Java + Spring Tutorials
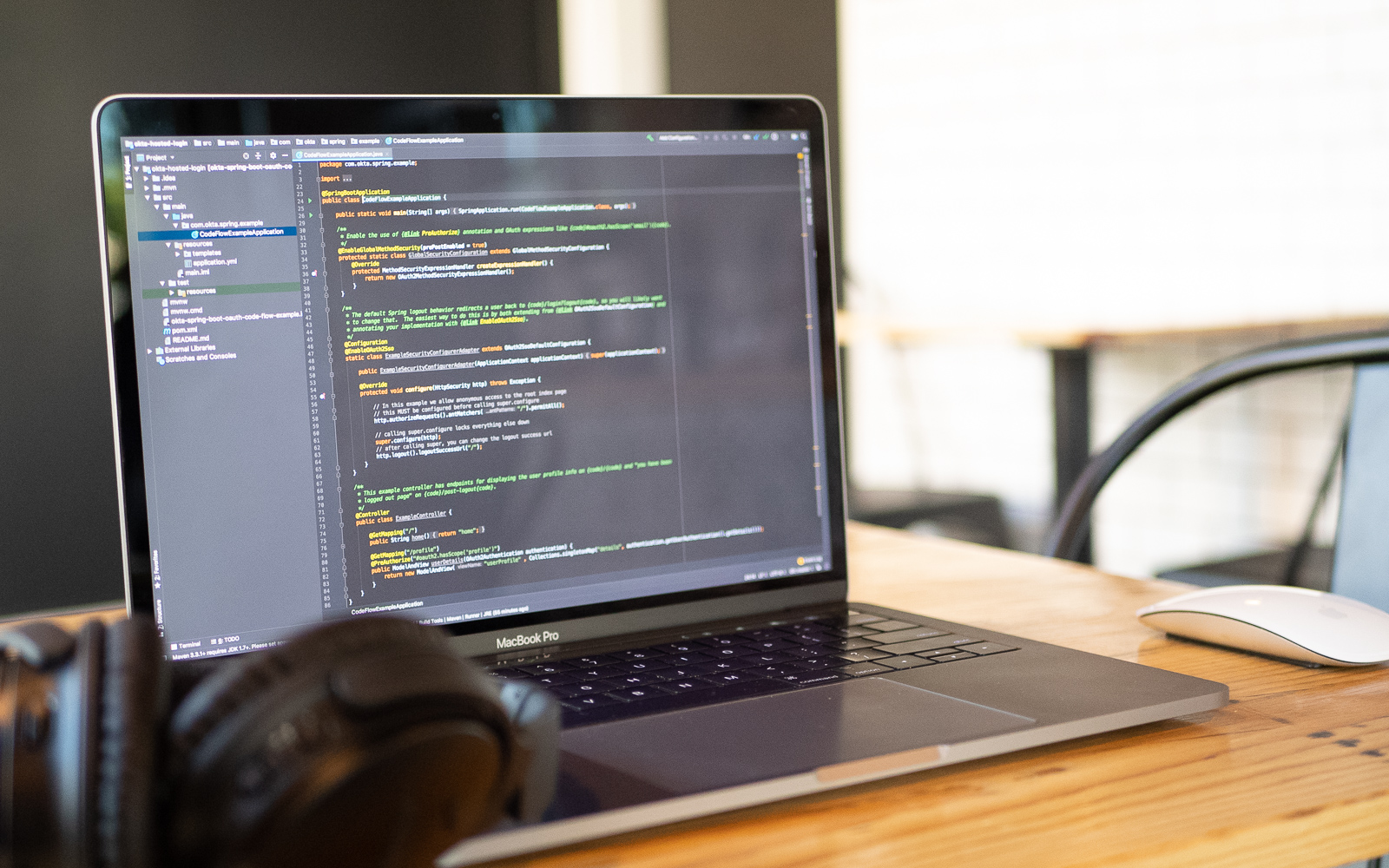
Spring Boot, and the Spring framework in general, are the core tools for the modern Java developer. They abstract away many nuances of development and architecture, allowing you to focus on building your business logic, aka, the parts of your app that will move the needle for your organization.
