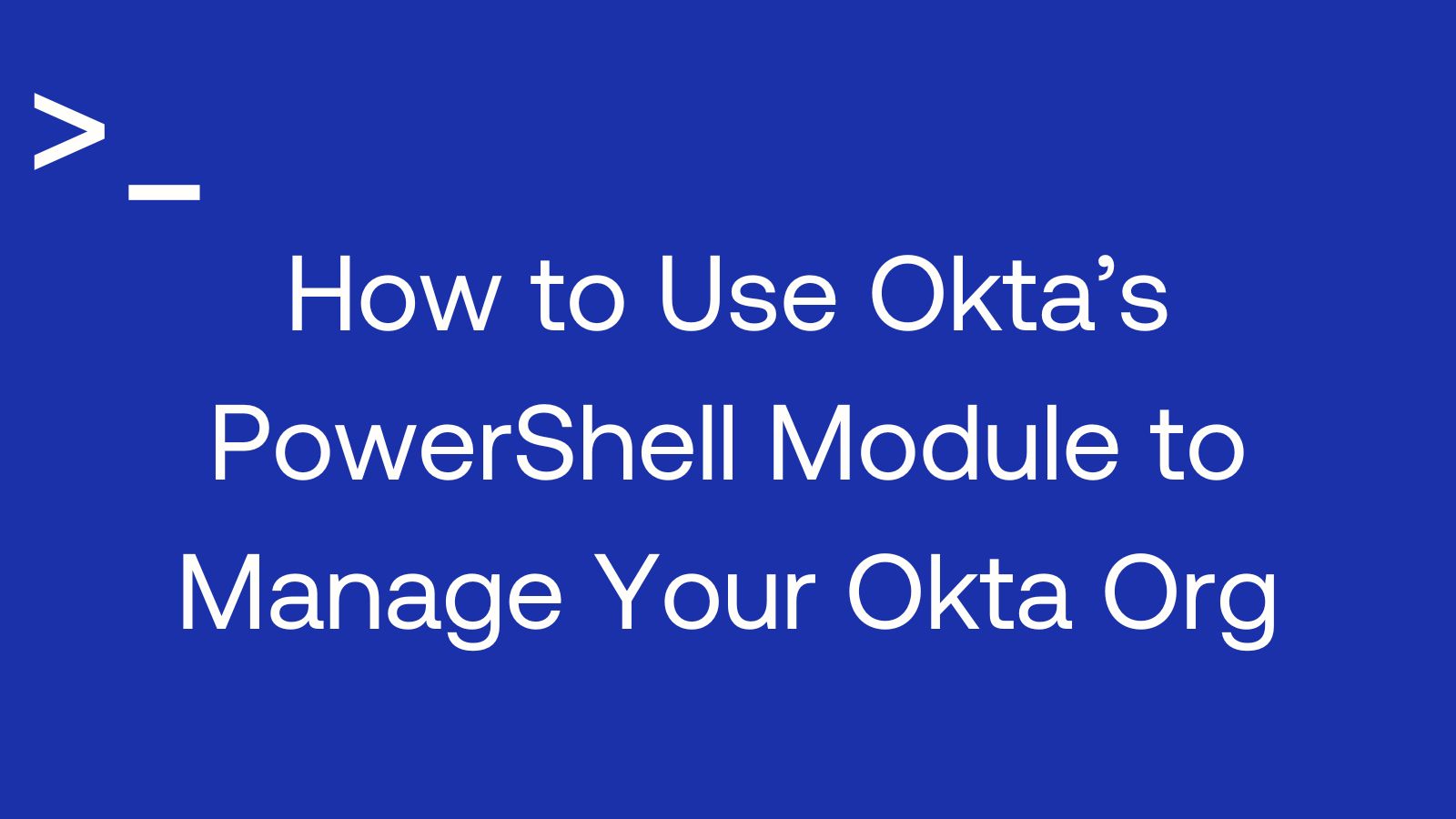
PowerShell is a powerful command-line interface for automating tasks, scripting, and managing systems. Okta offers an official PowerShell module, an extremely powerful tool for administering your Okta org. In this blog post, we’ll explore how to utilize this. You’ll need a PowerShell terminal for your OS and the Okta PowerShell module. Install it through the PS Gallery, Chocolatey Package Manager, or the GitHub repository. Follow the instructions in the GitHub repository’s ReadMe to install the...
How to Manage User Lifecycle with .NET and SCIM
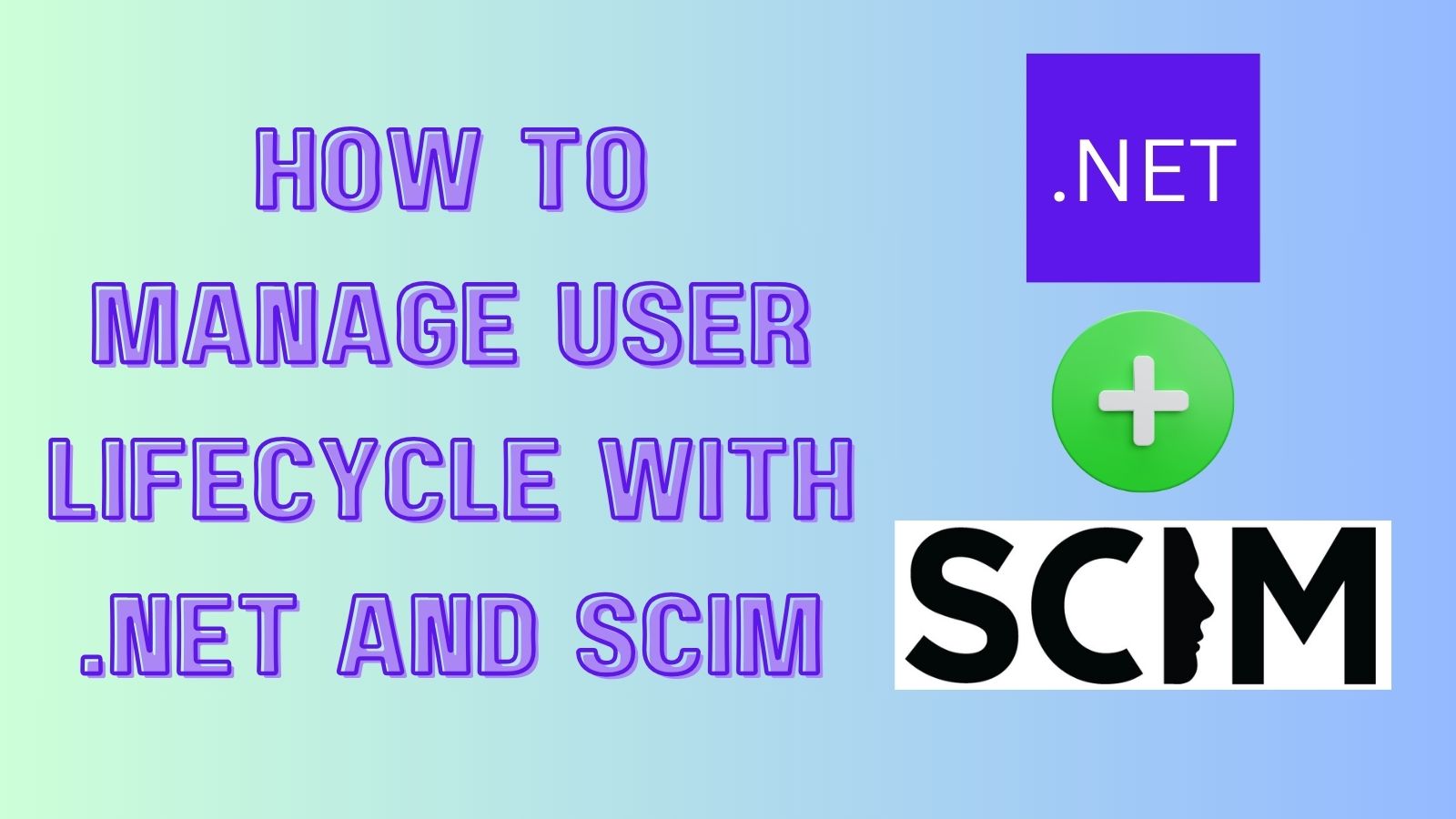
Several challenges exist in provisioning users for a growing company across systems and applications. It can be time-consuming, error-prone, challenging to sync users across domains, and potentially a security risk to keep track of existing profiles. Fortunately, a protocol called SCIM (System for Cross-domain Identity Management) standardizes user information across systems, making it possible to sync users. Also, combined with a SCIM-compliant Identity Provider (IdP), it securely automates common user lifecycle operations. In this tutorial,...
How to Add Authentication to .NET MAUI Apps
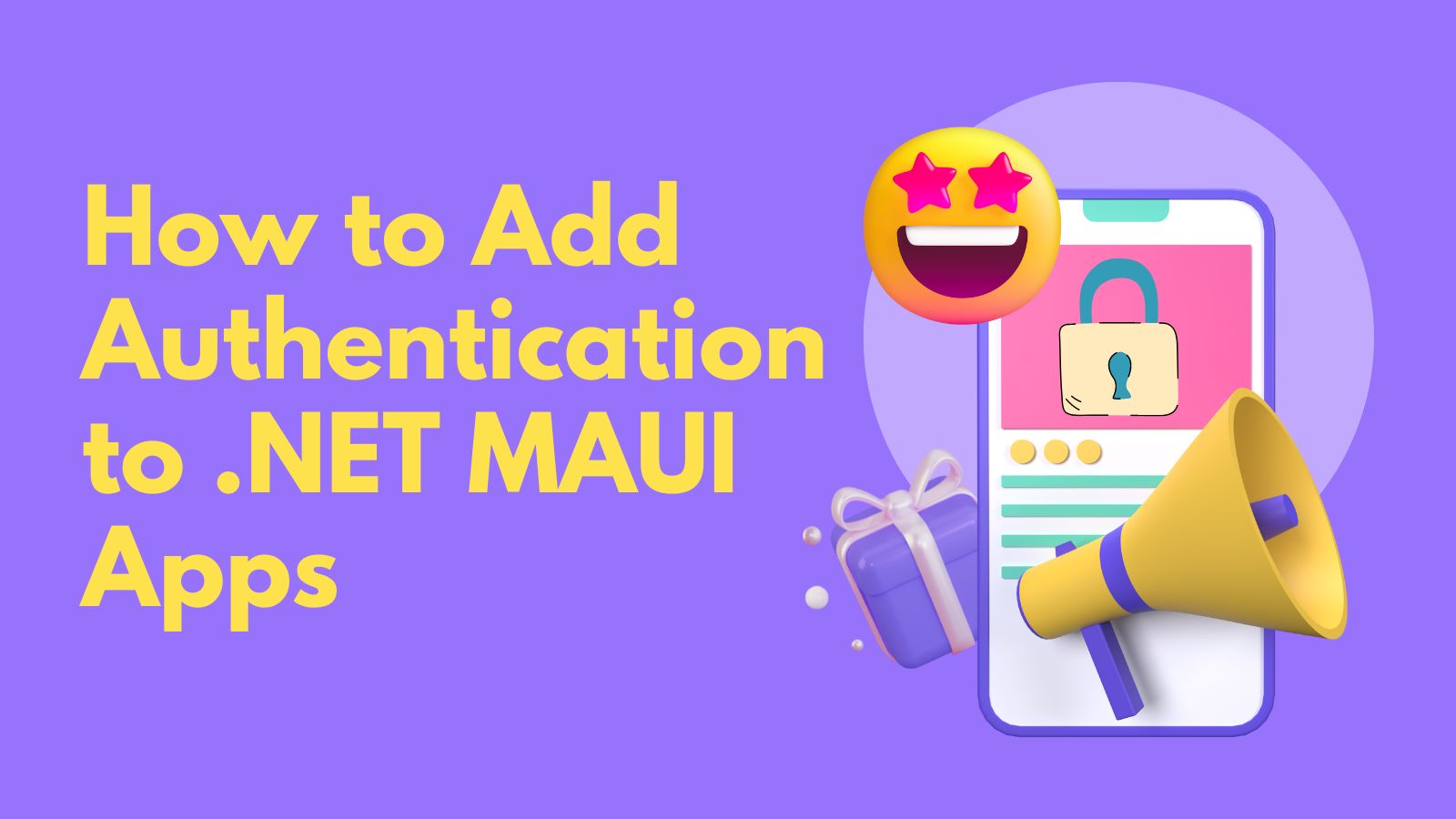
Have you ever been inspired by a colleague’s work and decided to explore a new tool or technology? That’s what happened to me when my colleague, Andrea Chiarelli, wrote a blog post “Add Authentication to .NET MAUI Apps with Auth0.” As someone who is always looking for ways to improve my skills and stay up-to-date with the latest trends in technology, I was intrigued by his post and decided to try MAUI, but this time...
How to Secure User Data in Azure Cosmos DB
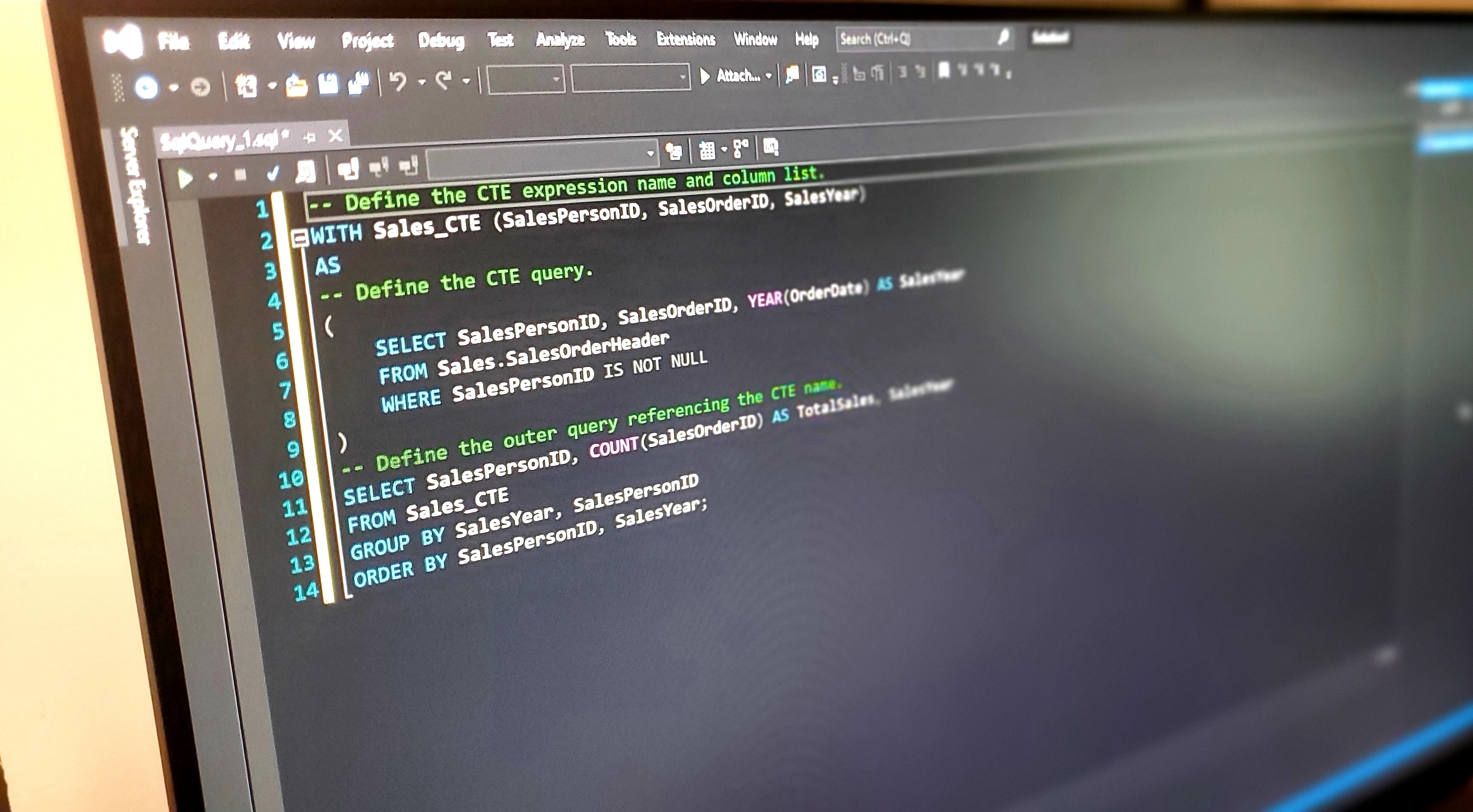
Cosmos DB is a cloud database product from Microsoft that offers scalable and high-performance services. The core product runs on a proprietary NoSQL database that should look familiar to experienced MongoDB developers. Microsoft offers several APIs in addition to the core Cosmos DB API. These include APIs for: SQL MongoDB Gremlin Cassandra The shift to serverless database operations is one of the most obvious advantages of migrating. Cosmos DB can automatically scale your throughput based...
Secure Your .NET 6 Web API
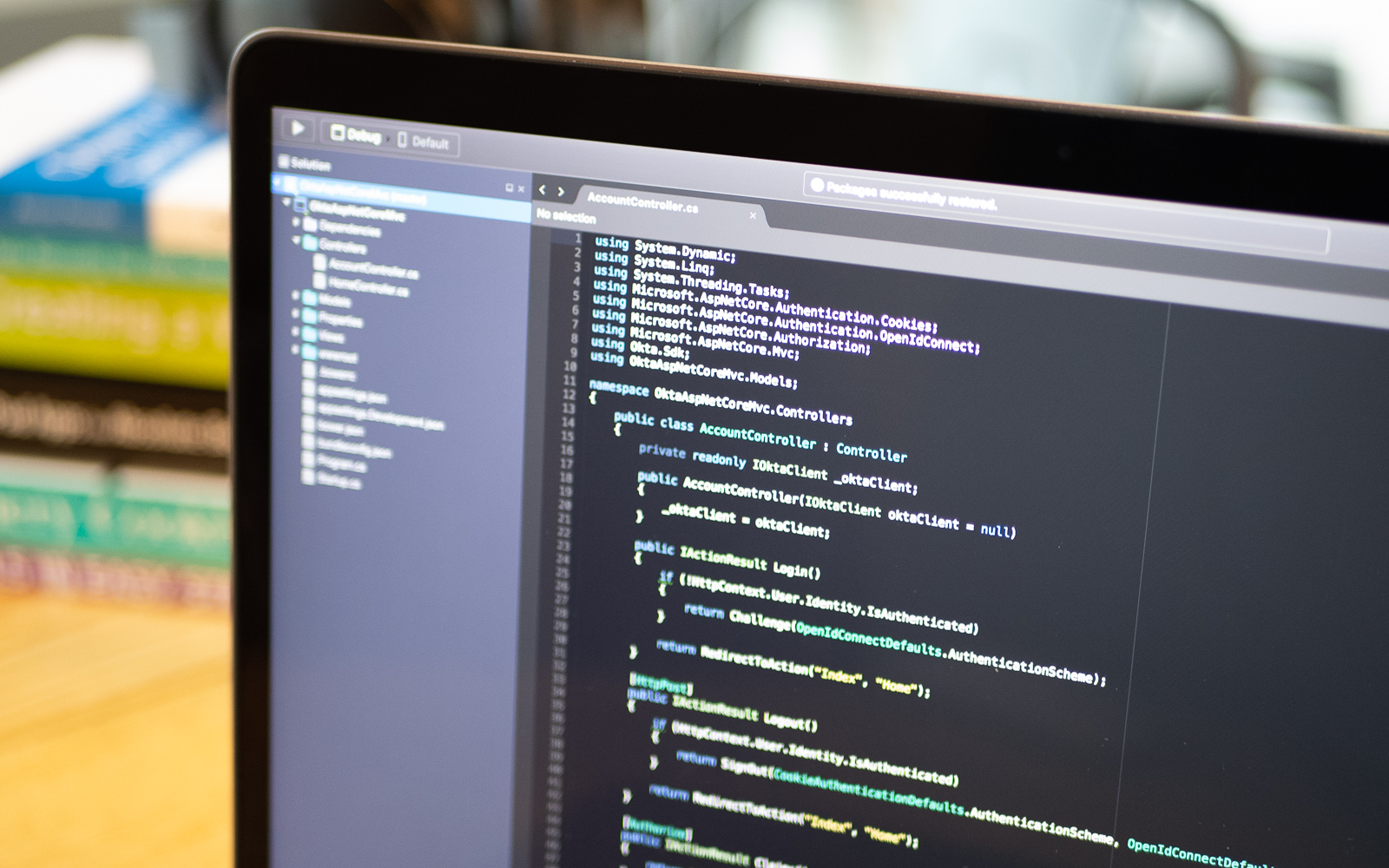
.NET 6 is here and many of us are making preparations to update .NET 5 codebases to .NET 6. As part of this review, today you will learn how to implement the client credentials flow in ASP.NET Core Web API. What is the client credentials flow The client credentials flow is a server-to-server flow that allows applications to request resources on behalf of itself rather than a user. The client credentials flow requires the client...
Managing Multiple .NET Microservices with API Federation
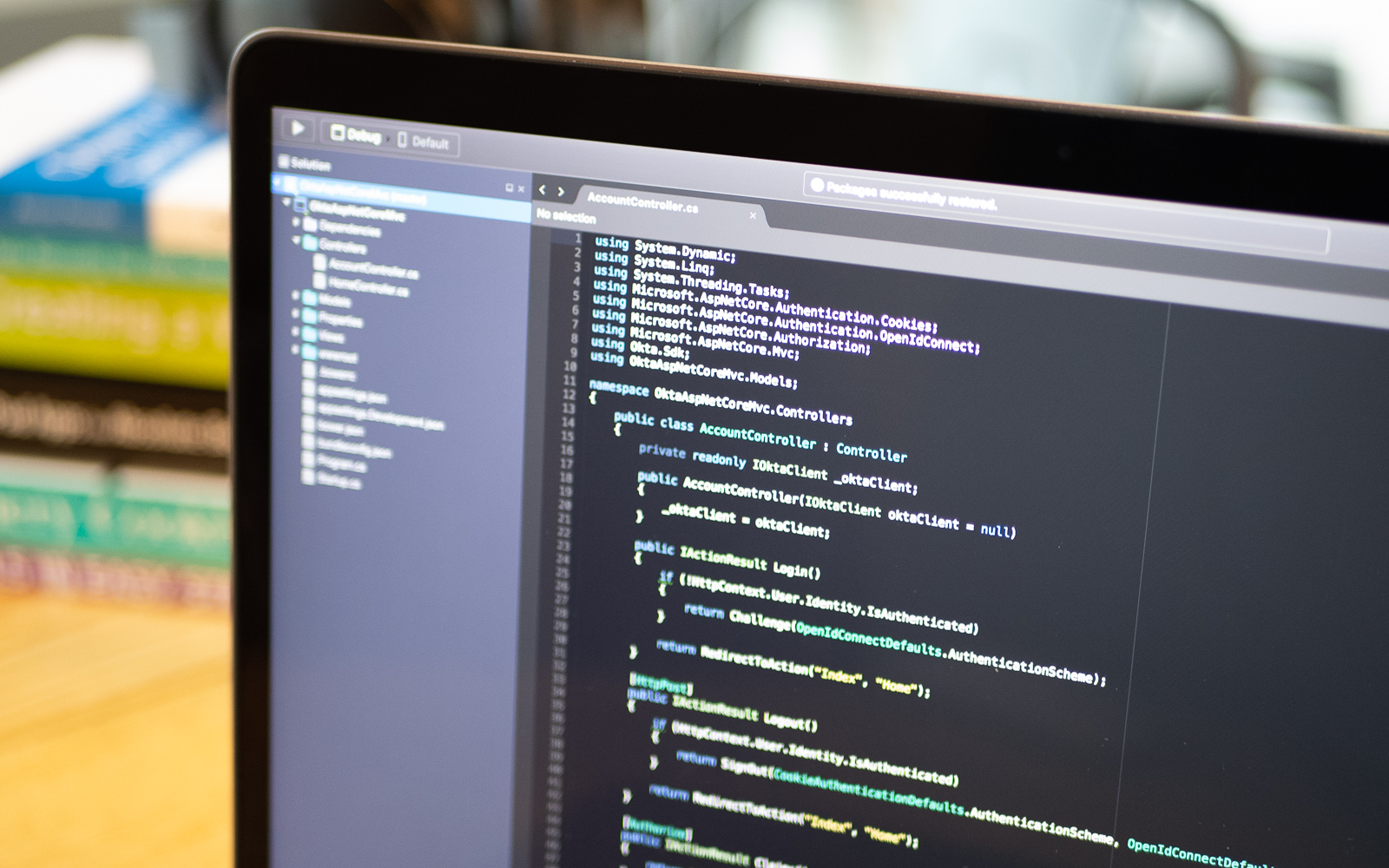
For large enterprise companies, boundaries around each team are crucial for maintaining systems that are owned by software engineers. This is accomplished by allowing individual teams to own their systems and expose them via APIs. You also need to keep certain types of cross-cutting concerns centralized, like select security controls, logging, and routing. If you don’t, every API across different teams has to implement common controls like rate-limiting, logging, and authentication. This can lead to...
Comparison of Dependency Injection in .NET
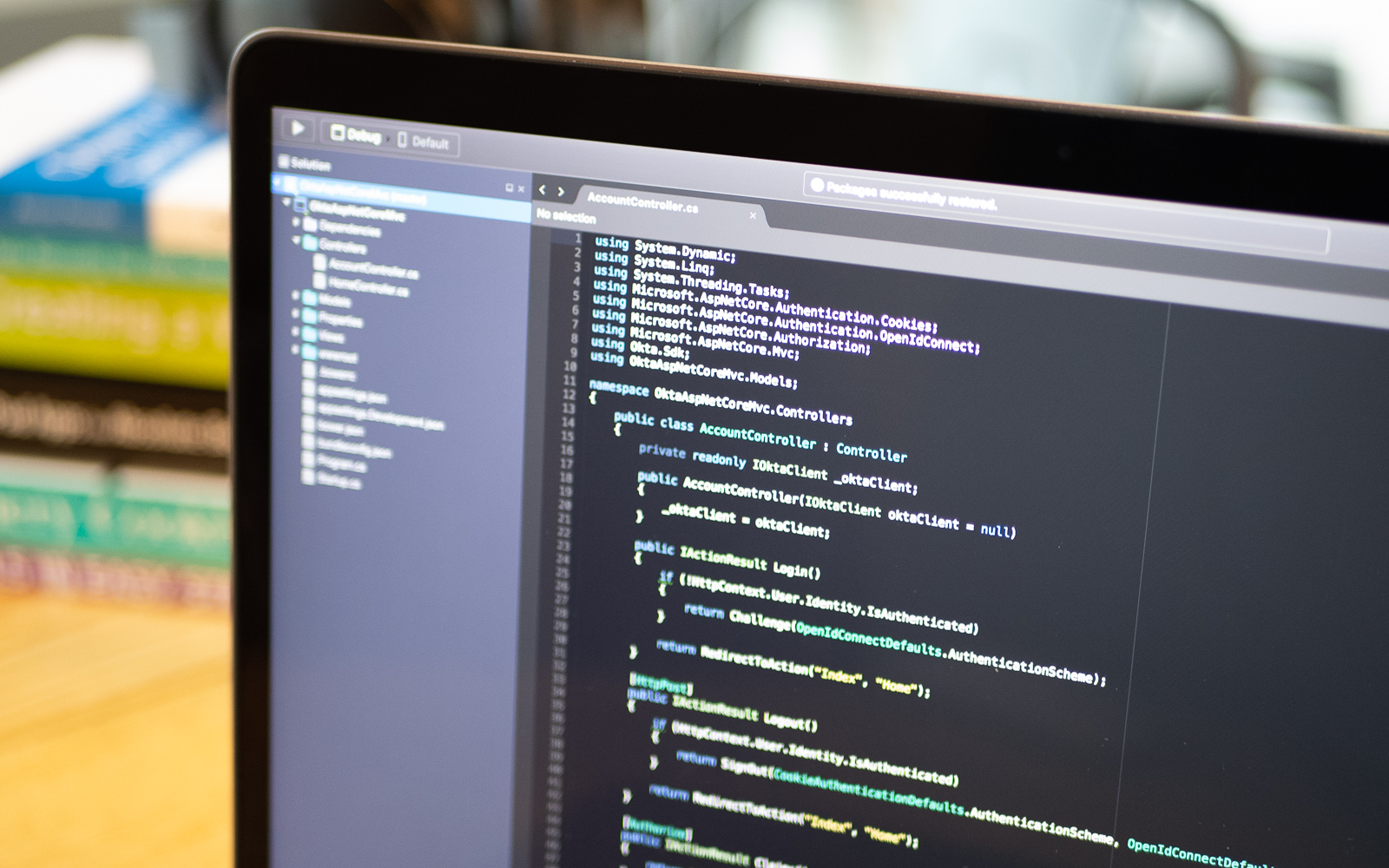
Why you should care about dependency injection Dependency injection is a tried and proven design pattern for producing code that is testable, readable, and reusable. This is achieved by creating (or increasing) a separation of concerns, where each class has a dedicated area of responsibility. Dependency injection is just one design pattern used to achieve inversion of control in which the calling code, or client, has no knowledge of the inner workings of the dependency,...
How to Deploy a .NET Container with AWS ECS Fargate
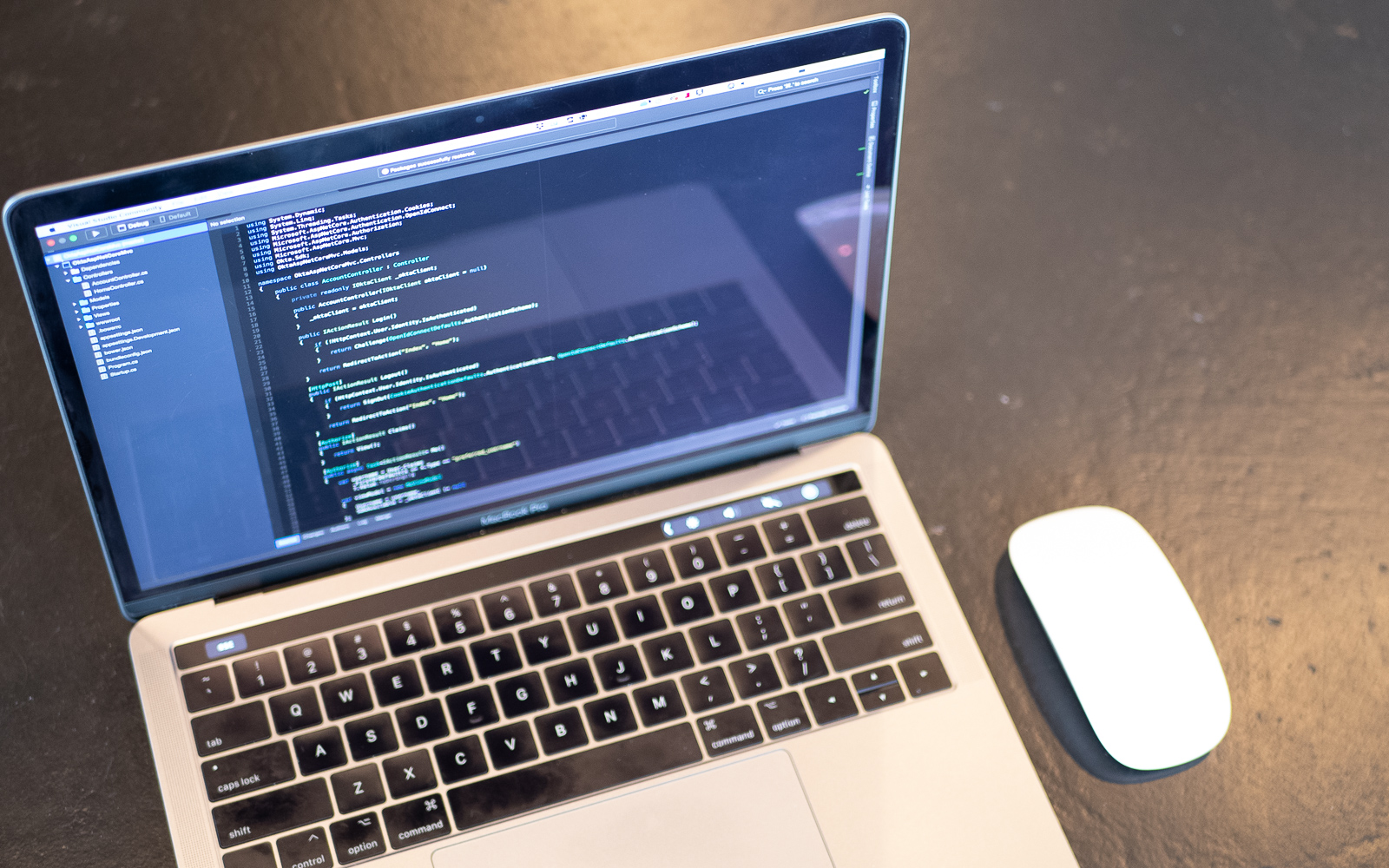
In a previous article, we learned how to host a serverless .NET application using AWS Lambda. We talked about the history of serverless and how companies are using these types of technology to simplify delivering APIs and functionality faster than traditional methods. Some problems will arise in this type of application when you need more capability than standard HTTP requests like GET, POST, PUT, DELETE, etc. Web Sockets is a great example of this. Table...
