Articles tagged angular
Secure OAuth 2.0 Access Tokens with Proofs of Possession
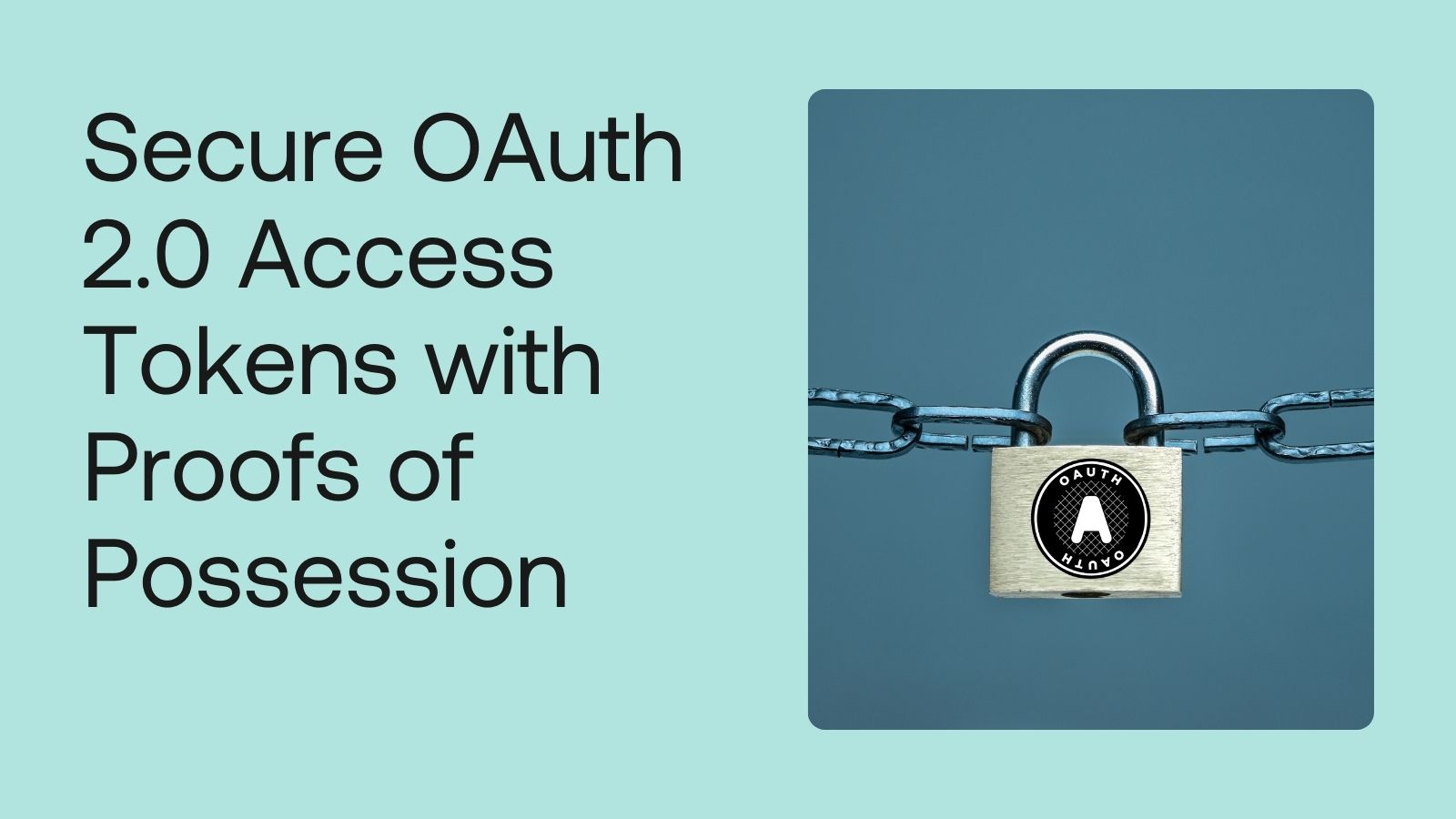
In OAuth, a valid access token grants the caller access to resources and the ability to perform actions on the resources. This means the access token is powerful and dangerous if it falls into malicious hands. The traditional bearer token scheme means the token grants anyone who possesses it access. A new OAuth 2.0 extension specification, Demonstrating Proof of Possession (DPoP), defines a standard way that binds the access token to the OAuth client sending...
Add Step-up Authentication Using Angular and NestJS
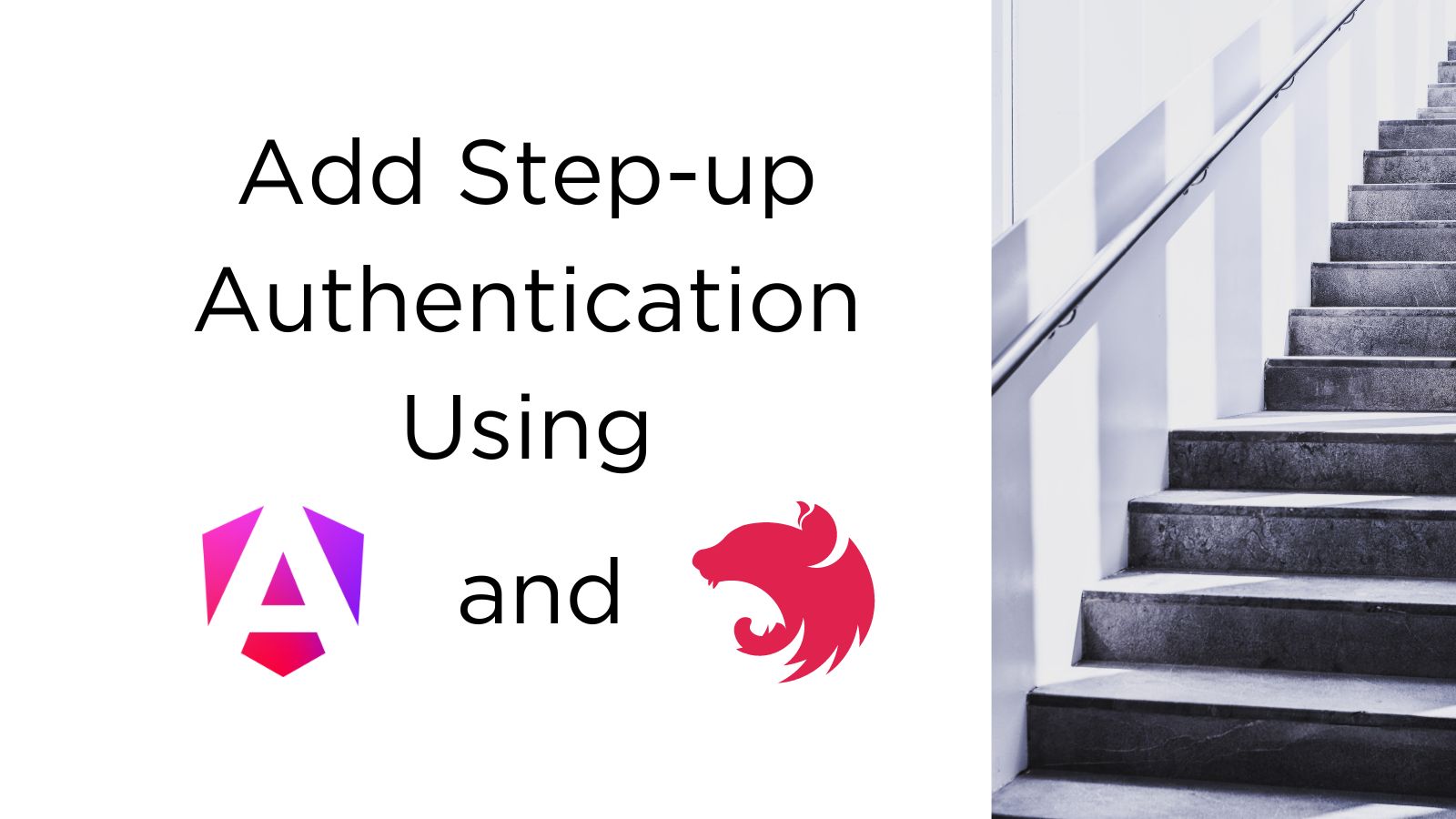
The applications you work on expect good authentication as a secure foundation. In the past, we treated authentication as binary. You are either authenticated or not. You had to set the same authentication mechanism for access to your application without a standard way to change authentication mechanisms conditionally. Consider the case where sensitive actions warrant verification, such as making a large financial transaction or modifying top-secret data. Those actions require extra scrutiny! Note: In May...
Flexible Authentication Configurations in Angular Applications Using Okta
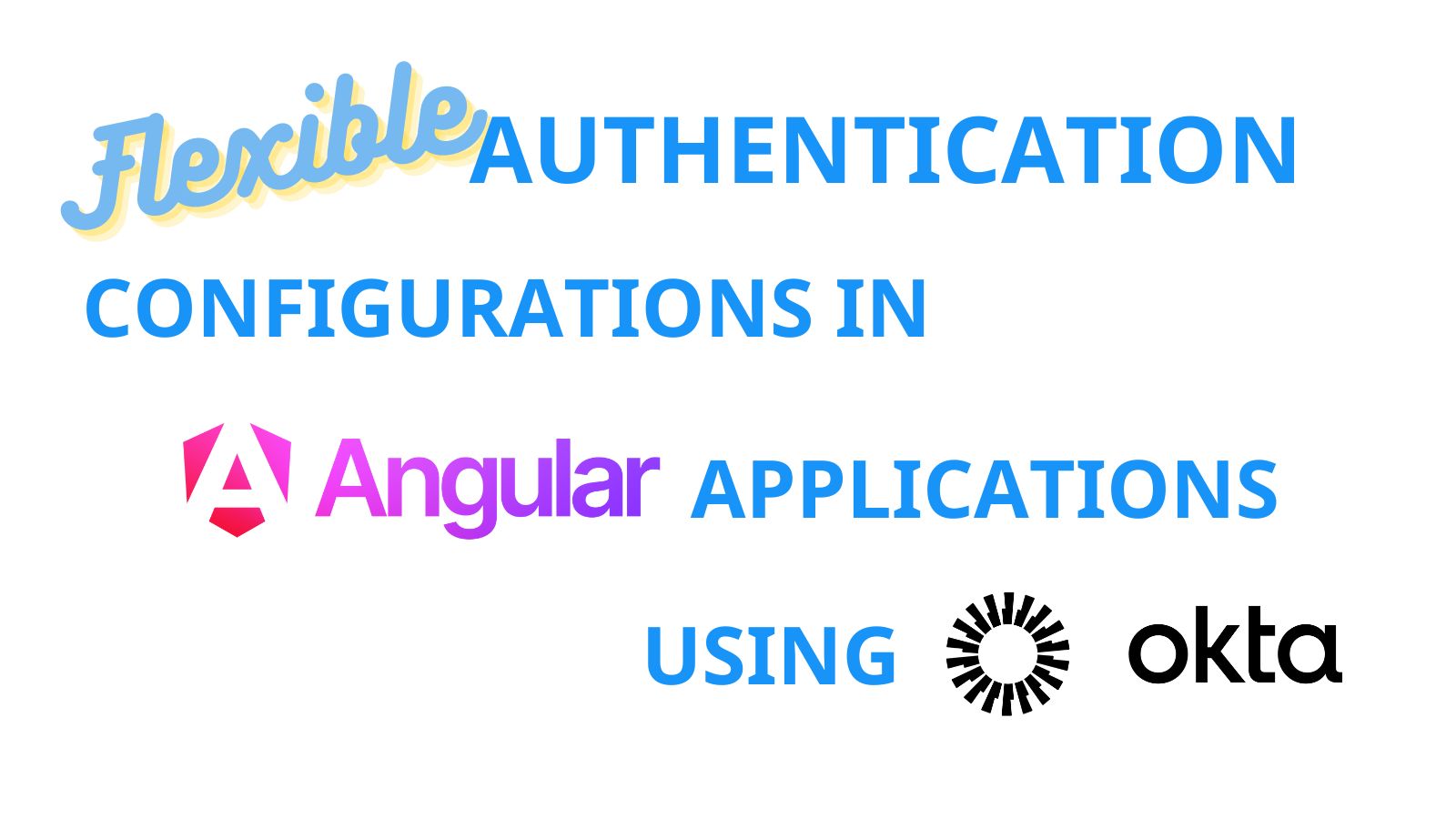
Are you ready to hear about the ultimate flexibility in configuring authentication properties in the Okta Angular SDK? You’ll want to check out this excellent new feature and walk through the steps of adding authentication using Okta to Angular applications. Note: In May 2025, the Okta Integrator Free Plan replaced Okta Developer Edition Accounts, and the Okta CLI was deprecated. We preserved this post for reference, but the instructions no longer work exactly as written....
How Authentication and Authorization Work for SPAs
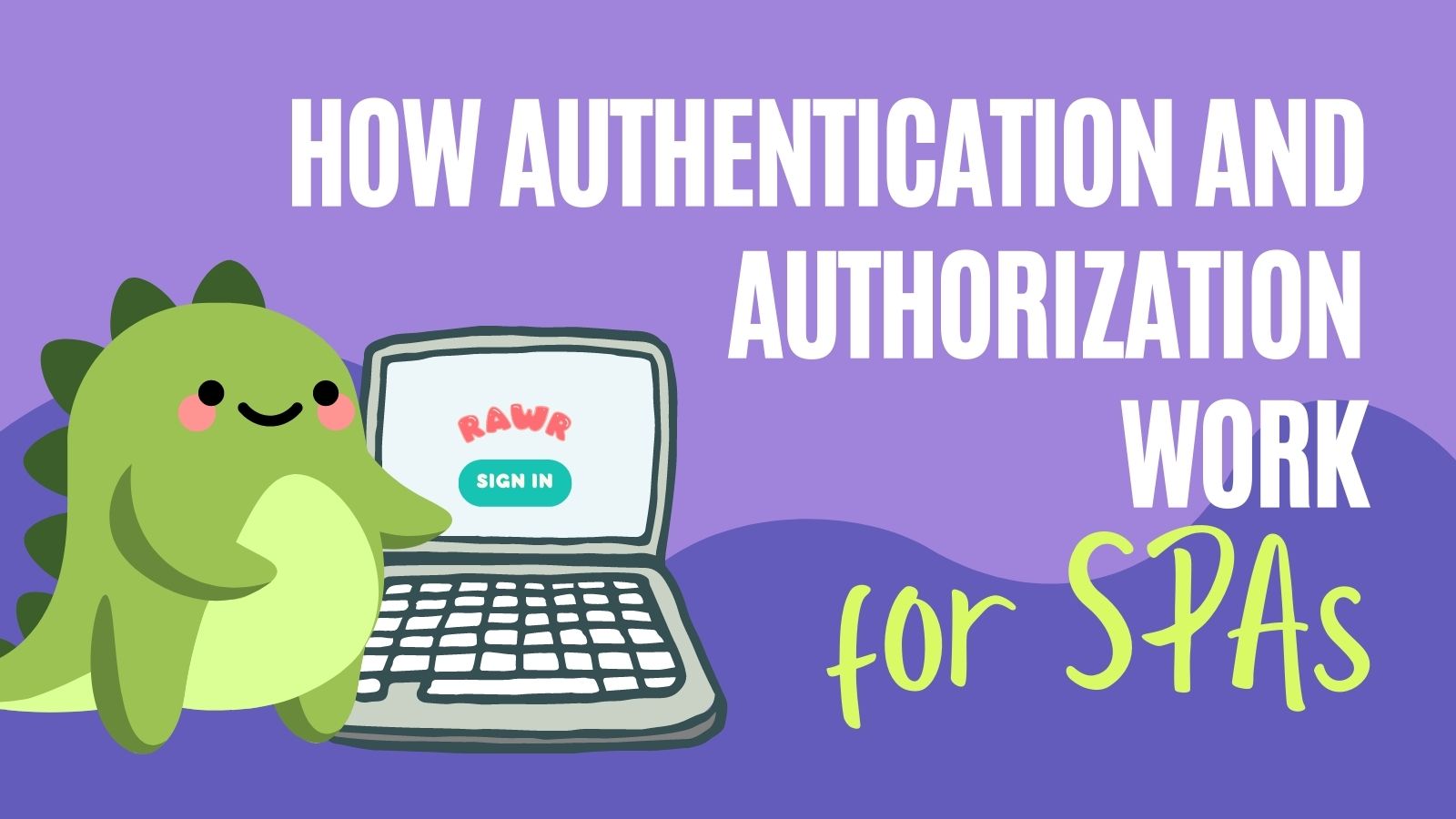
Adding authentication to public clients such as Single Page Applications (SPA) and JavaScript applications can be a source of confusion. Identity Providers like Okta try to help you via multiple support systems. Still, it can feel like a lot of work. Especially since you’re responsible for way more than authentication alone in the applications you work on! As part of authentication, your client application makes multiple calls to an authorization server, and you get back...
