Articles tagged spring
How to Deploy Java Microservices on Amazon EKS Using Terraform and Kubernetes
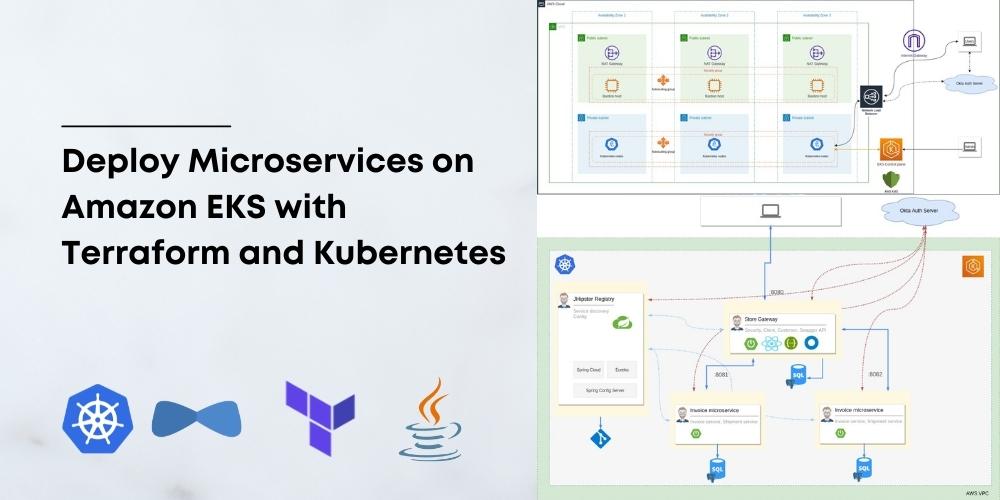
When it comes to infrastructure, public clouds are the most popular choice these days, especially Amazon Web Services (AWS). If you are in one of those lucky or unlucky (depending on how you see it) teams running microservices, then you need a way to orchestrate their deployments. When it comes to orchestrating microservices, Kubernetes is the de-facto choice. Most public cloud providers also provide managed Kubernetes as a service; for example, Google provides Google Kubernetes...
Cloud Native Java Microservices with JHipster and Istio
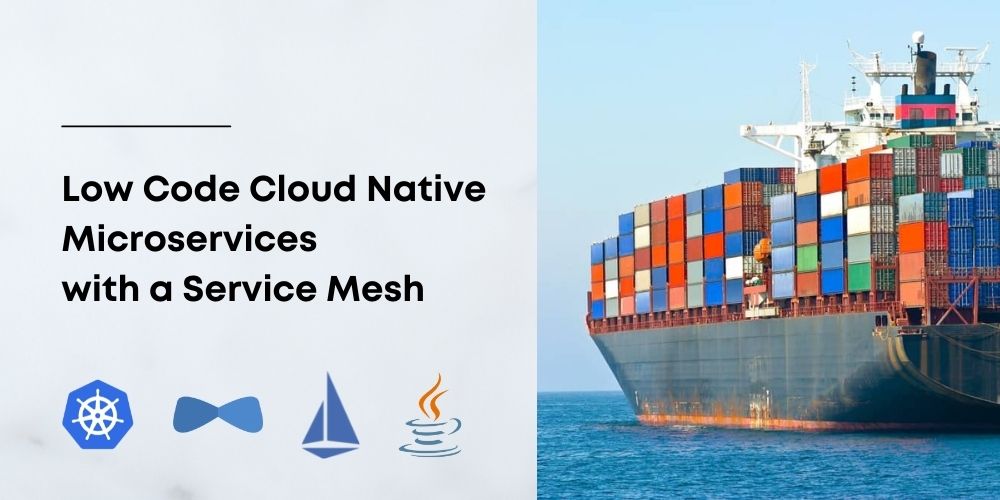
Microservices are not everyone’s cup of tea, and they shouldn’t be. Not every problem can or should be solved by microservices. Sometimes building a simple monolith is a far better option. Microservices are solutions for use cases where scale and scalability are important. A few years ago, microservices were all the rage, made popular, especially by companies like Netflix, Spotify, Google, etc. While the hype has died down a bit, genuine use cases still exist....
Three Ways to Run Your Java Locally with HTTPS
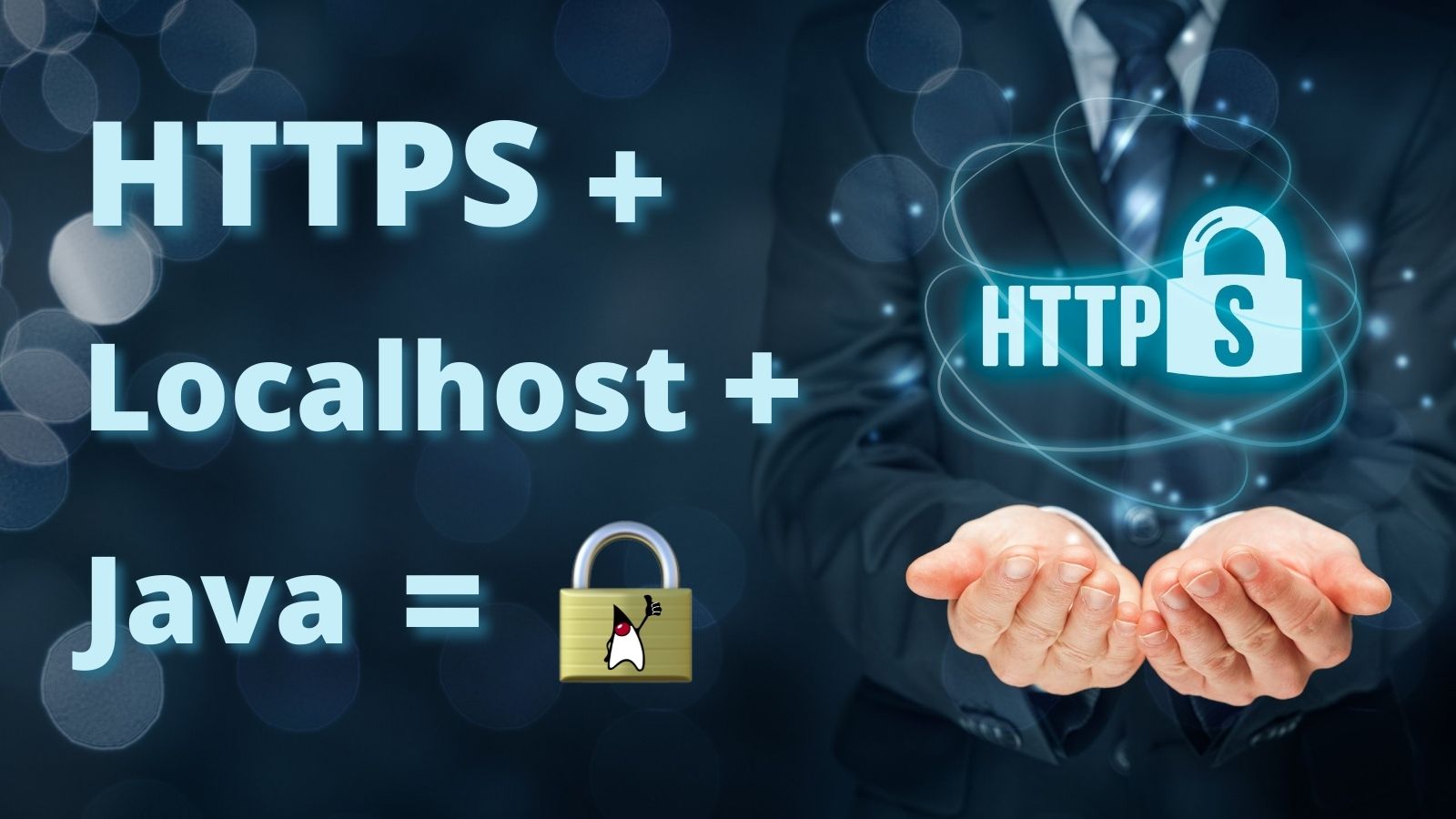
If you’re developing a web application, chances are you want your dev environment as close to production as possible. One of the often-overlooked differences between local development and production servers is the use of Transport Layer Security (TLS), or Hypertext Transfer Protocol Secure (HTTPS). In this post, I’ll cover three different options to get your local Java app running with TLS in no time! Table of Contents Start with a simple Java application HTTPS using...
Learn How to Build a Single-Page App with Vue and Spring Boot
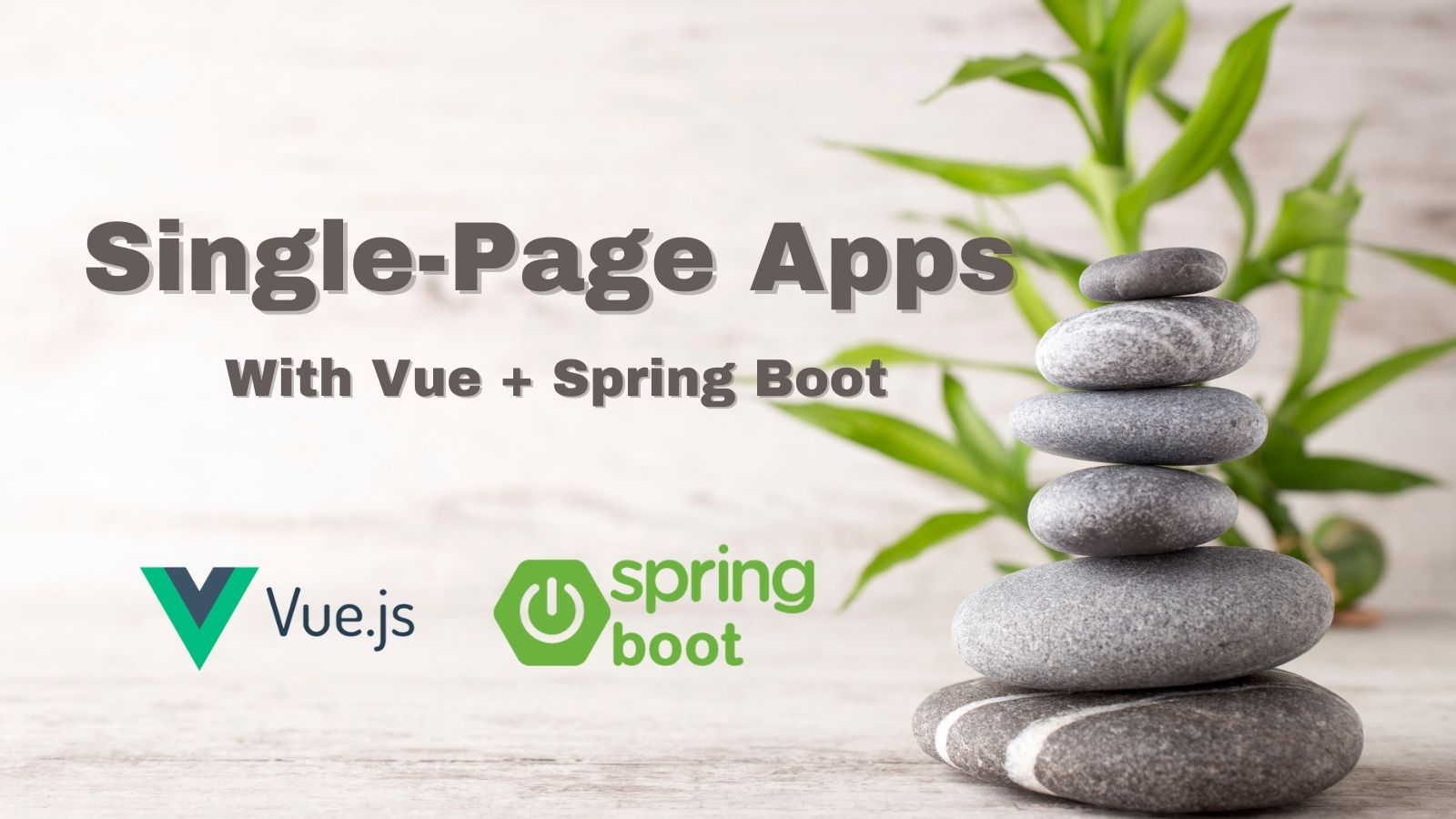
In this tutorial, you are going to create a single-page application (SPA) that uses a Spring Boot resource server and a Vue front-end client. You’ll see how to configure Spring Boot to use JSON Web Tokens (JWT) for authentication and authorization, with Okta as an OAuth 2.0 and OpenID Connect (OIDC) provider. You’ll also see how to bootstrap a Vue client app with the Vue CLI and how to secure it using the Okta Sign-In...
Session Clustering for OAuth 2.0 Applications
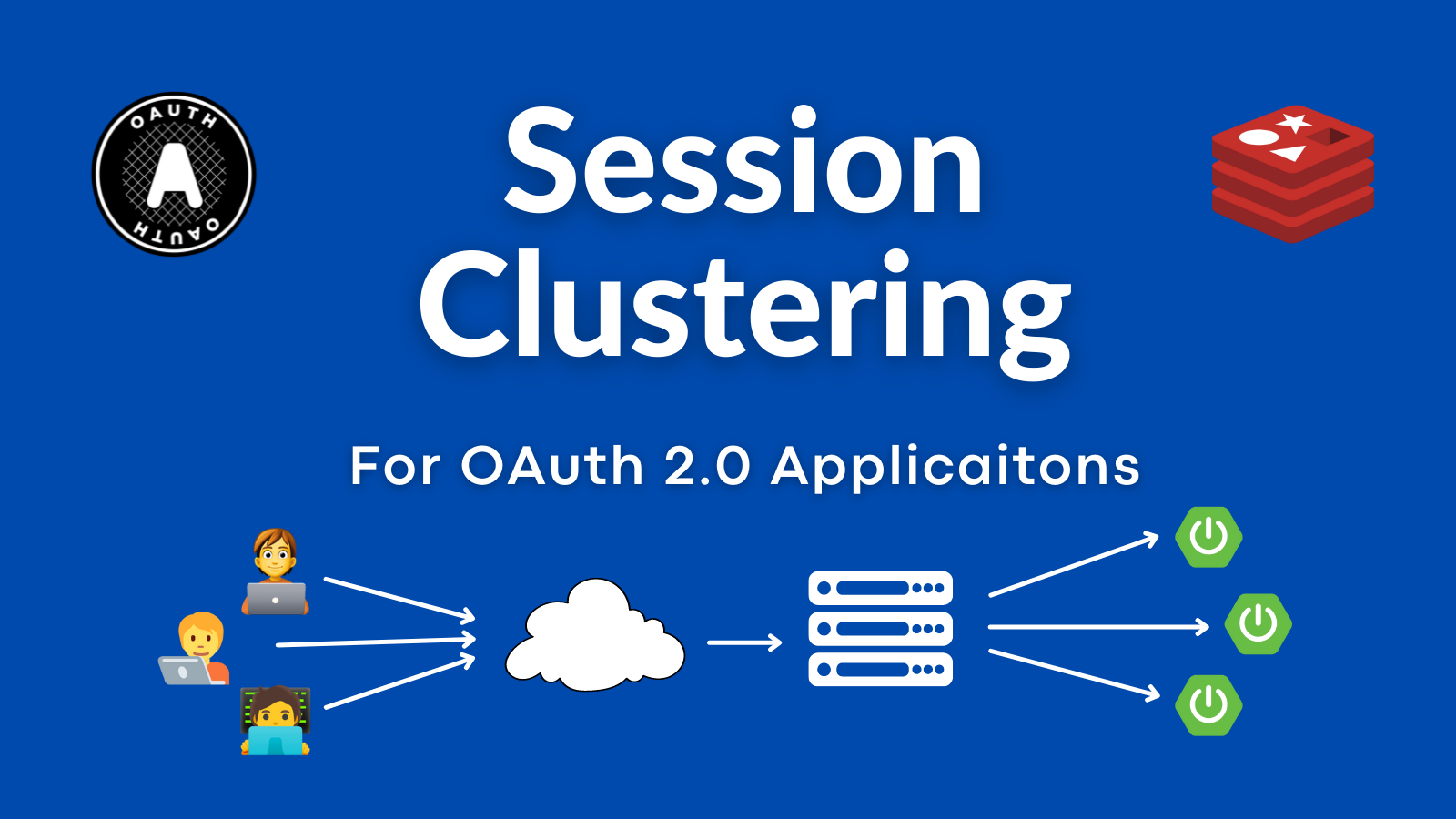
A common OAuth 2.0 question we get: "How do I deal with OAuth in a load-balanced application?" The short answer: There’s nothing specific about session clustering for OAuth. The longer answer is—you likely still need to worry about cluster session management. This post will discuss how an OAuth login relates to your application’s session. And we’ll build a simple, secure, load-balanced application to demonstrate. Note: In May 2025, the Okta Integrator Free Plan replaced Okta...
