Articles tagged react
Secure OAuth 2.0 Access Tokens with Proofs of Possession
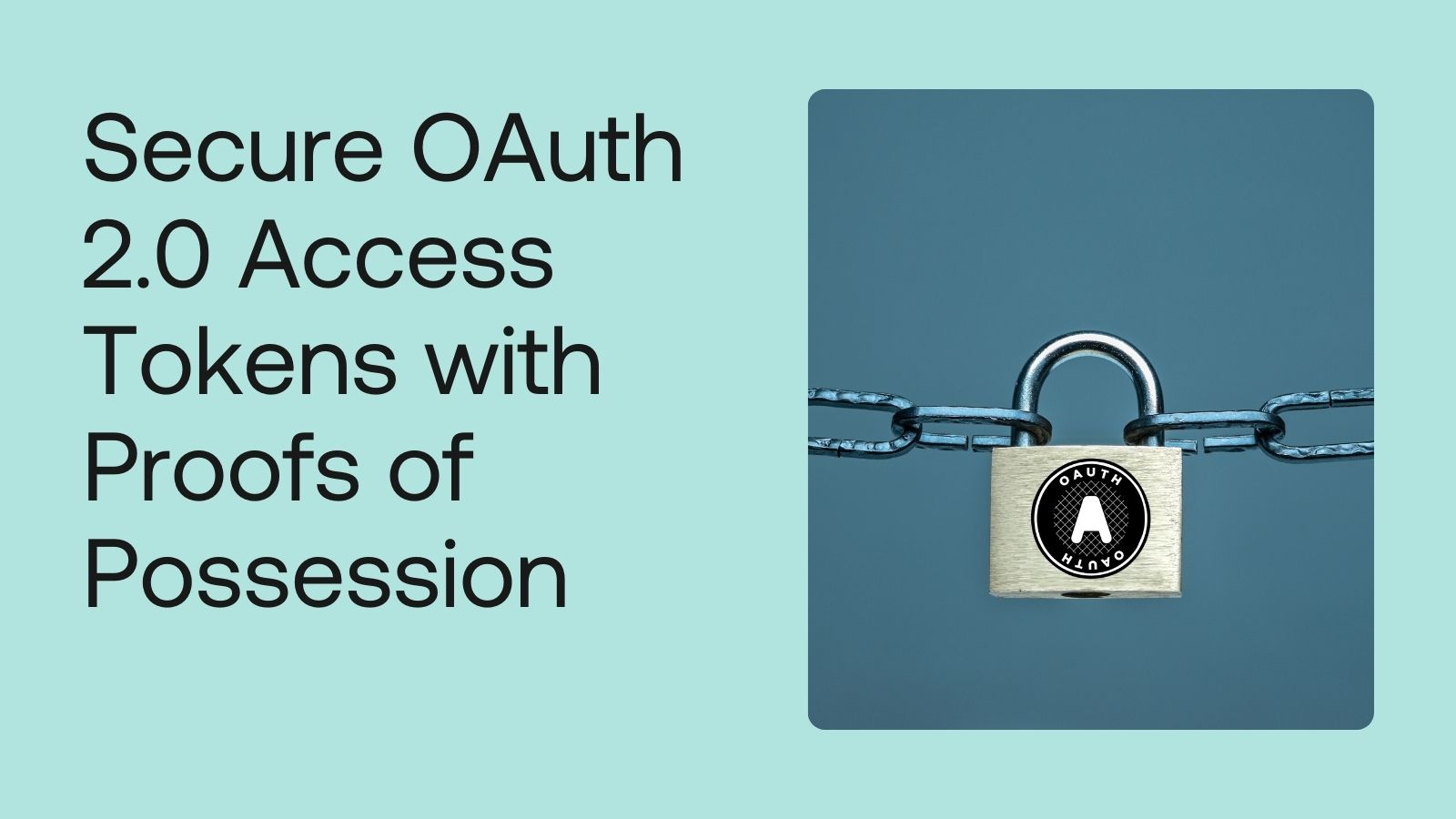
In OAuth, a valid access token grants the caller access to resources and the ability to perform actions on the resources. This means the access token is powerful and dangerous if it falls into malicious hands. The traditional bearer token scheme means the token grants anyone who possesses it access. A new OAuth 2.0 extension specification, Demonstrating Proof of Possession (DPoP), defines a standard way that binds the access token to the OAuth client sending...
Enterprise Maturity Workshop: Automate with no-code Okta Workflows
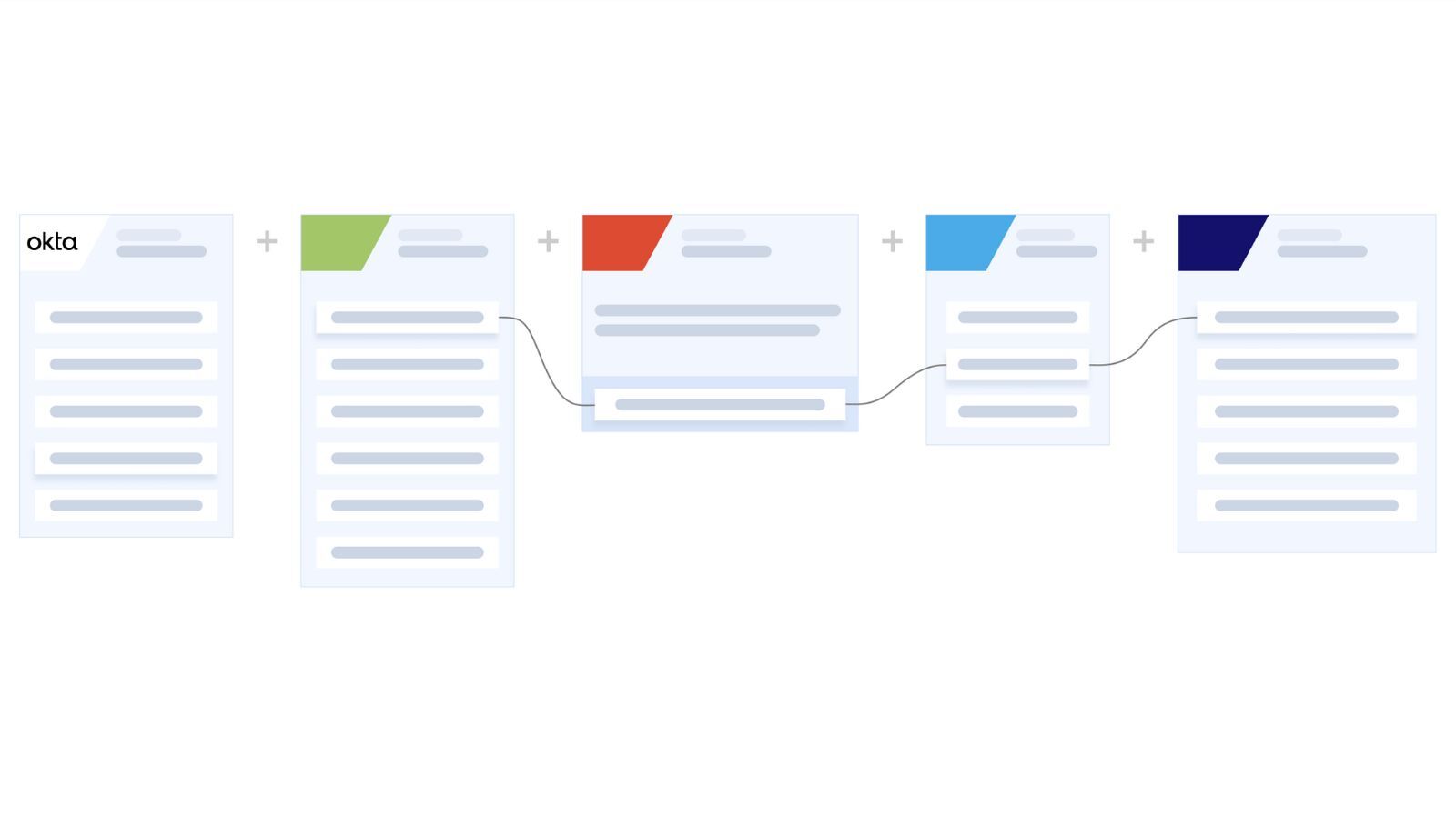
This tutorial is part of the on-demand workshop series. In this workshop, you’ll enhance the base Todo application by creating an automated report using Okta’s no-code Workflows platform. Table of Contents What is Okta Workflows? Getting access to Okta Workflows Creating a flow Okta Workflows building blocks Setting up the Todo application Enhancing the Todo application with a new API Launching the API in a local tunnel Building the Todo Report flow Updating the flow...
Enterprise-Ready Workshop: Authenticate with OpenID Connect
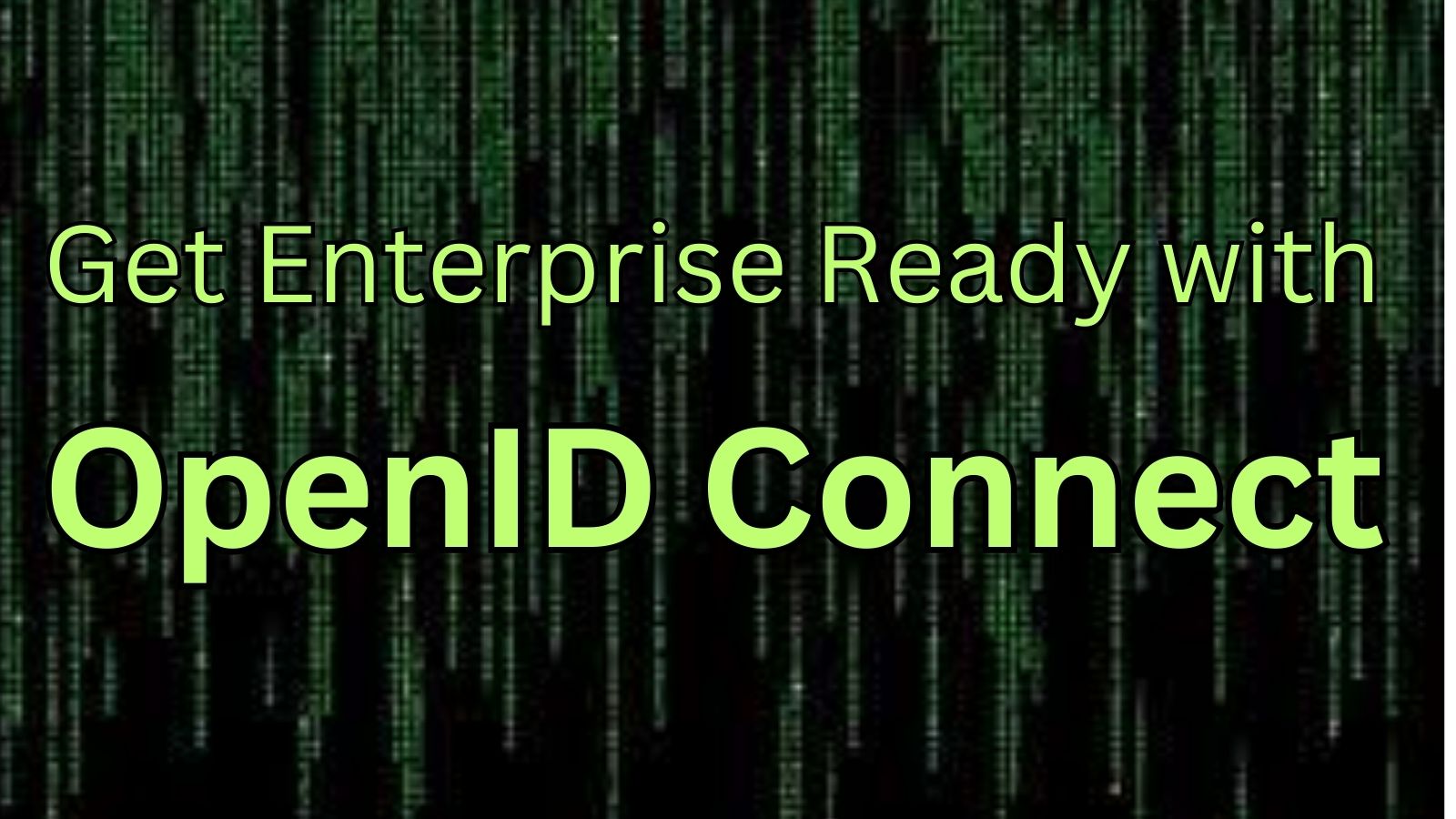
This workshop is part of our Enterprise Readiness Workshop series. In this workshop, you will be wearing the hat of a SaaS developer who will up-level his/her app to allow users (from your big enterprise customers) to log on using their own company credentials without providing a password directly to your app. When any enterprise customer considers buying your software to enhance their employees’ productivity, their IT and security teams want to make sure employees...
How to Get Going with the On-Demand SaaS Apps Workshops
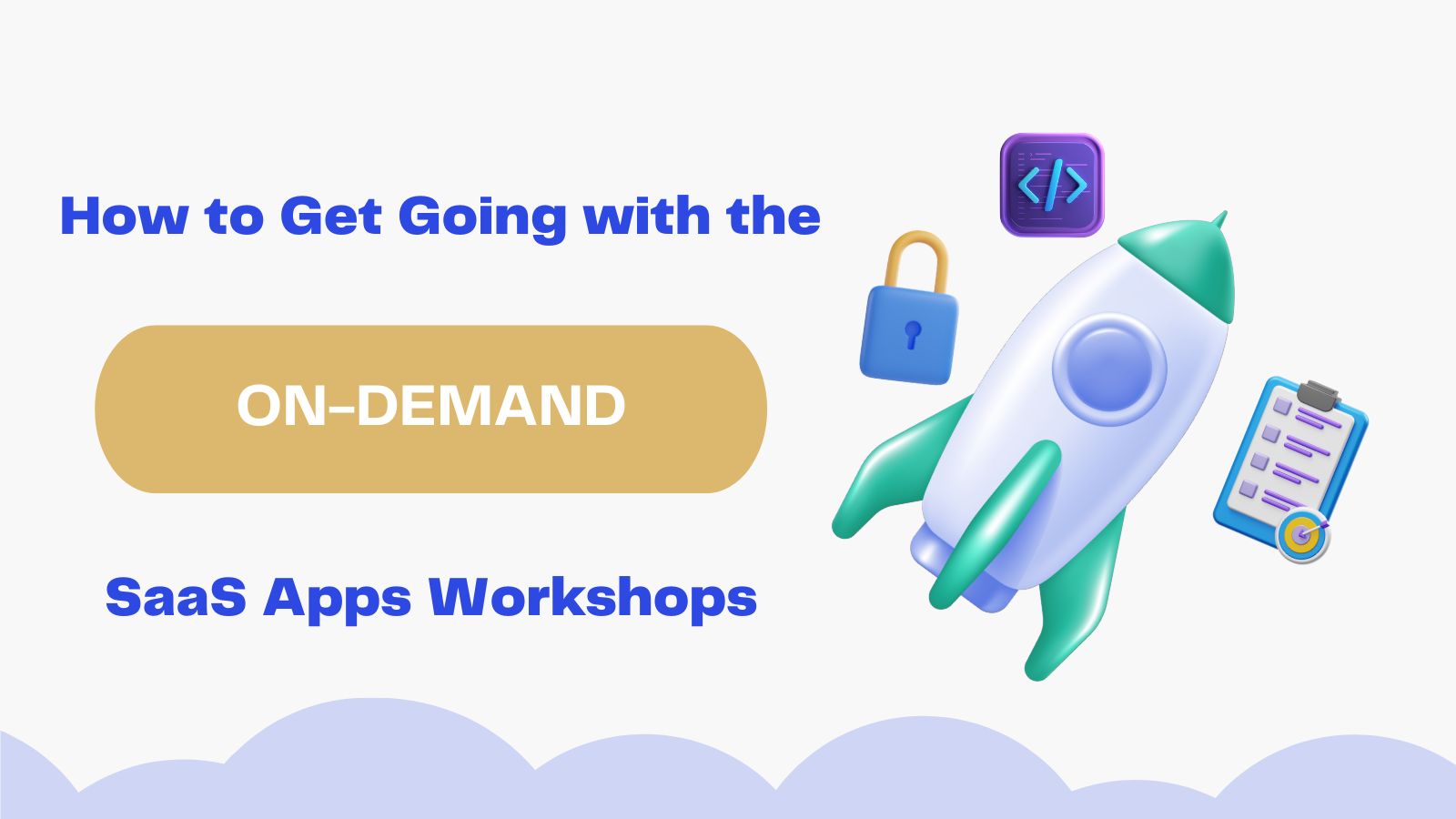
Having an enterprise-ready SaaS application means your application supports authentication best practices, can scale across multiple customers and users, has automated means to re-create environments, and can securely add enhancements and value-adds your customers expect. Join this free virtual workshop series where we take your SaaS application on a journey of enterprise-ready identity — you’ll wear the hat of a SaaS developer preparing your Todo application to support enterprise-level customers who want to use your...
How Authentication and Authorization Work for SPAs
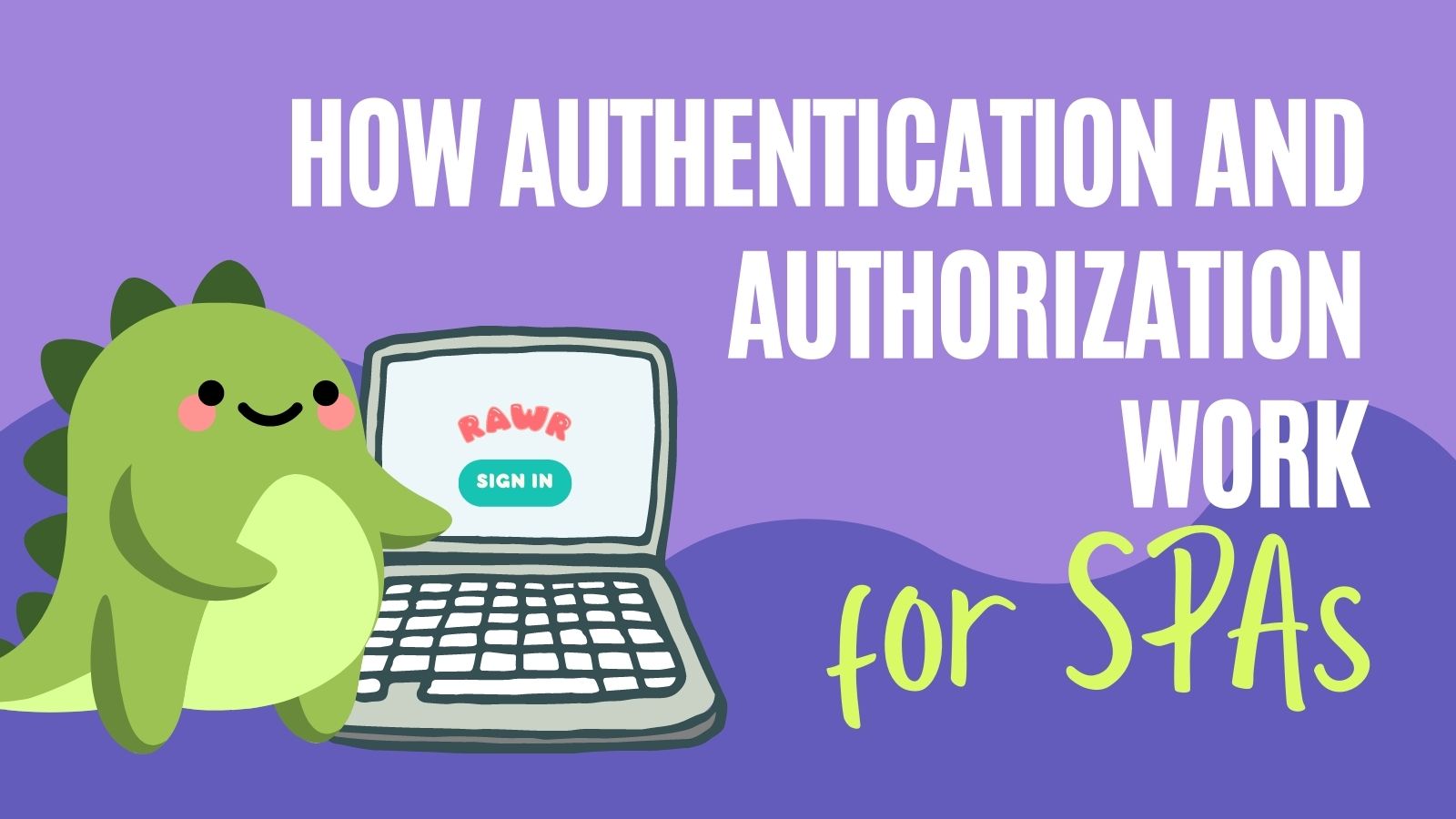
Adding authentication to public clients such as Single Page Applications (SPA) and JavaScript applications can be a source of confusion. Identity Providers like Okta try to help you via multiple support systems. Still, it can feel like a lot of work. Especially since you’re responsible for way more than authentication alone in the applications you work on! As part of authentication, your client application makes multiple calls to an authorization server, and you get back...
How to Build a Secure React and Fastify API App
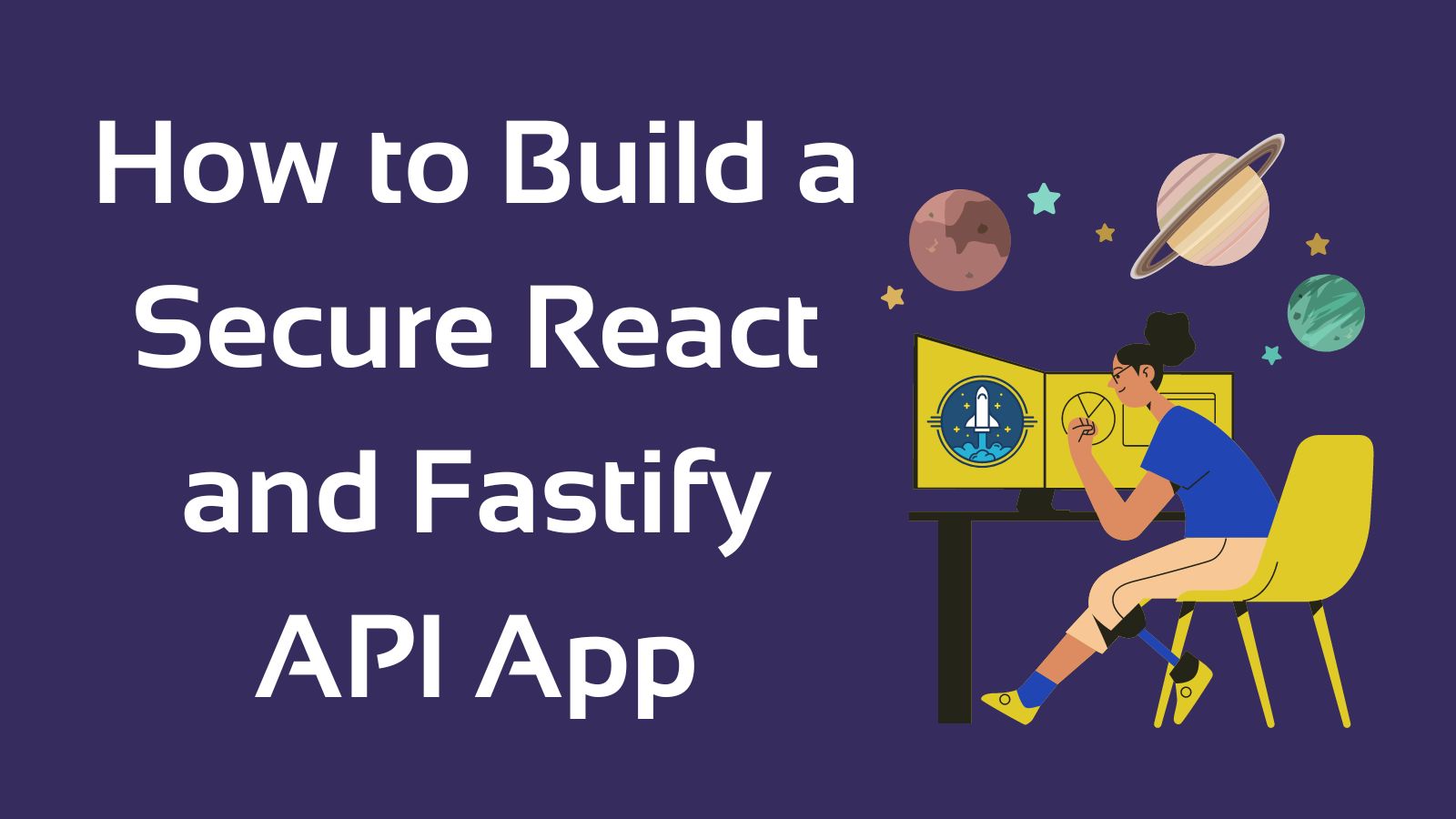
The National Aeronautics and Space Administration (NASA) is an independent agency of the US federal government, responsible for space exploration and research, with field facilities across the United States. In this tutorial, we’ll set up an app to keep track of what NASA facilities we’ve visited and which ones we still want to check out. Our app will be a monorepo with Okta authentication, using React for the frontend and Fastify for the backend. Fastify...