Streamline Your Okta Configuration in Angular Apps
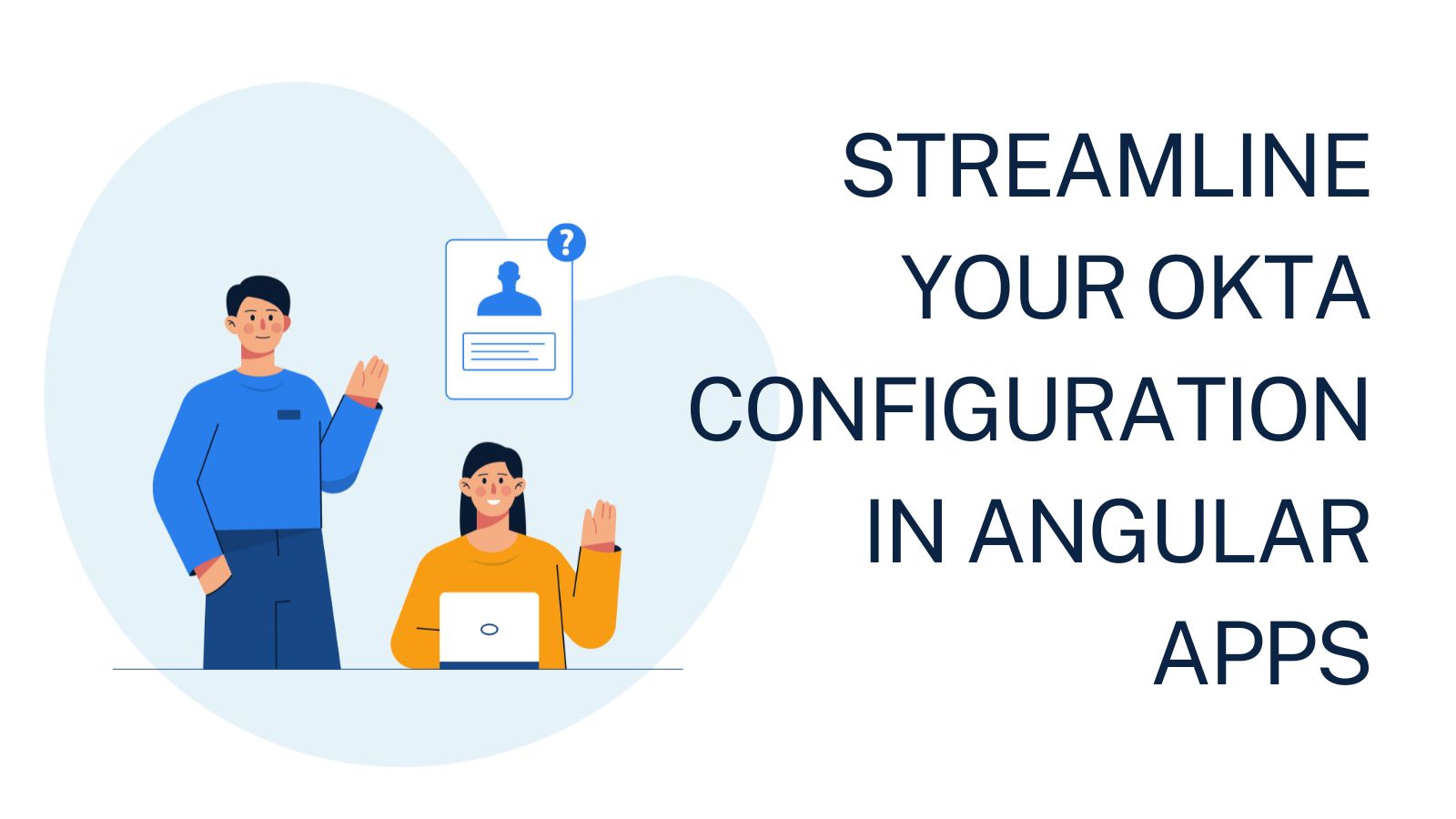
The Okta Angular SDK supports a new and improved configuration method to pass in the required properties for incorporating Okta in your Angular applications. Now, you can add Okta to your Angular application using the forRoot pattern! The forRoot pattern in Angular The forRoot pattern helps ensure services defined in NgModules aren’t duplicated across the application. This is especially noteworthy if you have a module that both provides services and also has component and directive...
Practical Uses of Dependency Injection in Angular
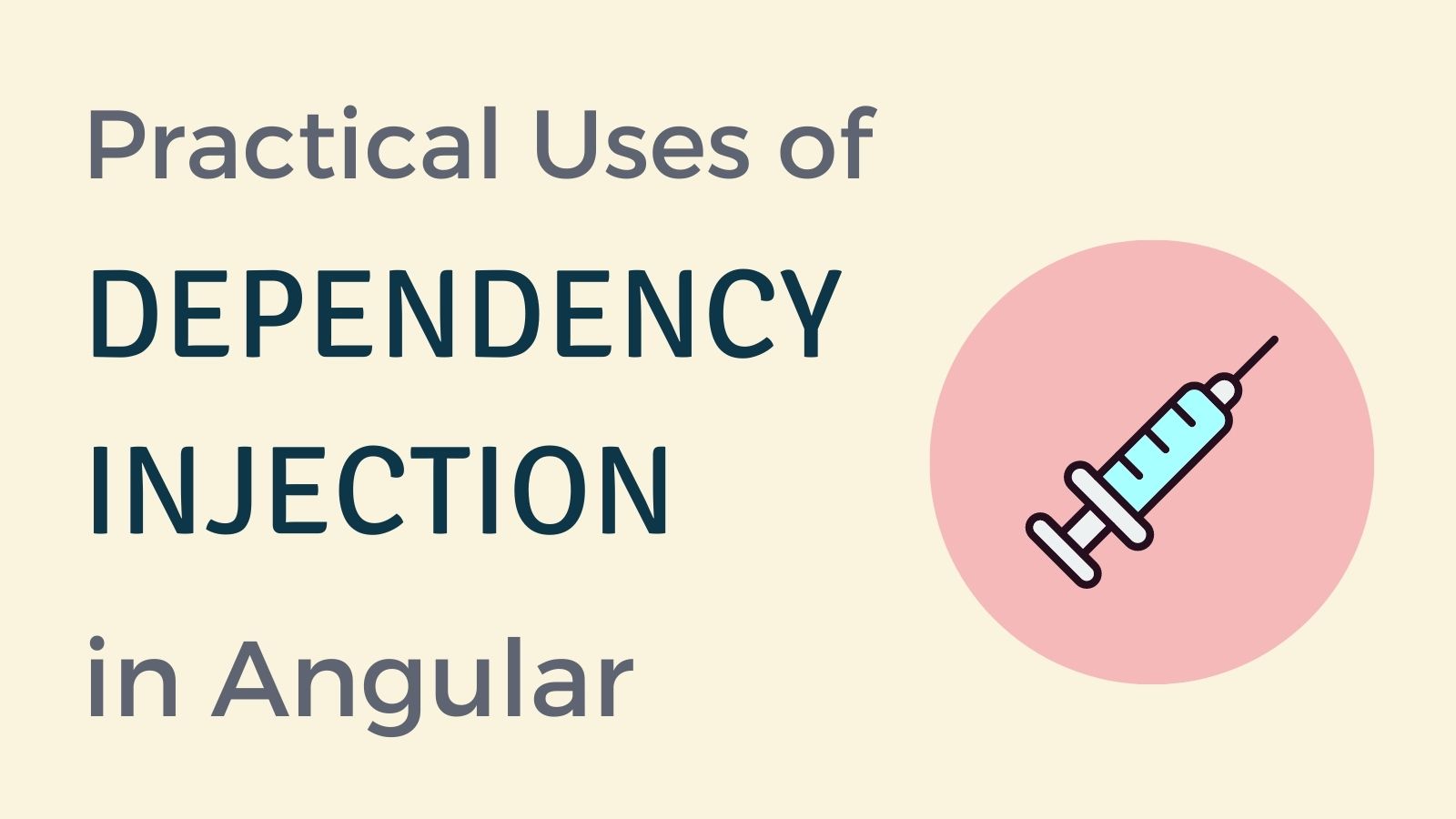
Angular has an extensive system that uses *providers” to add and configure dependencies to the application you’re building. To create providers, you use the built-in Dependency Injection (DI) system. This post will cover Angular’s powerful DI system at a high level and demonstrate a few practical use cases and strategies for configuring your dependencies. Let’s get practical! Table of Contents Quick overview of Dependency Injection Angular’s Dependency Injection system Injection tokens in Angular Configuring providers...
Protect Your Angular App From Cross-Site Scripting
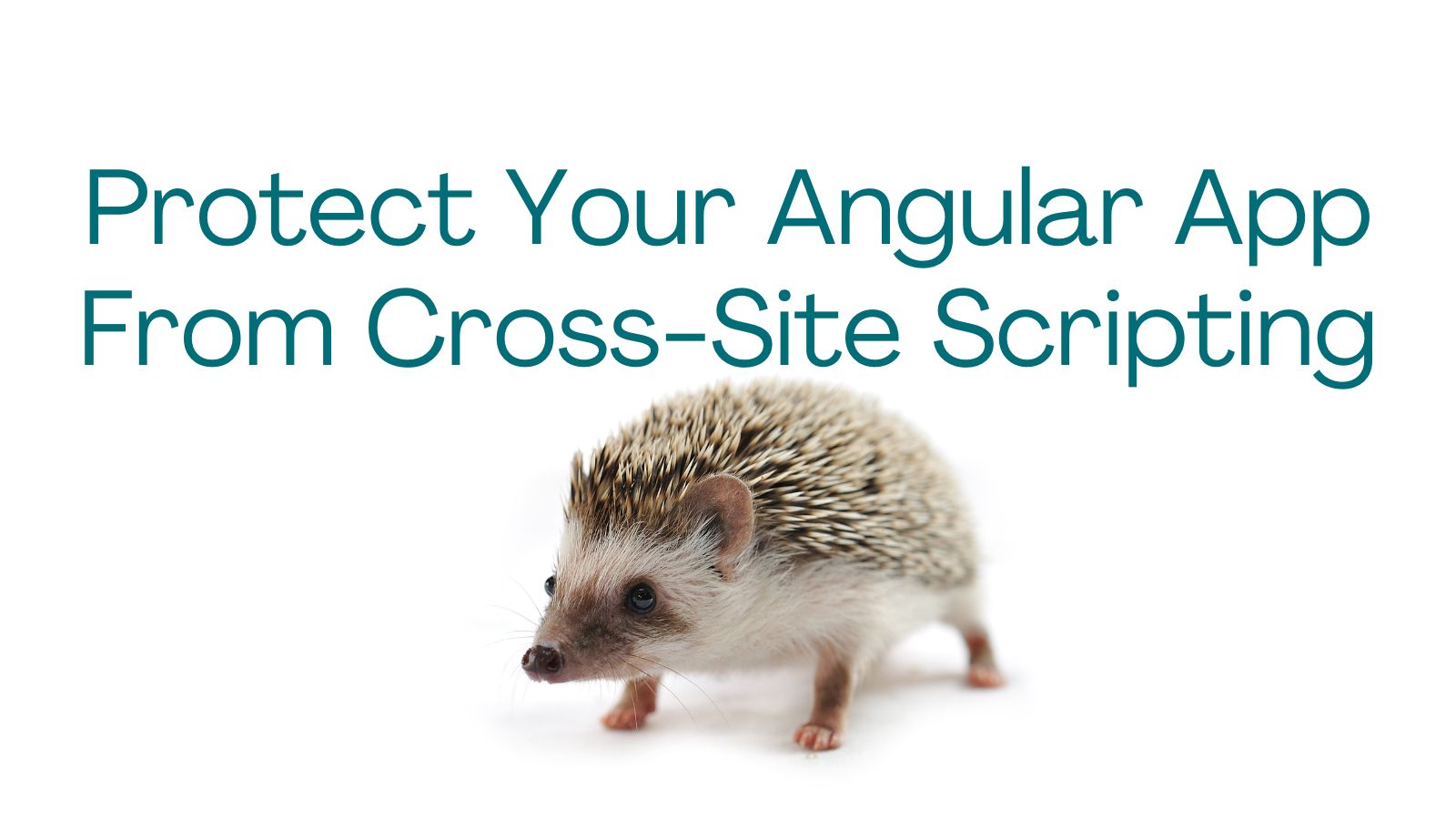
In the last post of this SPA security series, we covered Cross-Site Request Forgery (CSRF) and how Angular helps you with a mitigation technique. Posts in the SPA web security series 1. Defend Your SPA From Security Woes 2. Defend Your SPA From Common Web Attacks 3. Protect Your Angular App From Cross-Site Request Forgery 4. Protect Your Angular App From Cross-Site Scripting Next, we’ll dive into Cross-Site Scripting (XSS) and look at the built-in...
Protect Your Angular App From Cross-Site Request Forgery
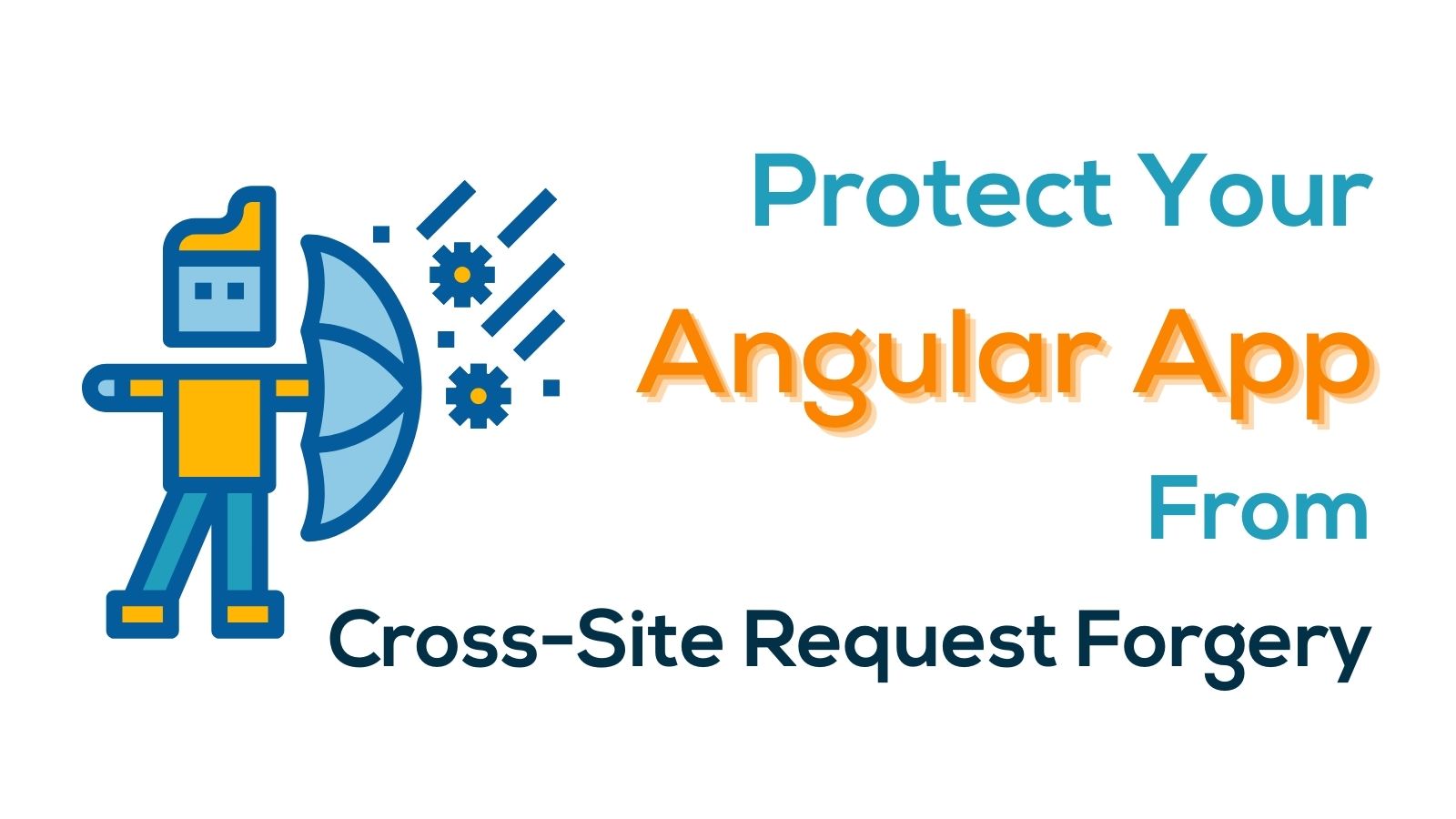
Previously, I wrote about web security at a high level and the framework-agnostic ways to increase safety and mitigate vulnerabilities. Posts in the SPA web security series 1. Defend Your SPA from Security Woes 2. Defend Your SPA from Common Web Attacks 3. Protect Your Angular App From Cross-Site Request Forgery 4. Protect Your Angular App From Cross-Site Scripting Now, I want to dive a little deeper into the vulnerabilities. In this short post, we’ll...