Spring Native in Action with the Okta Spring Boot Starter
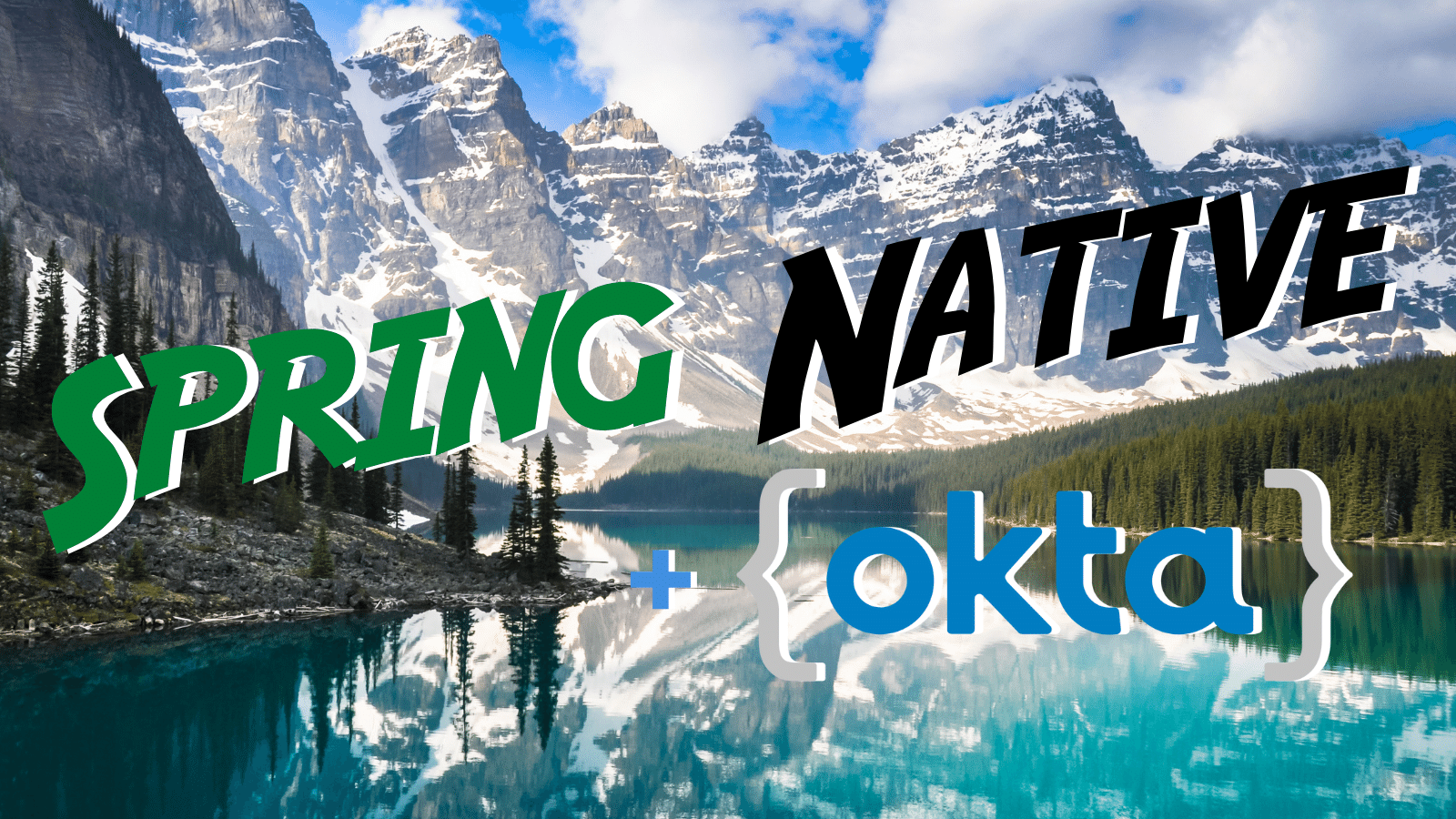
In the fall of 2020, the Spring team released a new experimental Spring Native project that gave Spring developers hope for faster startup times. Spring Native is all about converting your Spring applications to native executables. It leverages GraalVM to make it happen. This announcement was huge because the new kids on the block, Micronaut and Quarkus, produced native executables by default. I was really excited about Spring Native when I first heard about it....
Spring WebClient for Easy Access to OAuth 2.0 Protected Resources
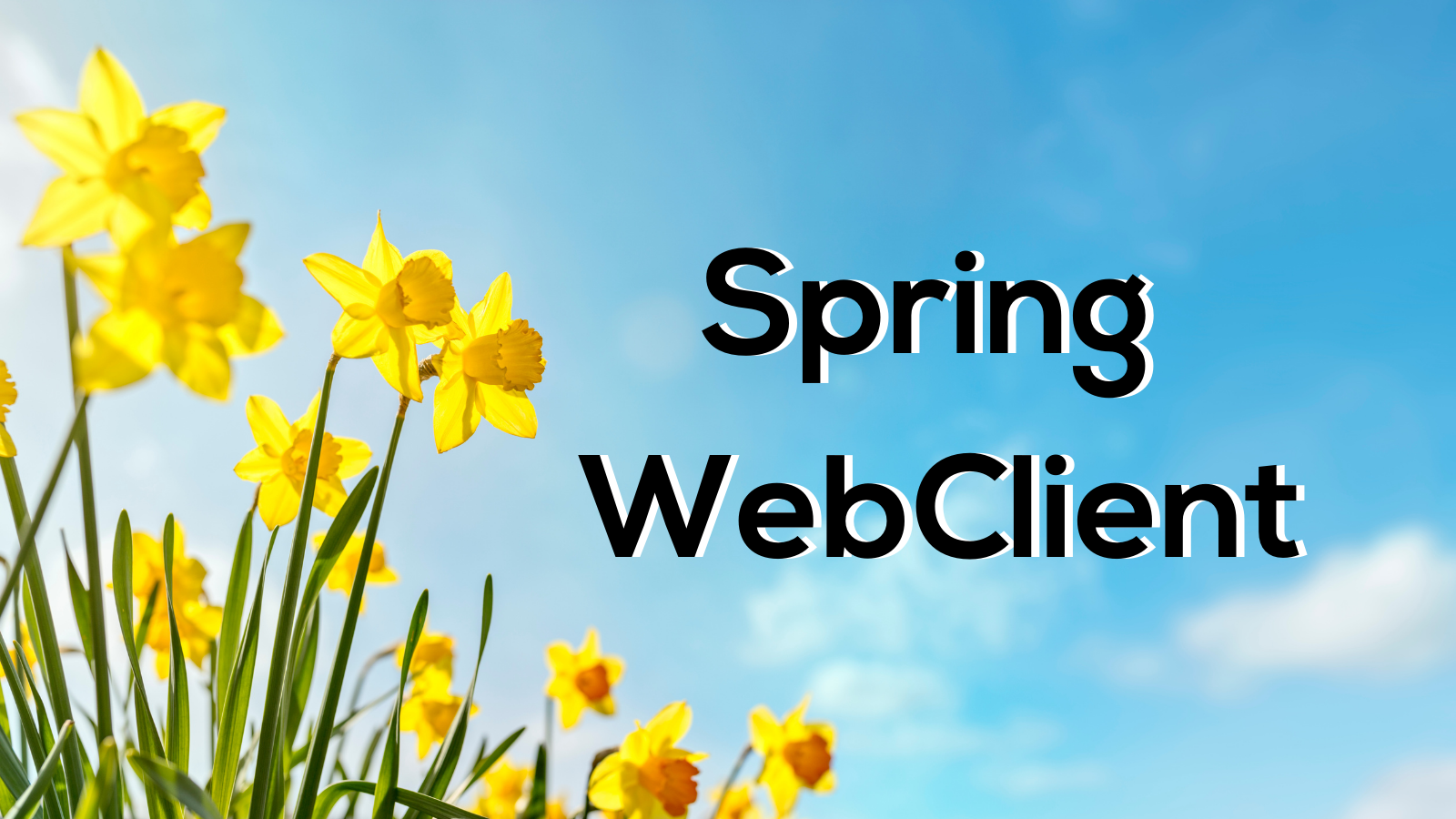
Spring ẀebClient was added as part of the reactive web stack WebFlux in Spring Framework 5.0. WebClient allows performing HTTP requests in reactive applications, providing a functional and fluent API based on Reactor, and enabling a declarative composition of asynchronous non-blocking requests without the need to deal with concurrency. One of its features is support for filter registration, allowing to intercept and modify requests, which can be used for cross-cutting concerns such as authentication, as...
Faster Spring Boot Testing with Test Slices
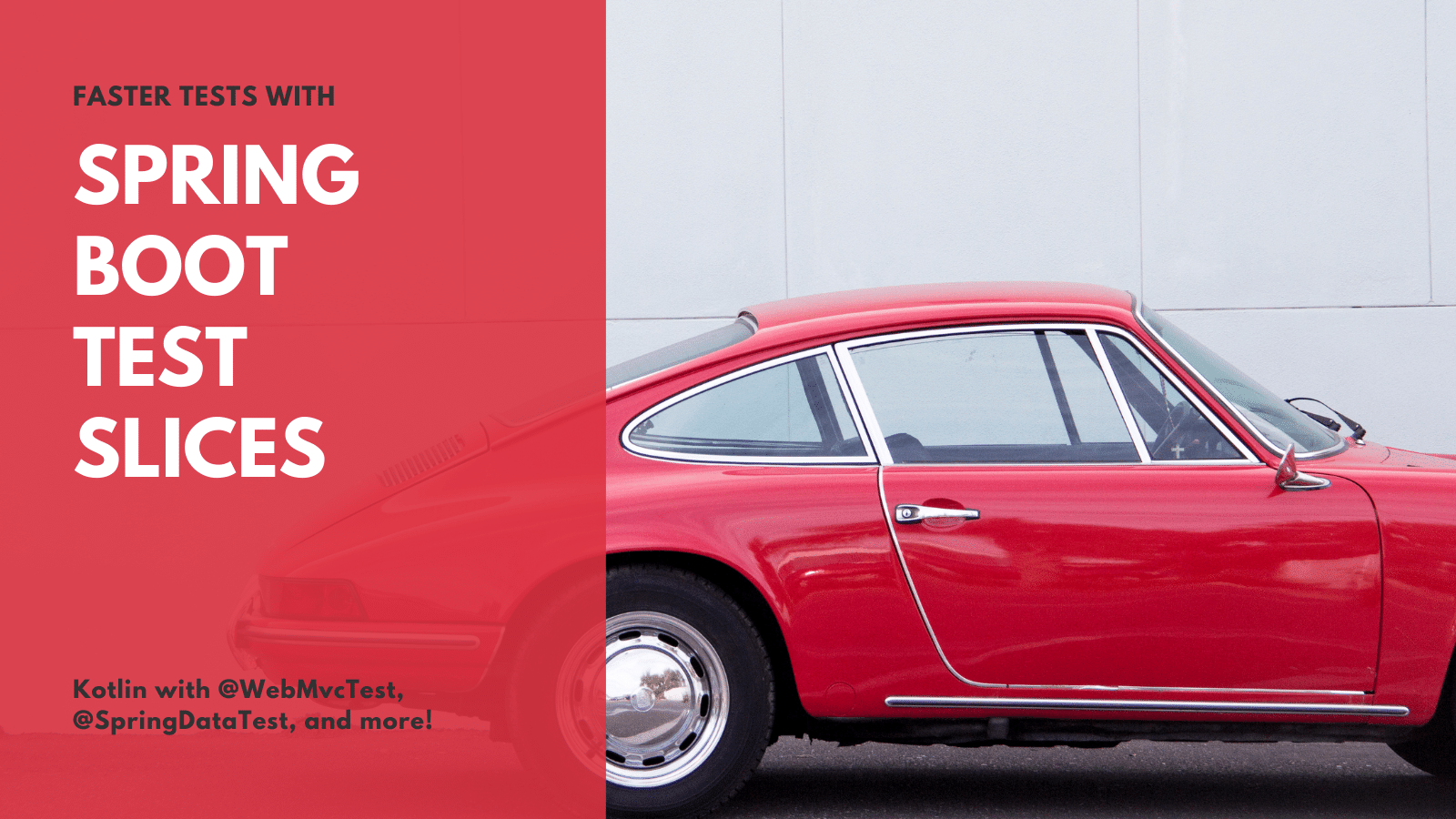
We know unit testing is a vital part of the software development process. We also know us developers love to debate techniques, frameworks, strategies, and how different layers and components need testing. Unit tests are the most valuable when they are stable, fast, and reproducible. Spring Boot is known to reduce boilerplate code and make development extremely efficient, but it can come with a cost when it comes down to the testing. Without prior optimization,...
Use Kong Gateway to Centralize Authentication
A customer once asked me: “Hey – Can Okta integrate with Kong?” Spoiler alert: You totally can integrate Kong with Okta using its OpenID Connect plugin. Still stuck wondering what an API gateway even is? Here’s a metaphor that works for me: You know that sci-fi movie trope in which you have a centralized hub that “jumps” you to other places in the galaxy? In that kind of system all the screening and security happens...