Use Redux to Manage Authenticated State in a React App
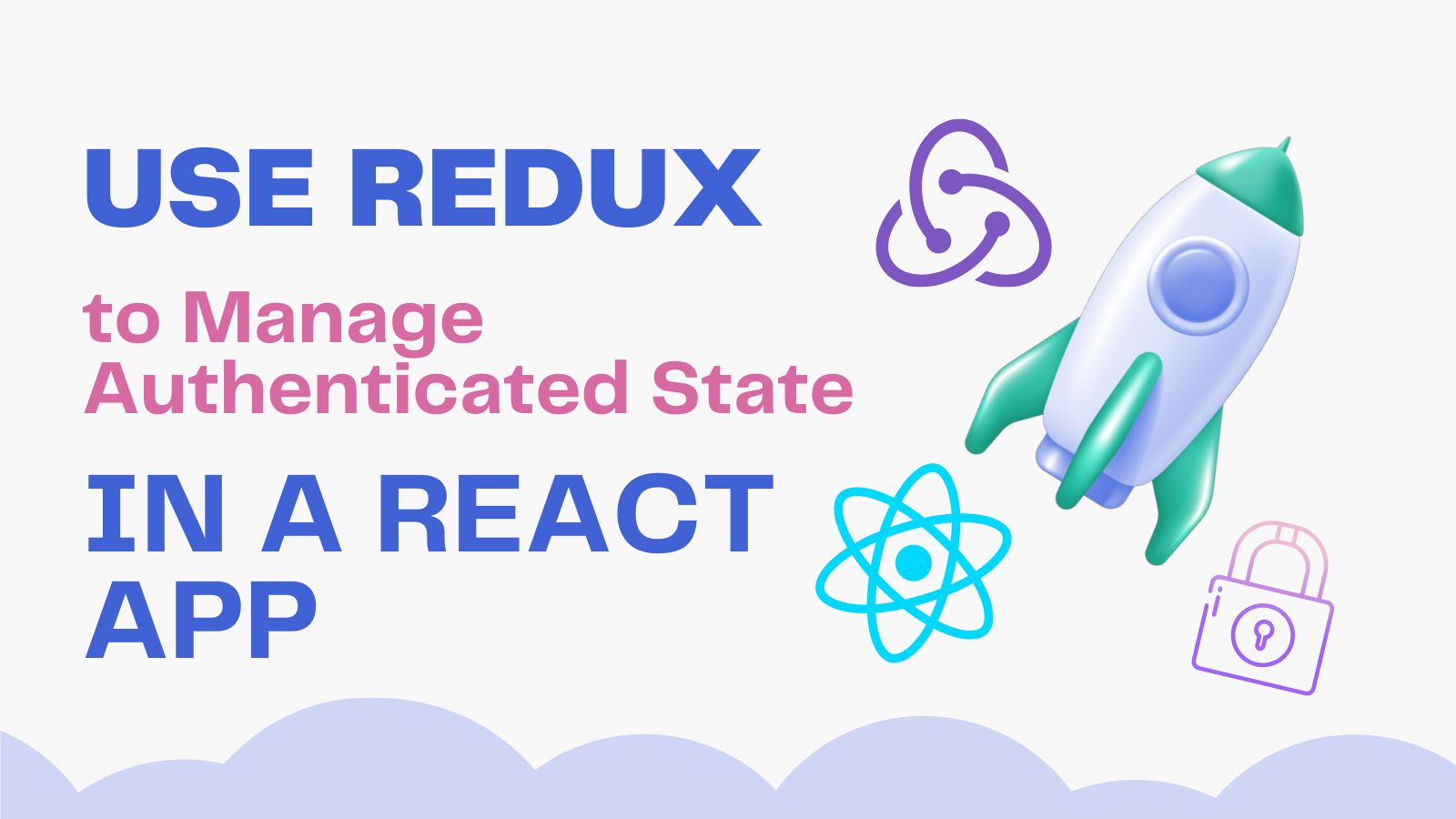
There are a myriad of state management options available for React. React provides the option of using the built-in Context for when you have a nested tree of components that share a state. There is also a built-in useState hook that will allow you to set local state for a component. For more complex scenarios where you need a single source of truth that changes frequently and is shared across large sections of your application,...
Defend Your SPA from Common Web Attacks
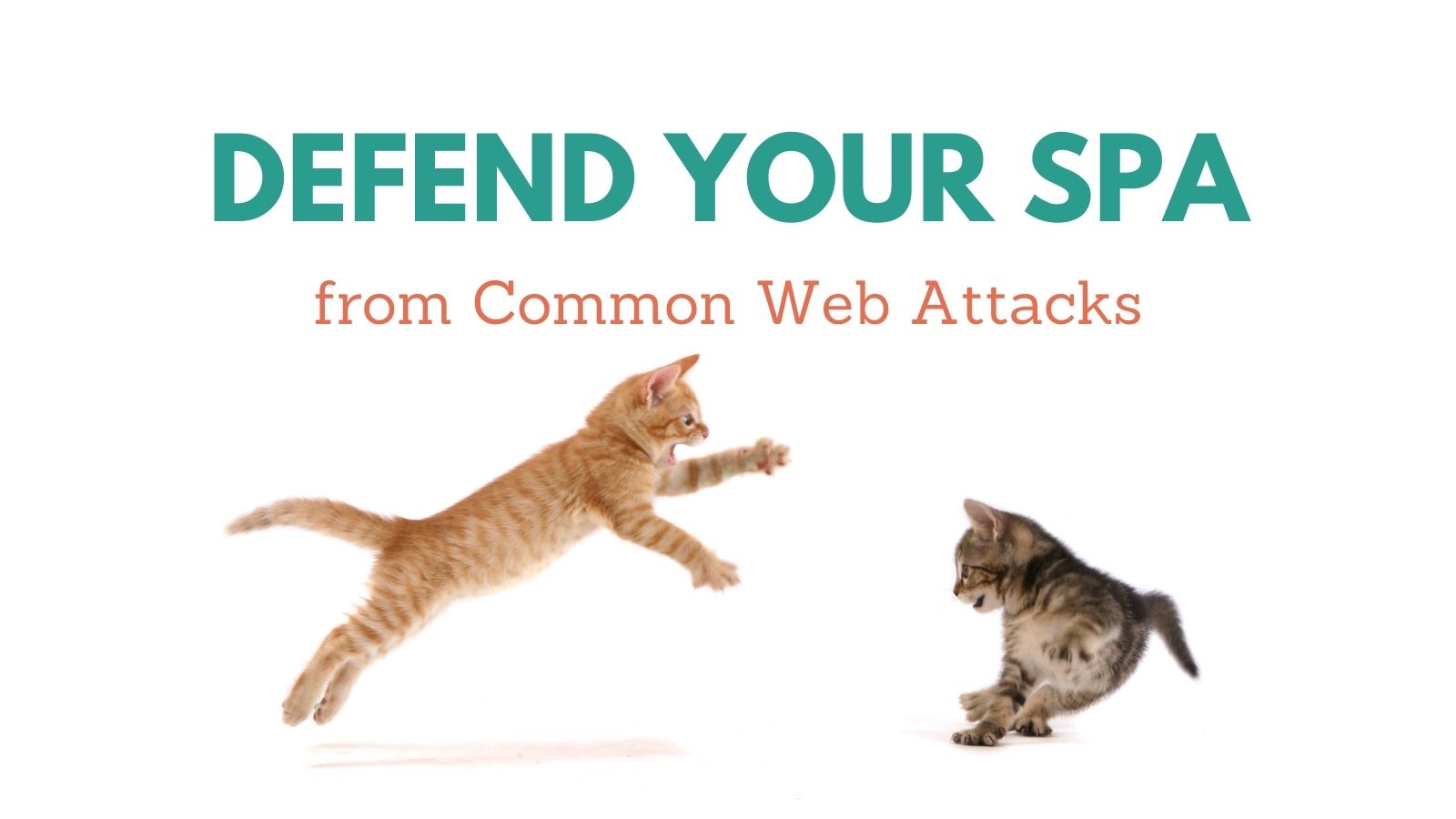
This is the second post in a series about web security for SPAs. In the last post, we laid the groundwork for thinking about web security and applying security mechanisms to our application stack. We covered the OWASP Top Ten, using secure data communication with SSL/TLS, using security headers to help enhance built-in browser mechanisms, keeping dependencies updated, and safeguarding cookies. Posts in the SPA web security series 1. Defend Your SPA from Security Woes...
Defend Your SPA from Security Woes
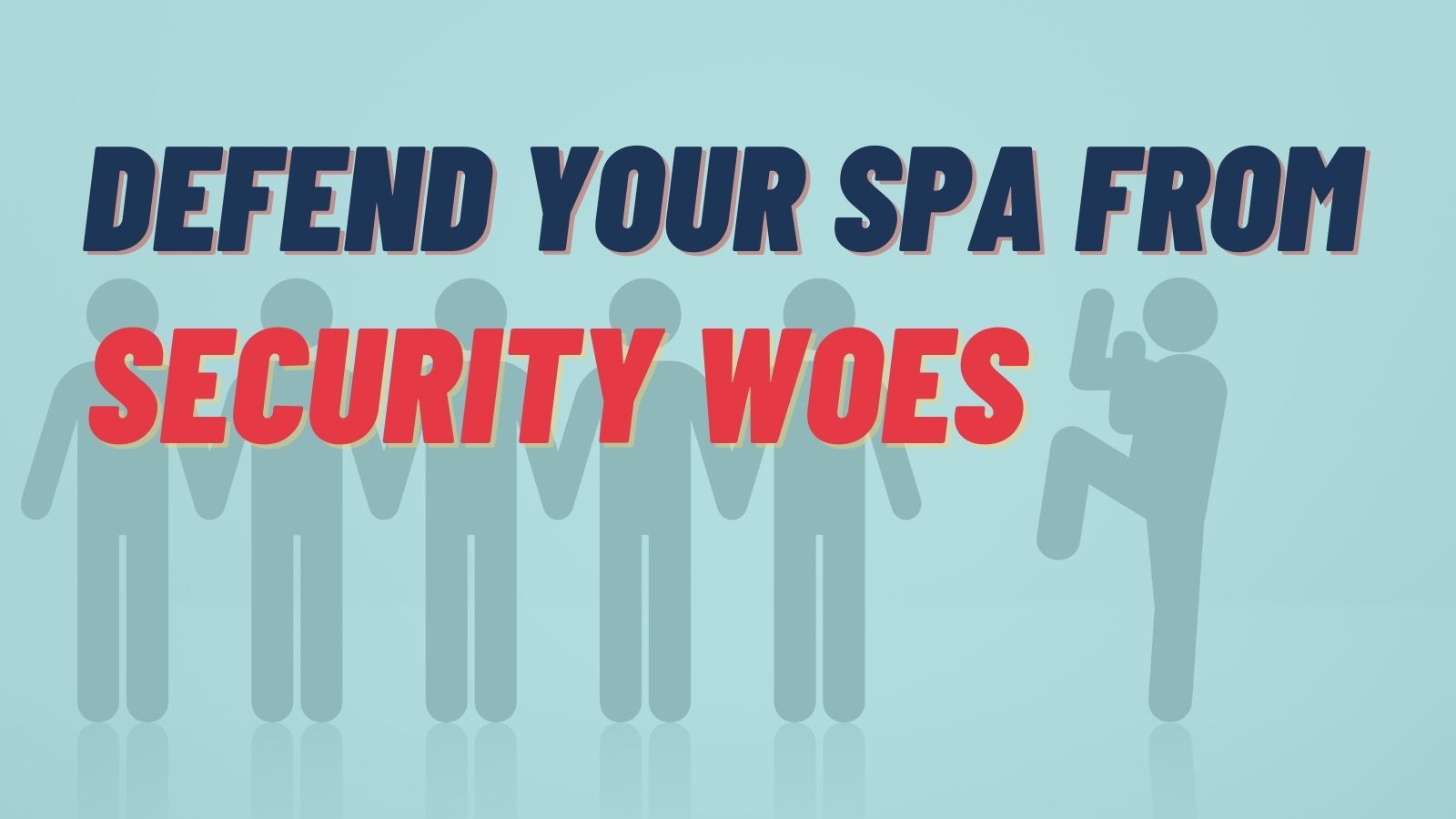
There’s a lot of information floating out there about web security. But when I read through the material, I noticed some information wasn’t up to date, or it was written specifically for traditional server-rendered web applications, or the author recommended anti-patterns. In a series of posts, I will cover web security concerns that all web devs should be aware of, emphasizing client-side applications, namely Single Page Applications (SPAs). Furthermore, I’m not going to get into...
Build a React App with Firebase Serverless Functions
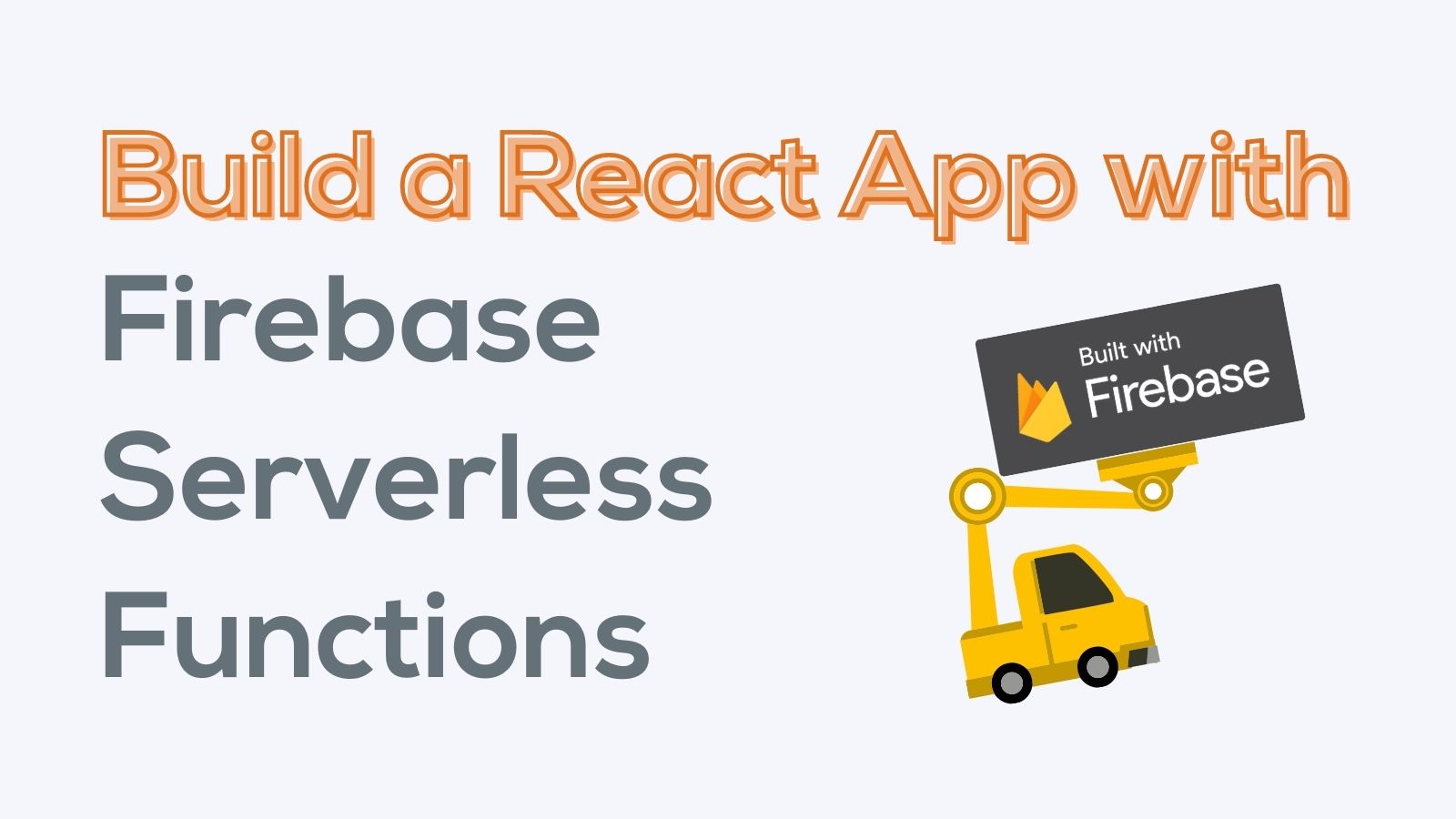
Firebase is an exciting cloud platform from Google available to businesses today. Firebase connects everything from simple static websites to IoT devices to AI and machine learning platforms. The platform provides various services to facilitate these connections, like storage and authentication. In this tutorial, you will learn about two core Firebase products: Cloud Functions for Firebase and Firebase Hosting. Hosting is for deploying static web applications. Functions are the Firebase serverless platform. You will create...
How to Deploy Java Microservices on Amazon EKS Using Terraform and Kubernetes
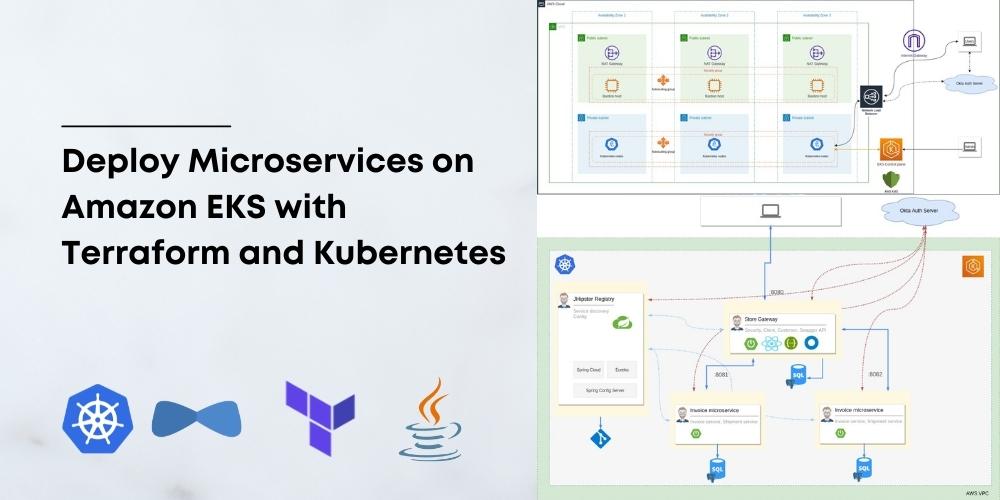
When it comes to infrastructure, public clouds are the most popular choice these days, especially Amazon Web Services (AWS). If you are in one of those lucky or unlucky (depending on how you see it) teams running microservices, then you need a way to orchestrate their deployments. When it comes to orchestrating microservices, Kubernetes is the de-facto choice. Most public cloud providers also provide managed Kubernetes as a service; for example, Google provides Google Kubernetes...
Use React and Spring Boot to Build a Simple CRUD App
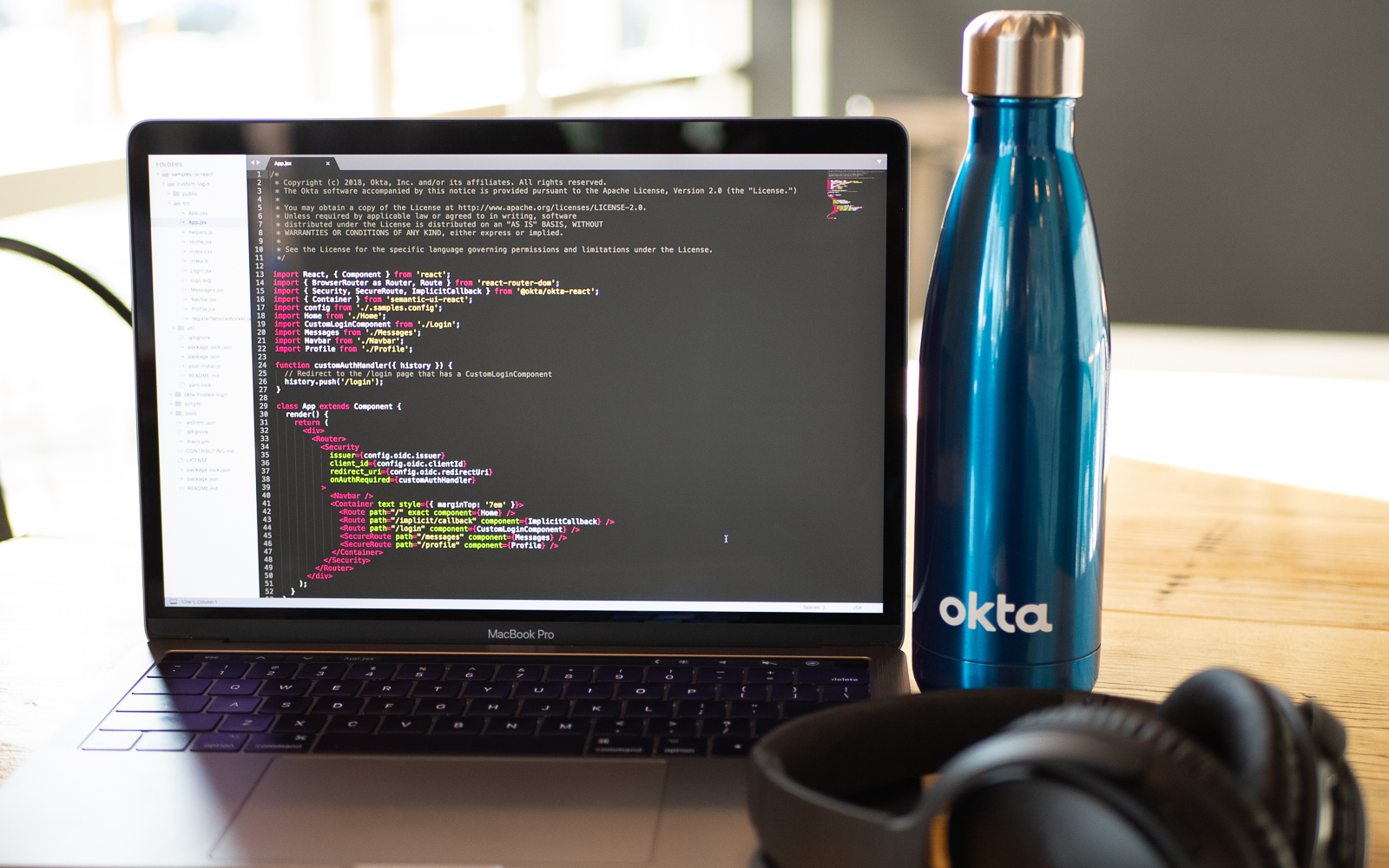
React was designed to make it painless to create interactive UIs. Its state management is efficient and only updates components when your data changes. Component logic is written in JavaScript, meaning you can keep state out of the DOM and create encapsulated components. Developers like CRUD (create, read, update, and delete) apps because they show a lot of the base functionality you need when creating an app. Once you have the basics of CRUD completed...