Using Azure Cognitive Services in a .NET App
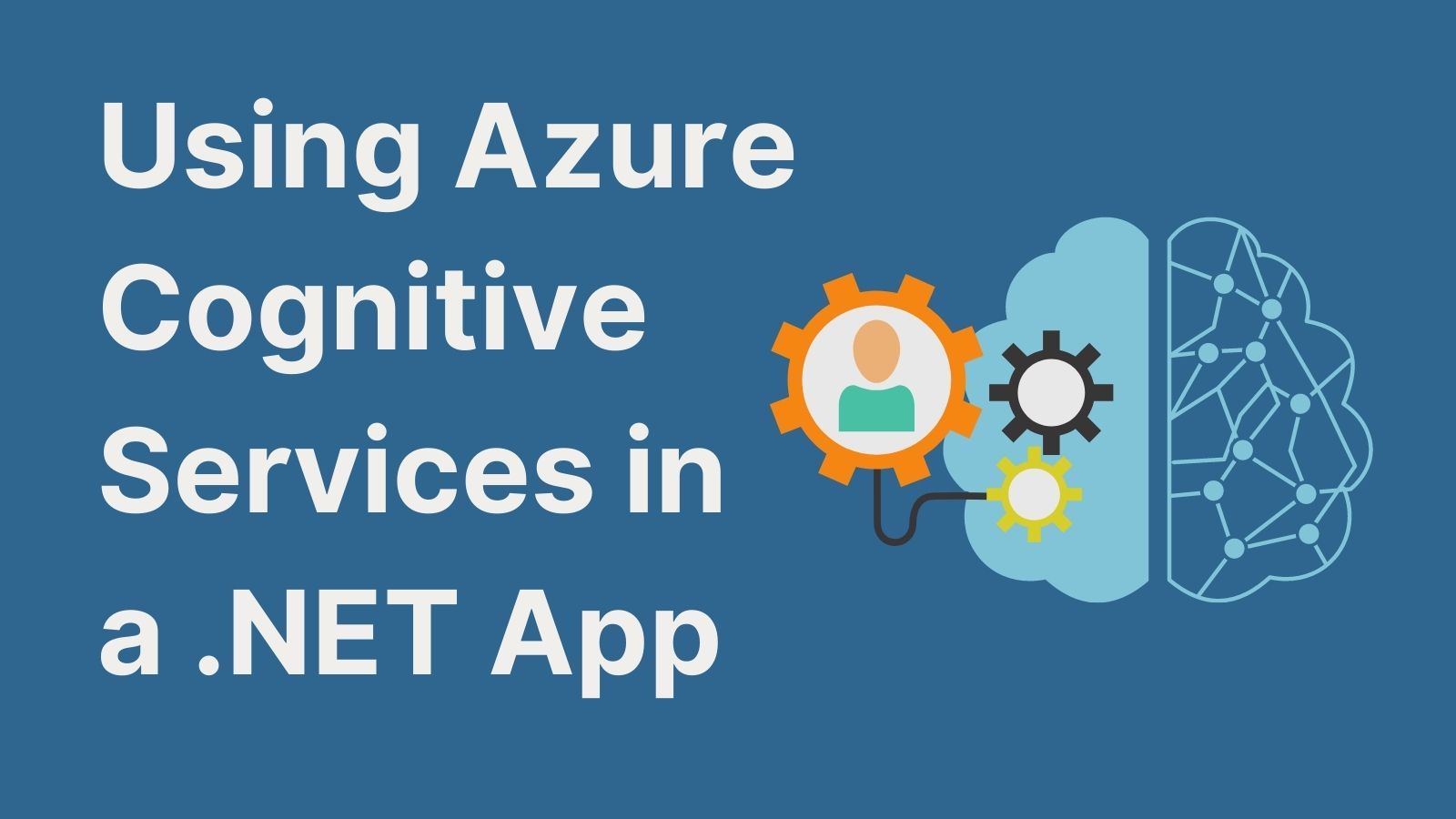
Azure Cognitive Services is a collection of cloud-based AI products from Microsoft Azure to add cognitive intelligence into your applications quickly. With Azure Cognitive Services, you can add AI capabilities using pre-trained models, so you don’t need machine learning or data science experience. Azure Cognitive Services has vision, speech, language, and decision-making services. In this article, you will learn how to use the Vision Face API to perform facial analysis in a .NET MVC application...
Secure Your .NET 5 Blazor Server App with MFA
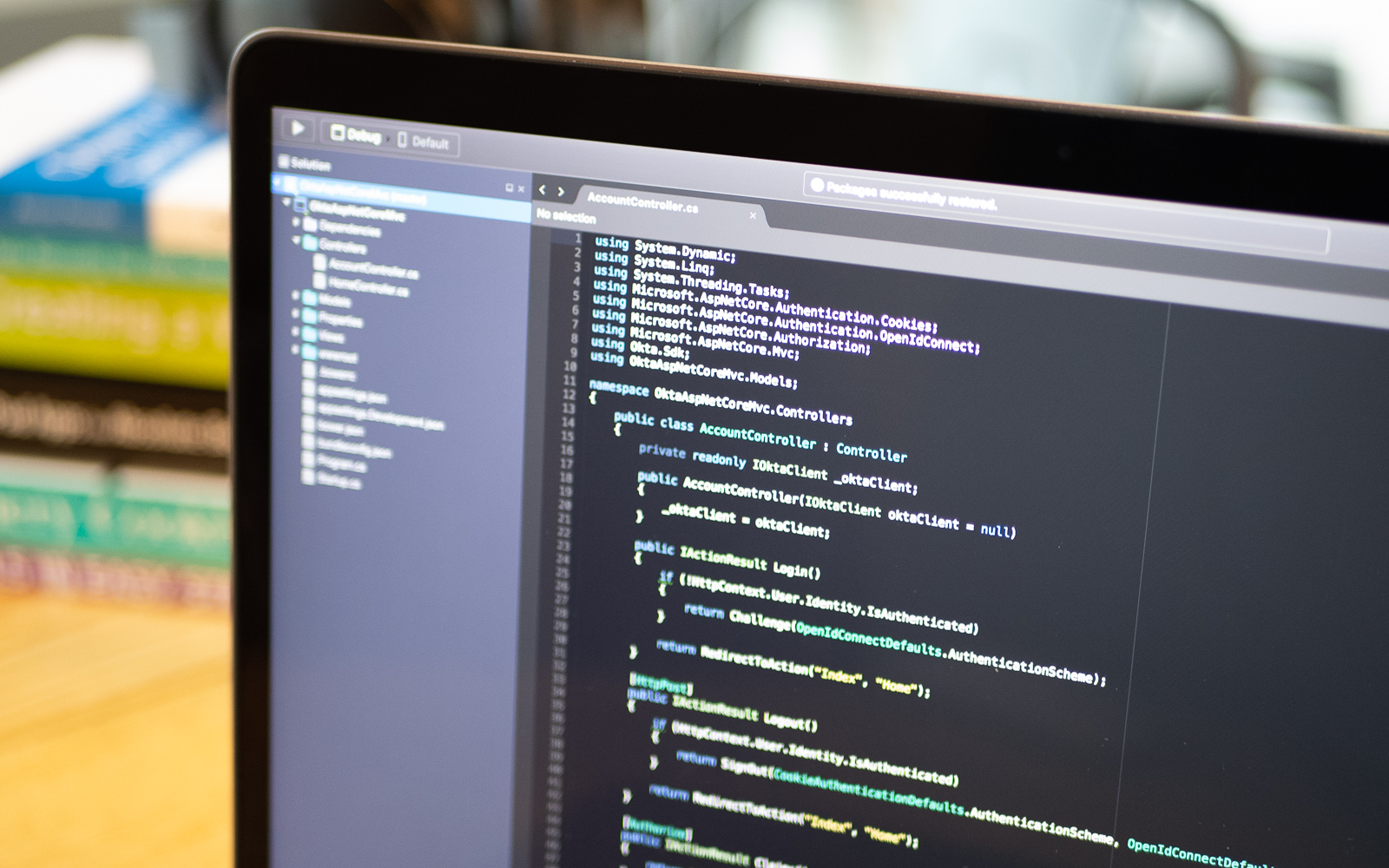
Introduction to Server Blazor apps Blazor is an exciting new technology from Microsoft that will allow developers to bring C# to clients. Server and client components are written in the same language and can be used and re-used interchangeably. Blazor comes in two flavors, server and client apps. In this tutorial you will be working with Server Blazor apps, where the C# code is run on the server, and messages are exchanged using SignalR. I’ll...
How to Write Cleaner, Safer Code with SonarQube, Docker and .NET Core
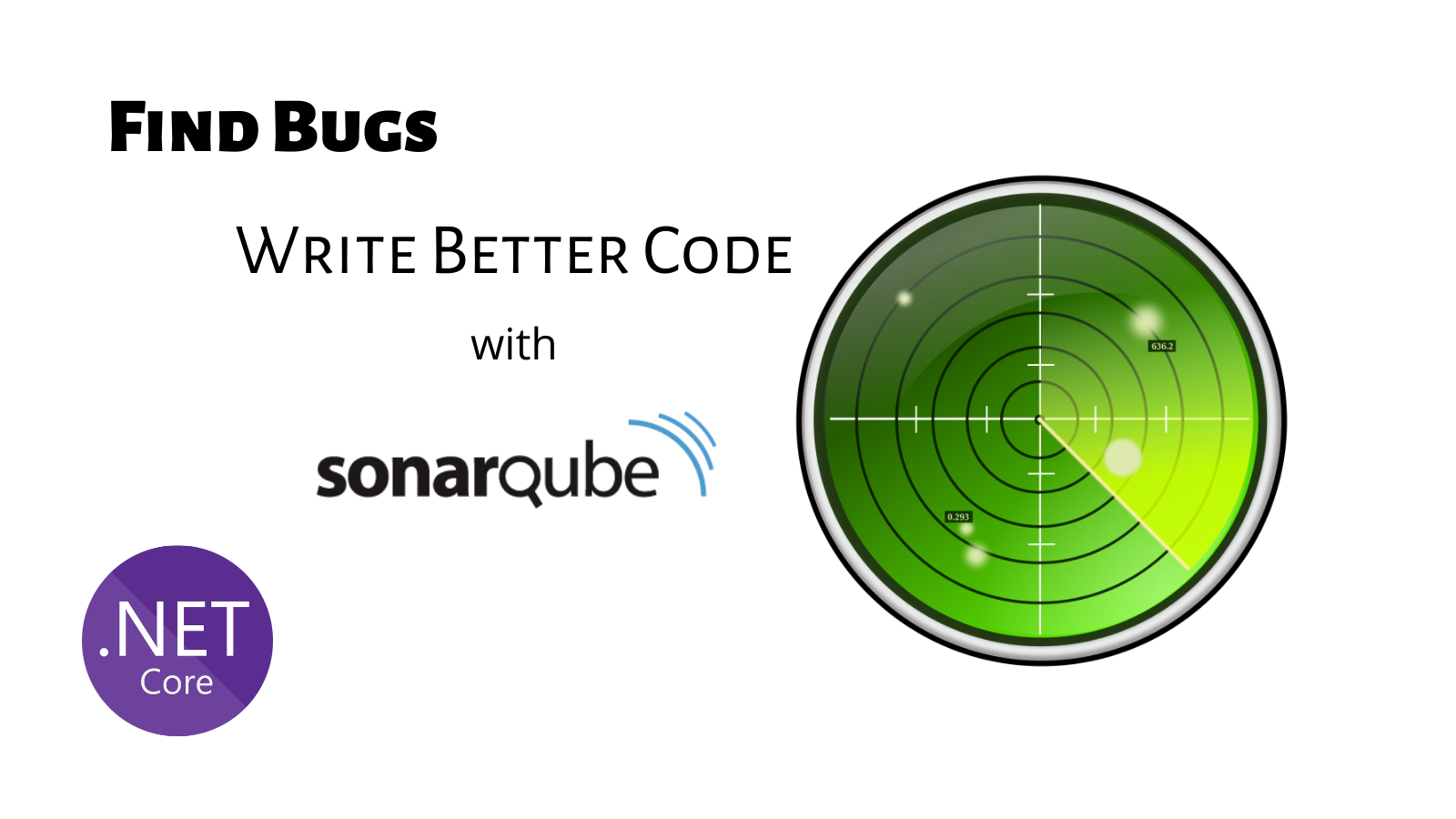
When it comes to code quality and code security, SonarQube is your teammate! This analysis tool is pretty straightforward to use, especially with some help from Docker. In this post, I’ll show you how to run a Docker container with SonarQube to analyze the code of a simple ASP.NET Core 3.0 application. Code analysis is a critical component of app development because it can identify security issues and other tricky bugs that might be overlooked...
Using AWS Toolkit for Visual Studio
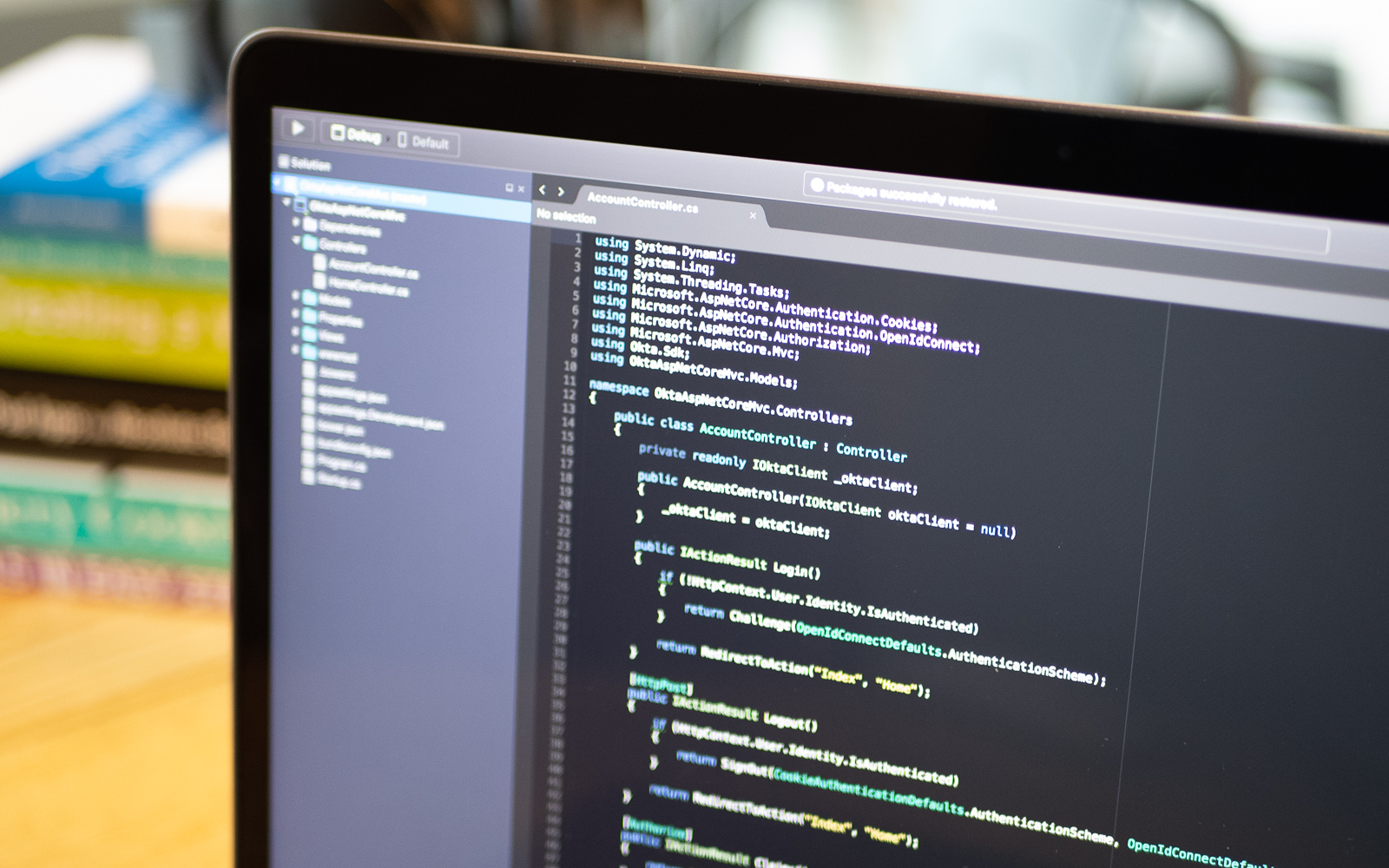
Amazon Web Services is one of the most popular cloud computing platforms on the planet. There’s a good chance you will need to work in an AWS environment, which means publishing to one of their web server services like Elastic Beanstalk. The AWS platform is enormous, and this can be an intimidating task for a developer. Luckily, Amazon has released their AWS Toolkit for Visual Studio 2013-2015 and AWS Toolkit for Visual Studio 2017-2019, which...
Web Forms Migration to Blazor in .NET Core
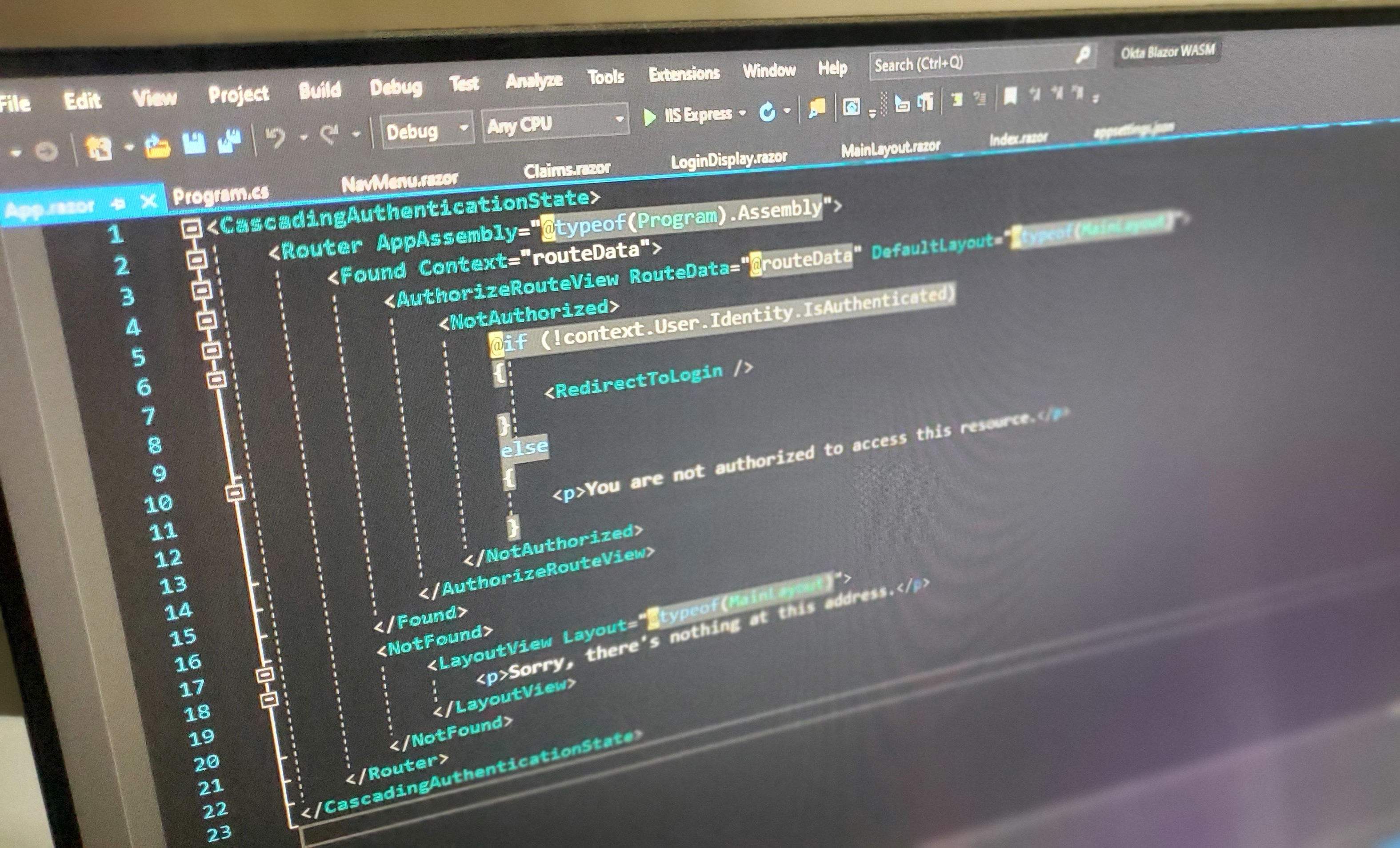
ASP.NET Web Forms framework has been the cornerstone technology of .Net for web development since the release of .Net Framework in 2002. ASP.NET Web Forms includes a layer of abstraction for developers so that you don’t need to care about HTML, JavaScript, or any other front-end technology. It provides a development flow similar to building desktop apps, a way for developers to build a web page by drag and drop, an event-driven programming model, and...
How to Toggle Functionality in C# with Feature Flags
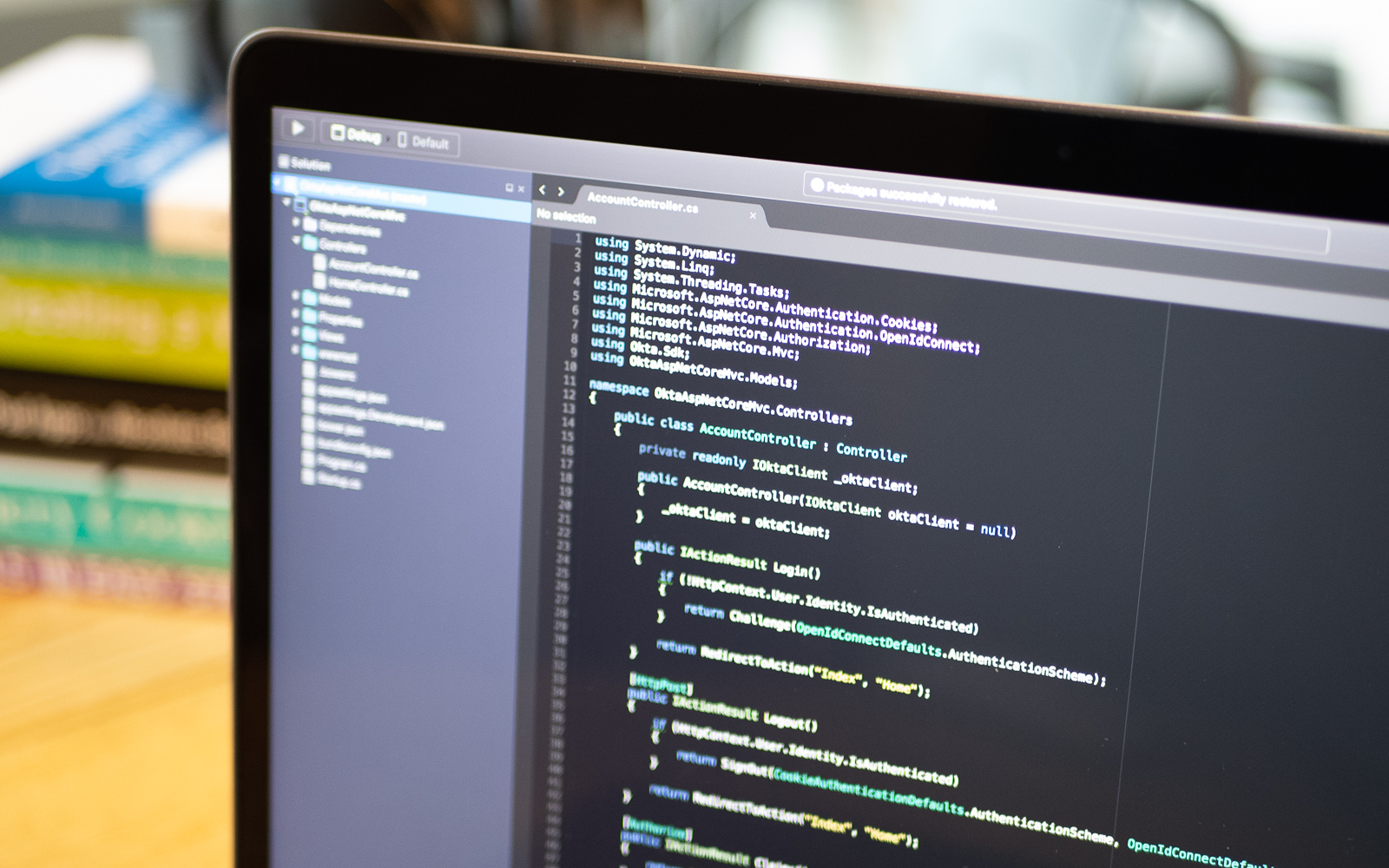
Toggling functionality using feature flags in .NET Core apps is quite straightforward, especially with a neat little feature flag management service. In this post, I’ll walk you through how to build a simple web application using Okta for user authentication and how to use ConfigCat to manage and access feature flags. What Are Feature Flags? Feature flags (aka. feature toggles) are a relatively new software development technique that enables development teams to turn features on...
Easy Xamarin Forms Auth with PKCE
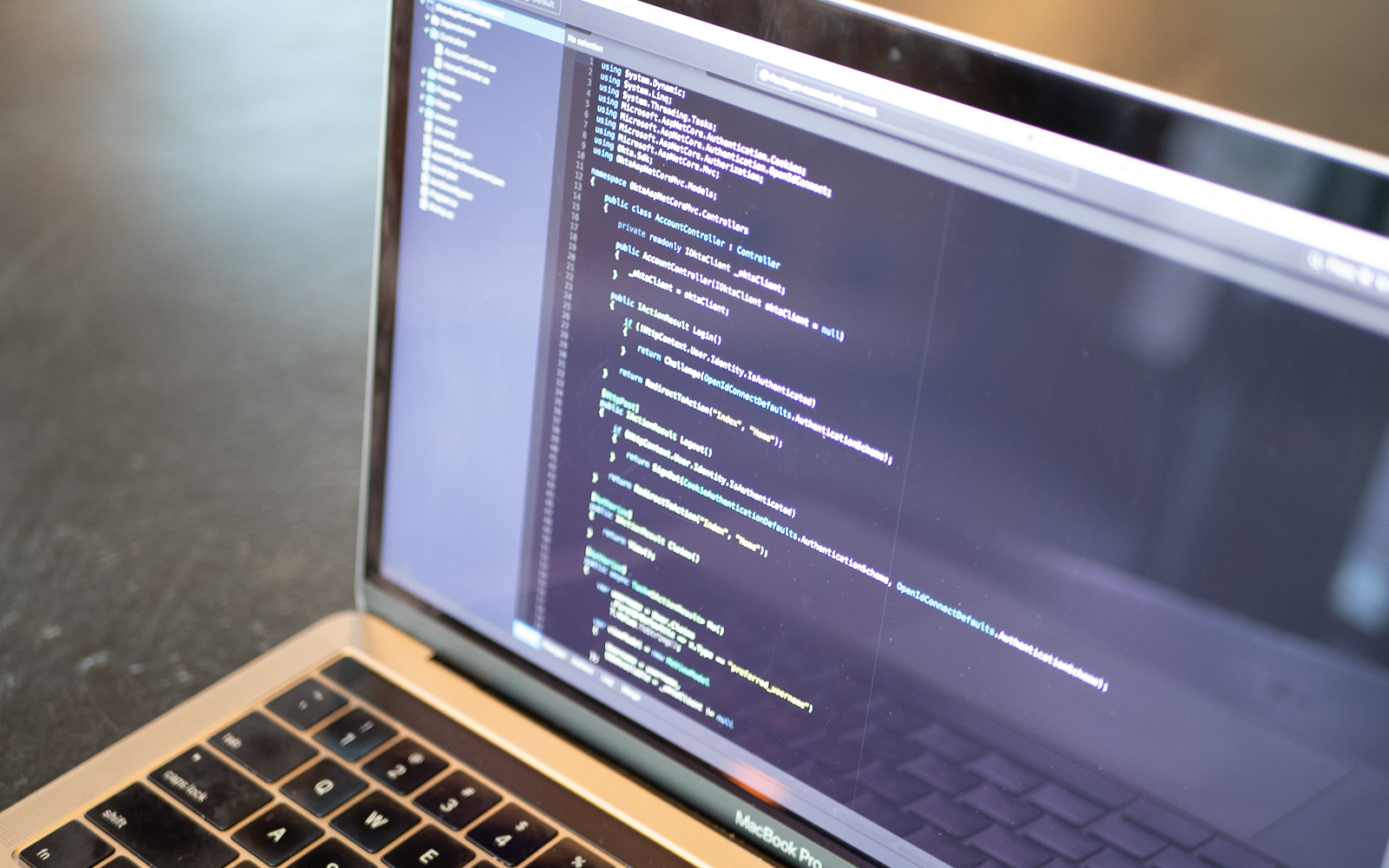
OAuth 2.0 is a protocol that controls authorization to access a secured resource such as a native app, web app, or API server. For native applications, the recommended method for controlling access between your application and a resource server is the Authorization Code flow with a Proof Key for Code Exchange (PKCE). In this article, you will learn how to build a basic cross-platform application with Xamarin.Forms and implement Authorization Code flow with PKCE using...
A Quick Guide to Elasticsearch for .NET
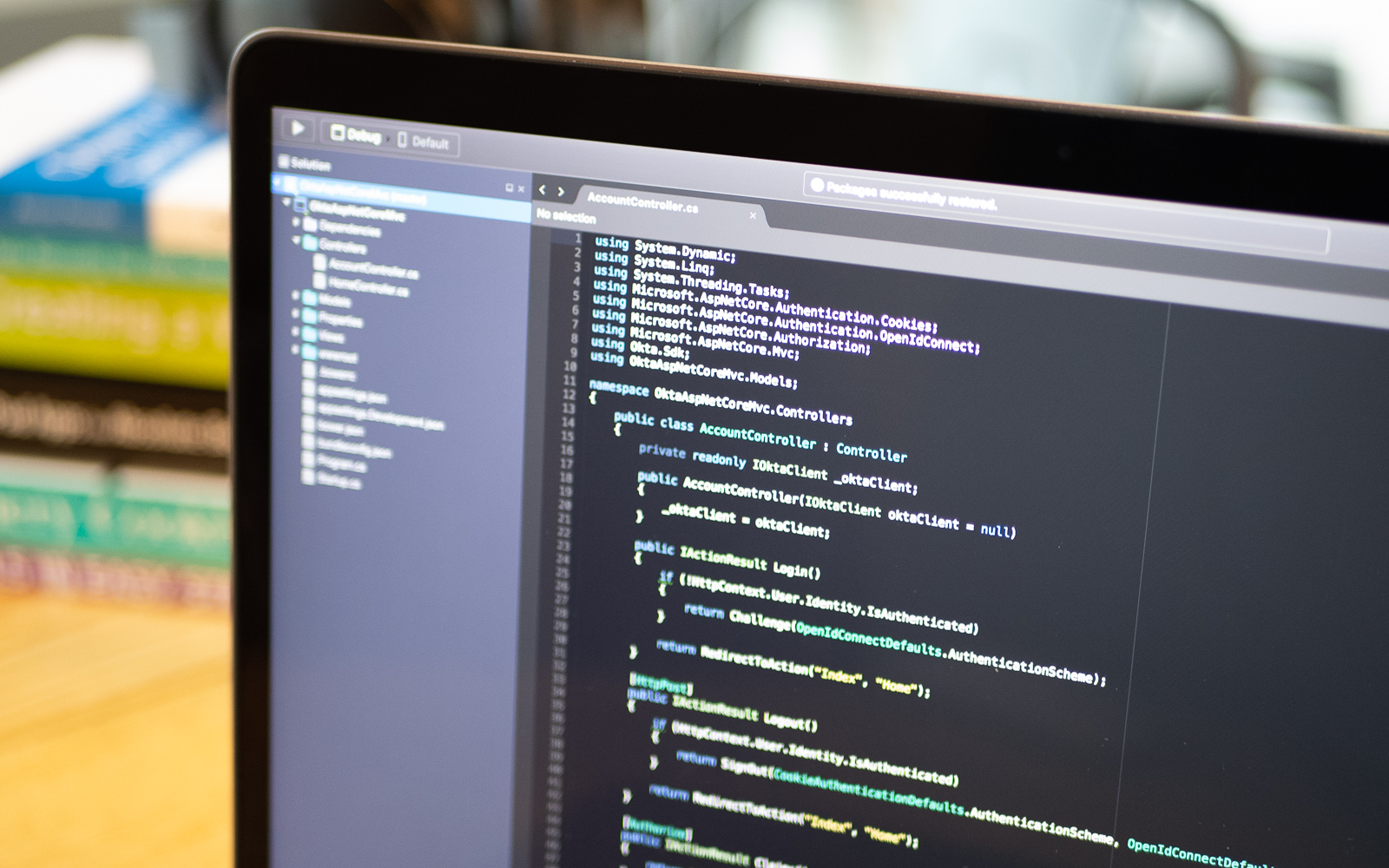
Implementing search functionality in your .NET Core apps doesn’t have to be hard! Using Elasticsearch makes it easy to develop fast, searchable apps. In this post, I’ll walk you through building a simple web application using Okta (for user authentication), Elastic Cloud (the official Elasticsearch hosting provider), and the fabulous Elasticsearch NEST SDK. Why Use Elasticsearch? Elasticsearch is an analytics and search engine based on the Apache Lucene library. It is developed in Java, following...