Scaling Secure Applications with Spring Session and Redis
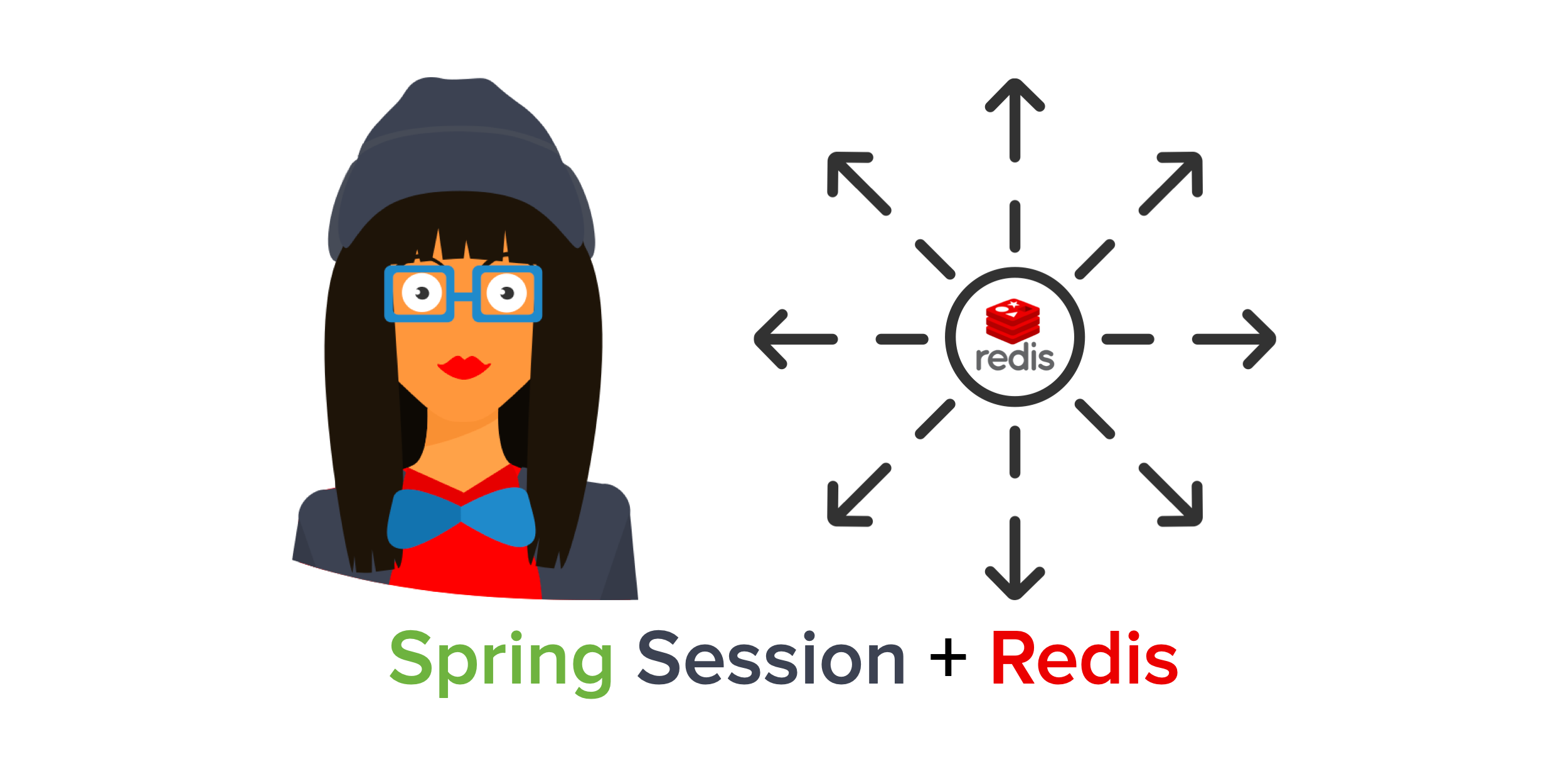
Spring Boot and Spring Security have delighted developers with their APIs for quite some time now. Spring Security has done an excellent job of implementing OAuth and OpenID Connect (OIDC) standards for the last few years. If you’re using Spring Security’s default authorization code flow with OIDC, it’ll establish a session on the server and serve up old fashion session cookies. If you want to scale your services, you’ll need to share session information. This...
Test in Production with Spring Security and Feature Flags
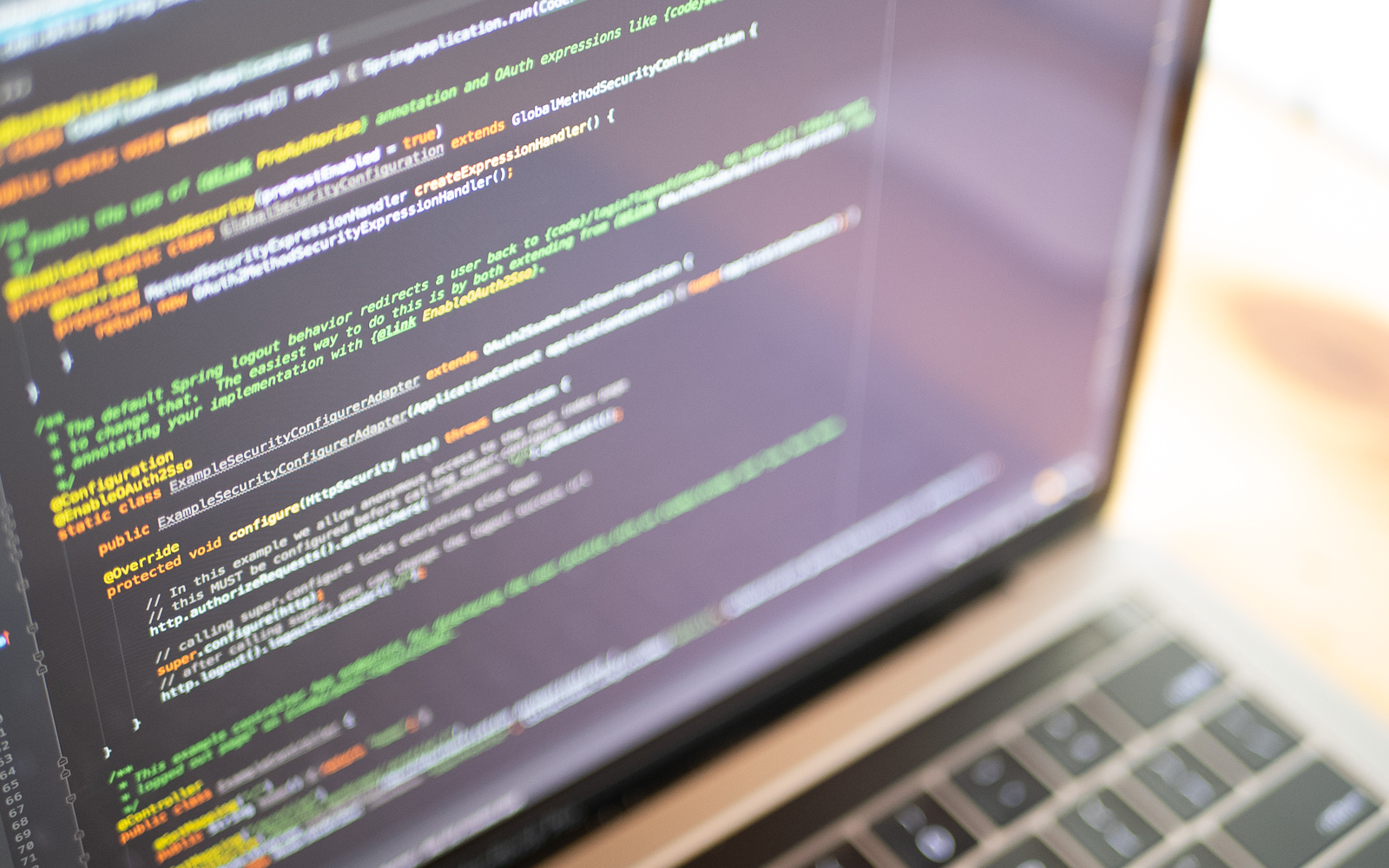
Okta is an Identity and Access Management platform. The TL;DR: you offload the responsibility for secure authentication and authorization to Okta so you can focus on the business logic of the app you’re building. Okta and Spring Boot already go together like peanut butter and chocolate. Add in feature flags care of Split, and you can test new capabilities for your app without having to redeploy. That’s testing in production the smart way! And, you...
OAuth 2.0 Patterns with Spring Cloud Gateway
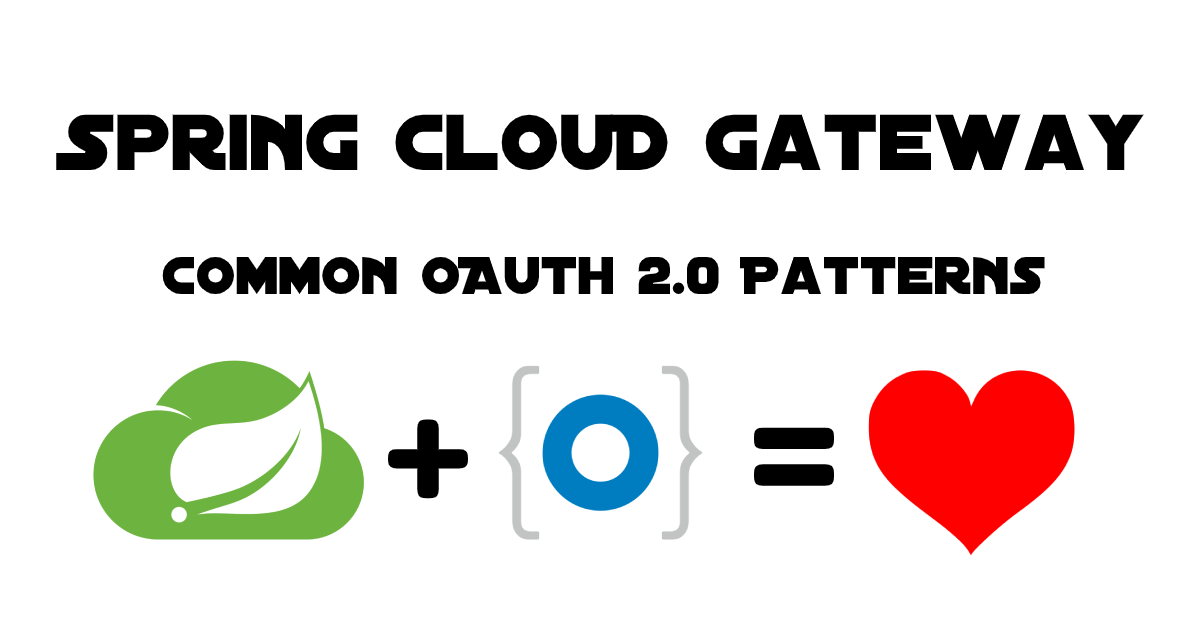
Note: In May 2025, the Okta Integrator Free Plan replaced Okta Developer Edition Accounts, and the Okta CLI was deprecated. We preserved this post for reference, but the instructions no longer work exactly as written. Replace the Okta CLI commands by manually configuring Okta following the instructions in our Developer Documentation. Spring Cloud Gateway is the Reactive API Gateway of the Spring Ecosystem, built on Spring Boot, WebFlux, and Project Reactor. Its job is to...
JWT vs Opaque Access Tokens: Use Both With Spring Boot
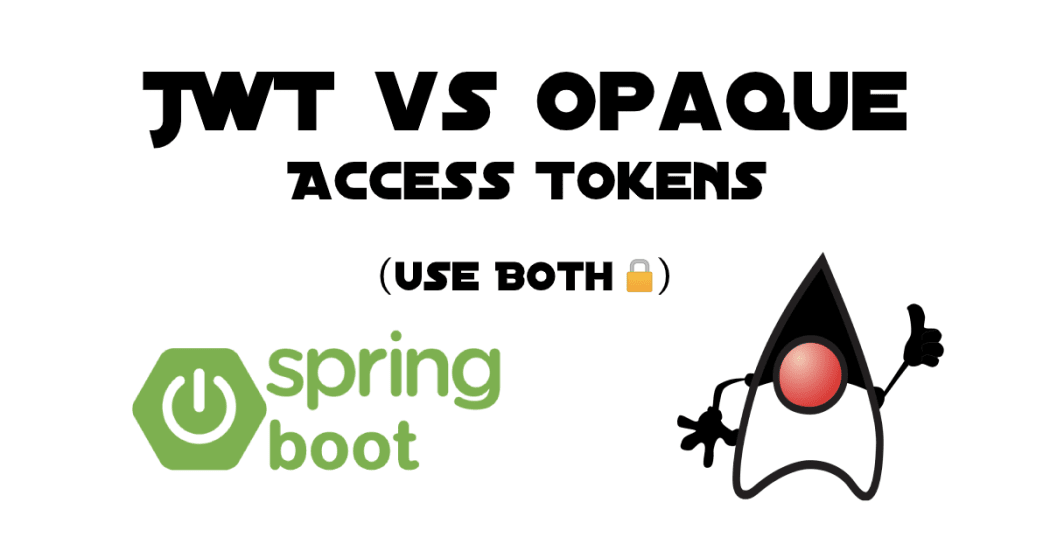
The topic of validating an OAuth 2.0 access tokens comes up frequently on this blog. Often we talk about how to validate JSON Web Token (JWT) based access tokens; however, this is NOT part of the OAuth 2.0 specification. JWTs are so commonly used that Spring Security supported them before adding support for remotely validating tokens (which is part of the OAuth 2.0 specification.) In this post, you will build a simple application that takes...
Build a CRUD App with Vue.js, Spring Boot, and Kotlin
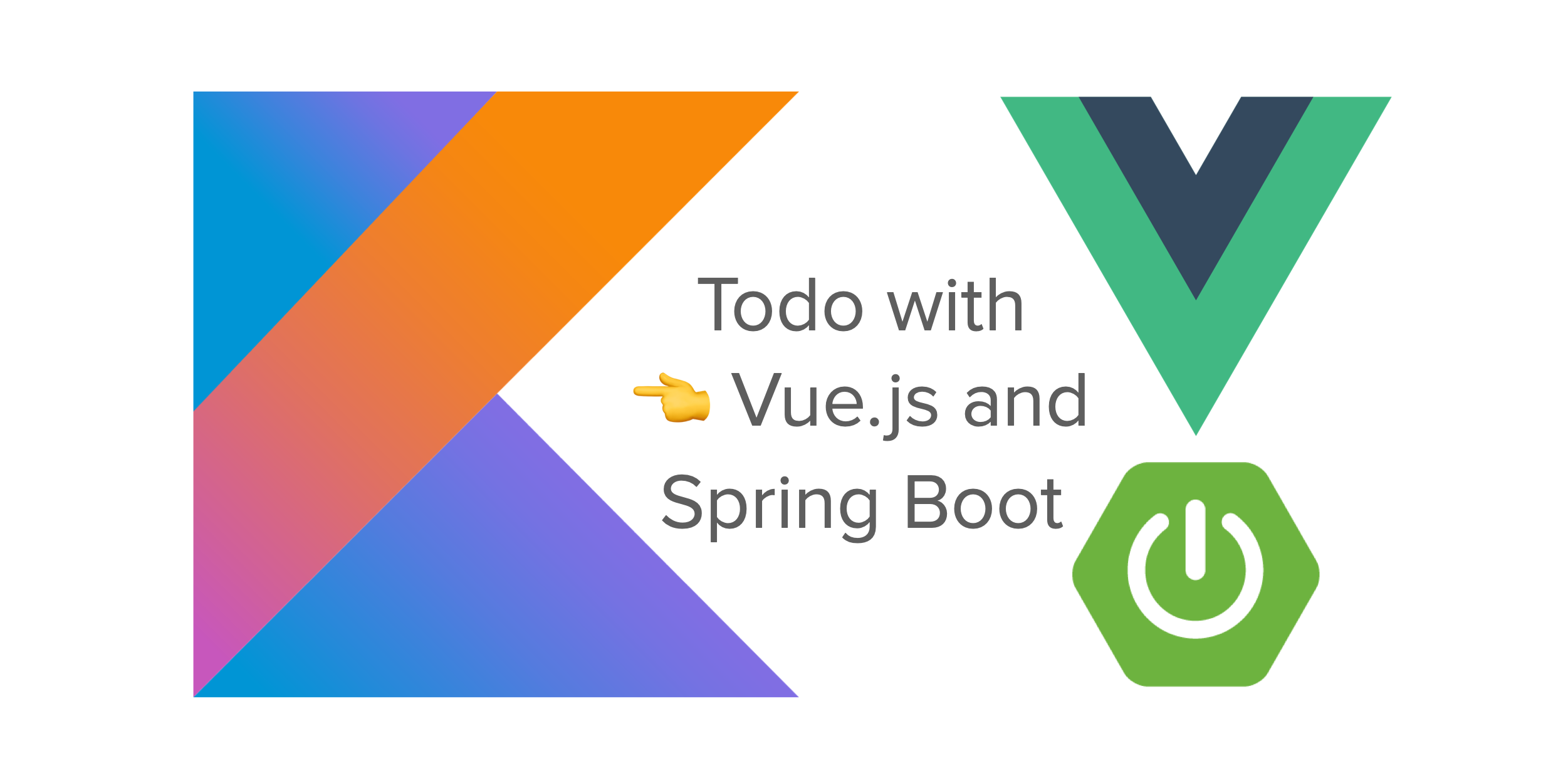
Much like React or Angular, Vue.js is a JavaScript view library. When coupled with a state management library like MobX, Vue.js becomes a full-featured application framework. Vue.js is designed to be incrementally adoptable, so you can use as much or as little of it as you like. Like React, Vue.js utilizes a virtual DOM to streamline processing so that it renders as little as possible on each state update. In my experience, Vue is far...