Cloud Native Java Microservices with JHipster and Istio
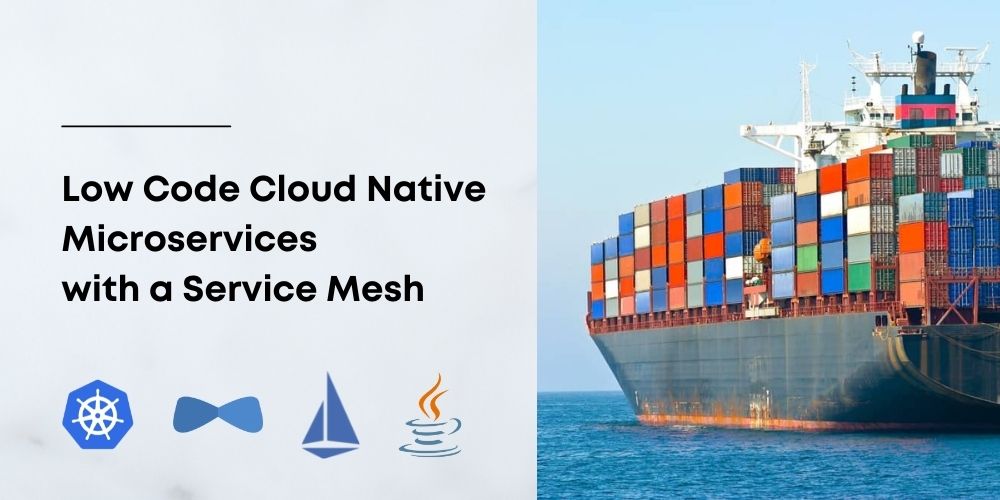
Microservices are not everyone’s cup of tea, and they shouldn’t be. Not every problem can or should be solved by microservices. Sometimes building a simple monolith is a far better option. Microservices are solutions for use cases where scale and scalability are important. A few years ago, microservices were all the rage, made popular, especially by companies like Netflix, Spotify, Google, etc. While the hype has died down a bit, genuine use cases still exist....
How to Build and Deploy a Serverless React App on Azure
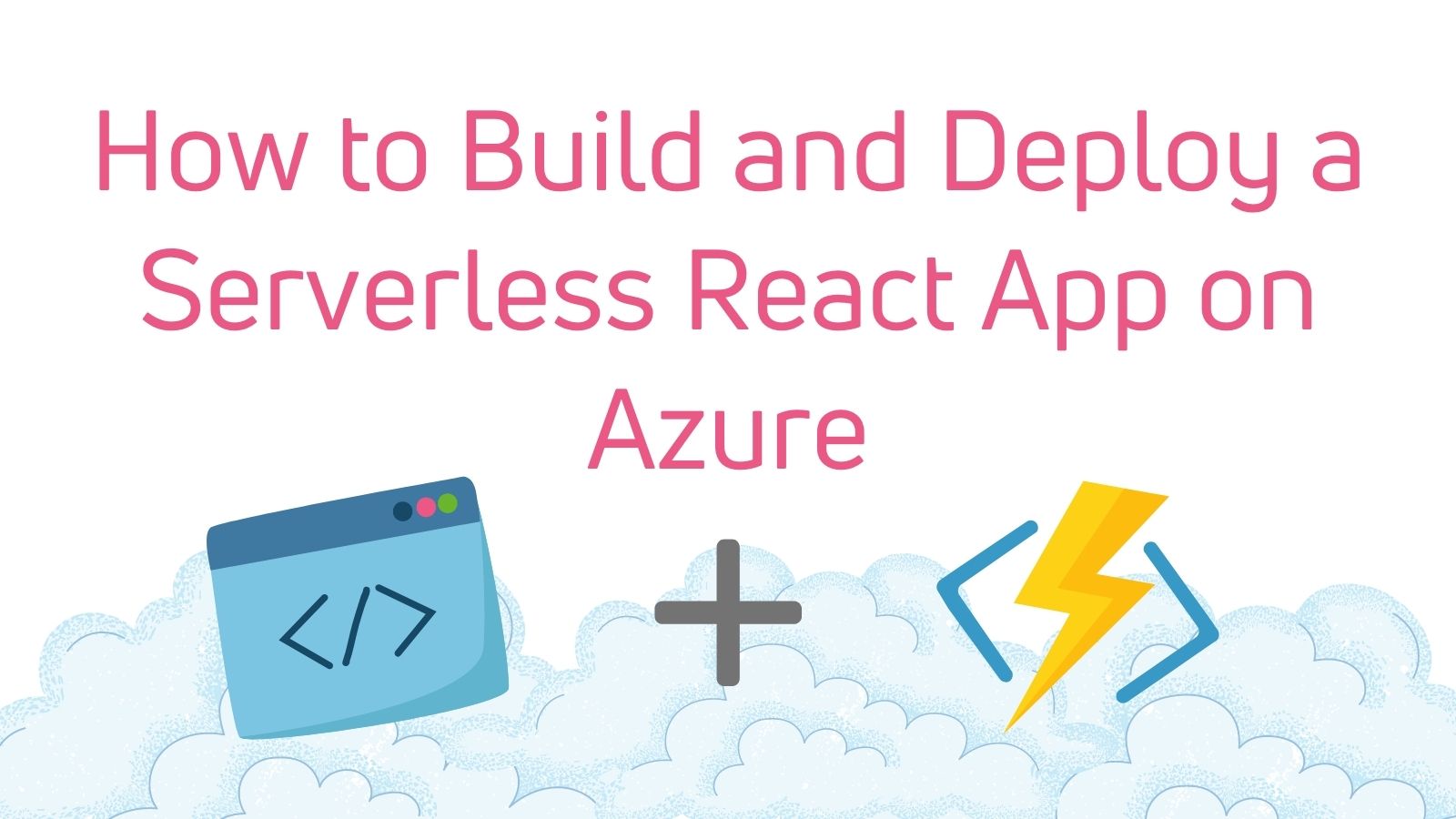
Microsoft’s Azure platform has as many high-tech products as anyone could ever want, including the Azure Static Web Apps service. As the name suggests, the platform hosts static web apps that don’t require a back end. Azure supports React, Angular, Vue, Gatsby, and many more, out of the box. However, you may run into situations where you want some back-end support, such as when you need the backend to run one or two API calls....
Creating a TypeScript React Application with Vite
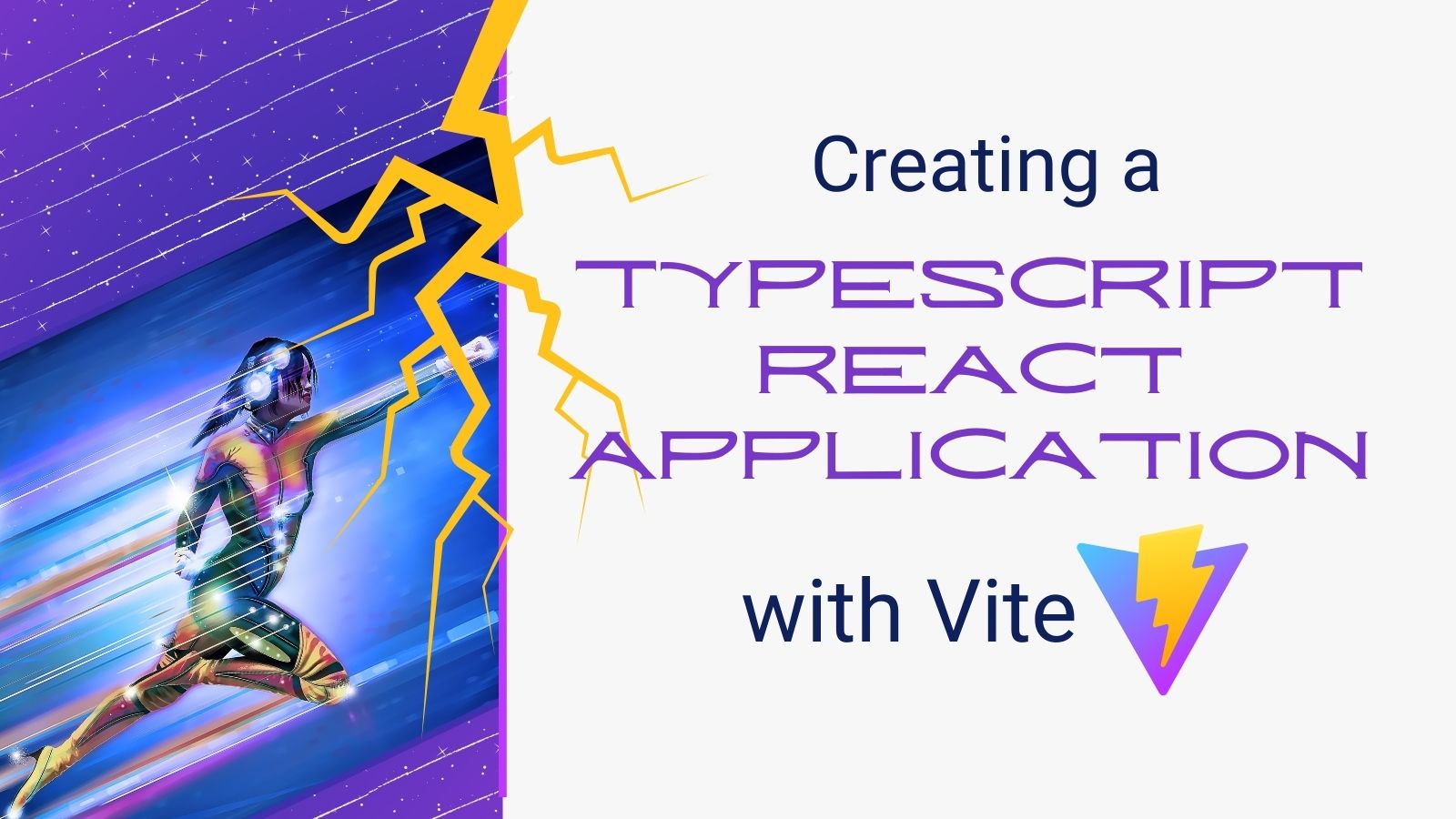
Front-end applications are becoming ever bigger and more complex. It is not uncommon for a React app to have hundreds or even thousands of components. As the project size increases, build times become increasingly important. In large projects, you may have to wait up to a minute for the code to be translated and bundled into a production package run in the browser. The compile and load times for the development server are also a...
How to Create a React App with Storybook
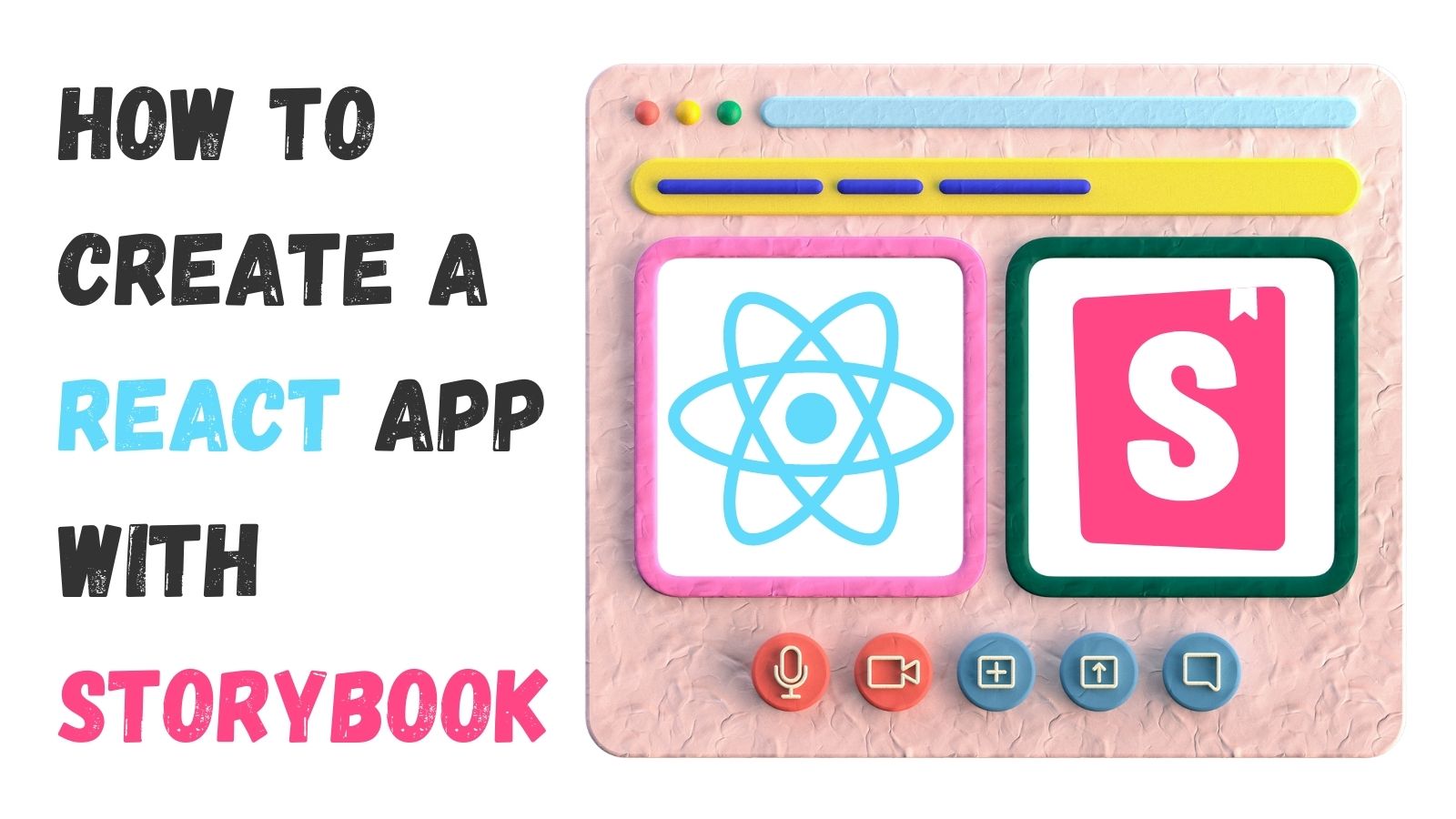
UI designers and front-end developers are tasked with creating clean and consistent user interfaces. At the same time, testing is a cornerstone of software development. Each part of a software project is tested individually and isolated from the other elements in unit tests. This practice has been challenging to achieve in the context of user interfaces. Now Storybook provides an open-source framework that lets you test UI components in isolation from the rest of the...
Full Stack Java with React, Spring Boot, and JHipster
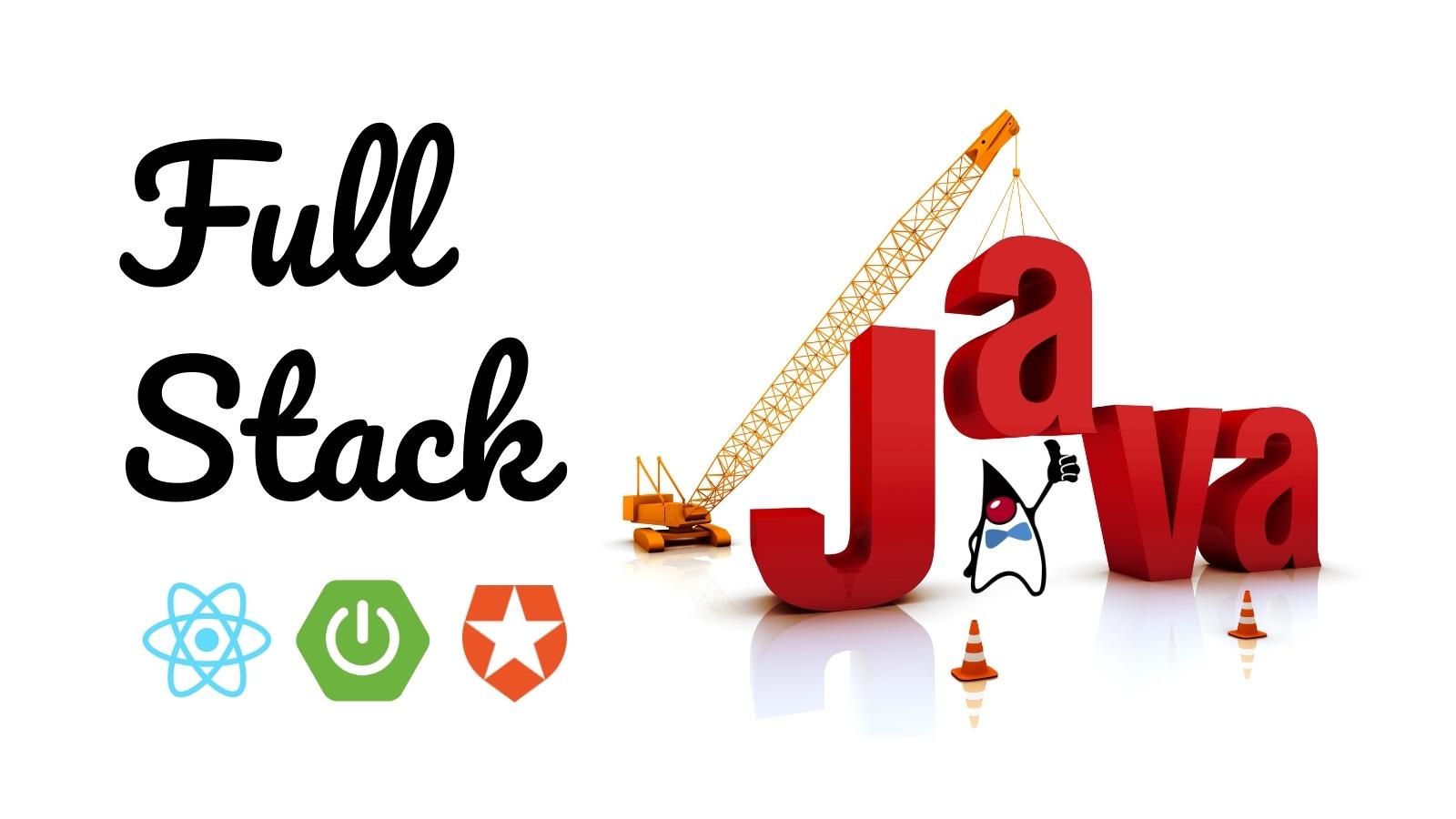
If you search for “Full Stack Java” on the internet, you’ll likely find a lot of recruiting, courses, and jobs. Being a full stack developer can be exciting because you can create the backend and frontend of an app all by yourself. There is business logic and algorithms as well as styling, making things look good, and securing everything. It also pays pretty well. Today, I’m going to show you how you can be a...
Build a Secure SPA with React Routing
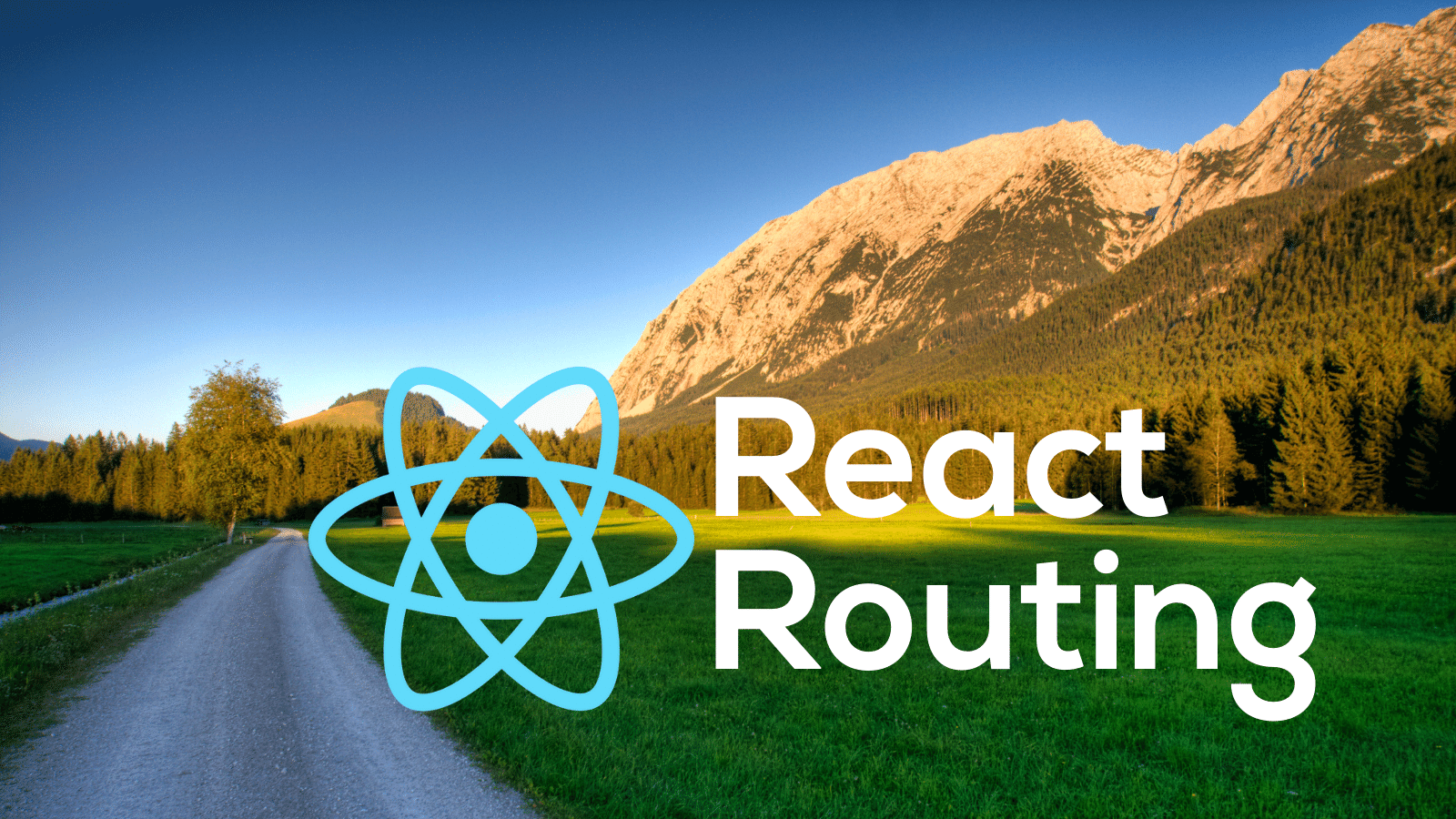
When building an SPA (single page application) with React, routing is one of the fundamental processes a developer must handle. React routing is the process of building routes, determining the content at the route, and securing it under authentication and authorization. There are many tools available to manage and secure your routes in React. The most commonly used one is react-router. However, many developers are not in a situation where they can use the react-router...
Create a Secure Chat Application with Socket.IO and React
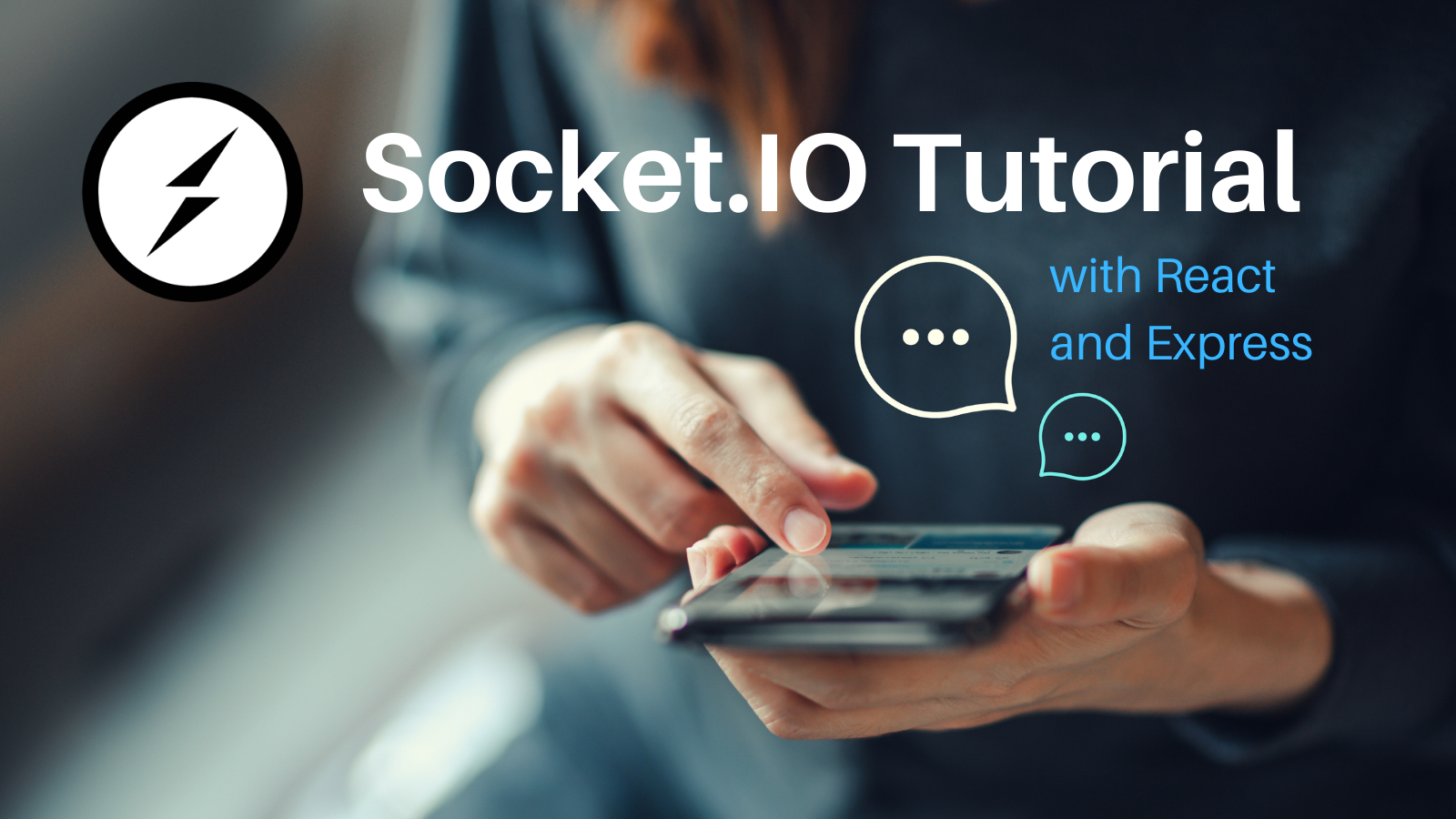
The HTTP protocol powers the web. Traditionally, HTTP is a request-response protocol. This means that a client requests data from a server, and the server responds to that request. In this model, a server will never send data to a client without having been queried first. This approach is suitable for many use cases that the web is used for. It allows loose coupling between clients and servers without the need to keep a persistent...