Update App Secrets with Jenkins CI and .NET Core
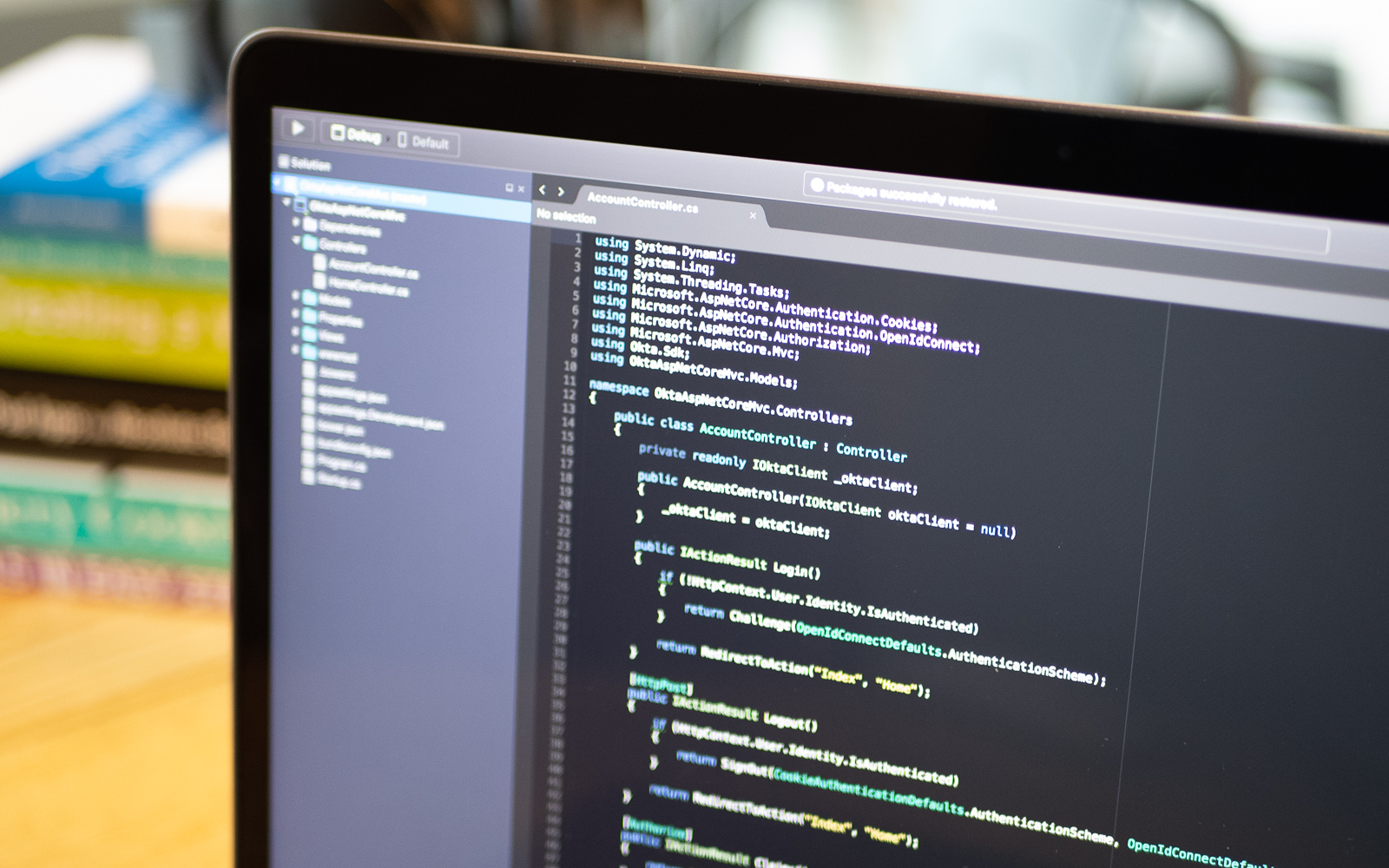
Introduction Jenkins is a free and open-source application that makes it easy to create CI/CD pipelines in almost any language or environment. Jenkins features a vast number of plugs to help create a CI/CD environment that is tailored to your technologies. In this application, you will create a .NET5 MVC web application and check it into a git repository. You will secure this application with Okta. Finally, you will set up a Jenkins project to...
Developer's Cheat Sheet for C# 9.0
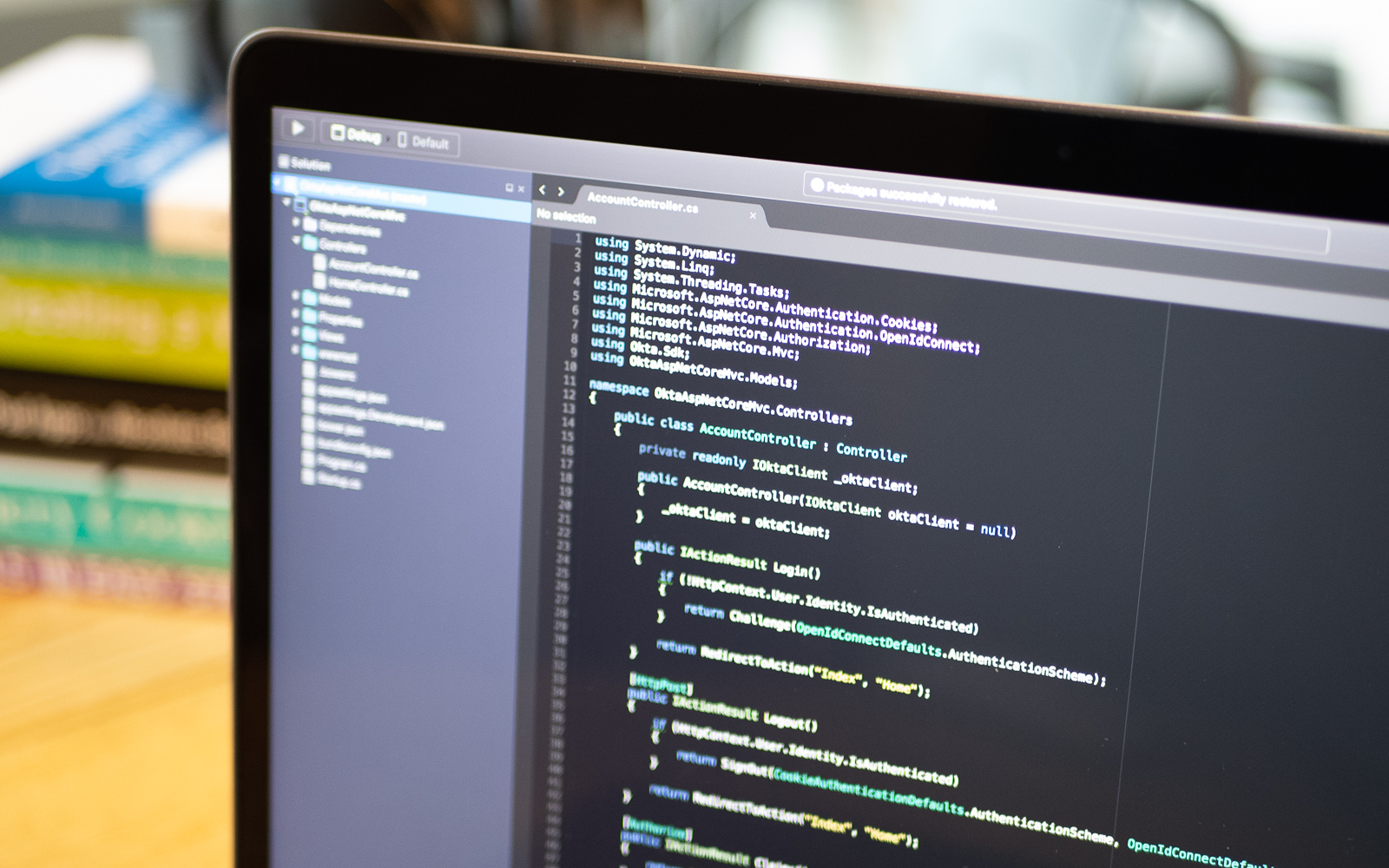
Introduction to C# 9 (and a bit of C# 8, too) Let’s start with a background on how C# 9 got here (implementation examples start in the next section). The last few years in computer science, we’ve observed the rising popularity of the #FreeLunchOver concept. The idea is that CPU technology, based on electrical signals and Von Neumann architecture, has reached its intrinsic limits. As long as integrated circuits were slower than light, we knew...
How to Write Secure SQL Common Table Expressions
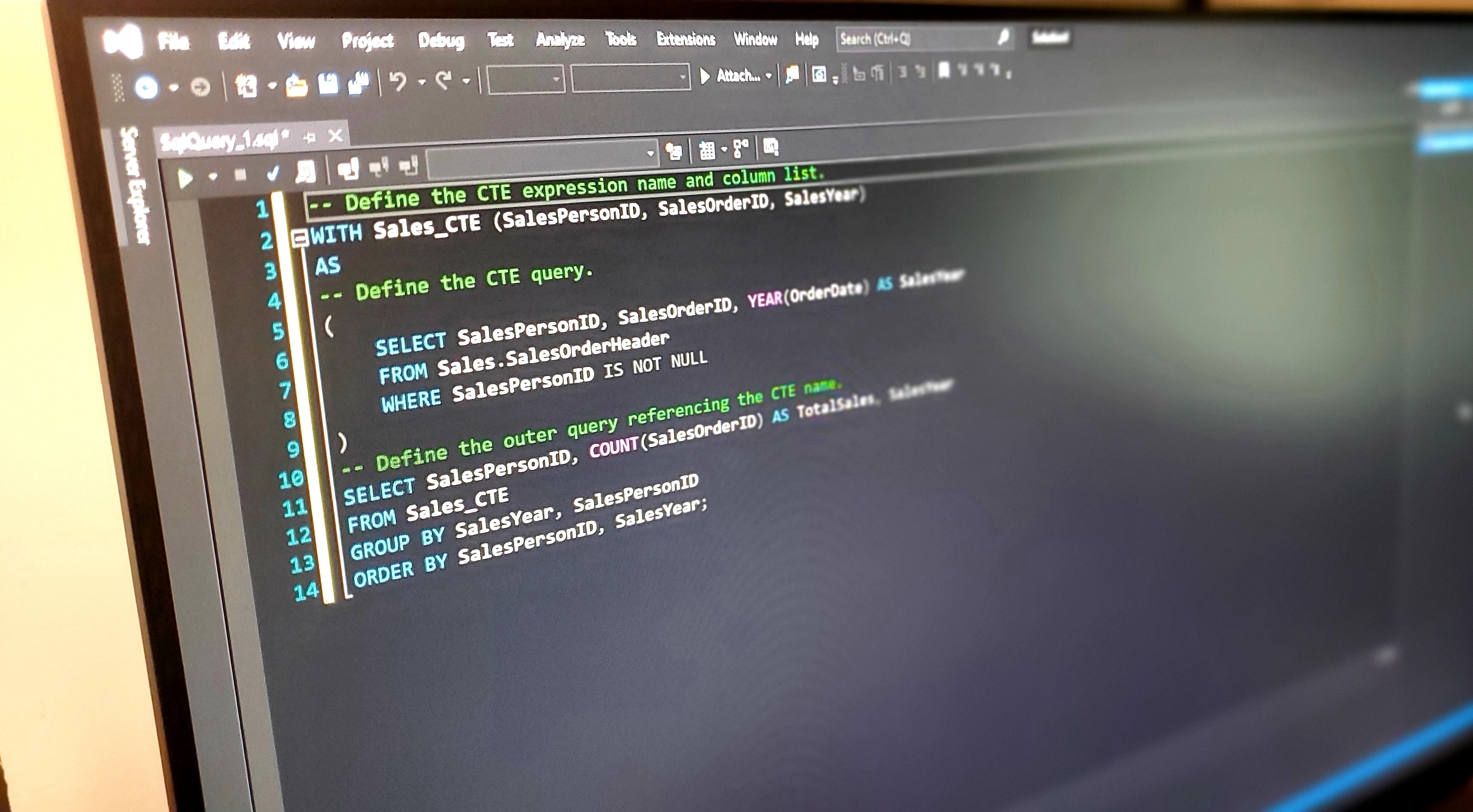
Common table expressions are a powerful feature of Microsoft SQL Server. They allow you to store a temporary result and execute a statement afterward using that result set. These can be helpful when trying to accomplish a complicated process that SQL Server isn’t well suited to handle. CTEs allow you to perform difficult operations in two distinct steps that make the challenge easier to solve. In this article, you will learn how to write common...
How to Use WebAuthn in C#
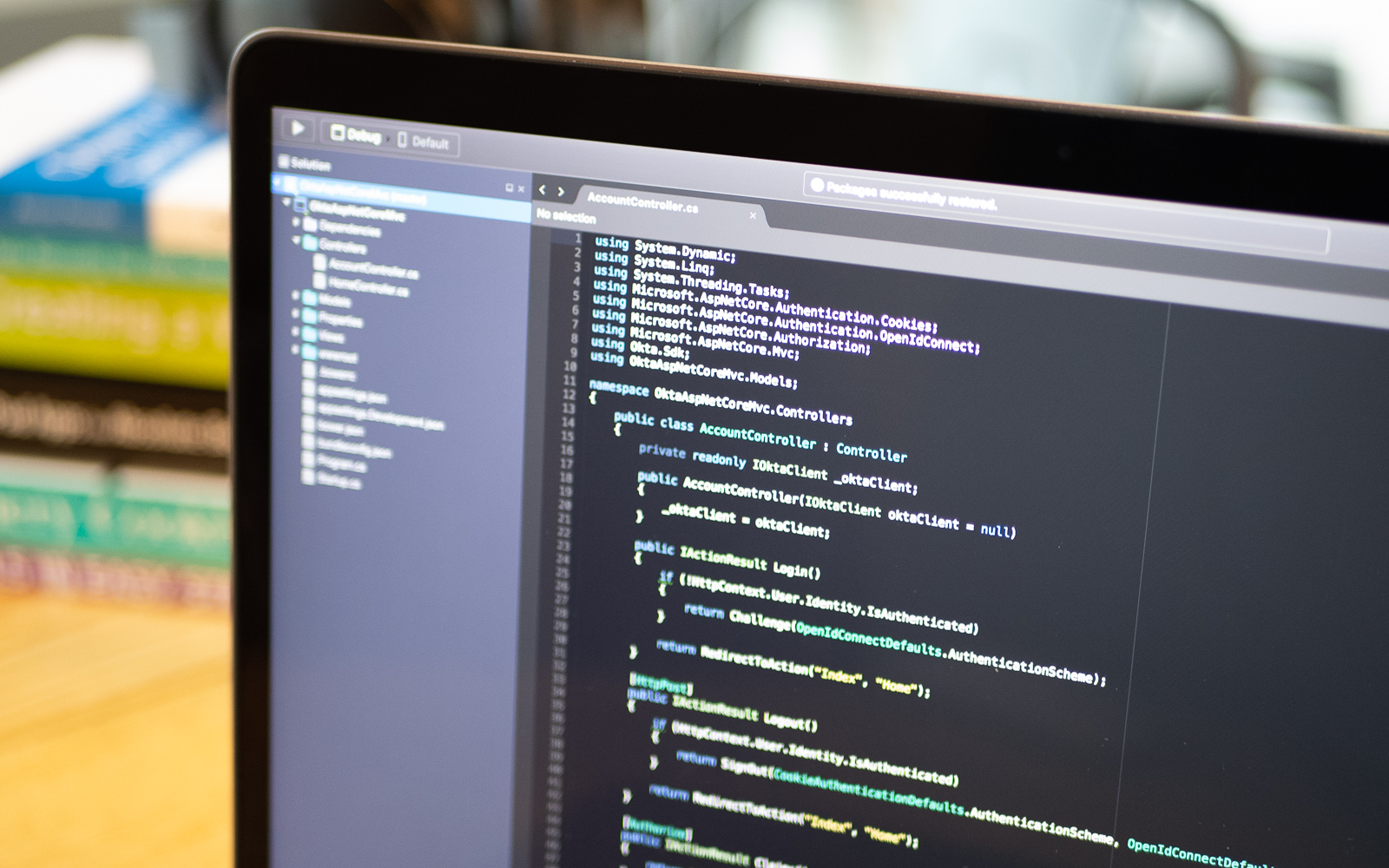
Nowadays, using a password for authentication is becoming less and less secure. Password attacks are becoming more sophisticated, and data breaches occur more frequently. Have I Been Pwned, the website where you can check if your account has been compromised in a data breach, contains more than 10 billion accounts and more than 600 million passwords. With 62% of users reusing passwords, a successful attack on one of the websites gives the attacker access to...
How to Support .NET Core SameSite + OAuth Apps on Linux
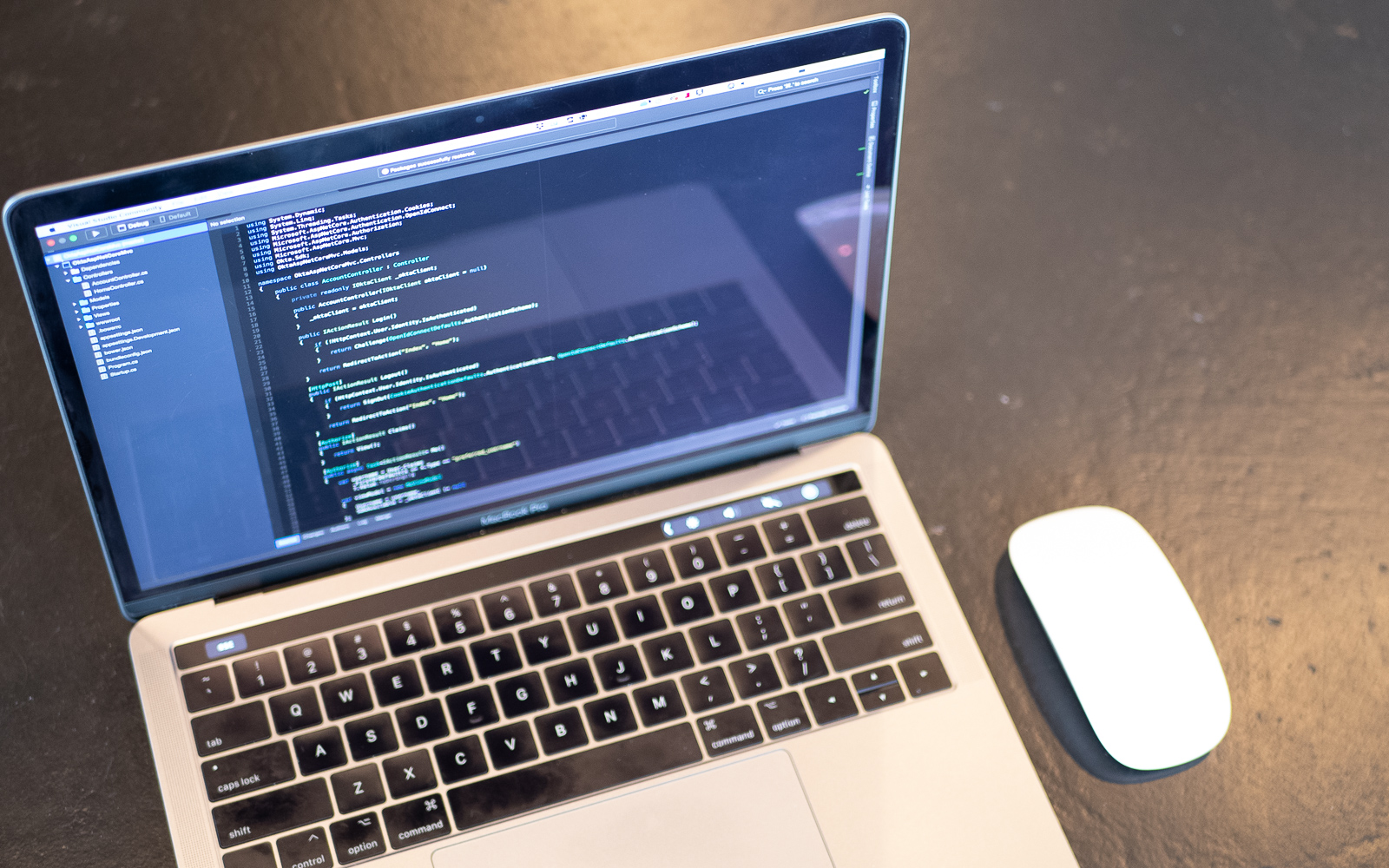
Google’s recent approach to SameSite cookie attributes caused a bit of confusion among developers. Especially in cases where handling redirects is necessary. After doing some research in the topic I’d like this article to be a guide on how to handle SameSite cookie attributes properly in production. This guide can serve as the basis for deploying an application to any Linux based environment—such as AWS Elastic Beanstalk, Google Cloud App Engine—or any VPS Linux deployment....
Introducing the Okta CLI
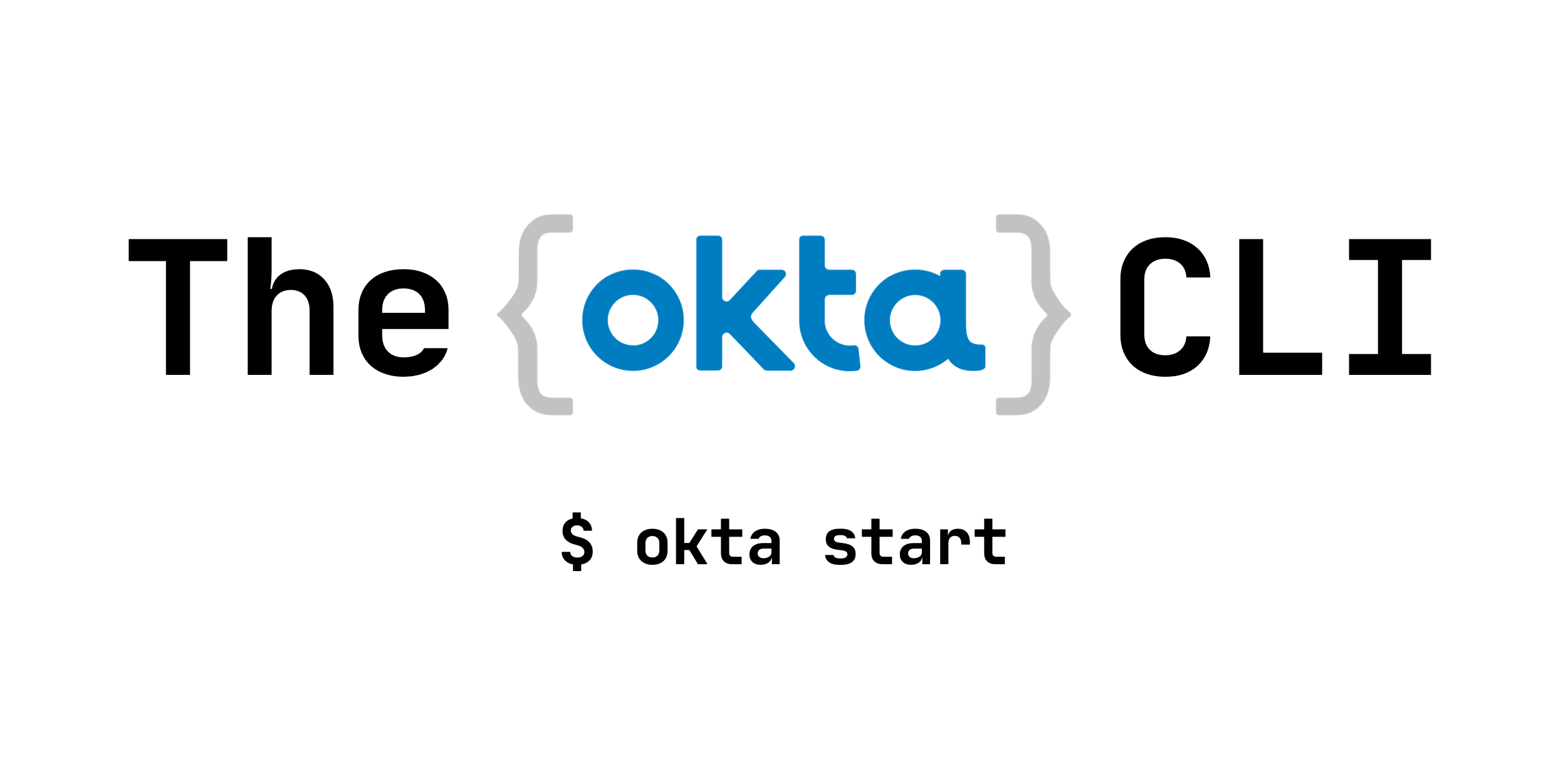
Okta is an Identity Management Platform that takes all the hassle out of authentication and authorization. It’s feature-packed with everything from workforce integrations for G-Suite to the latest version of OAuth 2.0 as-a-service for developers writing their own APIs. It can sometimes be a daunting task for developers to get started with Okta because of how feature-rich it is. Introducing the Okta CLI - made by developers for developers. Using the CLI tool, you can...
How to Deploy Your .NET Core App to Google Cloud, AWS or Azure
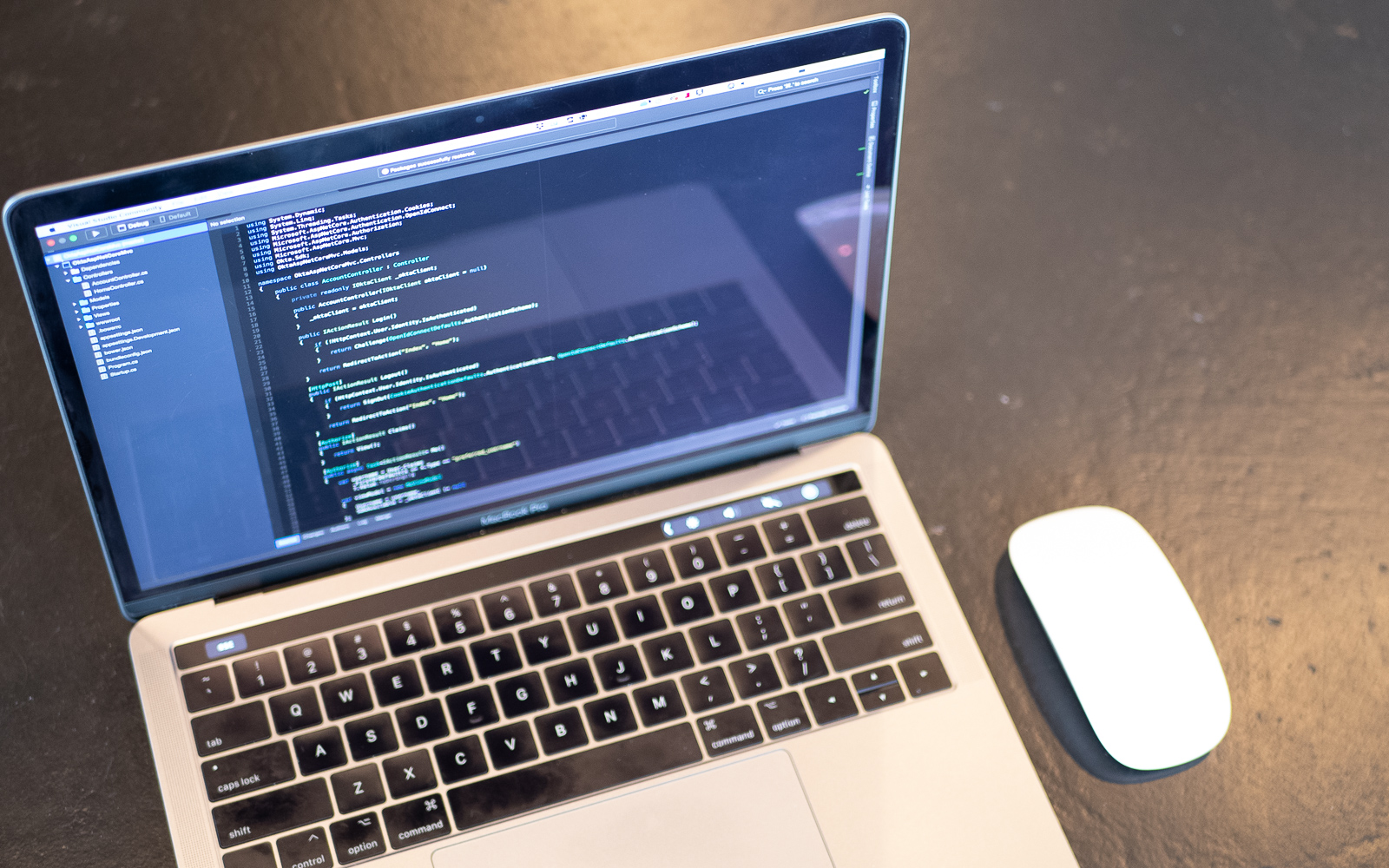
There has been a cut-throat competition between cloud hosts in the past few years - each attempting to earn the sympathy of developers and dev-ops by rolling out shiny new tools, plugins, and integrations. There are a gazillion how-to tutorials and guides in the community on using these tools. Sometimes when looking for a solution, it is hard to find the newest and simplest way. I never know if an article written last year is...
Rider for C# - The Best Visual Studio Alternative IDE
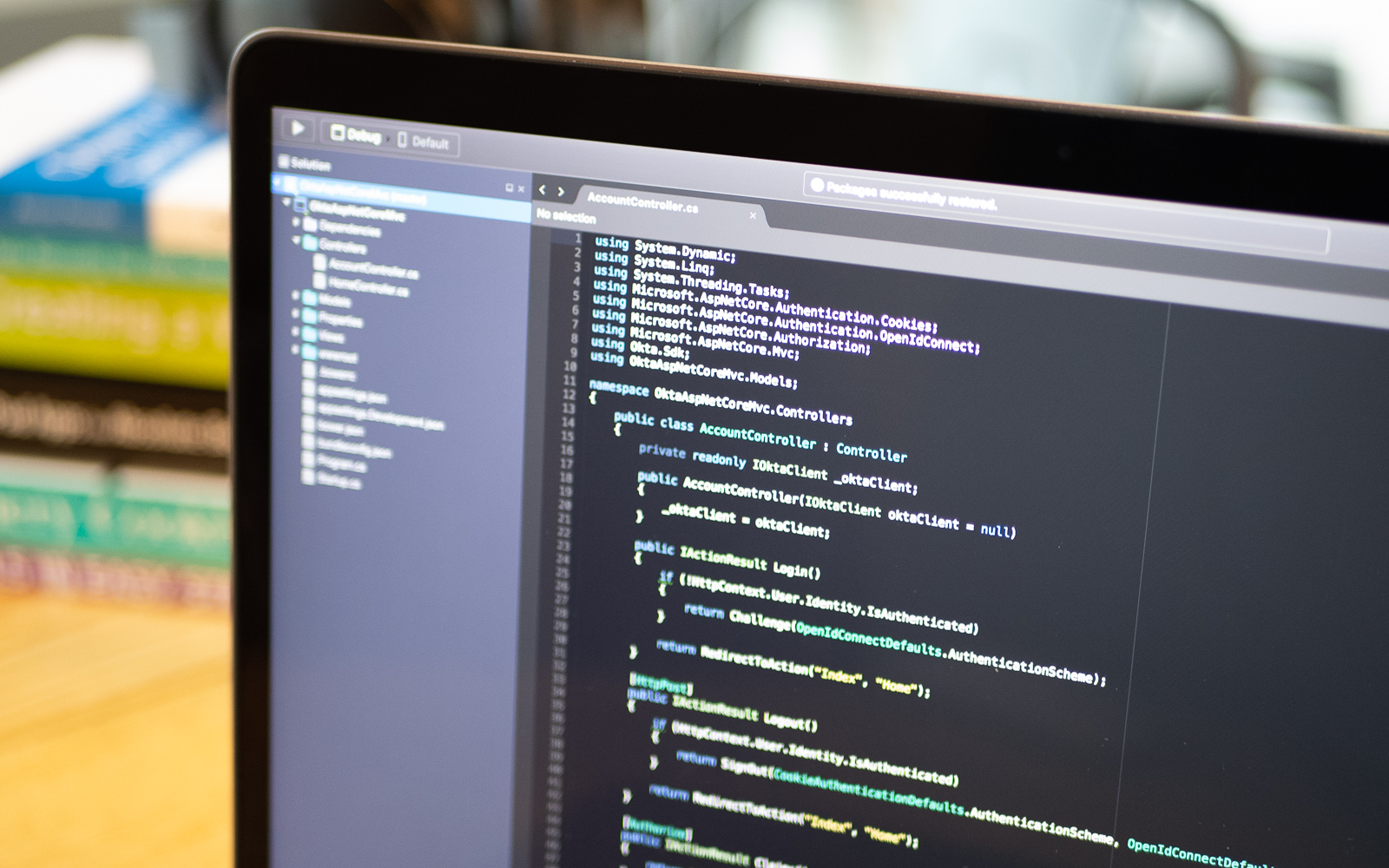
When it comes to developing .NET apps, Visual Studio has historically been the default choice for .NET developers for two main reasons: Visual Studio is the official integrated development environment (IDE) from Microsoft, and There was no viable alternative to Visual Studio This all changed about three years ago when JetBrains, the company behind Resharper, one of the most popular Visual Studio extensions, released a new, cross-platform, and innovative IDE - Rider. The Rider IDE...