Secure Secrets With Spring Cloud Config and Vault
OpenID Connect Logout Options with Spring Boot
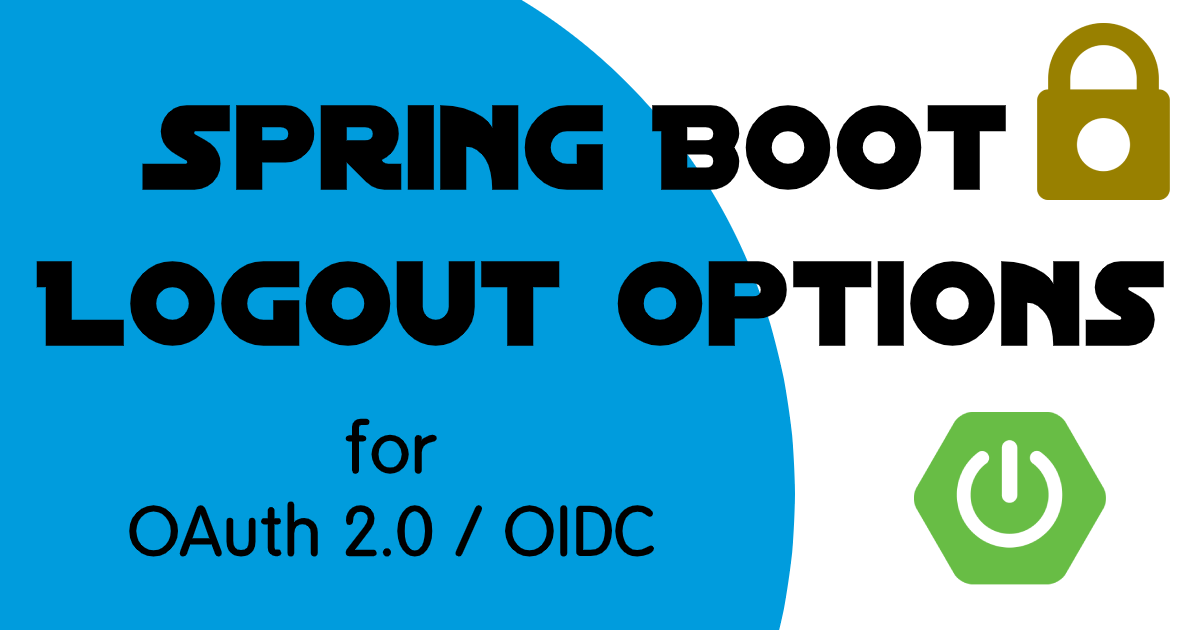
On the Okta blog, we spend much of our time talking about logging in. That is because once you configure your application to log in, the log out just works. But there are a few things you should consider when you’re thinking about your app’s logout configuration. In this post, I’ll walk through examples of the two logout options you have with Spring Security: the "default" session clearing logout, and relying party initiated logout. If...
Build Single Sign-on in Java
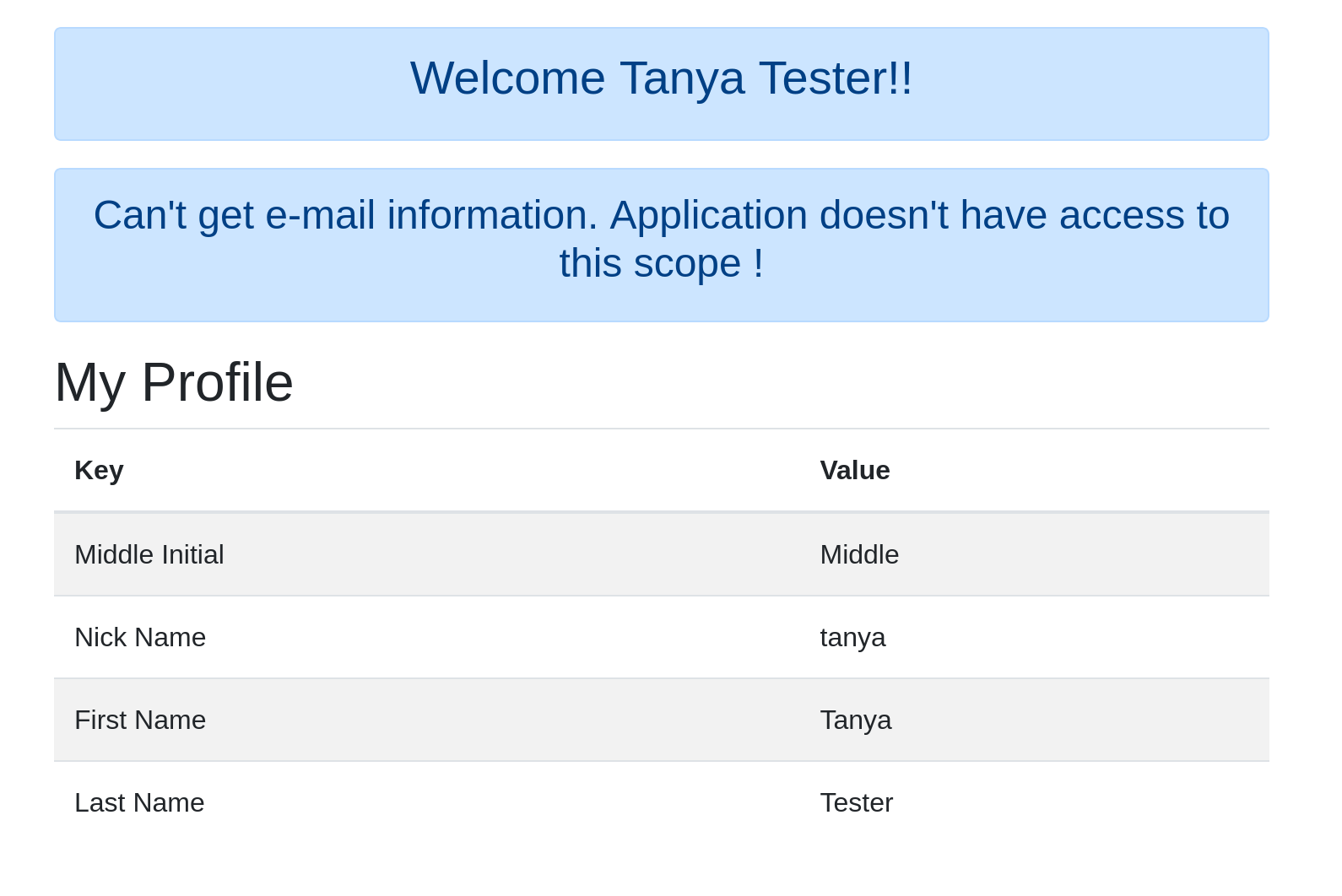
In modern app development, you quite frequently have a single resource server that provides data to multiple client applications. These applications may share a similar set of users, but need to enforce different permissions. For example, it’s possible that not all users of the first application should be allowed to access the second (think of, for example, an admin console application versus a client or user application). How would you implement this? One way to...
Deploy Your Spring Boot App the Right Way
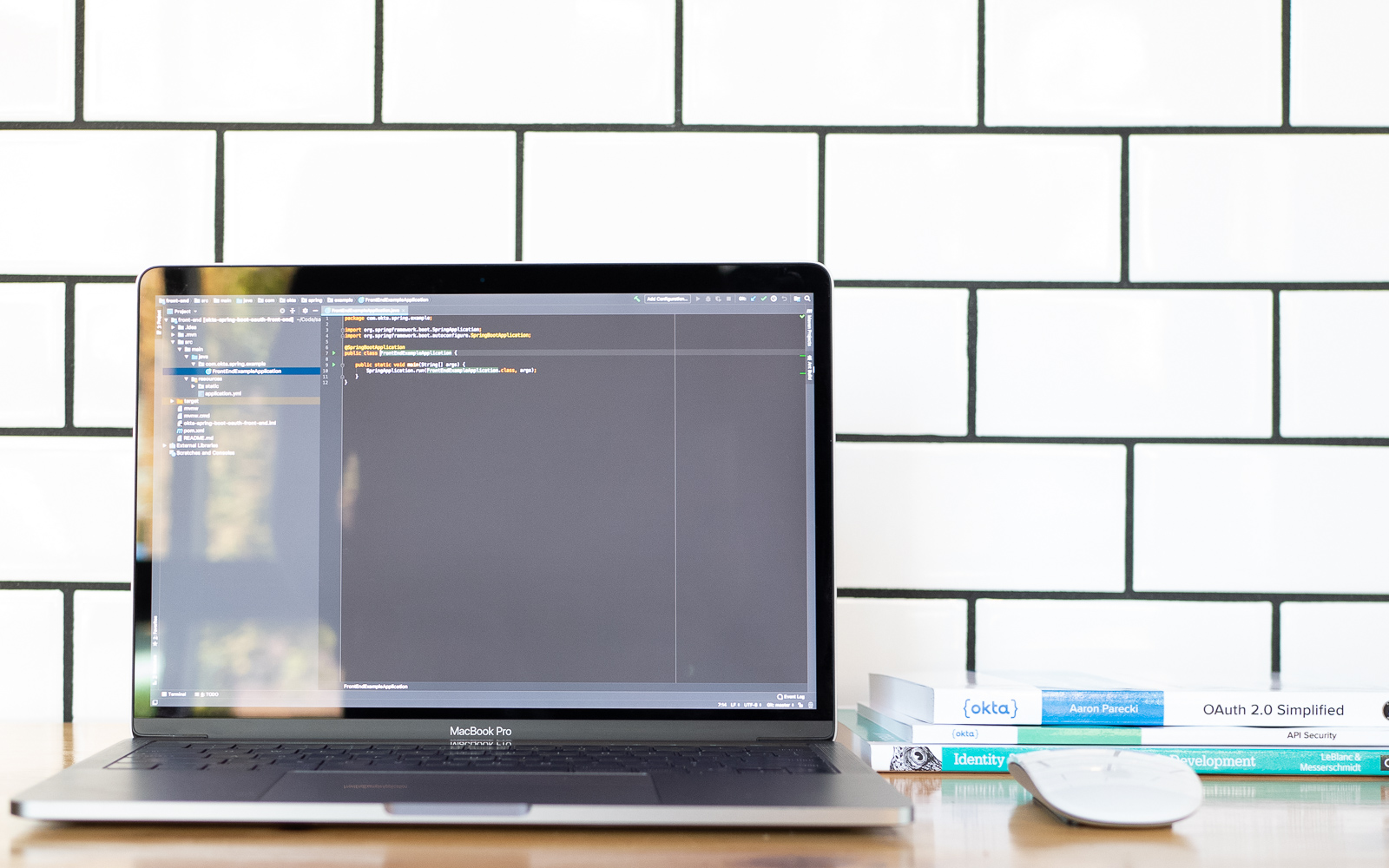
Spring Boot is an awesome solution to speed up the development cycle of your app. Have an idea and want to transform it into a Spring Boot app, but don’t know the best way to deploy it? Look no further, we will help you out! There are plenty of options to deploy Spring Boot applications. In this article. we will cover three of them: Azure Amazon Web Services Self-hosted We will use a simple application...
A Quick Guide to Java on Netty
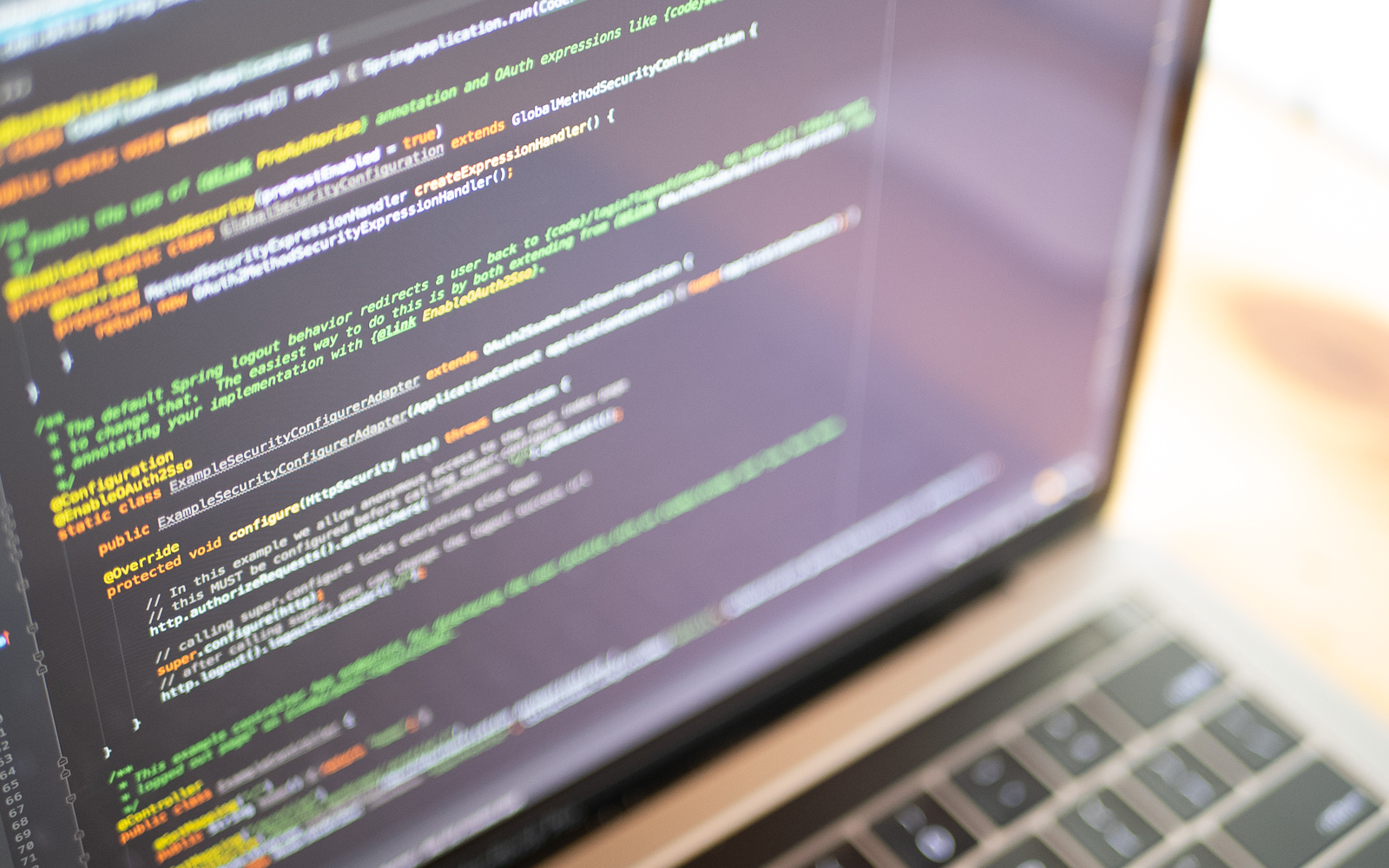
Netty is a non-blocking input/output (NIO) framework that makes it relatively simple to develop low-level network servers and clients. Netty provides an incredible amount of power for developers who need to work down on the socket level, for example when developing custom communication protocols between clients and servers. It supports SSL/TLS, has both blocking and non-blocking unified APIs, and a flexible threading model. It’s also fast and performant. Netty’s asynchronous, non-blocking I/O model is designed...