A Developer's Guide to Session Management in React
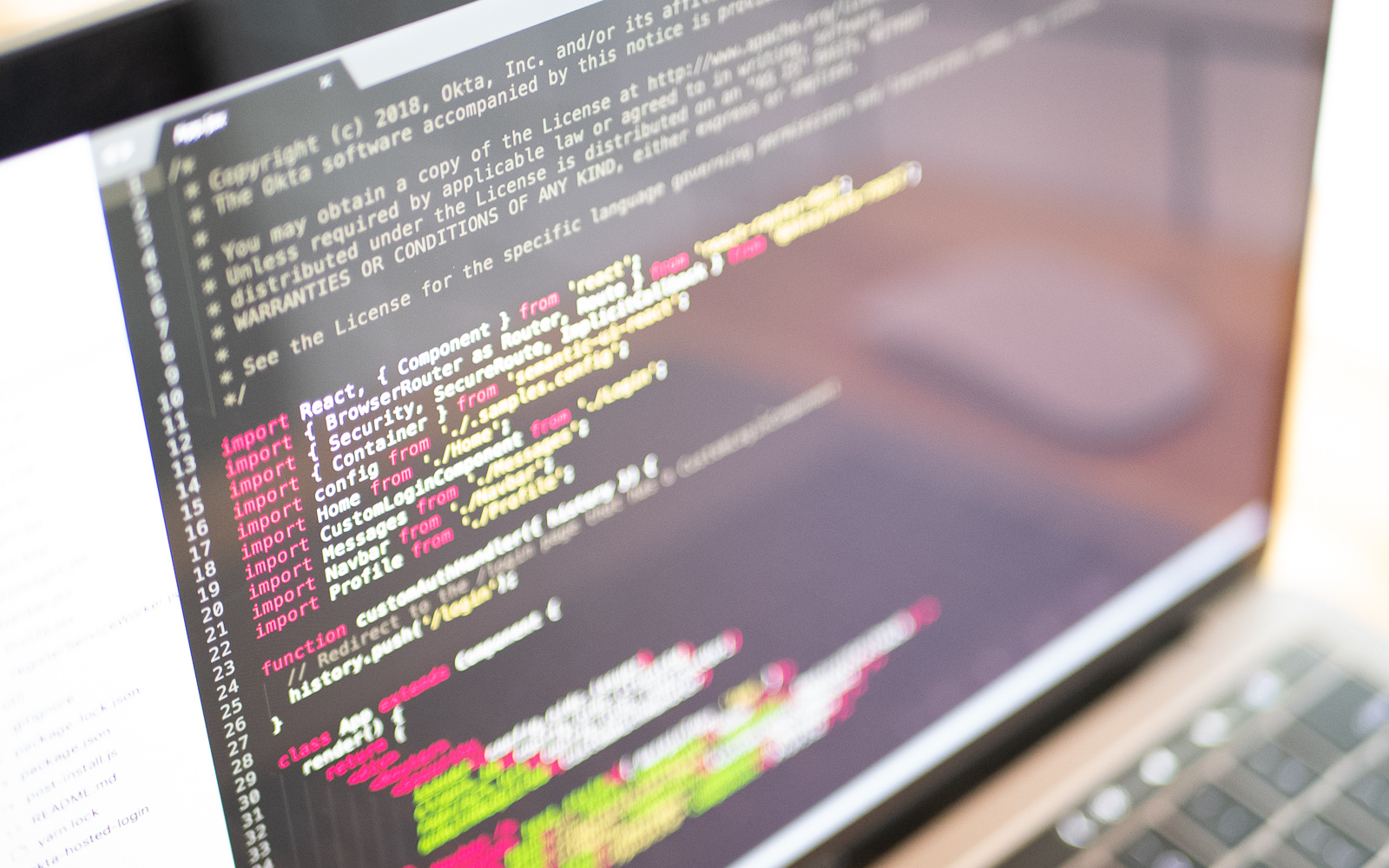
Sessions can be a challenging topic for developers of all skill levels. Many React developers never consider the internals of session management because so much of the work is abstracted away. But, it is important to understand what sessions are, how they work, and how best to manage and manipulate them. There are several different strategies for session management in React. In this article, you will learn the basics about sessions, how to manage them...
Easily Consume a GraphQL API from React with Apollo
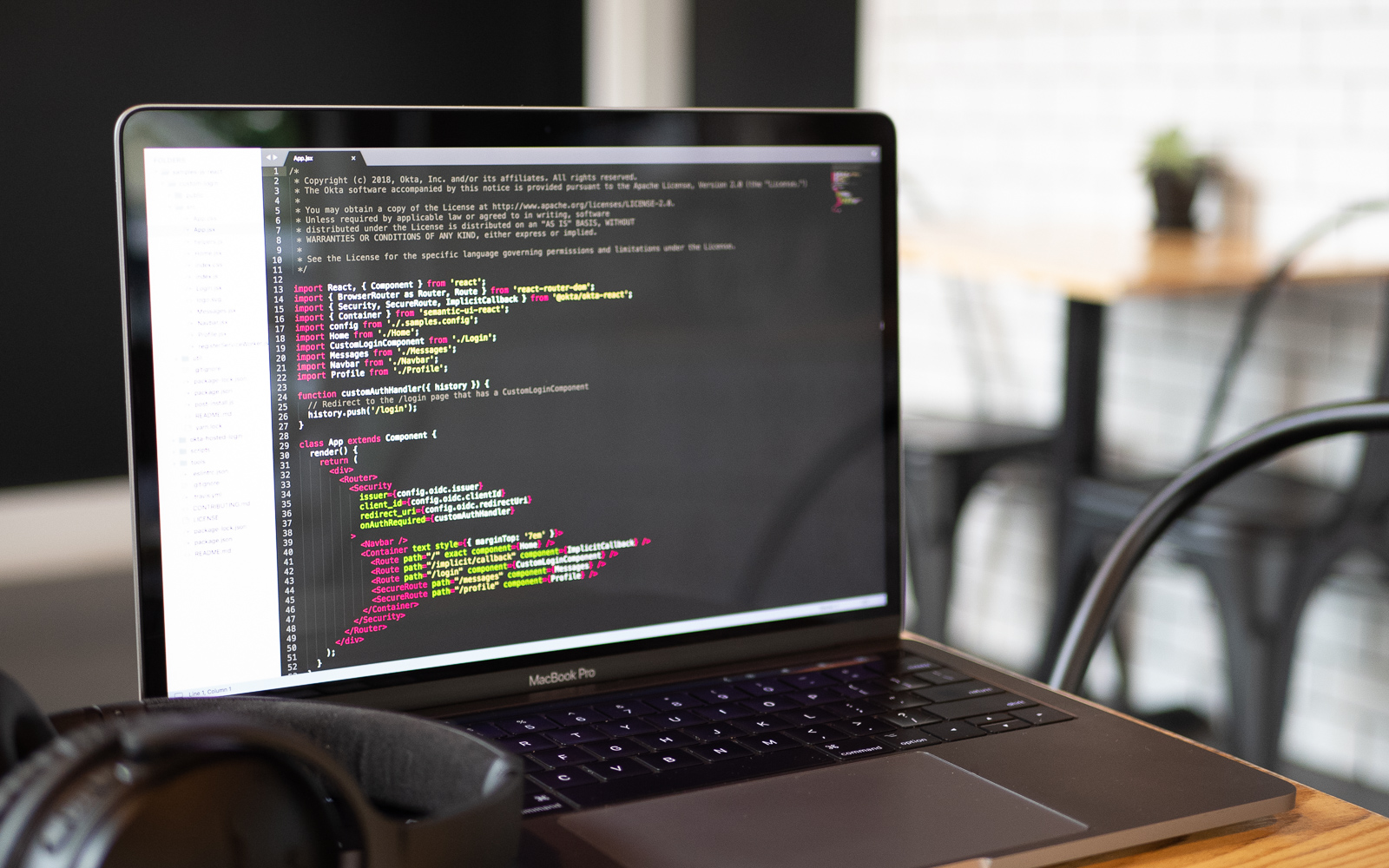
GraphQL is an incredibly powerful query language for APIs that helps improve performance and extensibility in your APIs. The query language is designed to allow developers to query exactly the data they need. As your API grows in size and scope, current consumers are unaffected by changes since their queries should return the same data. Apollo Client is a state management library for JavaScript. It fits seamlessly into React applications and can handle fetching, caching,...
A Quick Guide to React Login Options
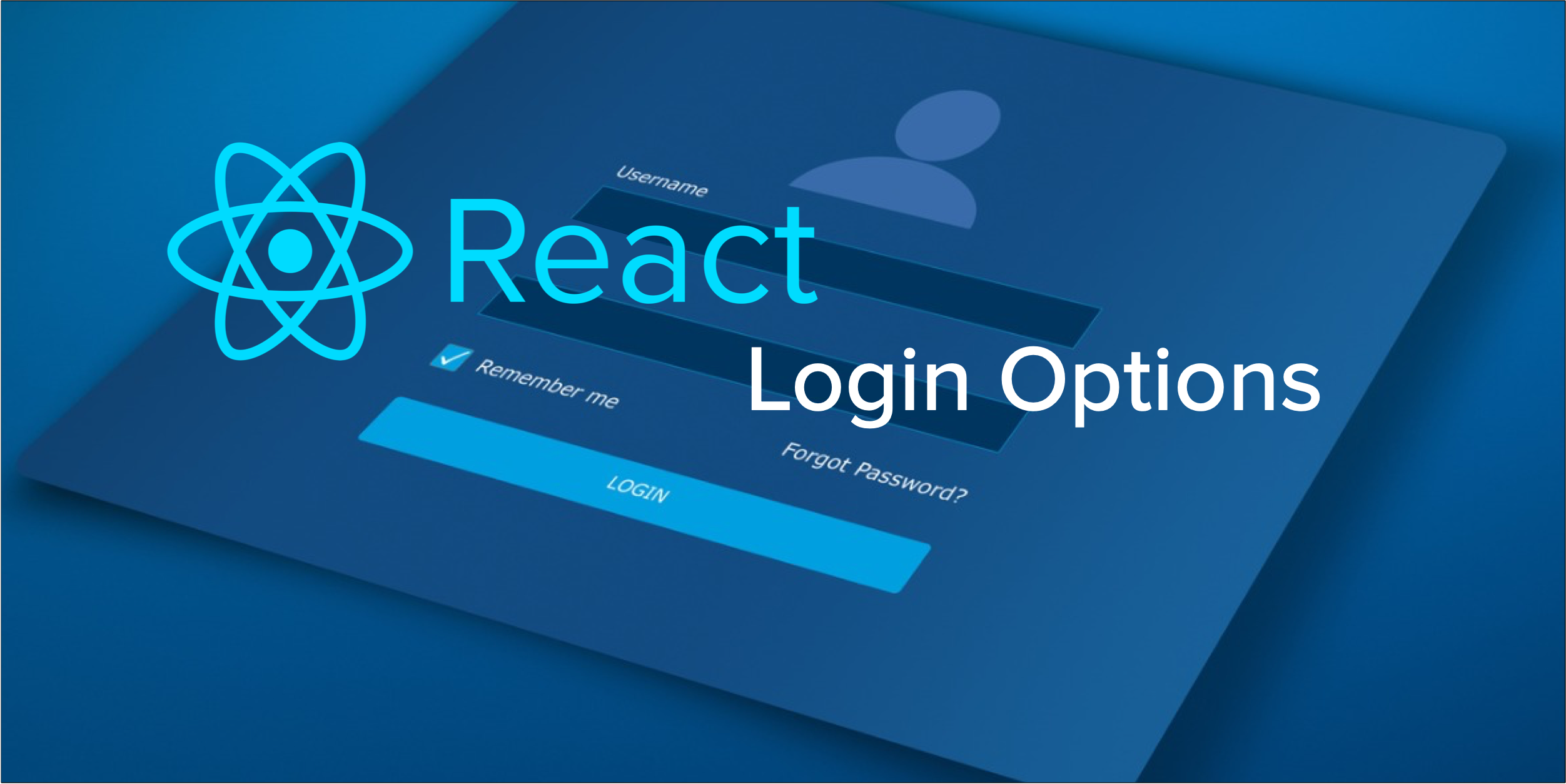
Almost any web app needs some sort of access control, usually implemented by user login. Choosing how user authentication is implemented depends on the type of application and its audience. In this post, I want to show you a few different ways of creating a login feature in a single-page React application using Okta. I will start with a login redirect. This is the easiest option to implement and is a good choice for some...
Quickly Consume a GraphQL API from React
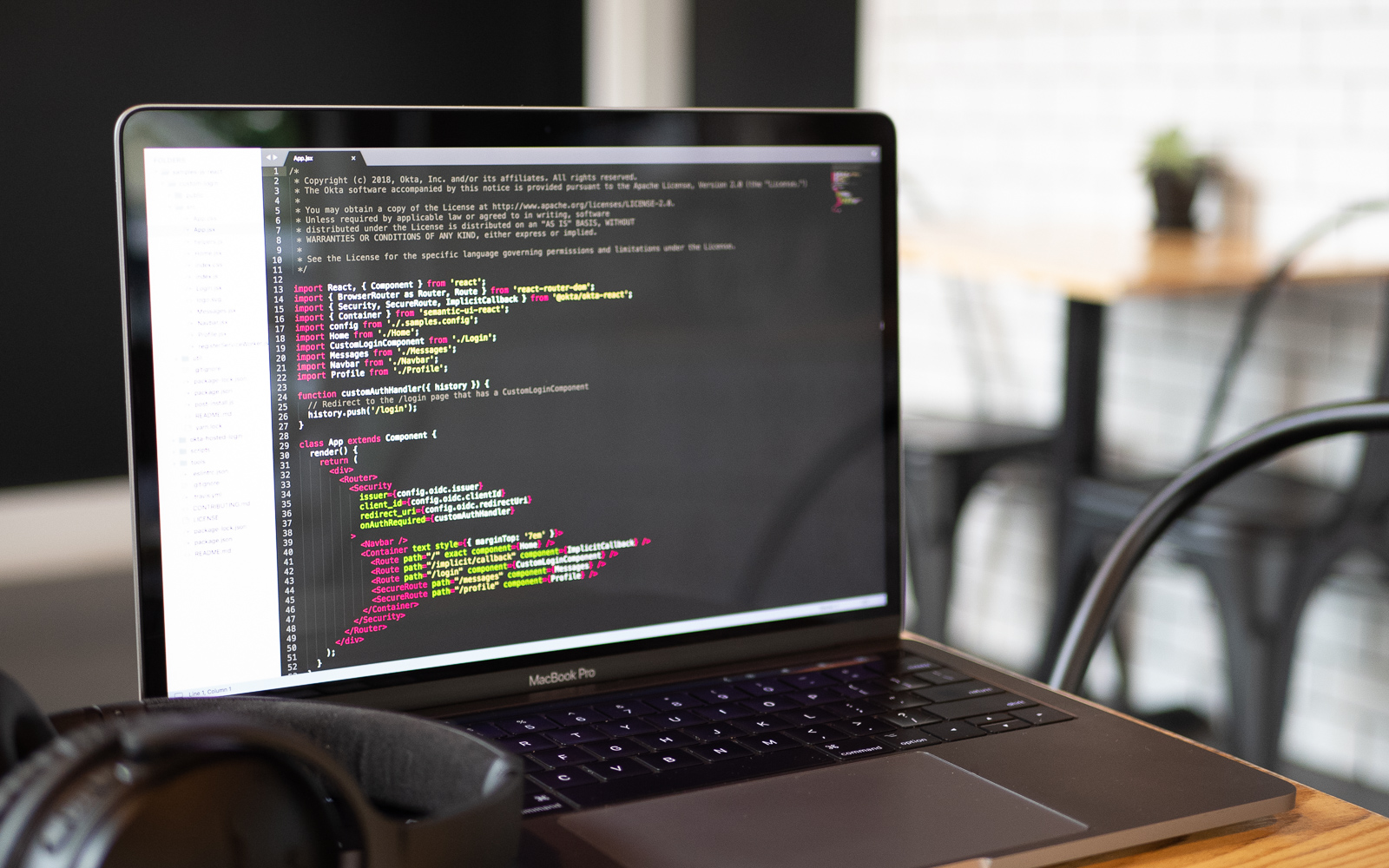
React.js is one of the most popular front-end javascript frameworks today. For most of its life, React has connected to REST APIs to fetch or save data on a server. GraphQL is a query language that aims to replace REST APIs by providing consumers with a clear description of the data in the API. By combining these two technologies, you can quickly connect to an API with your React.js application. There are many packages available...
Build a React App with ANT Design Principles
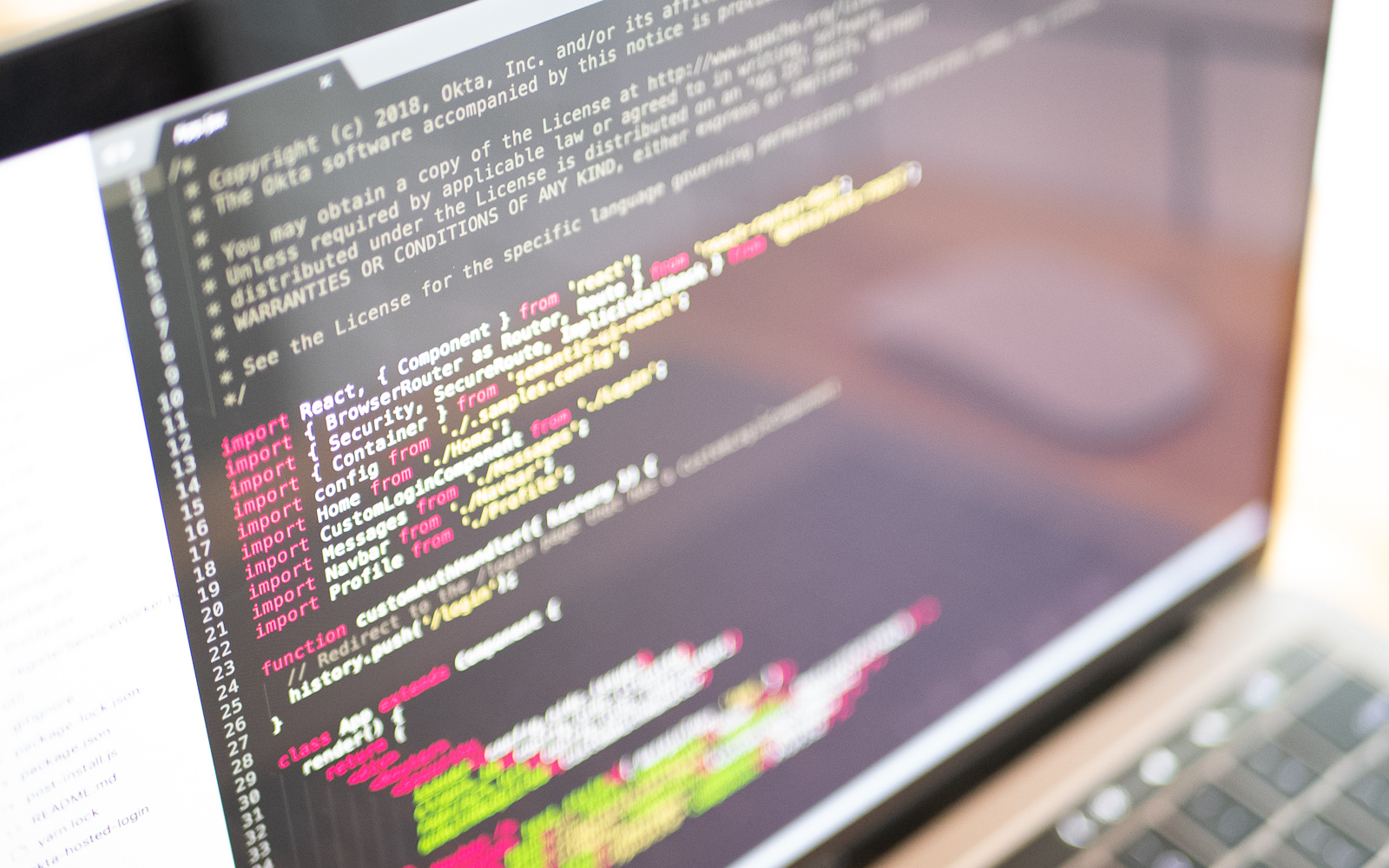
For years the go-to HTML/CSS framework of choice for developers was Bootstrap. A new contender has appeared in the form of Ant Design. Ant should feel familiar to veteran developers but it’s built on new principles. Their site spends a good amount of effort distinguishing between good and bad design. There is an emphasis on clarity and meaning. Ant Design is heavily based on psychological principles to anticipate—and be customized for—user behavior. Ant Design is...