Install .NET Core Apps on Linux in 5 Minutes
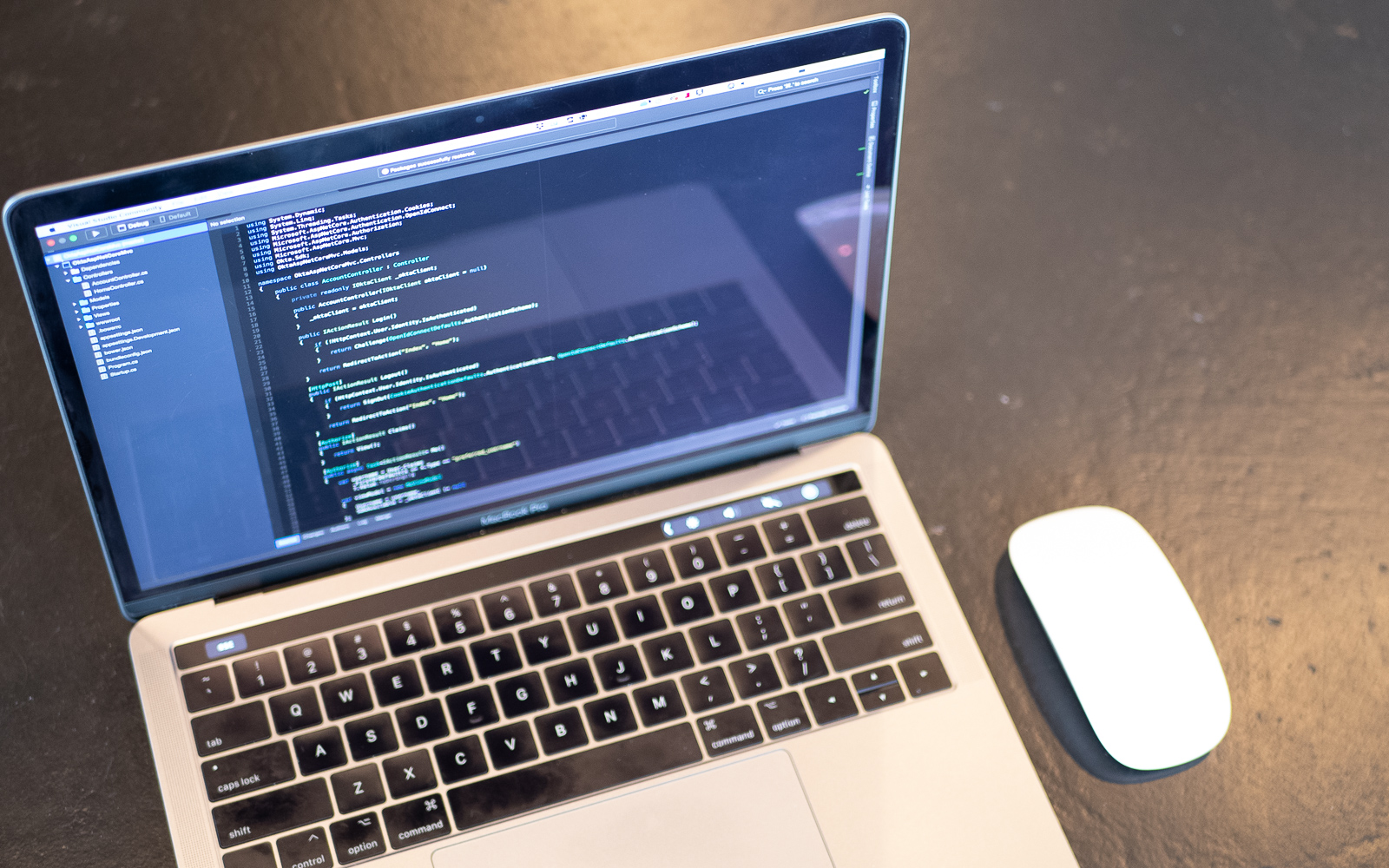
As a big fan of open source, I’m loving the fact that .NET Core is cross-platform. It opens up endless possibilities, from hobby projects, experiments, and proofs of concept, to massive high-load production applications that run on cost-effective infrastructure with high security and scalability. I usually get the simplest and cheapest $5/month Ubuntu-based virtual private server (VPS) from any cloud platform provider instead of the more complex and expensive container instances or cloud computing services....
How to Authenticate with SAML in ASP.NET Core and C#
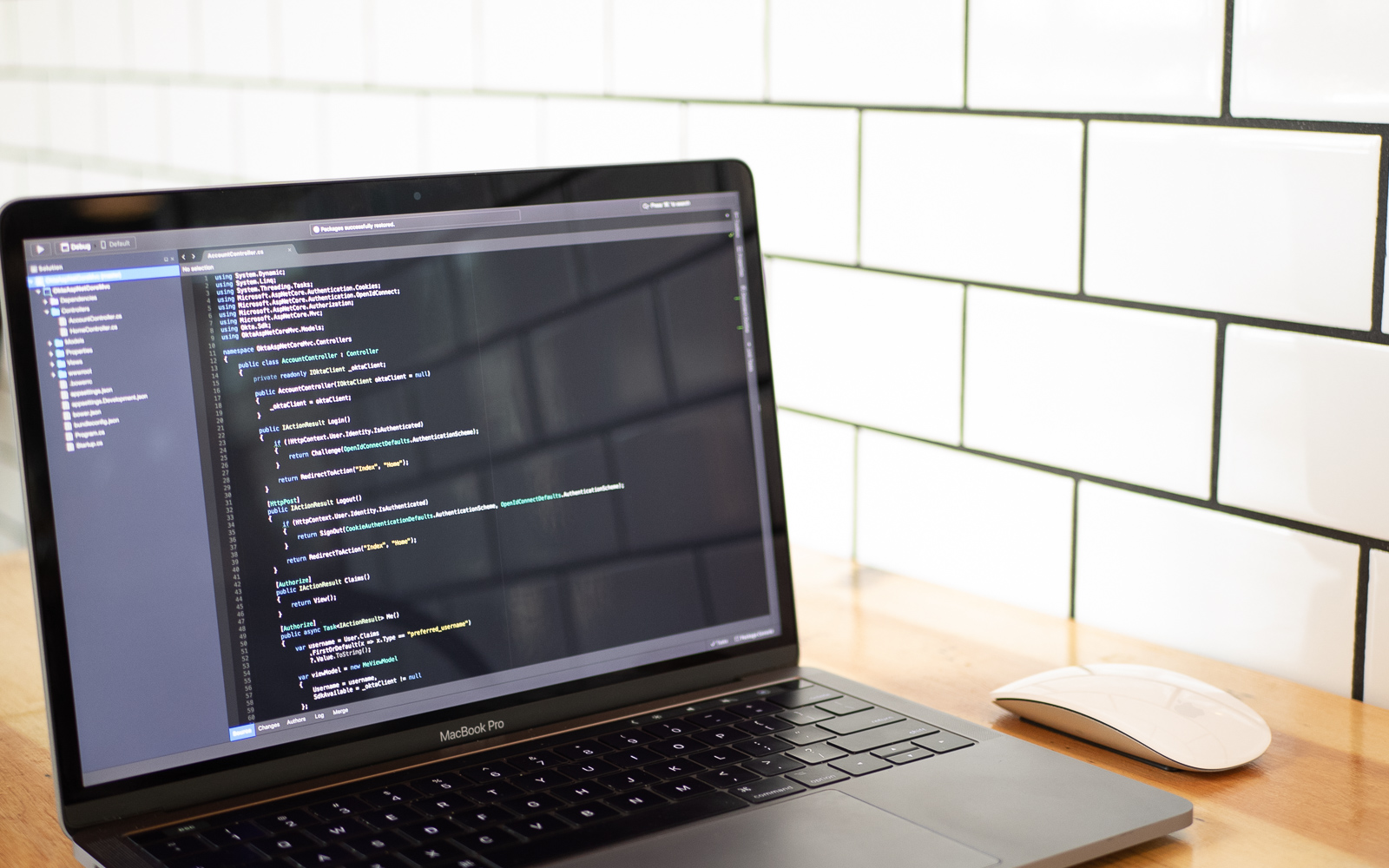
Security Assertion Markup Language, more commonly known as SAML, is an open standard for exchanging authentication and authorization data between parties. Most commonly these parties are an Identity Provider and a Service Provider. The primary use case for SAML has typically been to provide single sign-on (SSO) for users to applications within an enterprise/workforce environment. Up until the past few years, SAML was considered the industry standard—and proven workhorse—for passing an authenticated user into applications...
Deploy a .NET Container with Azure DevOps
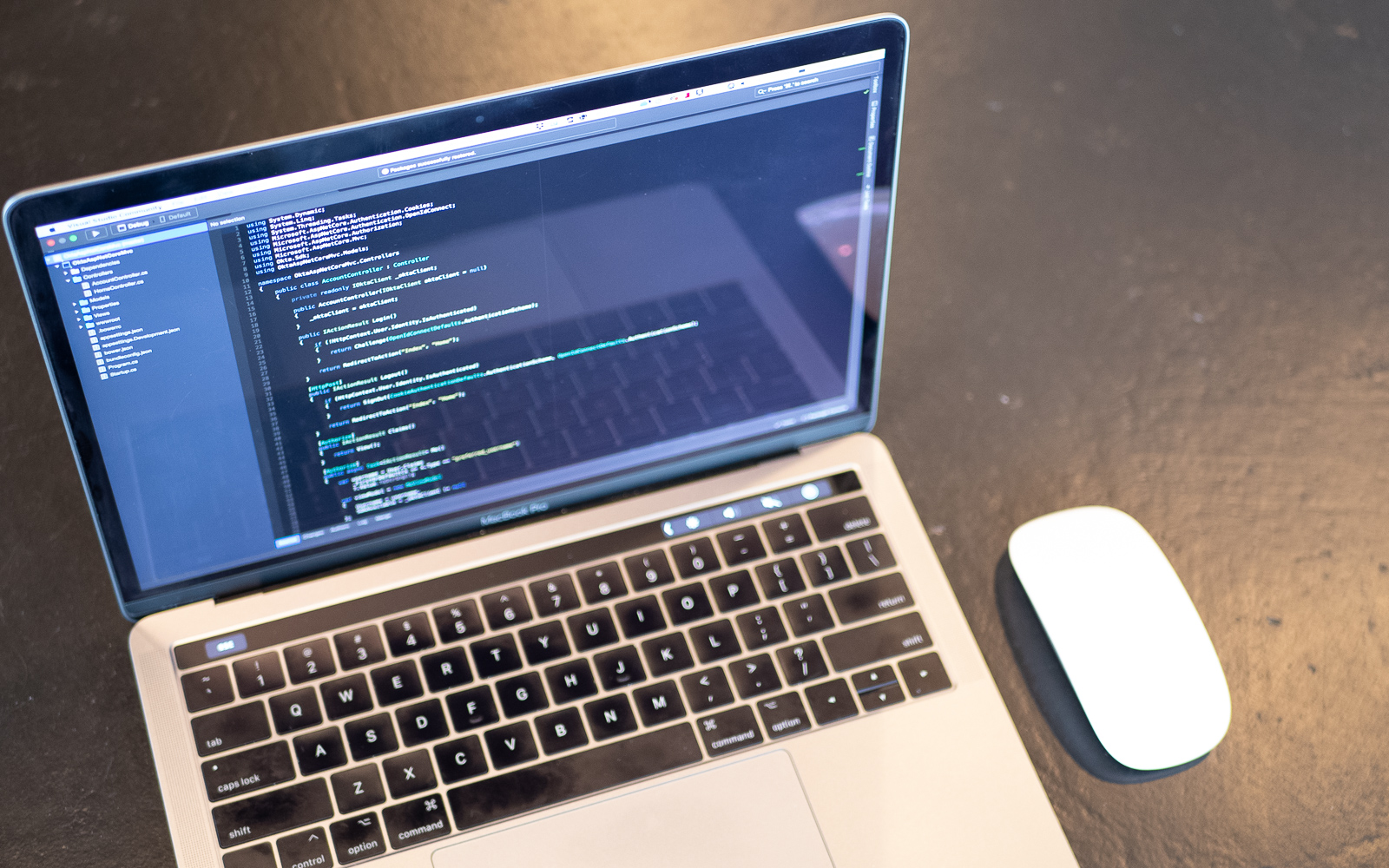
When I began programming (in the ’80s), computers weren’t equipped with a network card by default. The internet was almost unknown and modems were slow and noisy. The software was installed from stacks of flexible floppy disks. Today, computing resources are virtual. The internet is vital and there is an URL for everything. We live in the *aaS (* as a Service) era, where if you want something, there is likely one or more something...
How to Build Securely with Blazor WebAssembly (WASM)
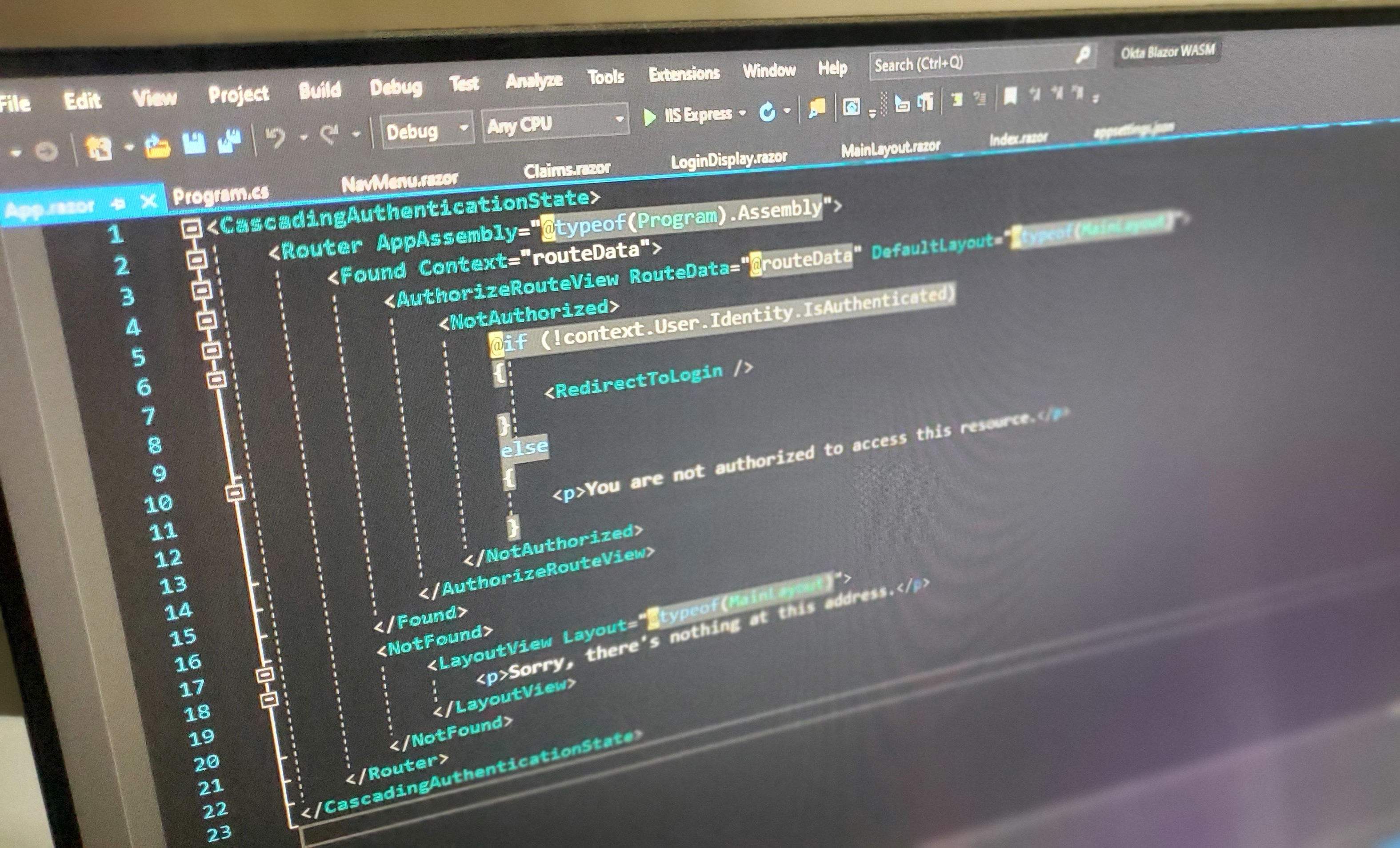
Last month I came out with a video tutorial on Blazor WebAssembly and I thought it would be good to follow up with a written tutorial as well. Youtube Link Because of the JS Interop, Blazor WASM has some key differences in the world of OAuth. Instead of thinking of this as a normal .NET back end application, you have to think of what Blazor resolves to in the browser - and that’s Javascript. Therefore,...
How to Adapt Your .NET App for SameSite
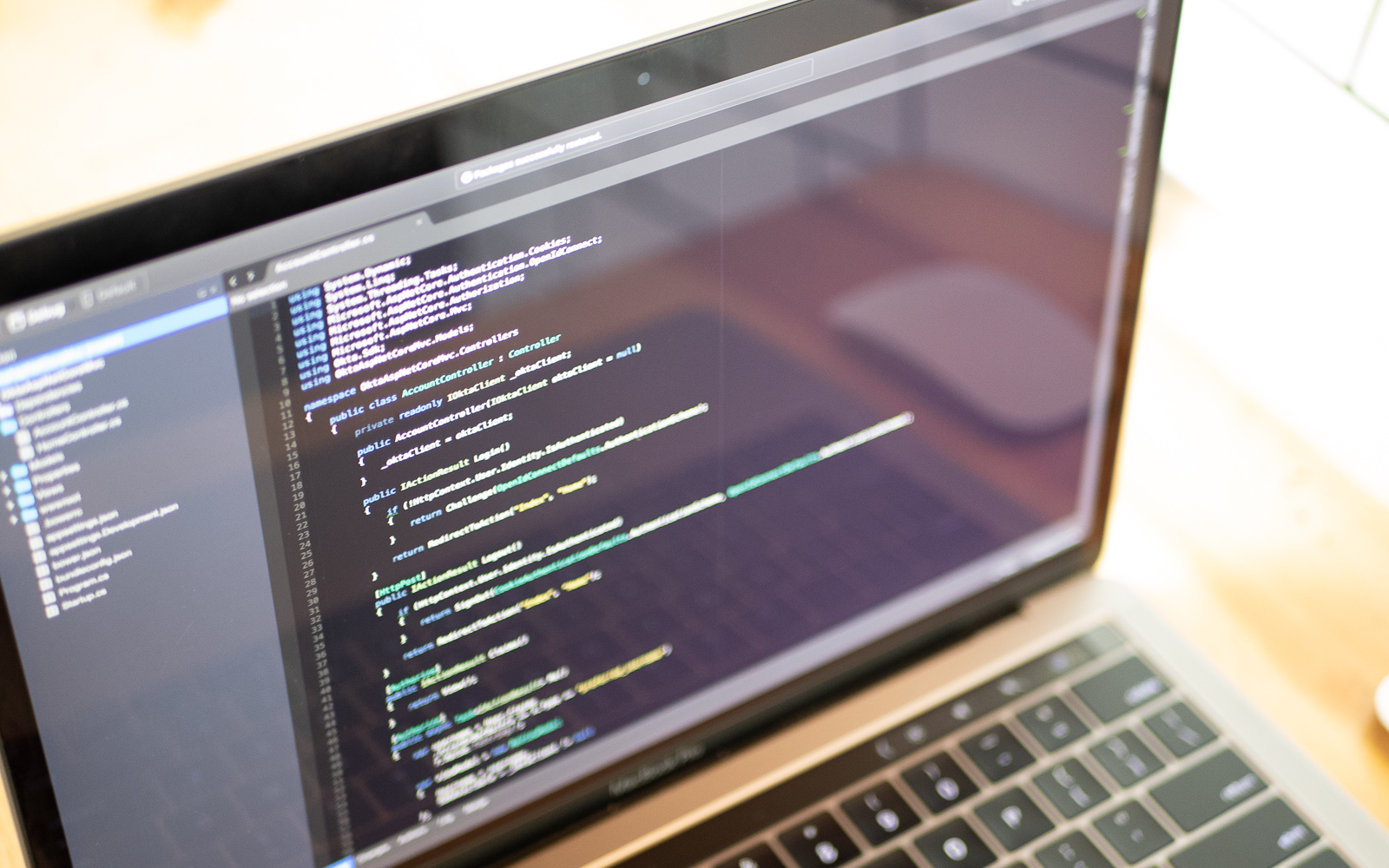
Have you run across an error message vaguely referencing SameSite in your .NET Apps? Read on, it’s time for a change to your code - and I’ll explain why. I like cookies, both the custard stuffed and the dry ones (which I use to dunk in my coffee or tea). This post is very much about cookies - only not the delicious, culinary ones. As in many other cases (think of the web, for example),...
How to Secure PII with Entity Framework Core
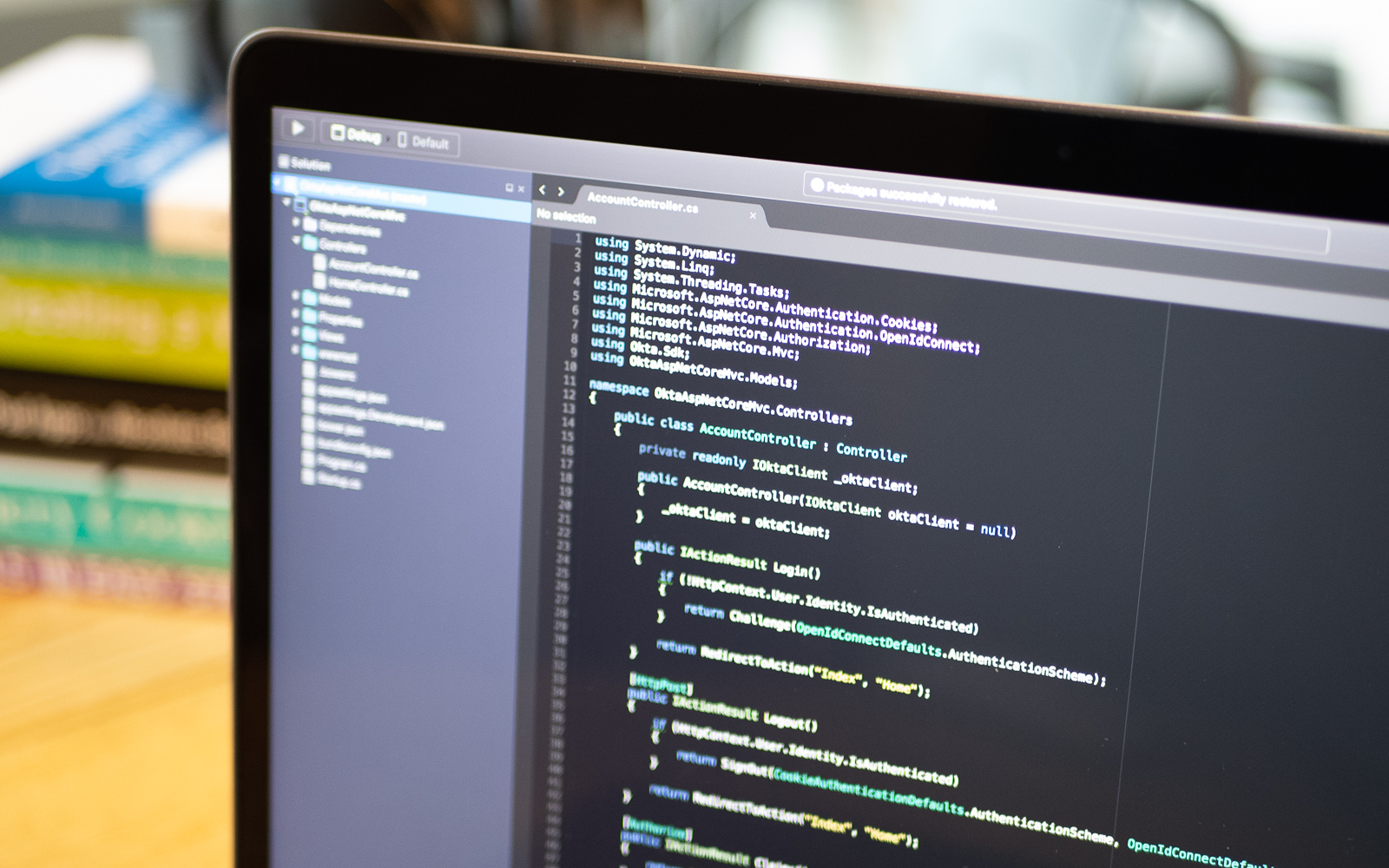
When the products we develop collect sensitive data, it’s essential to secure and safeguard it to protect user privacy and the user’s identity. Personally identifiable information (PII) is any data that can be used to identify a specific individual, such as name, email address, phone number, US Social Security number, birth date, and so on. Yet companies also need to store user-related data that is not as sensitive as PII. In this tutorial, you will...
Welcome Nick Gamb
My name is Nick Gamb and I am excited to be joining the Okta Developer Advocacy team for the .NET community. Who Am I At heart, I am just an inquisitive nerd who has had a very fortunate career getting to do many different things. I love video games, computers, programming, hacking, security, DevOps, data, film, photography, sound design, editing -it’s a long list. For the brave and interested, the longer, but still condensed, version...
Migrate Your ASP.NET Framework to ASP.NET Core with Okta
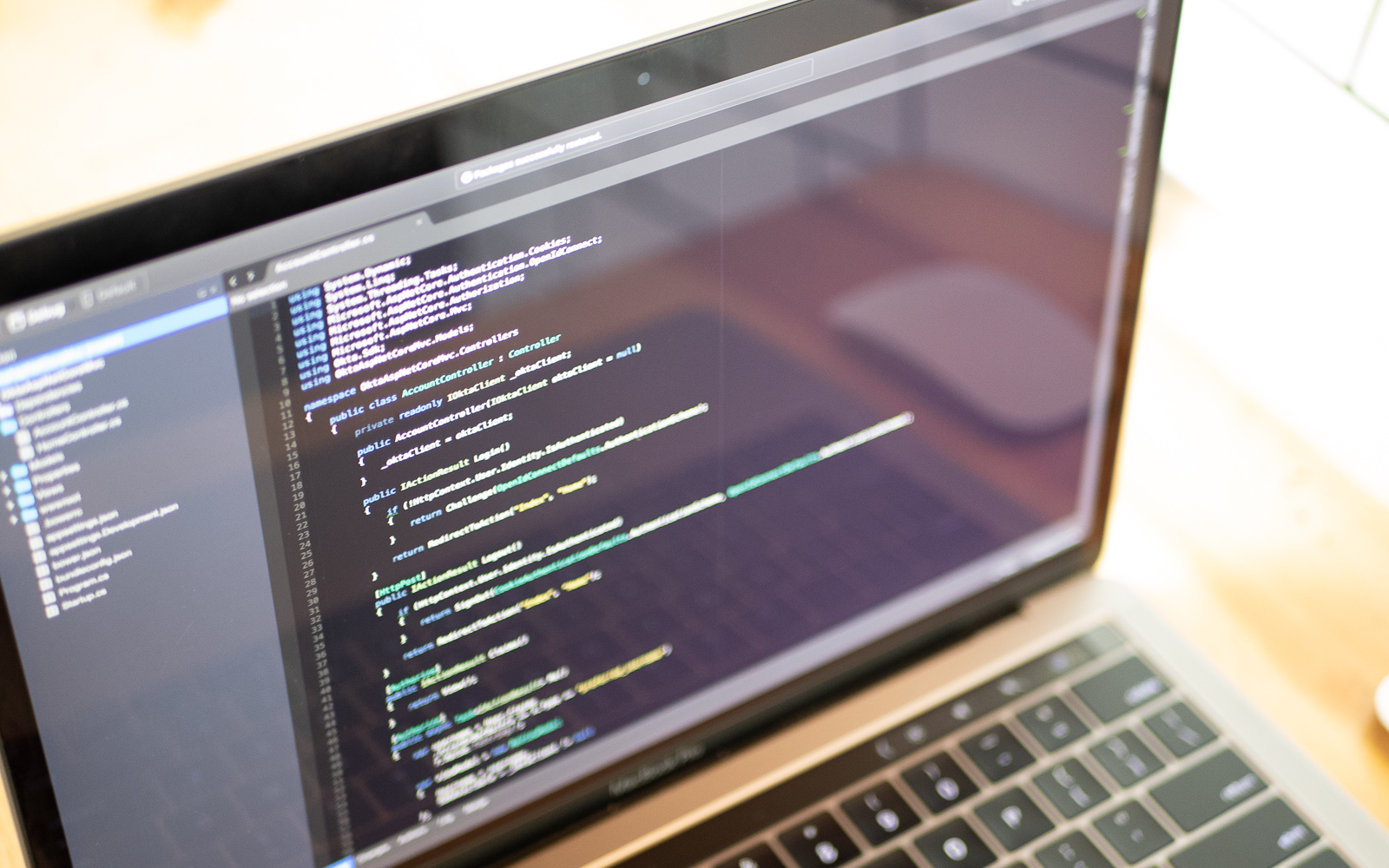
Ah, migration! Let’s say you have an ASP.NET application that has been running fine for years. You have kept up with the various .NET Framework updates and then suddenly you get told that you need to migrate to the latest and greatest, ASP.NET Core using .NET Core. .NET Core is the successor to the .NET Framework we’ve been using for years. It is open-source and supports cross-platform applications. To a veteran .NET developer it should...