Tutorial: Develop Apps with Secure WebSockets in Java
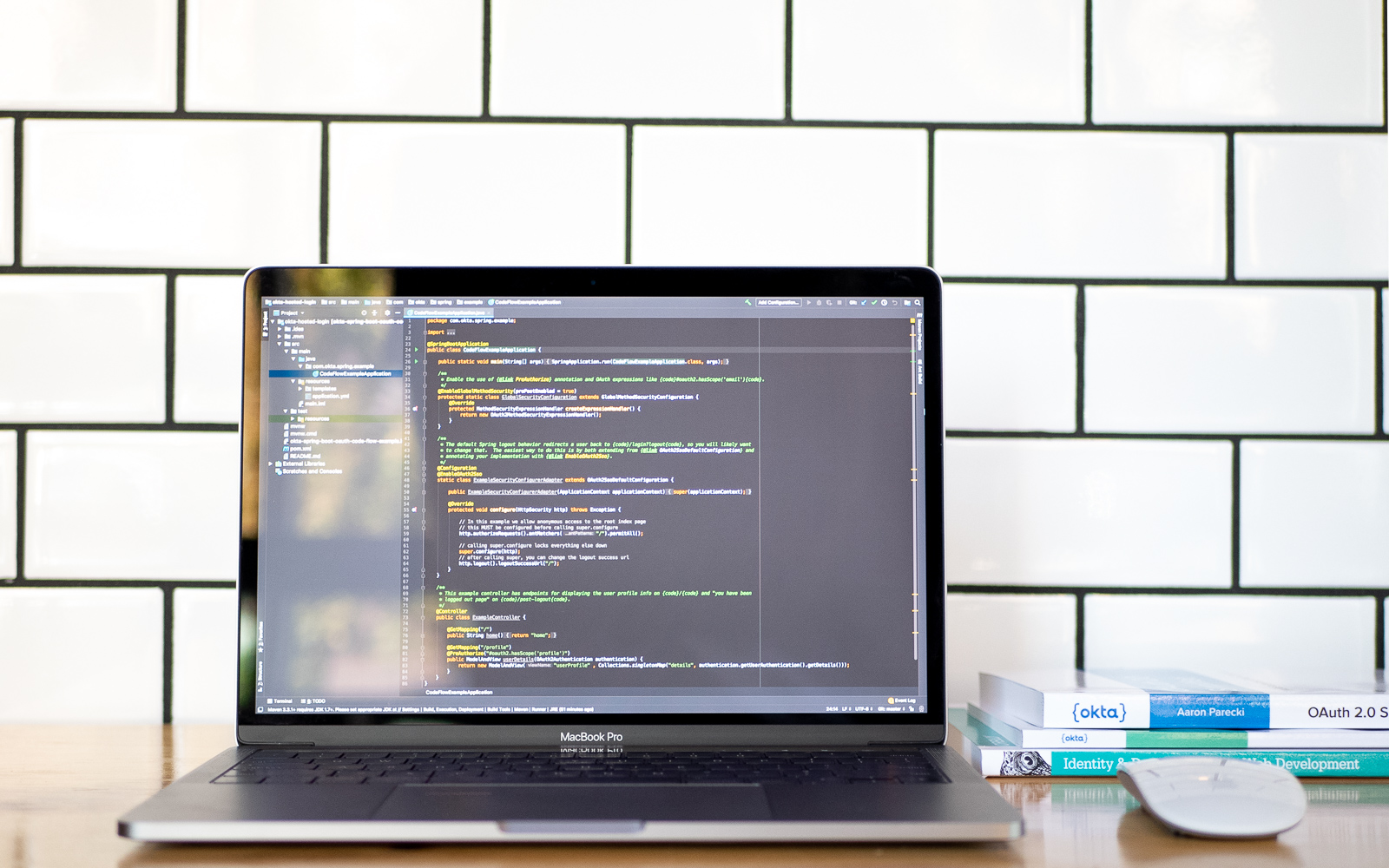
WebSockets is a modern transport layer technology that establishes a two-way communication channel between a client and a server, perfect for low-latency, high-frequency interactions. WebSockets tend to be used in collaborative, real-time or event-driven applications, where traditional client-server request-response architecture or long polling would not satisfy requirements. Use cases include stock trading and shared dashboard applications. In this tutorial, I’ll give you a quick overview of the WebSockets protocol and how it handles messages with...
Build a Simple CRUD App with Java and JSF
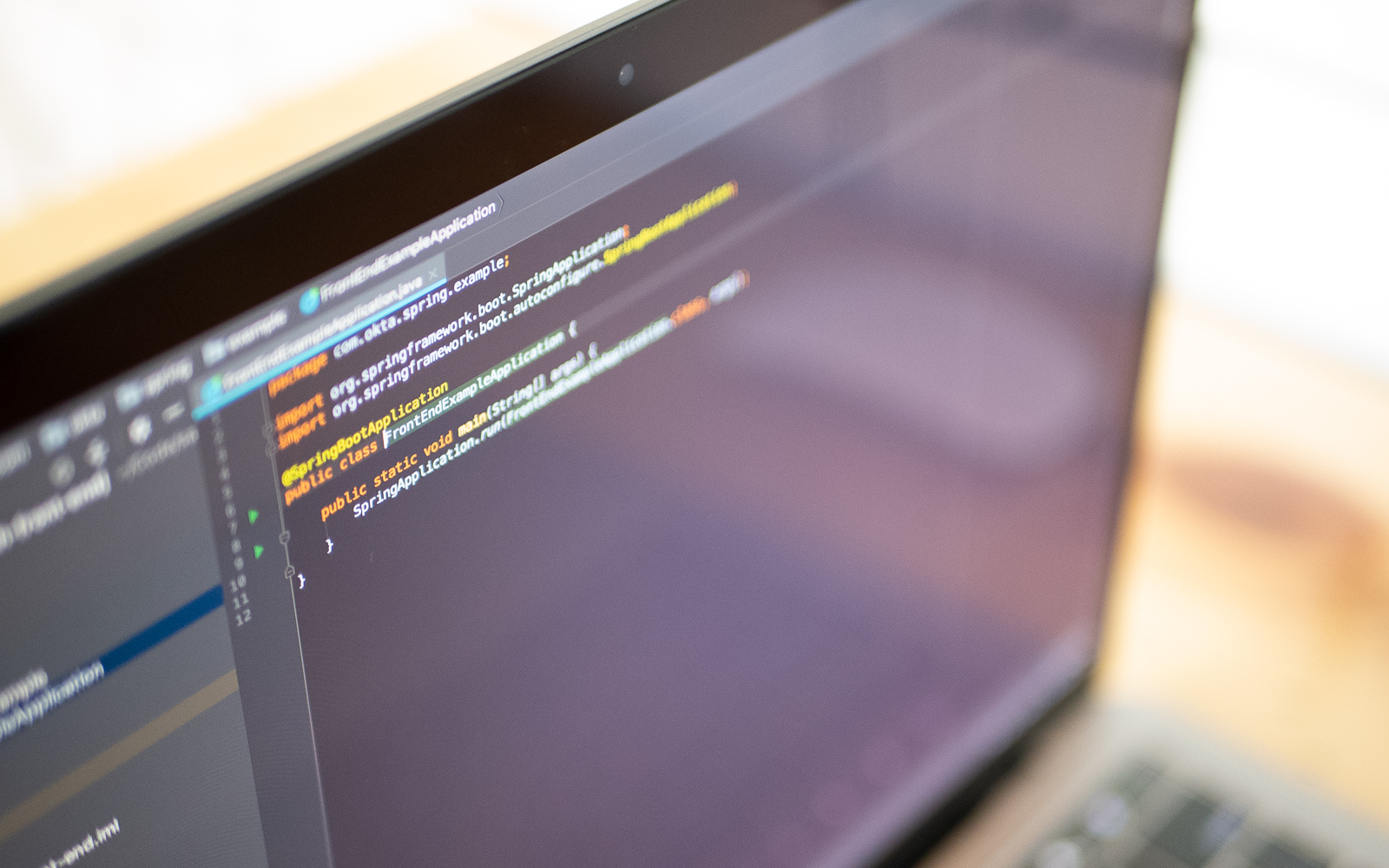
JavaServer Faces (JSF) is a Java framework for building Web applications, centered on components as the building blocks for the user interface. JSF benefits from a rich ecosystem of tools and vendors, as well as out of the box components and libraries that add even more power. Why use JSF instead of JavaServer Pages (JSP)? There are two primary reasons: First, JSF has more templating capabilities, since it doesn’t write your view directly as it...
Build an Application with Spring Boot and Kotlin
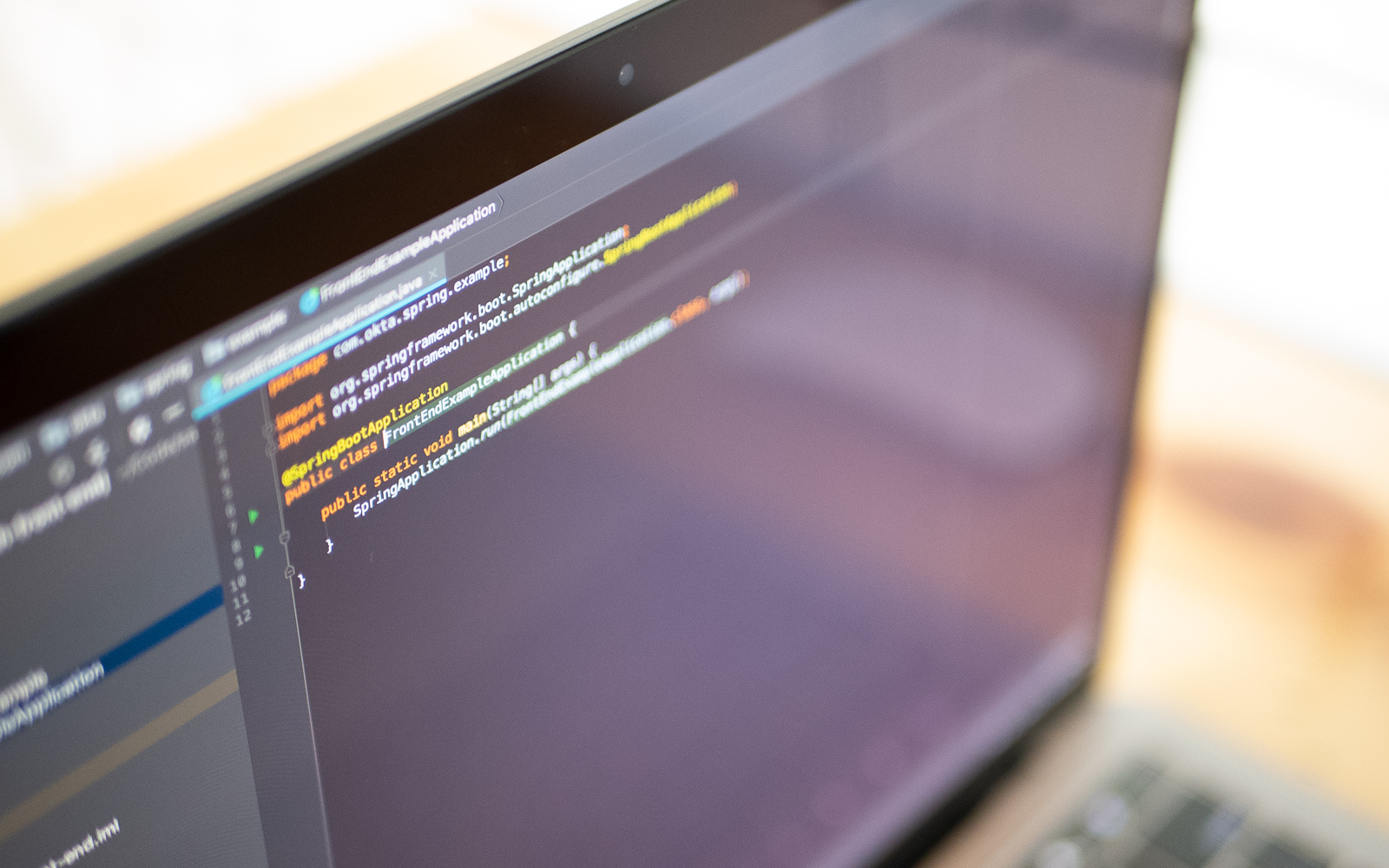
In 2011, JetBrains, the company behind IntelliJ, decided to create a modern language that would run inside the Java Virtual Machine and address common concerns with Java at the time like its verbosity. This project became Kotlin, a quickly growing and popular language. Google then announced official support for Kotlin on Android, further accelerating its adoption. Many companies started to replace Java with Kotlin as their main language to take advantage of the new features...
Make Java Tests Groovy With Hamcrest
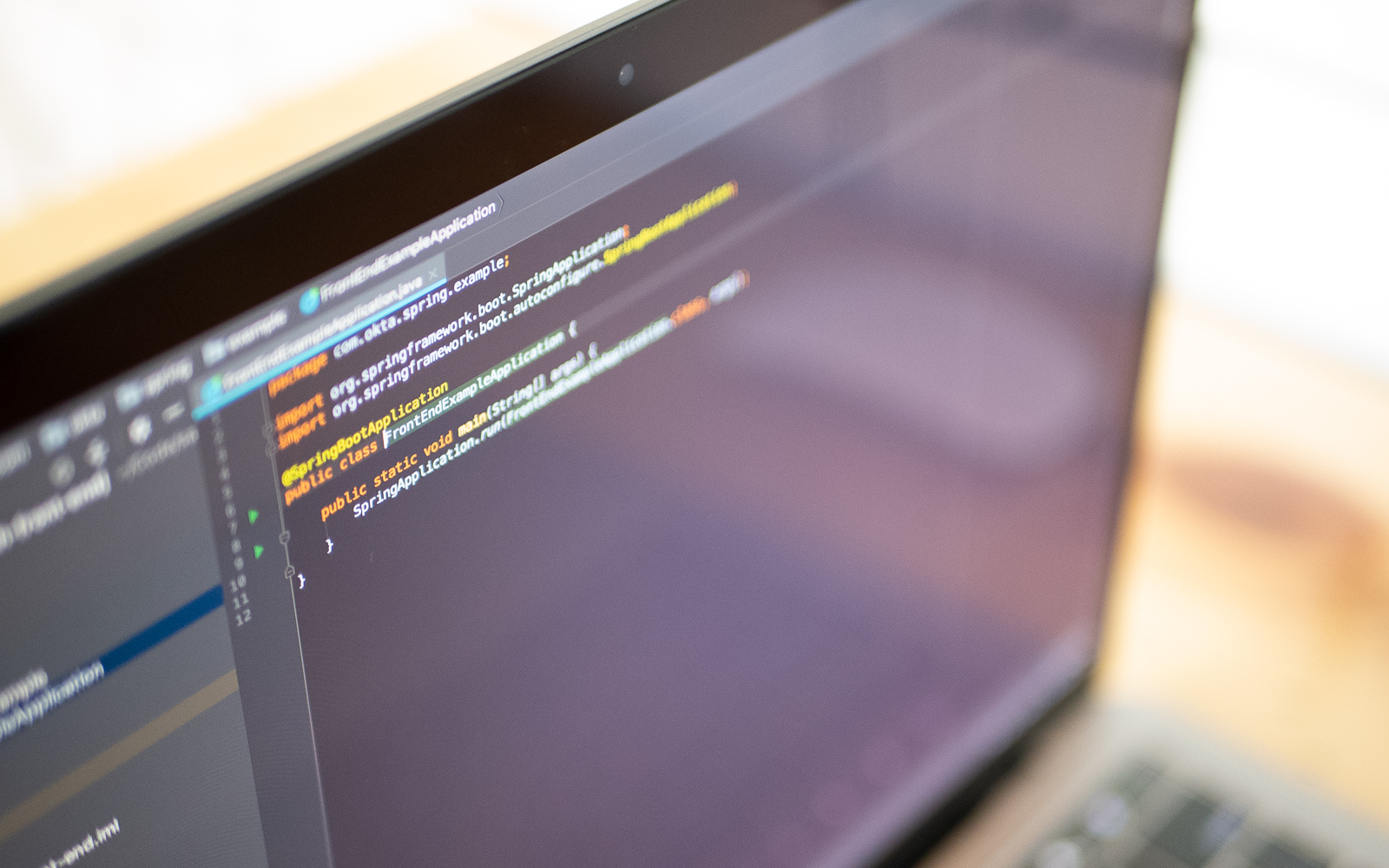
My favorite way to test Java code is with Groovy. Specifically, writing tests in Groovy with Hamcrest. In this post, I’ll walk through how to test a simple Spring Boot application with these tools. Groovy is an optionally typed dynamic language for the JVM, and can be compiled statically. That is a mouthful and I’ll explain this as we go, but for now think of Groovy as Java with lots of sugar. Groovy is a...