Build a Simple React Application Using Hooks
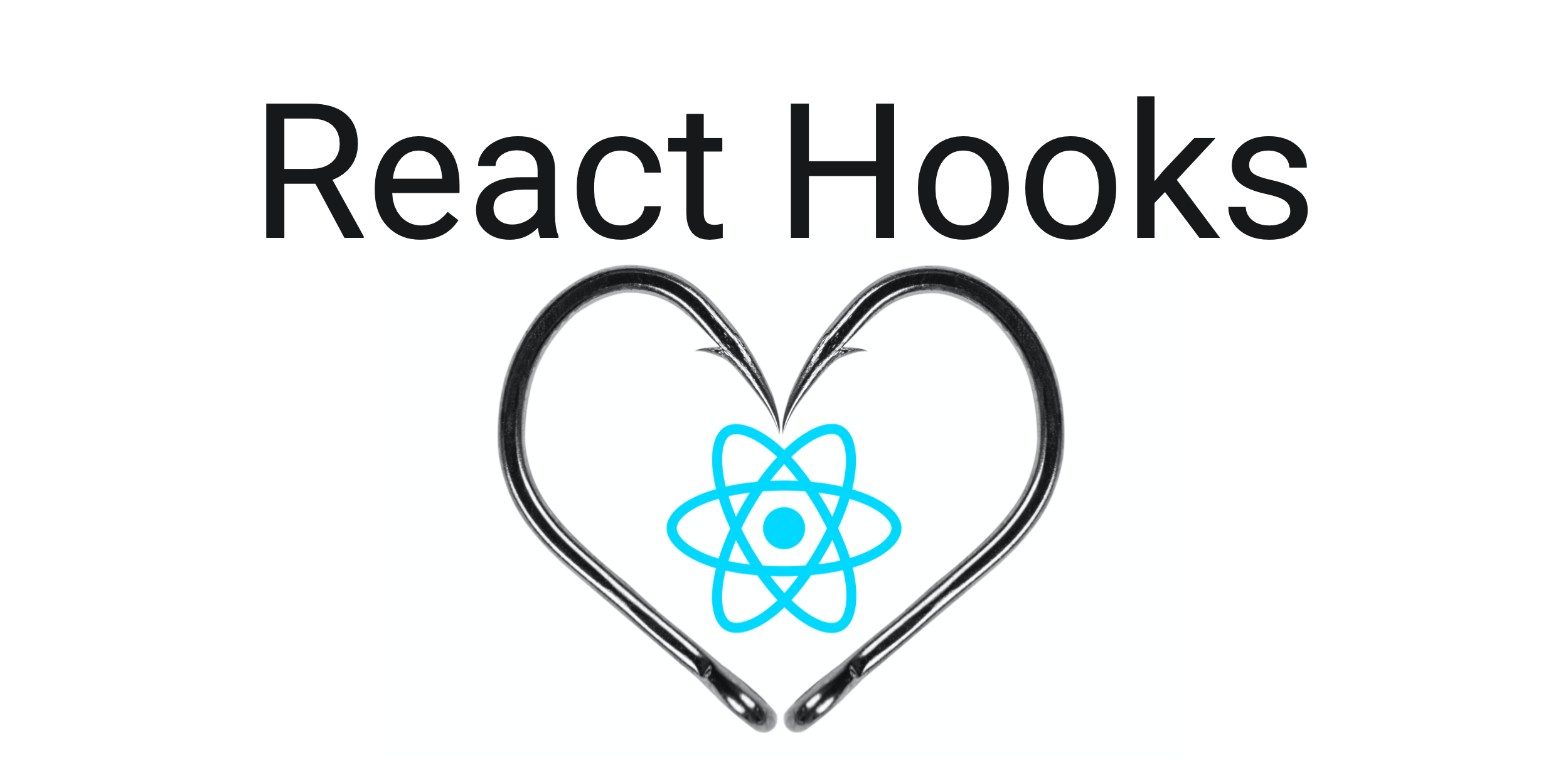
If you have been developing React applications, then you probably know that there are two ways of creating React components. You can create a component class that extends from React.Component. You then have to implement specific methods such as render() that renders the component. The alternative is to create a functional component. This type of component is simply a JavaScript function that returns a rendered element. Functional components are much shorter, they contain less boilerplate...
How to Use CSS Grid to Build a Responsive React App
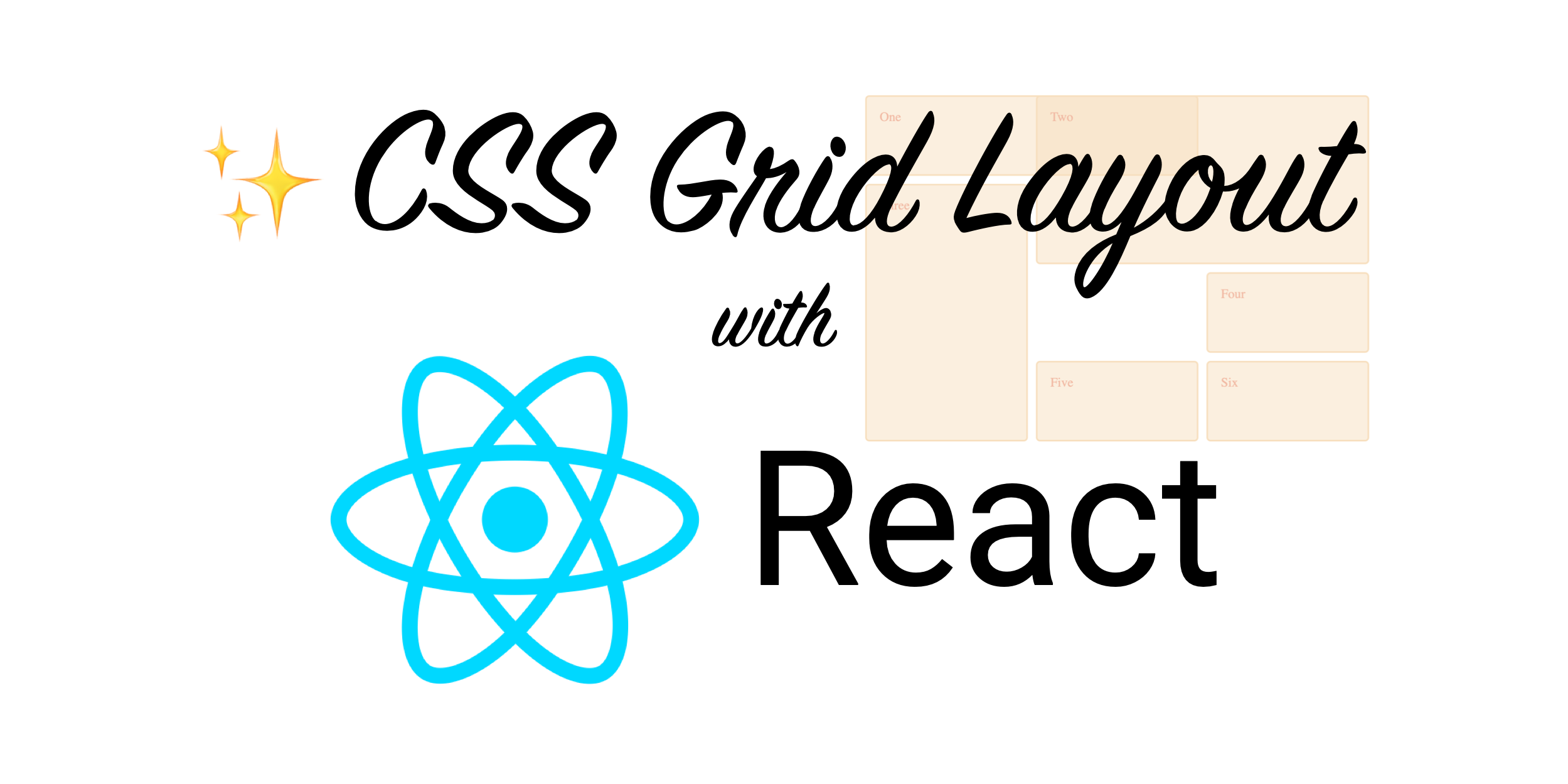
Do you remember the days when people used tables to arrange content on websites? HTML tables were ideal for arranging content in a grid-style layout. But they had a serious problem. They mixed content with style, prohibited semantic markup, and made it hard for screen readers to sort the information in a meaningful way. After dropping the use of tables, the first solution was to use the CSS float to position elements on a web...
Heroku + Docker with Secure React in 10 Minutes
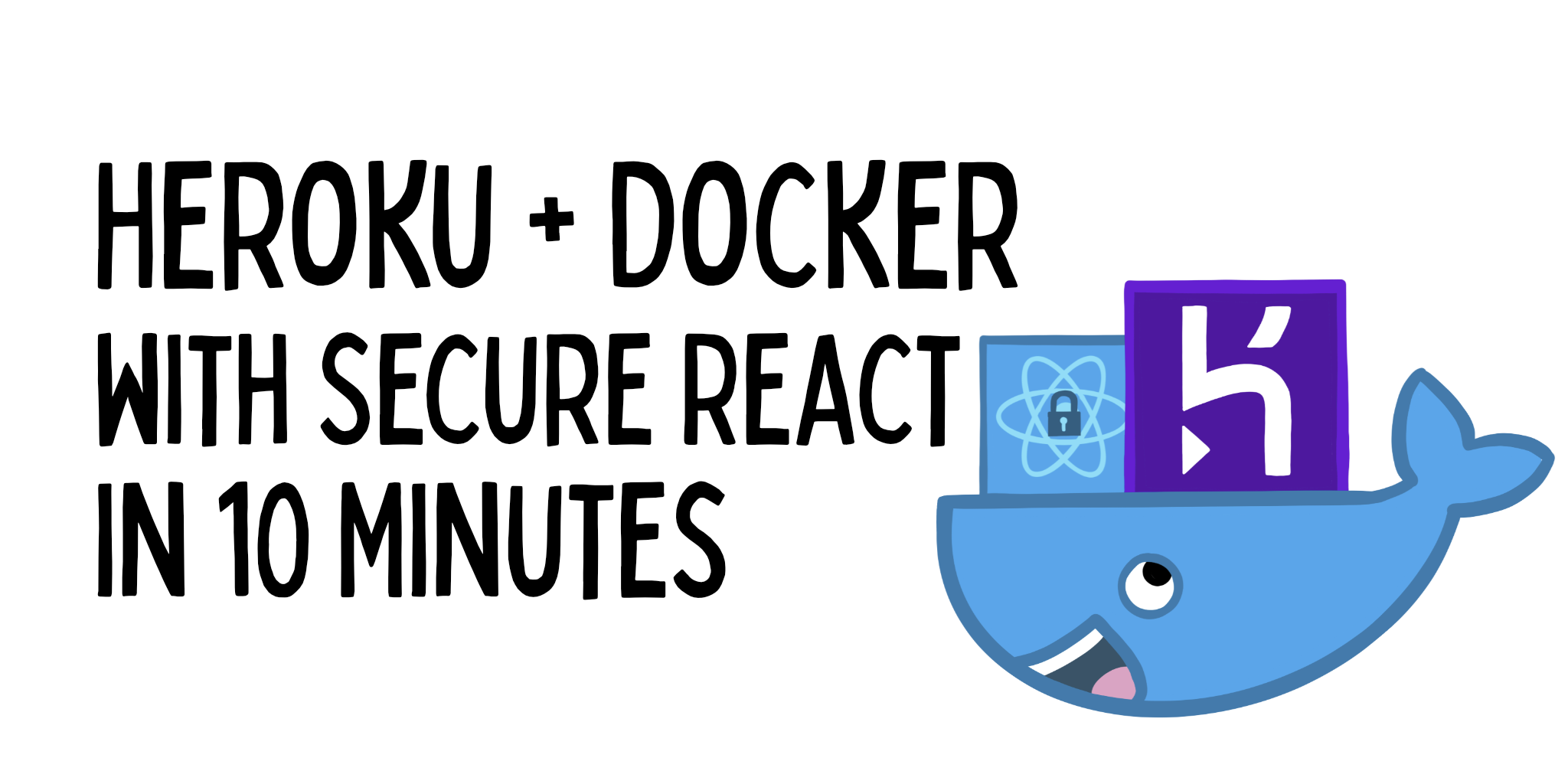
You’ve built a React app, but now you need to deploy it. What do you do? First, it’s probably best to choose a cloud provider as they’re typically low-cost and easy to deploy to. Most cloud providers offer a way to deploy a static site. Heroku supports static sites, easily deploys apps with Git, and provides a CLI that developers love. A built React app is just JavaScript, HTML, and CSS. They’re static files that...
How to Move from Consuming Higher-Order Components to React Hooks
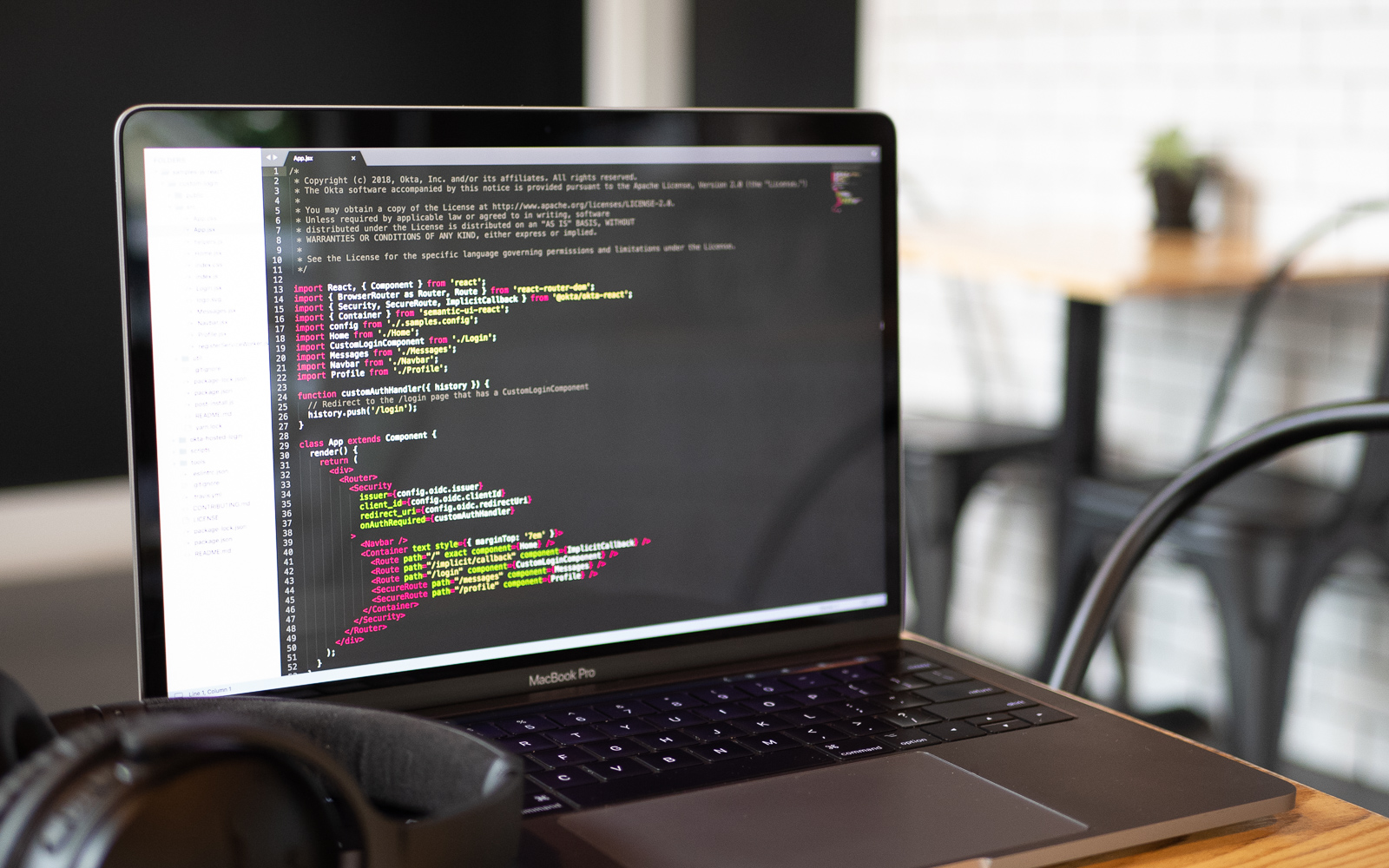
Let’s face it. Higher-Order Components (HOCs) allow developers to really take advantage and extend the functionality of their React components, but they can be cumbersome once you have more than one that you want to use within a component. It’s mostly because of the way they’re used. Not only do you have to use a class component in order, but you also use the HOC by wrapping them around your components. Wrapping the component code...
Build a React App with Styled Components
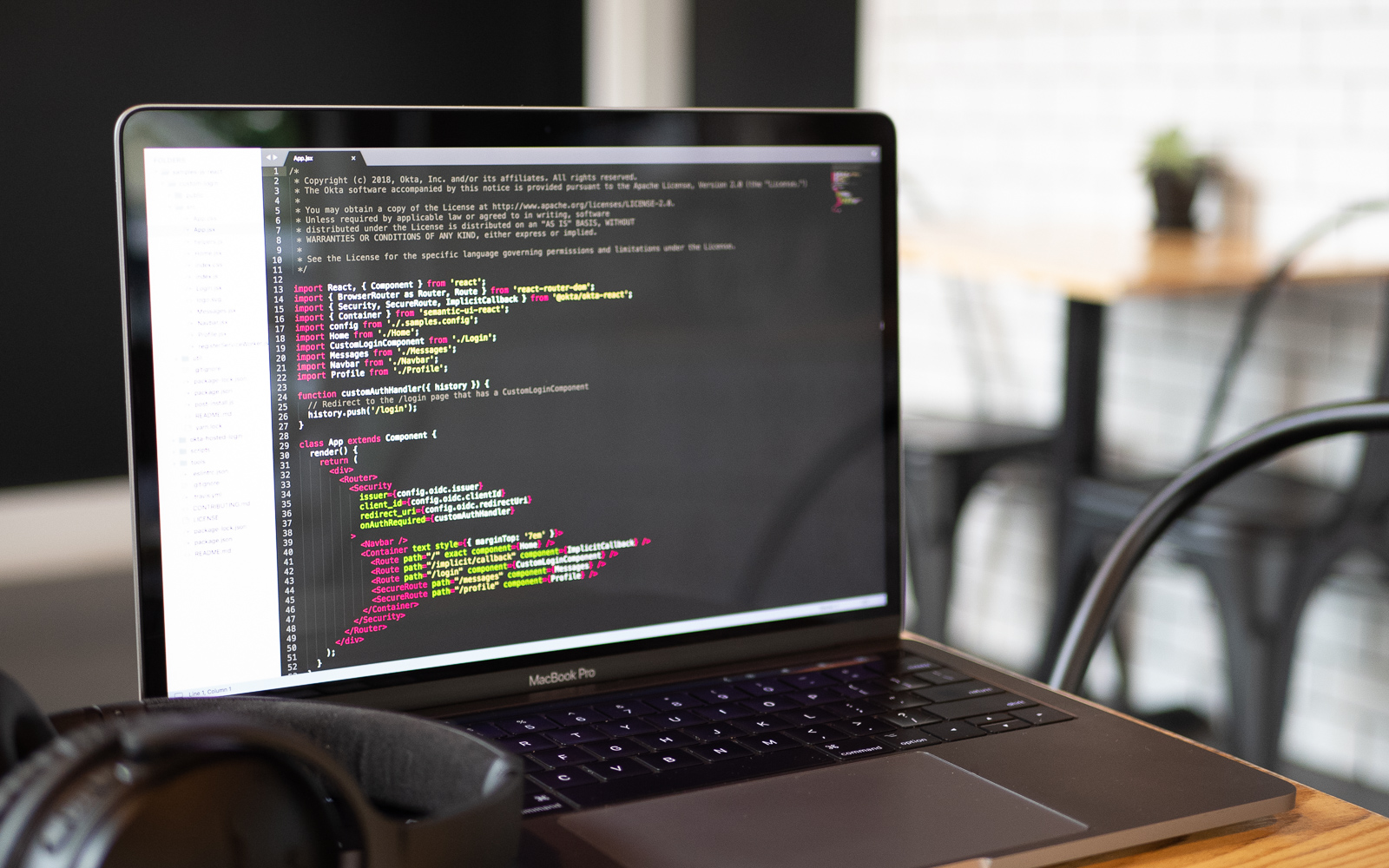
When you create React applications, at some point you have to make a decision on how to organize your CSS styles. For larger applications, you’ll have to modularize the stylesheets. Tools such as Sass and Less let you divide up your styles into separate files and provide lots of other features that make writing CSS files more productive. But some problems remain. The tools separate your styles from your components, and keeping the styles up-to-date...
Build a Secure Blog with Gatsby, React, and Netlify
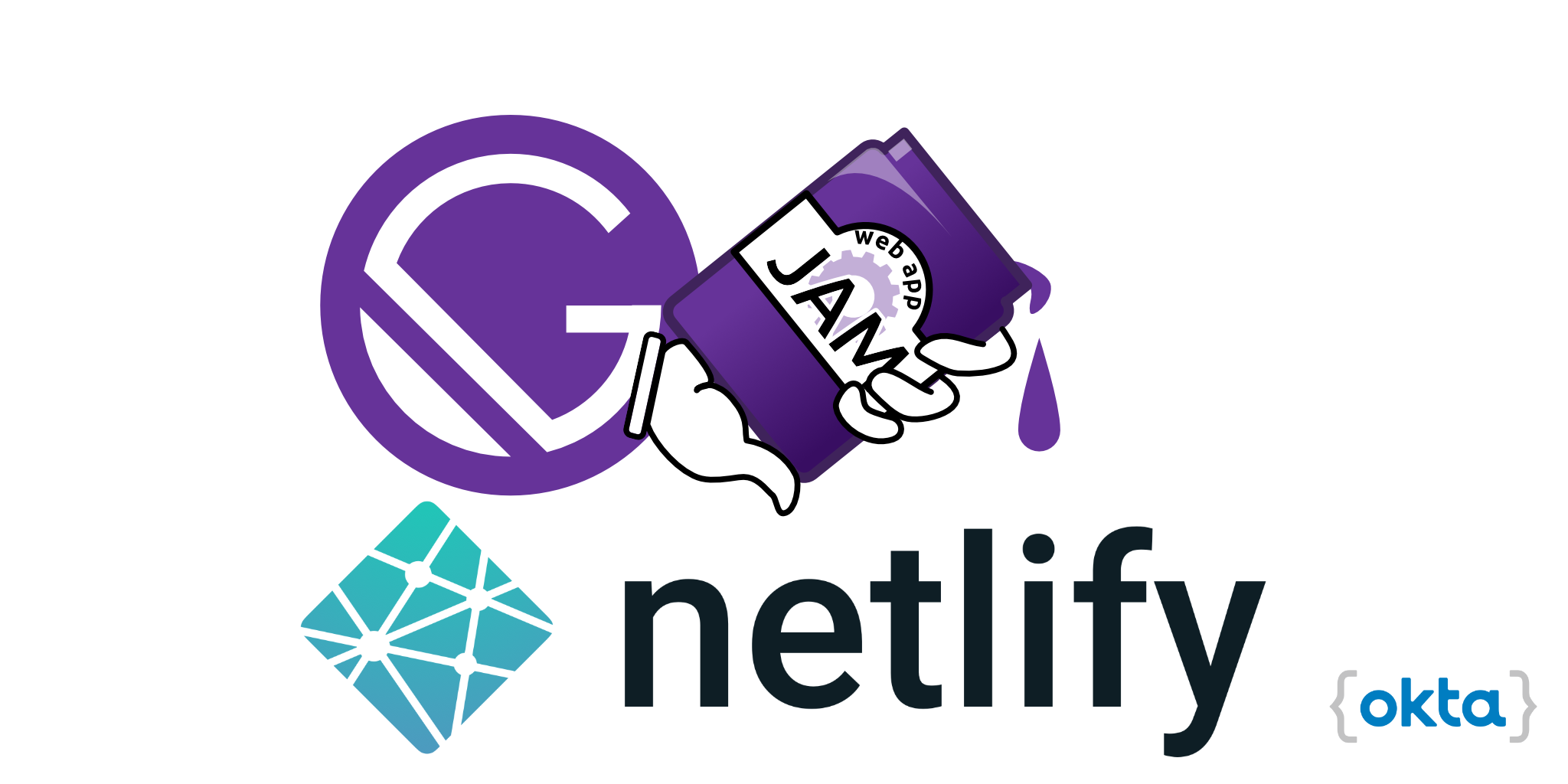
Gatsby is a tool for creating static websites with React. It allows you to pull your data from virtually anywhere: content management systems (CMSs), Markdown files, APIs, and databases. Gatsby leverages GraphQL and webpack to combine your data and React code to generate static files for your website. JAM - JavaScript, APIs, and Markup - apps are delivered by pre-rendering files and serving them directly from a CDN, removing the requirement to manage or run...