Defend Your SPA from Common Web Attacks
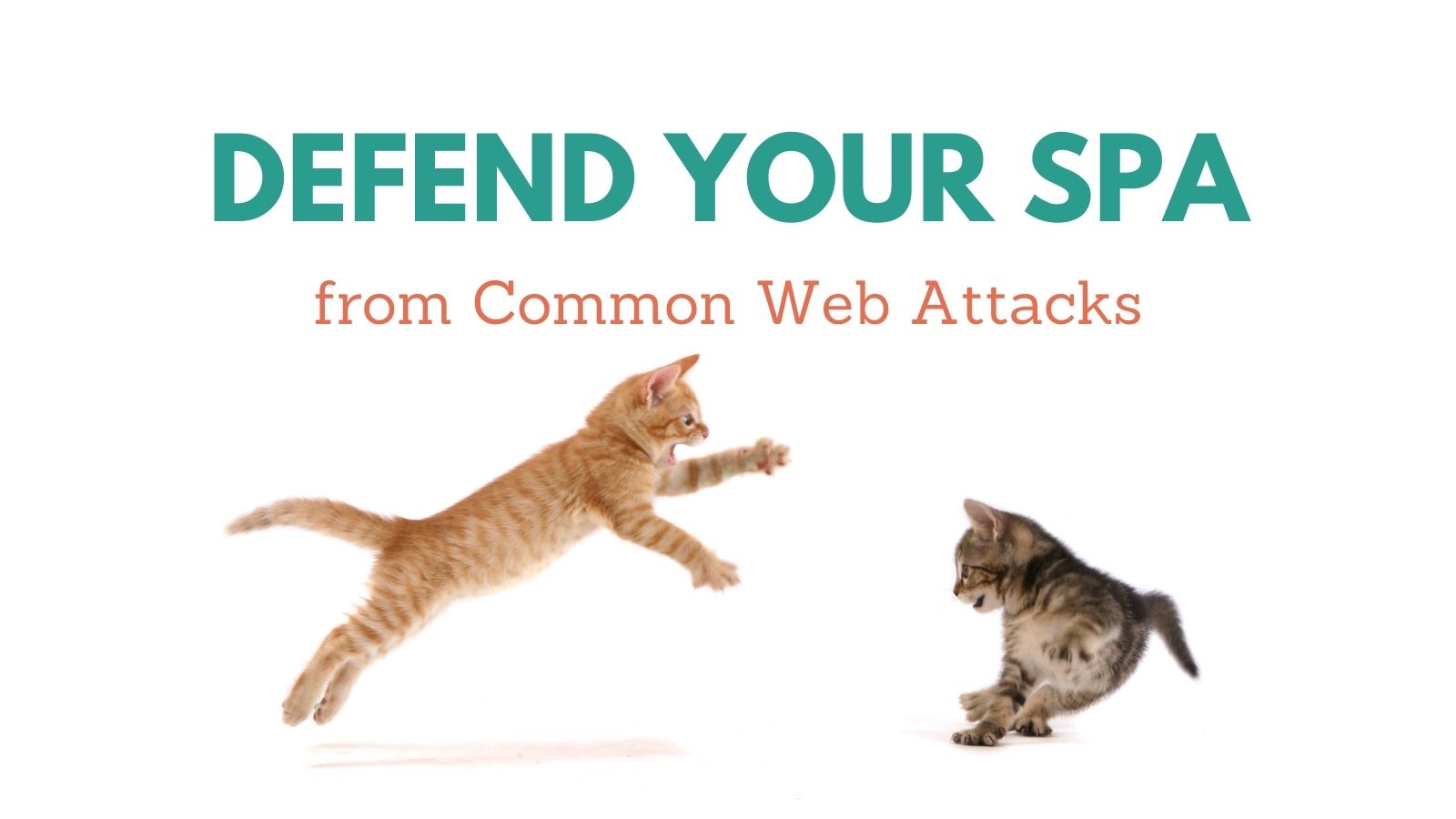
This is the second post in a series about web security for SPAs. In the last post, we laid the groundwork for thinking about web security and applying security mechanisms to our application stack. We covered the OWASP Top Ten, using secure data communication with SSL/TLS, using security headers to help enhance built-in browser mechanisms, keeping dependencies updated, and safeguarding cookies. Posts in the SPA web security series 1. Defend Your SPA from Security Woes...
Defend Your SPA from Security Woes
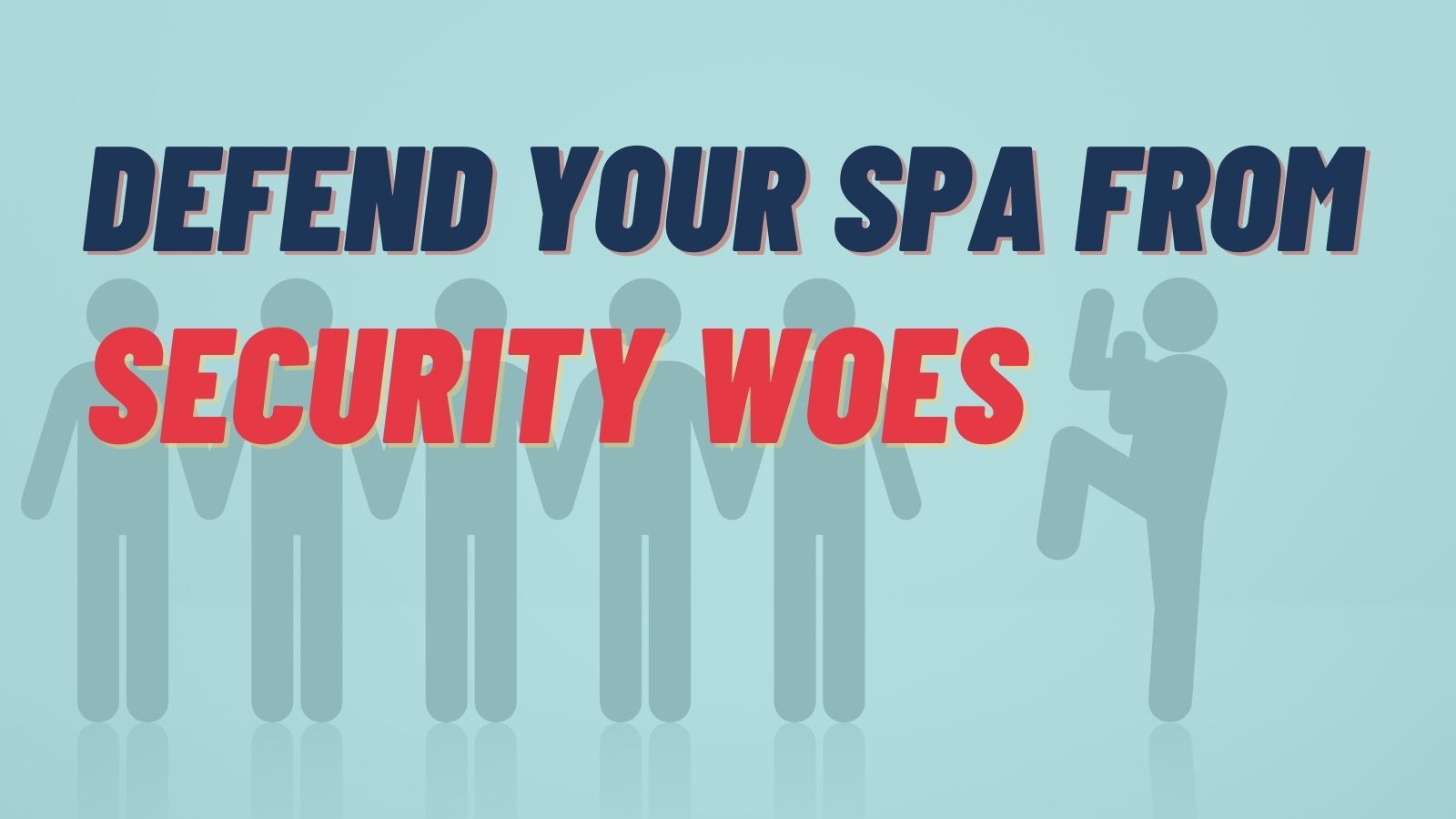
There’s a lot of information floating out there about web security. But when I read through the material, I noticed some information wasn’t up to date, or it was written specifically for traditional server-rendered web applications, or the author recommended anti-patterns. In a series of posts, I will cover web security concerns that all web devs should be aware of, emphasizing client-side applications, namely Single Page Applications (SPAs). Furthermore, I’m not going to get into...
Secure and Deploy Micro Frontends with Angular
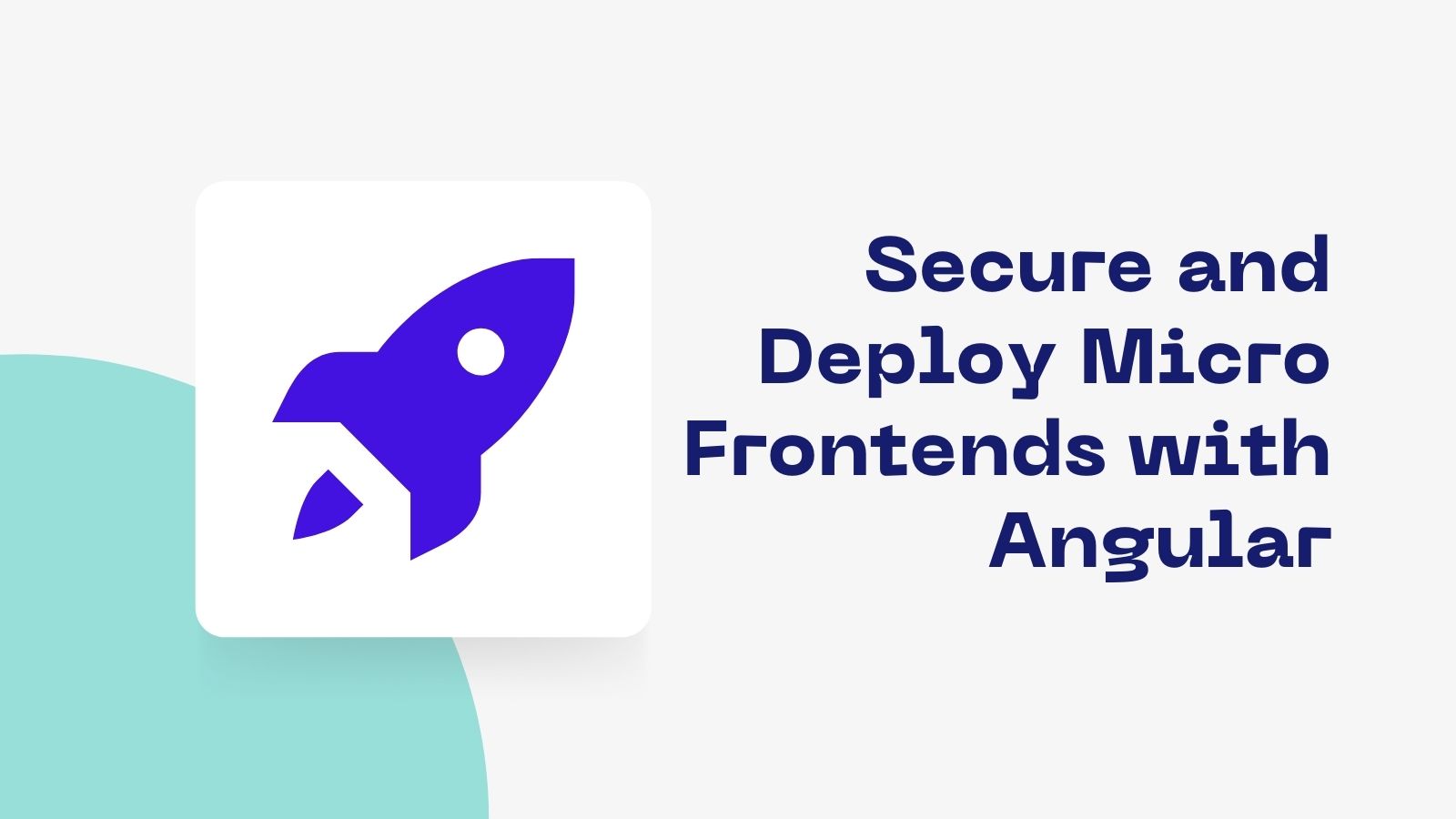
Micro frontends continue to gain interest and traction in front-end development. The architecture models the same concept as micro services - as a way to decompose monolithic front-end applications. And just like with micro services, micro frontends have complexities to manage. This post is part two in a series about building an e-commerce site with Angular using micro frontends. We use Webpack 5 with Module Federation to wire the micro frontends together, demonstrate sharing authenticated...
How to Build Micro Frontends Using Module Federation in Angular
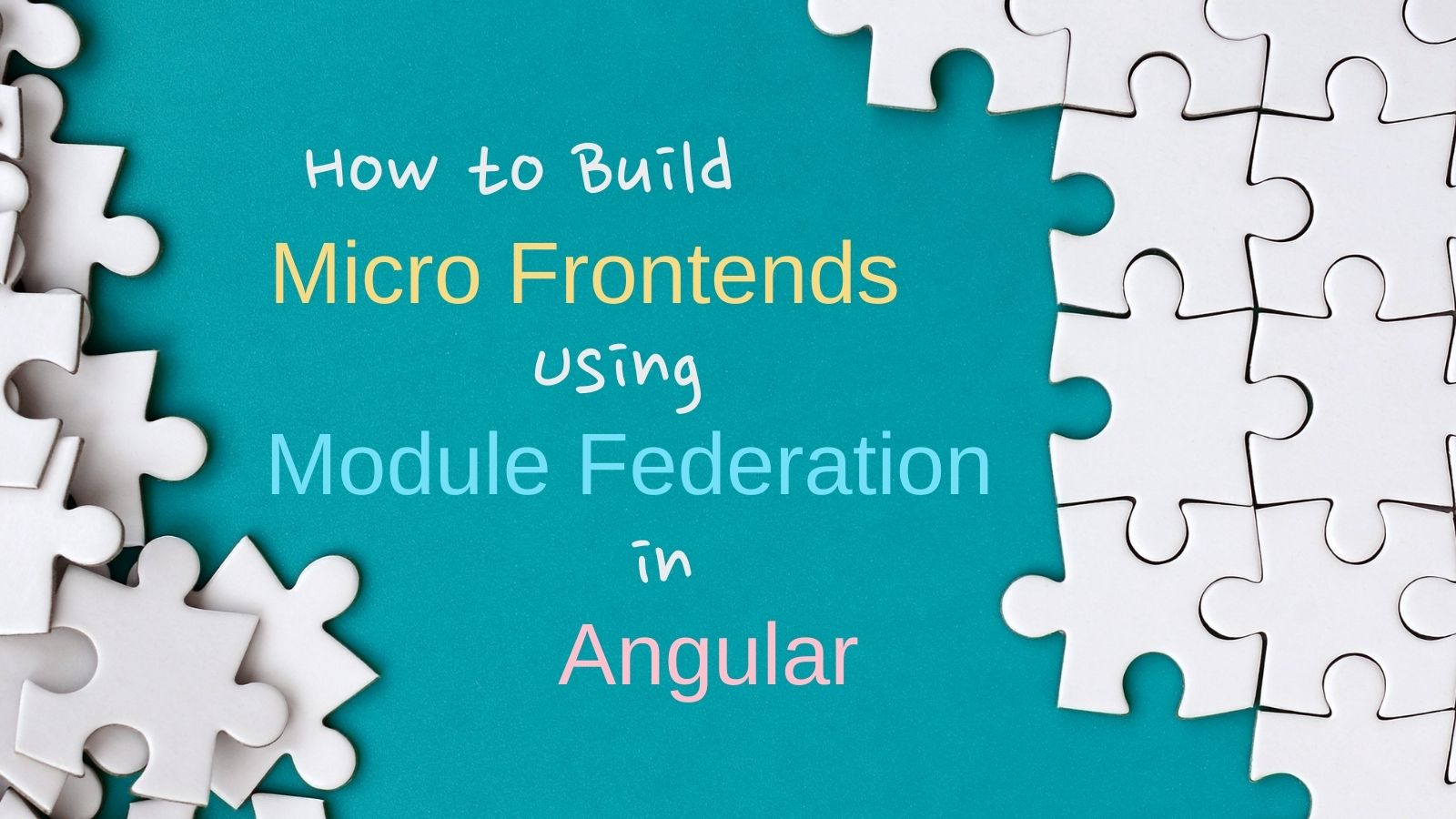
The demands placed on front-end web applications continue to grow. As consumers, we expect our web applications to be feature-rich and highly performant. As developers, we worry about how to provide quality features and performance while keeping good development practices and architecture in mind. Enter micro-frontend architecture. Micro frontends are modeled after the same concept as microservices, as a way to decompose monolithic frontends. You can combine micro-sized frontends to form a fully-featured web app....
Three Ways to Configure Modules in Your Angular App
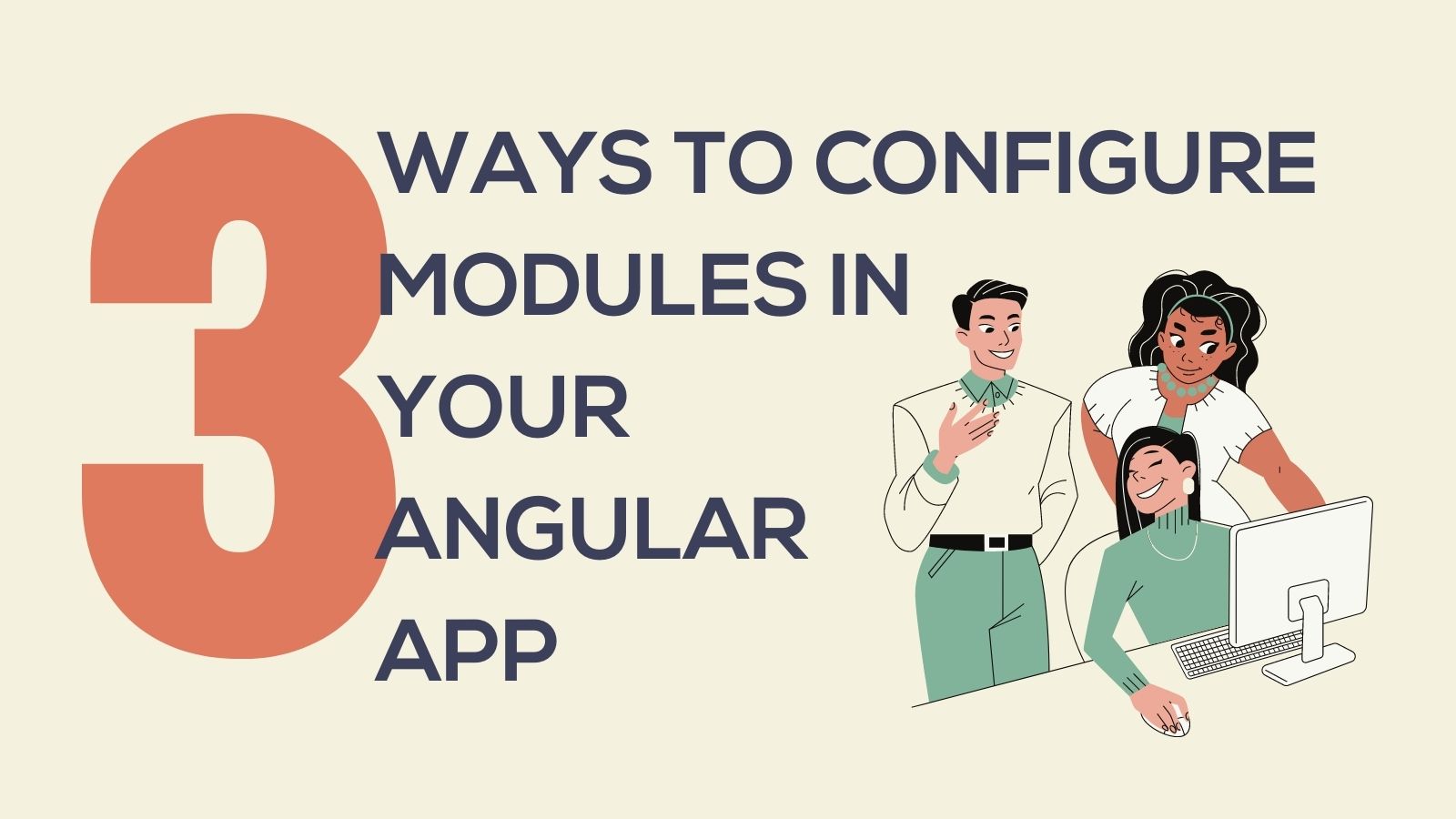
Configurations are part of a developer’s life. Configuration data is information your app needs to run and may include tokens for third-party systems or settings you pass into libraries. There are different ways to load configuration data as part of application initialization in Angular. Your requirements for configuration data might change based on needs. For example, you may have one unchanging configuration for your app, or you may need a different configuration based on the...