Here at Okta we believe in that abstraction, and that a big part of modern development is using the right tools to make the job not just easier, but better, and more securely done. What follows is a list of Java and Spring tutorials that we think can make you a better developer, and make the apps you build more secure. Some are tutorials our team has built, and many others are from experts around the web. Enjoy!
Spring + Spring Boot
Spring Boot is our fave, so we’ll start here.
- Spring Boot 2.1: Outstanding OIDC, OAuth 2.0, and Reactive API Support
- Deploy a Spring Boot Application into Tomcat
- Data Persistence with Hibernate and Spring
- Build a Web App with Spring Boot and Spring Security in 15 Minutes
- A Guide to Caching in Spring
- Spring MVC Tutorial
- Getting Started with Forms in Spring MVC
- How to Remote Debug Spring Boot Applications
- Logging with Spring Boot and Elastic Stack
Java
Because sometimes you have to go back to basics.
- Which Java SDK Should You Use?
- Learning Java as a First Language
- Better, Faster, Lighter Java with Java 12 and JHipster 6
- i18n in Java, Spring Boot, and JavaScript
- Tutorial: Create and Verify JWTs in Java
- Free Cookbook of 166 Java EE Code Examples
- Service Virtualization Meets Java: Hoverfly Tutorial
Securing Your Java and Spring Apps
Security is what we do, so let us help make your apps more secure!
- 10 Excellent Ways to Secure Your Spring Boot App
- A Quick Guide to Spring Boot Login Options
- A Quick Guide to OAuth 2.0 with Spring Security
- Simple Token Authentication for Java Apps
- Add User Authentication to Your Spring Boot App in 15 Minutes
- Secure Your Spring Boot Application with Multi-Factor Authentication
- Easy Single Sign-on with Spring Boot and OAuth 2.0
- Test Your Spring Boot Apps with JUnit 5
- Upgrading Spring Security and OAuth Tests Through the Eyes of a Java Hipster
- Migrate Your Spring Boot App to the Latest and Greatest Spring Security and OAuth 2.0
- Add Social Login to Your JHipster App
- Add Social Login to your Spring Boot 2.0 App
Spring with Frontend Technologies
If Spring is the peanut butter, React, Angular, and Vue are the jelly.
- Angular 8 + Spring Boot 2.2: Build a CRUD App Today!
- Build a Basic CRUD App with Angular 7 and Spring Boot 2.1
- Deploy Your Spring Boot + Angular PWA as a Single Artifact
- The Hitchhiker’s Guide to Testing Spring Boot APIs and Angular Components with Wiremock, Jest, Protractor, and Travis CI
- Use React and Spring Boot to Build a Basic CRUD App
- Build a Photo Gallery PWA with React, Spring Boot, and JHipster
- Full Stack Reactive with Spring Webflux, WebSockets, and React
- Build a Simple CRUD App with Spring Boot and Vue.js
- Bootful Development with Spring Boot and Vue
- Introduction to Thymeleaf in Spring
REST vs GraphQL in Spring
Which is best for what you’re building?
- REST with Spring Tutorial
- Create a Secure Spring REST API
- Build a Java REST API with Java EE and OIDC
- Build a Secure API with Spring Boot and GraphQL
- Error Handling for REST with Spring
SQL, Microservices, Mobile Apps, and More
Advanced topics for specific needs.
- Java Microservices with Spring Boot and Spring Cloud
- Java Microservices with Spring Cloud Config and JHipster
- Build a Microservices Architecture with Spring Boot and Kubernetes
- Secure Service-to-Service Spring Microservices with HTTPS and OAuth 2.0
- Build Spring Microservices and Dockerize them for Production
- Get Started with Reactive Programming in Spring
- Build a Reactive App in Spring Boot with MongoDB
- Build Reactive APIs with Spring Webflux
- Spring Boot with PostgreSQL, Flyway, and JSONB
- Spring Persistence Tutorial
- Build a Basic App with Spring Boot and JPA using PostgreSQL
- Build a Mobile App with React Native and Spring Boot
- Add CI/CD to Your Spring Boot App with Jenkins X and Kubernetes
- An Overview of MicroProfile Configuration
Looking for more awesome Java and Spring Boot tutorials? Follow us on Twitter for the latest from the @OktaDev team and all our friends!
Okta Developer Blog Comment Policy
We welcome relevant and respectful comments. Off-topic comments may be removed.