Articles tagged aspnetcore
How to Secure User Data in Azure Cosmos DB
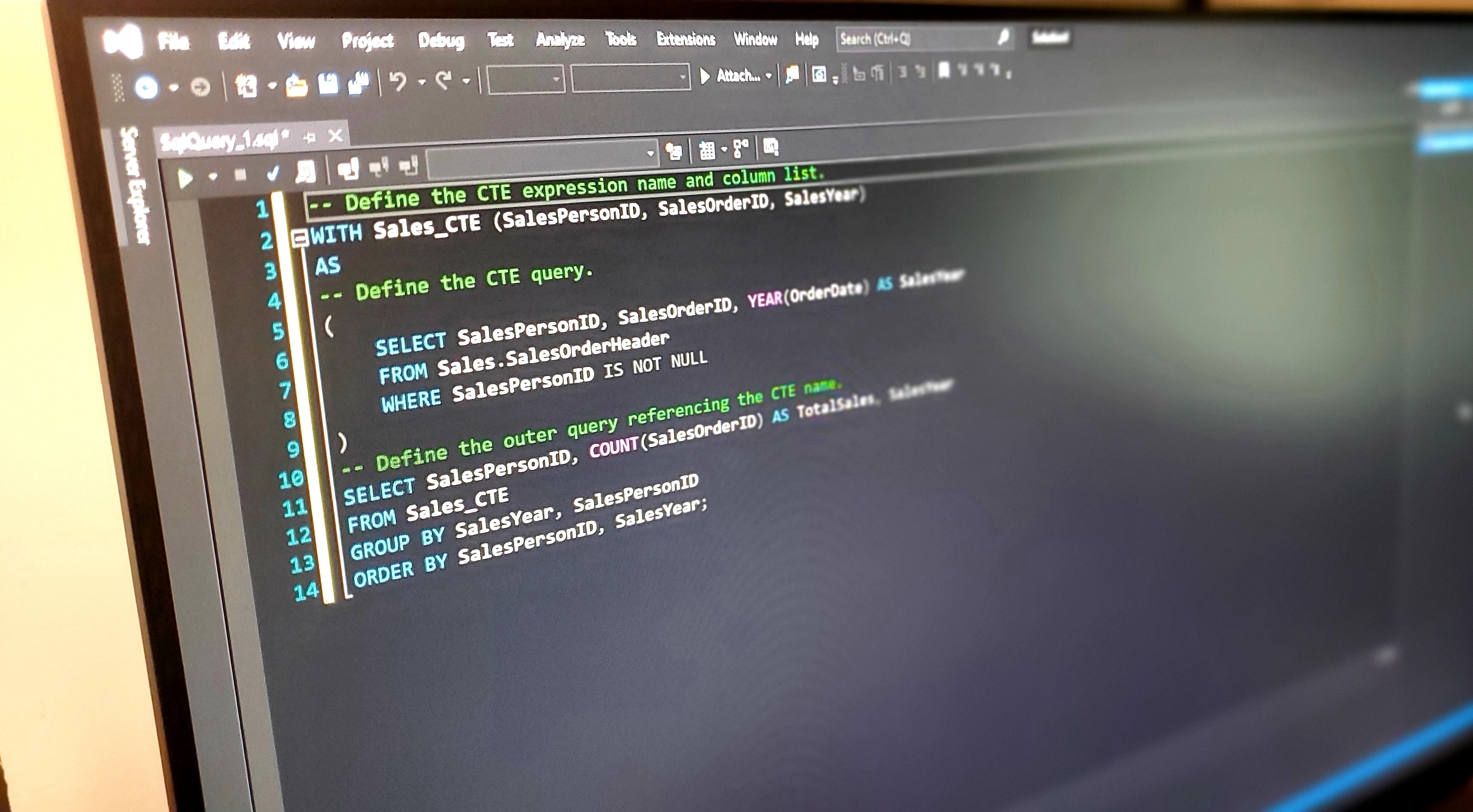
Cosmos DB is a cloud database product from Microsoft that offers scalable and high-performance services. The core product runs on a proprietary NoSQL database that should look familiar to experienced MongoDB developers. Microsoft offers several APIs in addition to the core Cosmos DB API. These include APIs for: SQL MongoDB Gremlin Cassandra The shift to serverless database operations is one of the most obvious advantages of migrating. Cosmos DB can automatically scale your throughput based...
Secure Your .NET 6 Web API
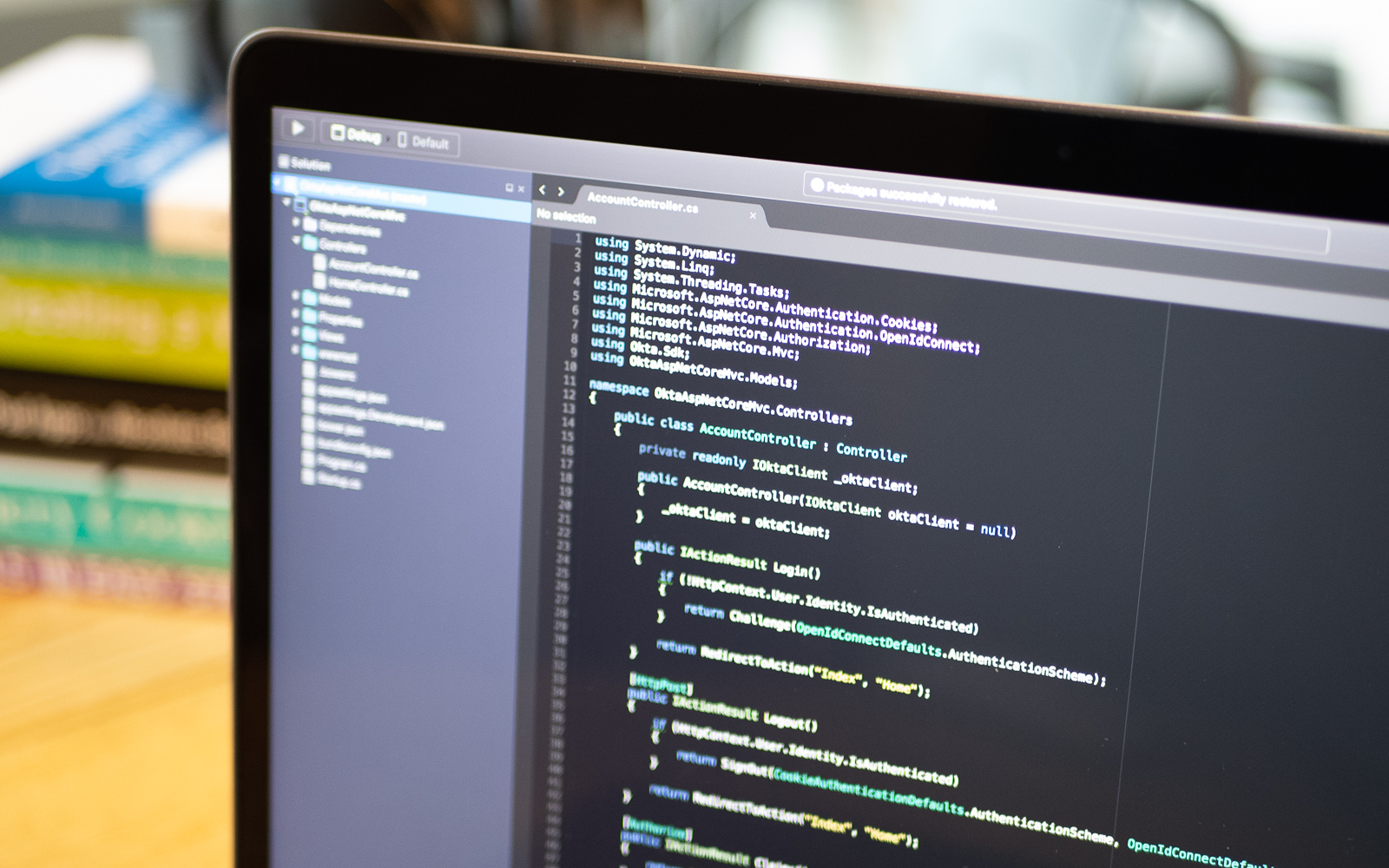
.NET 6 is here and many of us are making preparations to update .NET 5 codebases to .NET 6. As part of this review, today you will learn how to implement the client credentials flow in ASP.NET Core Web API. Note: In May 2025, the Okta Integrator Free Plan replaced Okta Developer Edition Accounts, and the Okta CLI was deprecated. We preserved this post for reference, but the instructions no longer work exactly as written....
Managing Multiple .NET Microservices with API Federation
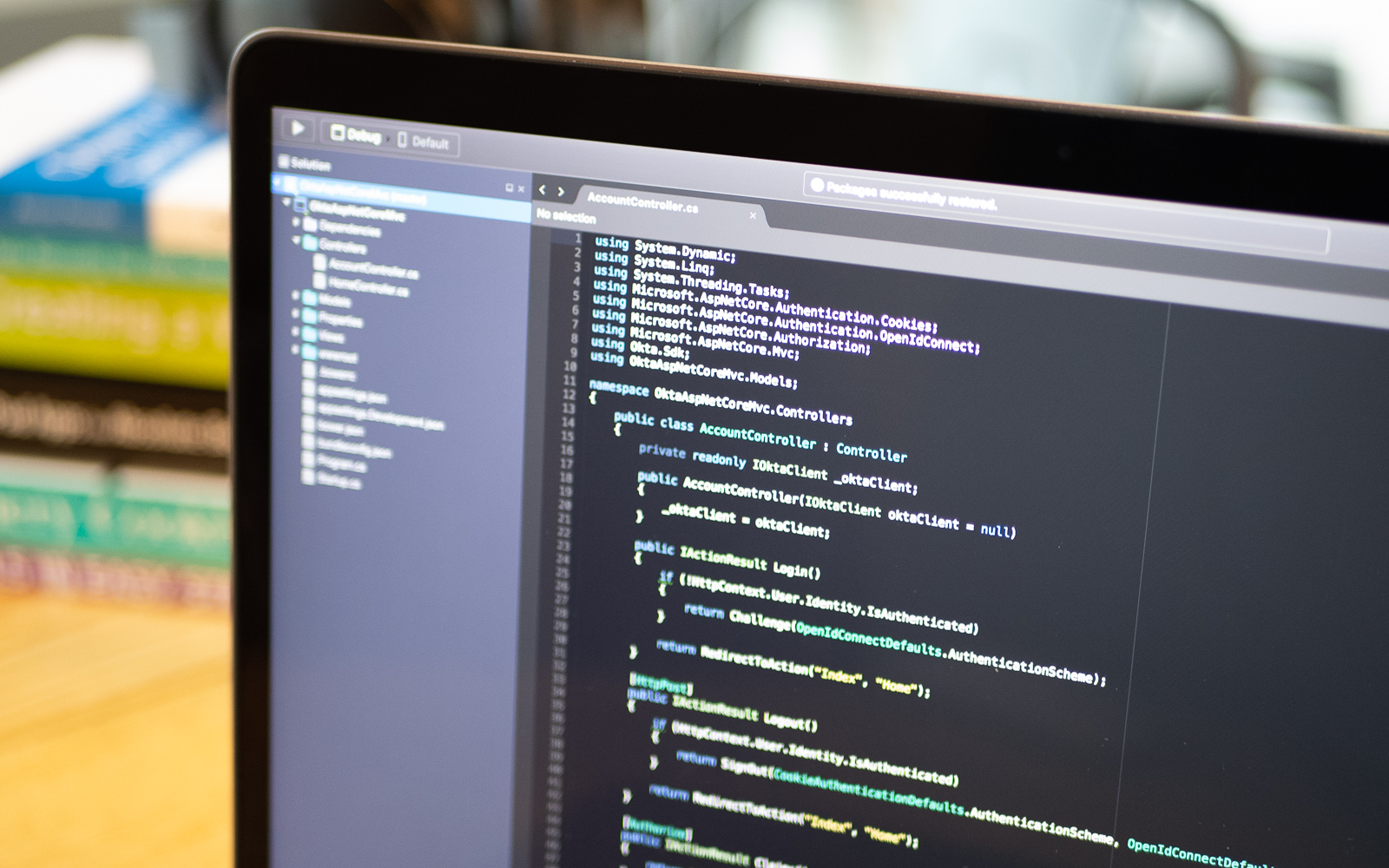
For large enterprise companies, boundaries around each team are crucial for maintaining systems that are owned by software engineers. This is accomplished by allowing individual teams to own their systems and expose them via APIs. You also need to keep certain types of cross-cutting concerns centralized, like select security controls, logging, and routing. If you don’t, every API across different teams has to implement common controls like rate-limiting, logging, and authentication. This can lead to...
Comparison of Dependency Injection in .NET
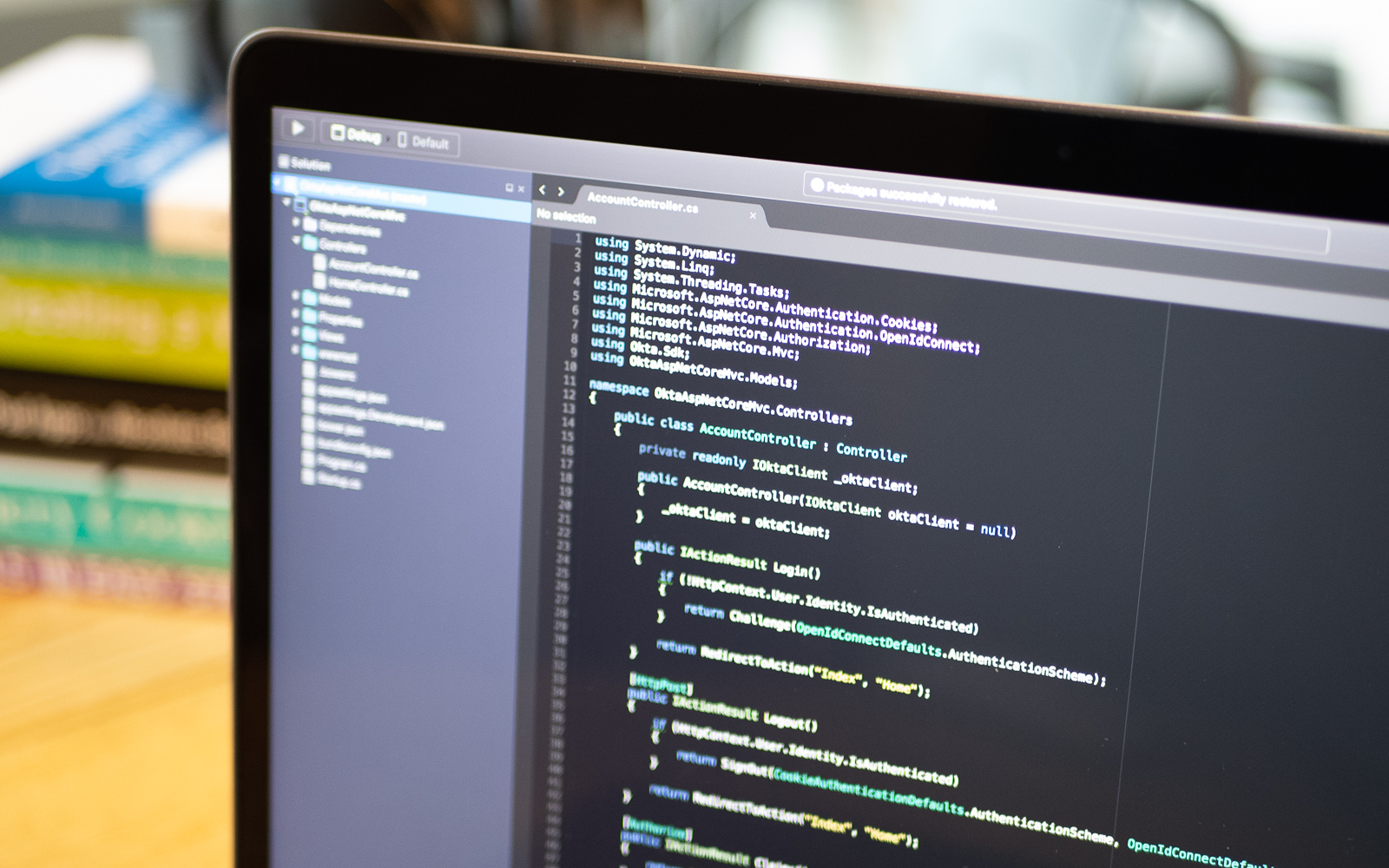
Why you should care about dependency injection Dependency injection is a tried and proven design pattern for producing code that is testable, readable, and reusable. This is achieved by creating (or increasing) a separation of concerns, where each class has a dedicated area of responsibility. Dependency injection is just one design pattern used to achieve inversion of control in which the calling code, or client, has no knowledge of the inner workings of the dependency,...
Using Azure Cognitive Services in a .NET App
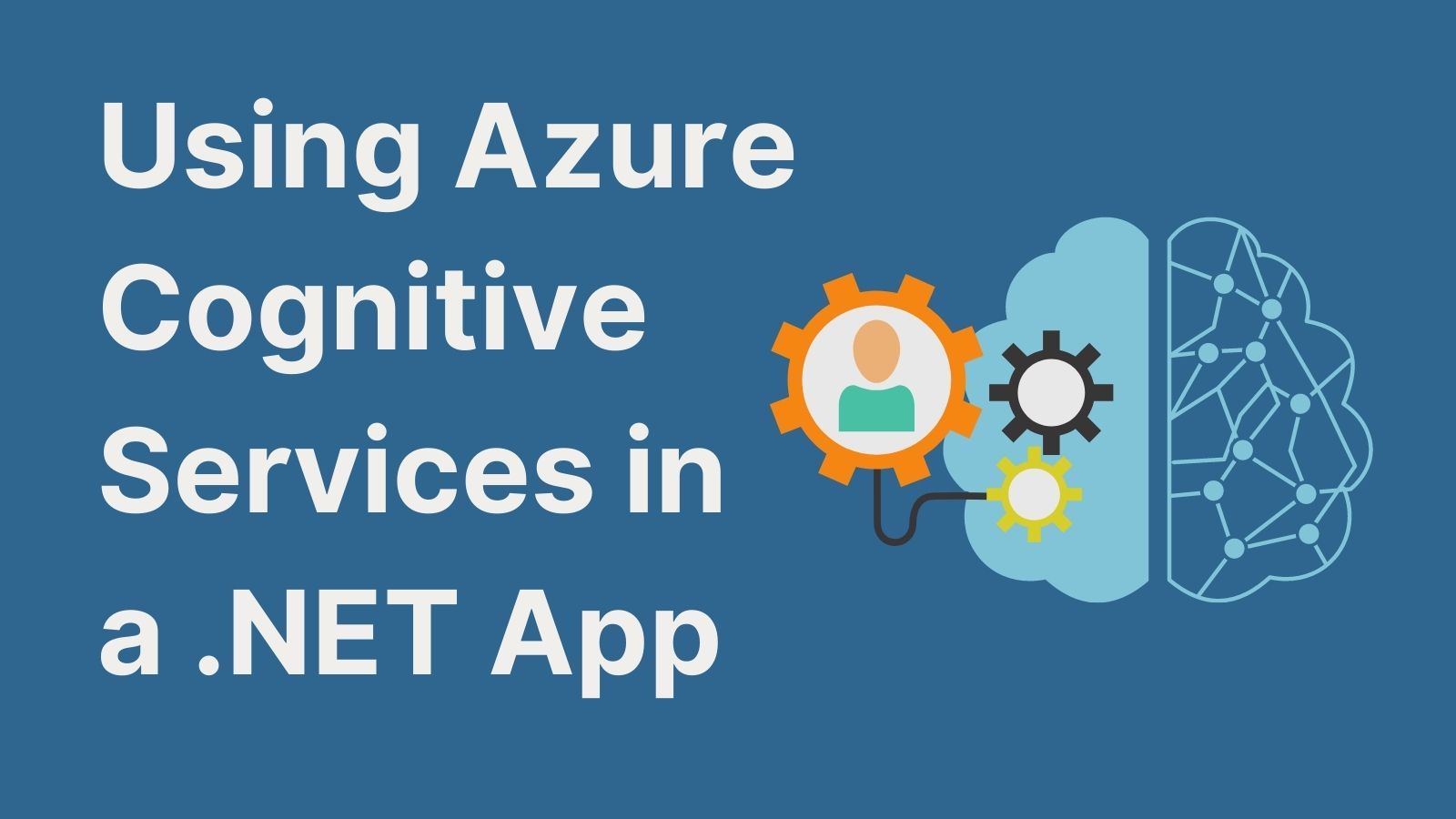
Azure Cognitive Services is a collection of cloud-based AI products from Microsoft Azure to add cognitive intelligence into your applications quickly. With Azure Cognitive Services, you can add AI capabilities using pre-trained models, so you don’t need machine learning or data science experience. Azure Cognitive Services has vision, speech, language, and decision-making services. In this article, you will learn how to use the Vision Face API to perform facial analysis in a .NET MVC application...