Build Reusable React Components
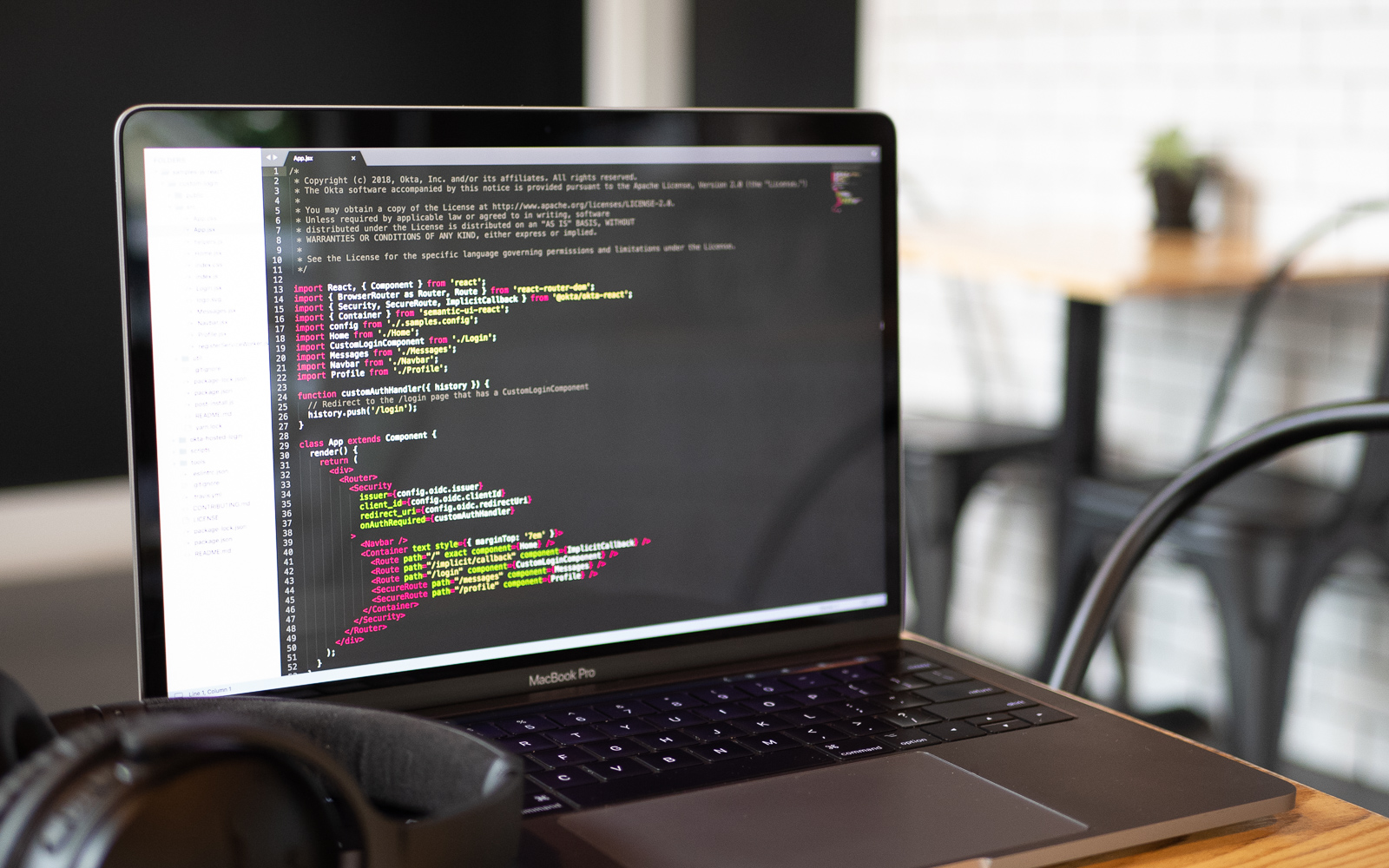
Who doesn’t love beer? When you drink a great beer you want to tell someone. You definitely want to be able to remind yourself of the great beers you’ve had. Enter Brewstr, a beer rating application that allows you to enter a beer you’re drinking and give it a rating. This way, you know what to get next time since there’s no way you’ll remember it later. React gives the ability to create a component...
Build a CRUD Application with Kotlin and React
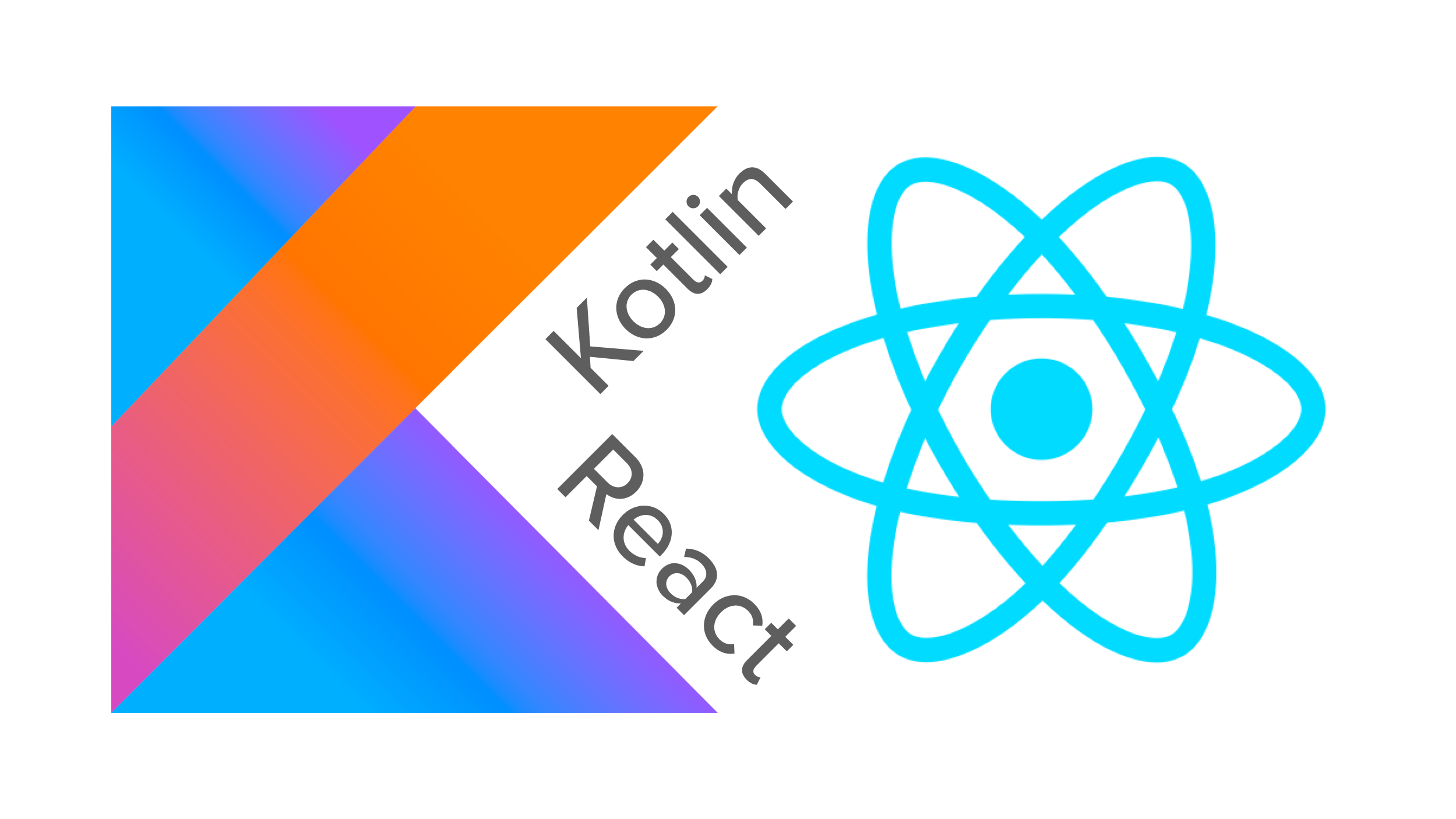
In this tutorial, you’re going to build a client and server application using React for the frontend and Kotlin with Spring Boot for the backend. You’ll first build the app unsecured before securing it using Okta. To secure the React frontend, you’ll use OAuth 2.0 login, and for the backend, you’ll use a JSON Web Token and Spring Boot’s resource server OAuth implementation. This tutorial covers a lot of ground. It also uses a lot...
Use Sass with React to Build Beautiful Apps
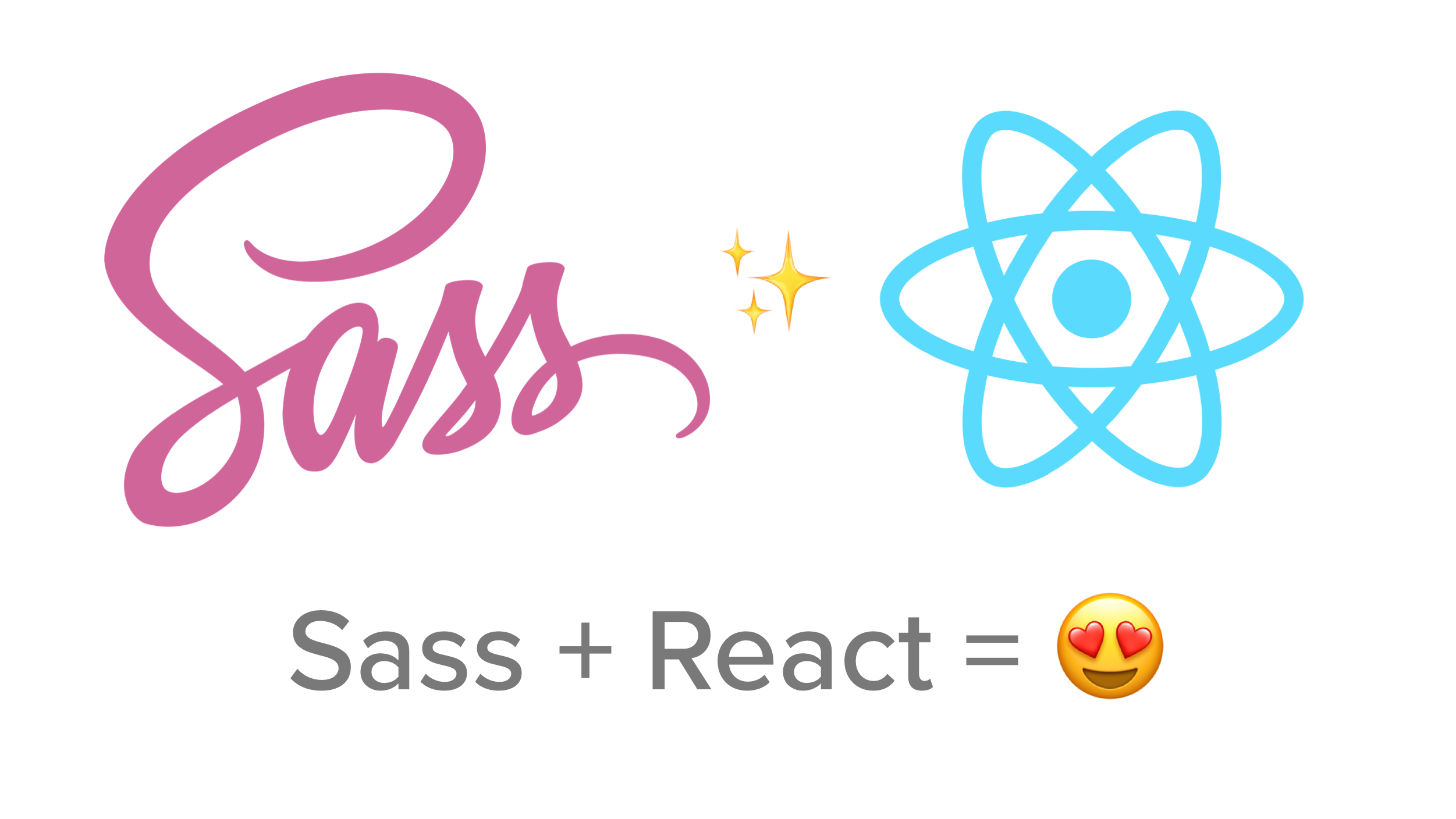
When you are developing web applications with React, you know that writing the JavaScript code is only half of the story. The other half is implementing the design using style sheets. When your application becomes larger, using plain CSS style sheets can become tedious and unmaintainable. Sass is one of the most popular alternatives to CSS. It extends the CSS language with variables, mixins, and many other features. It also lets you divide up the...
A Quick Guide to Integrating React and GraphQL
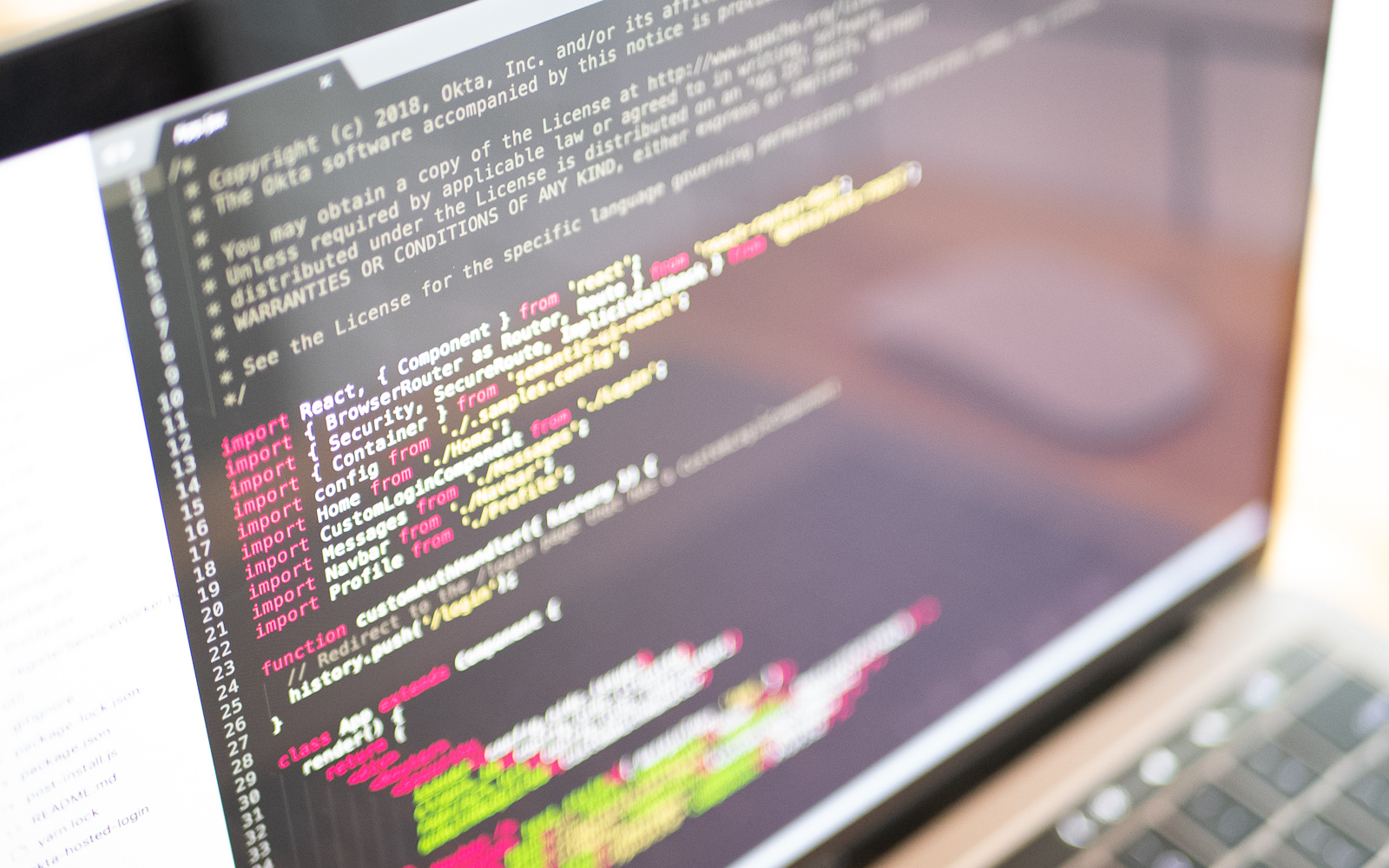
If your application consumes a ReST API from React, the default setup will give you ALL the data for a resource. But if you want to specify what data you need, GraphQL can help! Specifying exactly the data you want can reduce the amount of data sent over the wire, and the React applications you write can have less code filtering out useless data from data you need. There are a lot of GraphQL clients...
Create a React Native App with Login in 10 Minutes
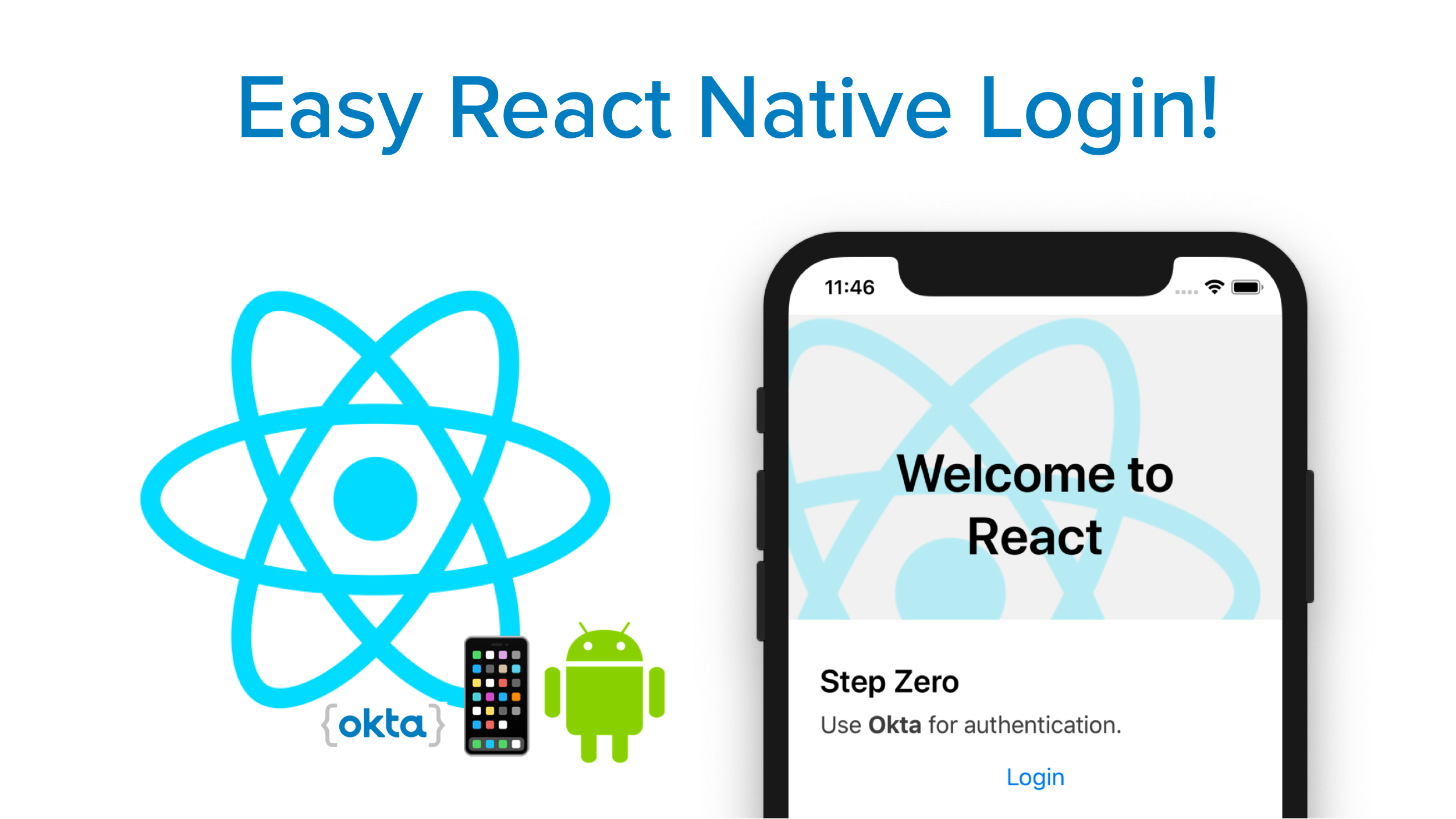
React Native is a mobile app development framework that allows you to use React to build native iOS and Android mobile apps. Instead of using a web view and rendering HTML and JavaScript, it converts React components to native platform components. This means you can use React Native in your existing Android and iOS projects, or you can create a whole new app from scratch. In this post, I’ll show you how to add a...
JWTs in React for Secure Authentication
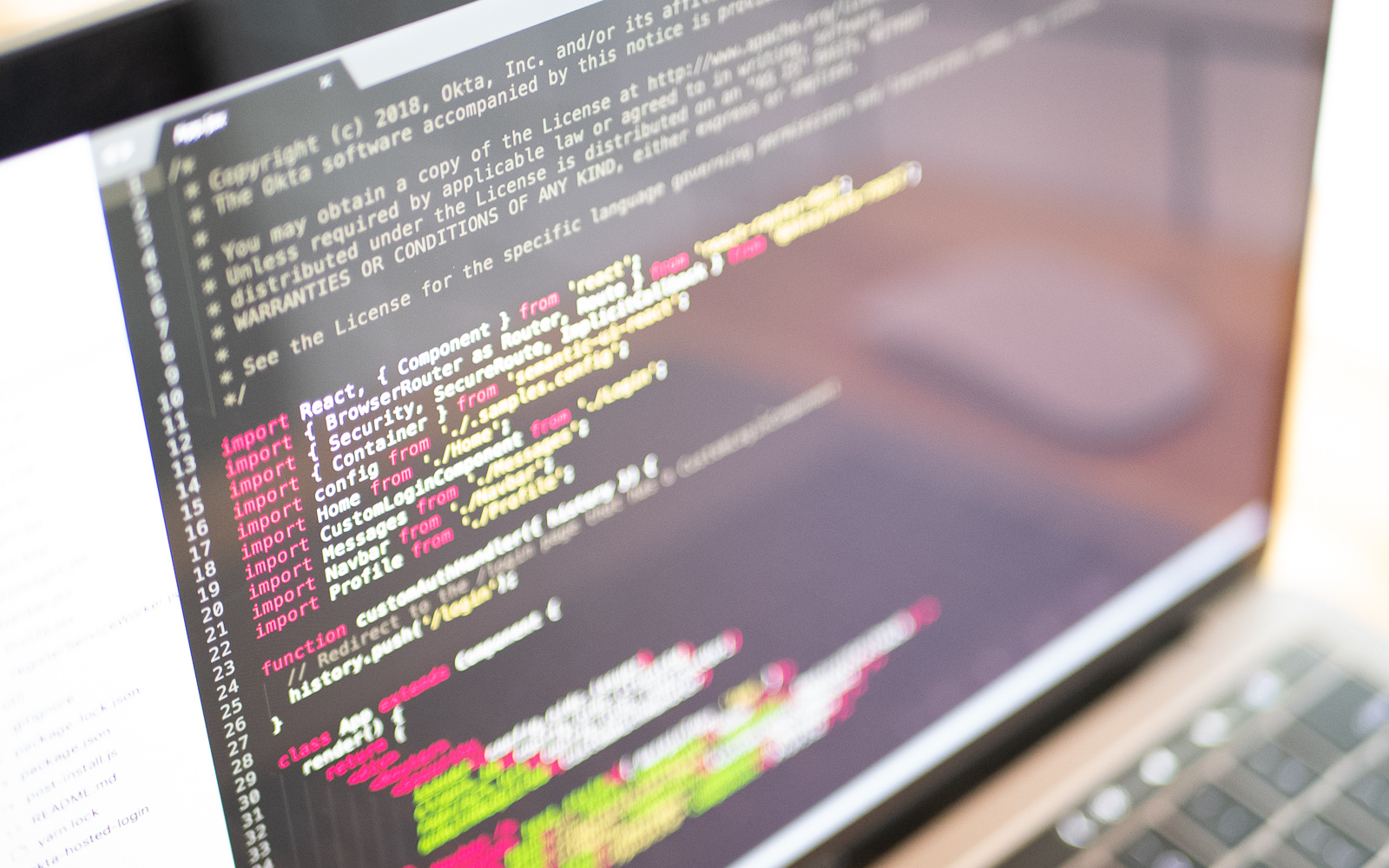
Although authentication is a common requirement for web apps, it can be difficult to get it right, especially if you’re by yourself or part of a small team. That’s why many sites choose to use OAuth 2.0 to let a third-party handle authentication for them. They just need to know how to decode a JSON Web Token (JWT), rather than how to store a bunch of user information and pray they aren’t the next company...