Use Spring Boot and MySQL to go Beyond Authentication
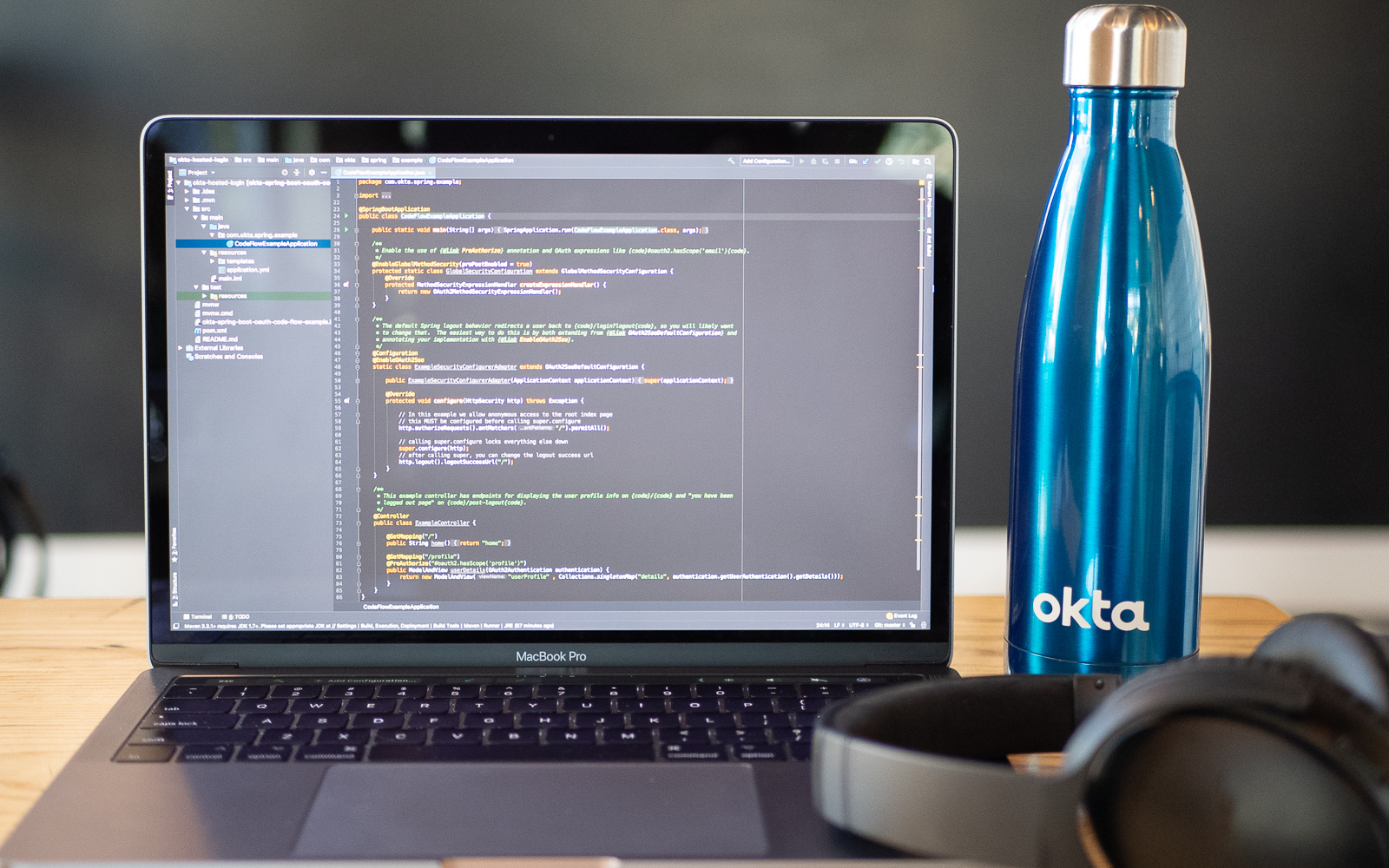
In this post, we will walk through how to build a simple CRUD application using Spring Boot, MySQL, JPA/Hibernate and Okta OpenID Connect (OIDC) Single Sign-On (SSO). The Java Persistence API (JPA) provides a specification for persisting, reading, and managing data from your Java object to relational tables in the database. The default implementation of JPA via Spring Boot is Hibernate. Hibernate saves you a lot of time writing code to persist data to a...
Spring Method Security with PreAuthorize
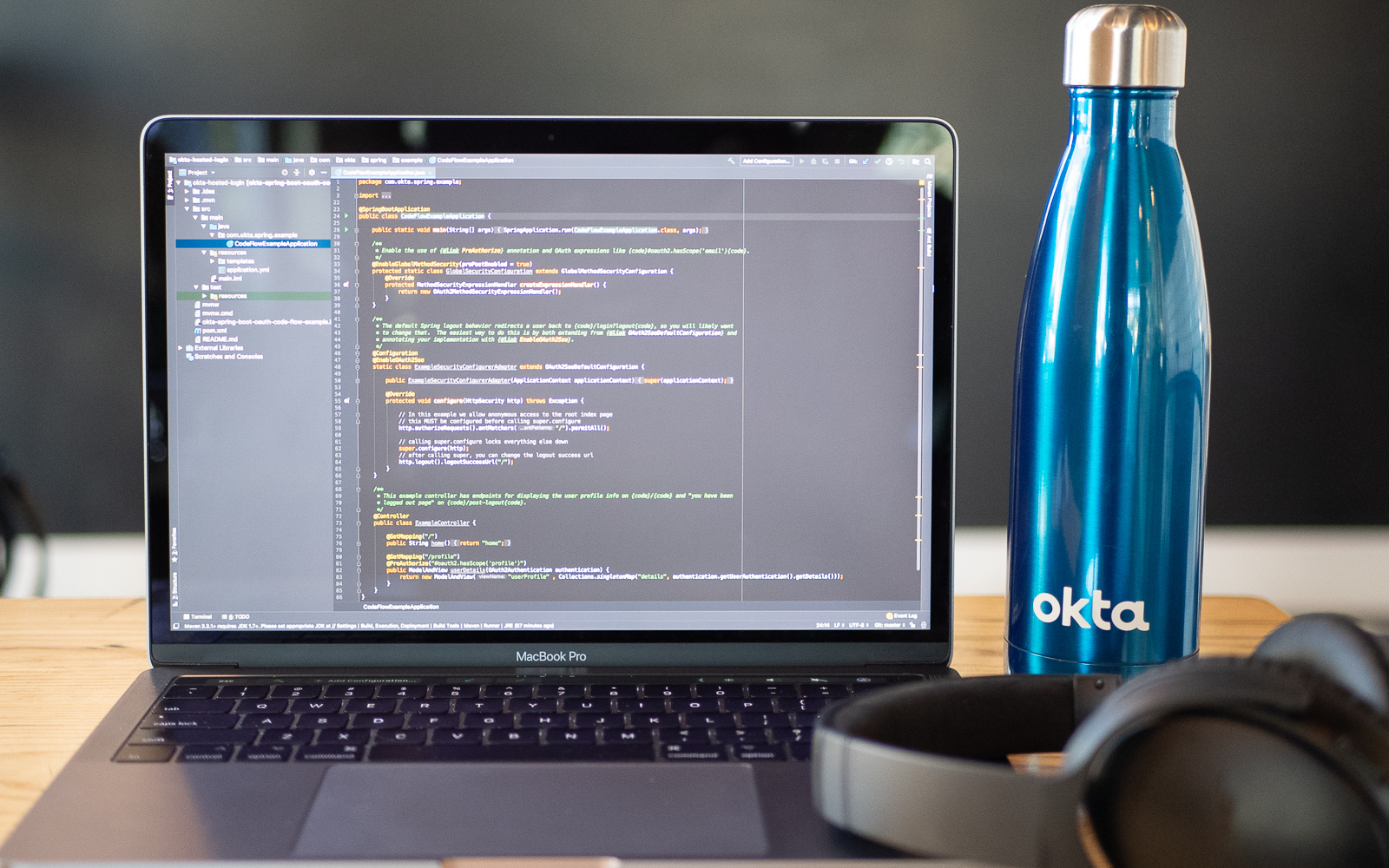
This tutorial will explore two ways to configure authentication and authorization in Spring Boot using Spring Security. One method is to create a WebSecurityConfigurerAdapter and use the fluent API to override the default settings on the HttpSecurity object. Another is to use the @PreAuthorize annotation on controller methods, known as method-level security or expression-based security. The latter will be the main focus of this tutorial. However, I will present some HttpSecurity code and ideas by...
Simple Authentication with Spring Security
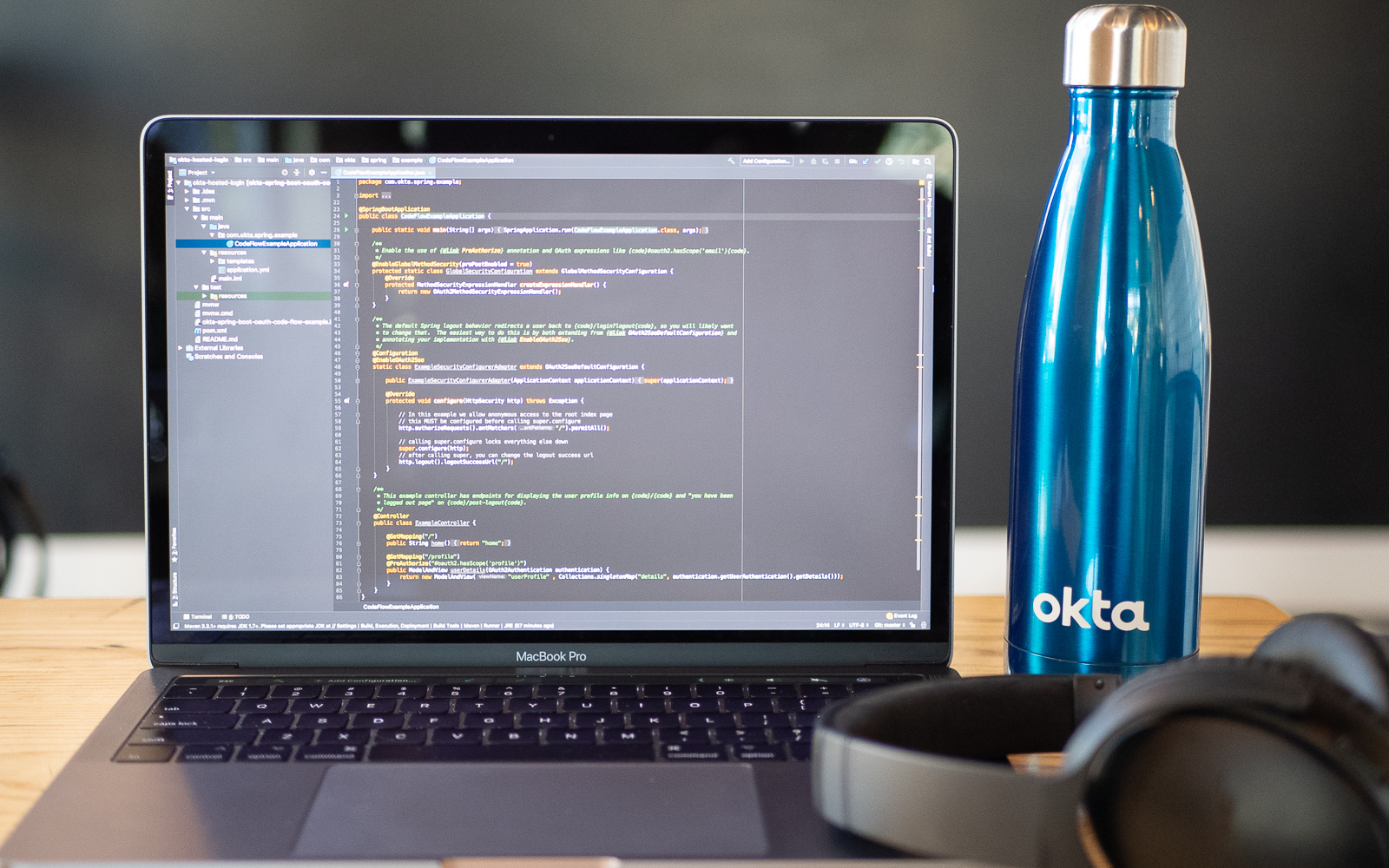
Authentication is vital to all but the most basic web applications. Who is making the request, wanting data, or wanting to update or delete data? Can you be sure that the request is coming from the stated user or agent? Answering this question with certainty is hard in today’s computer security environment. Fortunately, there is absolutely no reason to reinvent the wheel. Spring Boot with Spring Security is a powerful combination for web application development....
Java + Spring Tutorials
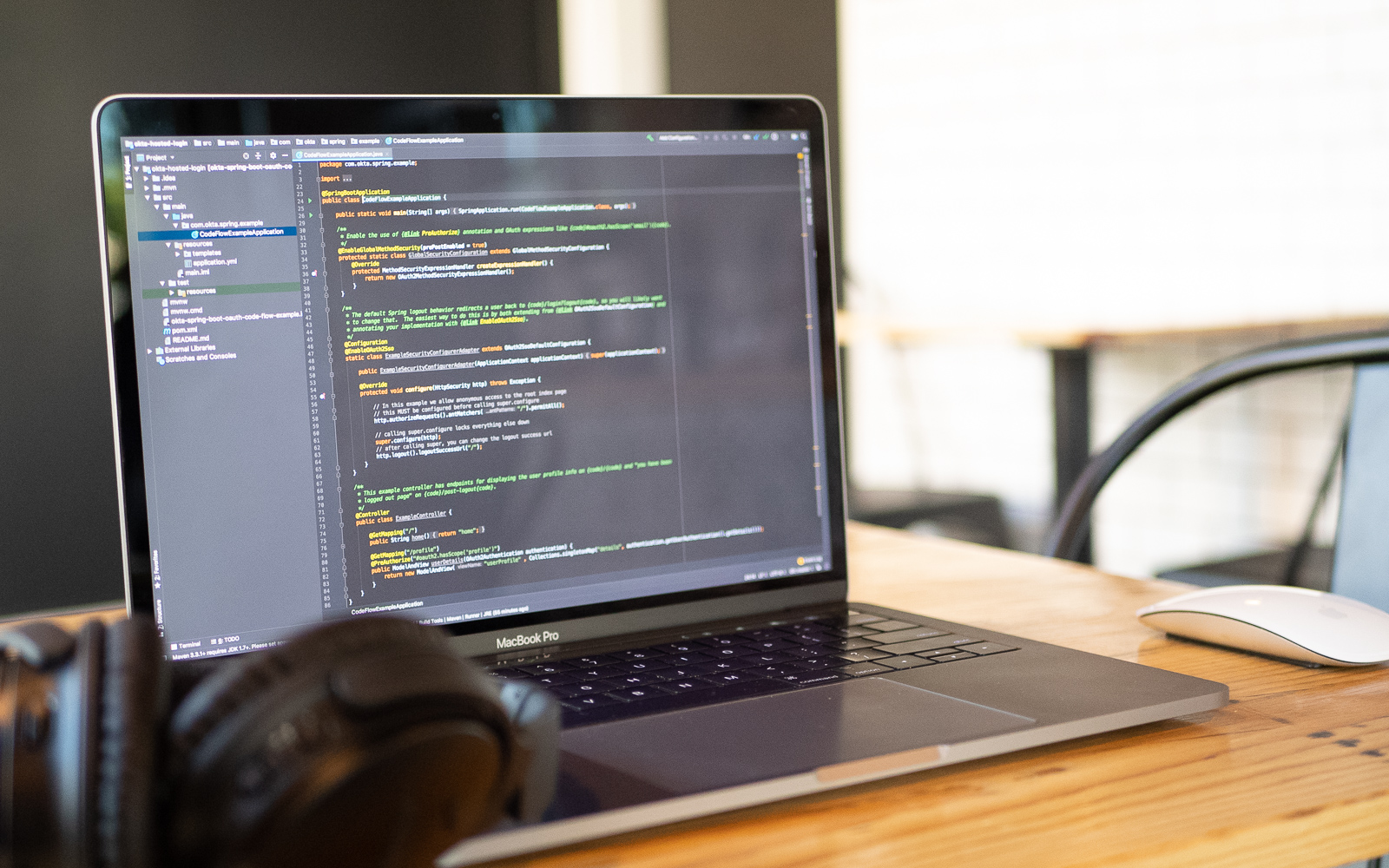
Spring Boot, and the Spring framework in general, are the core tools for the modern Java developer. They abstract away many nuances of development and architecture, allowing you to focus on building your business logic, aka, the parts of your app that will move the needle for your organization. Here at Okta we believe in that abstraction, and that a big part of modern development is using the right tools to make the job not...