Add OpenID Connect to Angular Apps Quickly
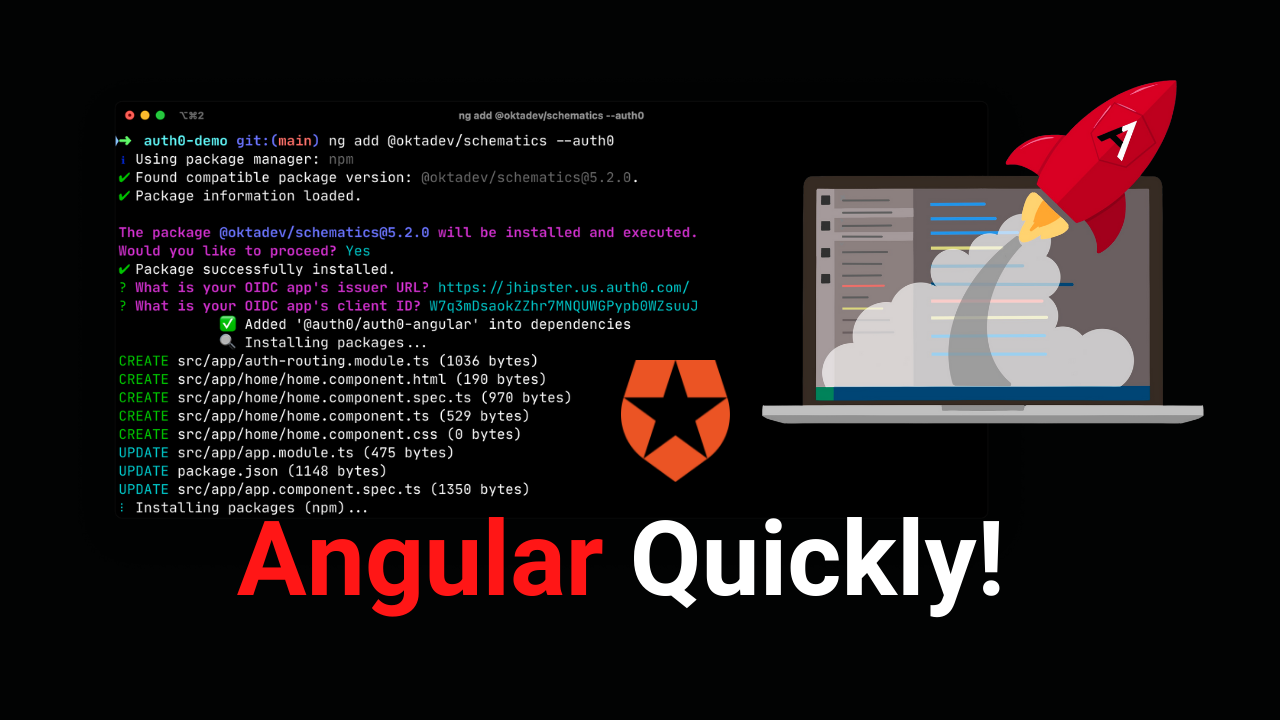
AngularJS 1.0 was released in October 2010. At the time, it was considered one of the most revolutionary and popular web frameworks ever to see the light of day. Developers loved it, and created many apps with it. However, as a pioneer in the JS framework space, AngularJS had some growing pains and significant issues. The team went back to the drawing board for a major breaking release with Angular 2. It took two years...
Loading Components Dynamically in an Angular App
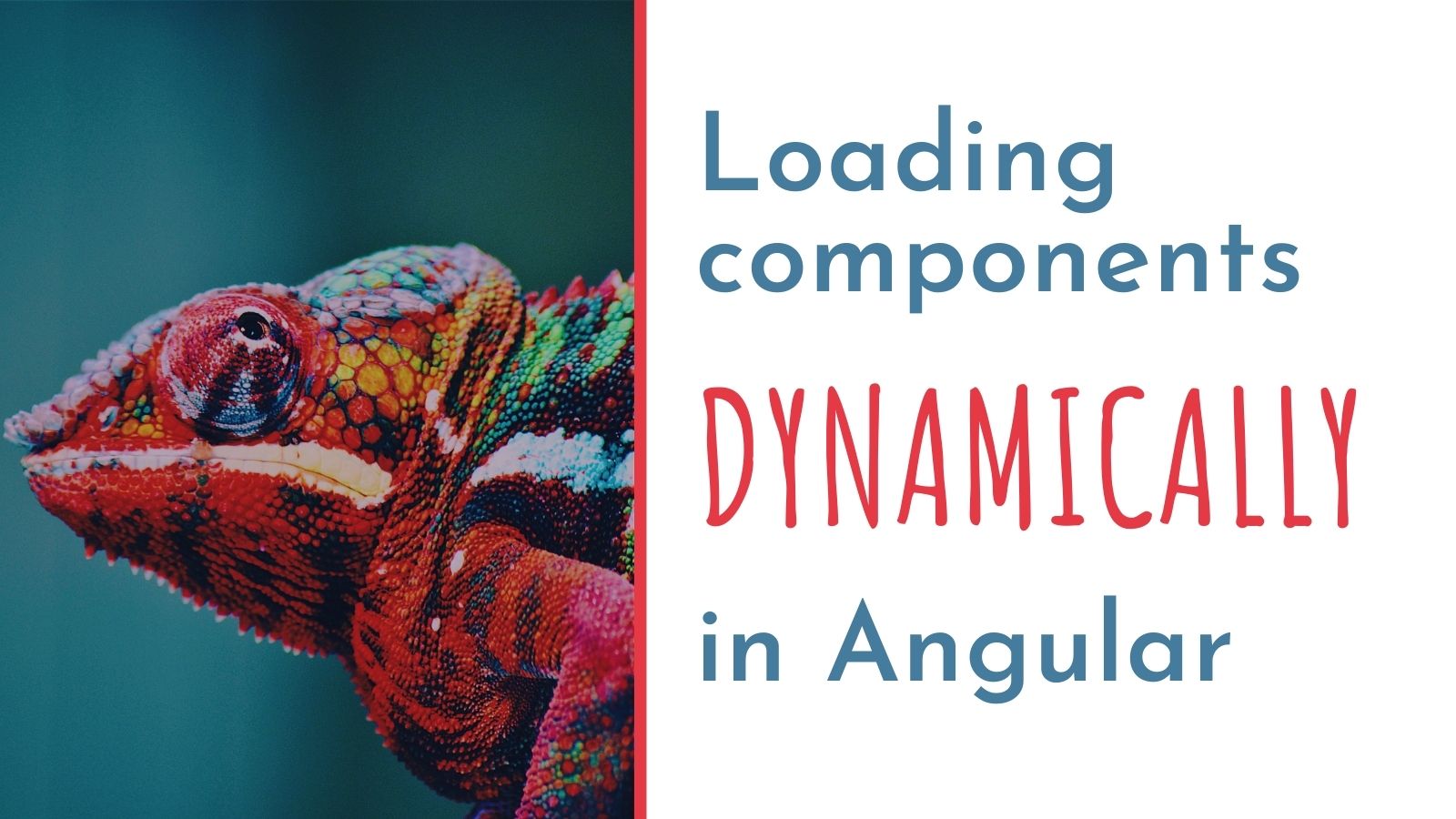
Businesses have unique and complex needs. In addition to the user or organization-specific data to show, there might be a need to display different views and content conditionally. The conditions might include the user’s role or which department they belong to. The information about a user might be part of the authenticated user’s ID token as a profile claim. In Angular, you can show different components or even parts of templates conditionally using built-in directives...
What You Need to Know about Angular v13
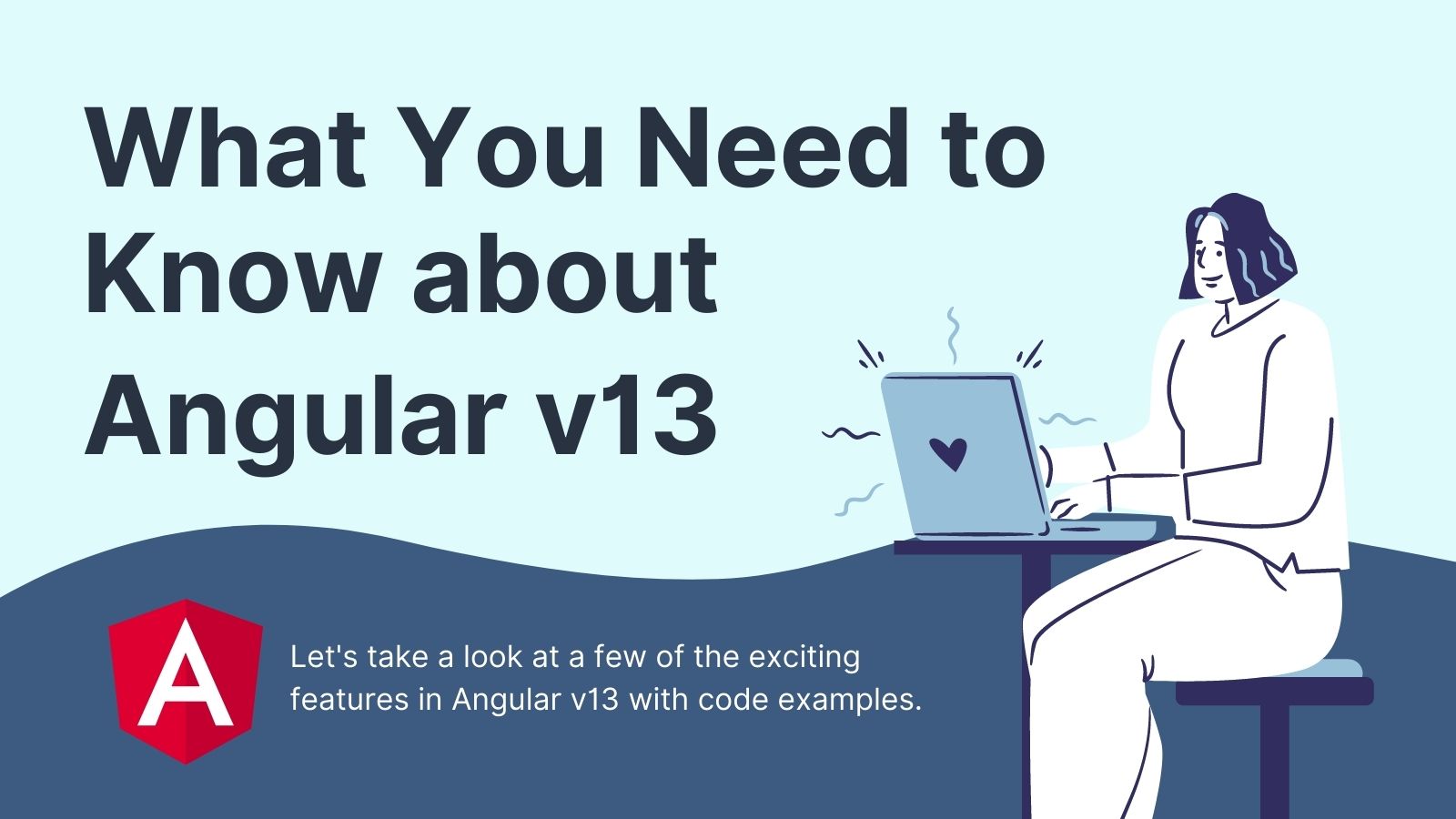
Angular v13 has arrived! And with it come a lot of exciting new features and updates. Angular continues to improve runtime performance, decrease compilation time, promote good software development practices, enhance developer experience, and keep up to date with dependencies such as TypeScript and RxJS. Is anyone else excited about RxJS v7?! 🤩 Let’s take a look at a few of the many new exciting features in Angular v13 with some code examples using authentication....
A Quick Guide to Angular and GraphQL
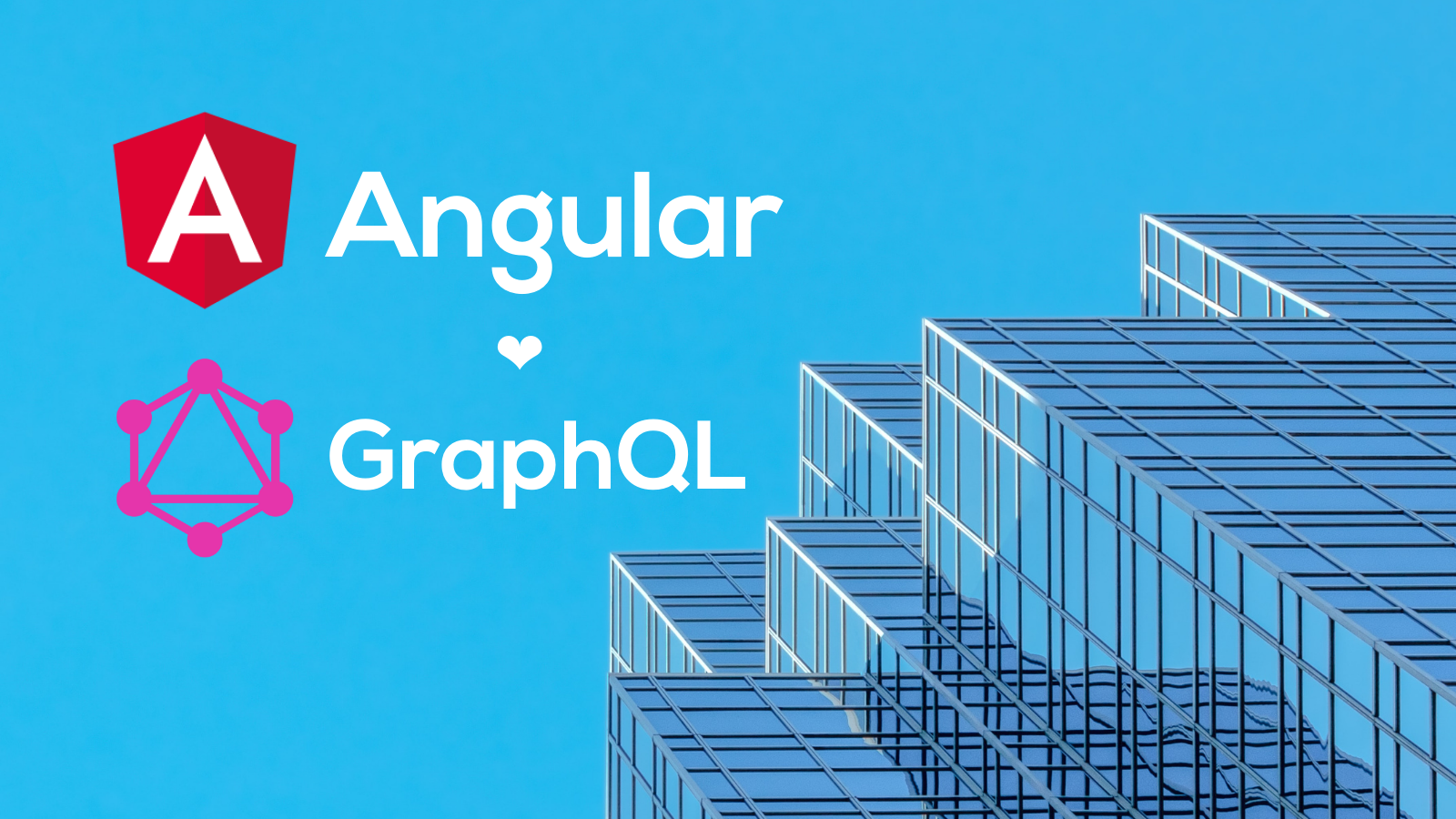
Over the past five years, GraphQL has established itself as the most popular alternative to REST APIs. GraphQL has several advantages over traditional REST-based services. First of all, GraphQL makes the query schema available to the clients. A client that reads the schema immediately knows what services are available on the server. On top of that, the client is able to perform a query on a subset of the data. Both of these features make...