10x Your Development with the Azure CLI
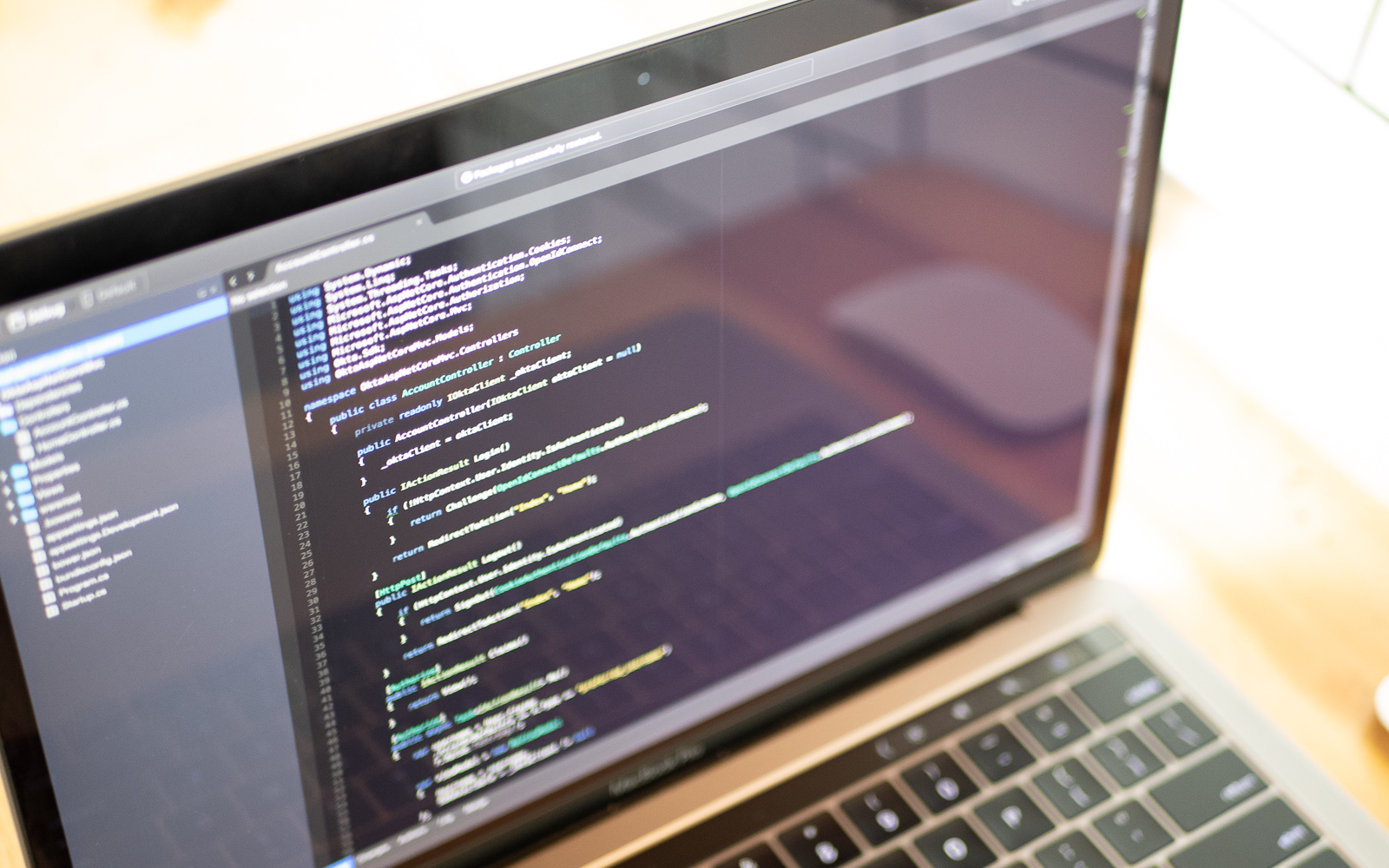
Back in the days of DOS, software developers couldn’t count much on fancy tools. There were no graphical interfaces, and everything was purely text-based. I remember using brief as an editor for my C source files (C++ didn’t exist yet), and compiling the code from the command line with the Aztec C compiler. The most advanced concept of a non-trivial software project was based on makefiles. The idea of grabbing a mouse and moving it...
Super Simple GraphQL Tutorial with C#
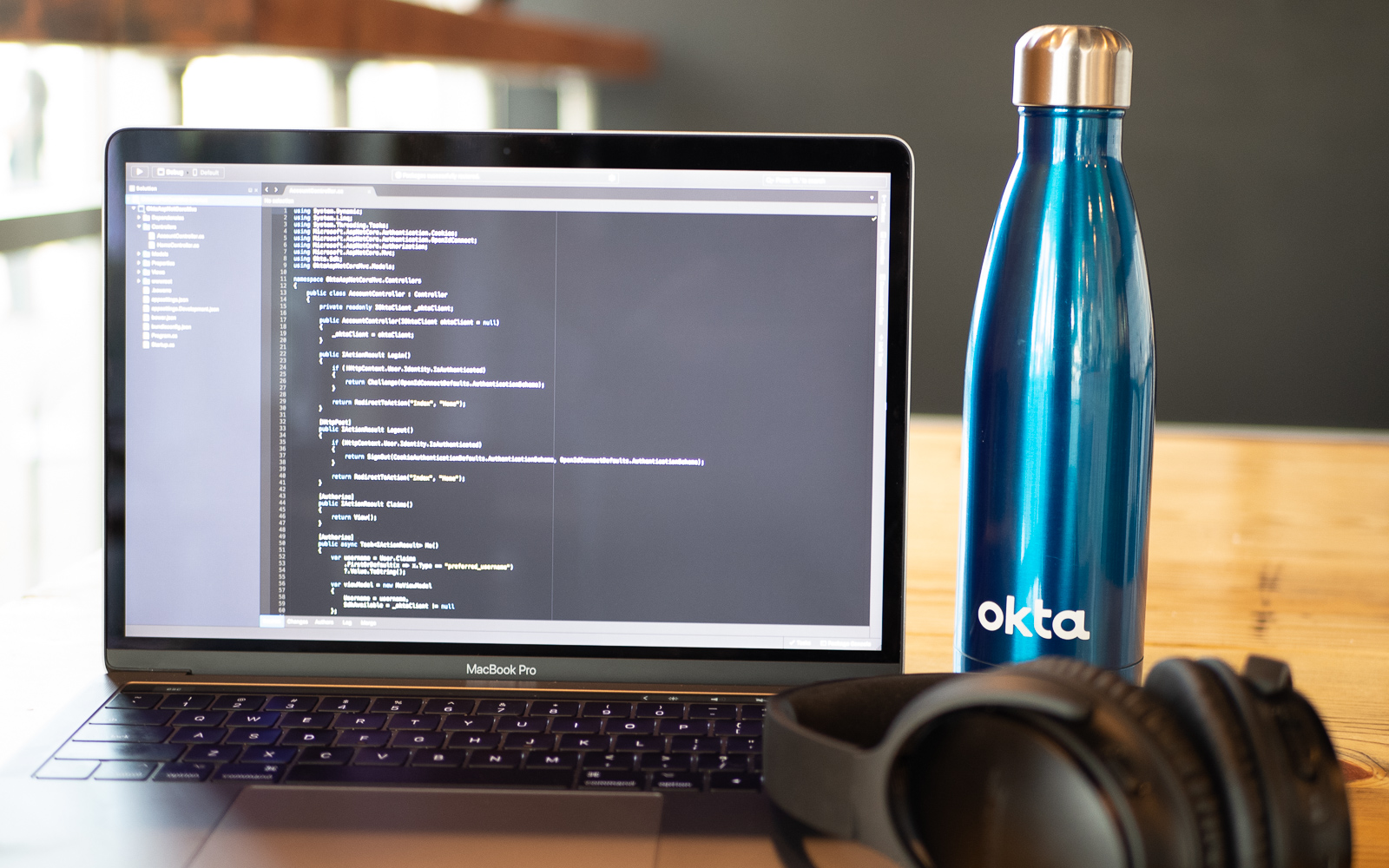
In today’s connected world, APIs are becoming an essential part of software and hardware companies. APIs allow companies to expose their services to third-party developers and integrate with other services. As more and more APIs are built and exposed to the internet, it is essential to secure them from unauthorized access. In this tutorial, you will learn how to build an ASP.NET Core 3.1 Web API using GraphQL and secure it with Okta. ASP.NET Core...
Easy Xamarin Essentials with Web Authenticator
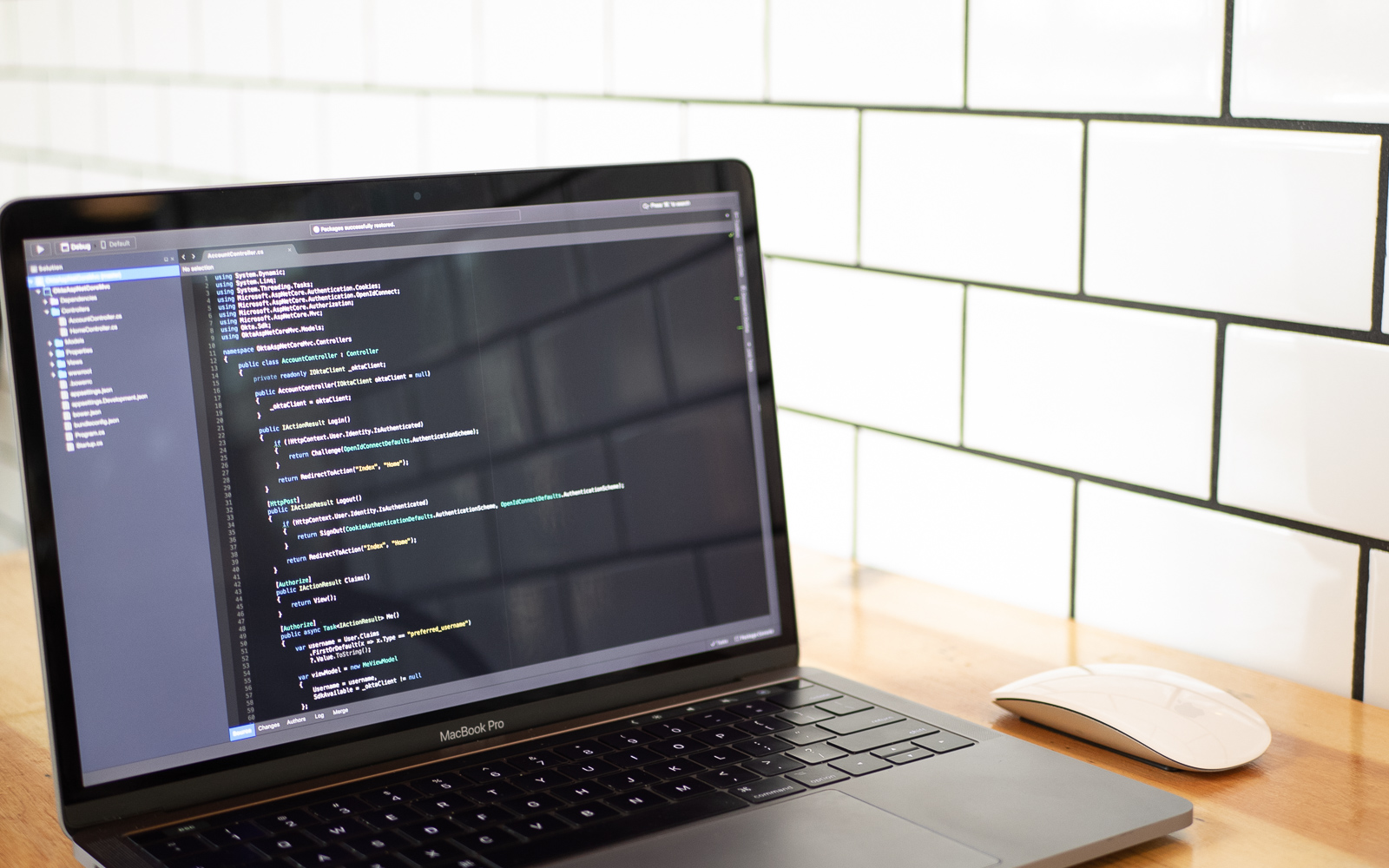
Authentication is an important part of today’s mobile applications, but securely implementing authentication in a mobile app can be a daunting task. In this article, you will learn how to create a basic cross-platform application with Xamarin.Forms and use the WebAuthenticator class from Xamarin.Essentials together with Okta to quickly and easily add user login to your mobile app. To continue you will need: A basic knowledge of C# Visual Studio 2019 An Okta Developer Account...
Build a Basic CRUD App with ASP.NET Core 3.0 and MongoDB
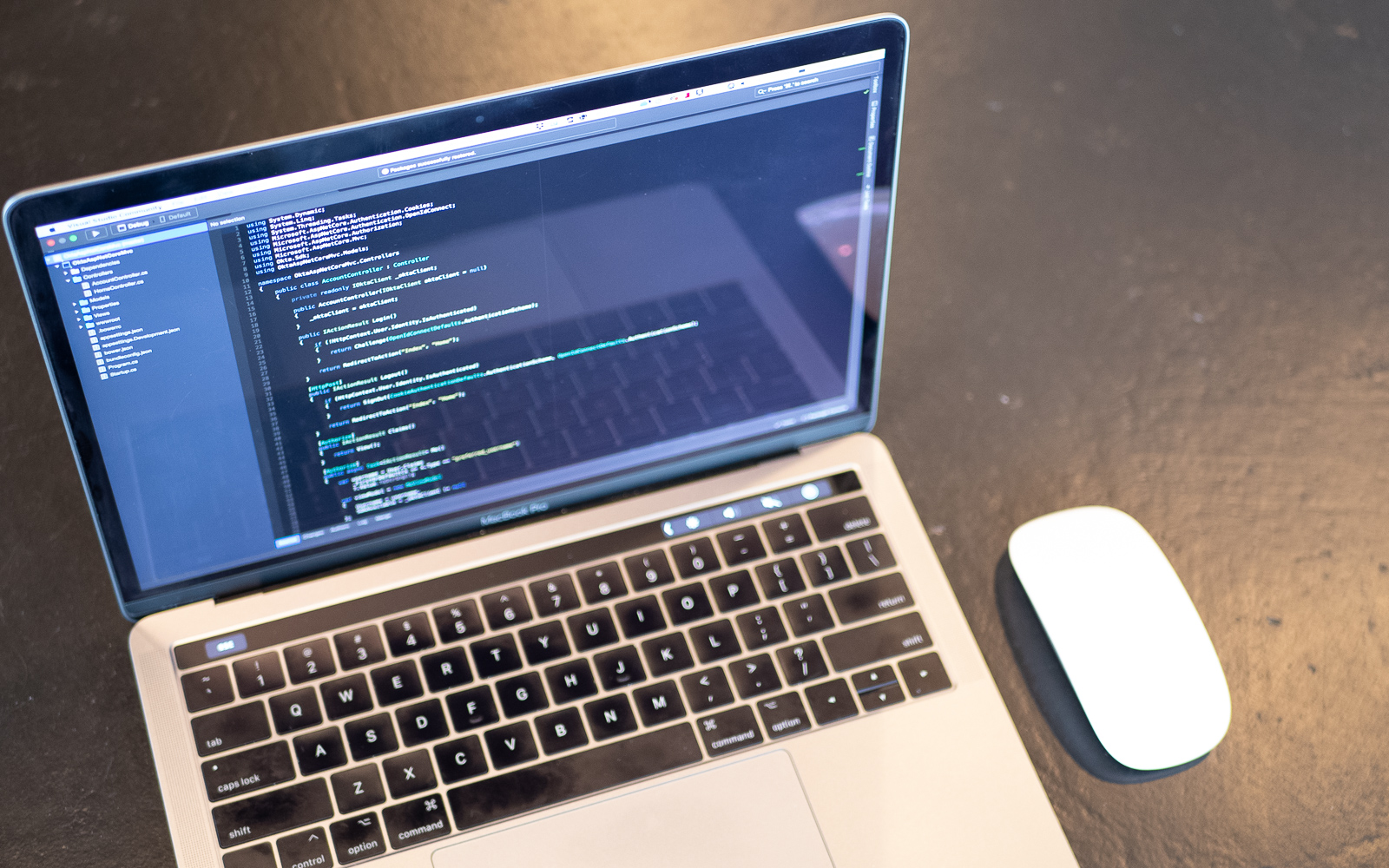
Document databases have become increasingly popular due to their speed and ability to store huge amounts of data or semi-structured data. MongoDB has emerged as a leader in the document database space and, as a consequence, it may be necessary for developers to learn how to interact with MongoDB from .NET applications. This post strips away all the arguments that obscure how document databases can easily serve as a datastore for .NET applications. The app...
Deploy a .NET Container with AWS Fargate
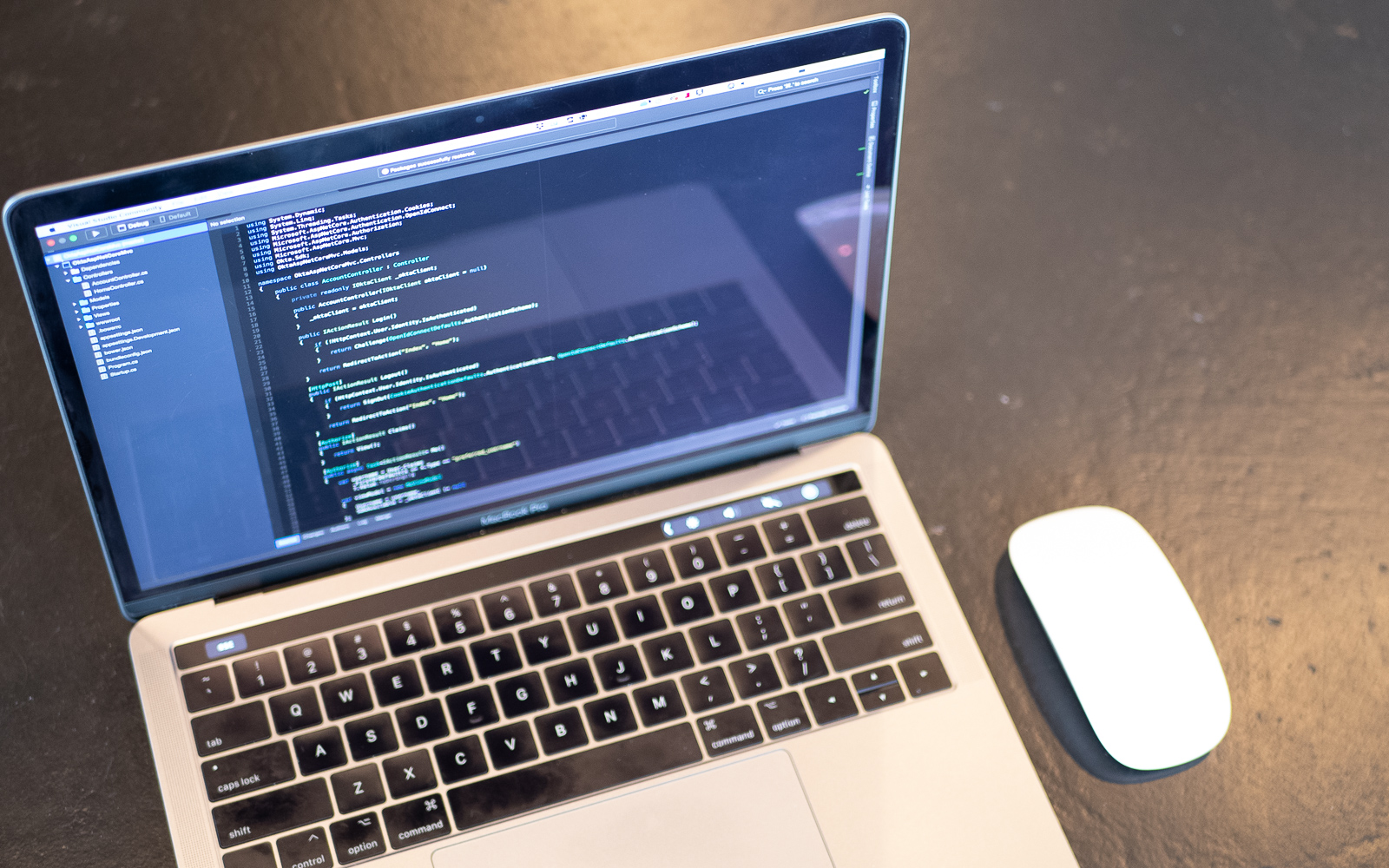
In the last article I wrote, I showed you how to host a serverless .NET application using AWS Lambda. In the article, we talked about the history of serverless and how companies are using these types of technology to simplify the process of delivering APIs and functionality faster than traditional methods. There are some problems that will start to arise in this type of application when you need more capability than your standard HTTP protocols...
How to Authenticate an AWS Lambda Function in C#
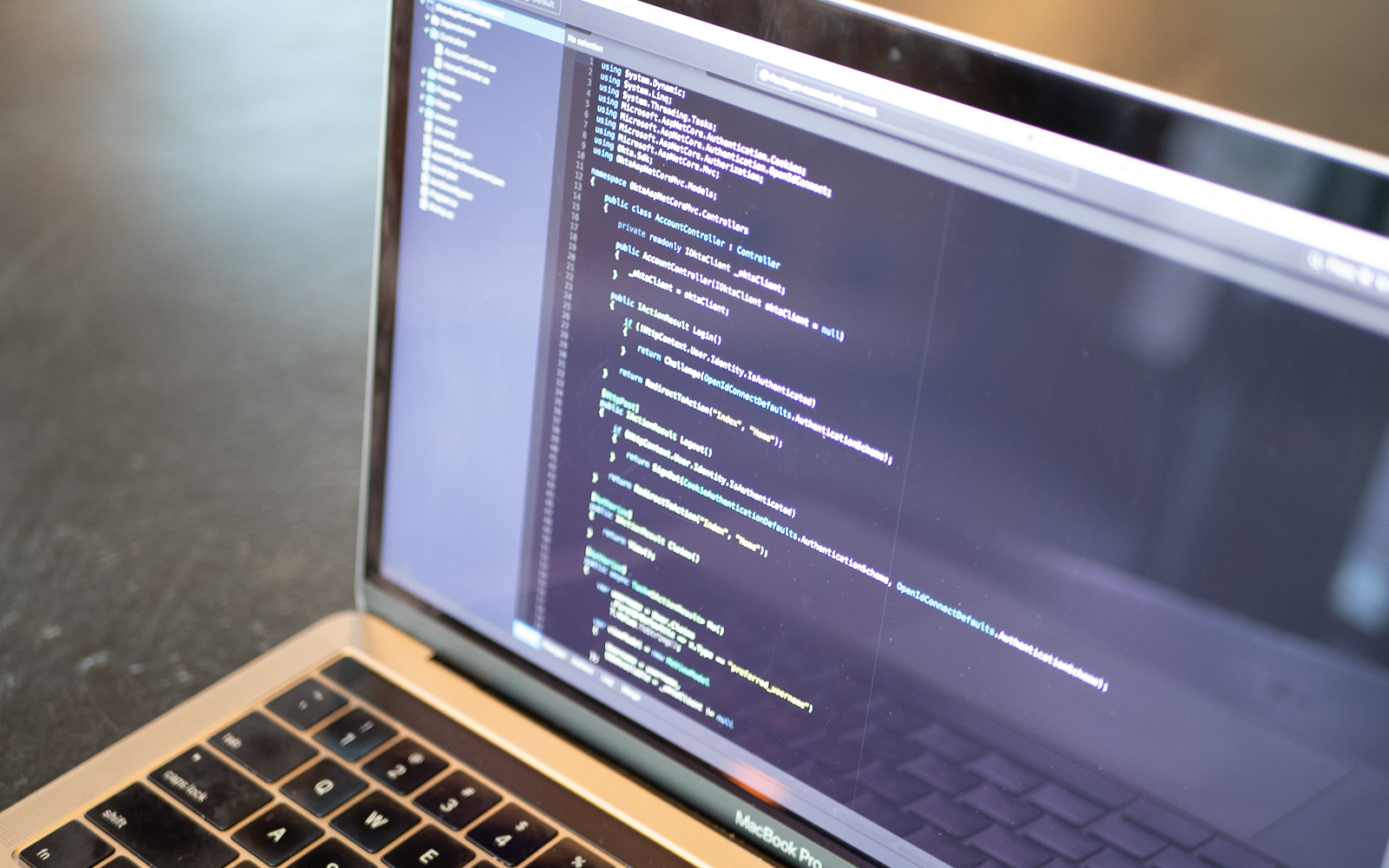
Serverless was only first coined as a concept 8 years ago in 2012 by Ken Fromm in The future of the world is serverless. Even with the rise of cloud computing, the world still revolves around servers. That won’t last, though. Cloud apps are moving into a serverless world, and that will bring big implications for the creation and distribution of software and applications. If you come from traditional service architecture roots of on-premises or...
How to Master the Filestream in C#
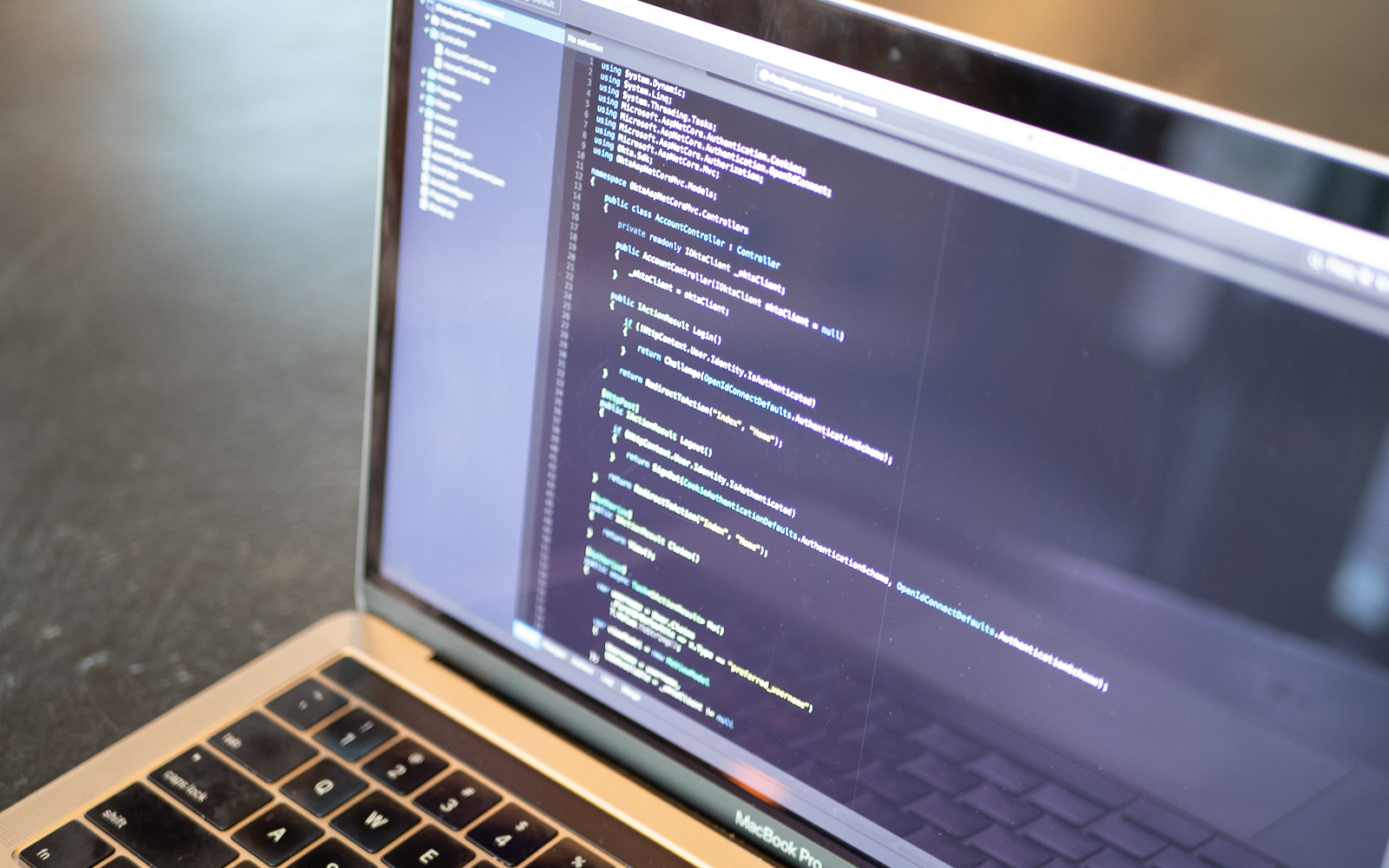
We live in a world that moves fast. Compared to the mid 90s through early 2000s, we have incredibly intelligent technology. Effectively, we have super-computers in our pockets. Our actual, modern supercomputers would have seemed like works of fiction just two decades ago. Not only is our ability to compute fast, but so is our data - with cellular 4G averaging 18.1 Mbps and 5G coming in at an average of 111.8 Mbps, at the...
The Most Exciting Promise of .NET 5
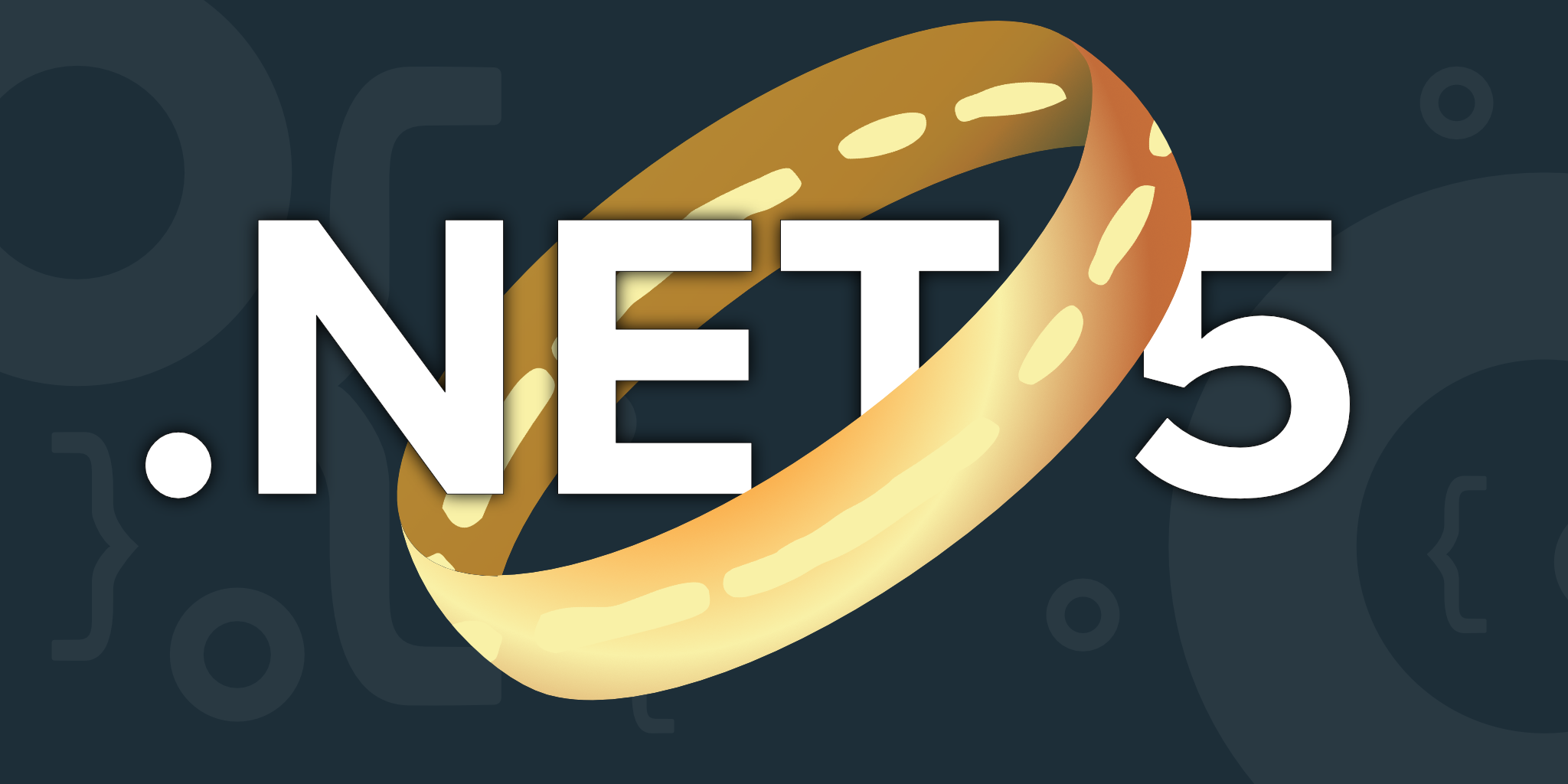
It’s time to get jazzed about the future of the .NET ecosystem! It’s hard to believe that .NET only came out in 2002. There are so many versions of the framework, rapidly changing the places a C# developer could create and support. The entire ecosystem just turned 18, and it has been confusing at times with versioning. I fell in love with how powerful the platform was, but often, choosing to upgrade to the latest...