Secure Your .NET 5 Blazor Server App with MFA
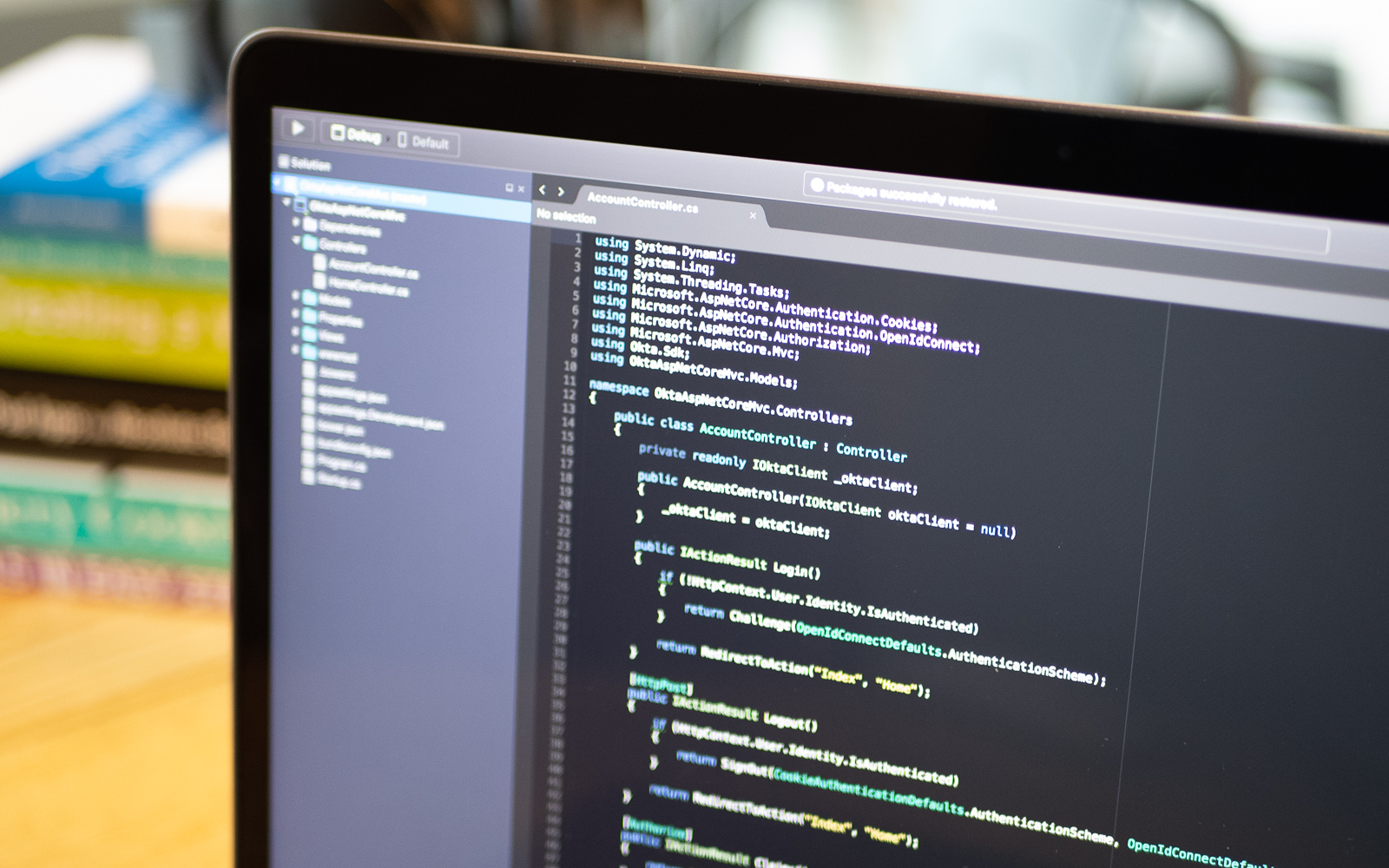
Note: In May 2025, the Okta Integrator Free Plan replaced Okta Developer Edition Accounts, and the Okta CLI was deprecated. We preserved this post for reference, but the instructions no longer work exactly as written. Replace the Okta CLI commands by manually configuring Okta following the instructions in our Developer Documentation. Introduction to Server Blazor apps Blazor is an exciting new technology from Microsoft that will allow developers to bring C# to clients. Server and...
How to Write Cleaner, Safer Code with SonarQube, Docker and .NET Core
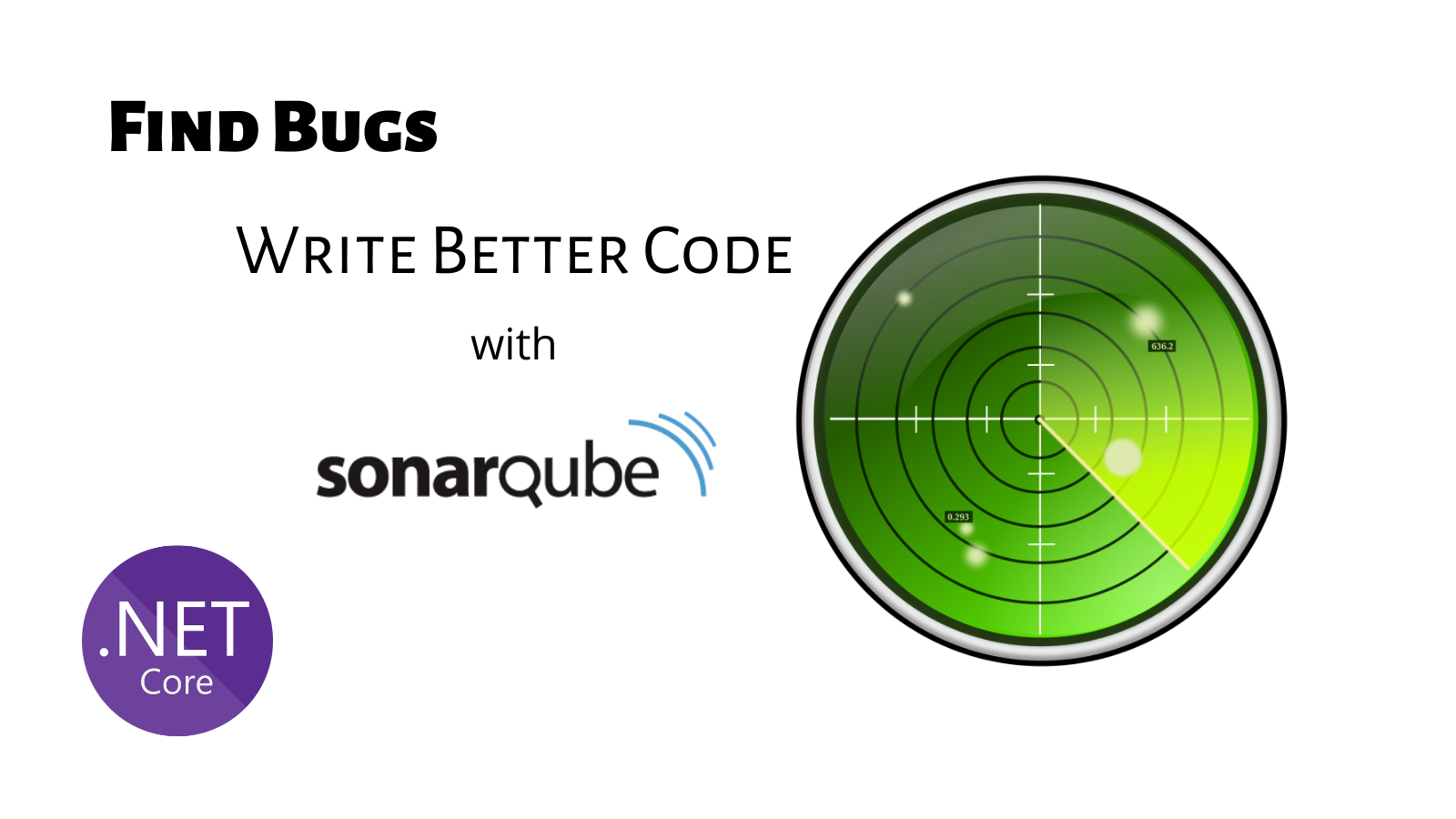
When it comes to code quality and code security, SonarQube is your teammate! This analysis tool is pretty straightforward to use, especially with some help from Docker. In this post, I’ll show you how to run a Docker container with SonarQube to analyze the code of a simple ASP.NET Core 3.0 application. Code analysis is a critical component of app development because it can identify security issues and other tricky bugs that might be overlooked...
Web Forms Migration to Blazor in .NET Core
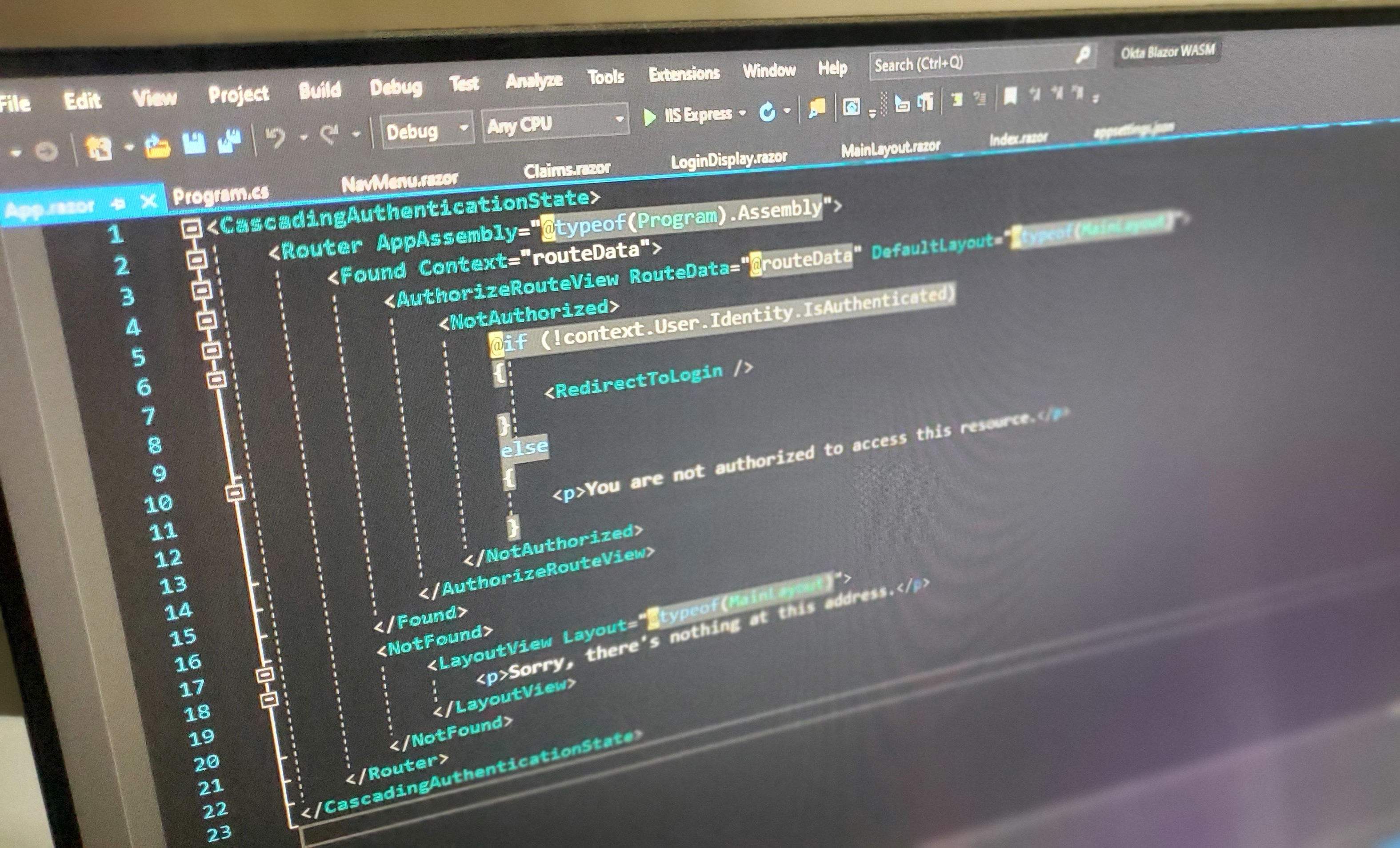
ASP.NET Web Forms framework has been the cornerstone technology of .Net for web development since the release of .Net Framework in 2002. ASP.NET Web Forms includes a layer of abstraction for developers so that you don’t need to care about HTML, JavaScript, or any other front-end technology. It provides a development flow similar to building desktop apps, a way for developers to build a web page by drag and drop, an event-driven programming model, and...
How to Toggle Functionality in C# with Feature Flags
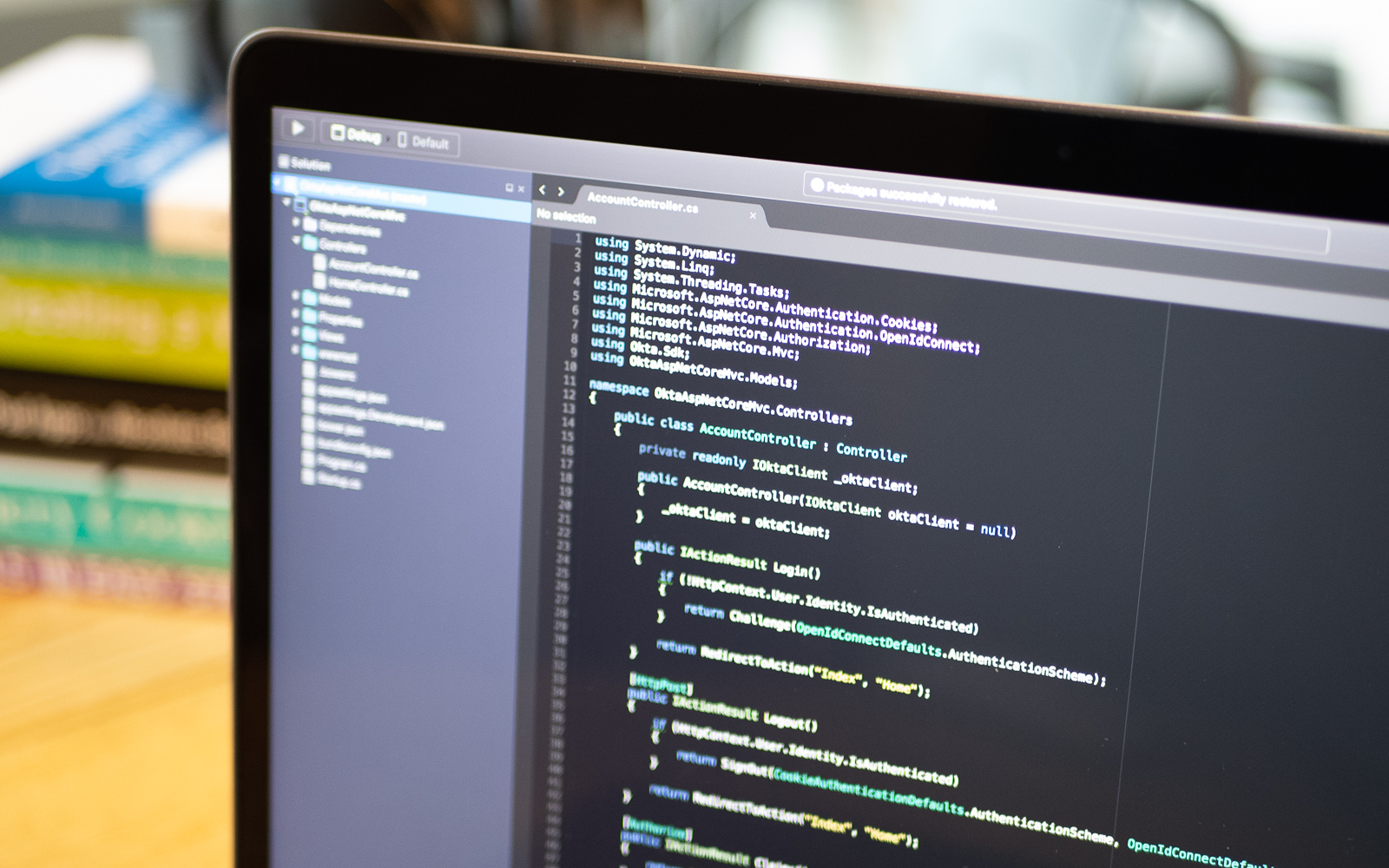
Toggling functionality using feature flags in .NET Core apps is quite straightforward, especially with a neat little feature flag management service. In this post, I’ll walk you through how to build a simple web application using Okta for user authentication and how to use ConfigCat to manage and access feature flags. What Are Feature Flags? Feature flags (aka. feature toggles) are a relatively new software development technique that enables development teams to turn features on...
A Quick Guide to Elasticsearch for .NET
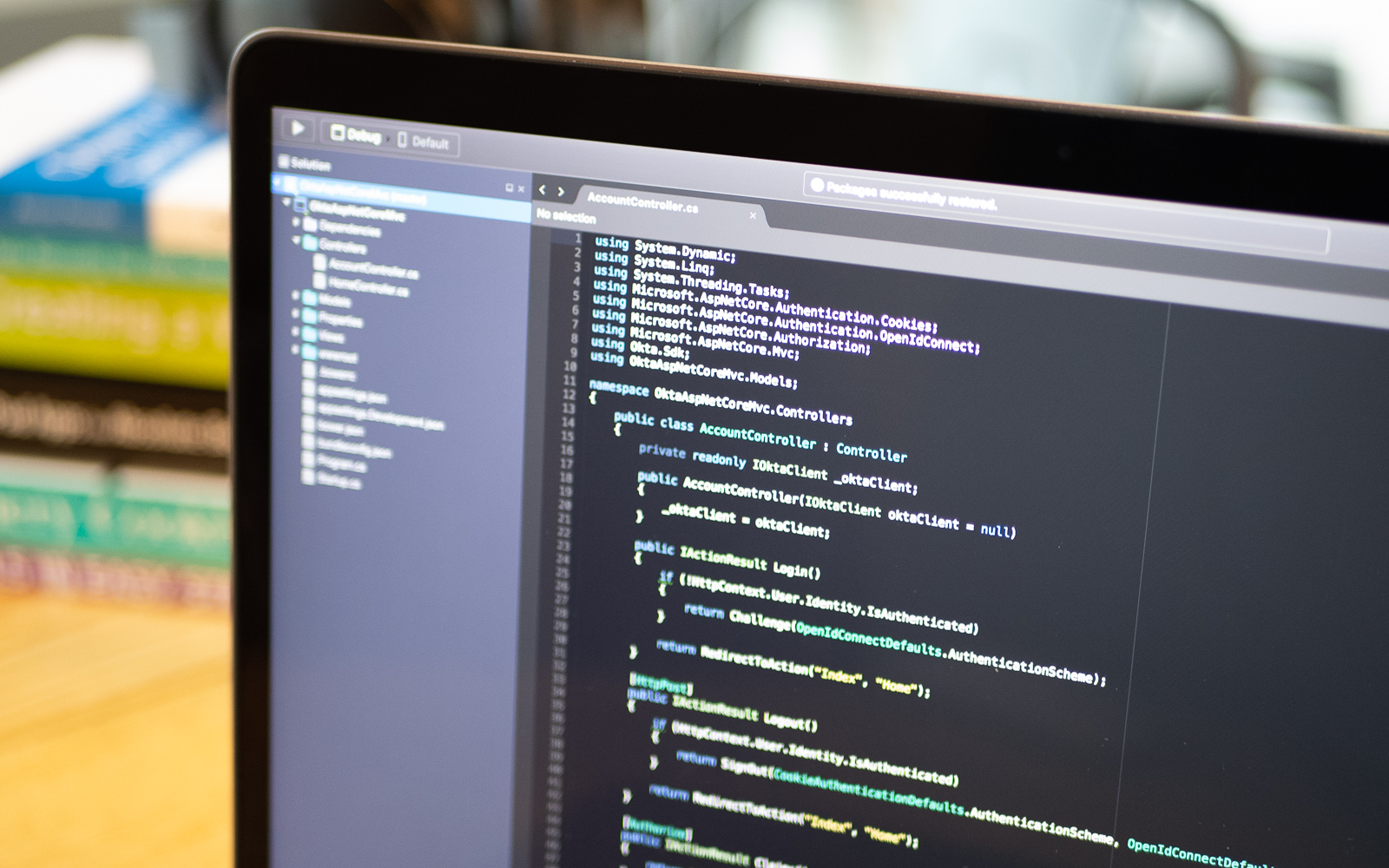
Implementing search functionality in your .NET Core apps doesn’t have to be hard! Using Elasticsearch makes it easy to develop fast, searchable apps. In this post, I’ll walk you through building a simple web application using Okta (for user authentication), Elastic Cloud (the official Elasticsearch hosting provider), and the fabulous Elasticsearch NEST SDK. Note: In May 2025, the Okta Integrator Free Plan replaced Okta Developer Edition Accounts, and the Okta CLI was deprecated. We preserved...