Flying Into Okta
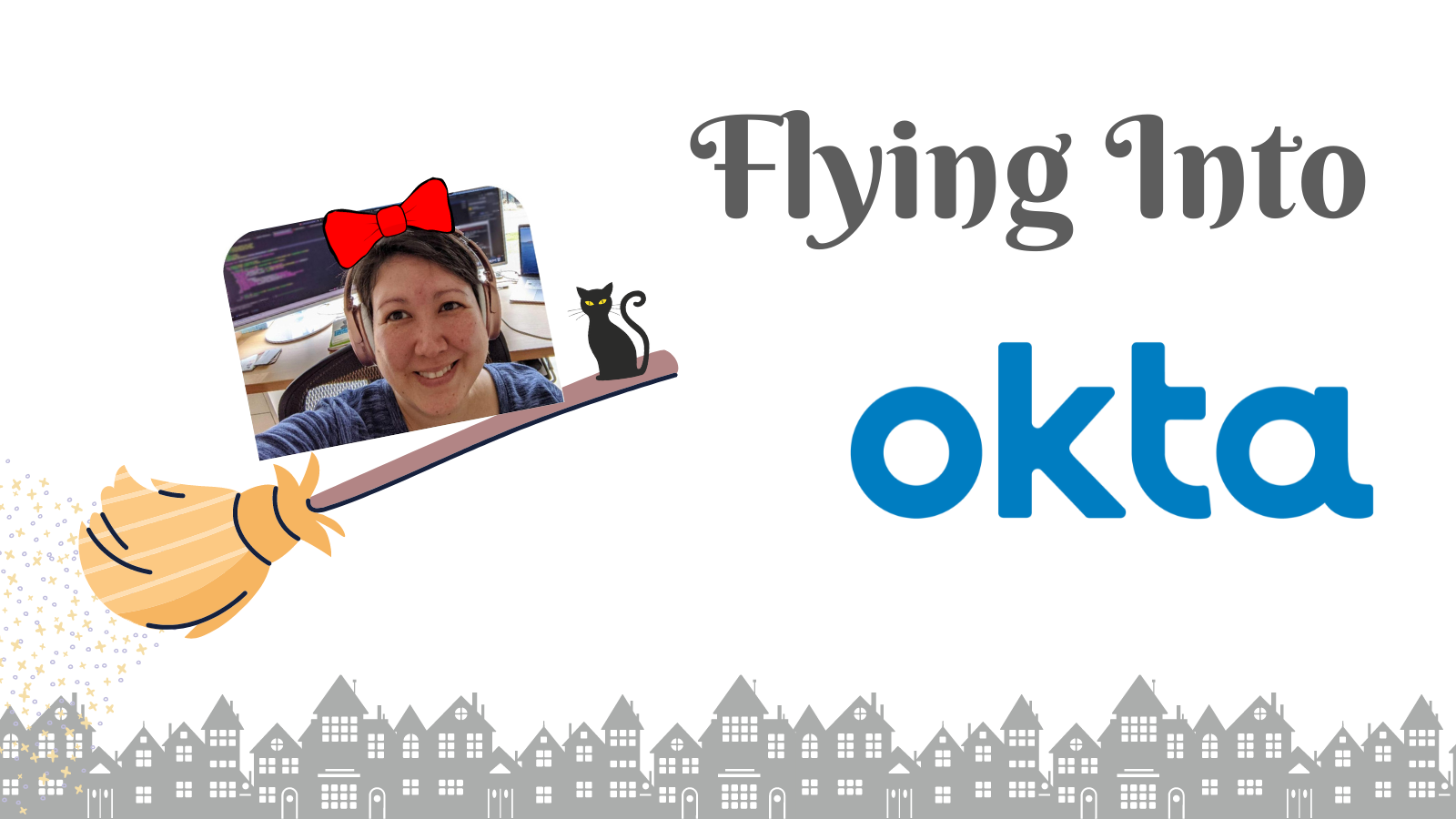
“Just follow your heart and keep smiling.” – Kiki’s Delivery Service I’m embarking on a new adventure and entering the wide world of Developer Advocacy at Okta! Much like Kiki setting out on her journey, I’m full of enthusiasm and curiosity and am ready to fly. I’m thrilled to be here at Okta and looking forward to everything. Now, I just need to get a talking cat… “Smile. We have to make a good first...
Use the Okta CLI to Quickly Build Secure Angular Apps
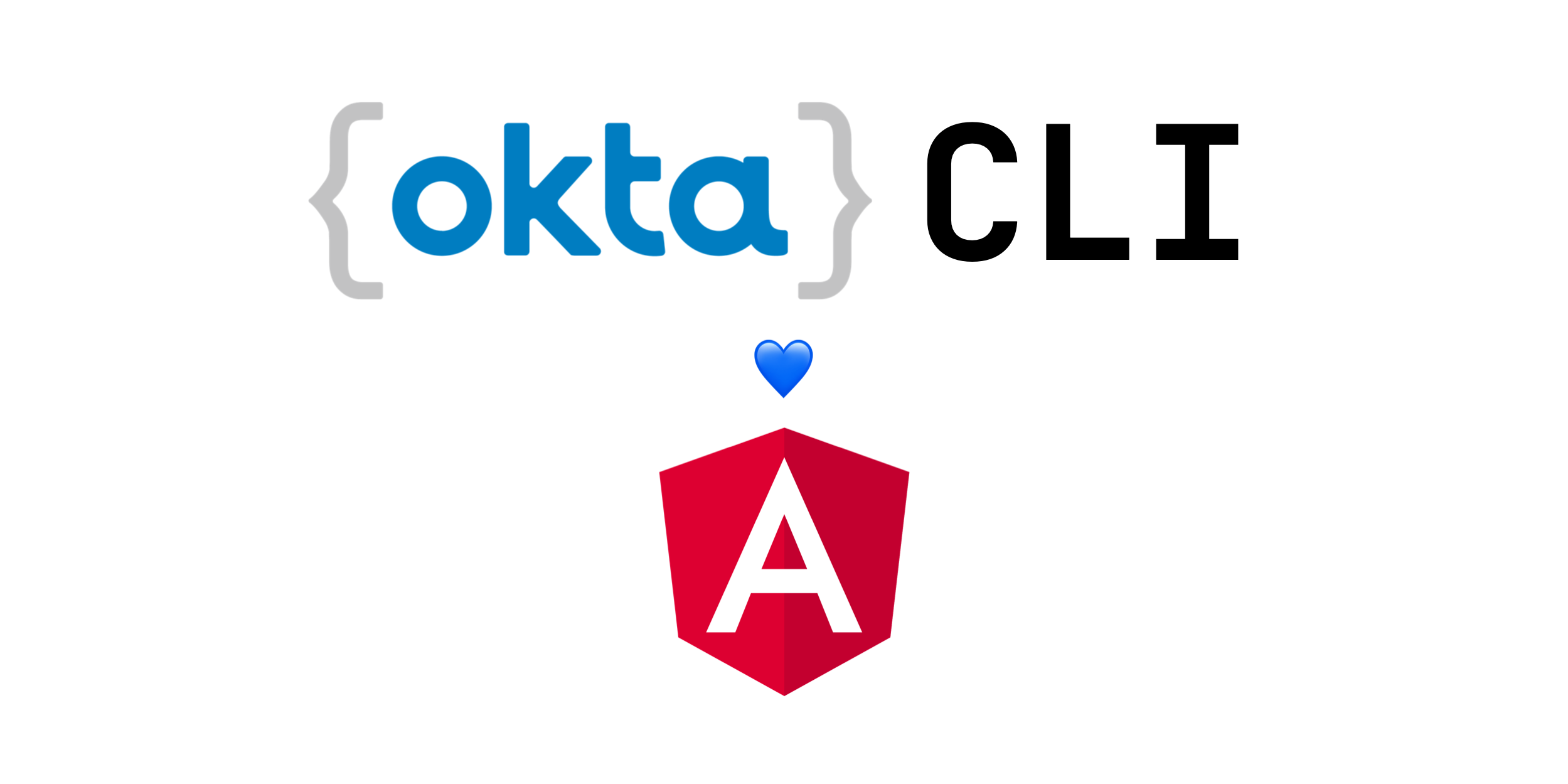
The Okta CLI is a new tool we’ve created here at Okta. It’s designed to streamline creating new Okta accounts, registering apps, and getting started. Wwwhhaaattt, you might say?! That’s right, it’s super awesome! To show you how easy it is, I created a screencast that shows you how to use it with Angular. To create the same app as the one shown in this video, you’ll need to run okta start angular --branch widget....
Ionic + Sign in with Apple and Google
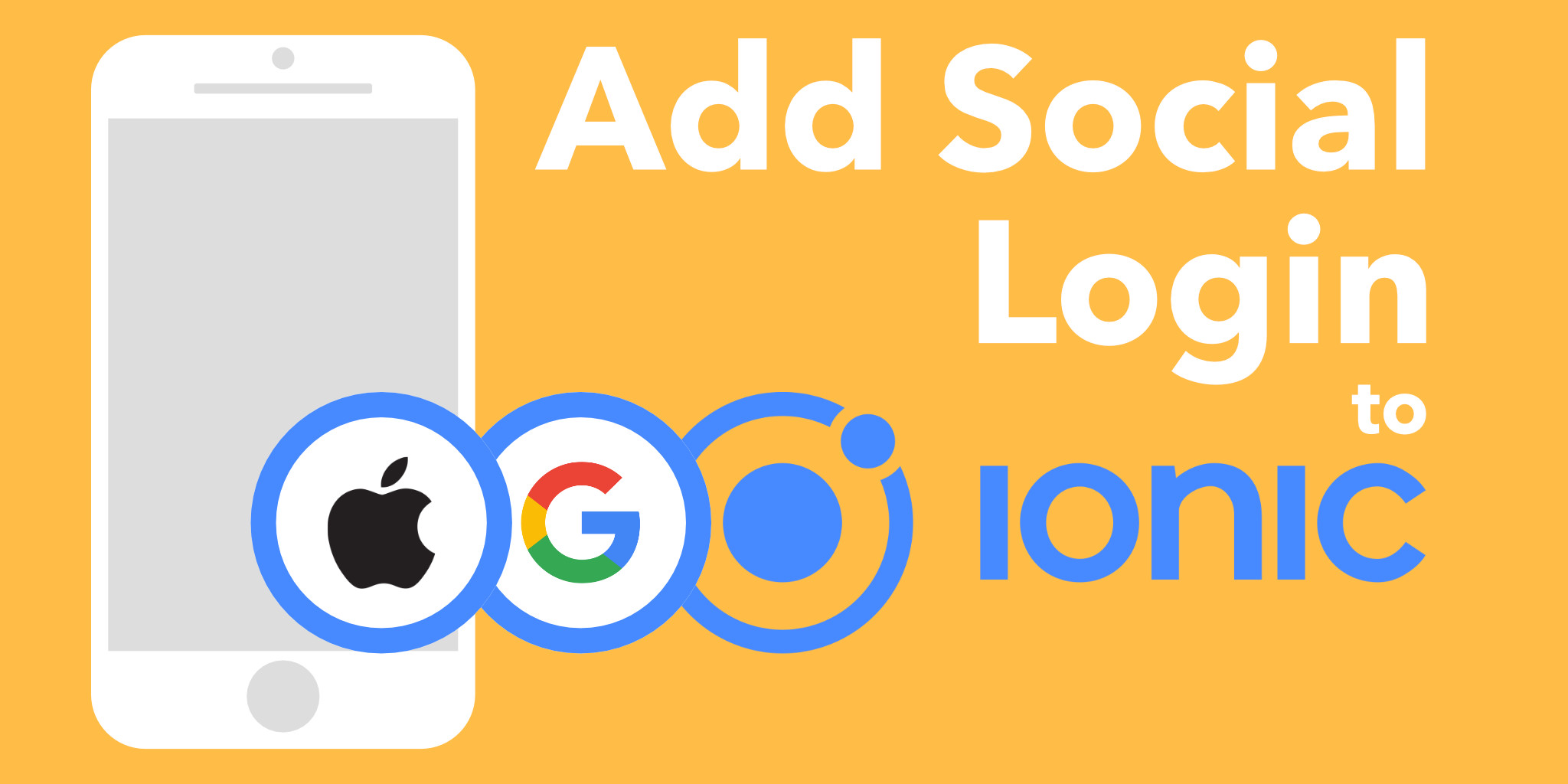
Apple announced a Sign in with Apple service at its WWDC developer conference in June 2019. If you’re familiar with social login with Google or Facebook, it’s very similar. Most of these identity services use OAuth and OpenID Connect (OIDC), and Apple’s implementation is similar. Today I’d like to show you how to develop a mobile application with Ionic, add OIDC authentication, retrieve the user’s information, and add social login (aka federated identity) with Apple...
Angular + Docker with a Big Hug from Spring Boot
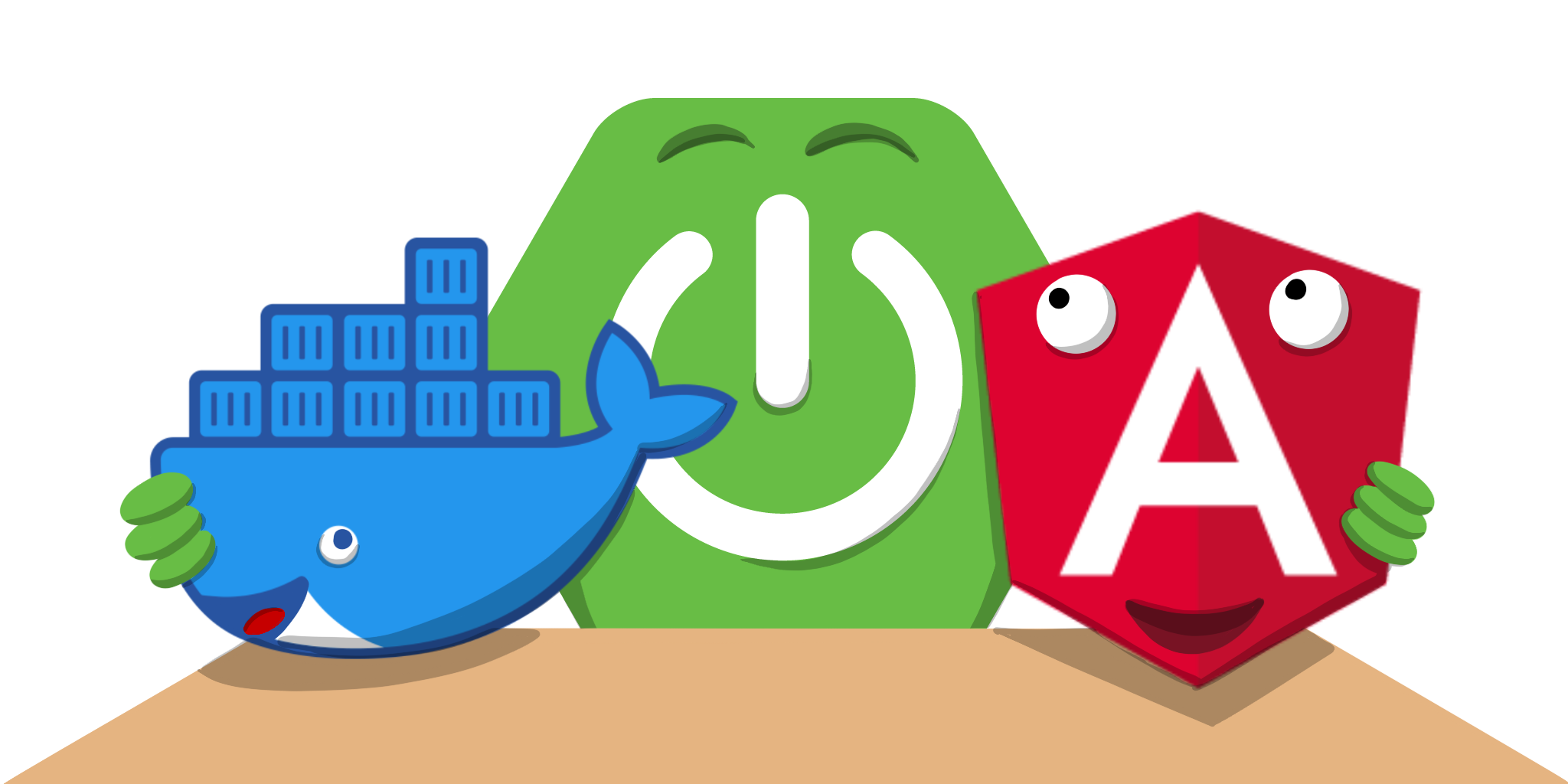
Building modern apps with Angular and Spring Boot is a common practice these days. In fact, if I use Google’s Keyword Planner to look up popular search terms, it’s one of the most popular combinations. The results in the list below are average monthly searches and not limited by location. This tutorial is the fourth and final in a series on Angular and Spring Boot in 2020. In the first one, I showed how to...