Migrate Your Spring Boot App to the Latest and Greatest Spring Security and OAuth 2.0
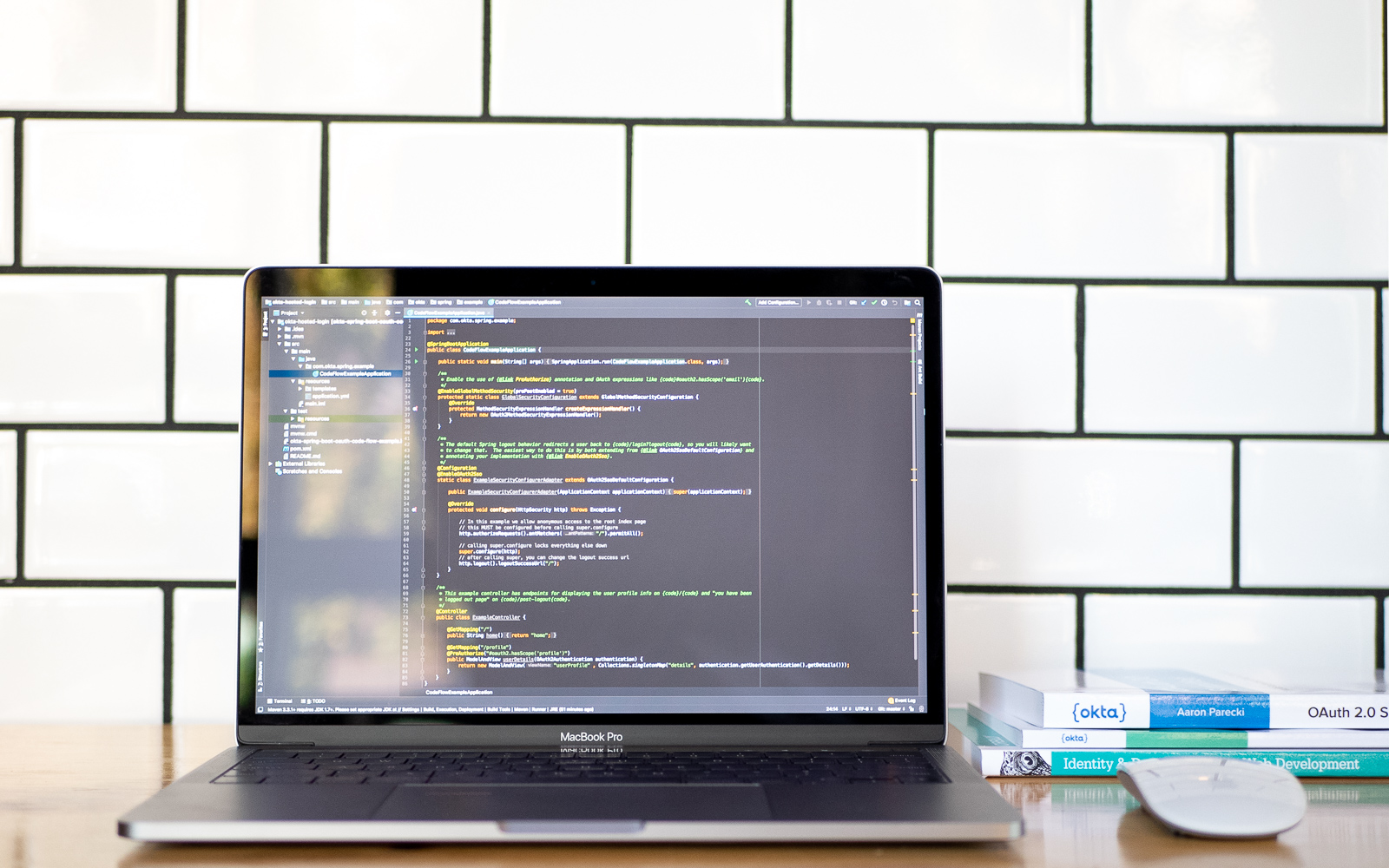
Spring Boot 1.5.x made it easier than ever before to integrate Spring Security with OAuth 2.0 into your application. Spring Boot 2.1.x dials it up to 11 by making OpenID Connect a first class citizen in the stack. In this post, you start with Spring Boot 1.5.19 and Spring Security 4.2.x. You integrate it with Okta’s OAuth service. From there, you move onto Spring Boot 2.1.3 and Spring Security 5.1. You’ll see how integrating with...
Add Social Login to Your JHipster App
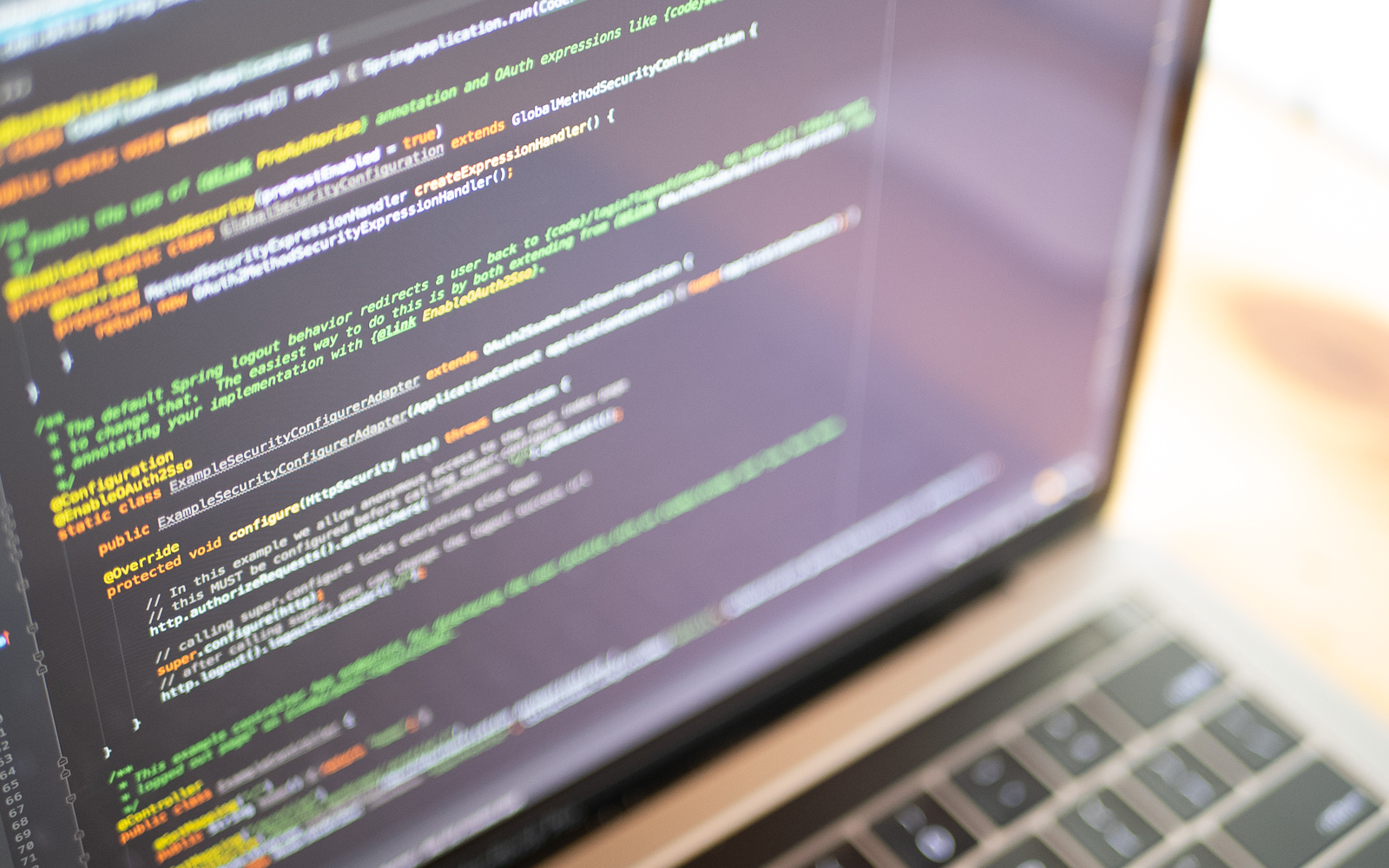
Social login is a great way to offer your customers a simple and secure authentication method. Why force them to create and forget yet another password? The vast majority of your users will have an account with Facebook or Google, so why no go ahead and let them use one of these accounts to log in? In this tutorial, you are going to integrate two social login providers: Google and Facebook. You are also going...
Data Persistence with Hibernate and Spring
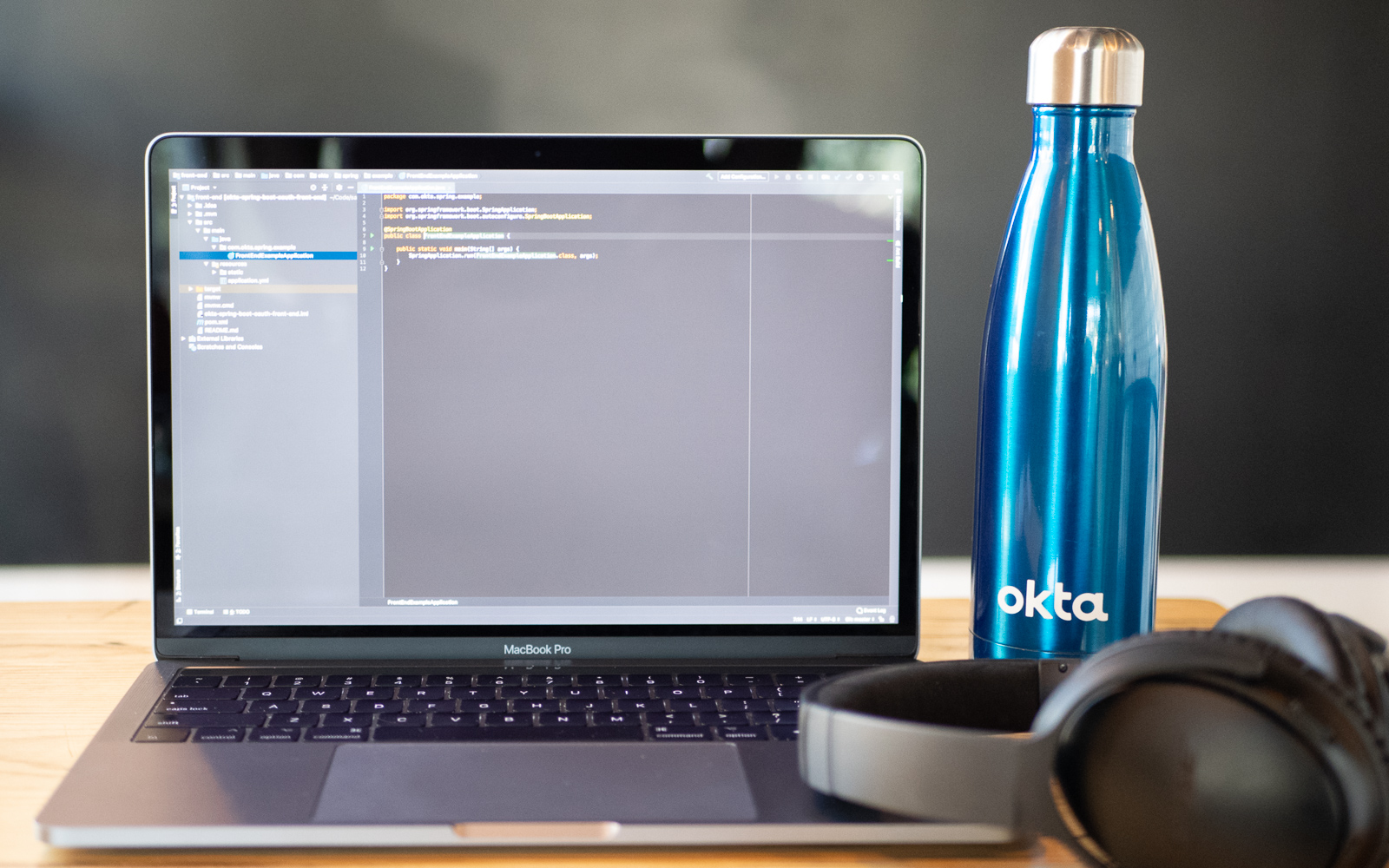
Note: In May 2025, the Okta Integrator Free Plan replaced Okta Developer Edition Accounts, and the Okta CLI was deprecated. We preserved this post for reference, but the instructions no longer work exactly as written. Replace the Okta CLI commands by manually configuring Okta following the instructions in our Developer Documentation. Java developers typically encounter the need to store data on a regular basis. If you’ve been developing for more than 15 years, you probably...
Build a Basic App with Spring Boot and JPA using PostgreSQL
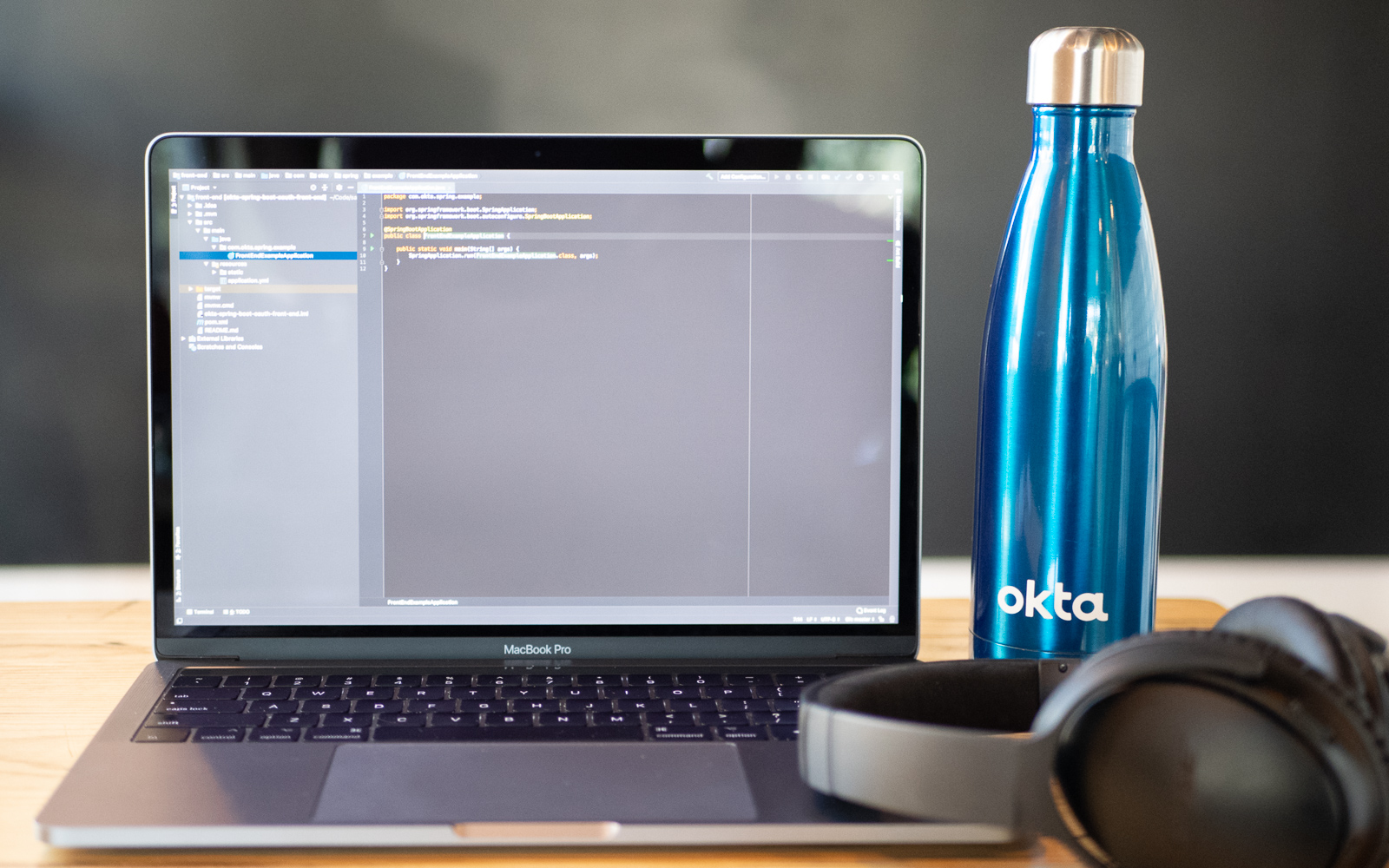
Every non-trivial application needs a way to save and update data: a resource server that is accessible via HTTP. Generally, this data must be secured. Java is a great language with decades of history in professional, enterprise development, and is a great choice for any application’s server stack. Within the Java ecosystem, Spring makes building secure resource servers for your data simple. When coupled with Okta, you get professionally maintained OAuth and JWT technologies easily...