Update App Secrets with Jenkins CI and .NET Core
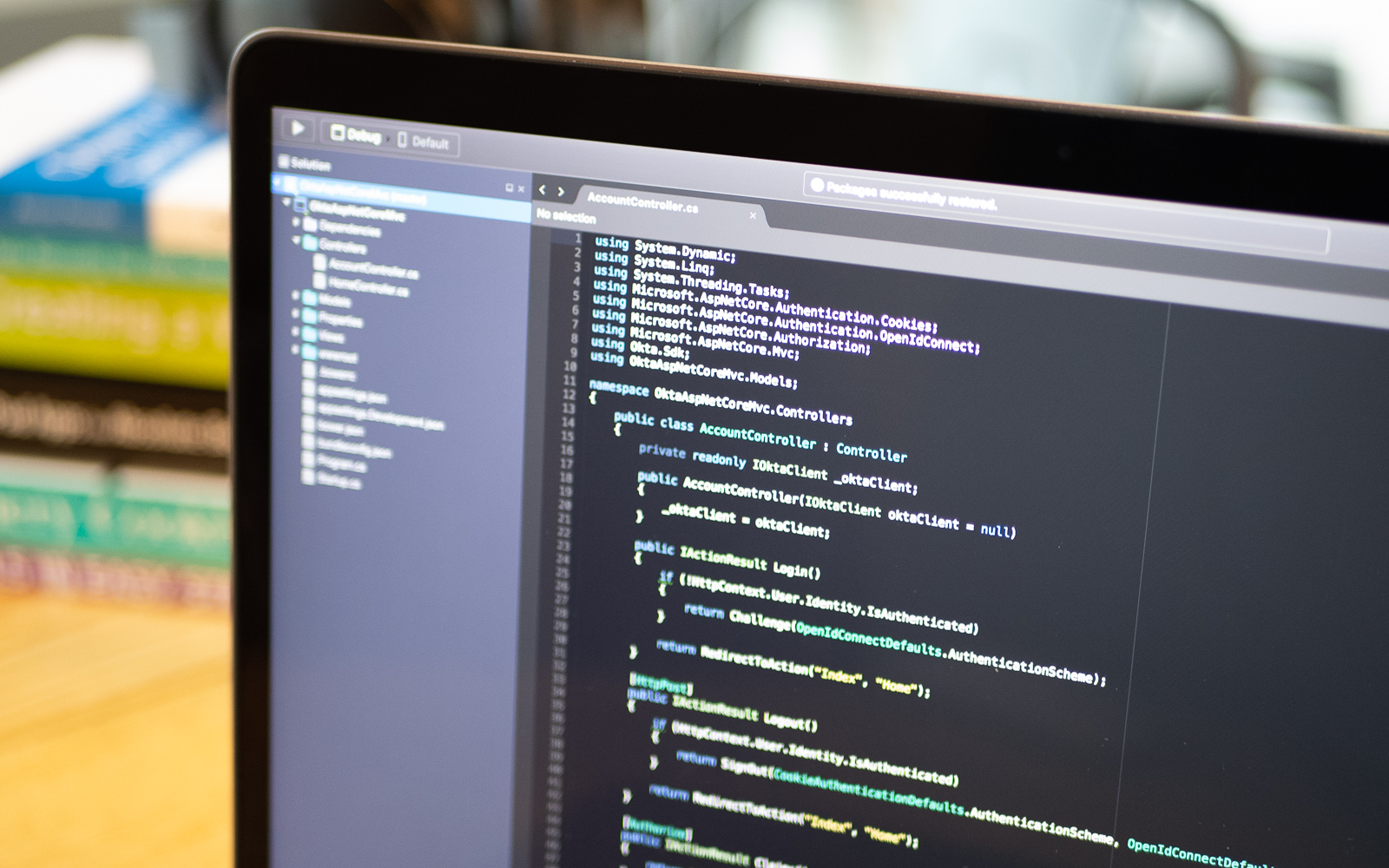
Note: In May 2025, the Okta Integrator Free Plan replaced Okta Developer Edition Accounts, and the Okta CLI was deprecated. We preserved this post for reference, but the instructions no longer work exactly as written. Replace the Okta CLI commands by manually configuring Okta following the instructions in our Developer Documentation. Introduction Jenkins is a free and open-source application that makes it easy to create CI/CD pipelines in almost any language or environment. Jenkins features...
Developer's Cheat Sheet for C# 9.0
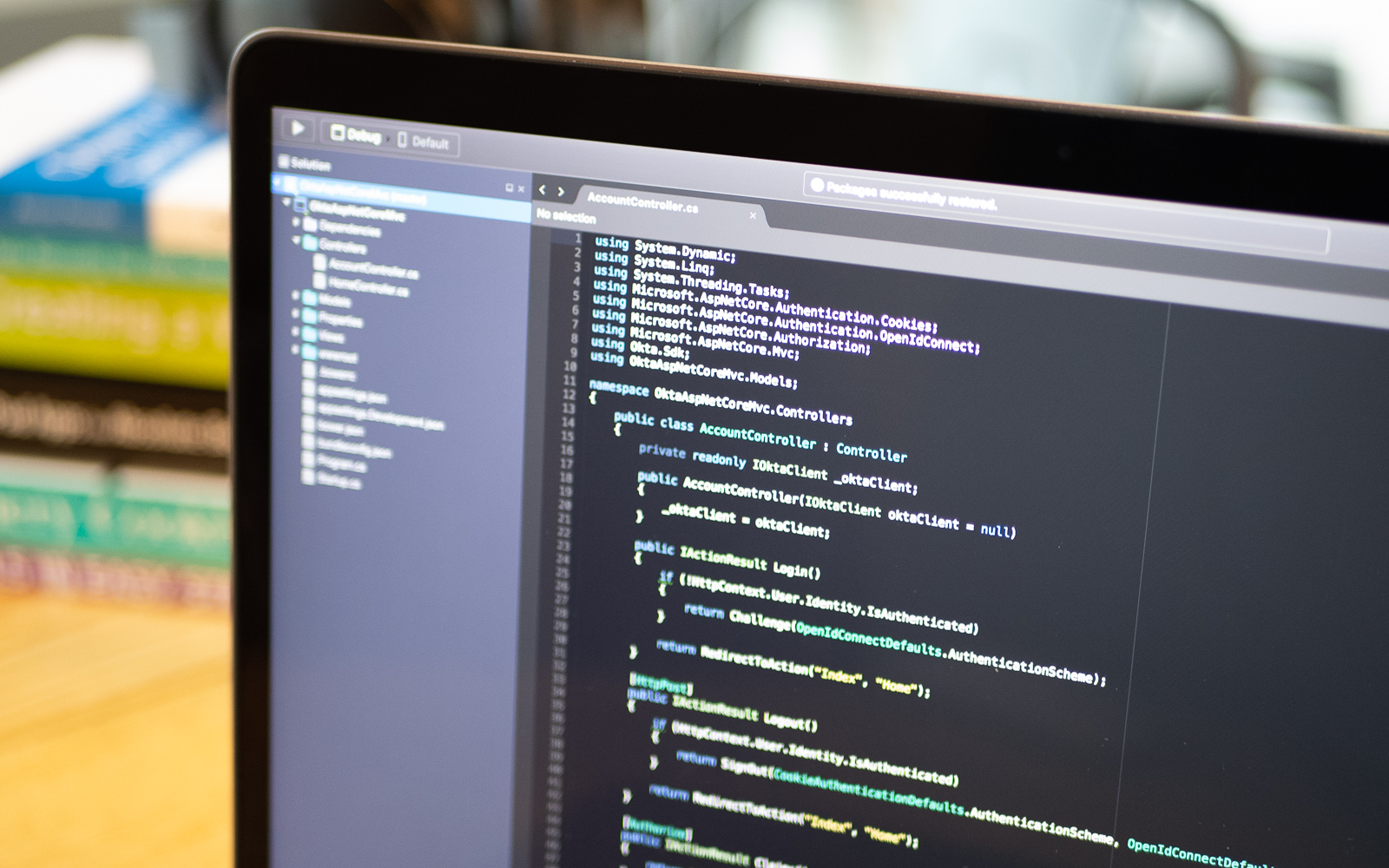
Introduction to C# 9 (and a bit of C# 8, too) Let’s start with a background on how C# 9 got here (implementation examples start in the next section). The last few years in computer science, we’ve observed the rising popularity of the #FreeLunchOver concept. The idea is that CPU technology, based on electrical signals and Von Neumann architecture, has reached its intrinsic limits. As long as integrated circuits were slower than light, we knew...
How to Write Secure SQL Common Table Expressions
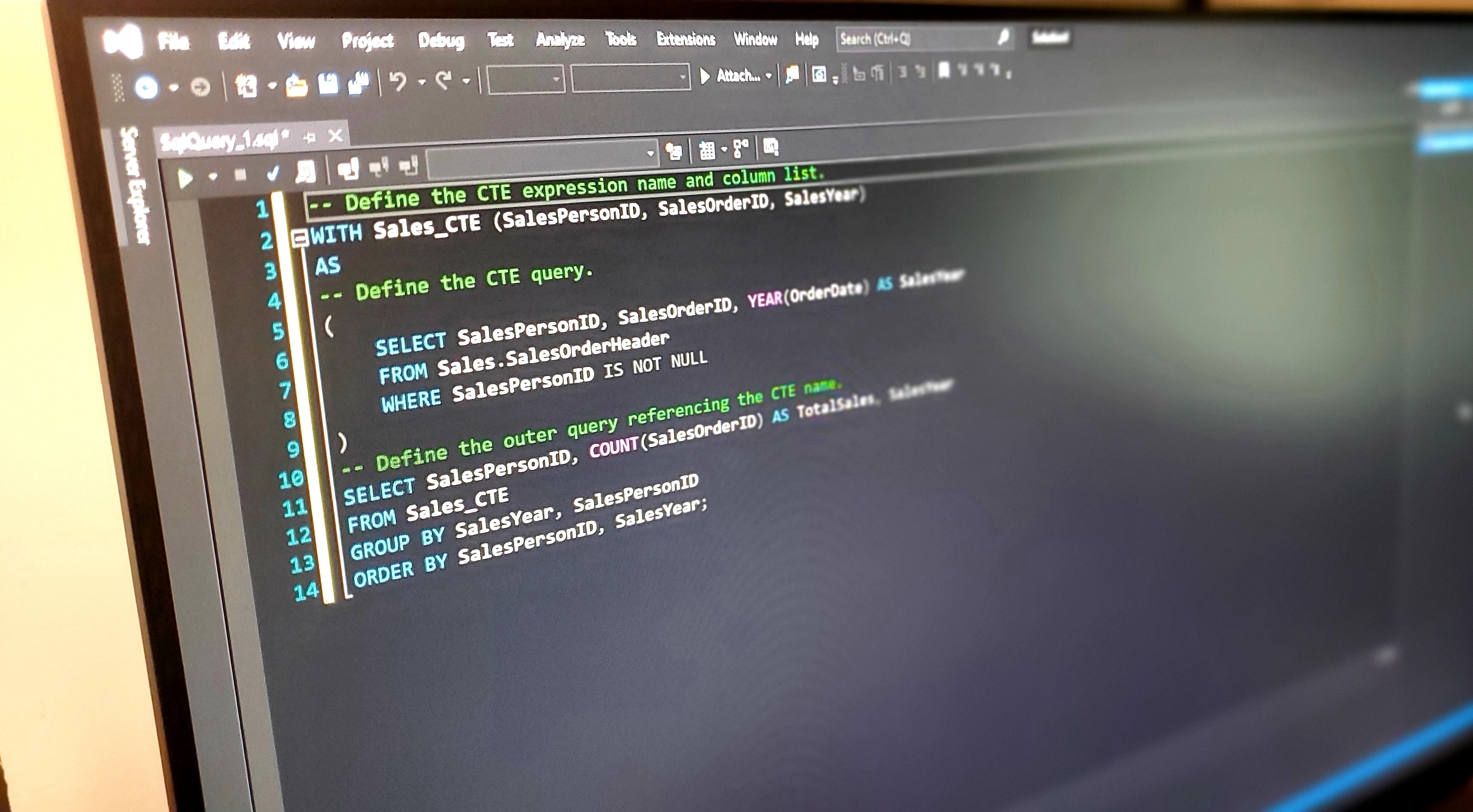
Common table expressions are a powerful feature of Microsoft SQL Server. They allow you to store a temporary result and execute a statement afterward using that result set. These can be helpful when trying to accomplish a complicated process that SQL Server isn’t well suited to handle. CTEs allow you to perform difficult operations in two distinct steps that make the challenge easier to solve. In this article, you will learn how to write common...
How to Use WebAuthn in C#
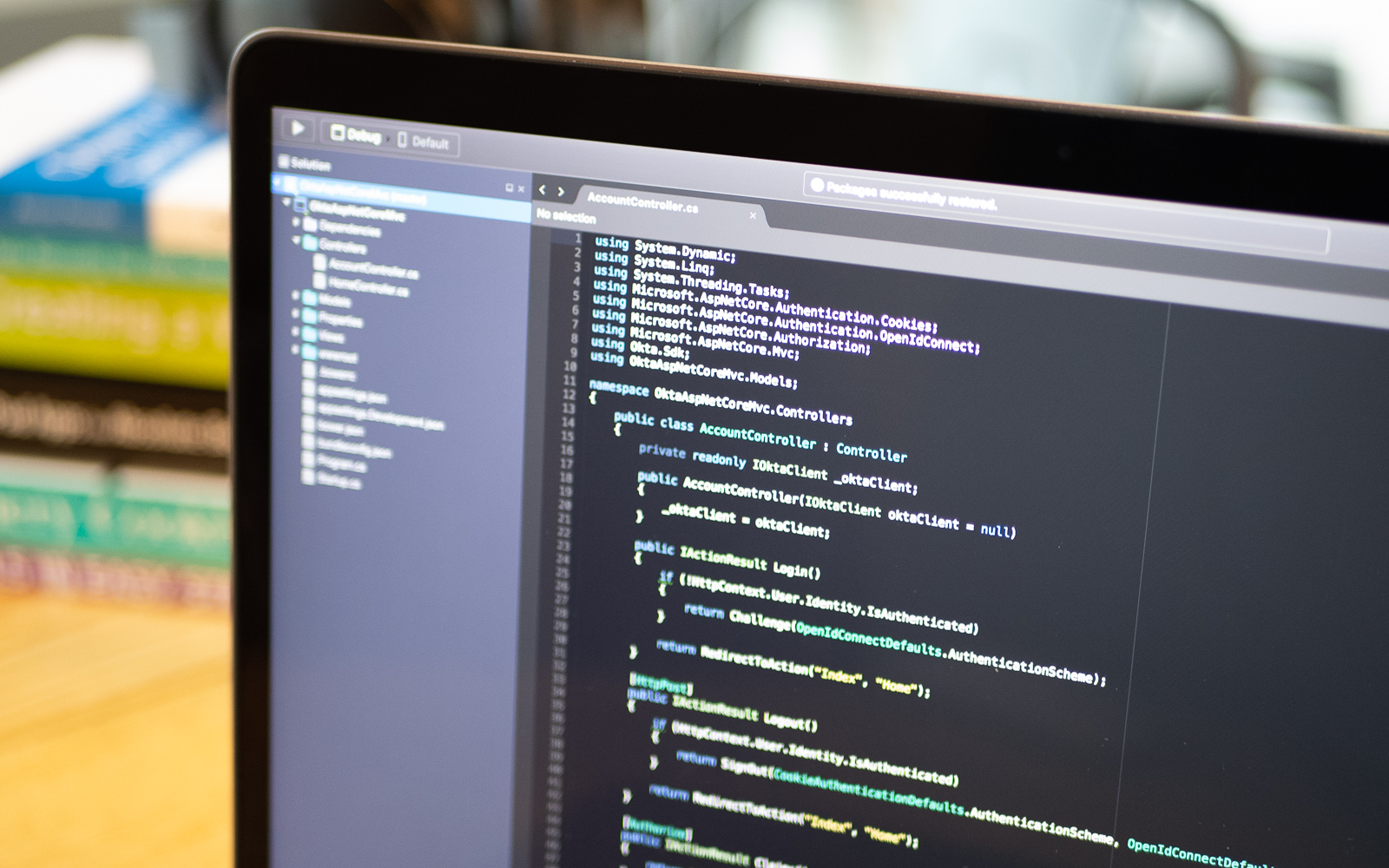
Nowadays, using a password for authentication is becoming less and less secure. Password attacks are becoming more sophisticated, and data breaches occur more frequently. Have I Been Pwned, the website where you can check if your account has been compromised in a data breach, contains more than 10 billion accounts and more than 600 million passwords. With 62% of users reusing passwords, a successful attack on one of the websites gives the attacker access to...