Angular Deployment with a Side of Spring Boot
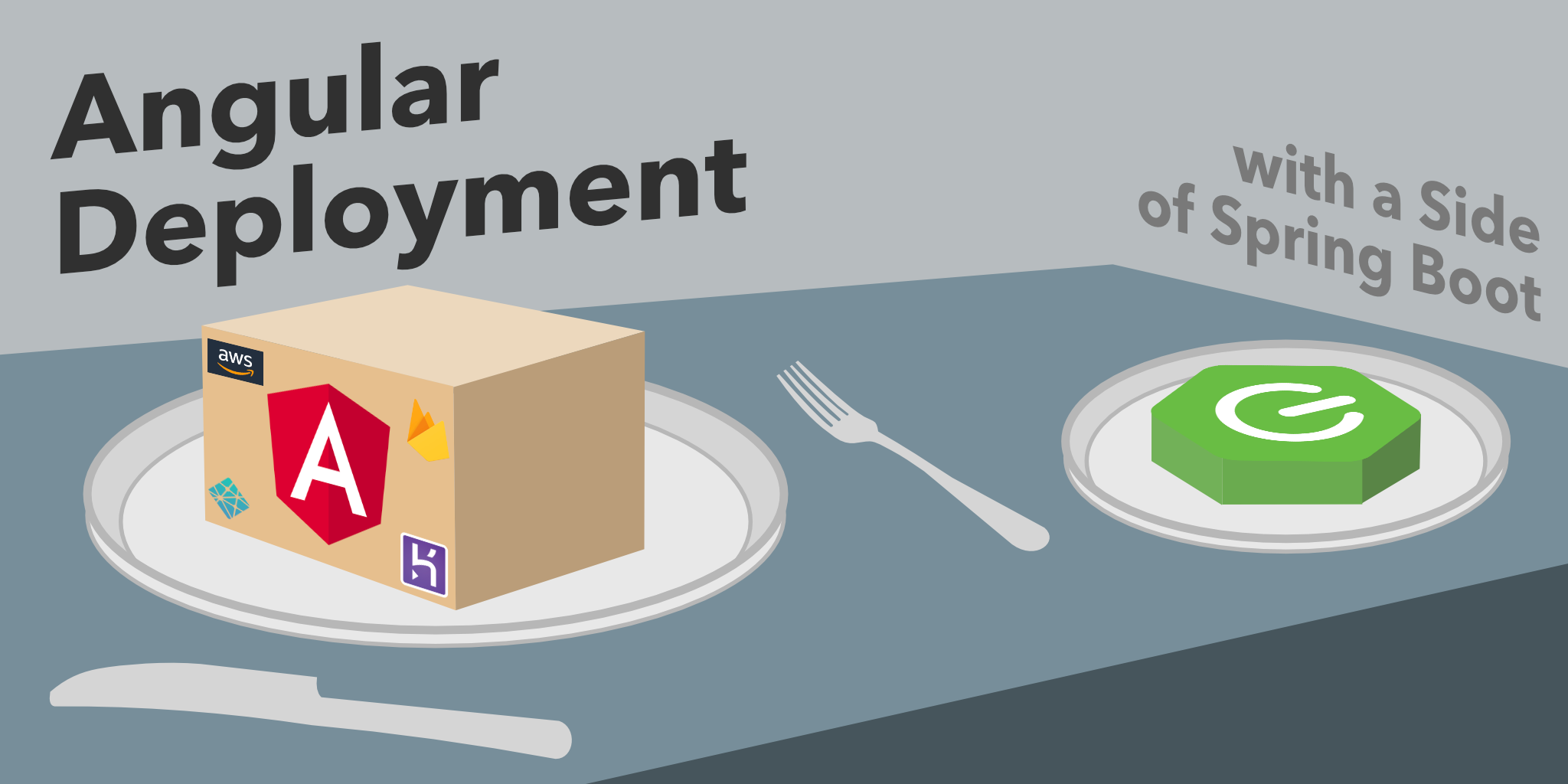
One of the more popular combinations of frontend and backend frameworks is Angular + Spring Boot. I’ve written several tutorials about how to combine the two—from keeping them as separate apps to combining them into a single artifact. But, what about deployment? Developers ask me from time-to-time, "What’s the best way to do Angular deployment?" In this tutorial, I’ll show you several options. I’ll start by showing you how to deploy a Spring Boot app...
Build Beautiful Angular Apps with Bootstrap
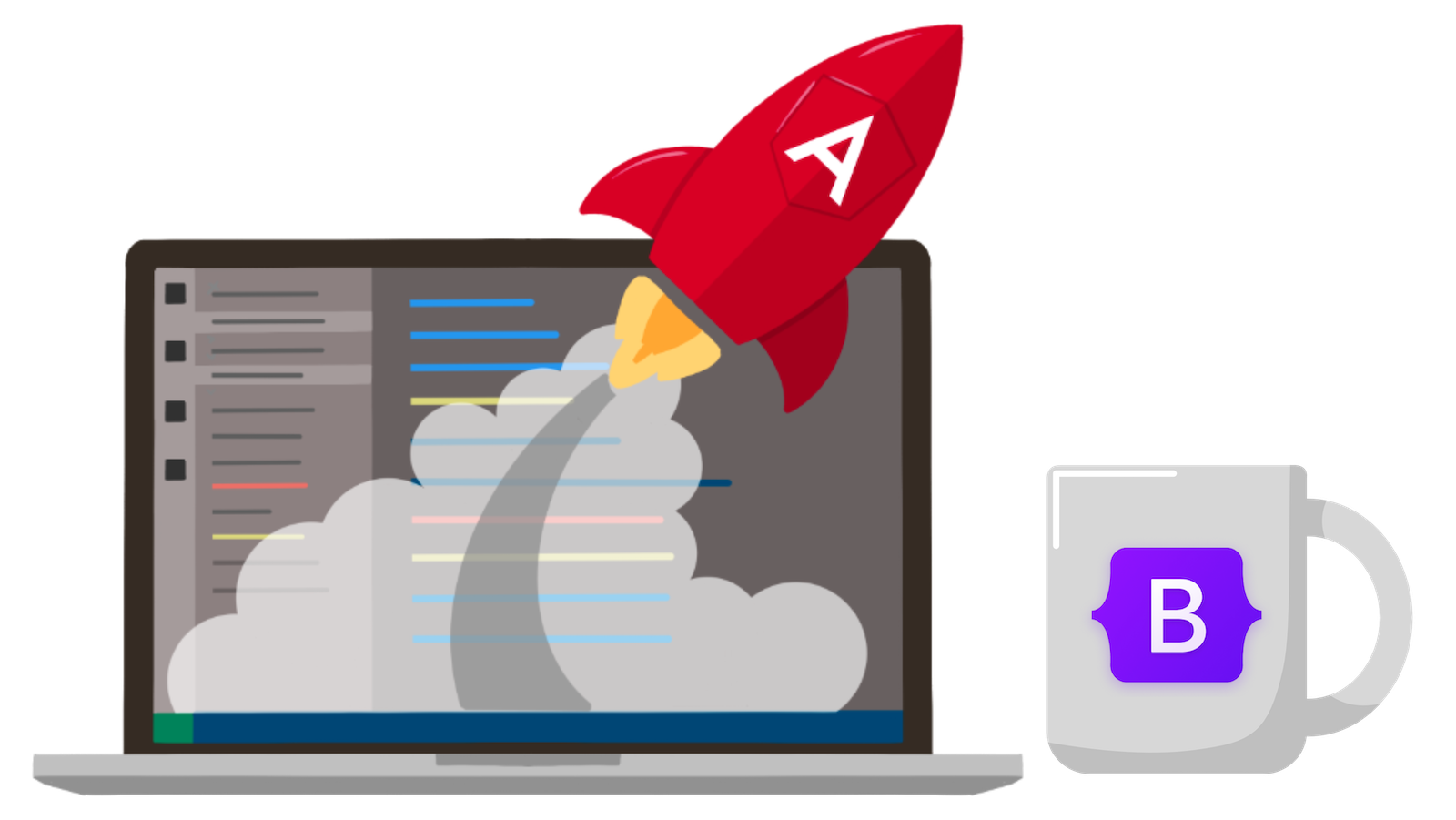
I’ve been a longtime fan of CSS frameworks since 2005. I led an open-source project called AppFuse at the time and wanted a way to provide themes for our users. We used Mike Stenhouse’s CSS Framework and held a design content to gather some themes we liked for our users. A couple of other CSS frameworks came along in the next few years, namely Blueprint in 2007 and Compass in 2008. However, no CSS frameworks...
What Is Angular Ivy and Why Is It Awesome?
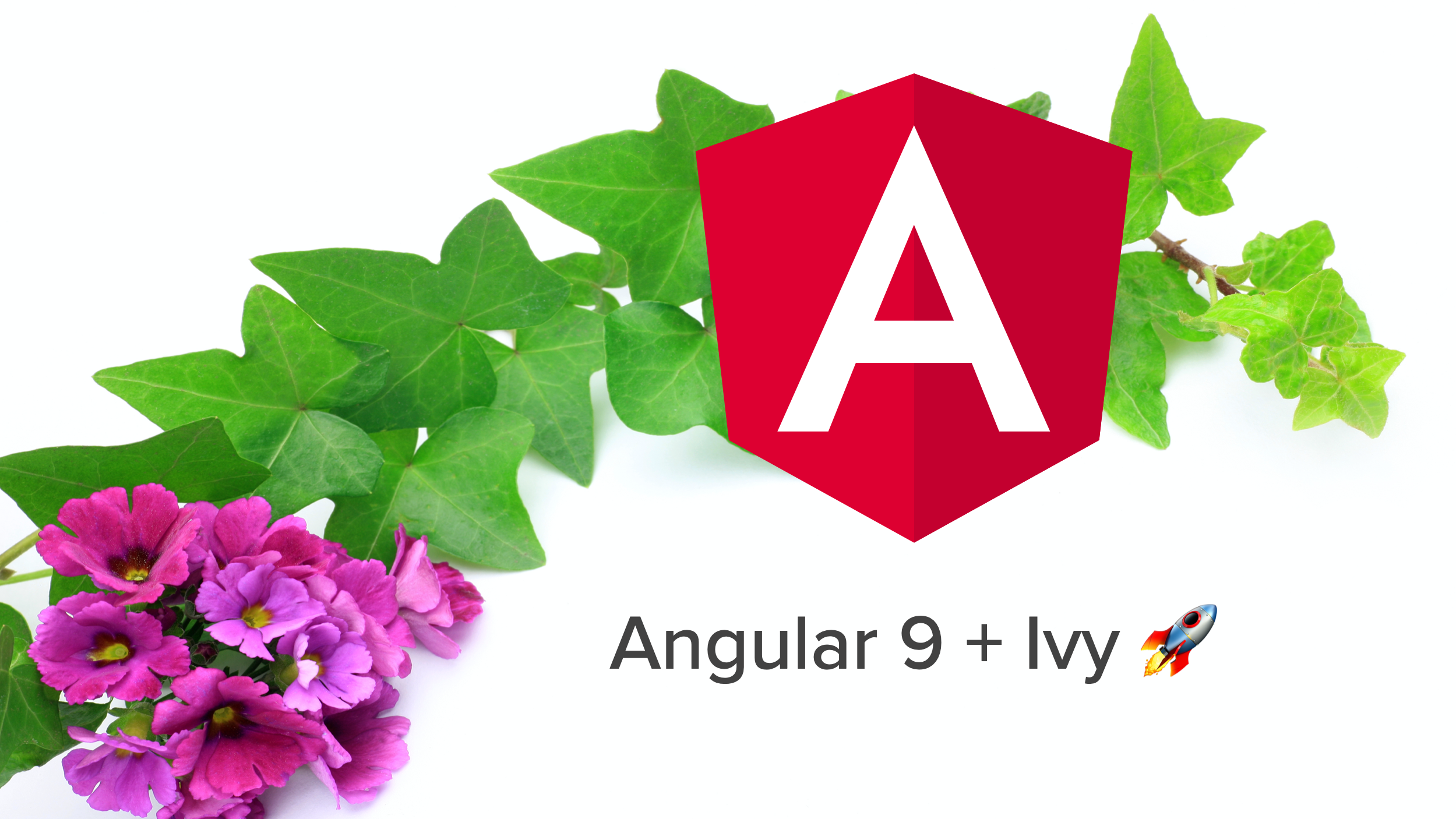
Over the last year or so, a new buzzword started floating around Angular forums and blogs. The word was Ivy. Ivy promises to make your application faster and smaller. But what exactly does this new technology do? Ivy is a complete rewrite of Angular’s rendering engine. In fact, it is the fourth rewrite of the engine and the third since Angular 2. But unlike rewrites two and three, which you might not have even noticed,...
Build a Beautiful App + Login with Angular Material
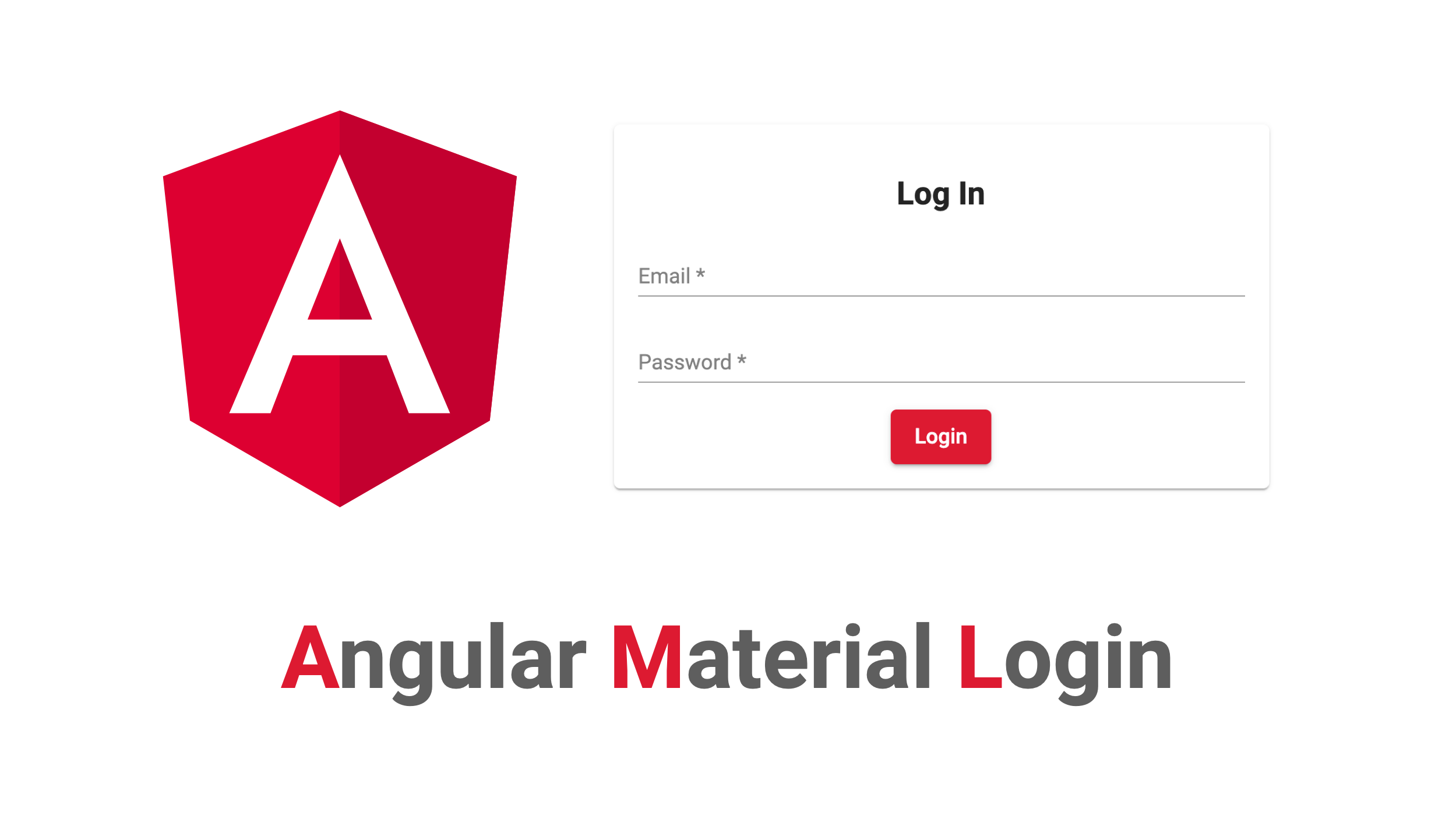
Usability is a key aspect to consider when creating a web application, and that means designing a clean, easy-to-understand user interface. Leveraging common design languages can help make that goal a reality with their recognizable components that many users will understand right away. However, unlike desktop apps where the operating system provides a set of uniform widgets, there has historically been no common design language to lean on for web app developers. That was until...