Build an Incredibly Fast Website with Dapper + C#
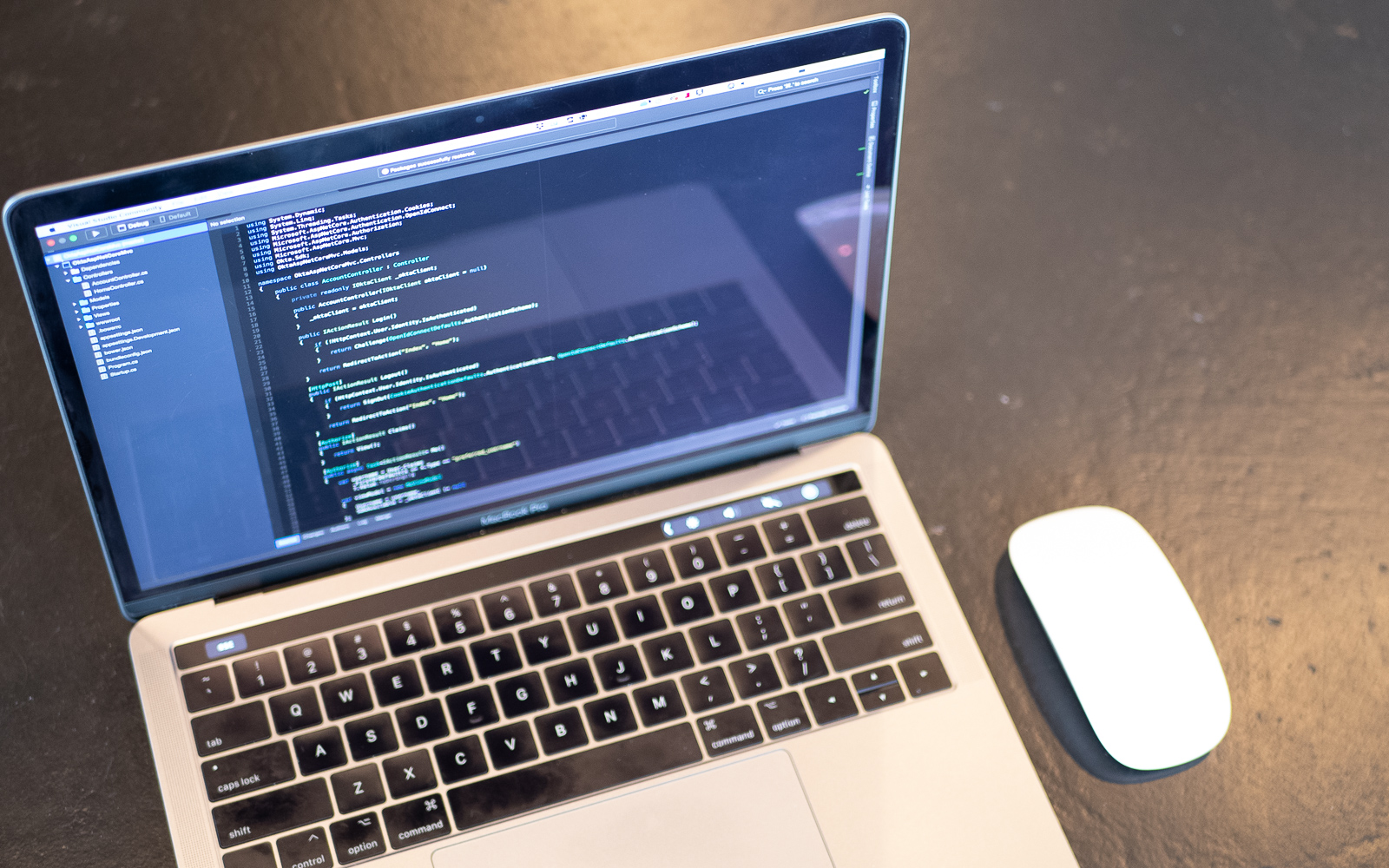
If you have been doing .NET development professionally for any length of time, you are probably familiar with Entity Framework for data access. At the time of this post, both Entity Framework and Entity Framework Core have over 85 million downloads on Nuget so it is definitely a popular framework. People love Entity Framework because it abstracts the way you interact with the database to make development easier. The problem, though, is that you trade...
Baking in Security with .NET CLI Templates
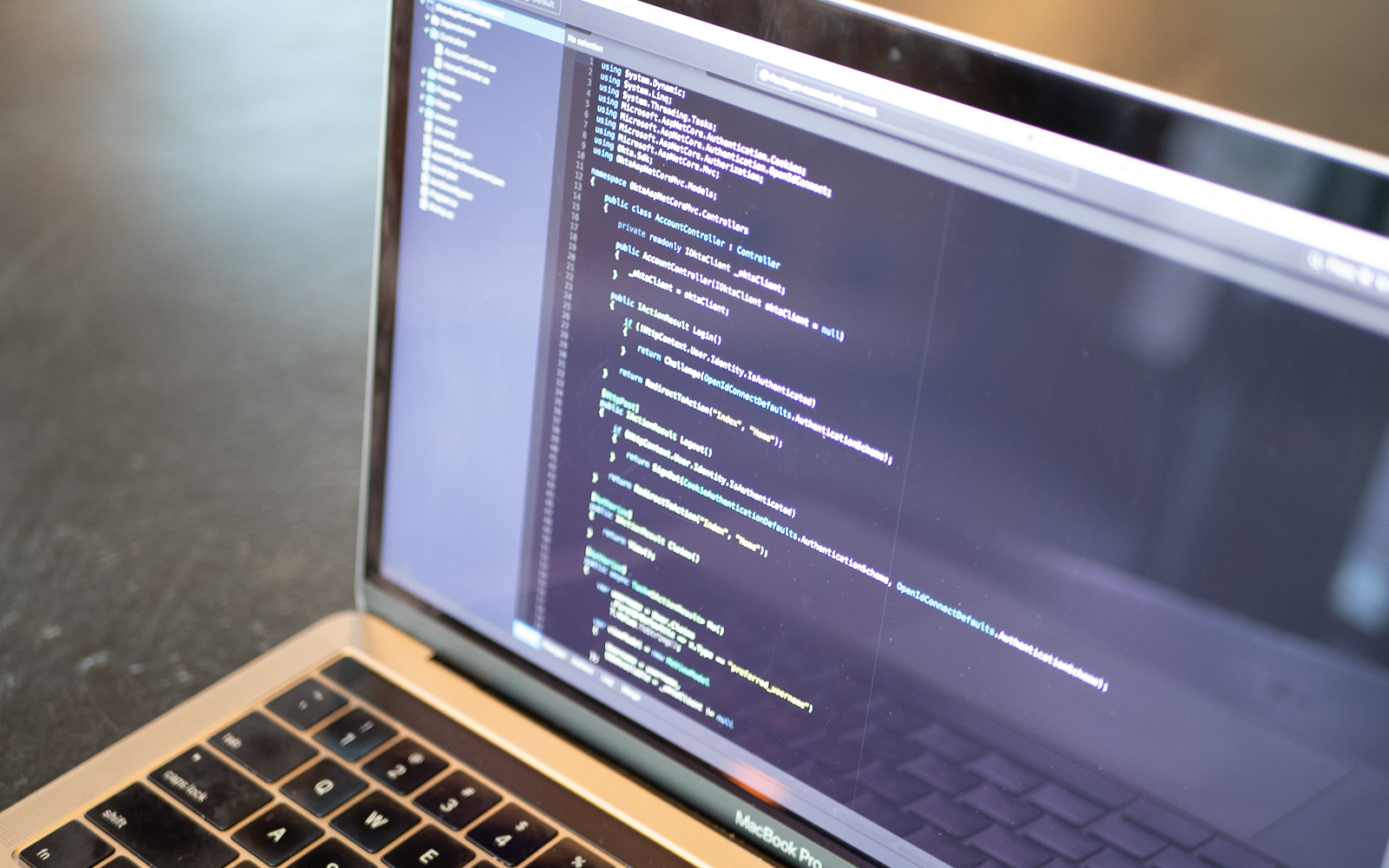
Let’s face it: developing good security is hard, unglamorous, and time-intensive. People tend to think about it as an afterthought instead of a priority. What if there were a way to make all your new projects more secure out of the box, and also make your company’s development processes easier and more repeatable in the process? Good news everyone, I have just the thing: .NET templates. You are going to love how much time and...
How I Learned to Love Default Implementations in C# 8.0
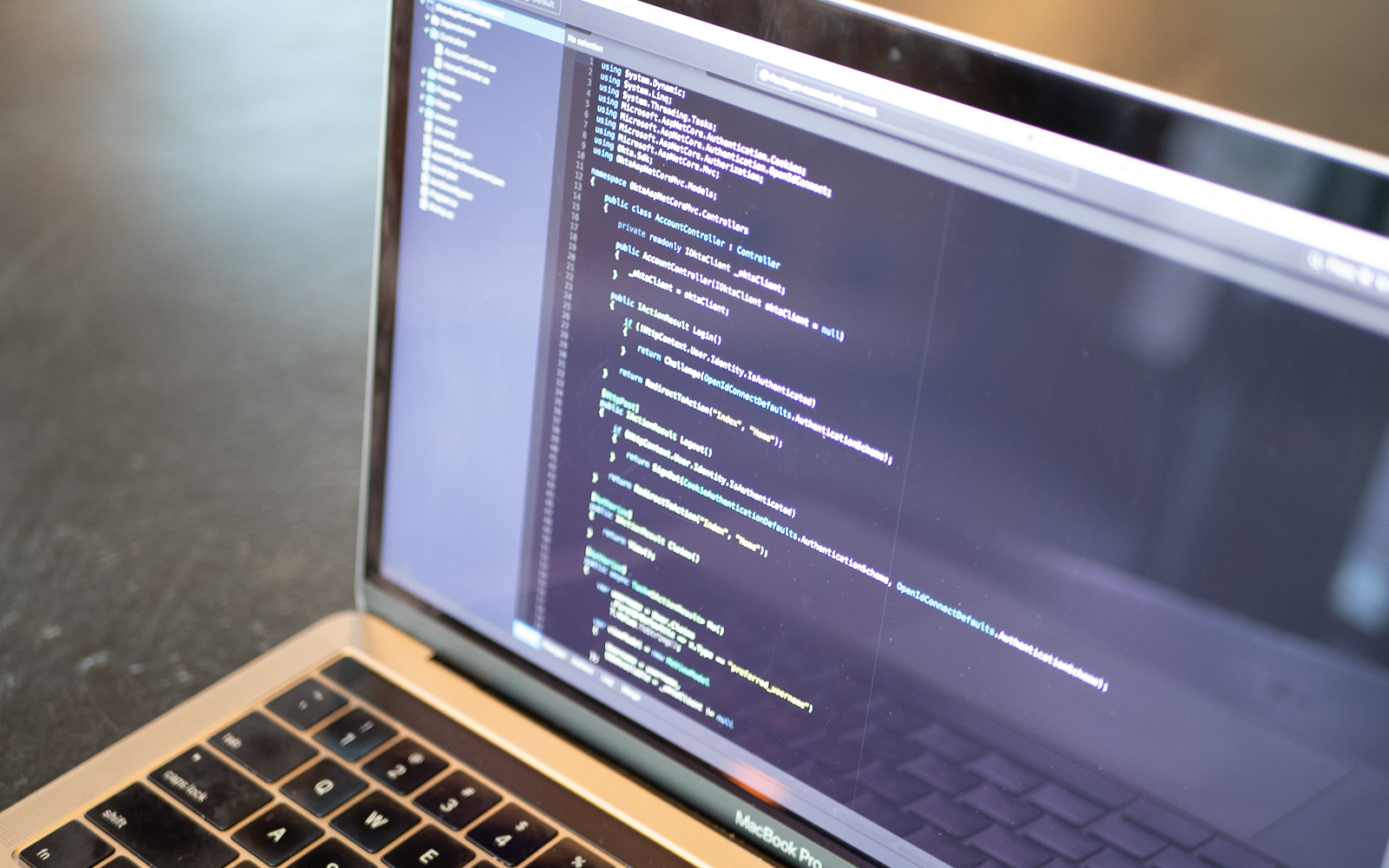
If you haven’t heard, C# 8.0 ships with a new feature that allows you to add default implementations to interfaces. If you’re like me, you may be thinking, “Why? Why would I want to add implementations to interfaces? Isn’t that what abstract classes are for? Doesn’t that go against everything that interfaces stand for?” My immediate reaction to this new feature was visceral and negative,, but I decided to investigate closer and… I think I...
Build a .NET App Quickly with Docker
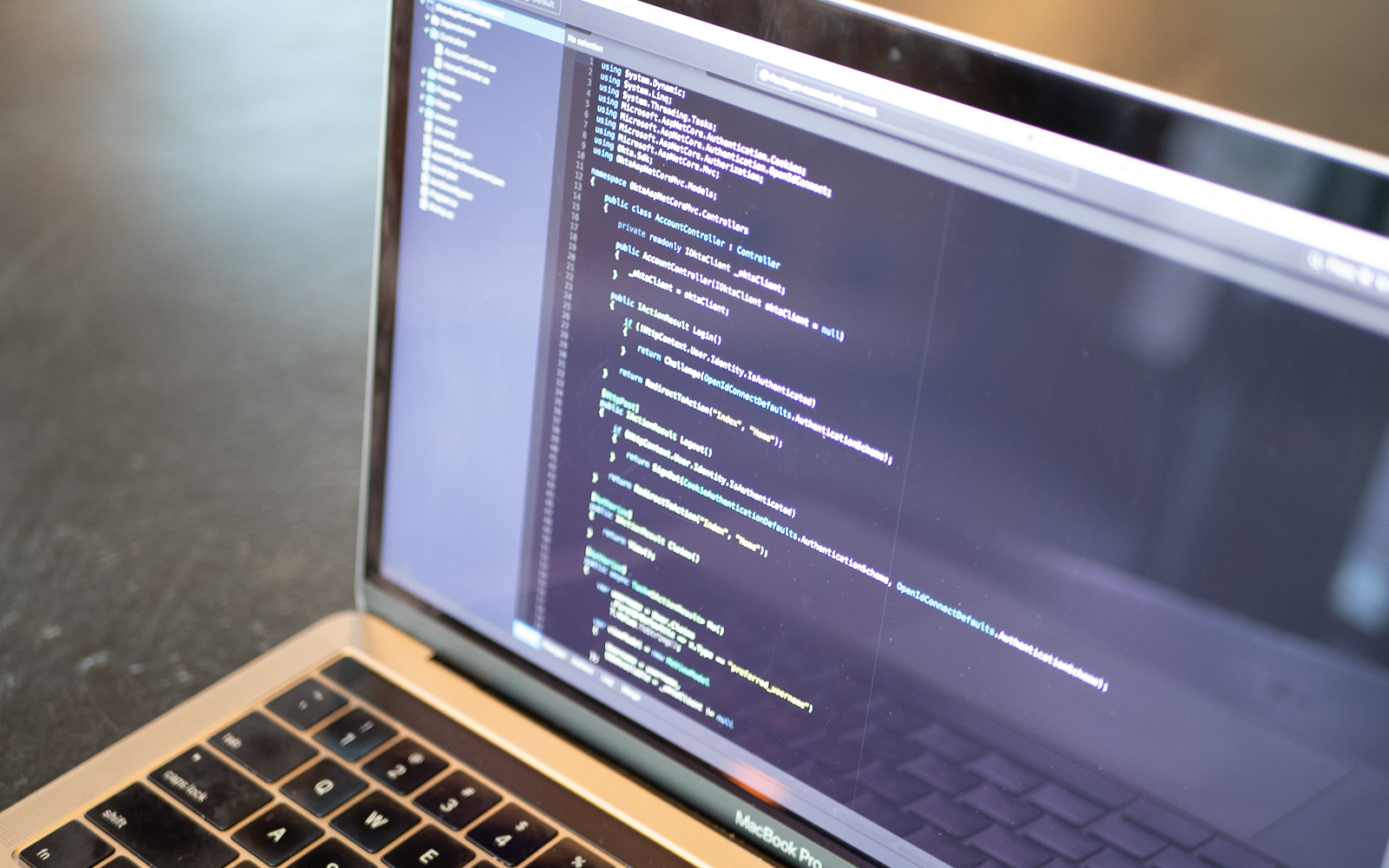
In this post, you’ll build a .Net Framework application with Visual Studio on Windows 10. You’ll then containerize your application so it can be reliably deployed and run on any instance of Docker for Windows. Since containers share the host operating system, you can only run containers compatible with your host operating system kernel. For Linux based applications, practically all versions of Linux share the same kernel, so as long as you’ve installed Docker, you’re...
Build a Simple .NET Core App on Docker
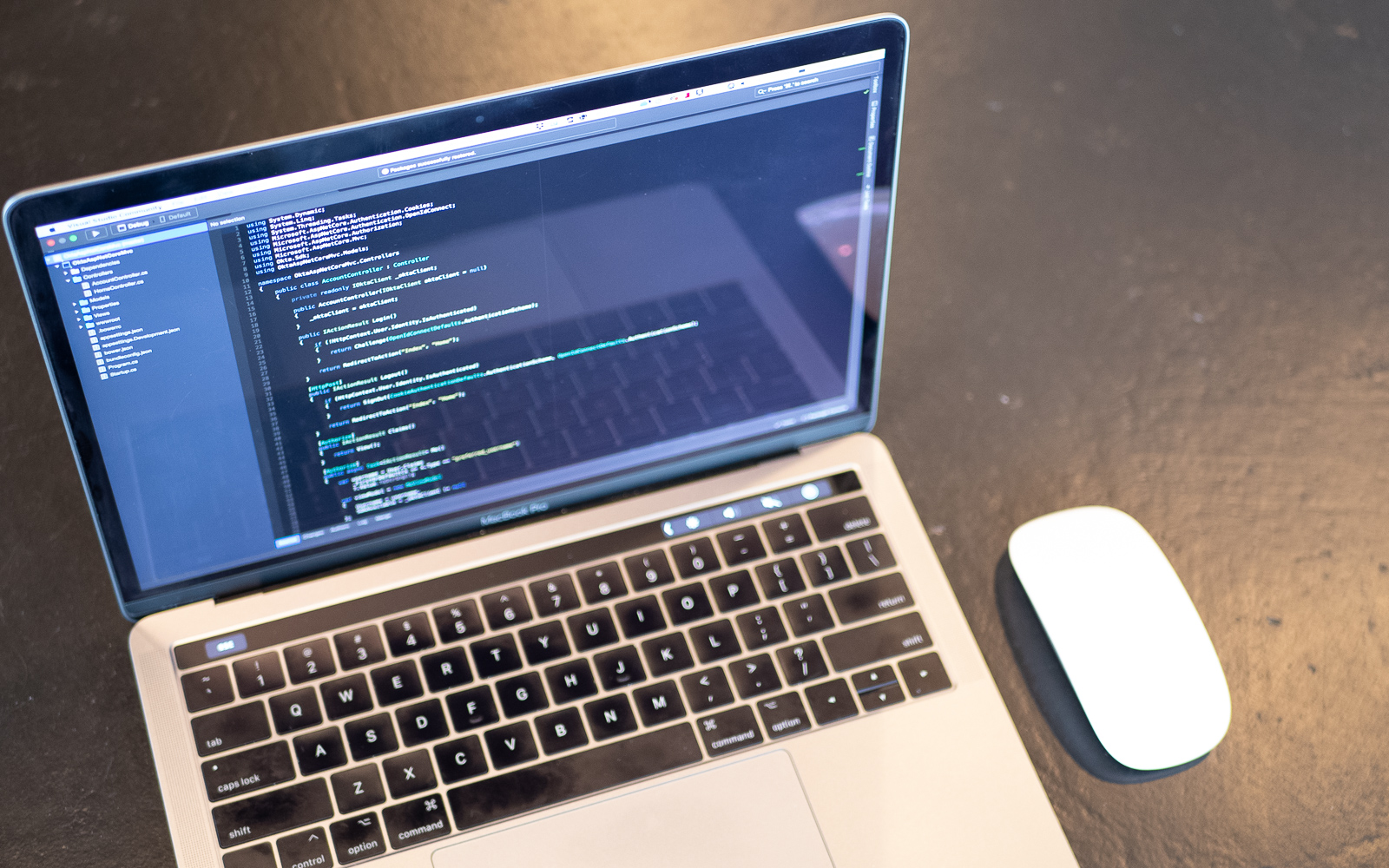
Wouldn’t it be great if stuff just worked? Especially in the ever-changing world of software. Chasing dependency issues and debugging arcane operating system errors - not a good use of time. One important aspect of “stuff just works” – reliability. Recently, the software community has made strides in test-driven development and continuous integration processes to drive up quality, and of course, that improves reliability. But it can only go so far. Operating systems perform many...
5 Reasons Why You Should Give Visual Studio for Mac Another Try
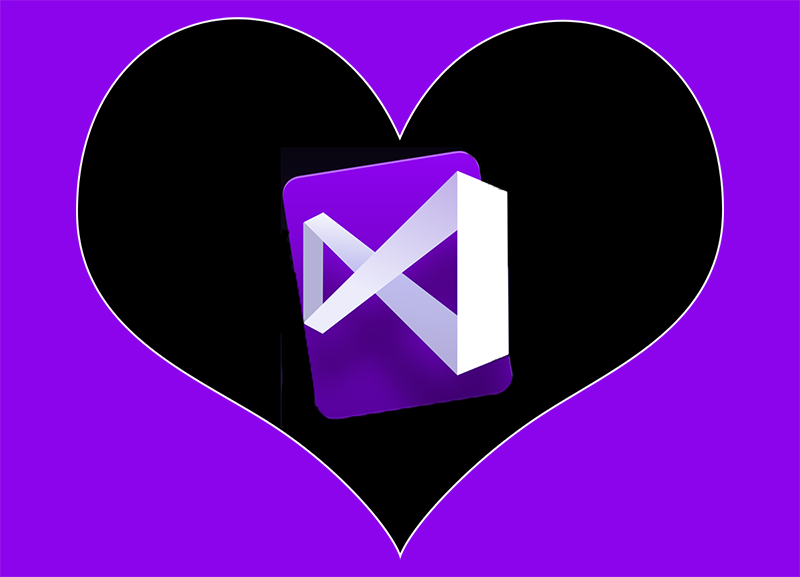
Visual Studio has not always been as user-friendly on the Mac as it is on a Windows machine. Lately, however, the stable release of VS for Mac is really starting to feel like a simple, but luxurious cousin to Visual Studio 2019. Different, but related. Installation on a Mac is quick, simple, and allows you to get into coding right away - whether you are already familiar or an Apple-only dev getting into something new...
Build a Simple CRUD App with ASP.NET Core, MySQL, and Twilio
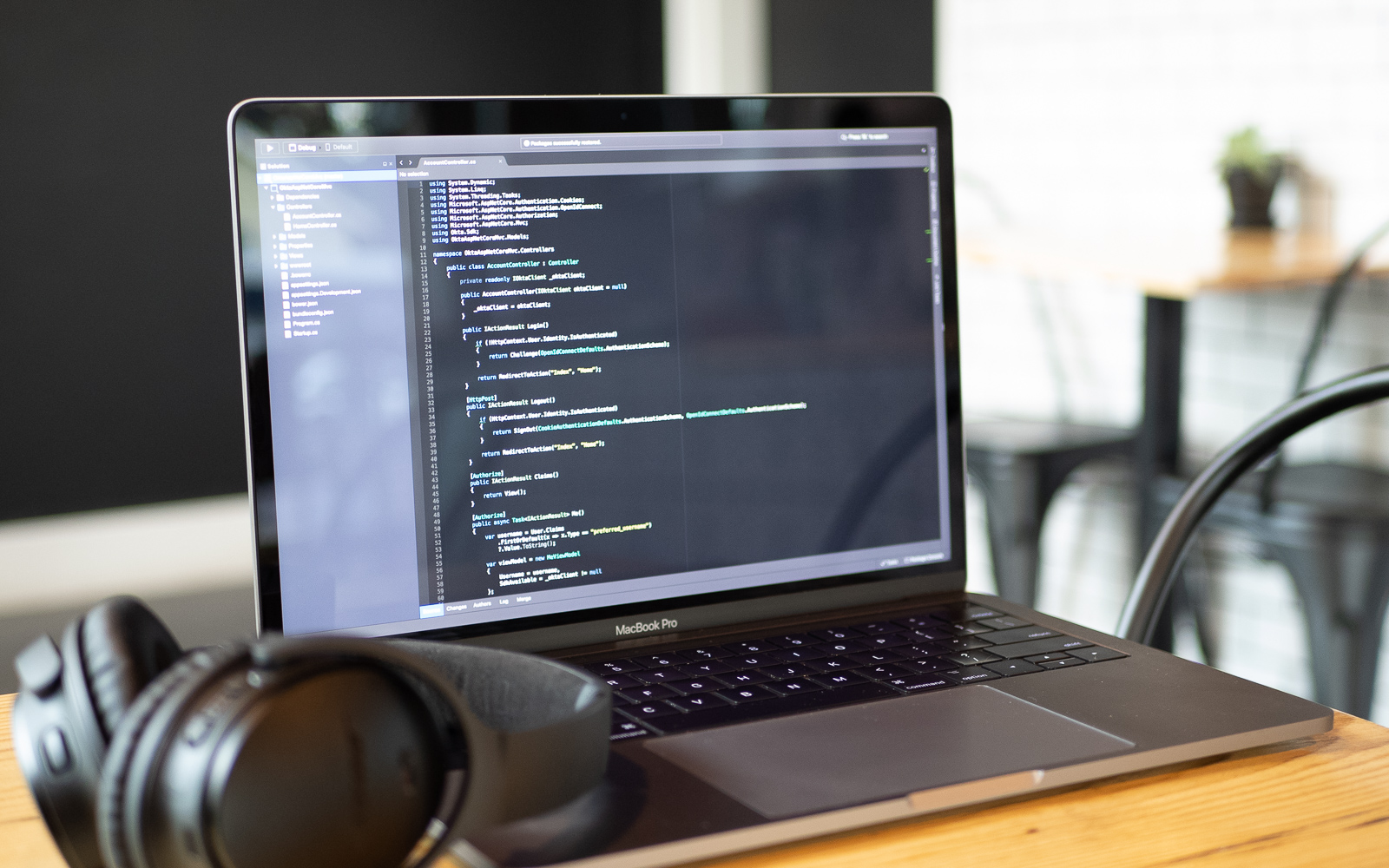
In this article, we’ll be building an MVC task management form with .NET Core 2.2. We’ll also be showing how to integrate with a MySQL database from MVC, and how to use Twilio to send SMS messages to your app that will add new tasks to your list. .NET Core and MySQL are both free and open source technologies. The new ASP.NET Core can run on Linux and in Linux Containers, and MySQL is one...
Use Azure Cosmos DB with Your ASP.NET App
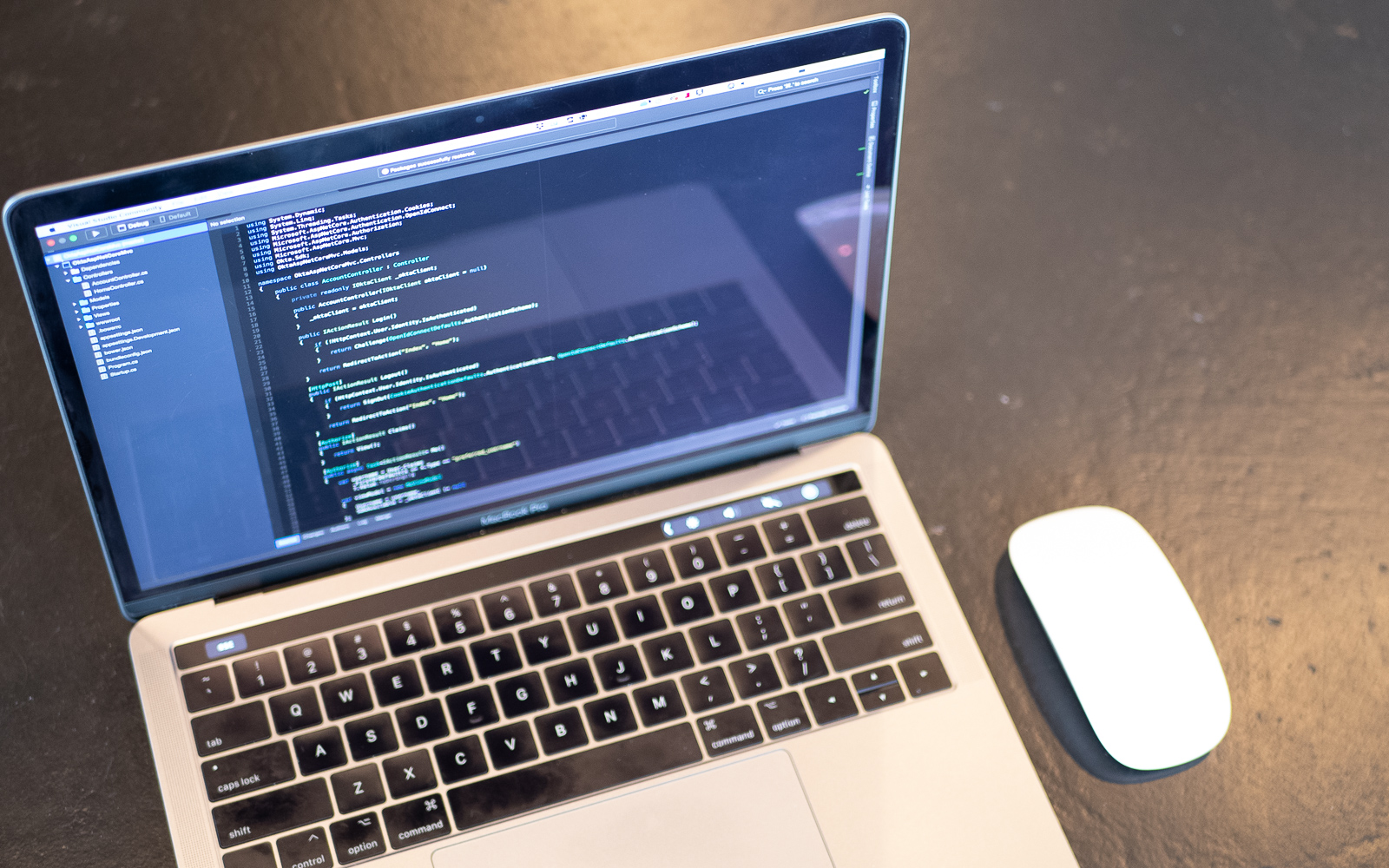
Cosmos DB is a planet-scale database capable of holding billions of records (“documents” using Cosmos jargon) without significant detriment to performance. In this article, you will learn how to use Azure Cosmos DB - a key-value store solution - your ASP.NET app instead of a relational database. To demonstrate their differences, check out a simple example query using both a relational database and Cosmos DB. When you query a relational database you get back a...
The Top 5 DevOps Automation Tools .NET Developers Should Know
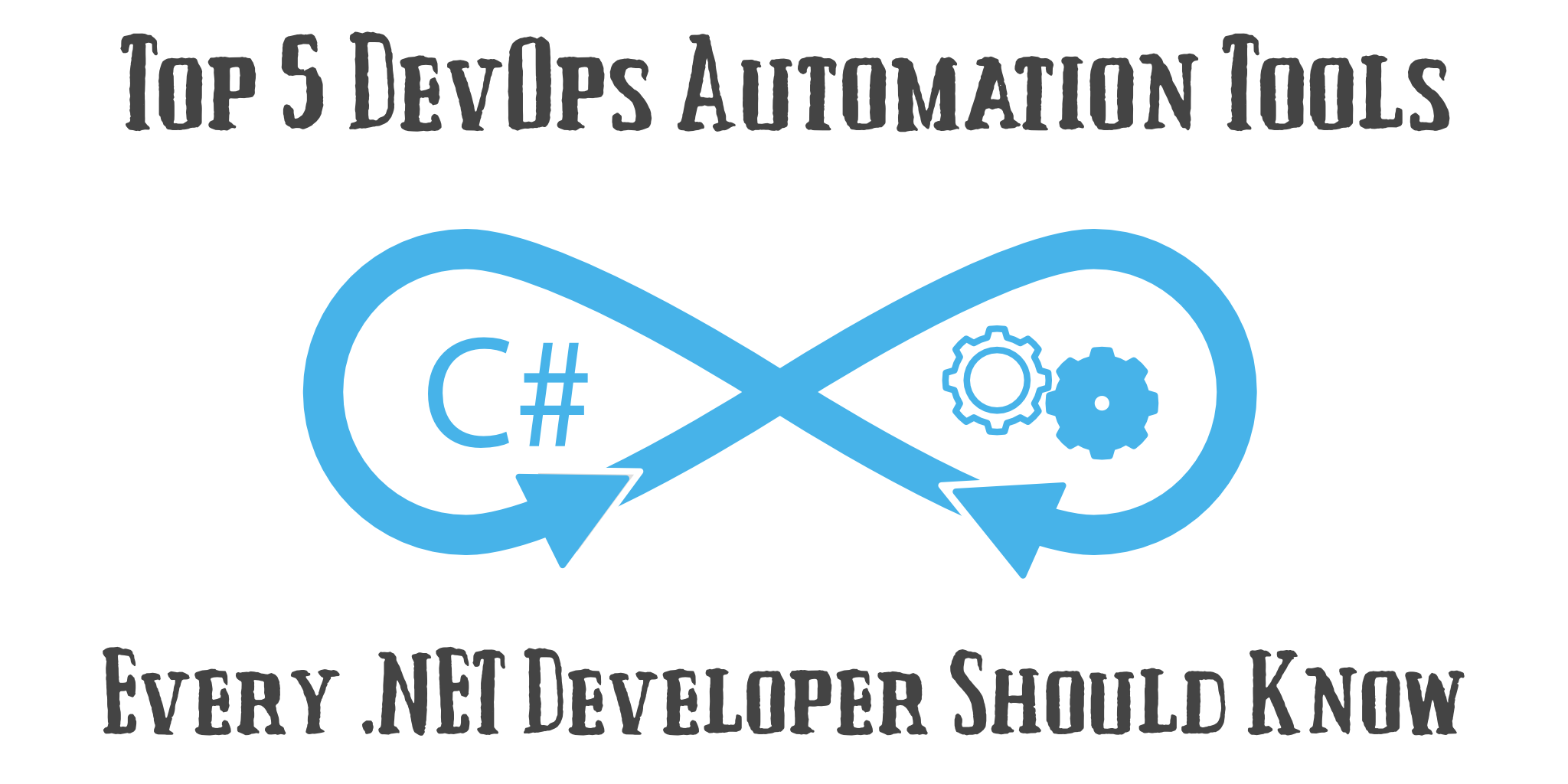
Not too long ago, deployments were done by hand - manually pushed to a physical server somewhere in a building your company owned. The software engineering world has come a long way since then, and we have more options than ever get our code and data live, automatically. Here are a few tools every C#/.NET developer should know that can help with that process, vetted by some of the best software leaders in the business....