Build a Simple CRUD App with Spring Boot and Vue.js
Add User Authentication to Your Spring Boot App in 15 Minutes
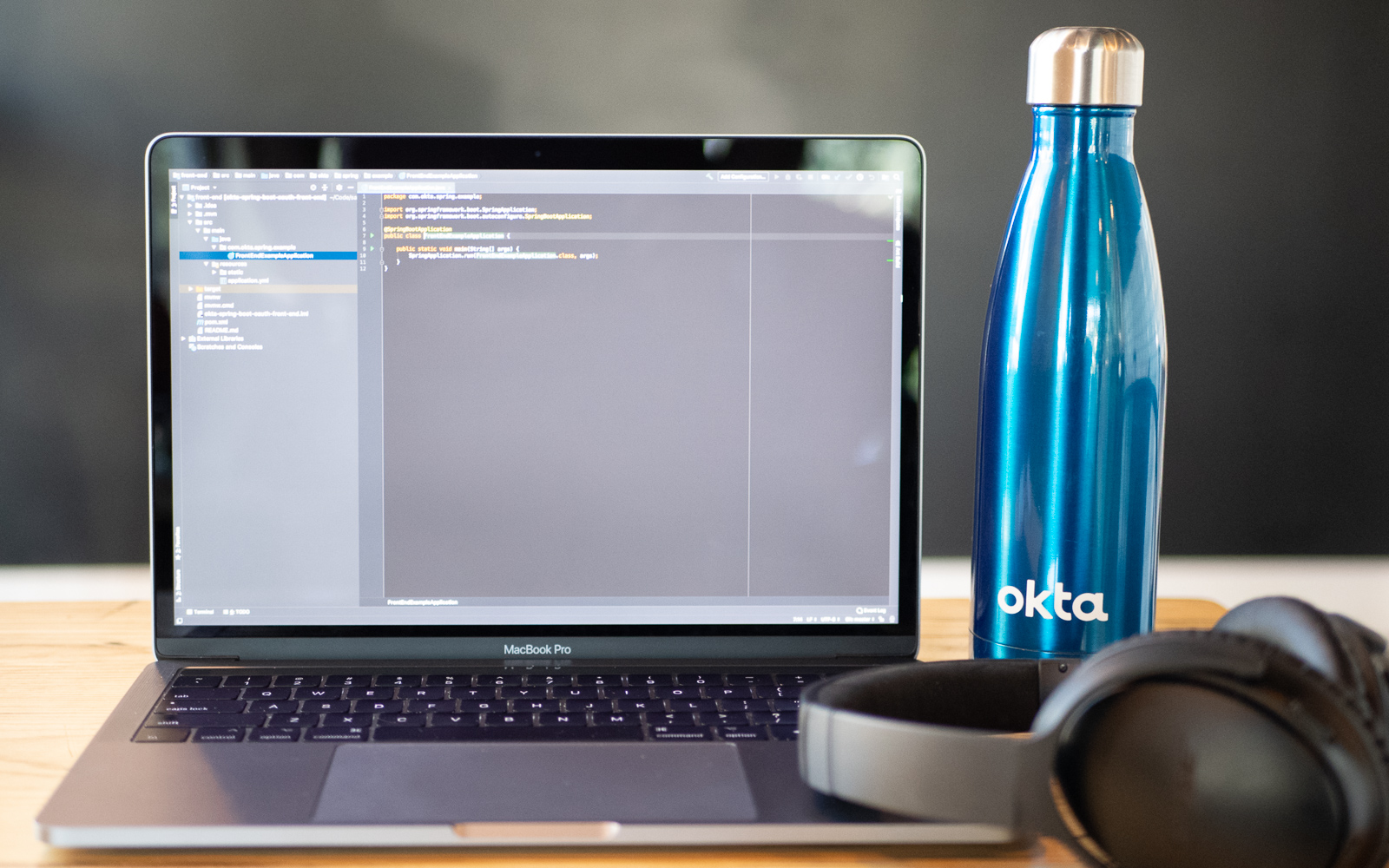
When’s the last time you had fun building a web application? We love Spring Boot because it makes it super easy to build a rich Java web application, and it can even be pretty fun. By combining Spring, Spring Boot, and Gradle, we have a complete build system that can develop, test, run, and deploy Spring applications in minutes. But what about user authentication and authorization, you’re probably thinking. Maybe with a sneer. Surely that’s...
Secure Your Spring Boot Application with Multi-Factor Authentication
OAuth 2.0 has quickly become an industry standard for third party authentication for web applications. It’s a super secure strategy, when implemented properly, but getting it right can be hard. Fortunately, you don’t have to go it alone. Okta has done it for you. This is one place where it’s definitely not worth re-inventing the wheel. And further, with all of the cybersecurity attacks today, just using a password, even a hard password, is not...
Build a Basic CRUD Application with Grails and Okta
Grails and Groovy can be a great alternative to Spring Boot, in some specific use cases. In this post, we’ll start with that basic Grails app that already has secure authentication via Okta, and add some additional features. In this tutorial you will: Define a simple data model Create domain classes for the models Create the necessary controllers and views Configure logging Configure the database so that the data is persisted between sessions Add some...
Build Server Side Authentication in Grails with OAuth 2.0 and Okta
What is Grails, what is Groovy, and why would we choose them over Spring Boot? In this post I’ll walk you through implementing server-side authentication in Grails using OAuth 2.0 and Okta. Before we dive in, however, I want to talk a little bit about why you’d be using Grails + Groovy in the first place, and how it can make your life easier in specific situations. Grails is an open source “convention over configuration”...
Secure Server-to-Server Communication with Spring Boot and OAuth 2.0
Most OAuth 2.0 guides are focused around the context of a user, i.e., login to an application using Google, GitHub, Okta, etc., then do something on behalf of that user. While useful, these guides ignore server-to-server communication where there is no user and you only have one service connecting to another one. The OAuth 2 client credentials grant type is exclusively used for scenarios in which no user exists (CRON jobs, scheduled tasks, other data...
Add Single Sign-on to Your Dropwizard Server in 15 Minutes
Dropwizard is recognized as the pioneer in turn-key Java API frameworks, and rivals Spring Boot for ease of adoption. Whether you’re interested in trying it out for the first time, or already have a mature platform built on top of Dropwizard, you can add secure authentication to your site in a matter of minutes. By combining Dropwizard’s production-ready essential libraries and Okta’s identity platform, you can construct a fully secured internet-facing web service with little...
Open Source Framework Samples and Quickstarts for Okta's Developer APIs
Developers love sample applications. It’s one thing to see the steps to create an application or feature; but when someone provides a working app you can just build and run it’s simply fantastic. Open source is near and dear to many developers today. Many of the frameworks we use to build applications are open source. It’s a great way to develop widely-used software and get contributions from your users. Okta’s Developer Experience (DevEx) team believes...
Secure a Spring Microservices Architecture with Spring Security and OAuth 2.0
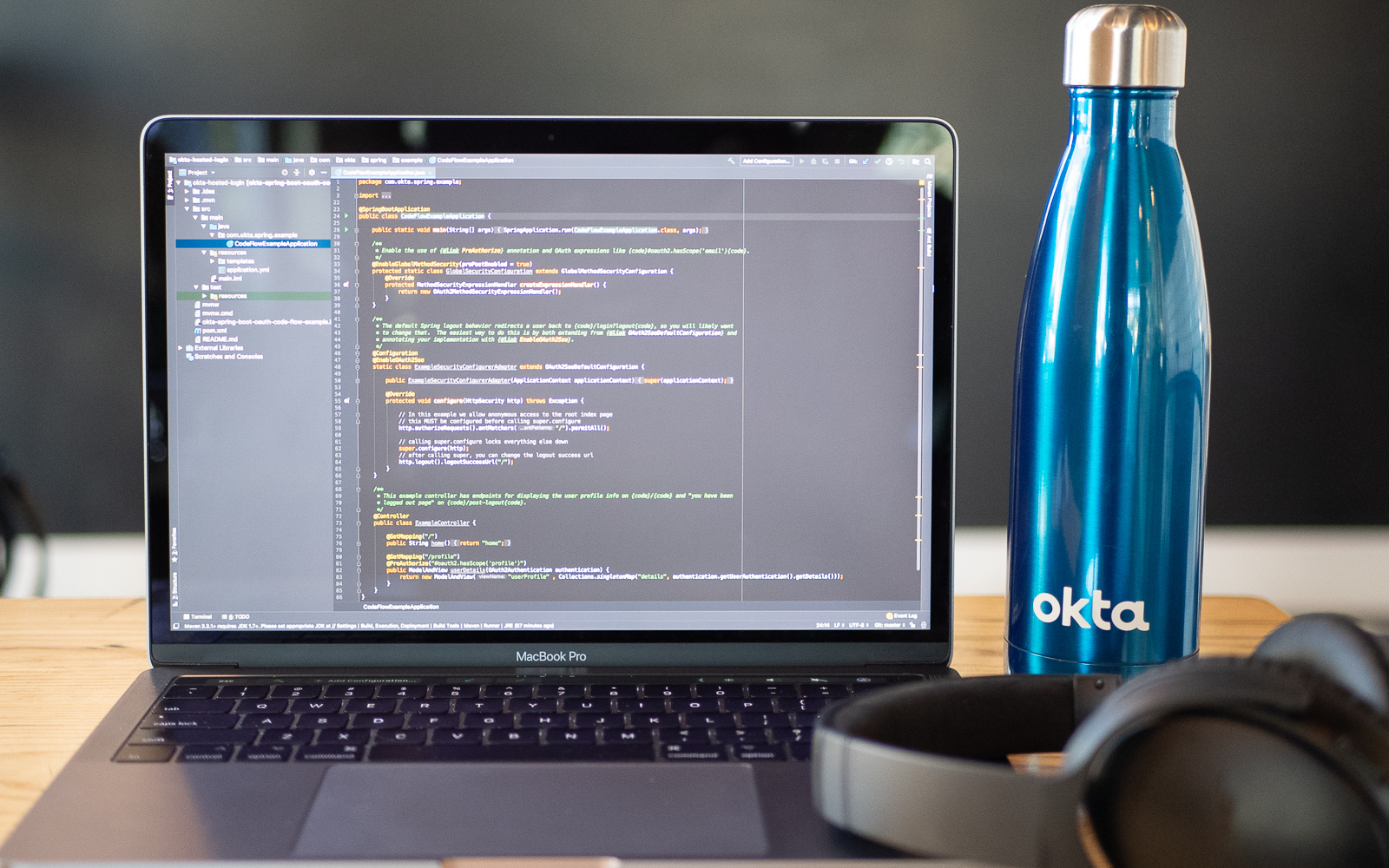
Building a microservices architecture with Spring Boot and Spring Cloud can allow your team to scale and develop software faster. It can add resilience and elasticity to your architecture that will enable it to fail gracefully and scale infinitely. All this is great, but you need continuous deployment and excellent security to ensure your system stays up-to-date, healthy, and safe for years to come. With Spring Security and its OAuth 2.0 support, you have everything...
Use Kong Gateway to Centralize Authentication
NOTE: The video and code in this post has just been updated for 2021! A customer once asked me: “Hey – Can Okta integrate with Kong?” My first thought was: “What’s Kong?” A Google result later, I was introduced to the Kong API Gateway – an open-source API Gateway and Microservices management layer. Spoiler alert: You totally can integrate Kong with Okta using its OpenID Connect plugin. Still stuck wondering what an API gateway even...
Add Single Sign-On to Your Spring Boot Web App in 15 Minutes
Need a secure web server right now? With Spring Boot and Okta, you can spin up an enterprise-quality REST server with complete user identity and authorization management in less than 20 minutes. Out of the box, Spring Boot and its Starter packages supply a near instant production-ready Tomcat server, and Okta hardens your APIs with a variety of OAuth flows just as fast. This tutorial will walk you through the complete process. The cherry on...
Secure your SPA with Spring Boot and OAuth
If you have a JavaScript single-page application (SPA) that needs to securely access resources from a Spring Boot application, you likely want to use the OAuth 2.0 implicit flow! With this flow your client will send a bearer token with each request and your server side application will verify the token with an Identity Provider (IdP). This allows your resource server to trust that your client is authorized to make the request. In OAuth terms...
Play Zork, Learn OAuth
In the early ’80s, some of the best “video” games were text-based adventures. These games would print out descriptive text of your surroundings and you would interact with the game using simple, but natural language commands like: “go north” or “take sword”. Fast forward some 30 years and a specification for an authorization framework called OAuth 2.0 was published. This framework allows an application to receive a token from an external party (like Okta) that...
Add Role-Based Access Control to Your App with Spring Security and Thymeleaf
User management functions are required by a wide variety of apps and APIs, and it’s a common use-case to partition access to parts of an application according to roles assigned to a user. This is the basis of role-based access control (RBAC). Okta manages these roles with groups. Users can belong to one or more groups. With the Okta Spring Security integration, these groups are automatically mapped to roles that can be called out in...
5 Tips for Building your Java API
Developers use APIs to for everything! You build APIs for your own apps to consume, or as a part of a microservices architecture. Bottom line, you’re building and using APIs to make your life easier. The ongoing effort to simplify development and work more efficiently, sometimes this also means looking for new libraries or processes (or more often less process). For many teams managing authentication and access control for their apps and APIs is more...
Let's Compare: JAX-RS vs Spring for REST Endpoints
Need to decouple your web service and client? You’re probably using REST endpoints, and if you’re a Java shop you’ve probably tried out JAX-RS, Spring REST, or both. But is one better than the other? In this post I’ll go over the differences between the two using basically the same code for an apples to apples comparison. In future posts I’ll show you how easy it is to secure these REST endpoints using Apache Shiro...
Secure a Spring Microservices Architecture with Spring Security, JWTs, Juiser, and Okta
You’ve built a microservices architecture with Spring Boot and Spring Cloud. You’re happy with the results, and you like how it adds resiliency to your application. You’re also pleased with how it scales and how different teams can deploy microservices independently. But what about security? Are you using Spring Security to lock everything down? Are your microservices locked down too, or are they just behind the firewall? This tutorial shows you how you can use...