Build a CRUD App with Angular 9 and Spring Boot 2.2
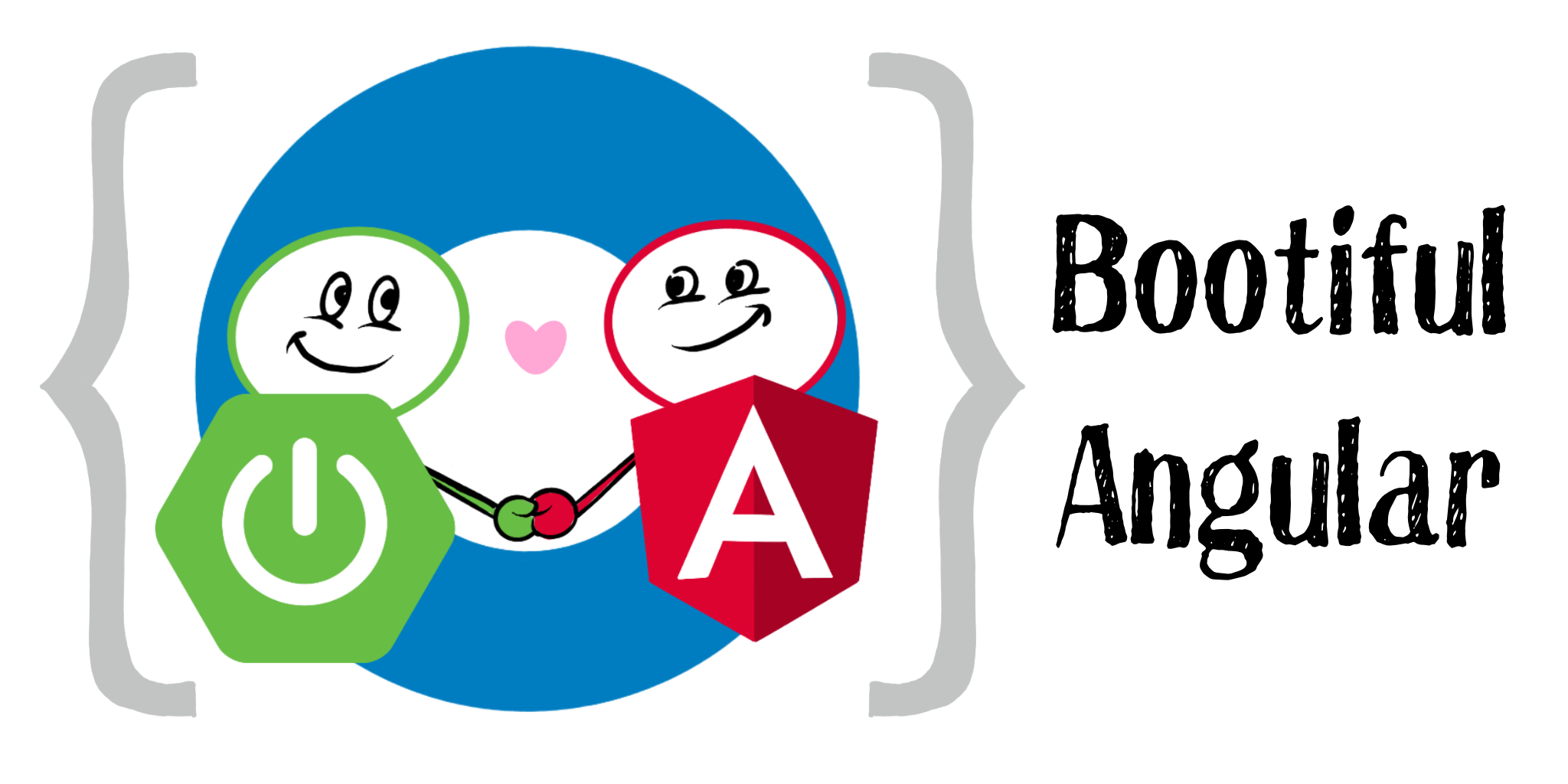
Angular is a web framework for building mobile and desktop applications. Its first version, AngularJS, was one of the first JavaScript MVC frameworks to dominate the web landscape. Developers loved it, and it rose to popularity in the early 2010s. AngularJS 1.0 was released on June 14, 2014. Angular 9 was recently released, giving Angular quite a successful run in the land of web frameworks. Spring Boot is one of the most popular frameworks for...
How to Customize Your Angular Build With Webpack
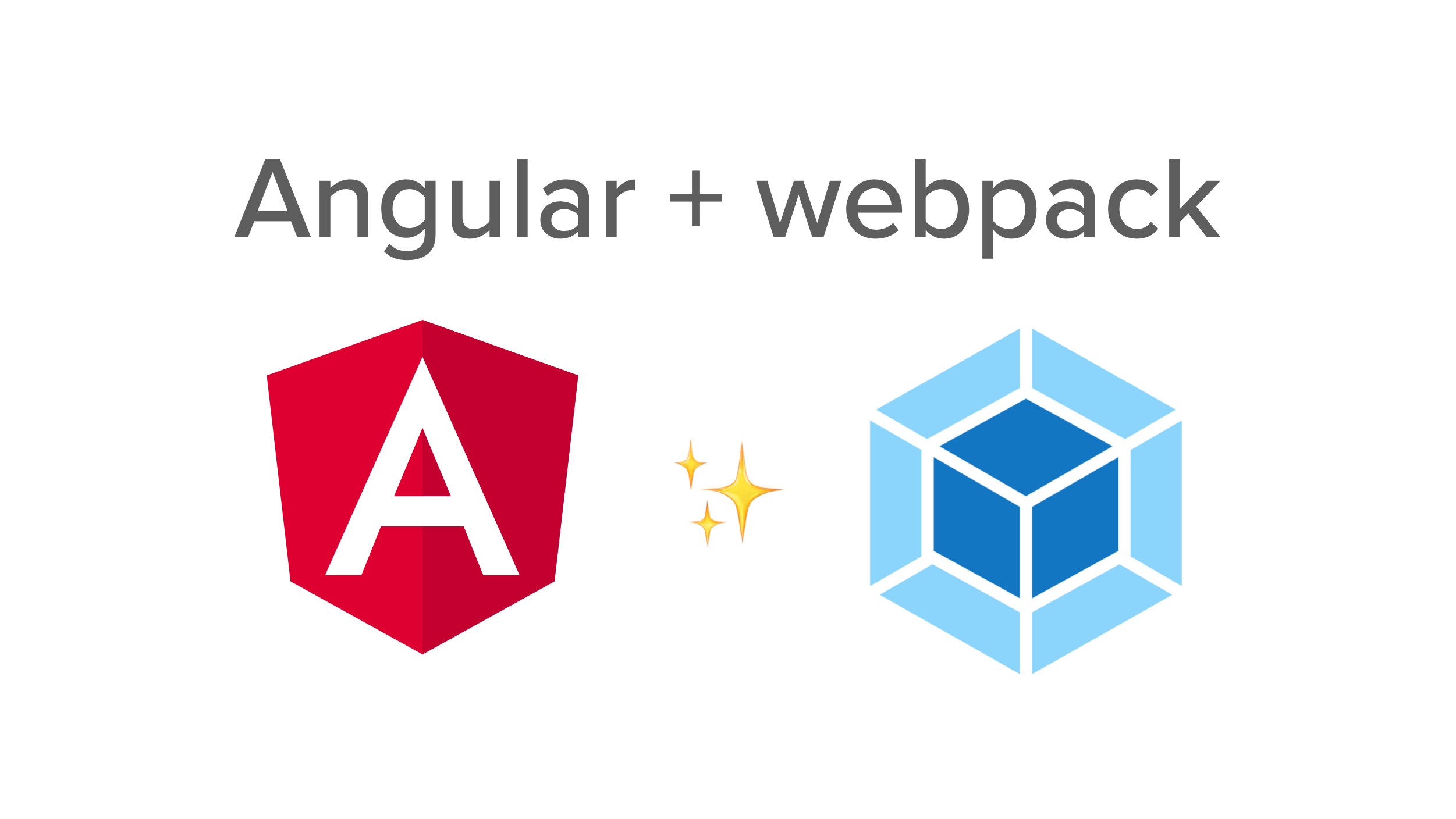
If you’re a frontend dev in the world today you’ve probably heard of (and possibly even used) webpack. The Angular build process uses webpack behind the scenes to transpile TypeScript to JavaScript, transform Sass files to CSS, and many other tasks. To understand the importance of this build tool, it helps to understand why it exists. Browsers have very limited support for JavaScript modules. In practice, any JavaScript application loaded into the browser should be...
How to Connect Angular and MongoDB to Build a Secure App
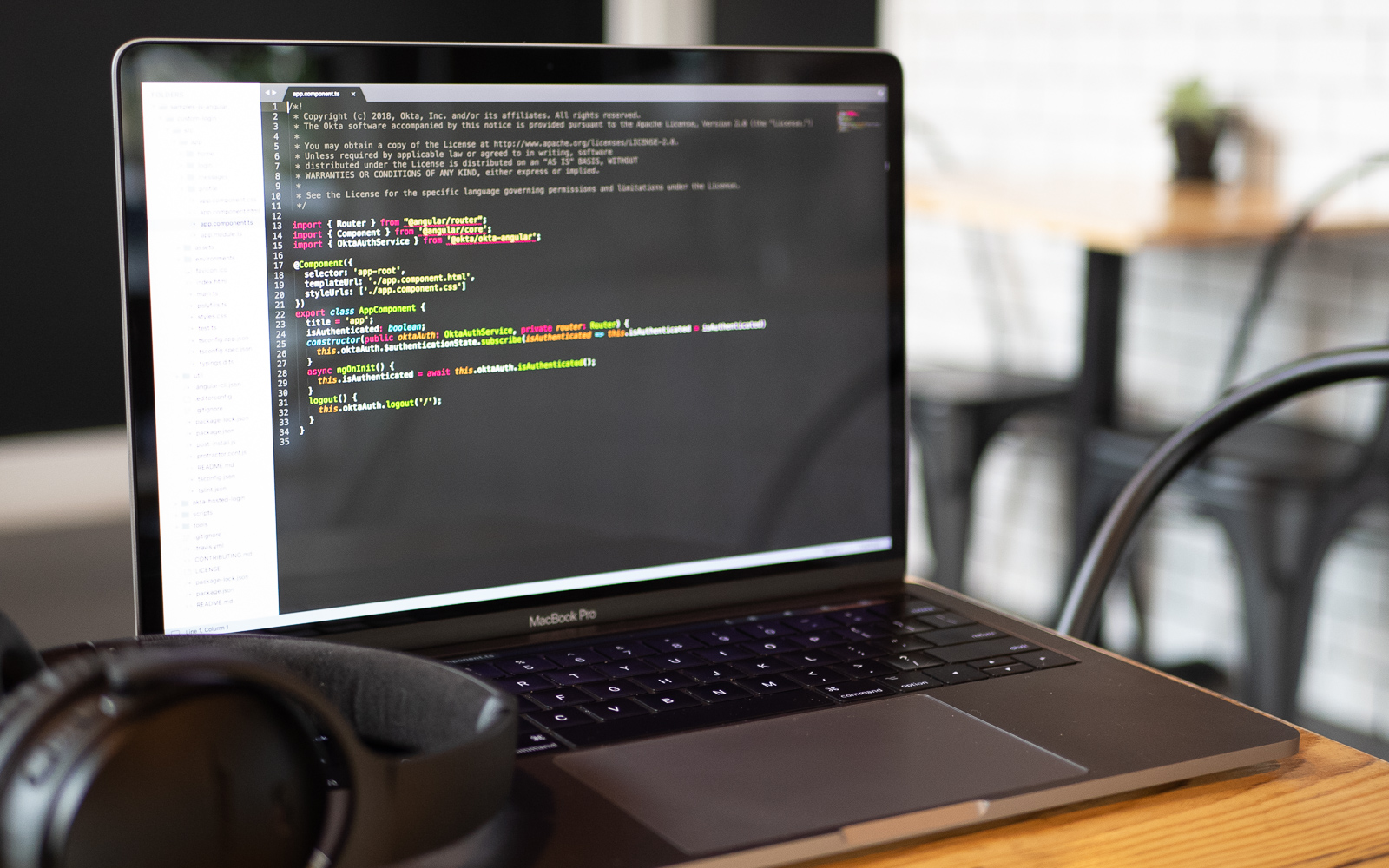
MongoDB is often the first suggestion when it comes time to select a NoSQL database. But what is a NoSQL database, and why would you want to use one in the first place? To answer this question, let’s step back and look at SQL databases and where they shine. SQL databases are a good choice if you have well-defined data that will not change much over time. They also allow you to define complex relationships...
How to Work with Angular and MySQL
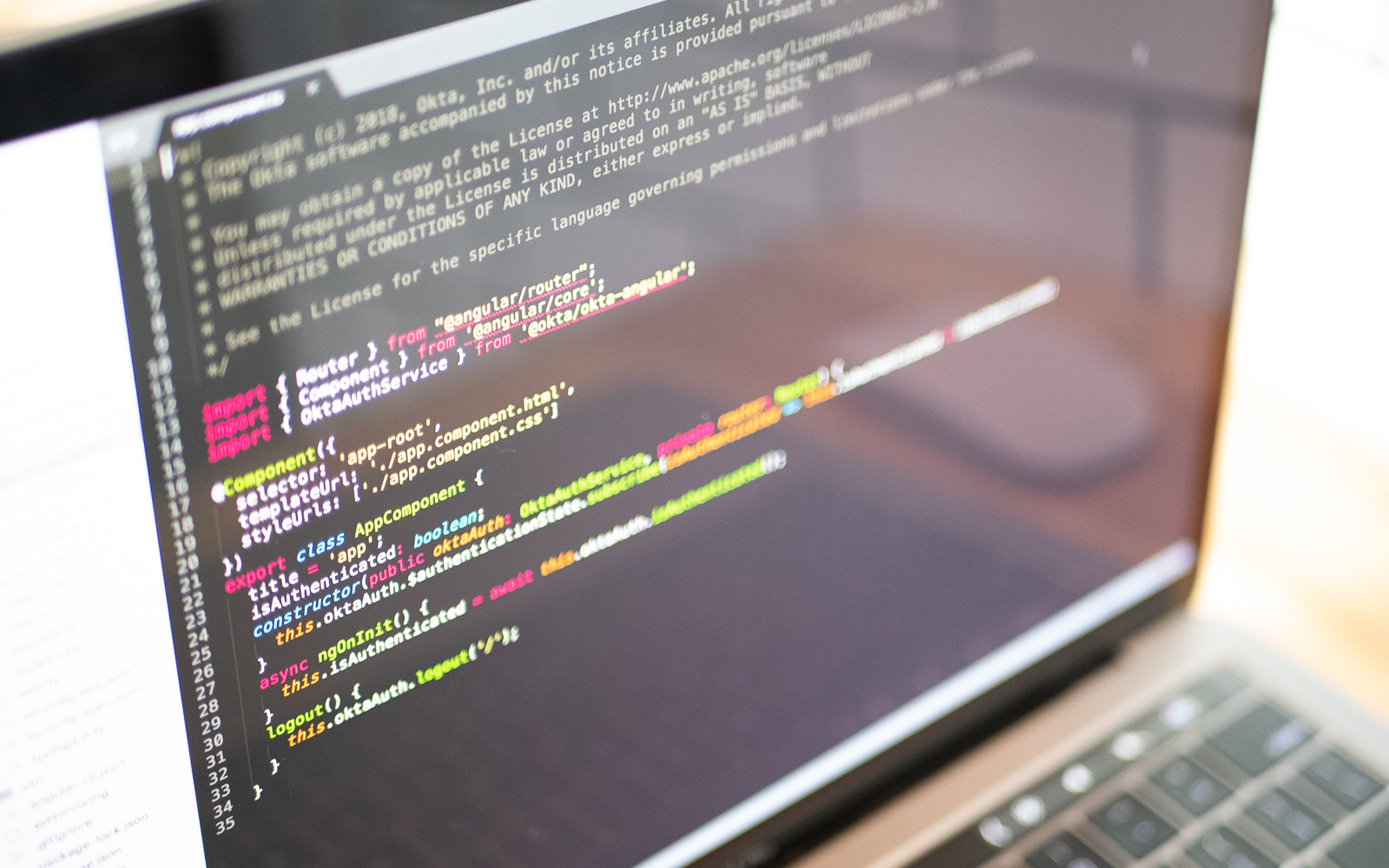
The MySQL database has been a reliable workhorse for web applications for many years. It is the M in the LAMP stack, and powers a huge number of web servers across the world. MySQL is also a relational database, with data stored in tables with strict data definitions. Rows in tables correspond to data entries and rows in one table can be referenced from another table through their index. Plenty of literature covers the fundamentals...
Build Mobile Apps with Angular, Ionic 4, and Spring Boot
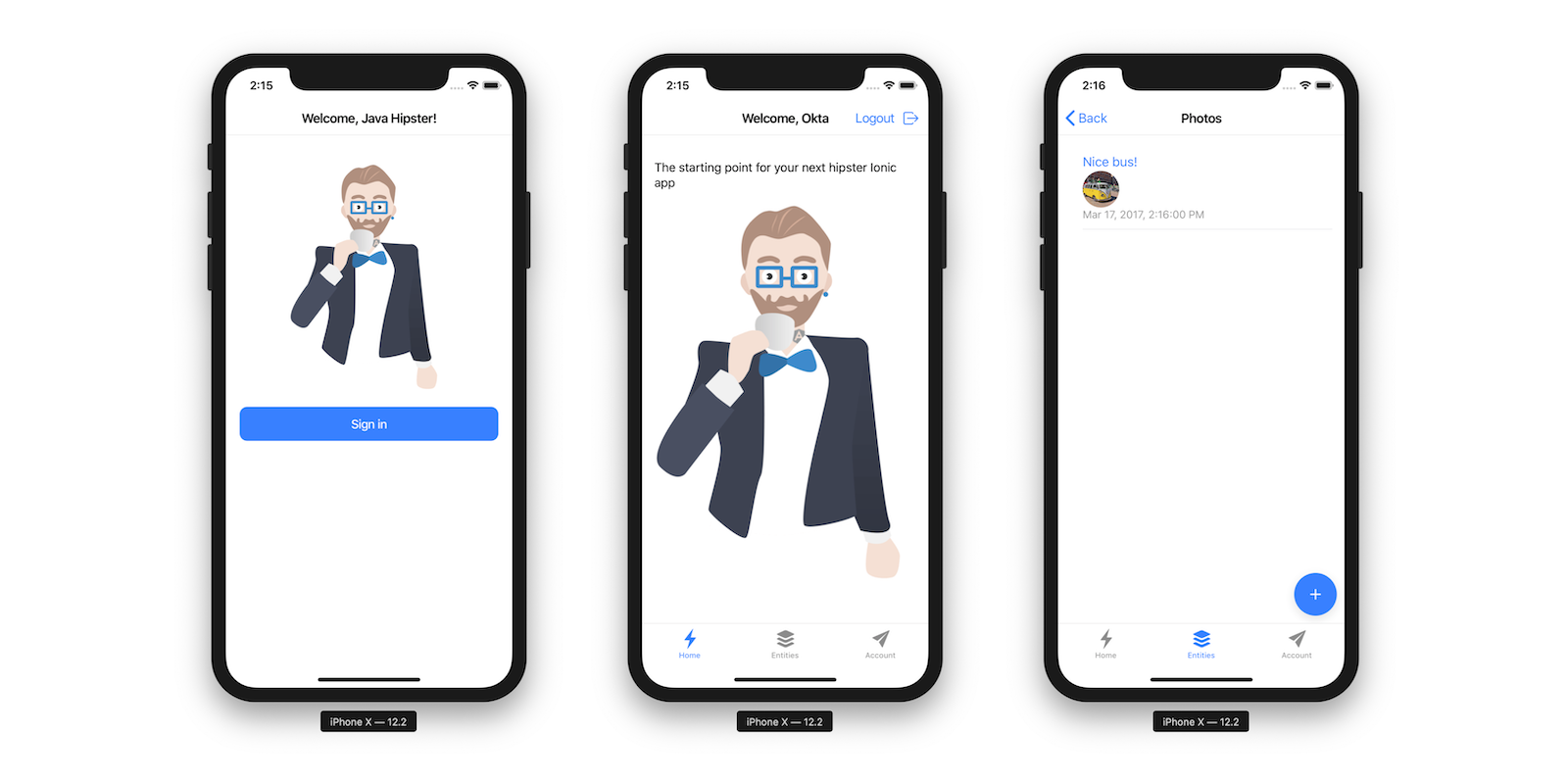
I’m a big fan of Ionic. I started using it several years ago when it was based on AngularJS. As a developer, I really liked it because I knew Angular. I found didn’t have to learn much more to be a productive developer with Ionic. What is Ionic? I’m glad you asked! Ionic is an open source project that allows you to build mobile apps using web tech. Technically, this is called a "hybrid" app...