Alexa, Sign In Every Time: Voice-Only Authentication Verification in .NET
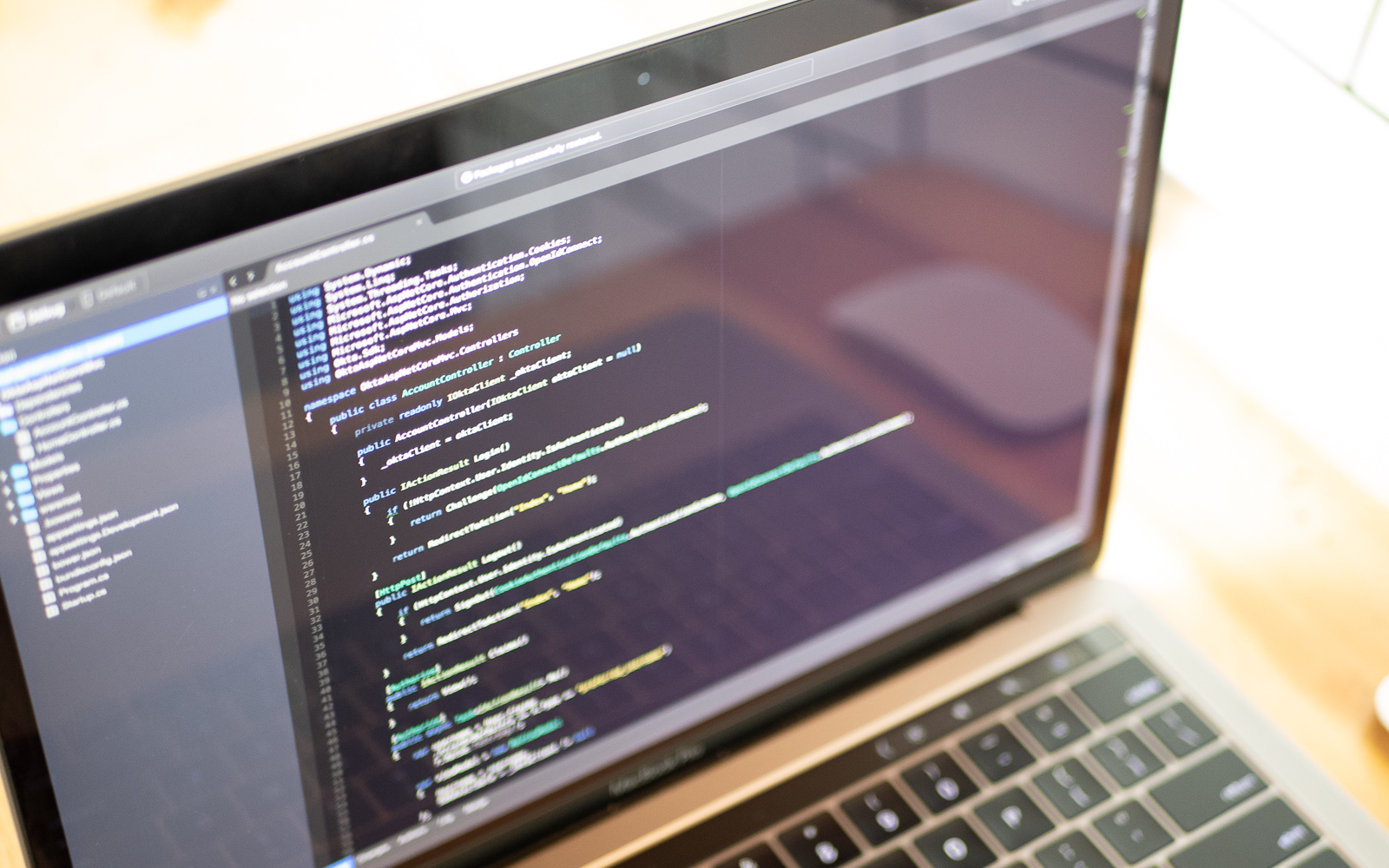
Authentication for voice, specifically Amazon’s Alexa, has its limitations. The initial Account Linking process Amazon provides for identification works for many scenarios, but it is only done once (signing into the skill via the Alexa mobile app or website), and all subsequent sessions with the skill are linked - no log-in screen presented again. I’ve often been asked what the options are for verifying every time a user engages with an Alexa skill. For example;...
AWS Lambda vs Azure Functions for C# Serverless
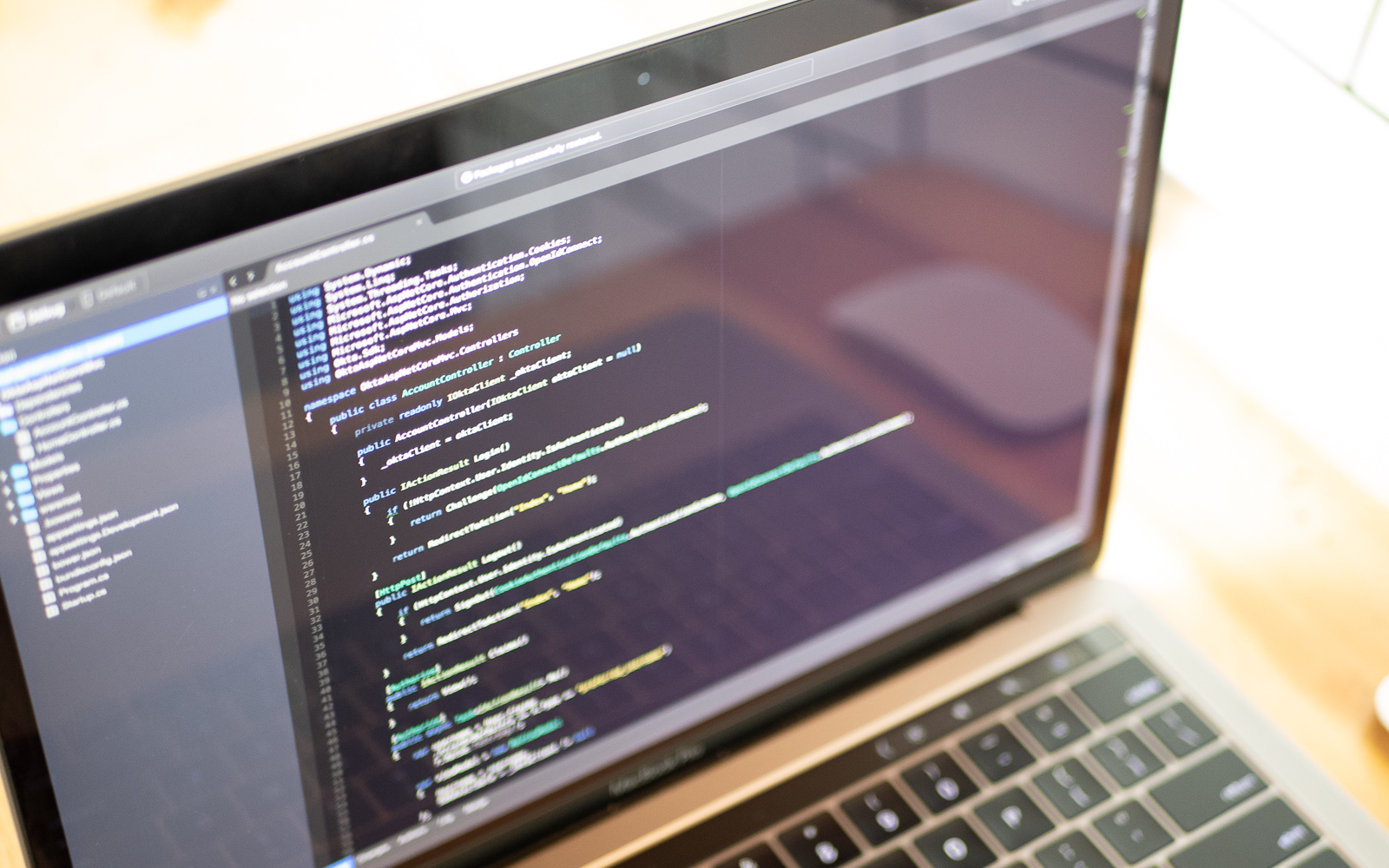
As a C# developer, I became interested in how using a serverless function could complement existing projects I had done in ASP.NET 4.x. Enhancing ecosystems by using it for new requirements - without starting over from scratch - really had appeal. AWS Lambda came along first, with Azure Functions emerging onto the scene a couple of years later. In this post, we will briefly examine my experience getting started on both after using the .NET...
Use Firebase with Your ASP.NET MVC App
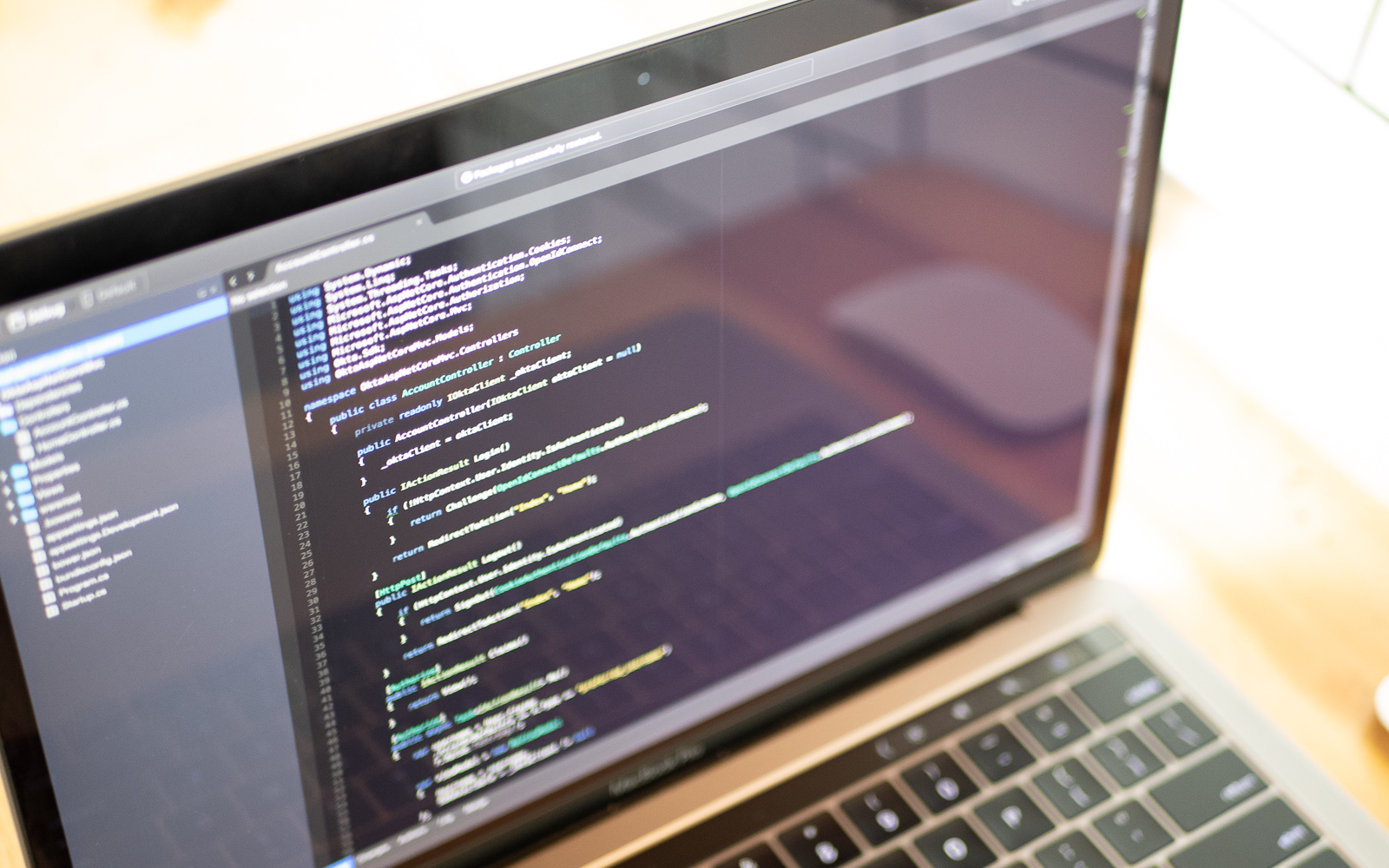
Working with databases hosted online has become easier over recent years. The emergence of Database as a Service (DaaS) specifically makes quick integrations much easier. It is important to keep application user data separate from personally identifiable information, especially in this day and age. When using a third party auth provider like Okta, user information like a name or email address can be stored by that provider directly in their system, benefitting from their oversight...
User Account Linking in Alexa with ASP.NET Web API
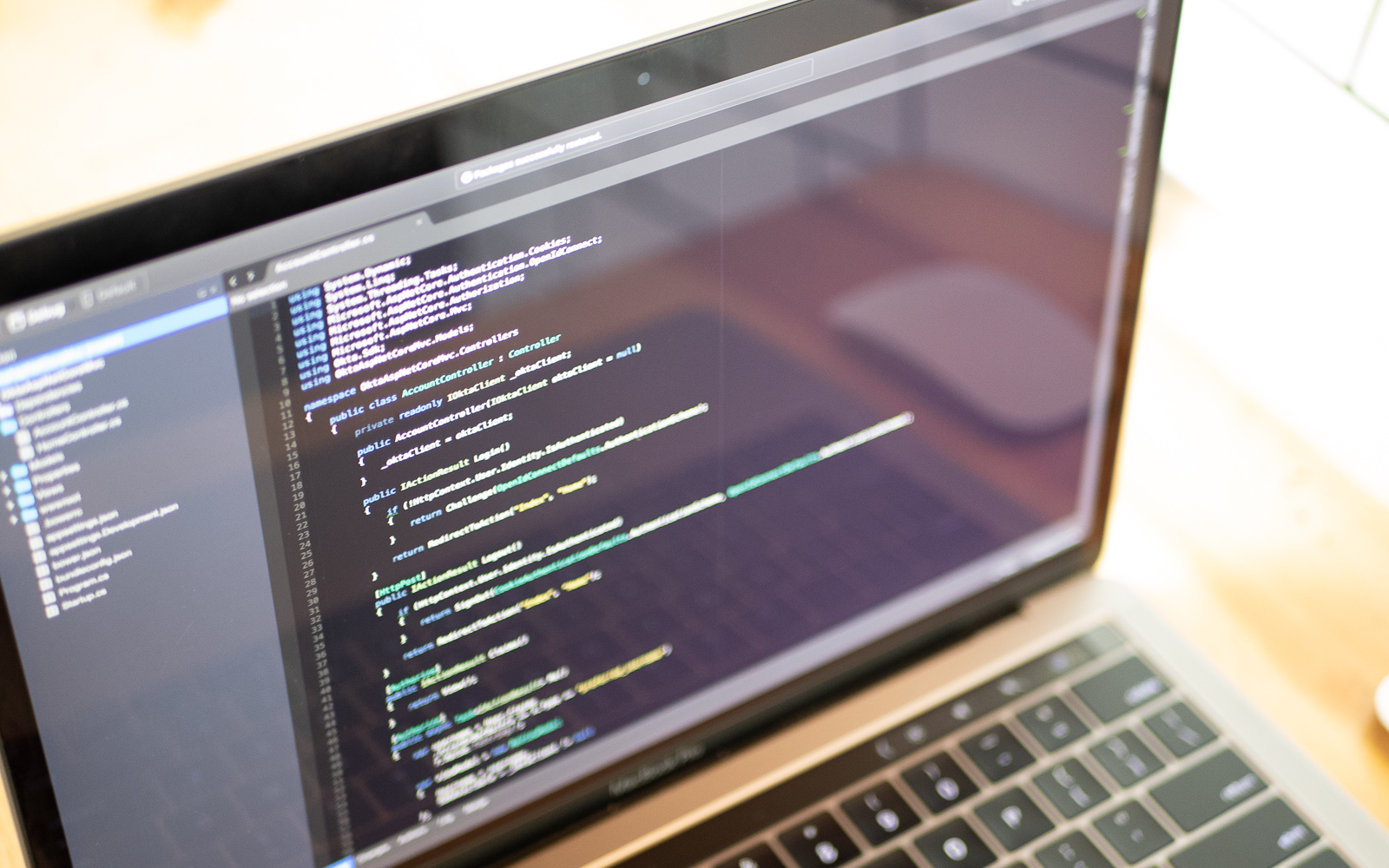
REST APIs are often written for enterprise-level companies as a way of allowing a client-side application to access core business logic. For many companies, simply extending their existing API by adding an endpoint for Alexa integration is the best way to manage that flow. For this example, you will use an existing Web API project on ASP.NET 4.7 and demonstrate how to link existing users to the Alexa skill the first time they access it,...
Build a CRUD App with ASP.NET Core and TypeScript
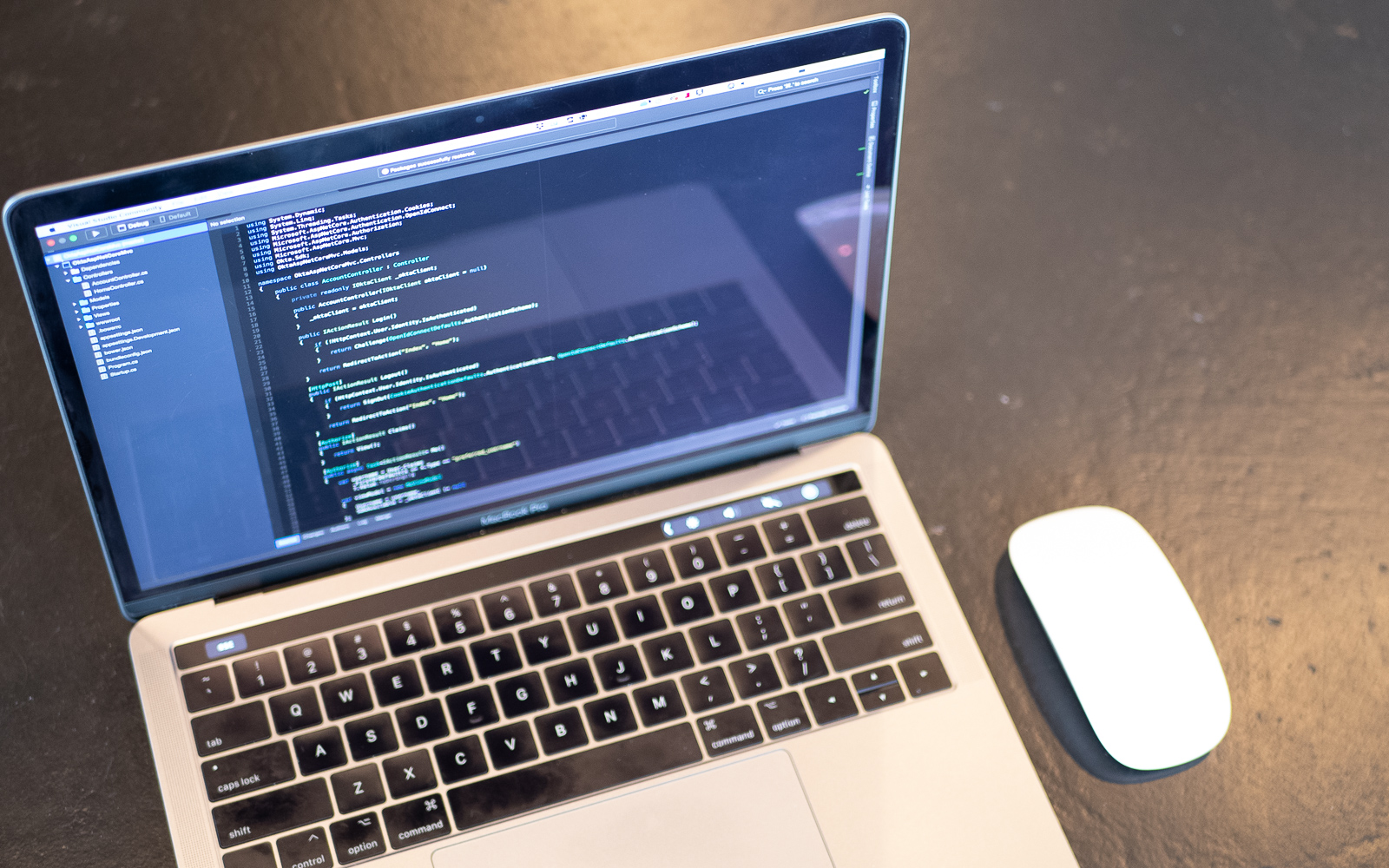
There are a lot of things for .NET developers to love about TypeScript. It has strong typing that .NET Developers are used to and the ability to use the latest JavaScript features. Since it is just a superset of JavaScript, the cost to switch is almost nothing. Getting Visual Studio to transpile the TypeScript when it builds your ASP.NET Core app is pretty simple as well. In this tutorial, you will build an ASP.NET Core...
Visual Studio 2019 Tips and Tricks
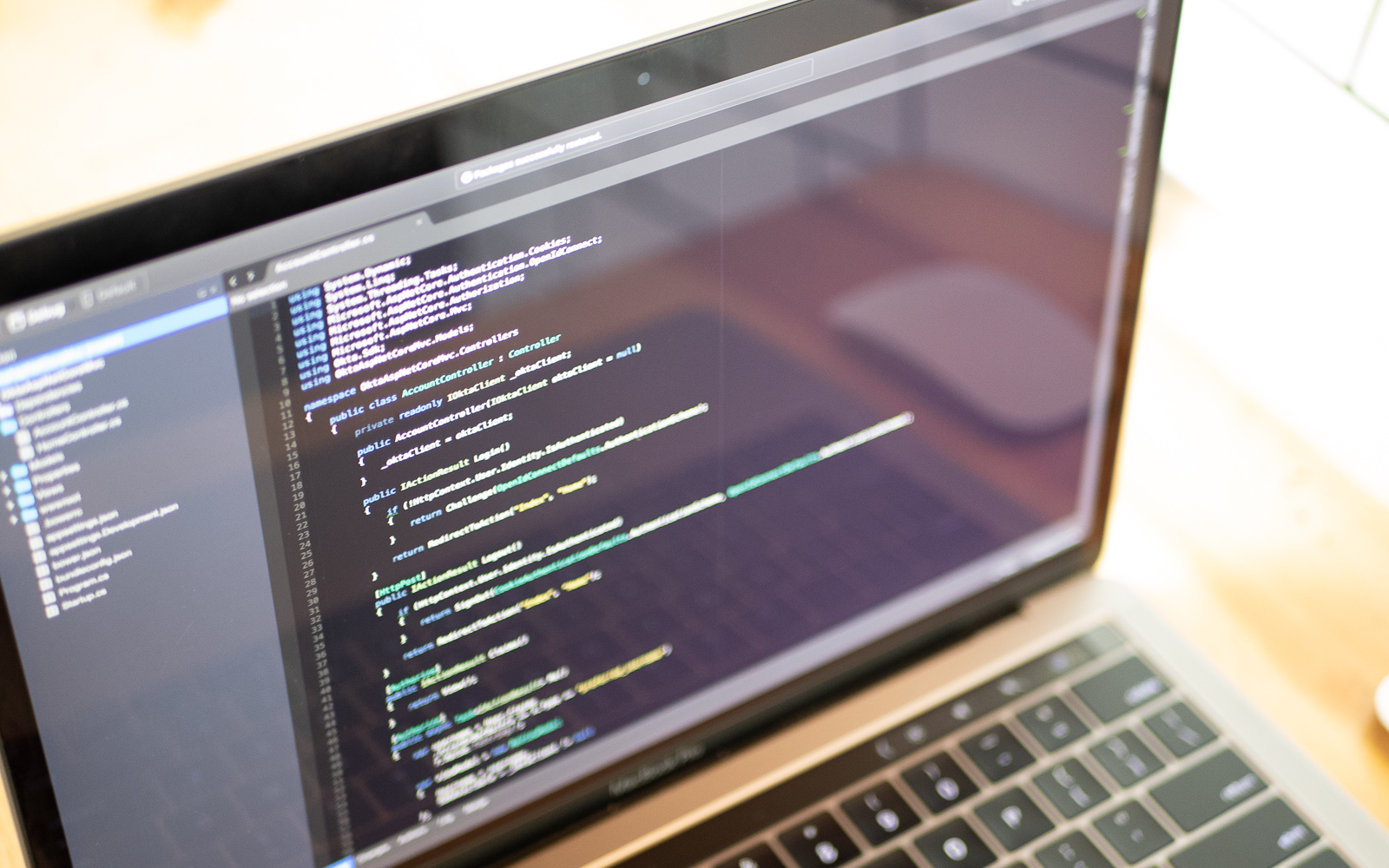
Microsoft recently released the preview of Visual Studio 2019 for Windows, and it’s got lots of improvements and features! After reading the release notes, I reached out to Allison Buchholtz-Au and Kendra Havens on the Visual Studio team at Microsoft to get an idea of what features should be configured immediately after downloading and installing this shiny new IDE. After they showed me a couple of quick tips, VS 2019 started to feel like the...
Build Secure Microservices with AWS Lambda and ASP.NET Core
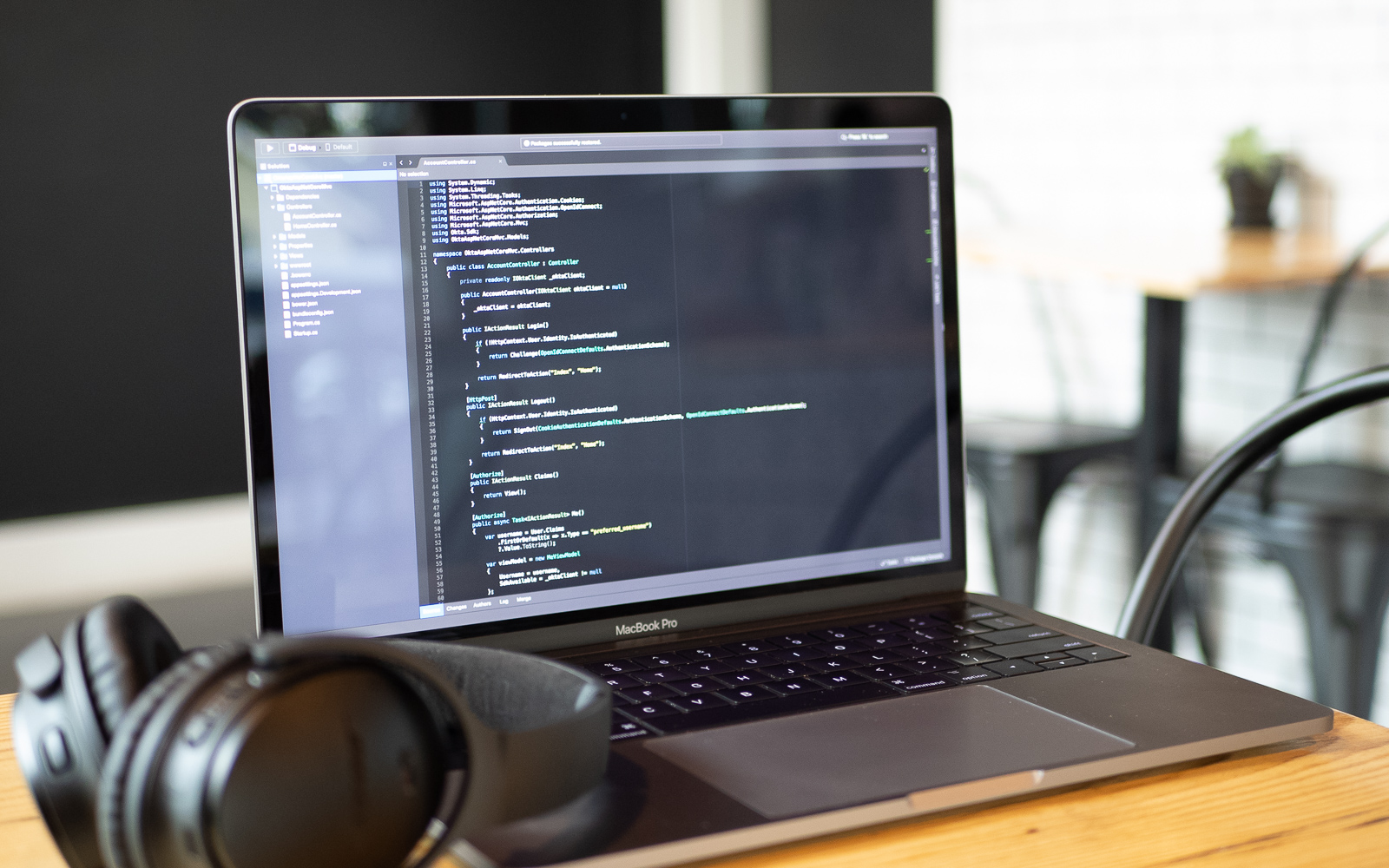
Microservices are fun to build and offer us a scalable path to overcoming problems with tightly coupled dependencies that plague monolithic applications. This post will walk you through building an AWS Lambda microservice written in C# with .NET Core 2.1, and communicating in JSON. We’re bringing together multiple exciting technologies here - microservices, serverless API via AWS Lambda, and authentication using Okta’s easy and convenient identity provider. Each of these technologies is deserving of their...
Build a REST API with ASP.NET Web API
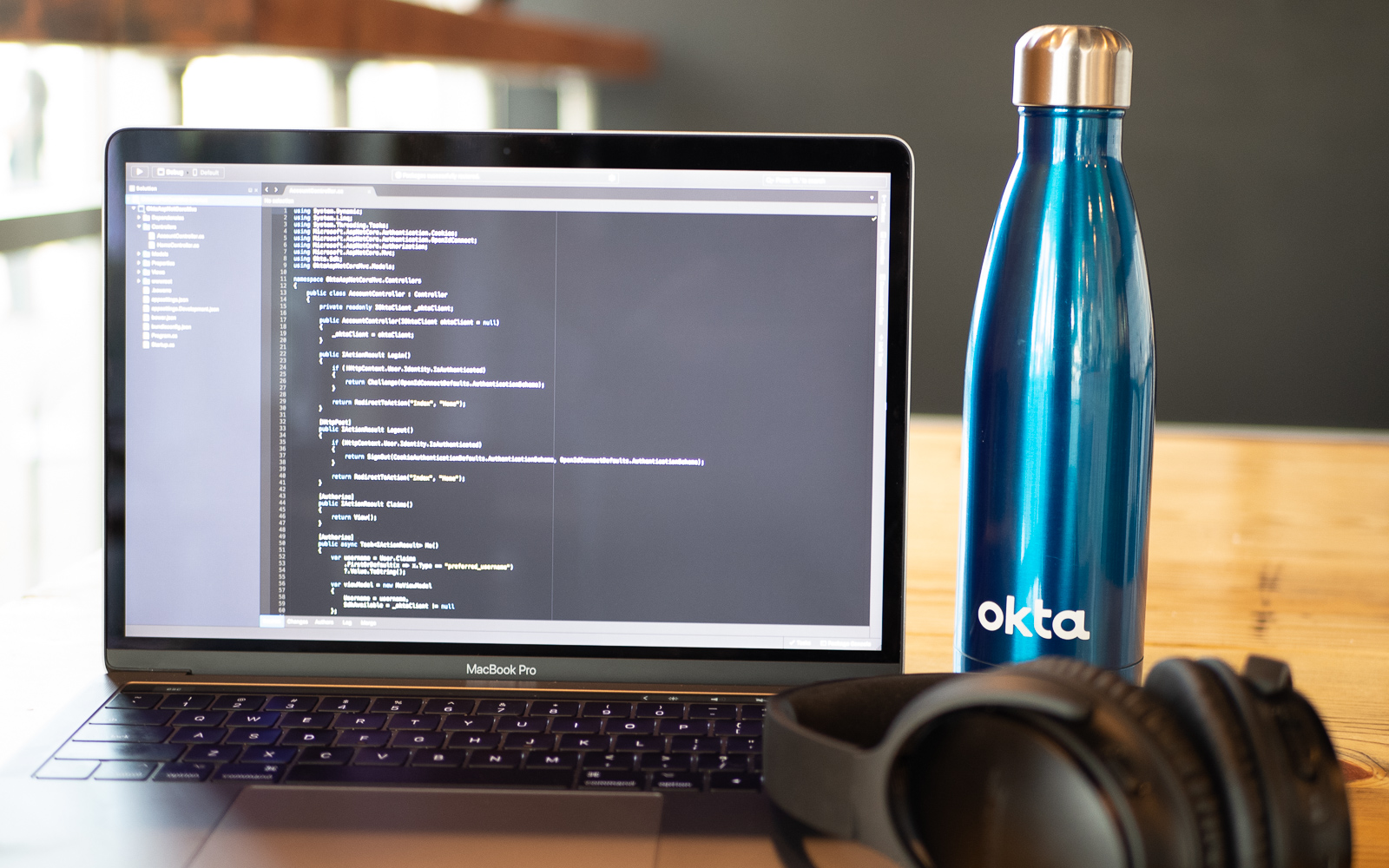
Do you need to build a REST API with ASP.NET Web API? If you’re creating a new API, you should probably create it with .NET Core. But it’s not always possible to use the latest and greatest technologies. If you’re working in an existing ASP.NET 4.x app, or the organization you work for hasn’t approved the use of .NET Core yet, you may need to build an API in .NET Framework 4.x. That is what...