Build a Secure React Application with JWTs and Redux
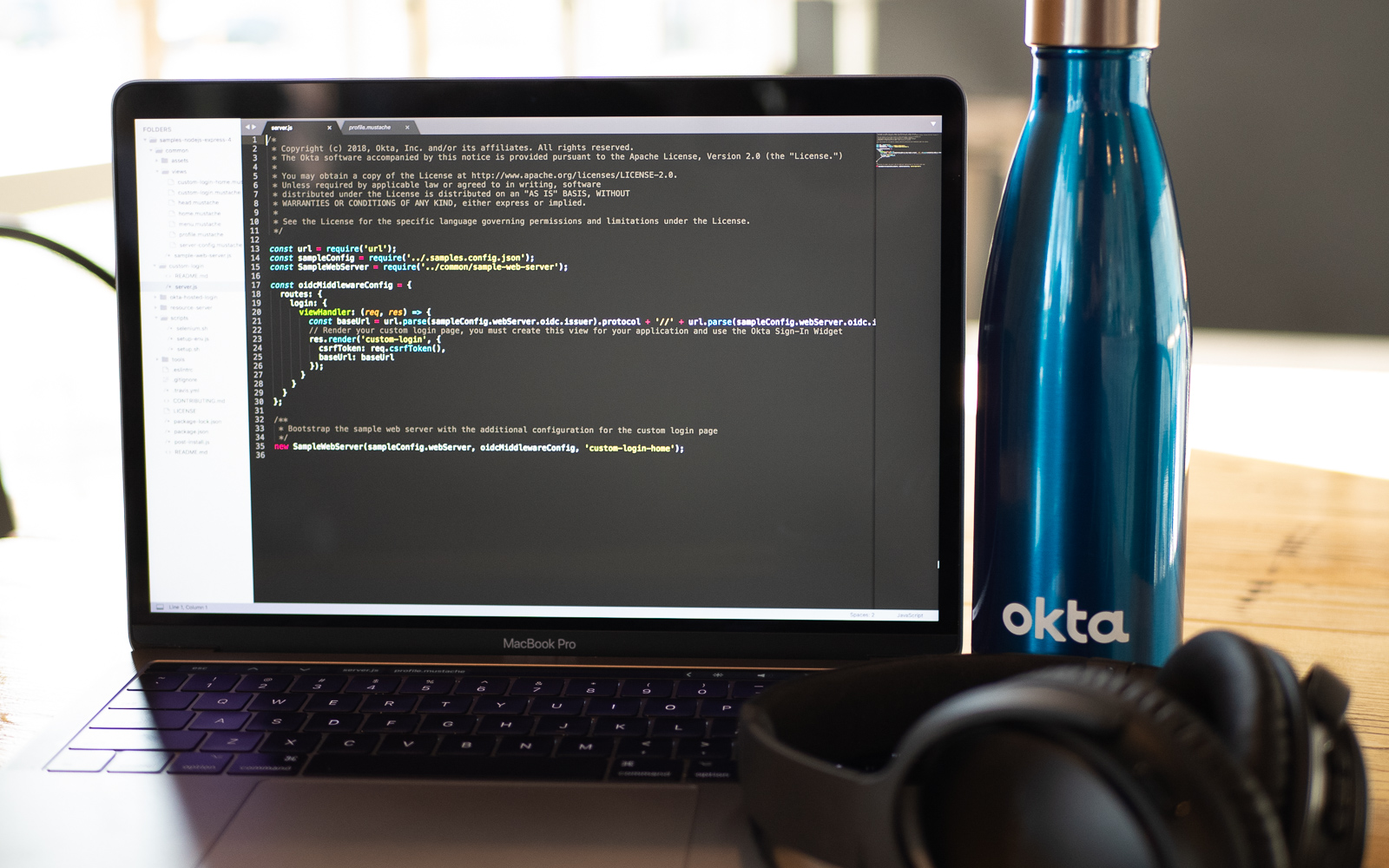
If you’ve worked with React at all, chances are you’ve at least heard of Redux. But you may not know what it is, how it fits in with React, or how to use it in your app. It can sometimes be complicated to set up but can be a very useful addition to a React app depending on your use cases. And if you’ve done much work with web apps, you also probably know how...
A Beginner's Guide to Redux
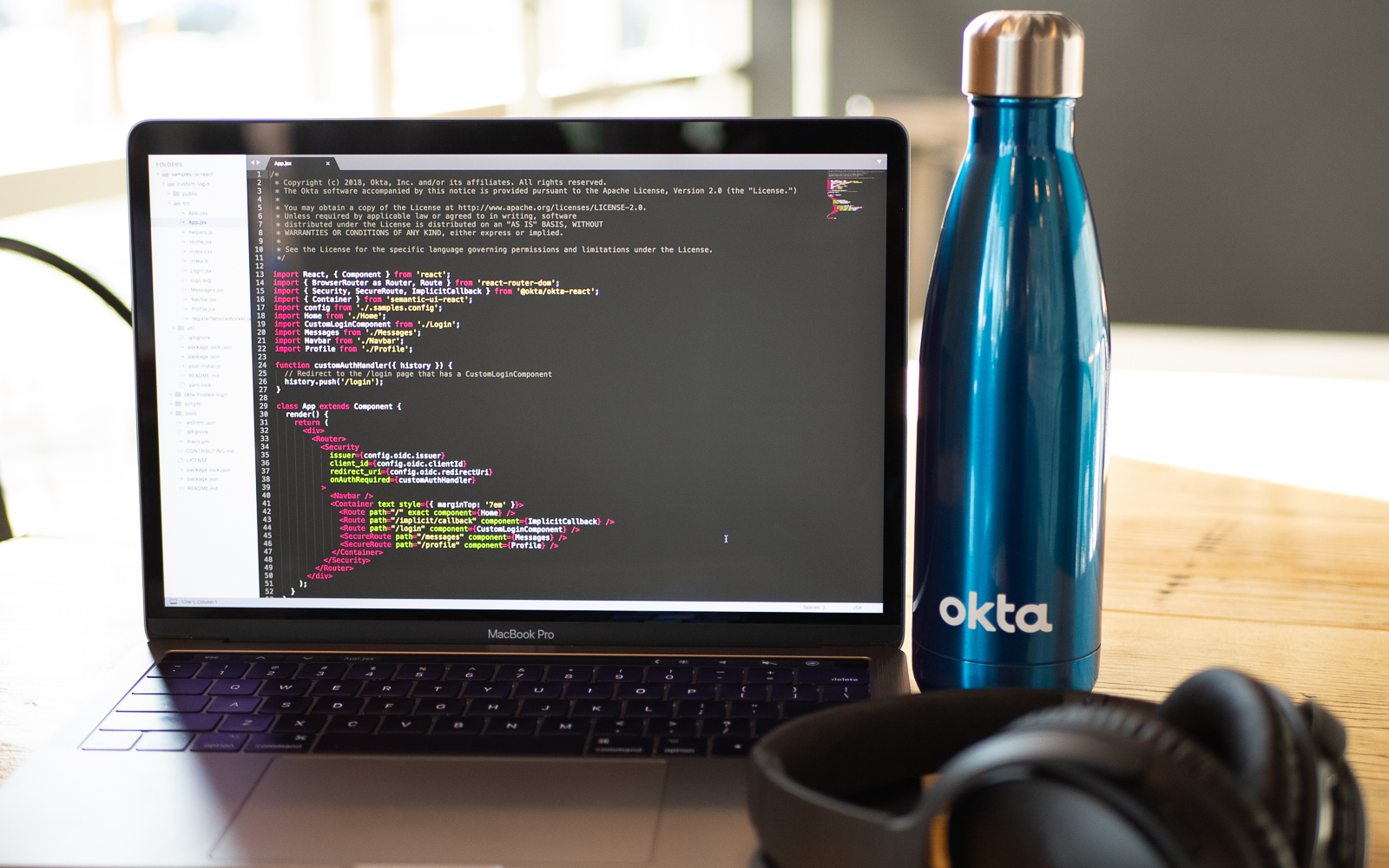
React has gained a lot of popularity over the last few years, and Redux is a term often heard in combination with it. While technically separate concepts, they do work quite nicely together. React is a component-based framework, often used to create a Single-Page App (SPA), but can also be used to add any amount of independent components to any website. Redux is a state management system with a great set of dev tools useful...
Simple User Authentication in React
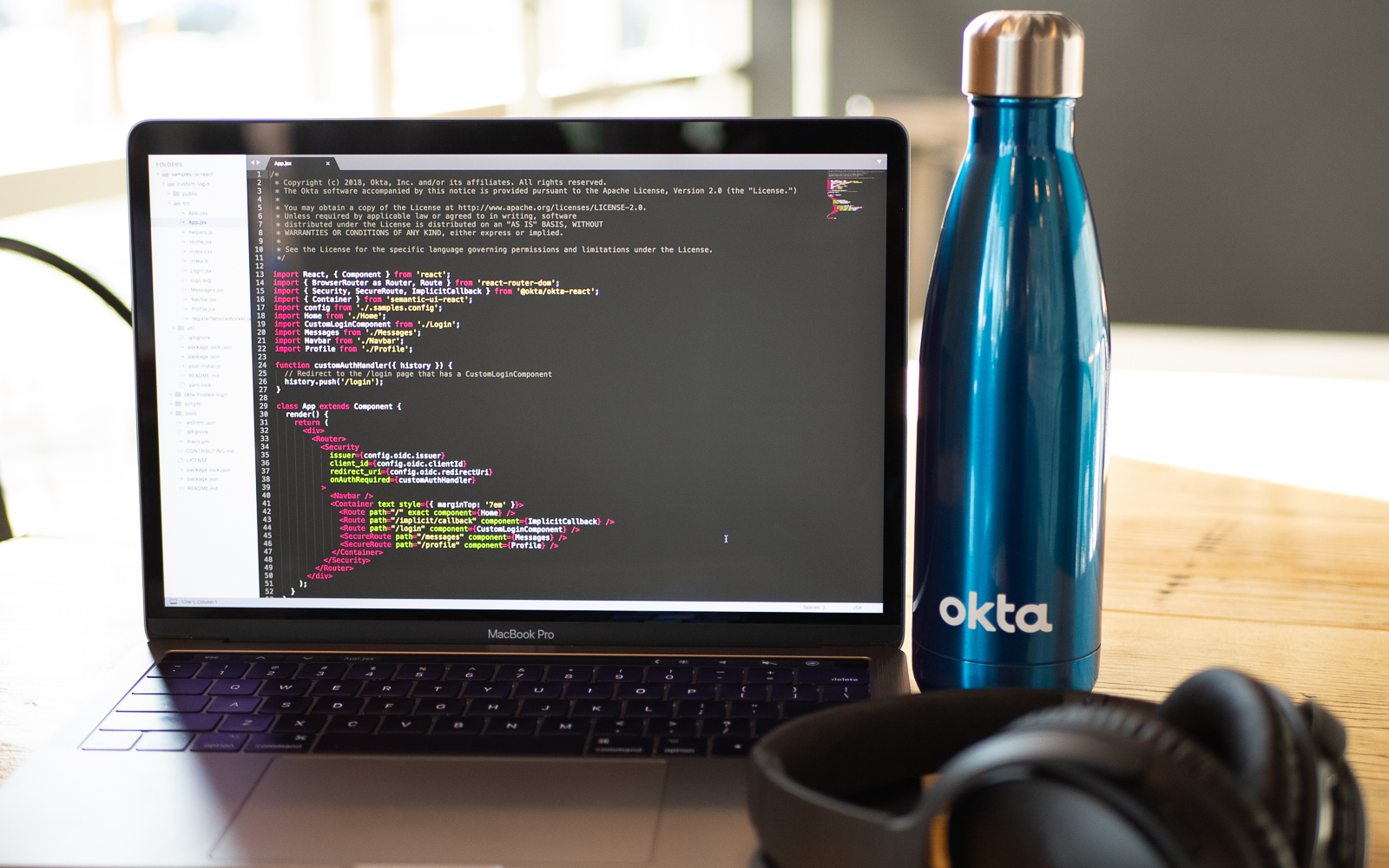
In 2019, it’s quite easy to find React components for pretty much everything. For example, if you want to add user authentication to your app, you can do so easily with Okta’s React component. Here I’ll walk you through creating a simple, fun React app that fetches random Chuck Norris jokes. I’ll then show you how you can add user authentication and customize your user experience, so the jokes will replace Chuck Norris’ name with...
Use Schematics with React and Add OpenID Connect Authentication in 5 Minutes
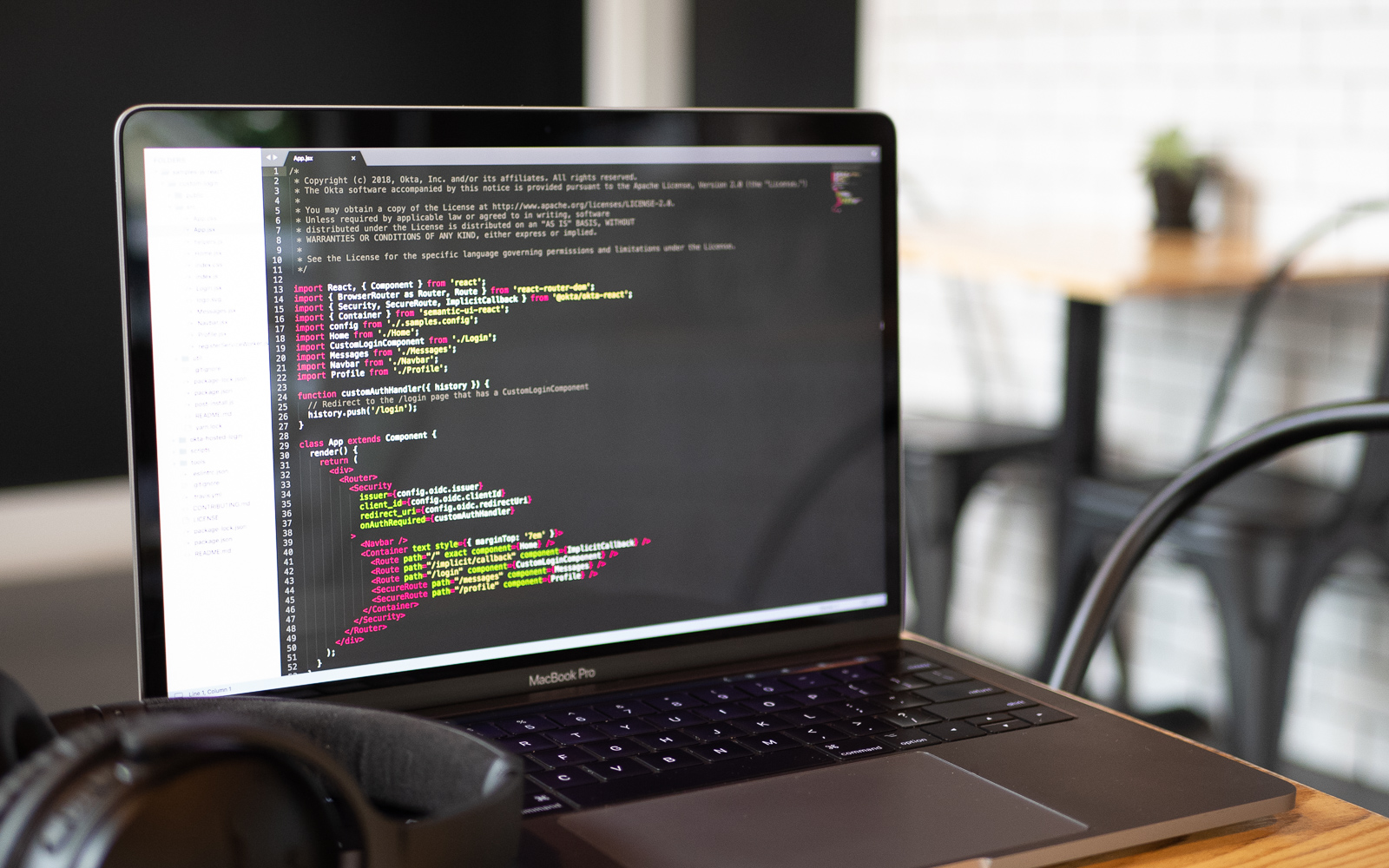
Developers love to automate things. It’s what we do for a living for the most part. We create programs that take the tediousness out of tasks. I do a lot of presentations and live demos. Over the past year, I’ve noticed that some of my demos have too many steps to remember. I’ve written them down in scripts, but I’ve recently learned it’s much cooler to automate them with tools powered by Schematics! Schematics is...
Build Simple Login in PHP
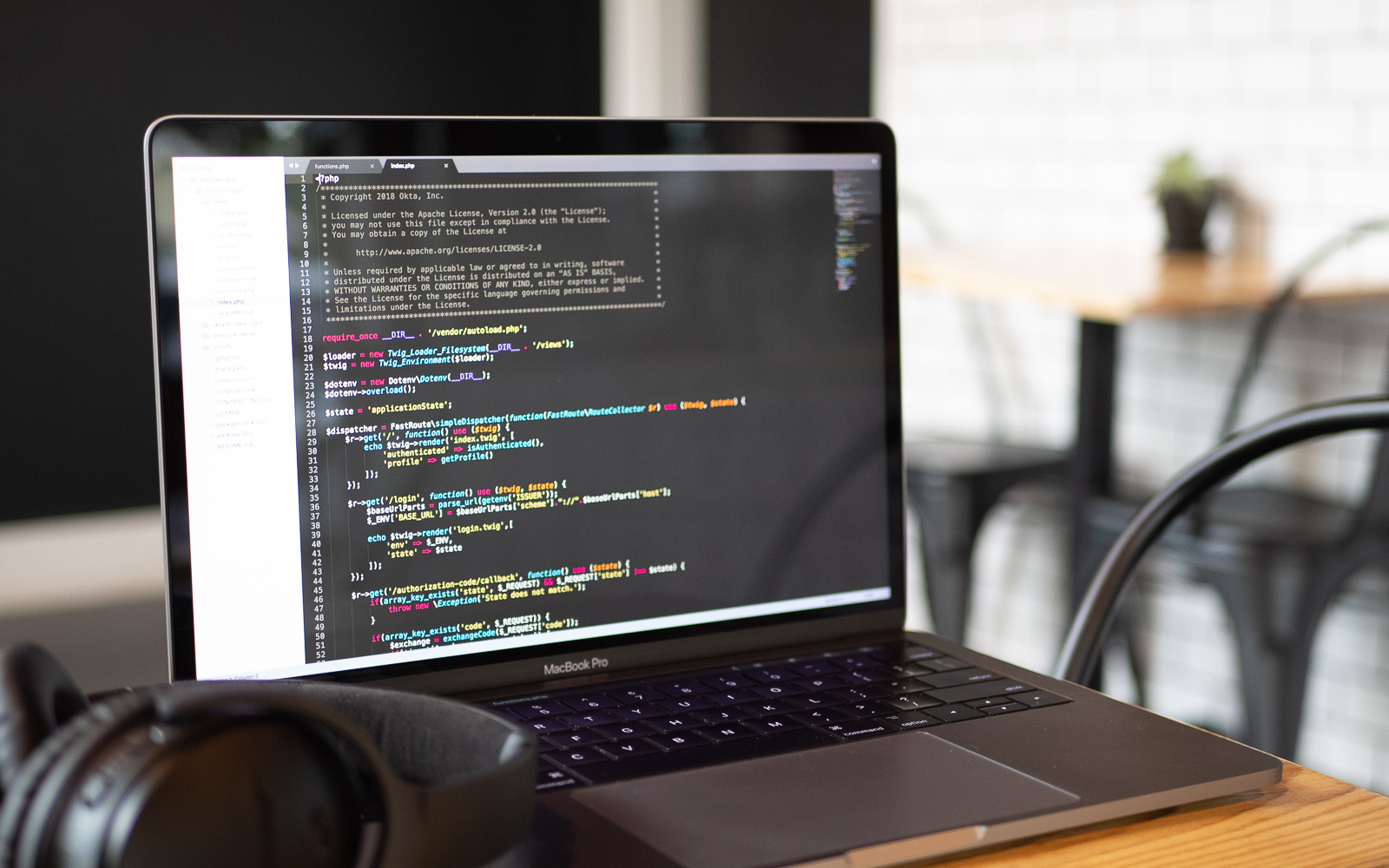
Building a user authentication system for your Web application from scratch can be a deceptively tricky job. It seems easy at first, but there are so many details you have to consider - hashing the passwords properly, securing the user sessions, providing a way to reset forgotten passwords. Most modern frameworks offer boilerplate code for dealing with all of these issues but even if you’re not using a framework, do not despair. In this article,...
Build a Simple CRUD App with Python, Flask, and React
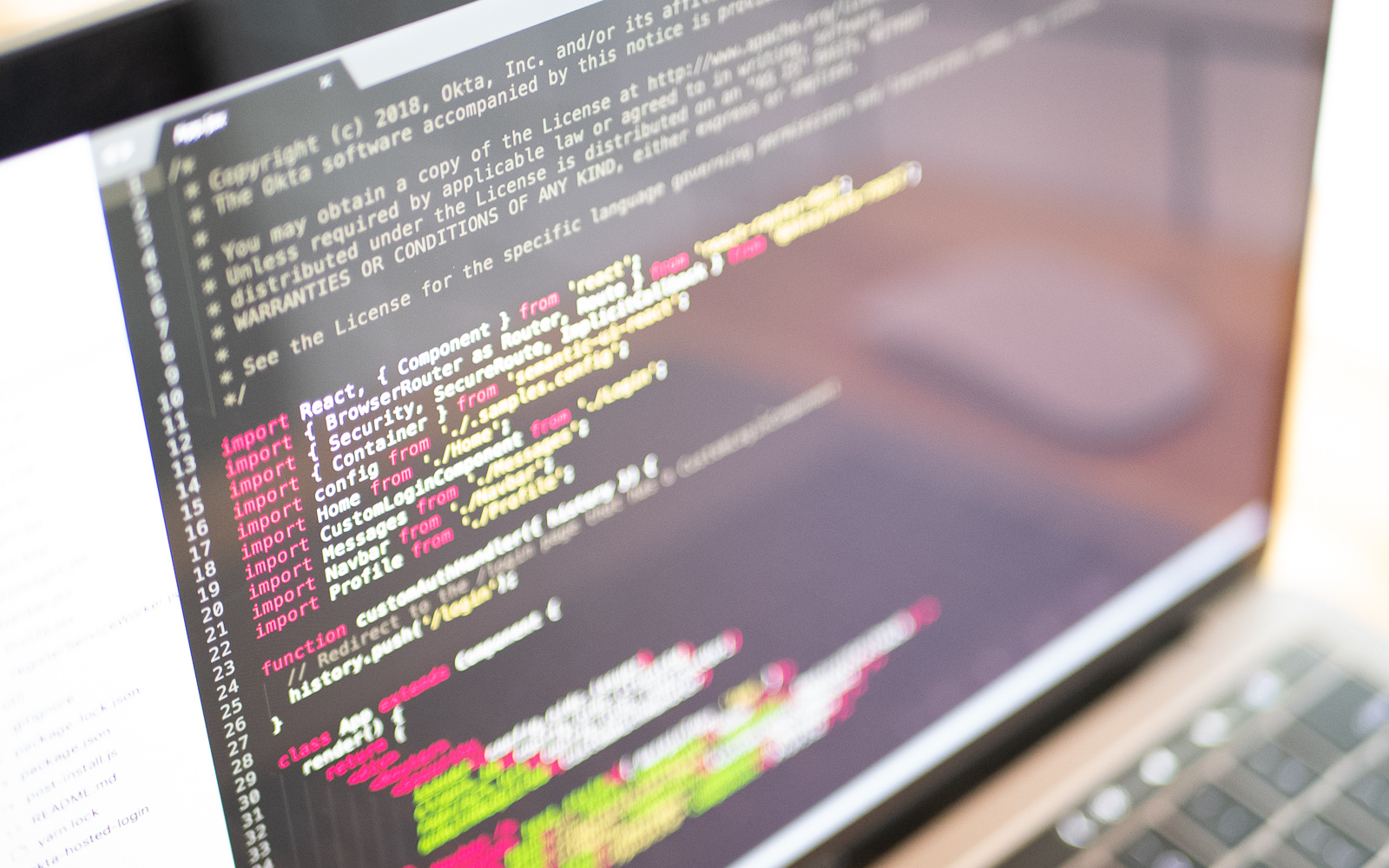
Note: In May 2025, the Okta Integrator Free Plan replaced Okta Developer Edition Accounts, and the Okta CLI was deprecated. We preserved this post for reference, but the instructions no longer work exactly as written. Replace the Okta CLI commands by manually configuring Okta following the instructions in our Developer Documentation. Today’s modern web applications are often built with a server-side language serving data via an API and a front-end javascript framework that presents the...