How to Support .NET Core SameSite + OAuth Apps on Linux
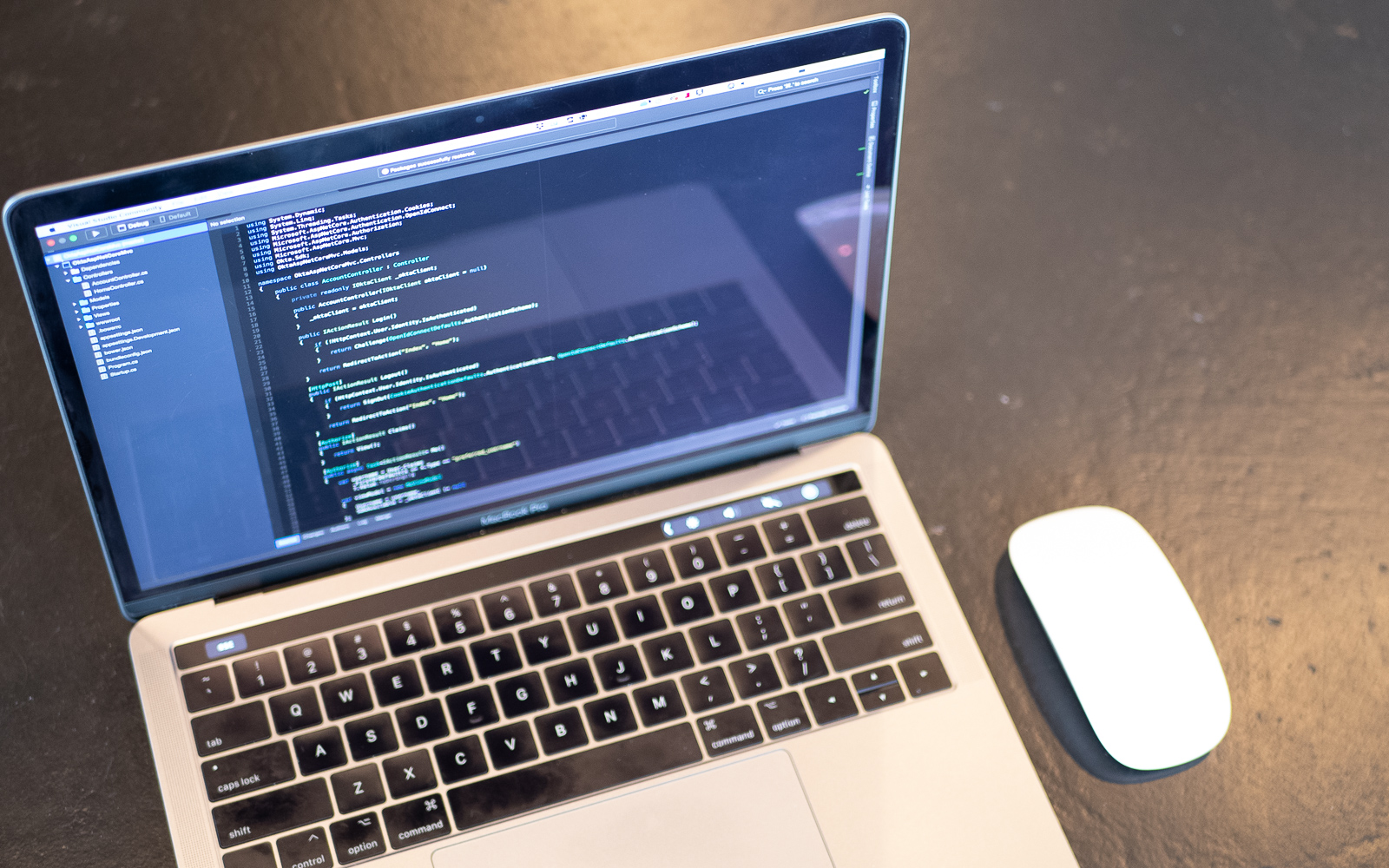
Google’s recent approach to SameSite cookie attributes caused a bit of confusion among developers. Especially in cases where handling redirects is necessary. After doing some research in the topic I’d like this article to be a guide on how to handle SameSite cookie attributes properly in production. This guide can serve as the basis for deploying an application to any Linux based environment—such as AWS Elastic Beanstalk, Google Cloud App Engine—or any VPS Linux deployment....
How to Deploy Your .NET Core App to Google Cloud, AWS or Azure
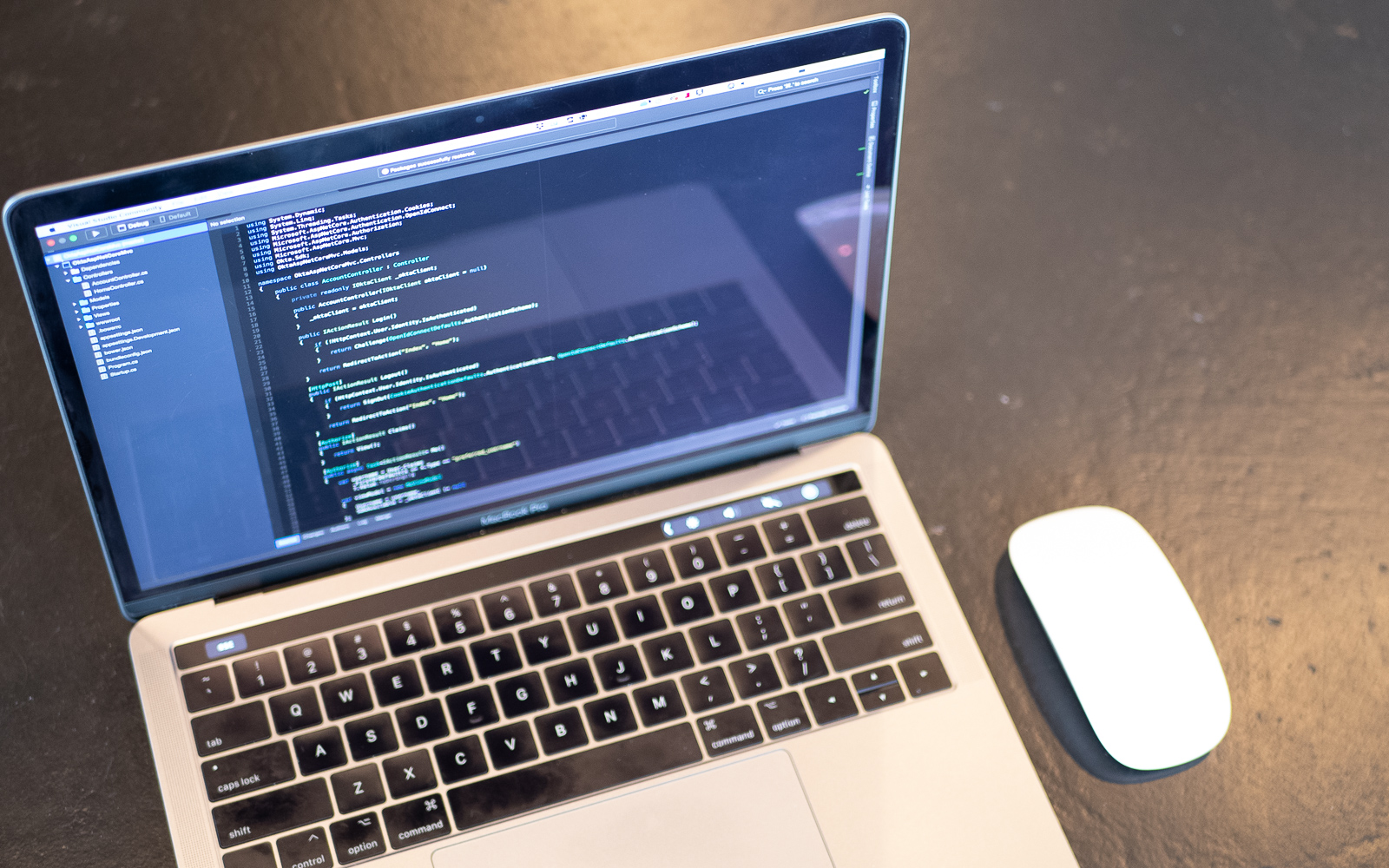
There has been a cut-throat competition between cloud hosts in the past few years - each attempting to earn the sympathy of developers and dev-ops by rolling out shiny new tools, plugins, and integrations. There are a gazillion how-to tutorials and guides in the community on using these tools. Sometimes when looking for a solution, it is hard to find the newest and simplest way. I never know if an article written last year is...
Rider for C# - The Best Visual Studio Alternative IDE
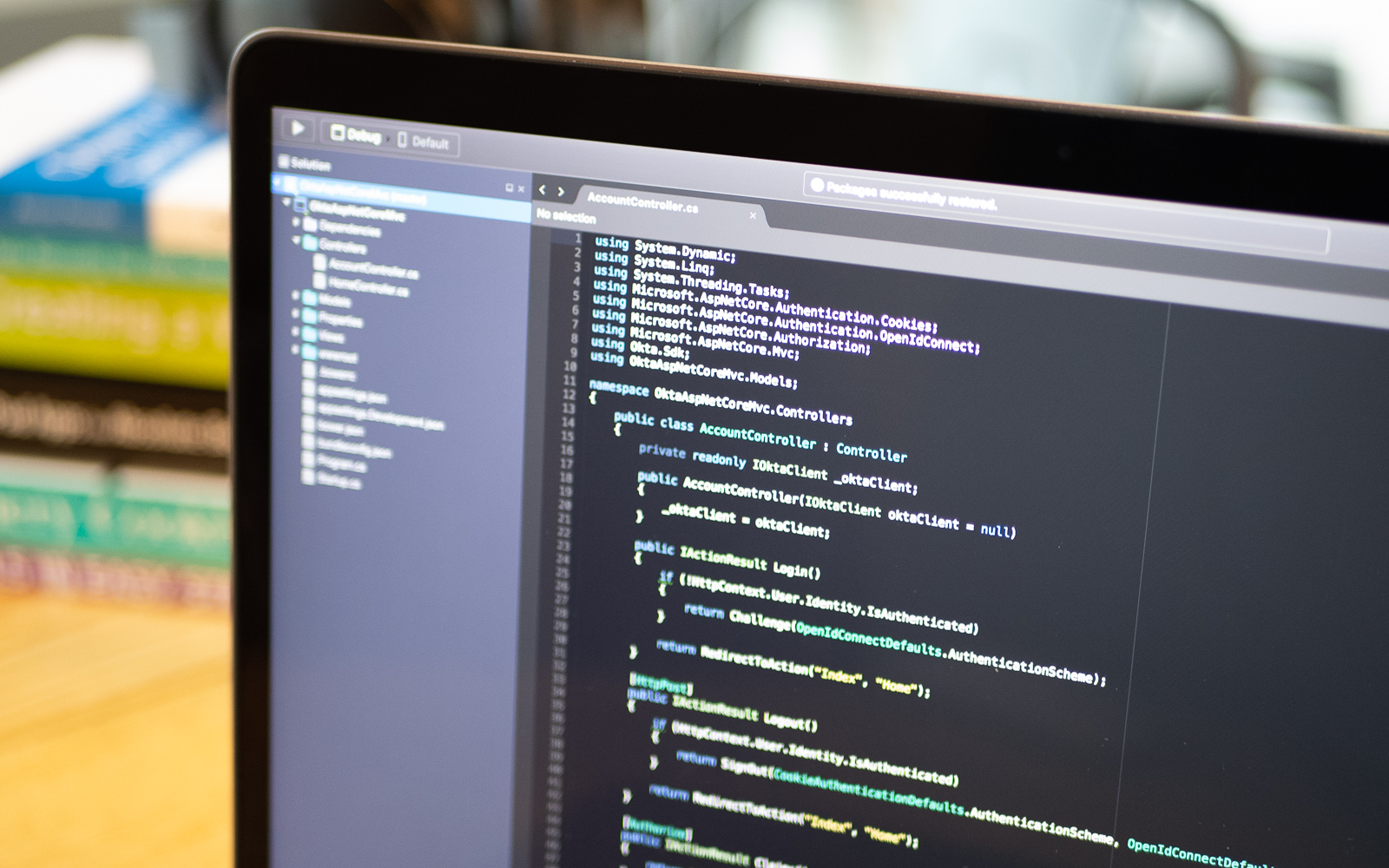
When it comes to developing .NET apps, Visual Studio has historically been the default choice for .NET developers for two main reasons: Visual Studio is the official integrated development environment (IDE) from Microsoft, and There was no viable alternative to Visual Studio This all changed about three years ago when JetBrains, the company behind Resharper, one of the most popular Visual Studio extensions, released a new, cross-platform, and innovative IDE - Rider. The Rider IDE...
Install .NET Core Apps on Linux in 5 Minutes
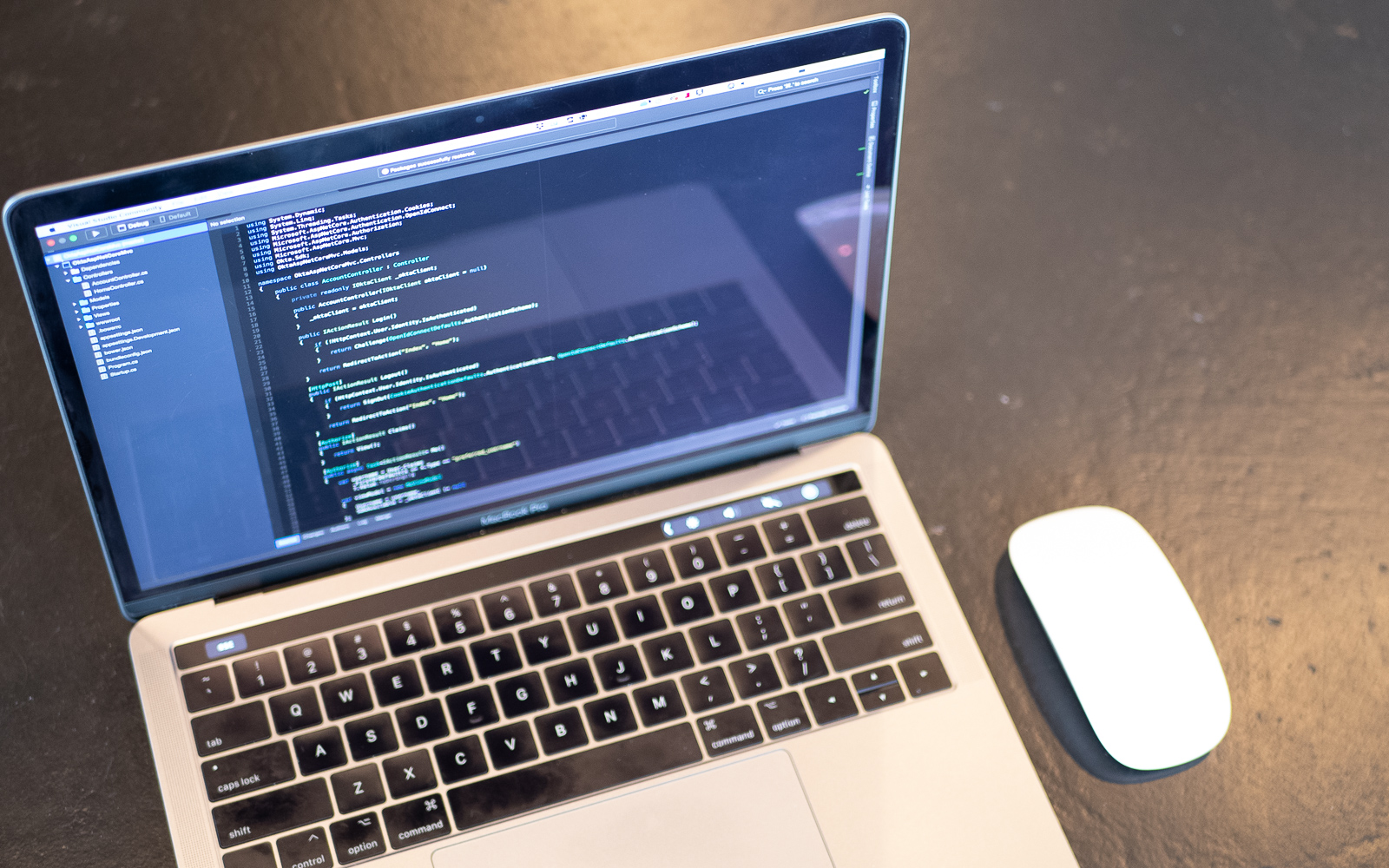
As a big fan of open source, I’m loving the fact that .NET Core is cross-platform. It opens up endless possibilities, from hobby projects, experiments, and proofs of concept, to massive high-load production applications that run on cost-effective infrastructure with high security and scalability. I usually get the simplest and cheapest $5/month Ubuntu-based virtual private server (VPS) from any cloud platform provider instead of the more complex and expensive container instances or cloud computing services....
How to Authenticate with SAML in ASP.NET Core and C#
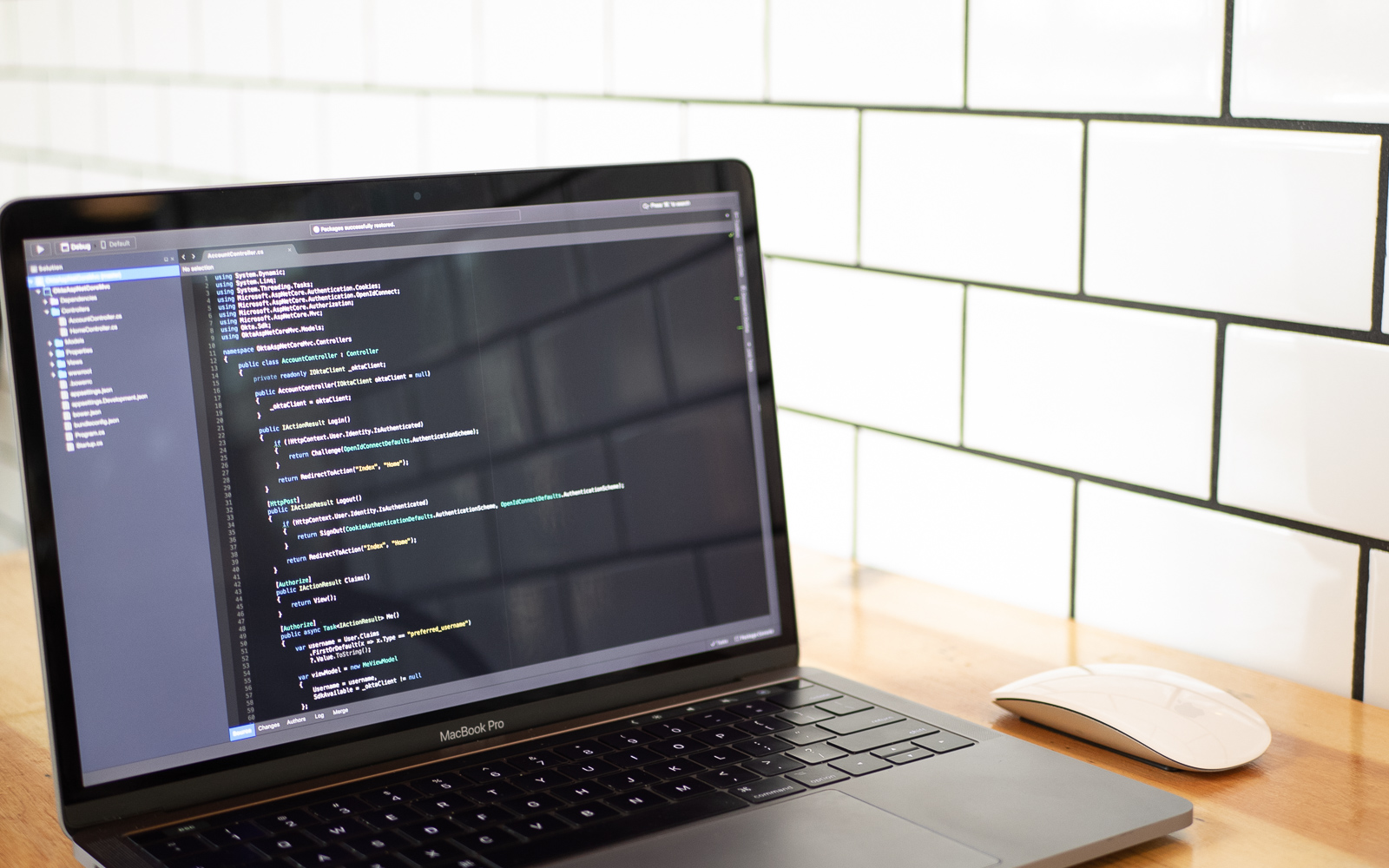
Security Assertion Markup Language, more commonly known as SAML, is an open standard for exchanging authentication and authorization data between parties. Most commonly these parties are an Identity Provider and a Service Provider. The primary use case for SAML has typically been to provide single sign-on (SSO) for users to applications within an enterprise/workforce environment. Up until the past few years, SAML was considered the industry standard—and proven workhorse—for passing an authenticated user into applications...