Build a CRUD App with ASP.NET MVC and Entity Framework
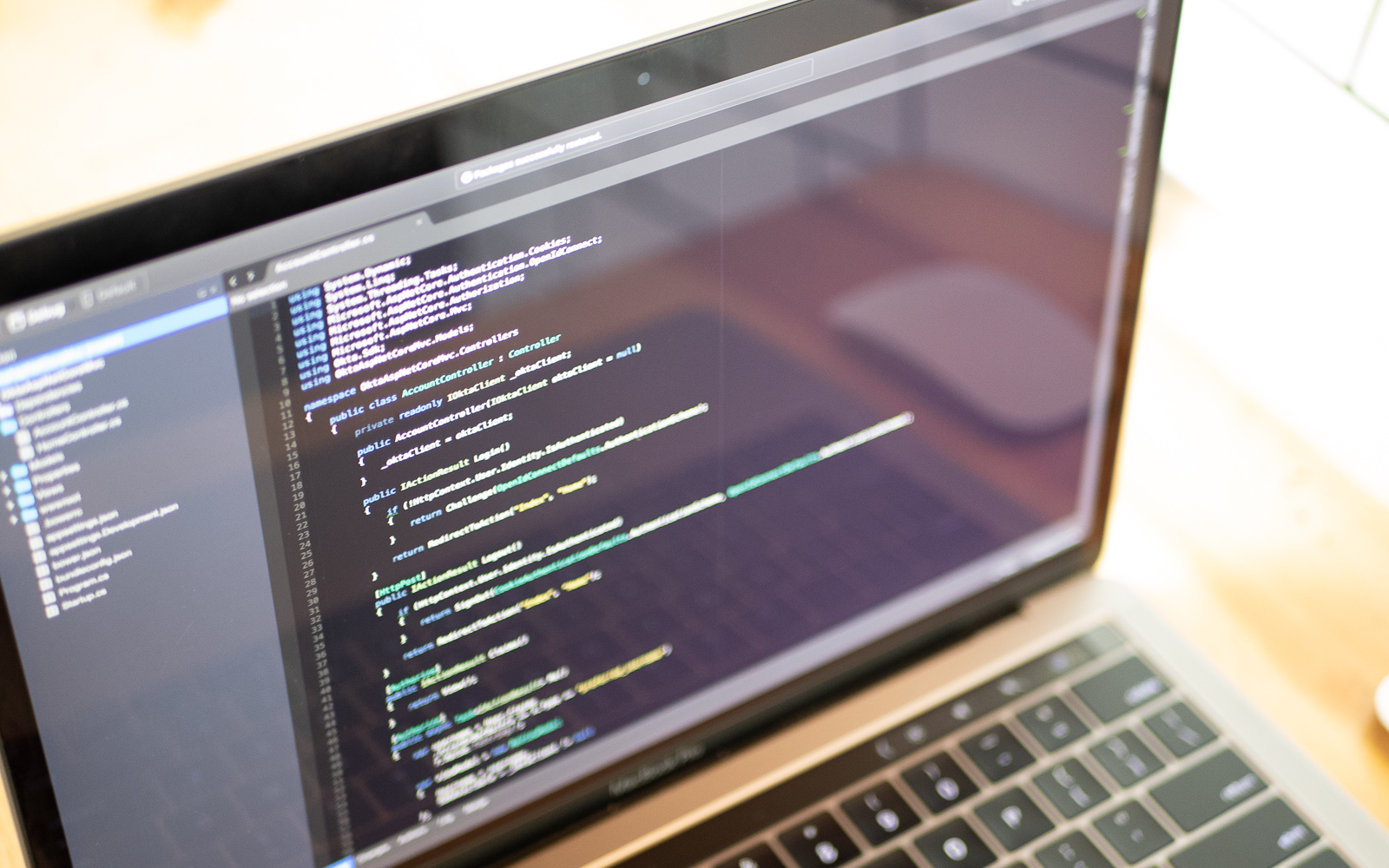
Interested in building a secure ASP.NET MVC website that allows users to handle their own data with ease? Let’s walk through creating a basic application that allows the creation, reading, updating, and deletion of data (CRUD) with Entity Framework by your users while managing them easily with Okta. For a fun example, we will create a web application that lists upcoming rocket launches for space enthusiasts! You will have everything you need to get up...
Build Your First Azure Function in Visual Studio Code
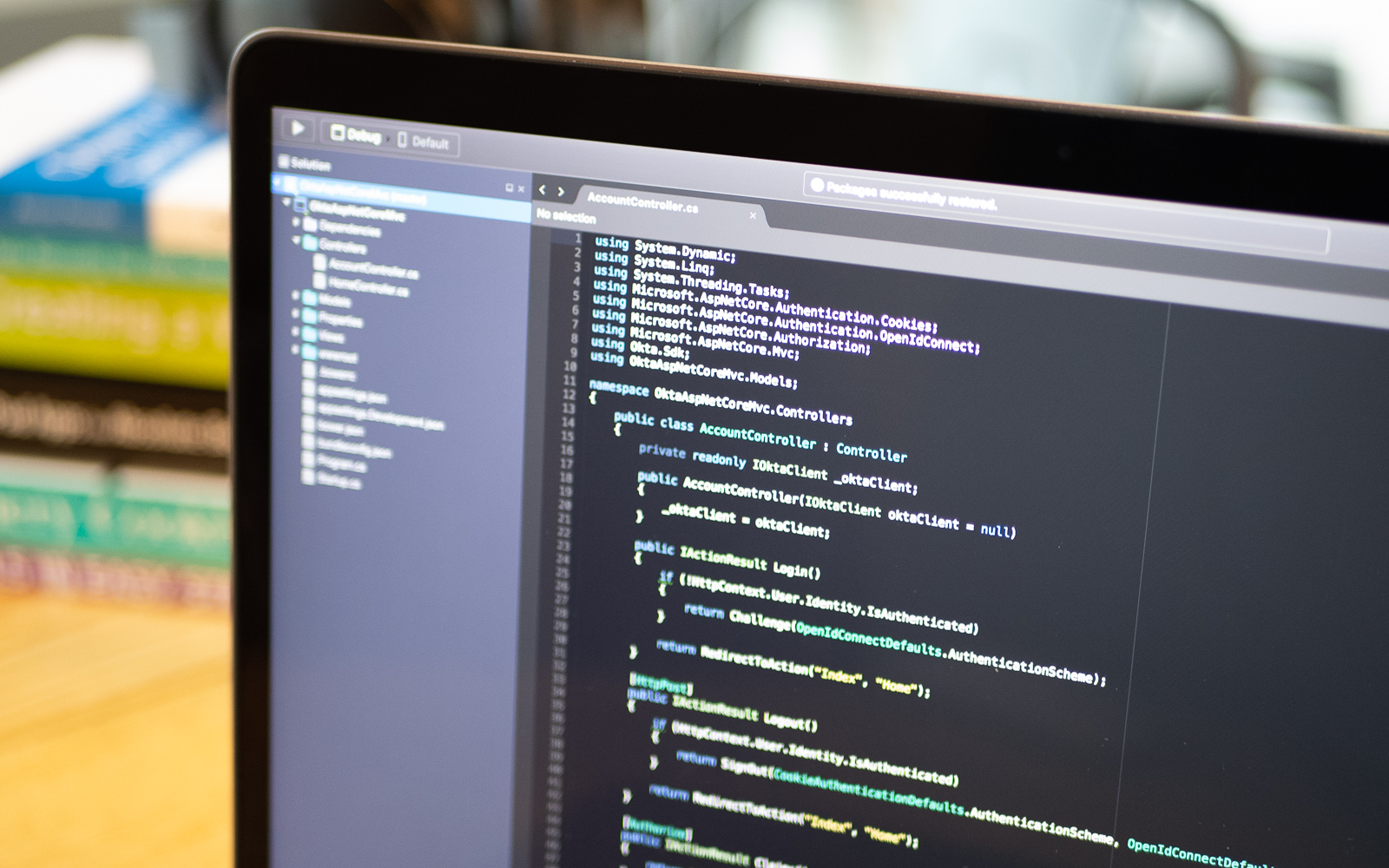
Inevitably it happens. You go to one of those bars that has three trillion beers on tap, and you stare hopelessly at the wall of taps trying to decide what to order. Panic no more! Hop Roulette is here to save you from embarrassment when the bartender asks, “What’ll it be?” Hop Roulette is a simple Azure Function that returns a random beer from an API. What use is this? It gives me a fun...
Create Login and Registration in Your ASP.NET Core MVC App
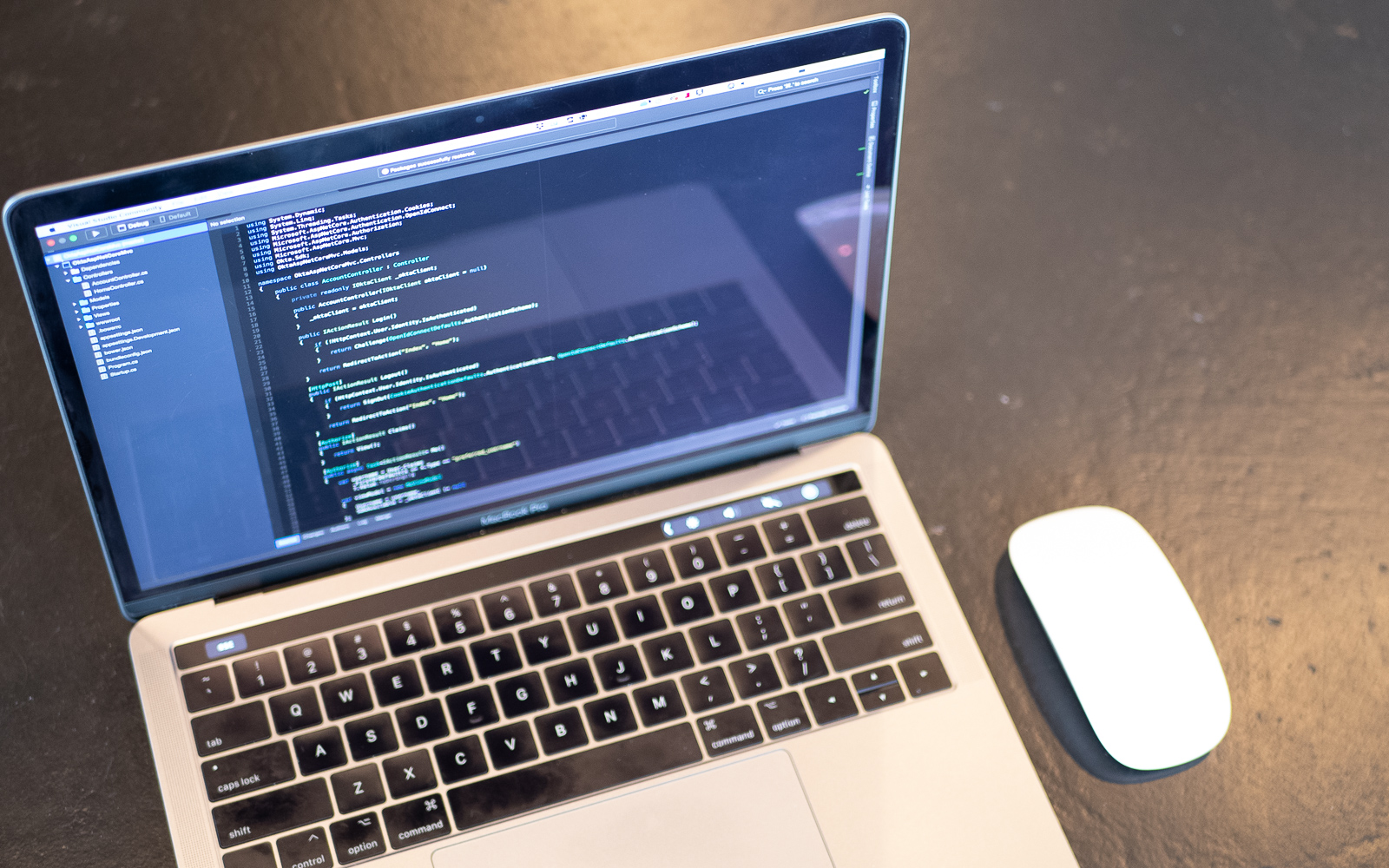
User authentication and authorization are common features in web applications, but building these mechanics has the potential to take a lot of time. Doing so requires setting up persistent storage for user information (in some type of database) and paying keen attention to potential security issues around sensitive operations like hashing passwords, password reset workflows, etc. - weeks of development time begin to add up before we ever get to the functionality that delivers value...
Go for liftoff at Okta!
T-minus 3… 2… 1… I’m blasting my way into the Developer Relations world like Alice discovering Wonderland and its fantastic cast of characters. I am so curious, and excited, and all of the feels that come with coming to Okta! Officially stepping into the Developer Avocado (er… pardon me, Advocate) role and bringing the love of .NET, voice and IOT to the party. Who is this Lady Nerd? Well, for starters I am obsessed with...
Build a Basic Website with ASP.NET MVC and Angular
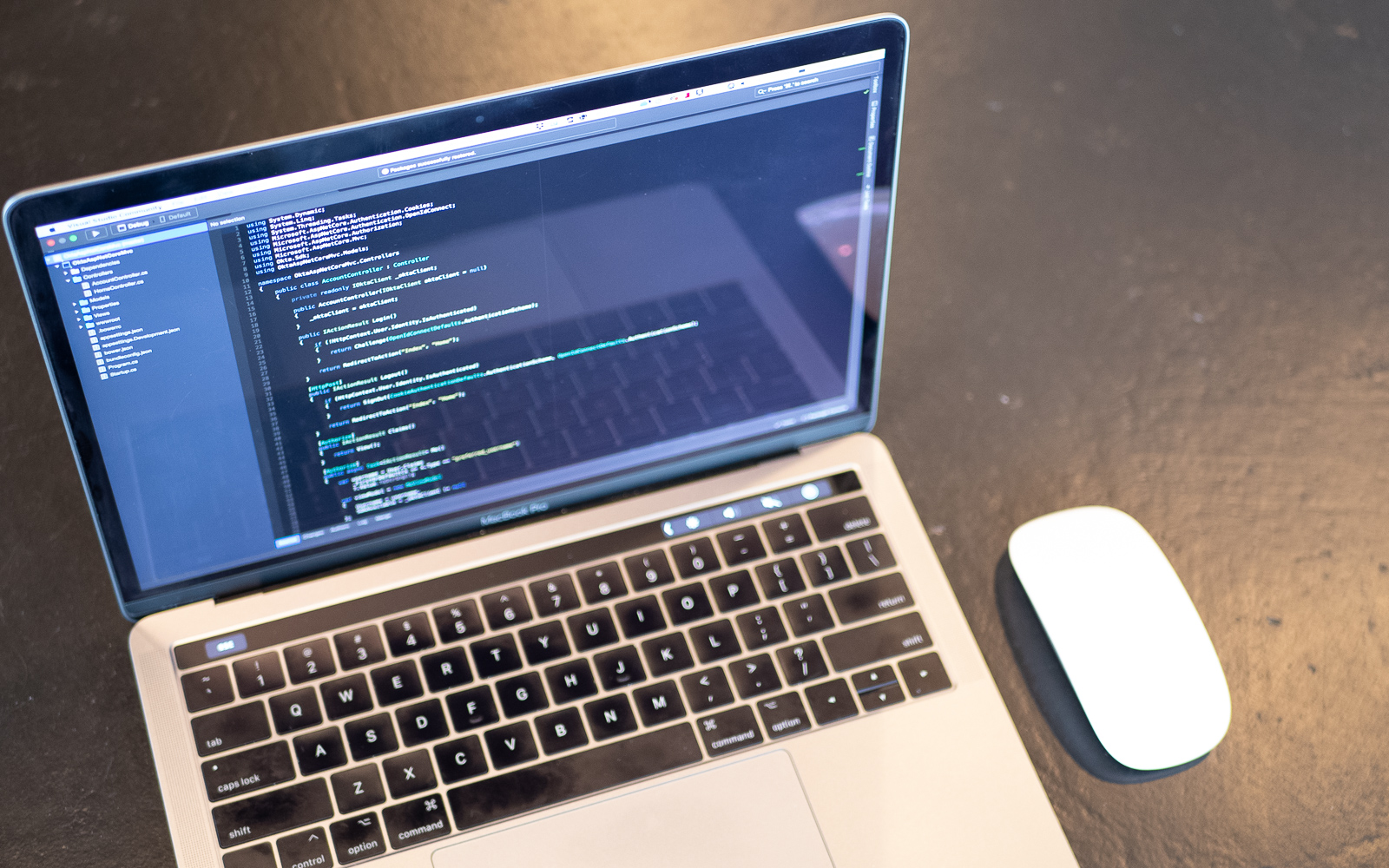
ASP.NET has been around for a long time. When Microsoft introduced ASP.NET MVC, it changed the way many developers approach their codebase. There is an excellent separation of concerns, a TDD friendly framework, and easy integration with JavaScript while maintaining full control over rendered HTML. On the client side, a lot of .NET developers prefer Angular because it comes with TypeScript and it’s a much closer language to C# than plain JavaScript. Angular is an...
Add Login to Your ASP.NET Core MVC App
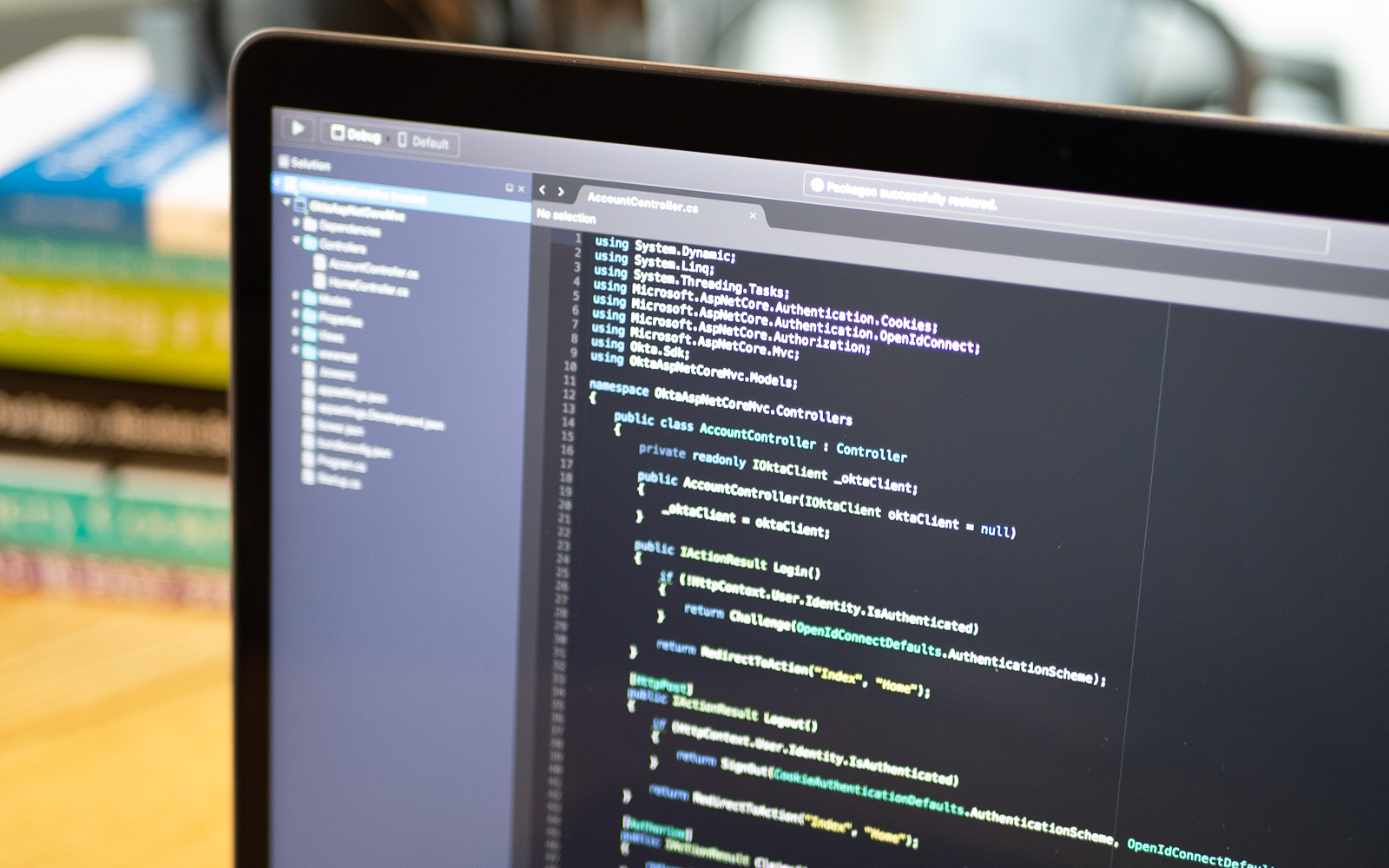
One of the improvements in the latest version of ASP.NET Core (MVC ) is a new and simplified authentication integration. However, managing authentication on your own is still a considerable burden. Not only do you need to handle signup and login, but you also have to set up the database, manage security aspects of registration and login, and take care of session management. Since the integration of external auth providers has never been more comfortable,...
Build a SPA with ASP.NET Core 2.1, Stripe, and Angular 6
Buying things on the Internet has become a daily activity and is a feature many new projects require. In this tutorial, I will show you how to build an app to sell tickets using an Angular 6 single page app (SPA) using an ASP.NET Core 2.1 backend API. You’ll build both the Angular and ASP.NET Core applications and run them from within VS Code. Let’s get to it! Upgrade to Angular 6 I love to...
Upgrade your ASP.NET Core 2.1 App to Angular 6
With the release of ASP.NET Core, there are several templates in the DotNet CLI. One of those templates is an Angular template that scaffolds a single page application built with Angular and ASP.NET Core. The problem with that template is that it scaffolds an Angular 4.2.5 project and Angular released Angular 6 in May of 2018! In this post, not only will I show you how to build a base CRUD app with ASP.NET Core...
Build a CRUD App with ASP.NET Framework 4.x Web API and Angular
Even with all the hype around ASP.NET Core, many .NET developers continue to develop applications with ASP.NET 4.x. The ASP.NET 4.X framework is still being developed, and will be supported for a long time to come. It’s a battle-tested web framework that has existed for over 15 years and is supported by a mature ecosystem. On the client side, many developers prefer Angular, and it is outstanding for building enterprise-level, feature rich, applications. The application...
Token Authentication in ASP.NET Core 2.0 - A Complete Guide
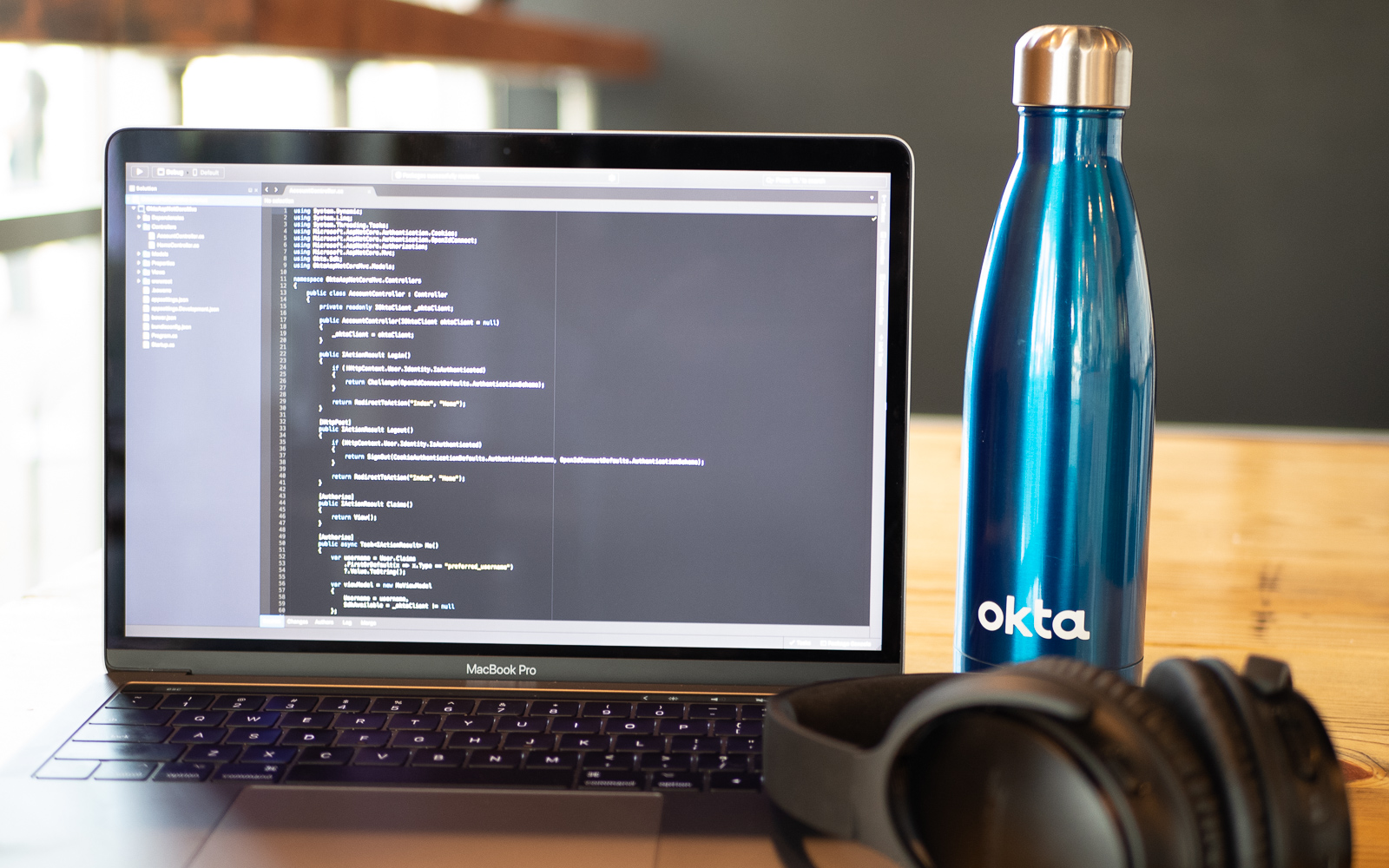
Token authentication has been a popular topic for the past few years, especially as mobile and JavaScript apps have continued to gain mindshare. Widespread adoption of token-based standards like OAuth 2.0 and OpenID Connect have introduced even more developers to tokens, but the best practices aren’t always clear. I spend a lot of time in the ASP.NET Core world and have been working with the framework since the pre-1.0 days. ASP.NET Core 2.0 has great...
How to Secure Your .NET Web API with Token Authentication
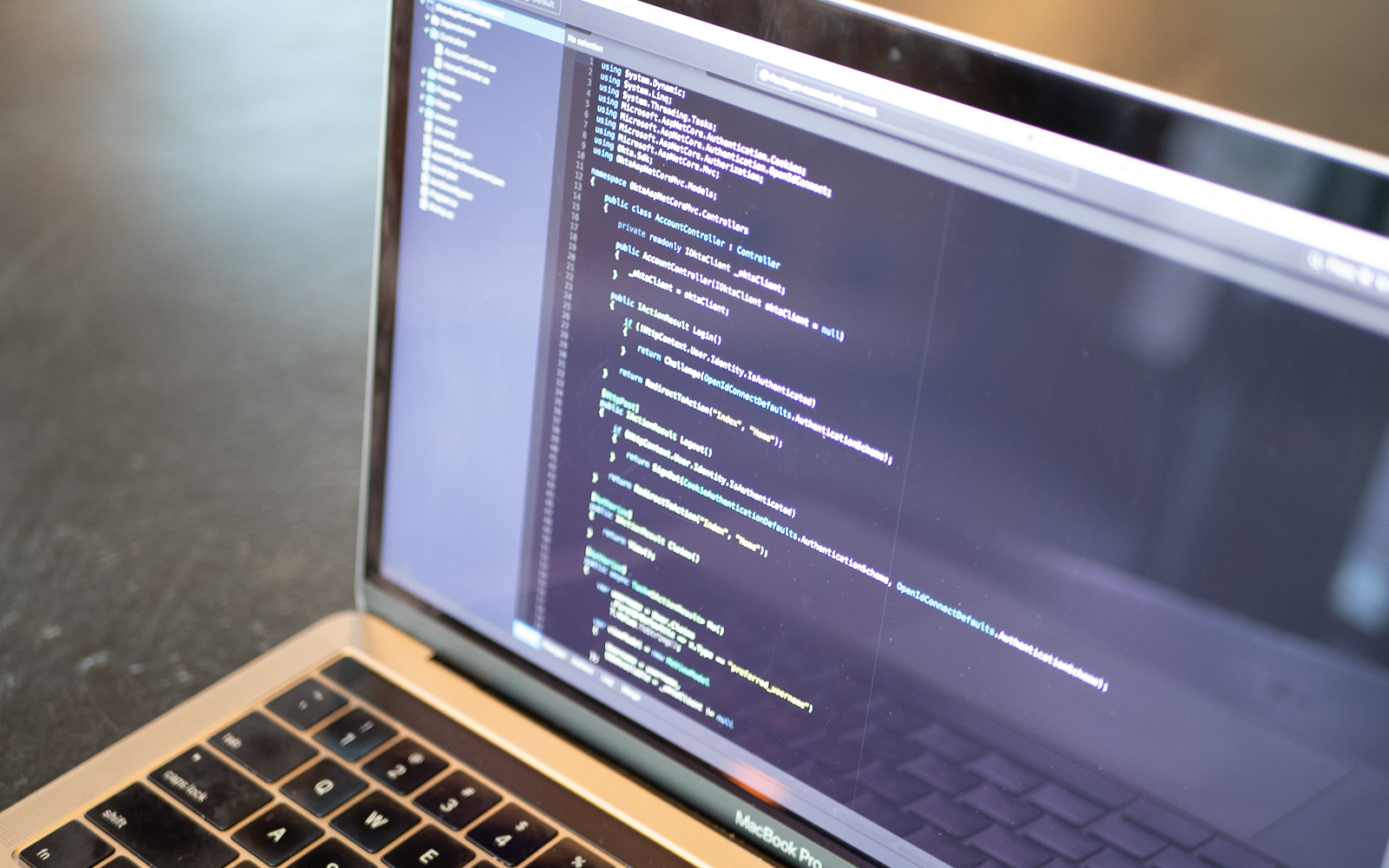
API security can be complex. In many cases, just because you’ve built an API that you want to make public, it doesn’t mean that you want just anybody accessing it. In most cases, you want fine-grained control over who can access the API, but setting up that kind of user management can be a daunting task: you’d have to create your own authorization service that can create API credentials for your users and have the...
Build a Secure To-Do App with Vue, ASP.NET Core, and Okta
I love lists. I keep everything I need to do (too many things, usually) in a big to-do list, and the list helps keep me sane throughout the day. It’s like having a second brain! There are hundreds of to-do apps out there, but today I’ll show you how to build your own from scratch. Why? It’s the perfect exercise for learning a new language or framework! A to-do app is more complex than “Hello...
Build an App for iOS and Android with Xamarin
Xamarin is a cross-platform technology that makes it possible to build native applications for Android and iOS using a single, shared codebase. Like other technologies such as React Native and NativeScript, it allows development teams to spend less time writing code for both platforms. Xamarin is open-source (and free). Under the hood, it uses Mono (a version of the Microsoft .NET runtime), so Xamarin apps are usually written in C#. You can build Xamarin apps...