Build a Basic CRUD App with Laravel and React
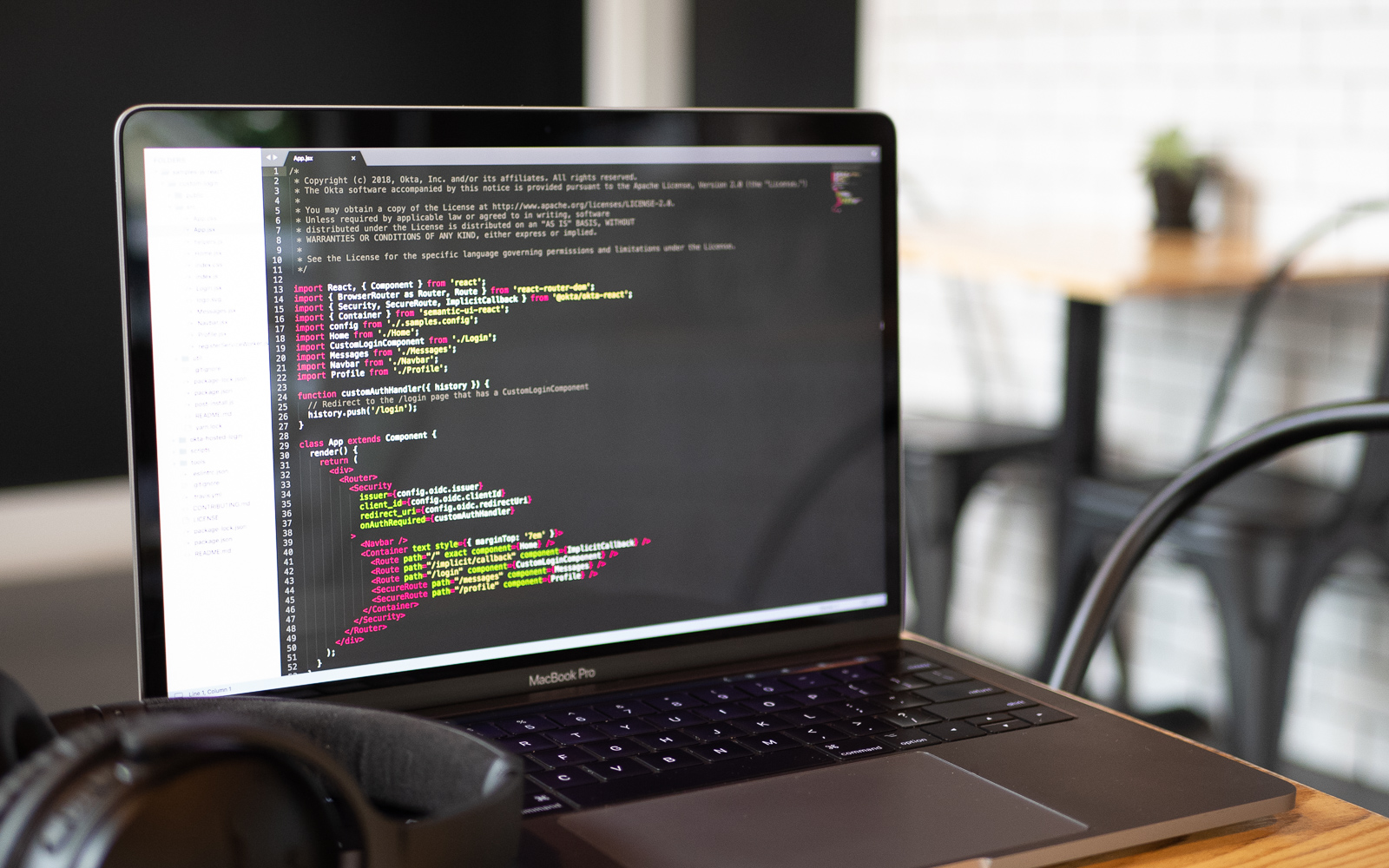
Laravel is an amazing web application framework which regularly tops the lists of best PHP frameworks available today. This is partly because its based on PHP which runs 80% of the web today and the learning curve is relatively small (despite it being packed with advanced features, you can understand the basic concepts easily). However, the real reason for its popularity is its robust ecosystem and abundance of high-quality learning resources available for free (like...
Full Stack Reactive with Spring WebFlux, WebSockets, and React
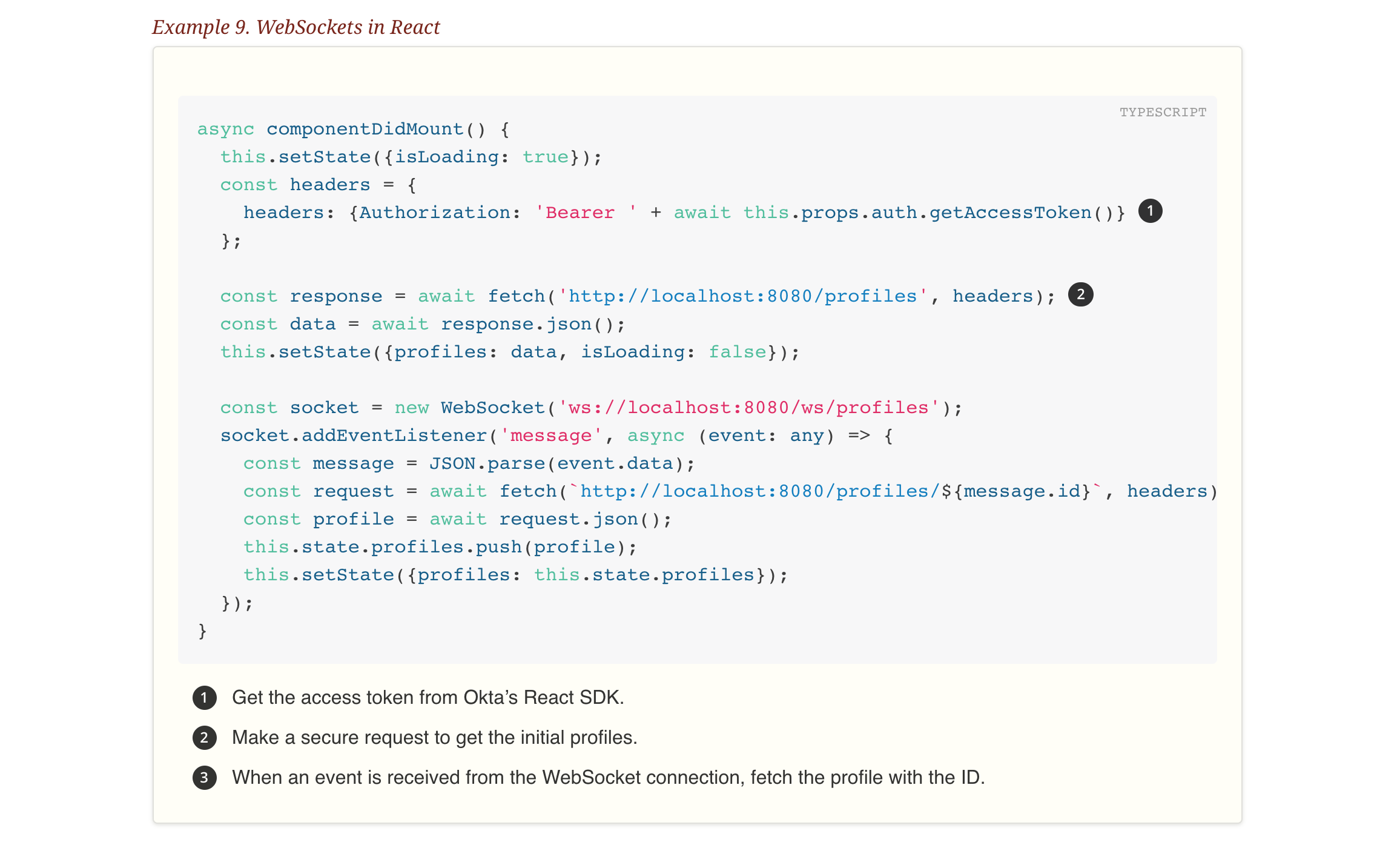
Spring WebFlux can be used to create a REST API with streaming data. Spring WebFlux can also be integrated with WebSockets to provide notifications that clients can listen to. Combining the two is a powerful way to provide real-time data streaming to JavaScript or mobile clients. Add React to the mix and you have an excellent foundation for a full-stack reactive architecture. React is a UI toolkit (similar to GWT) that lets you build components...
Tutorial: Build a Secure CRUD App with Symfony and React
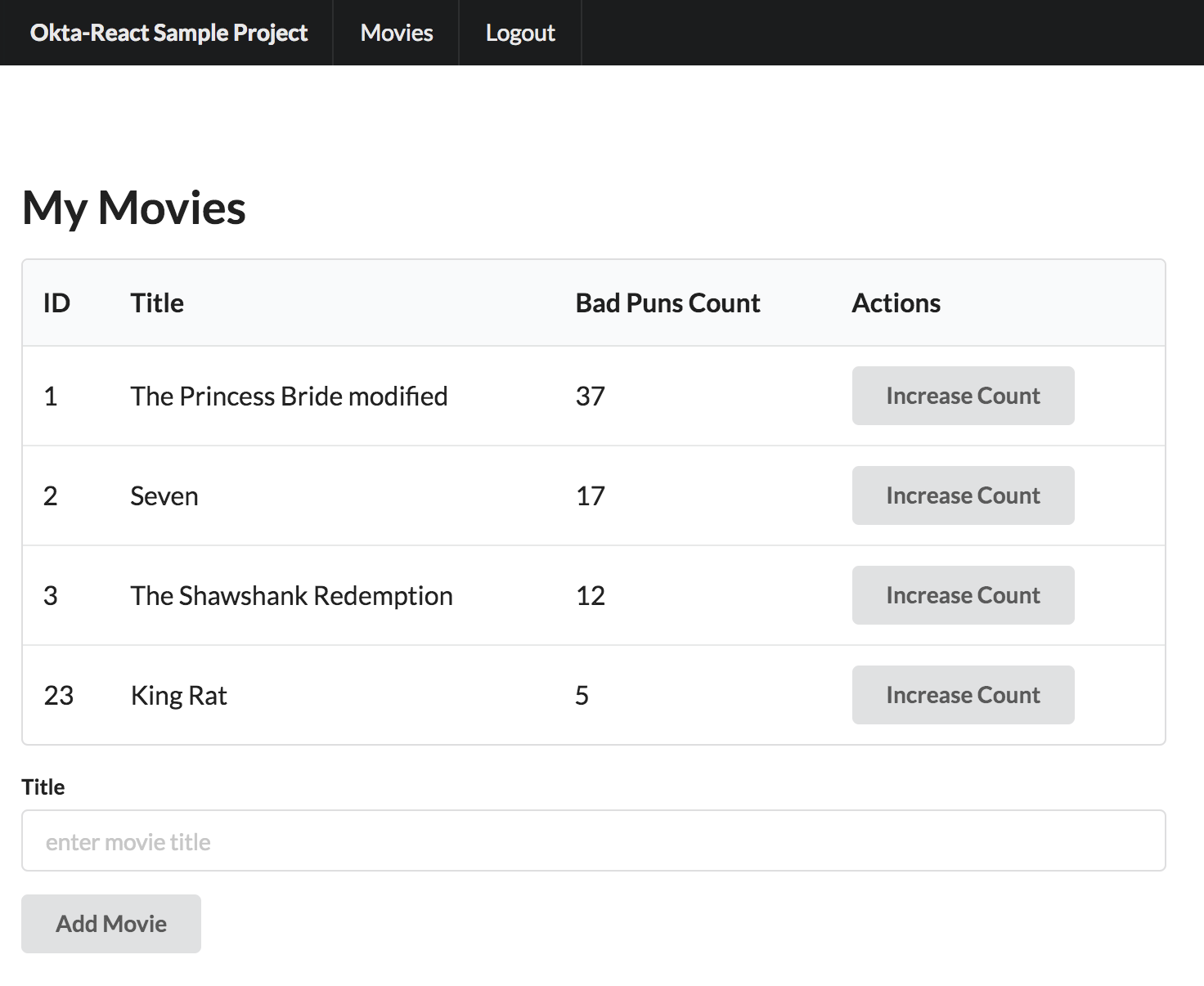
Building a modern single-page application can be a daunting task for a sole developer because of the sheer amount of different components you need to get in place – you need a backend API, a dynamic frontend, a decent user interface, and everything has to be secure and scalable. However, with the right tools in place, you can get started quickly without compromising quality or performance. Today I’ll show you how to create an app...
Use React and Spring Boot to Build a Simple CRUD App
Build a Health Tracking App with React, GraphQL, and User Authentication
I think you’ll like the story I’m about to tell you. I’m going to show you how to build a GraphQL API with Vesper framework, TypeORM, and MySQL. These are Node frameworks, and I’ll use TypeScript for the language. For the client, I’ll use React, reactstrap, and Apollo Client to talk to the API. Once you have this environment working, and you add secure user authentication, I believe you’ll love the experience! Why focus on...
Build a Basic CRUD App with Node and React
There are a lot of JavaScript frameworks out there today. It seems like I hear about a new one every month or so. They all have their advantages and are usually there to solve some sort of problem with an existing framework. My favorite to work with so far has been React. One of the best things about it is how many open source components and libraries there are in the React ecosystem, so you...
Build a Secure CRUD App with ASP.NET Core and React
These days it’s prevalent to have a “back-end” and a “front-end” allowing two (or more) teams to work on a project. Microsoft’s latest version of the ASP.NET Core framework is cross-platform and performant. Pairing it with the power and flexibility of Facebook’s React framework makes it a pretty stable platform. In this tutorial, I will show you how to build a secure CRUD (Create, Read, Update, and Delete) application using these two powerful technologies. When...
Build a Photo Gallery PWA with React, Spring Boot, and JHipster
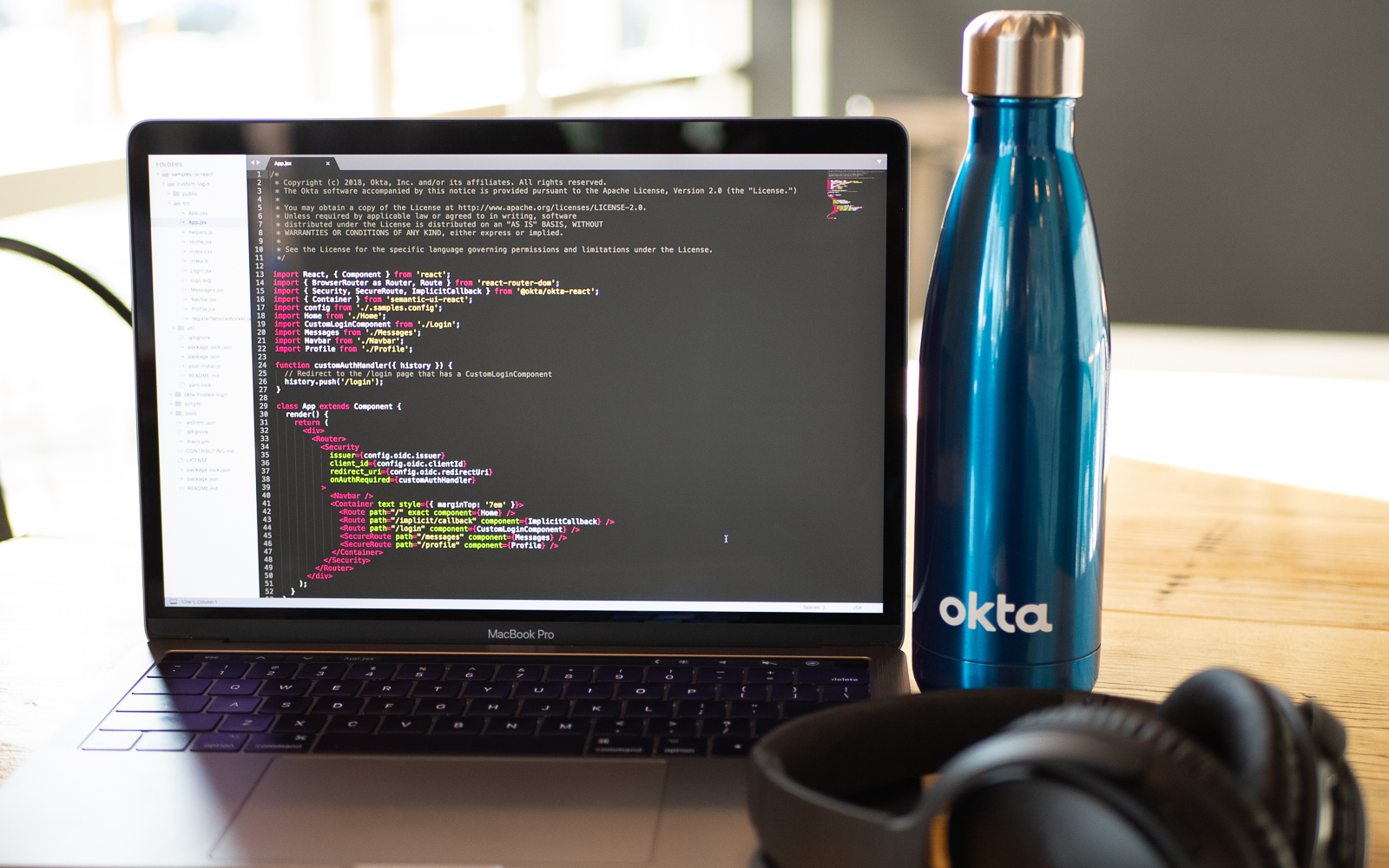
At its core, React is just a UI toolkit, ala GWT, but it has a very healthy ecosystem around it that provides everything you need to build a kick-ass progressive web app (PWA). PWAs are cool because if they’re done right, they can offer a native-like experience for your users, allowing them to install your app, and use it when it’s offline. But, “why React?” is what you’re probably asking yourself right now, right? Well,...
Build a React Native Application and Authenticate with OAuth 2.0
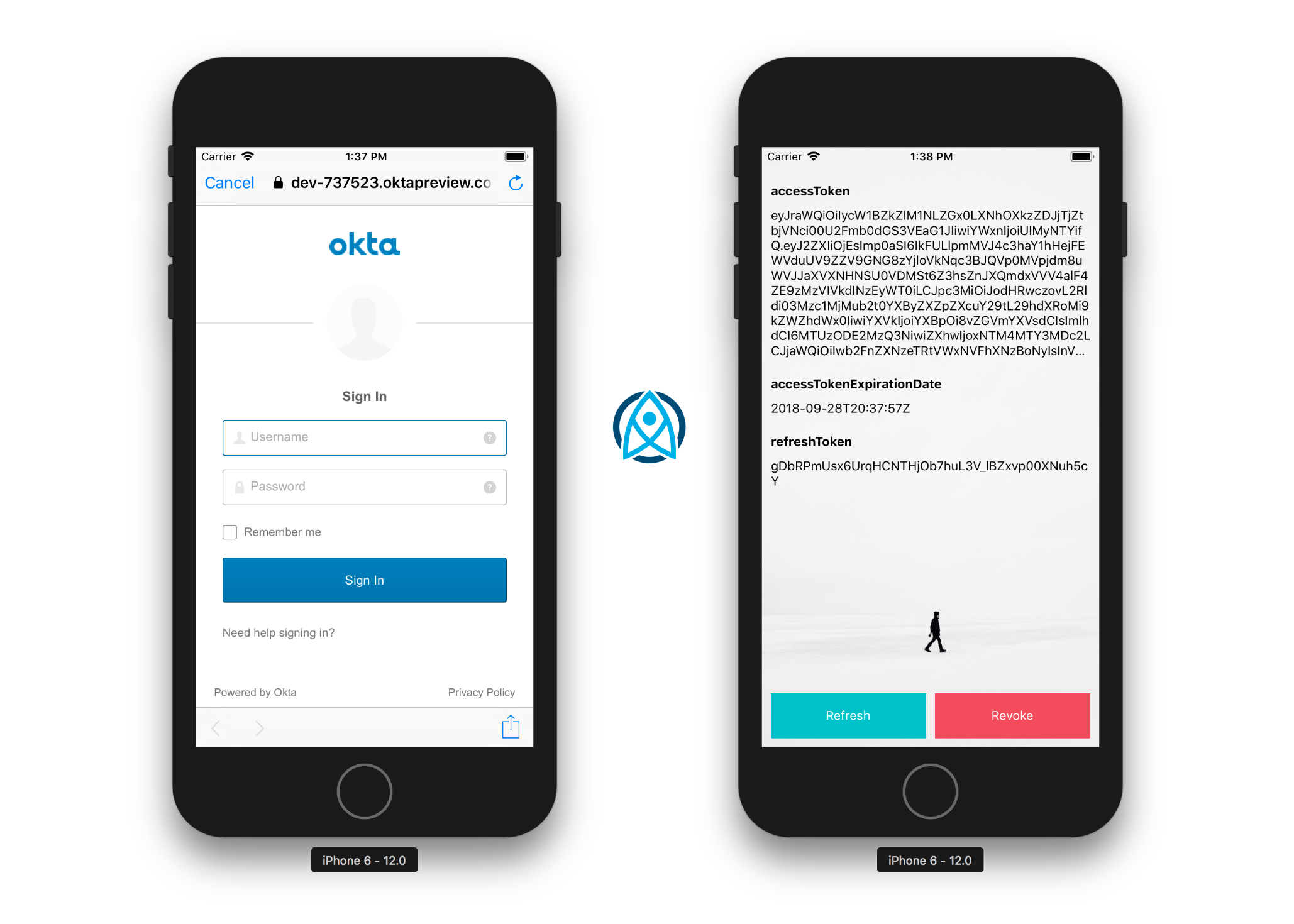
With Okta and OpenID Connect (OIDC) you can easily integrate authentication into a React Native application and never have to build it yourself again. OIDC allows you to authenticate directly against the Okta API, and this article shows you how to do just that in a React Native application. Today you’ll see how to log a user into your React Native application using an OIDC redirect via the AppAuth library. React Native is a pretty...
Open Source Framework Samples and Quickstarts for Okta's Developer APIs
Developers love sample applications. It’s one thing to see the steps to create an application or feature; but when someone provides a working app you can just build and run it’s simply fantastic. Open source is near and dear to many developers today. Many of the frameworks we use to build applications are open source. It’s a great way to develop widely-used software and get contributions from your users. Okta’s Developer Experience (DevEx) team believes...
Build User Registration with Node, React, and Okta
Today’s internet users expect a personalized experience. Developers must learn to develop websites that provide that personalized experience while keeping their user’s information private. Modern web applications also tend to have a server-side API and a client-side user interface. it can be challenging to get make both ends aware of the currently logged in user. In this tutorial, I will walk you through setting up a Node API that feeds a React UI, and build...
Two Approaches to Setting Up a MERN Stack Application
The trend I’ve seen in web applications is a backend API written in a server-side technology like Node, with a front-end single-page application written in something like React. The problem with these stacks is that it can be hard to run and deploy them as a single unit. The API and UI will need to be started, stopped and deployed separately. That can be a bit of a pain when developing, and if you are...
Bootiful Development with Spring Boot and React
React has been getting a lot of positive press in the last couple years, making it an appealing frontend option for Java developers! Once you learn how it works, it makes a lot of sense and can be fun to develop with. Not only that, but it’s wicked fast! If you’ve been following me, or if you’ve read this blog for a bit, you might remember my Bootiful Development with Spring Boot and Angular tutorial....
Build a Preact App with Authentication
React is a fast, and lightweight library, which has led to fast adoption across the SPA (single-page app) ecosystem. Preact is an even lighter-and-faster alternative to React, weighing in at a measly 3kb! For less complex applications, it can be a great choice. In this tutorial, you’ll build a basic Preact application with a couple of pages and user authentication using the Okta Sign-In Widget. Bootstrap Your App With PreactCLI To get your project started,...
The Ultimate Guide to Progressive Web Applications
Progressive Web Apps, aka PWAs, are the best way for developers to make their webapps load faster and more performant. In a nutshell, PWAs are websites that use recent web standards to allow for installation on a user’s computer or device, and deliver an app-like experience to those users. Twitter recently launched mobile.twitter.com as a PWA built with React and Node.js. They’ve had a good experience with PWAs, showing that the technology is finally ready...
Build a React Application with User Authentication in 15 Minutes
React has quickly become one of the most favored front-end web frameworks, and is second only to plain old HTML5, according to JAXenter. So it’s no surprise that developers are learning it, and employers are asking for it. In this tutorial, you’ll start with a very simple React app with a couple of pages and some routing built in, and add authentication using Okta’s Sign-In Widget. The Sign-In Widget is an embeddable Javascript widget that...