Deploy a .NET Container with Azure DevOps
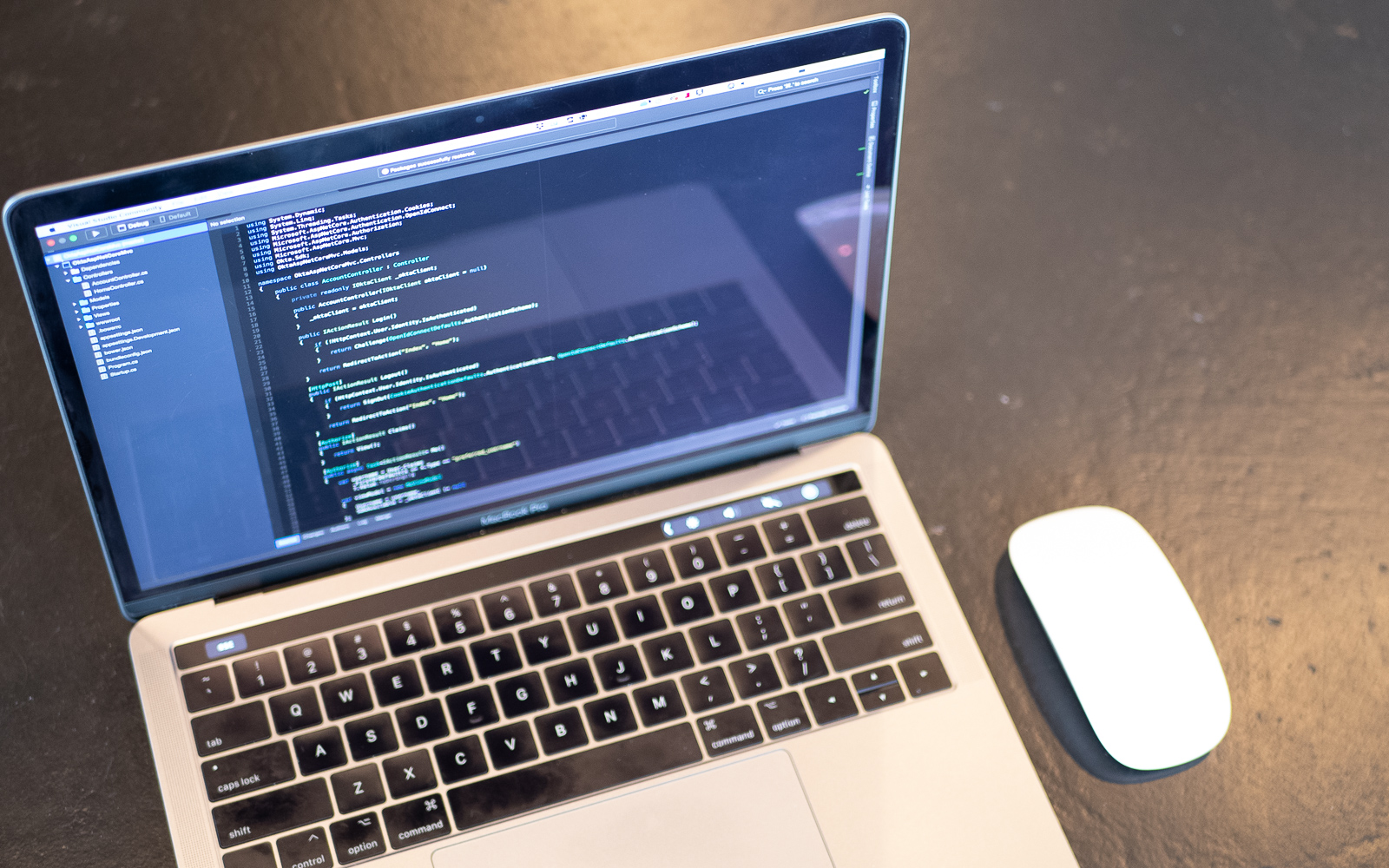
When I began programming (in the ’80s), computers weren’t equipped with a network card by default. The internet was almost unknown and modems were slow and noisy. The software was installed from stacks of flexible floppy disks. Today, computing resources are virtual. The internet is vital and there is an URL for everything. We live in the *aaS (* as a Service) era, where if you want something, there is likely one or more something...
How to Build Securely with Blazor WebAssembly (WASM)
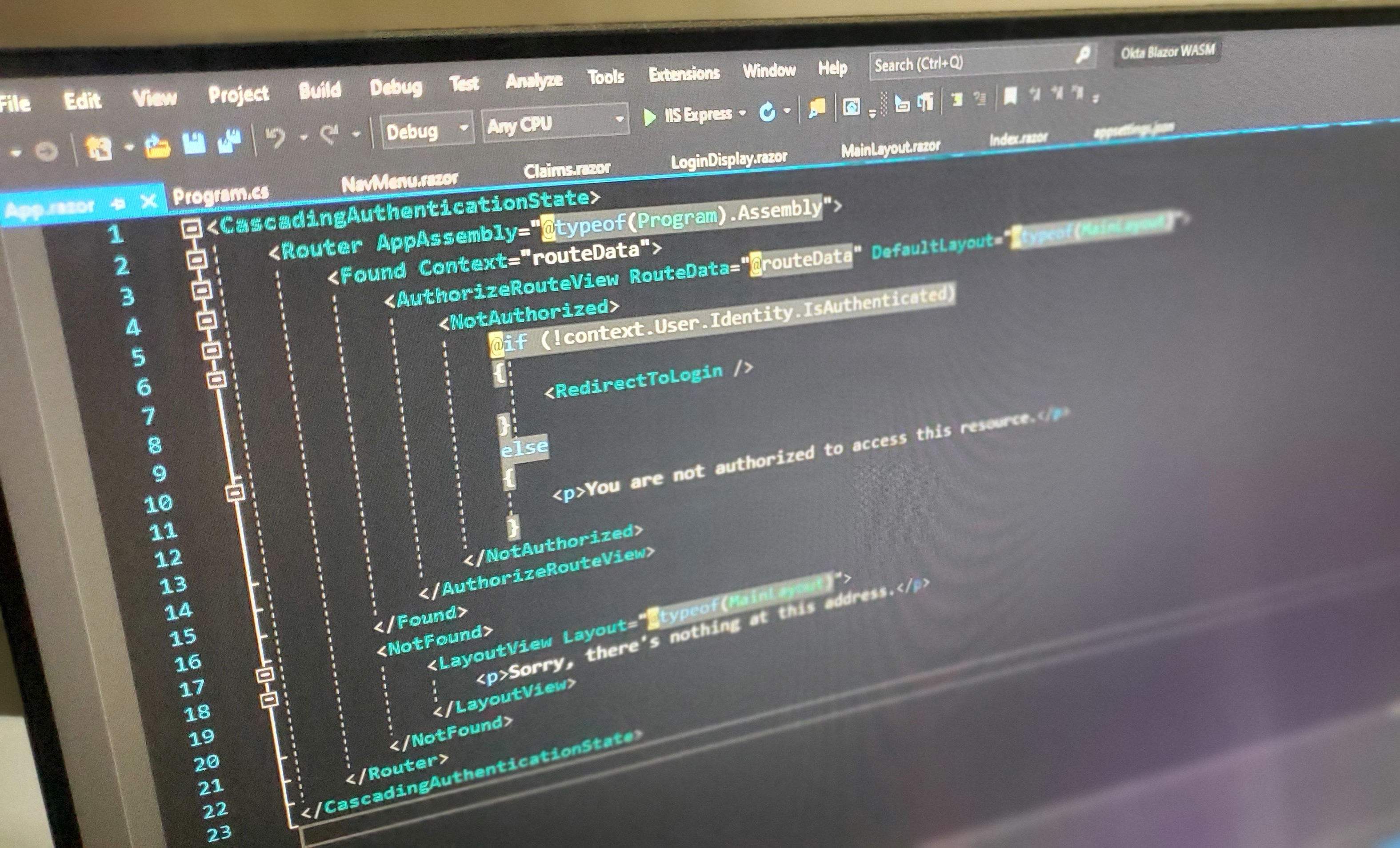
Last month I came out with a video tutorial on Blazor WebAssembly and I thought it would be good to follow up with a written tutorial as well. Youtube Link Because of the JS Interop, Blazor WASM has some key differences in the world of OAuth. Instead of thinking of this as a normal .NET back end application, you have to think of what Blazor resolves to in the browser - and that’s Javascript. Therefore,...
How to Adapt Your .NET App for SameSite
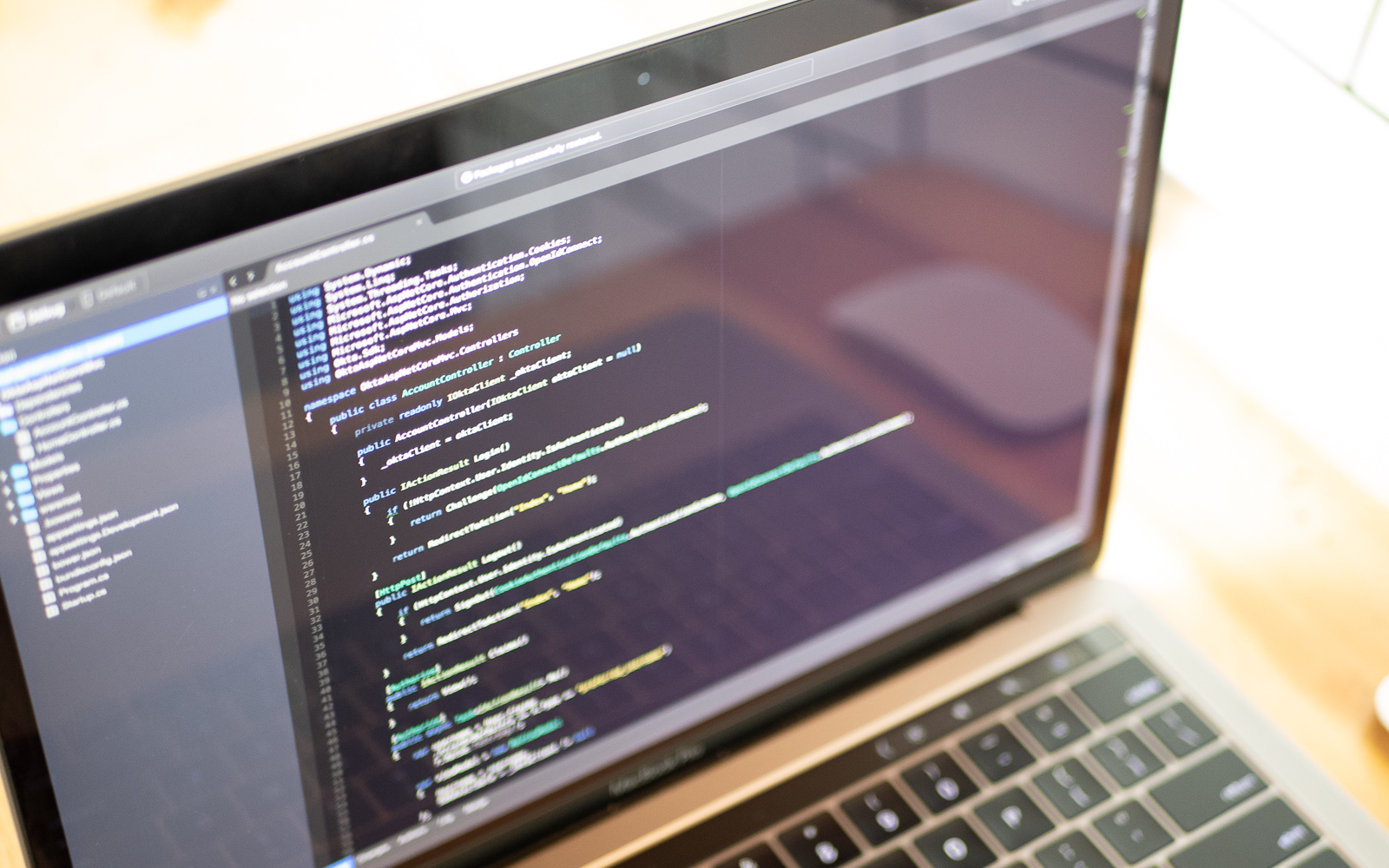
Have you run across an error message vaguely referencing SameSite in your .NET Apps? Read on, it’s time for a change to your code - and I’ll explain why. I like cookies, both the custard stuffed and the dry ones (which I use to dunk in my coffee or tea). This post is very much about cookies - only not the delicious, culinary ones. As in many other cases (think of the web, for example),...
Migrate Your ASP.NET Framework to ASP.NET Core with Okta
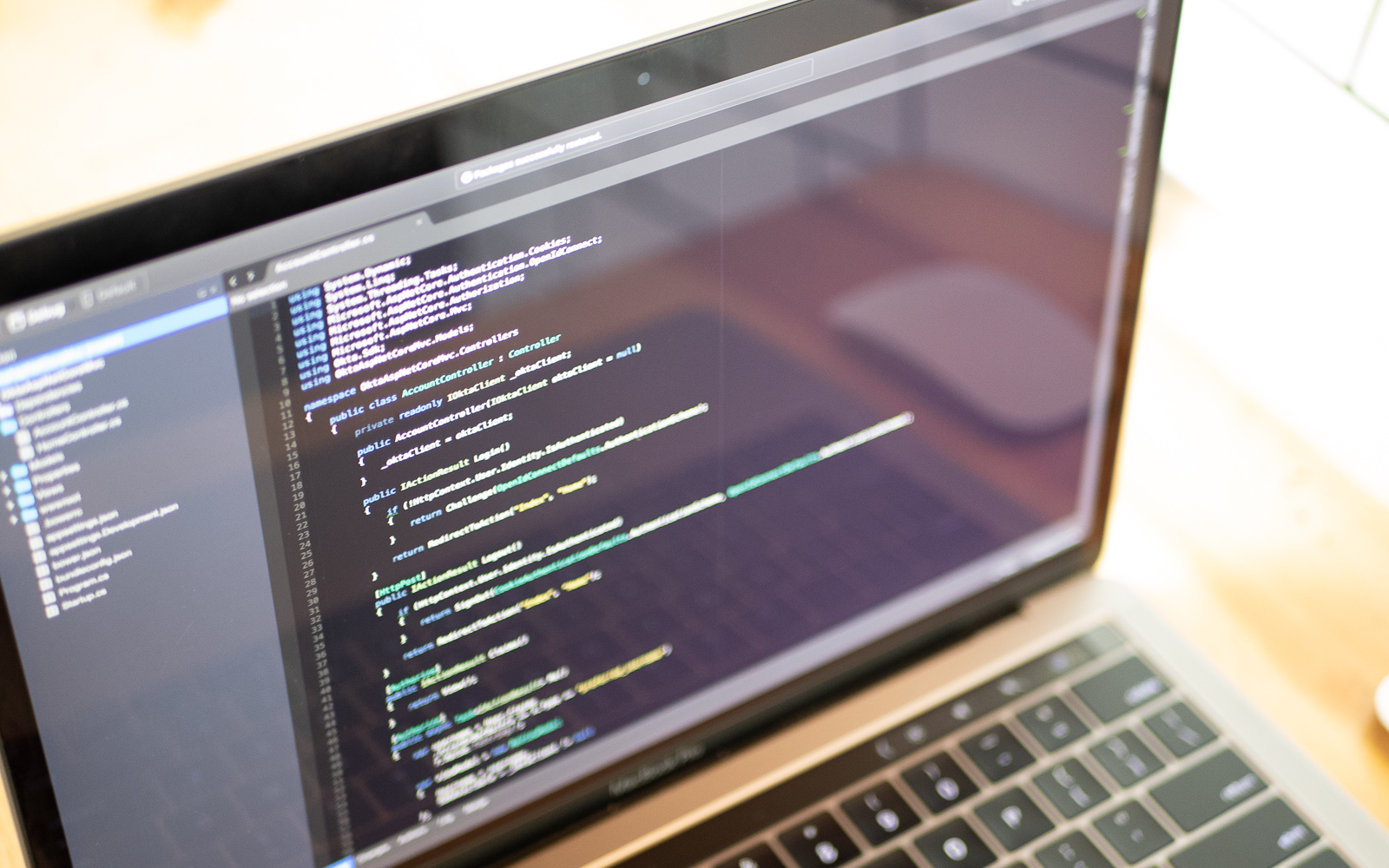
Ah, migration! Let’s say you have an ASP.NET application that has been running fine for years. You have kept up with the various .NET Framework updates and then suddenly you get told that you need to migrate to the latest and greatest, ASP.NET Core using .NET Core. .NET Core is the successor to the .NET Framework we’ve been using for years. It is open-source and supports cross-platform applications. To a veteran .NET developer it should...
How to Make a CRUD App with Entity Framework Core
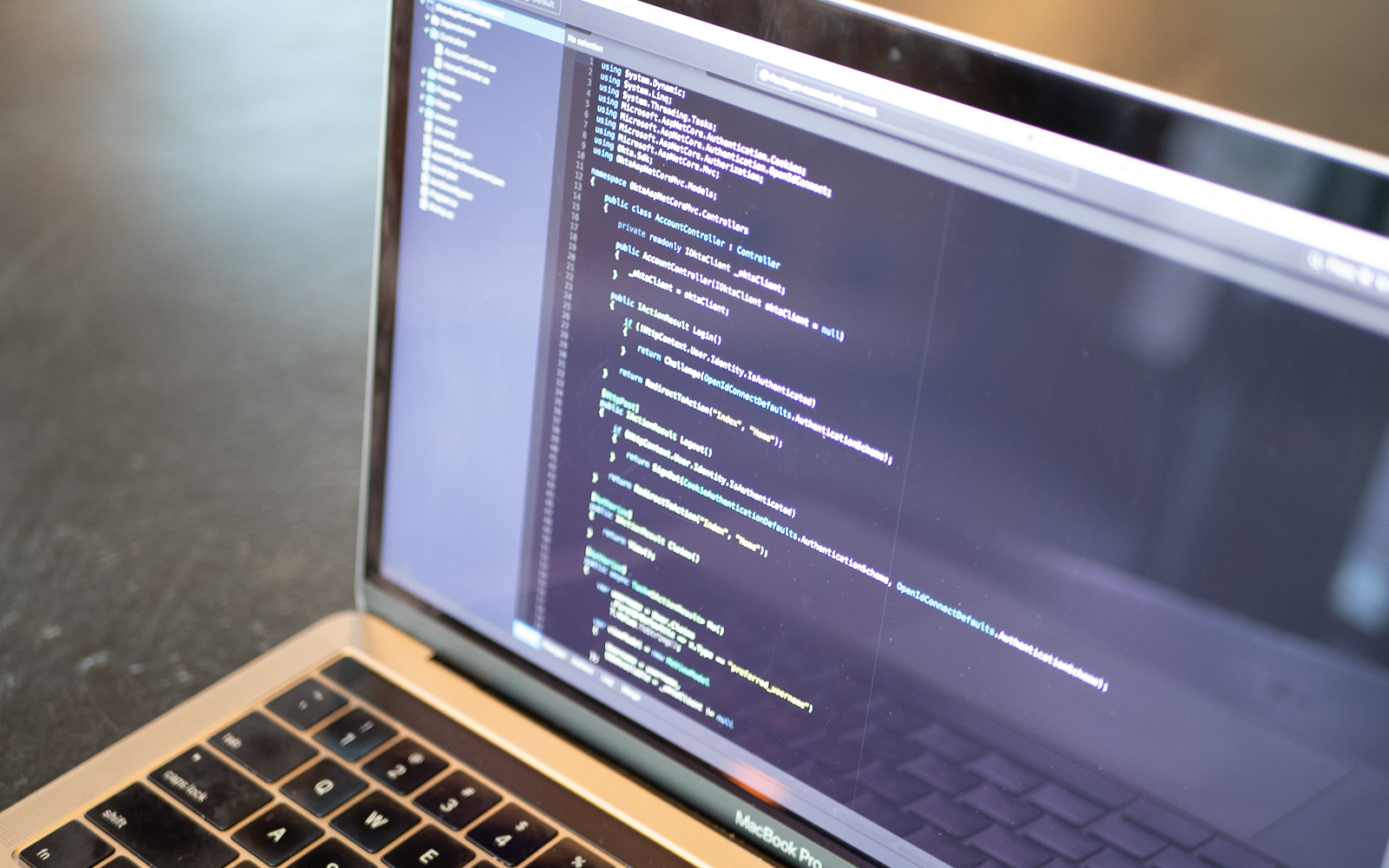
ASP.NET Core is Microsoft’s latest iteration of its web framework, which boasts many upgrades over the .Net Framework versions of ASP.NET. ASP.NET Core is cross-platform—meaning you can finally deploy your ASP.NET apps on Linux. It’s open-source which is a massive departure from Microsoft’s previous line of thinking. Most of the new framework should be familiar to veteran ASP.NET developers however there are some new tweaks to get used to. One of the most popular tools...
ASP.NET Core 3.0 MVC Secure Authentication
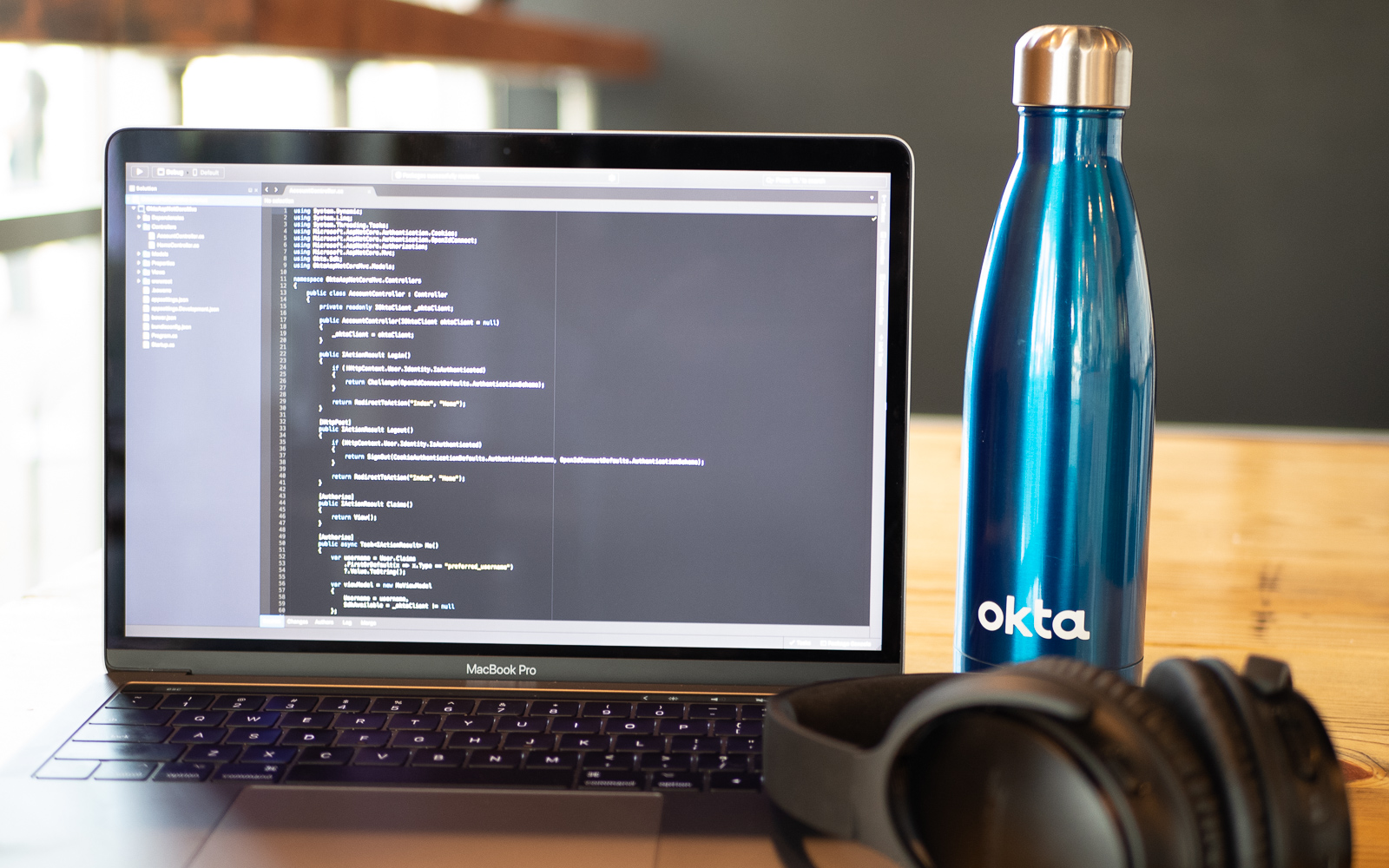
On September 23rd, Microsoft announced the third major release of its .NET Core framework. This new release boasts better performance, support for Windows Desktop apps, improved support for Docker containers, and more. Naturally, I was excited to see this new release and get authentication hooked into it with Okta! I put together this tutorial to demonstrate how to quickly and securely set up user management with Okta and OIDC (OpenID Connect) in an ASP.NET Core...
Build a Simple CRUD App with ASP.NET Core, MySQL, and Twilio
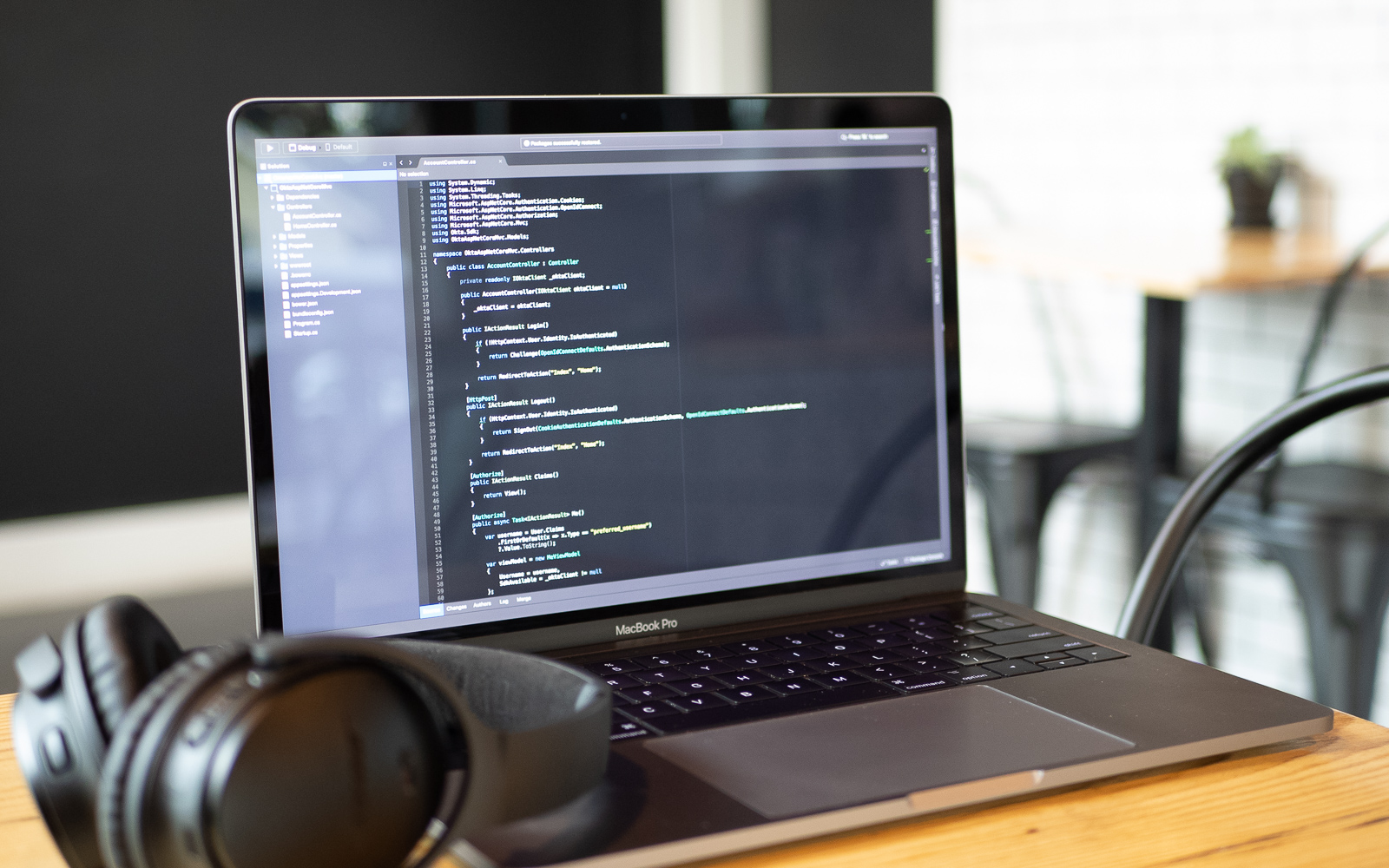
In this article, we’ll be building an MVC task management form with .NET Core 2.2. We’ll also be showing how to integrate with a MySQL database from MVC, and how to use Twilio to send SMS messages to your app that will add new tasks to your list. .NET Core and MySQL are both free and open source technologies. The new ASP.NET Core can run on Linux and in Linux Containers, and MySQL is one...
IInterface Considered Harmful
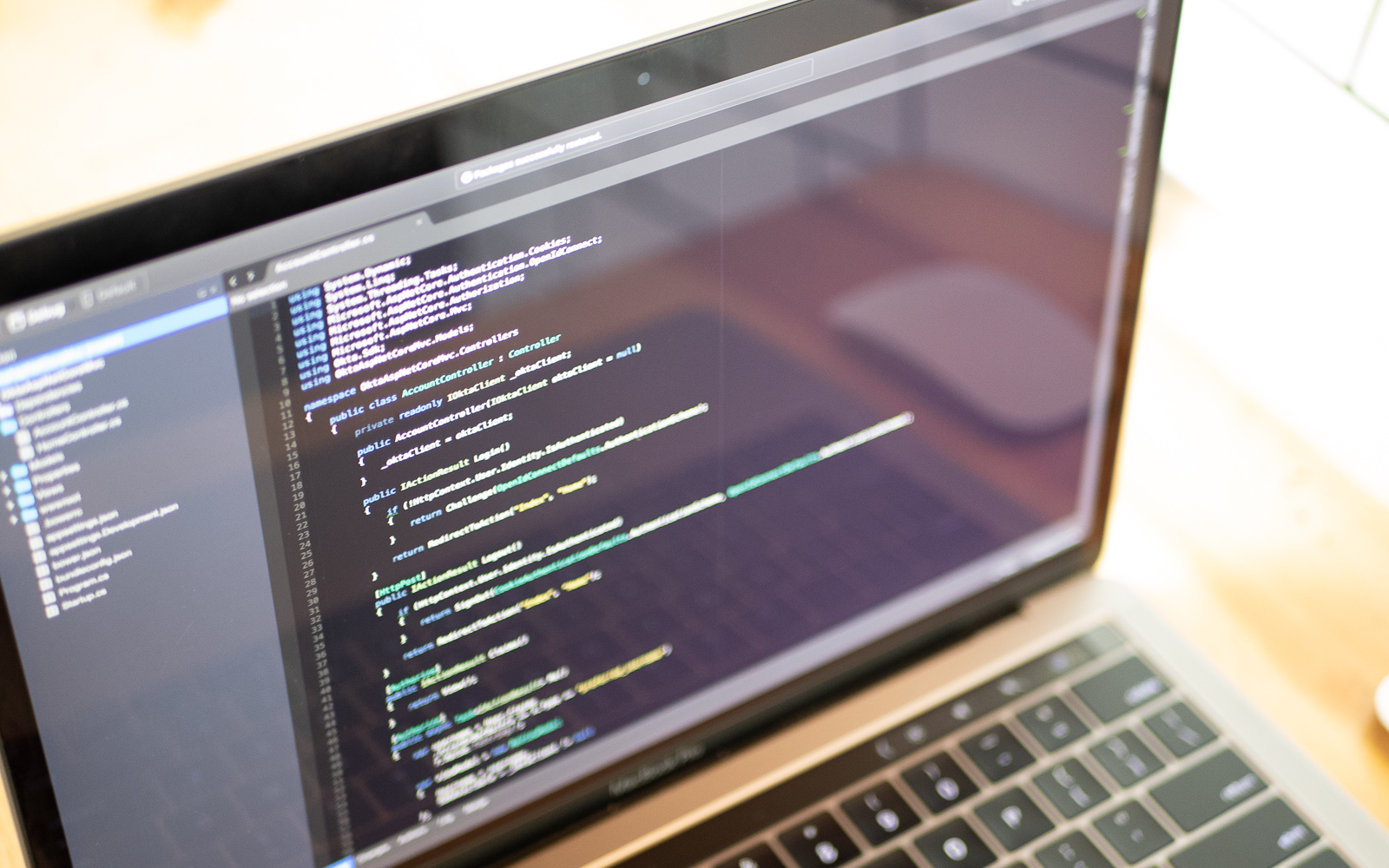
Developers have been lauded as being early adopters when it comes to technology products, but they seem to be late bloomers when it comes to dropping old habits. It took years of convincing and some guidance from Microsoft to get .NET developers to stop using Hungarian Notation in their programs, but there’s is one last “comfort blanket” it left: developers still use the “I” prefix for interfaces. I want it to stop. Let it go....
Build a CRUD App with ASP.NET Core 2.2 and SQL Server
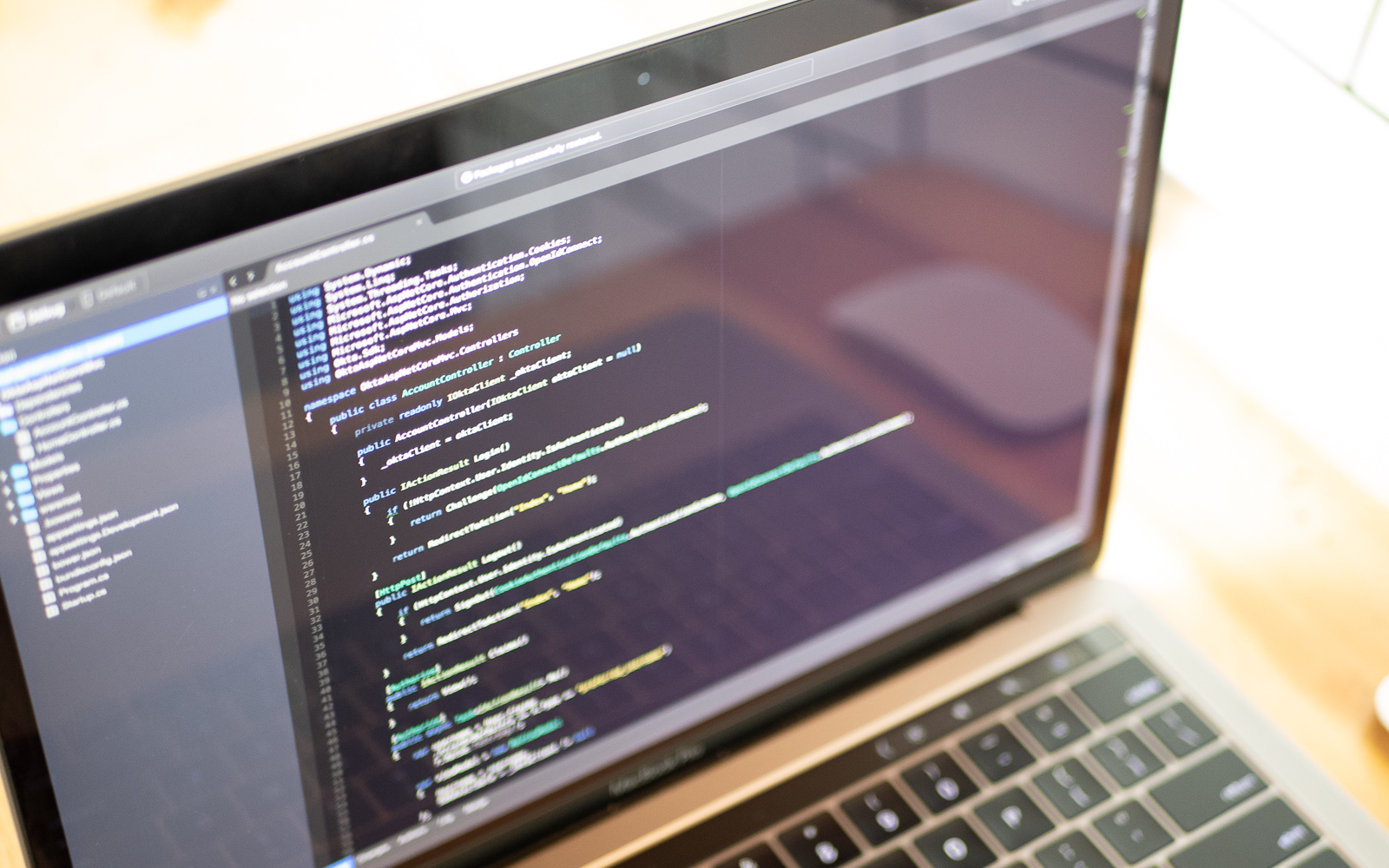
I’ve always said that you can tell a lot about a person by the kind of music they listen to. Don’t tell me you haven’t had serious doubts about whether you can be friends with someone when you find out that they like a particular band or artist. In that spirit, I created JudgeMyTaste, an ASP.NET Core web application where people can enter their favorite band or artist so that people on the Internet can...
Build a GraphQL API with ASP.NET Core
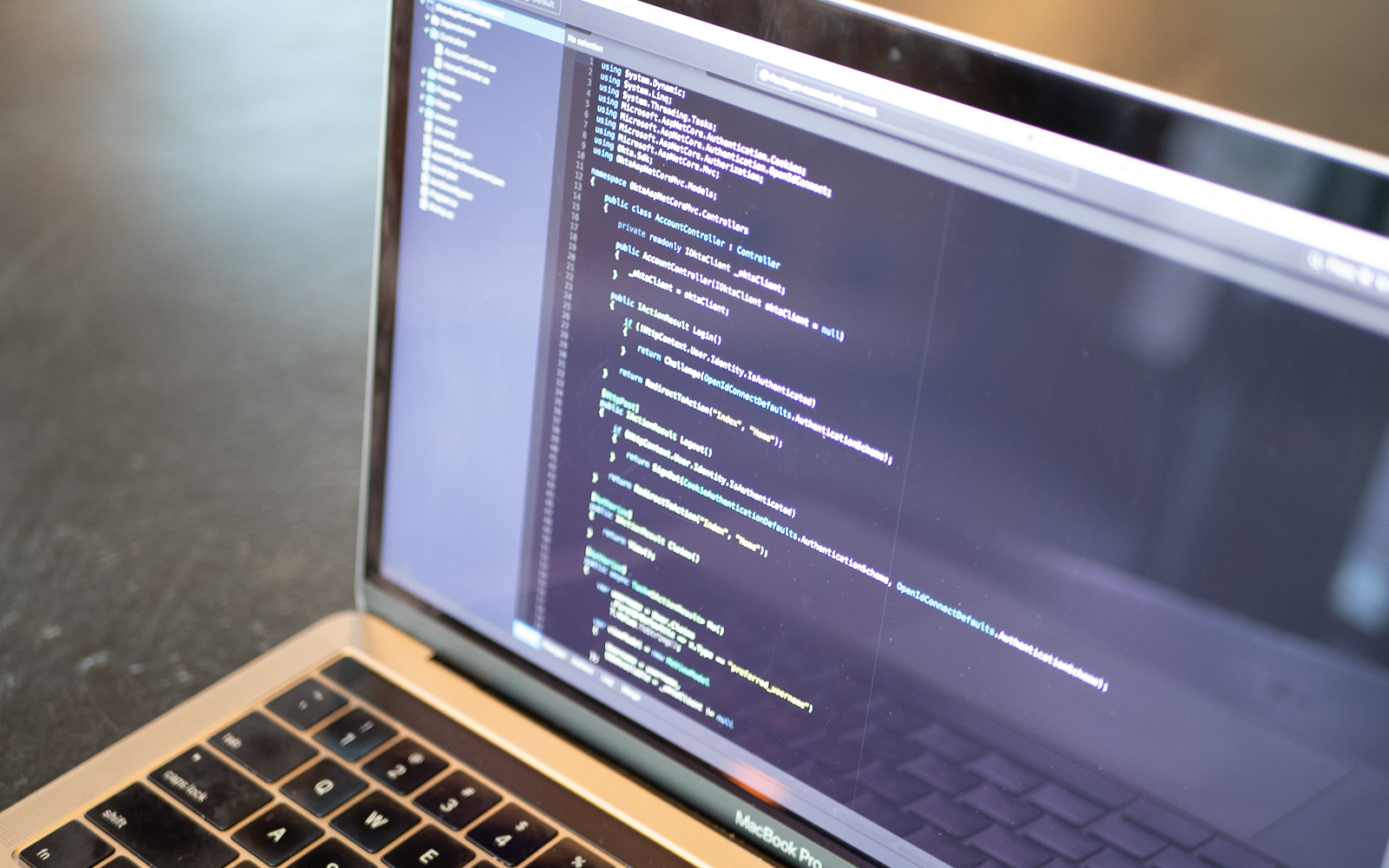
“There is no Frigate like a Book To take us Lands away…” – Emily Dickinson These days, people read more on social media than they do in books. Maybe the remedy is to make reading more social. To that end, we’ll create an API for books. This will be an ASP.NET Core 2.2 Web API using GraphQL, which will allow developers to create applications that consume the API securely. ASP.NET Core 2.2 provides the ability...
Build a REST API with ASP.NET Core 2.2
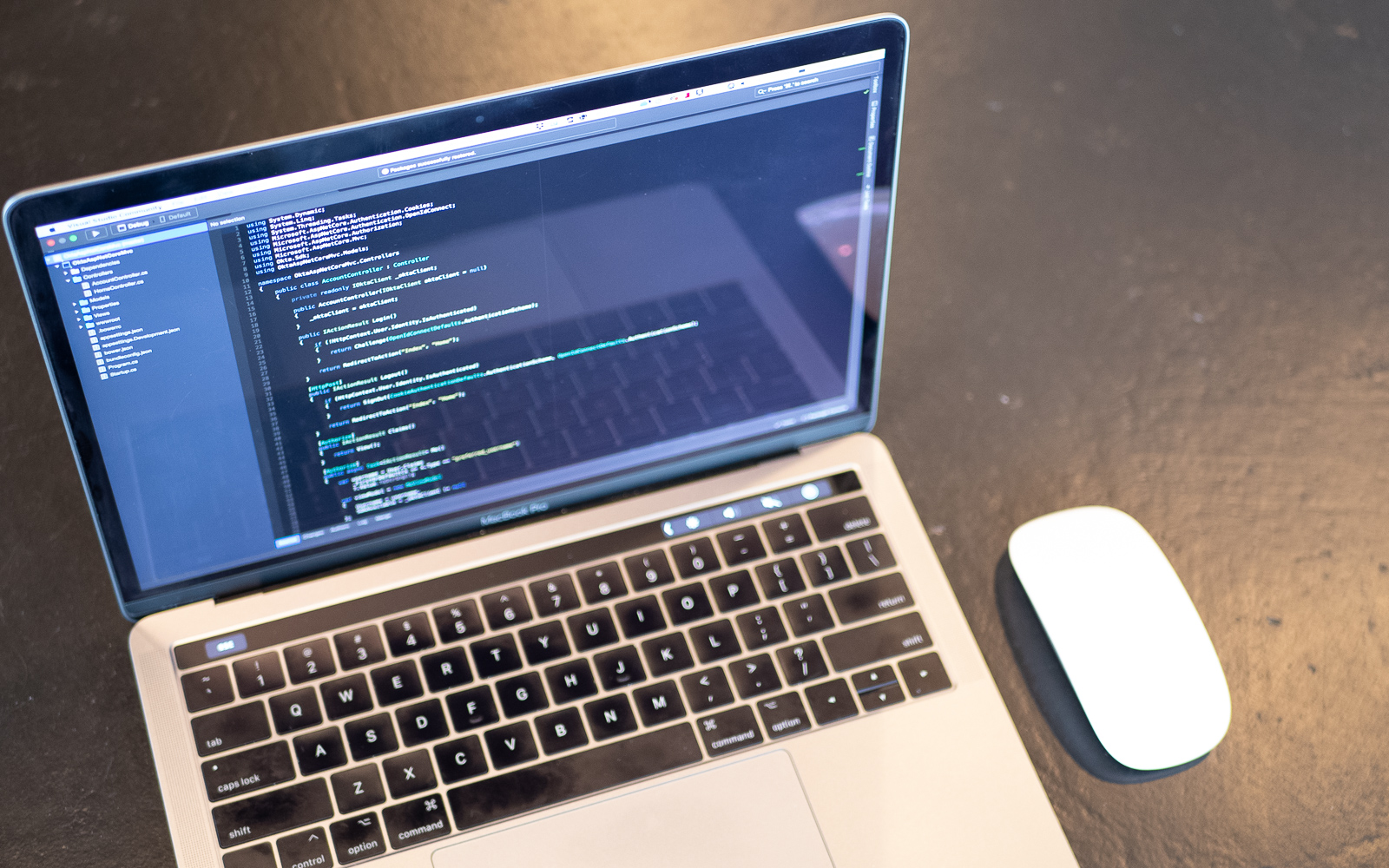
ASP.NET Core is entirely open source, free, has built-in DI and logging, works smoothly with a fantastic ORM and has tons of built-in features within Web API framework, and on top of that you get Microsoft support for free, maturity and flexibility of C# and ASP.NET, it’s evident that ASP.NET Core is easily one of the best picks for building REST APIs. Lots of folks keep a daily journal that is essentially a detailed log...
Build a CRUD App with ASP.NET Core 2.2 and Entity Framework Core
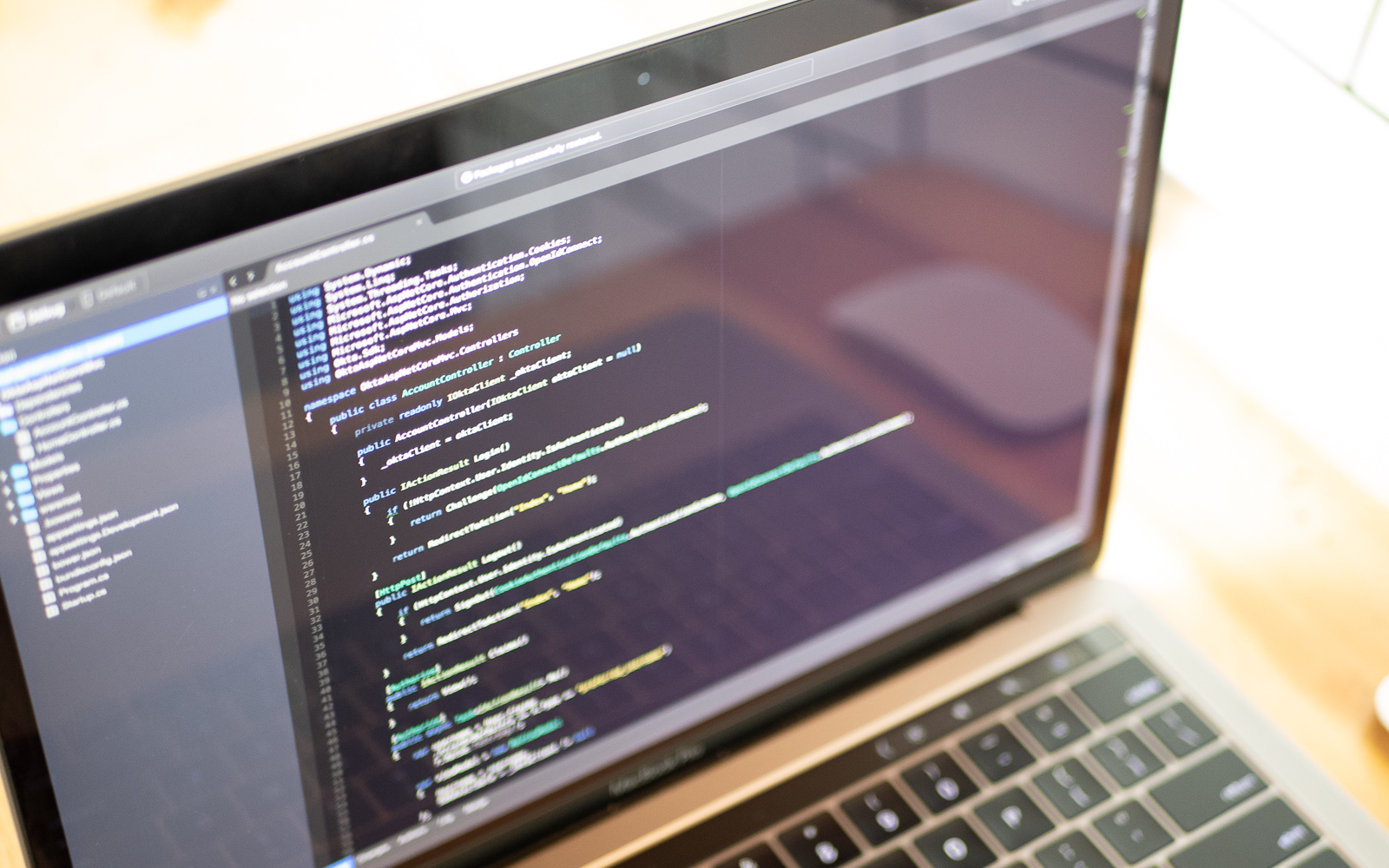
If you’re like me, you love music. Music is always streaming somewhere in my house at all times. I especially like going to see live music, but it can be hard to know where and when live music is happening. LiveMusicFinder is a web application that allows users to enter when and where some live music is going down. This beta version is very rough, but I will show you how I built it with...
Build a CRUD App with ASP.NET Core and TypeScript
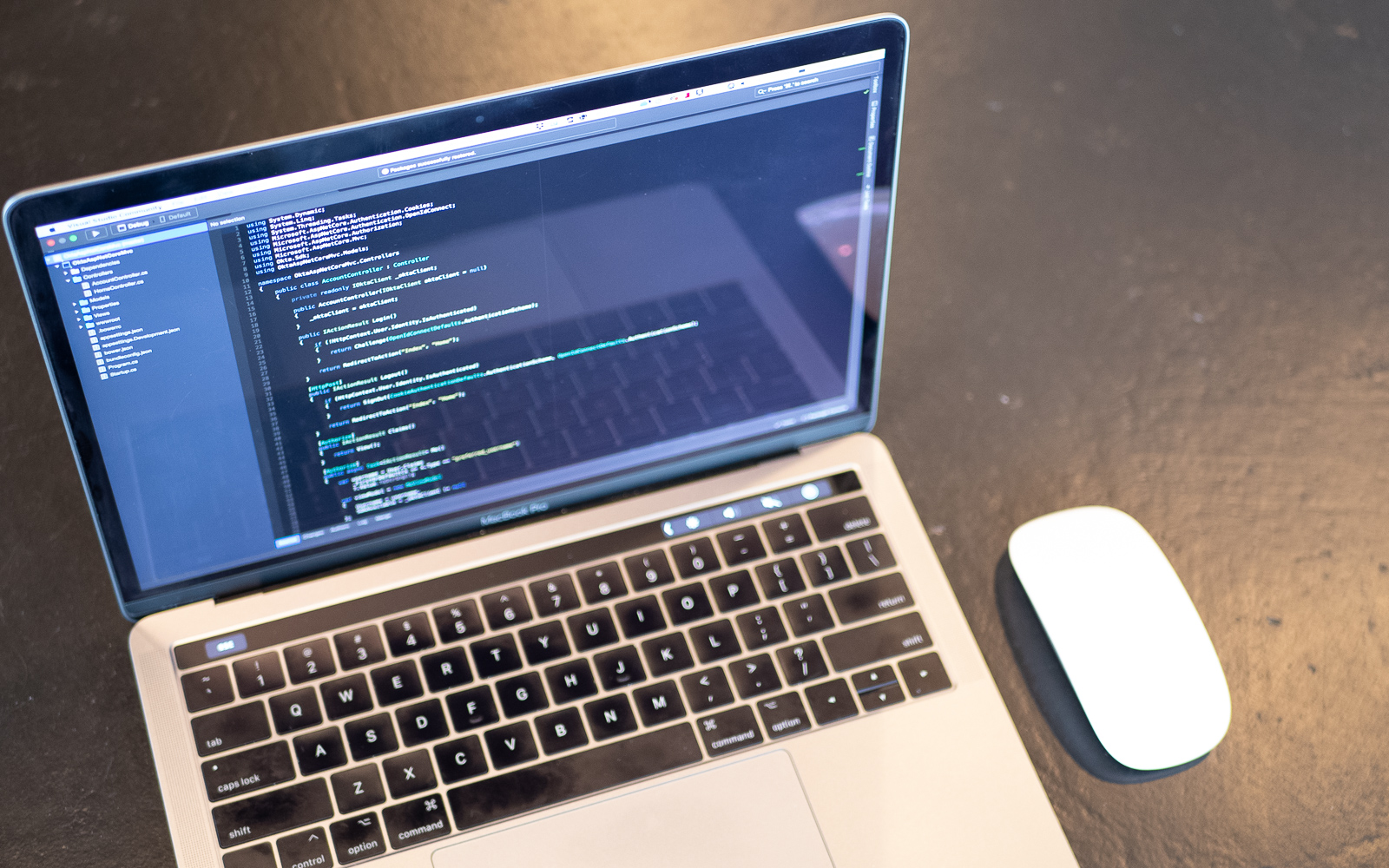
There are a lot of things for .NET developers to love about TypeScript. It has strong typing that .NET Developers are used to and the ability to use the latest JavaScript features. Since it is just a superset of JavaScript, the cost to switch is almost nothing. Getting Visual Studio to transpile the TypeScript when it builds your ASP.NET Core app is pretty simple as well. In this tutorial, you will build an ASP.NET Core...
Build Secure Microservices with AWS Lambda and ASP.NET Core
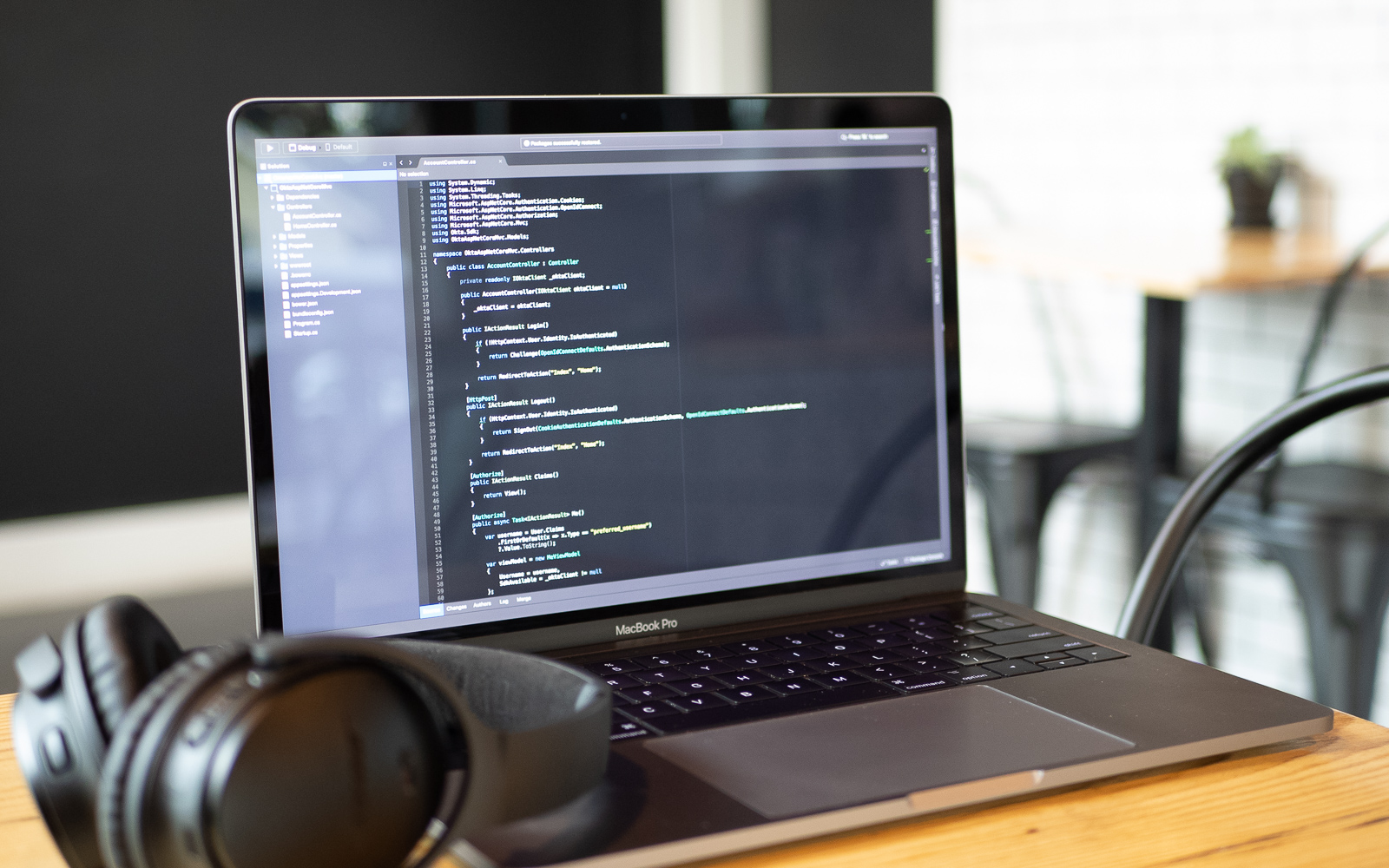
Microservices are fun to build and offer us a scalable path to overcoming problems with tightly coupled dependencies that plague monolithic applications. This post will walk you through building an AWS Lambda microservice written in C# with .NET Core 2.1, and communicating in JSON. We’re bringing together multiple exciting technologies here - microservices, serverless API via AWS Lambda, and authentication using Okta’s easy and convenient identity provider. Each of these technologies is deserving of their...
Create Login and Registration in Your ASP.NET Core MVC App
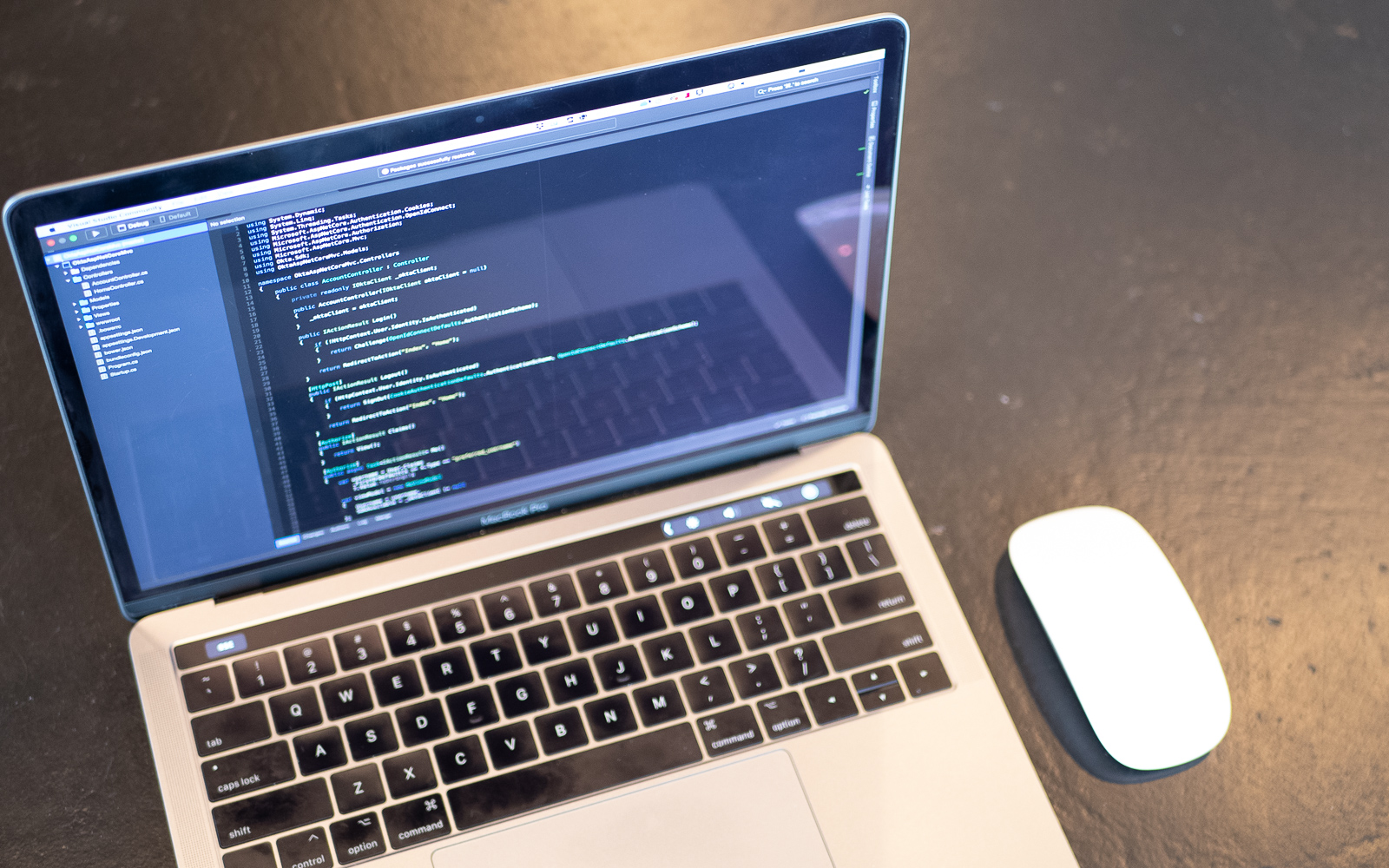
User authentication and authorization are common features in web applications, but building these mechanics has the potential to take a lot of time. Doing so requires setting up persistent storage for user information (in some type of database) and paying keen attention to potential security issues around sensitive operations like hashing passwords, password reset workflows, etc. - weeks of development time begin to add up before we ever get to the functionality that delivers value...
Go for liftoff at Okta!
T-minus 3… 2… 1… I’m blasting my way into the Developer Relations world like Alice discovering Wonderland and its fantastic cast of characters. I am so curious, and excited, and all of the feels that come with coming to Okta! Officially stepping into the Developer Avocado (er… pardon me, Advocate) role and bringing the love of .NET, voice and IOT to the party. Who is this Lady Nerd? Well, for starters I am obsessed with...
Navigating the ASP.NET Core Identity Landscape
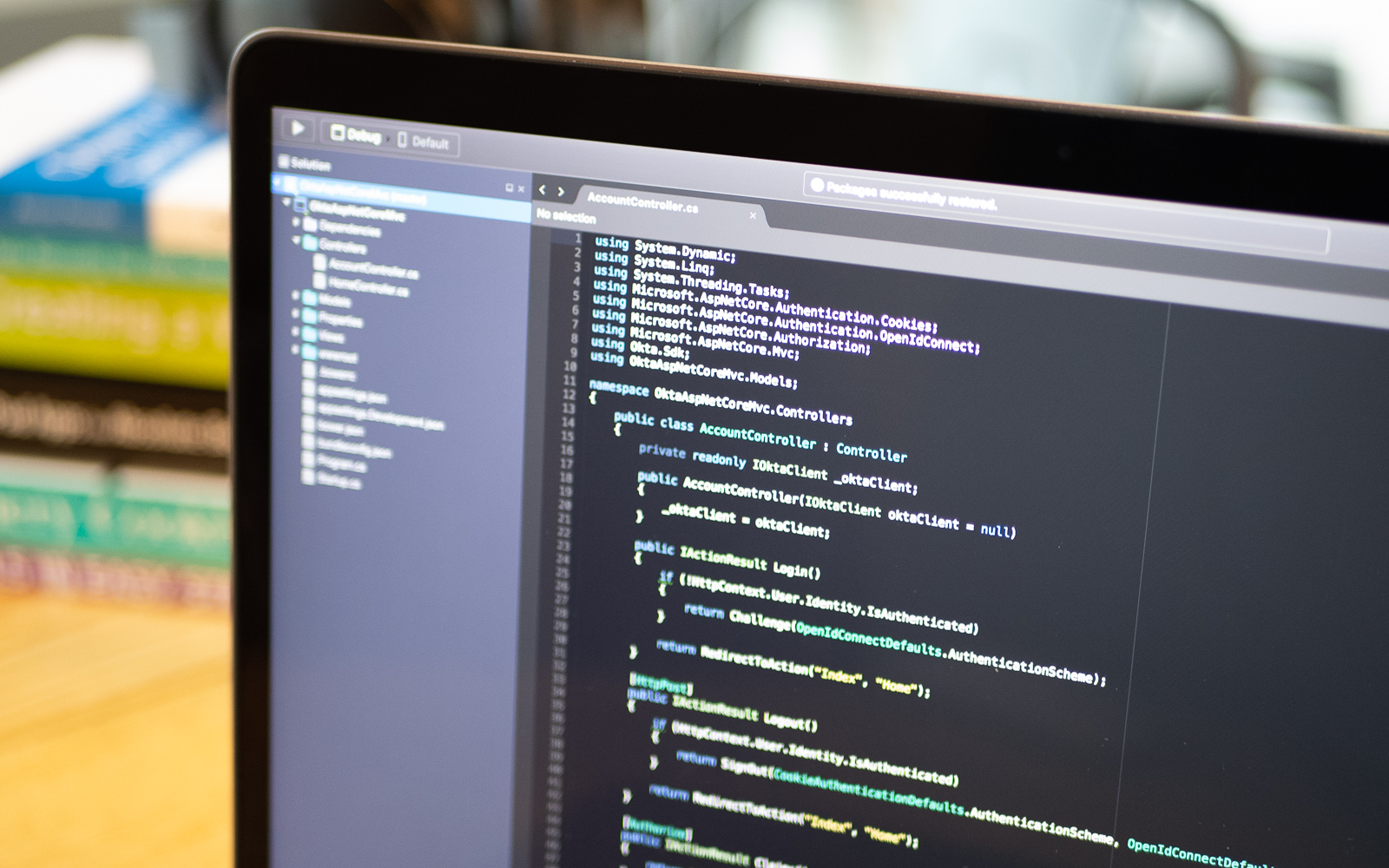
These days, users of web application expect a personalized, secure experience. They want to make sure that they can easily get to their own information, and that no one else can access it. Developers of these web applications want a simple way to manage users and be assured that they have secured their users’ information from prying eyes. For developers of web applications written in ASP.NET Core, there are several choices for managing user identities....
Add Login to Your ASP.NET Core MVC App
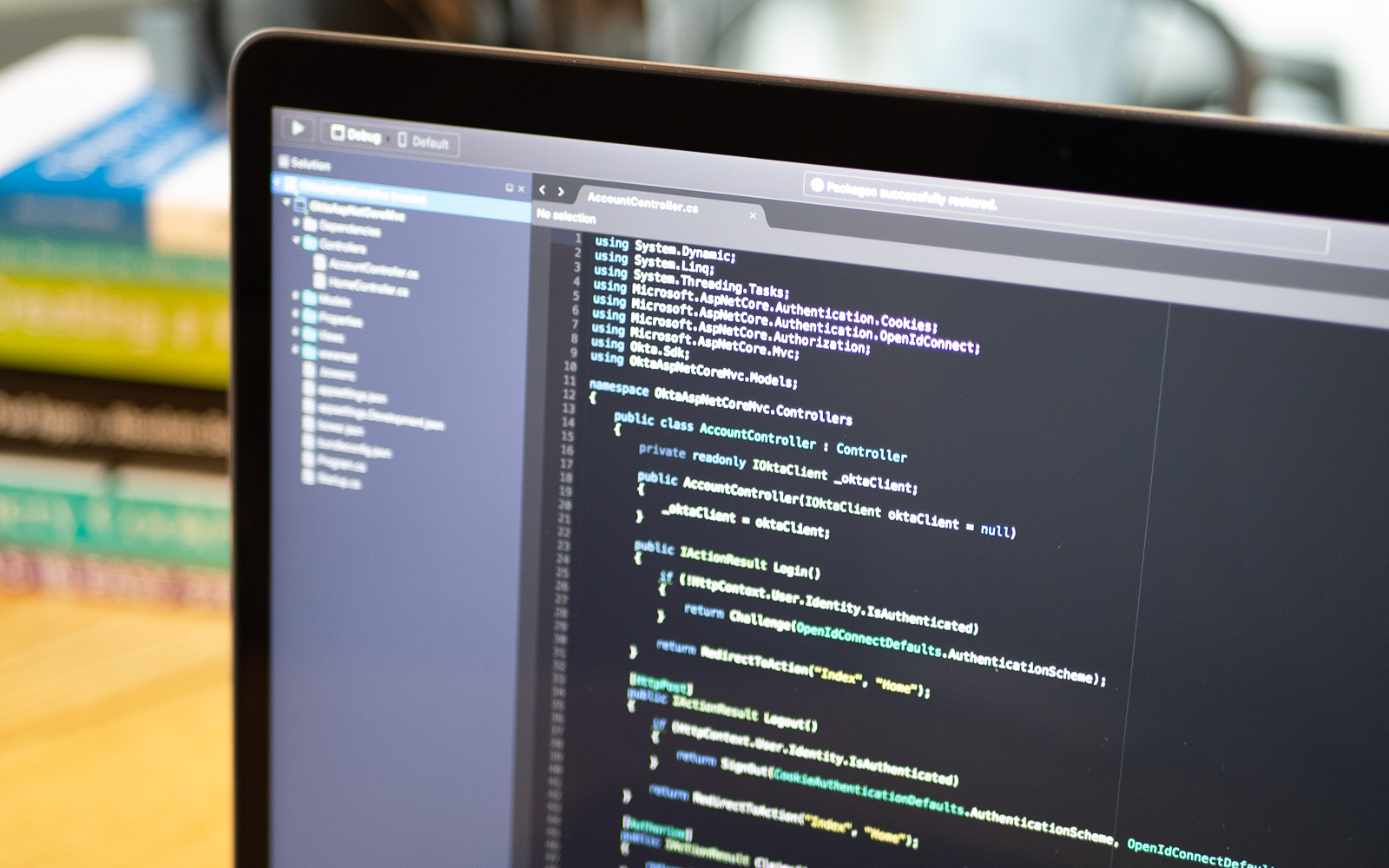
One of the improvements in the latest version of ASP.NET Core (MVC ) is a new and simplified authentication integration. However, managing authentication on your own is still a considerable burden. Not only do you need to handle signup and login, but you also have to set up the database, manage security aspects of registration and login, and take care of session management. Since the integration of external auth providers has never been more comfortable,...
Build a Simple CRUD App with ASP.NET Core and Vue
Keeping an eye on your daily calorie intake can be crucial to healthy lifestyle. There are a ton of apps on the market that will help you do this, but may be bloated with extra features or just full of ads. The app we’ll build today is a bare-bones stand-in for any of those, as a demonstration of these technologies, and a great stand-in if simple calorie tracking is all you really need. Note: In...
Deploy Your ASP.NET Core Application to Azure
One of the scariest features of Visual Studio is without a doubt right-click to publish. There are very few instances in which you’d actually want to make use of this “feature” and so many more in which it’s a terrible idea. In this post I’ll walk you through the right way to secure and deploy your new ASP.NET Core app to Azure. My method might not be as fast as right clicking to publish, but...
Build a CRUD App with ASP.NET Core and Angular
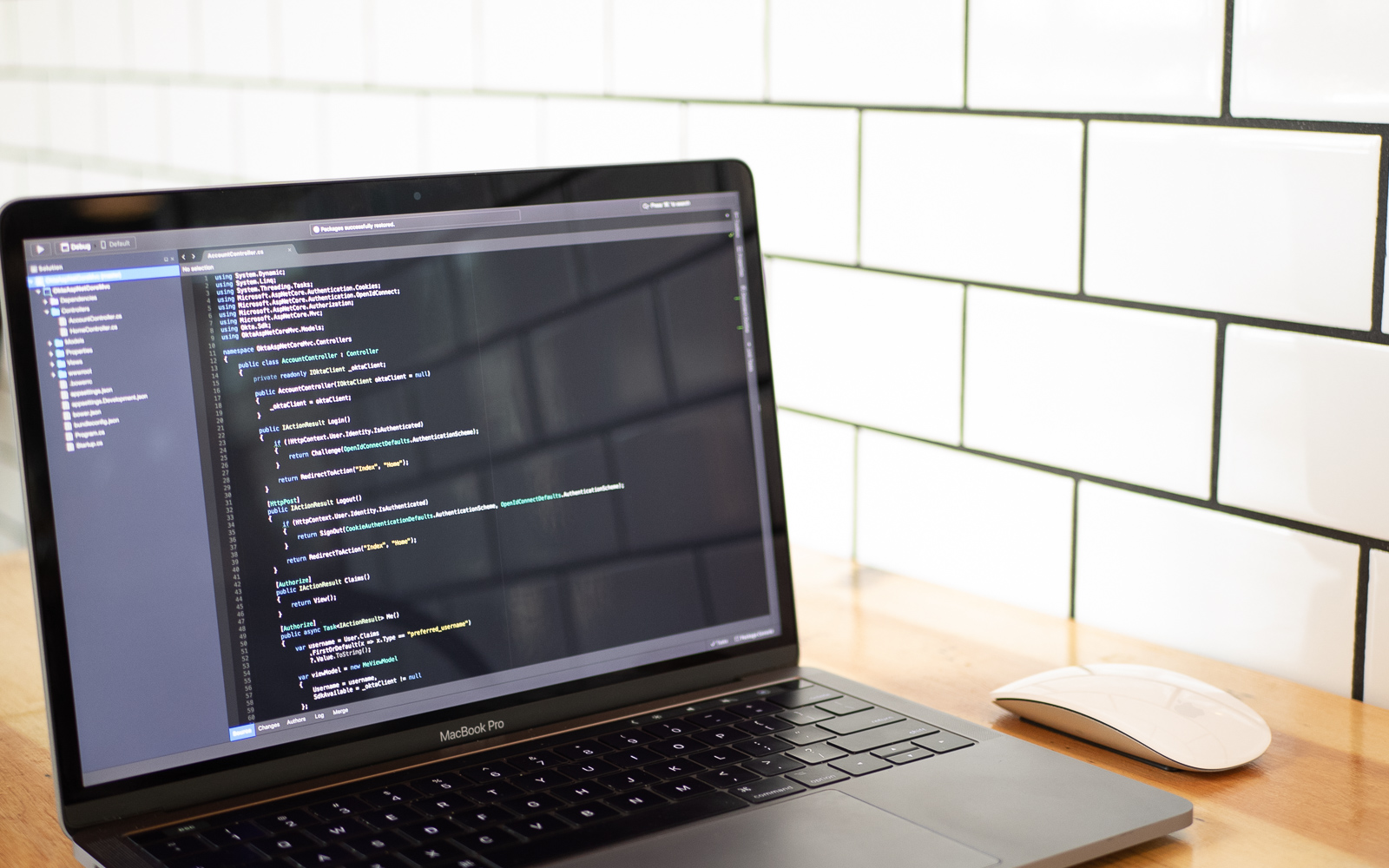
A lot of applications today are built with an API on the backend, and then a single page application on the front end. This is a good approach because it allows you a ton of flexibility. For example, if you get a requirement to build a native mobile client later on: it’s easy, you already have the server side in place. Today you’ll use ASP.NET Core 2.0 on the server side, and Angular 5 on...
Token Authentication in ASP.NET Core 2.0 - A Complete Guide
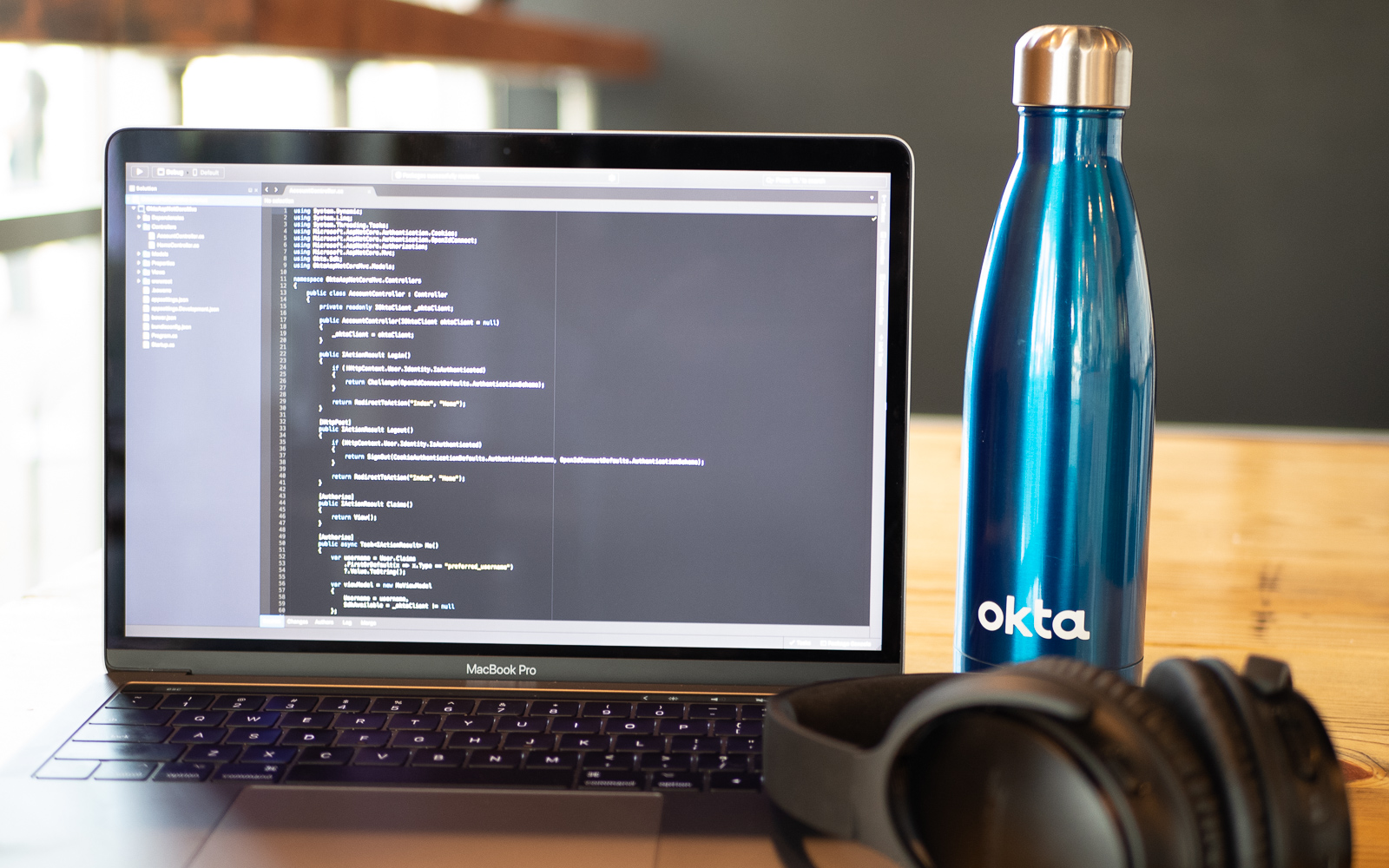
Token authentication has been a popular topic for the past few years, especially as mobile and JavaScript apps have continued to gain mindshare. Widespread adoption of token-based standards like OAuth 2.0 and OpenID Connect have introduced even more developers to tokens, but the best practices aren’t always clear. I spend a lot of time in the ASP.NET Core world and have been working with the framework since the pre-1.0 days. ASP.NET Core 2.0 has great...
Build a Secure To-Do App with Vue, ASP.NET Core, and Okta
I love lists. I keep everything I need to do (too many things, usually) in a big to-do list, and the list helps keep me sane throughout the day. It’s like having a second brain! There are hundreds of to-do apps out there, but today I’ll show you how to build your own from scratch. Why? It’s the perfect exercise for learning a new language or framework! A to-do app is more complex than “Hello...
User Authorization in ASP.NET Core with Okta
Authorization is the oft-forgotten piece of identity and access management. The fact is, almost every app needs more than just “are they signed in?” for authorization. Most times, you need to not only know who “they” are, but what access they are supposed to have. For instance, “are they in the administrator group?” or “are they in a group with some special privileges?” Today, you’ll learn how to do this with Okta in an ASP.NET...