Tutorial: User Login and Registration in Ionic 4
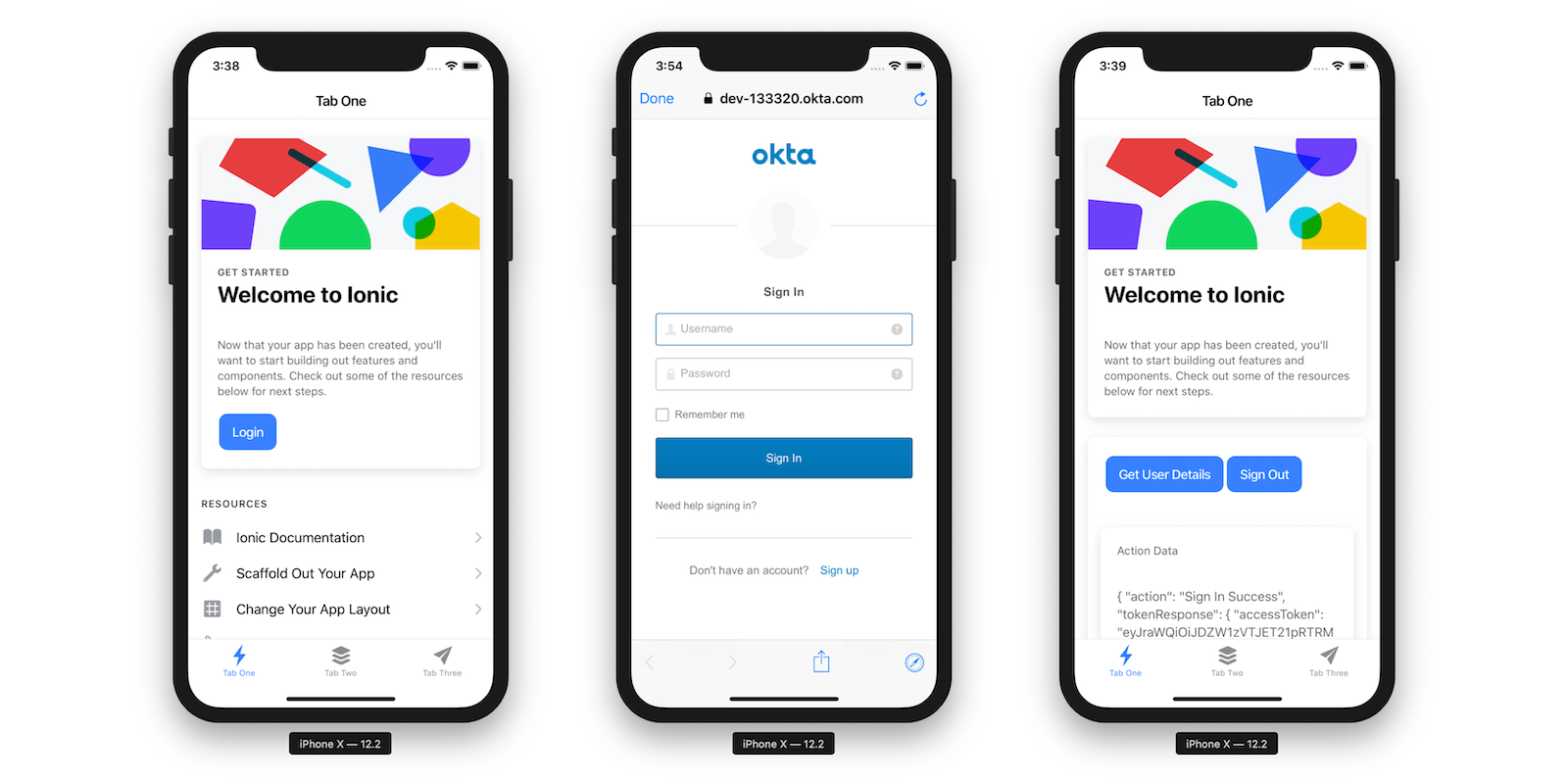
Ionic allows you to develop PWAs and hybrid mobile apps. PWAs are web applications that run in a browser and allow for offline capabilities via service workers. They can be installed on desktops and mobile devices, just like you install apps on your smartphone. Hybrid mobile apps are like native mobile apps, except they’re built using web technologies. Ionic 2 was based on AngularJS. Ionic 3 was based on Angular. Ionic 4 allows you to...
Angular Authentication with JWT
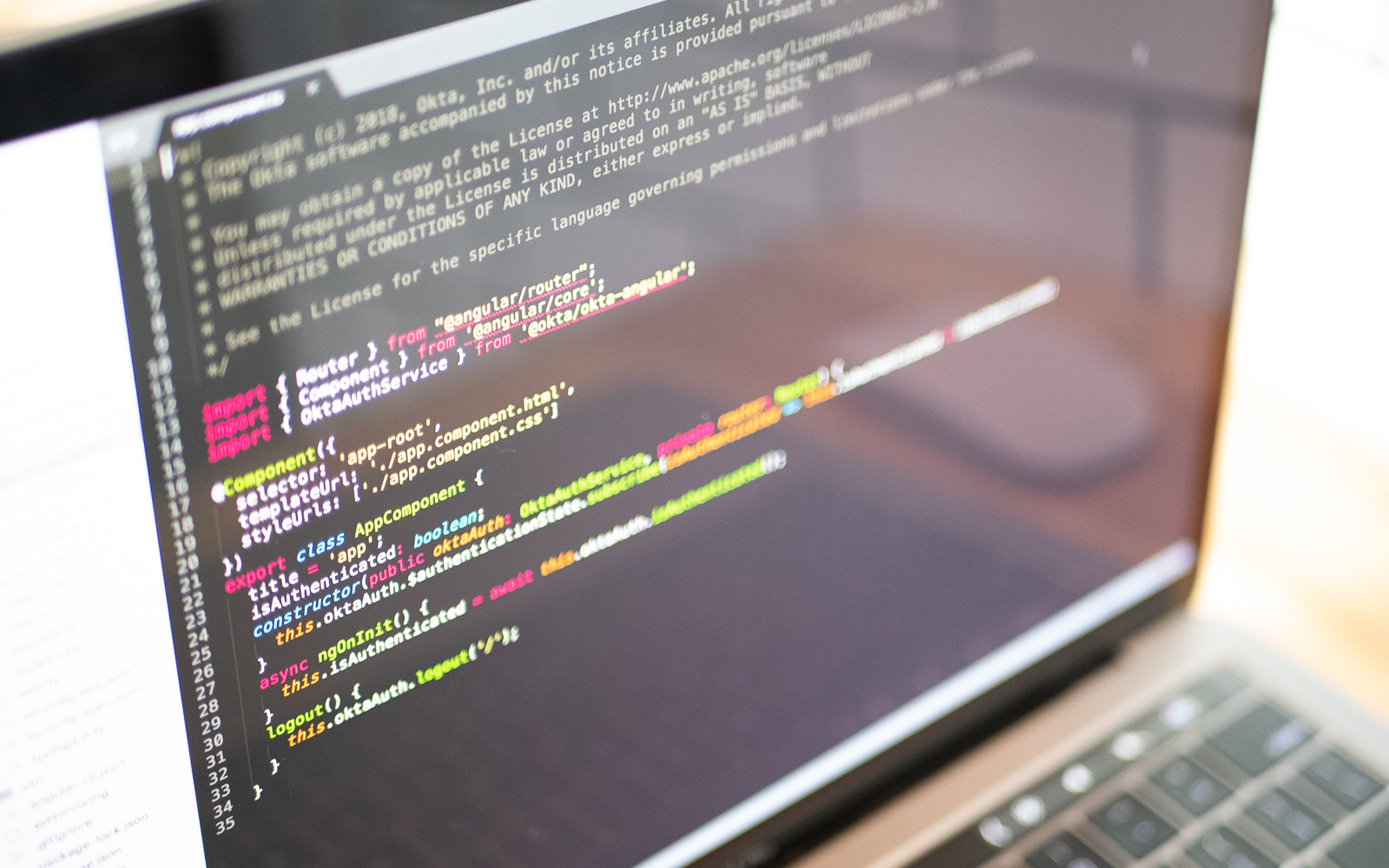
User registration and authentication are one of the features that almost no web application can do without. Authentication usually consists of a user entering using a username and a password and then being granted access to various resources or services. Authentication, by its very nature, relies on keeping the state of the user. This seems to contradict a fundamental property of HTTP, which is a stateless protocol. JSON Web Tokens (JWTs) provide one way to...
Angular 8 + Spring Boot 2.2: Build a CRUD App Today!
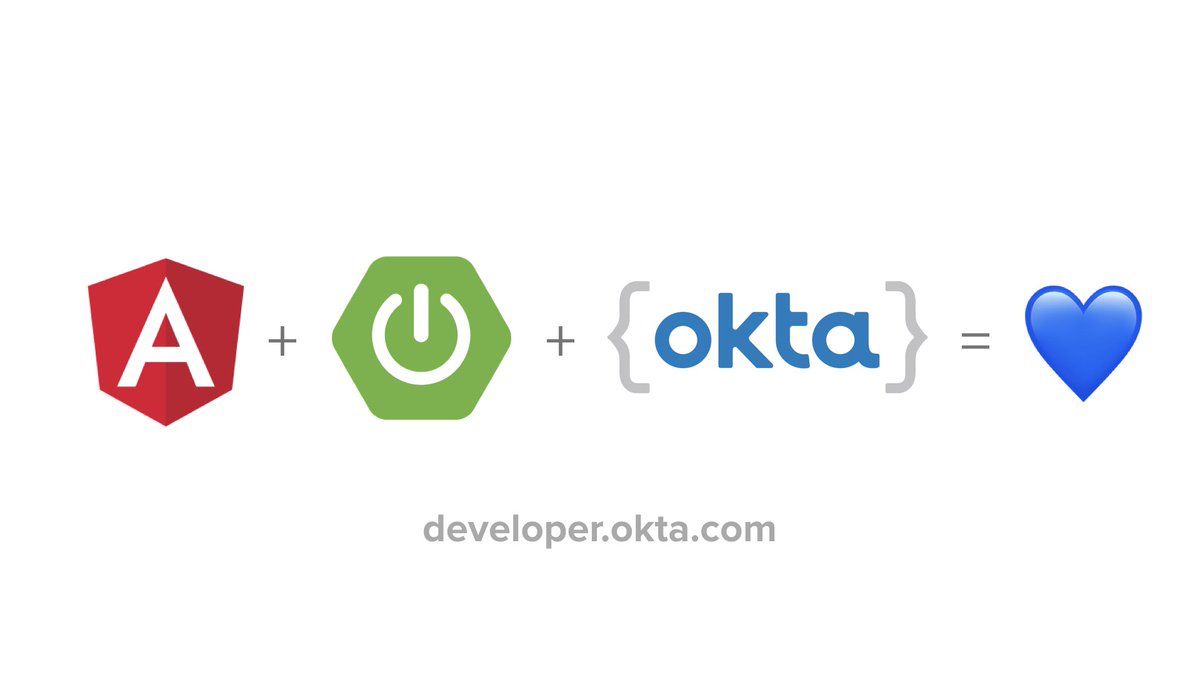
If you’ve been a Java developer for more than 15 years, you probably remember when there were a plethora of Java web frameworks. It started with Struts and WebWork. Then Tapestry, Wicket, and JSF came along and championed the idea of component-based frameworks. Spring MVC was released in 2004 (in the same month as Flex 1.0 and JSF 1.0) and became the de-facto standard in Java web frameworks over the next six years. Then along...
Angular MVC - A Primer
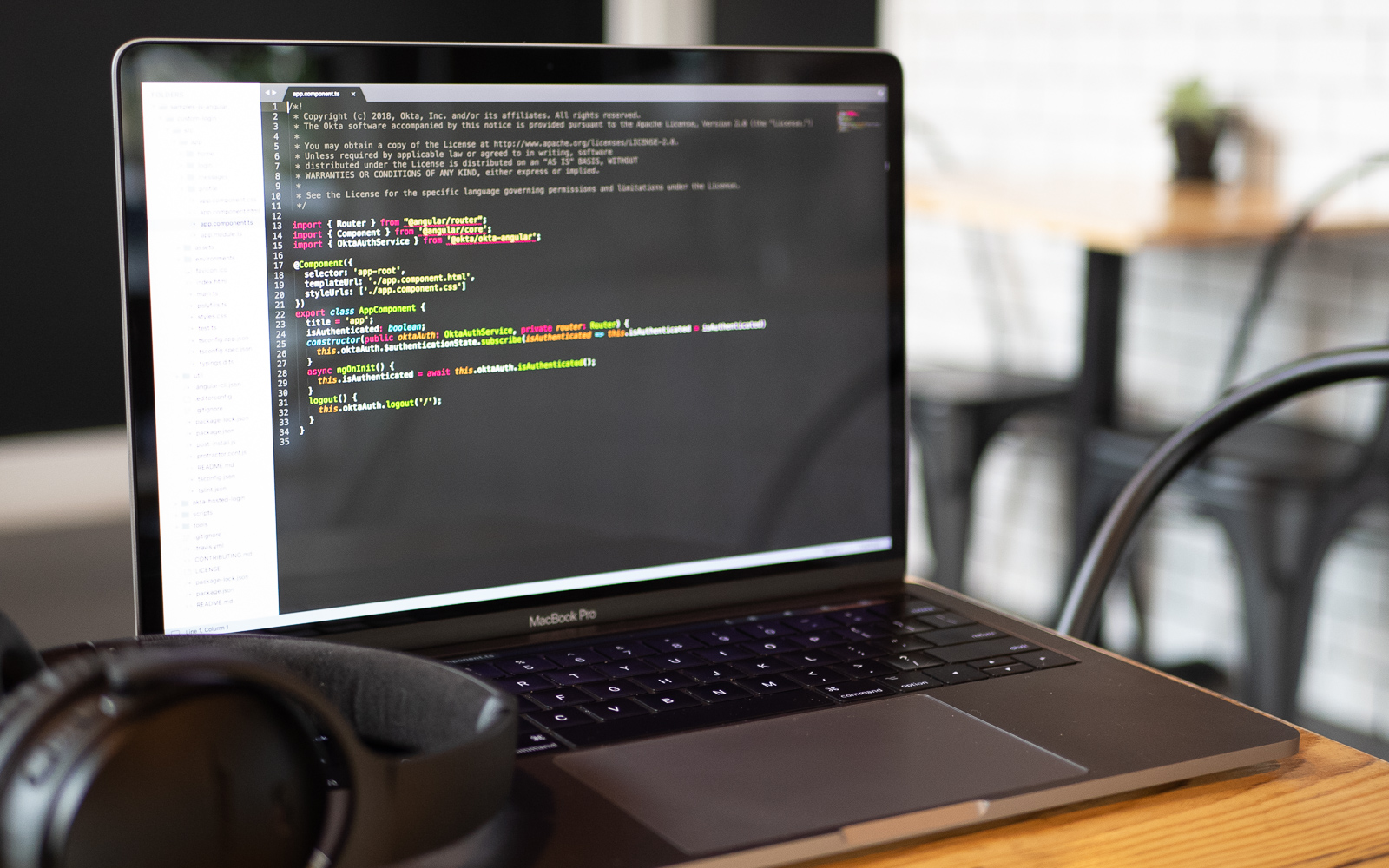
When designing software with a user interface, it is important to structure the code in a way that makes it easy to extend and maintain. Over time, there have been a few approaches in separating out responsibilities of the different components of an application. Although there is plenty of literature on these design patterns around, it can be very confusing for a beginner to understand the features of limitations of the different patterns and the...
Build a CRUD App with Python, Flask, and Angular
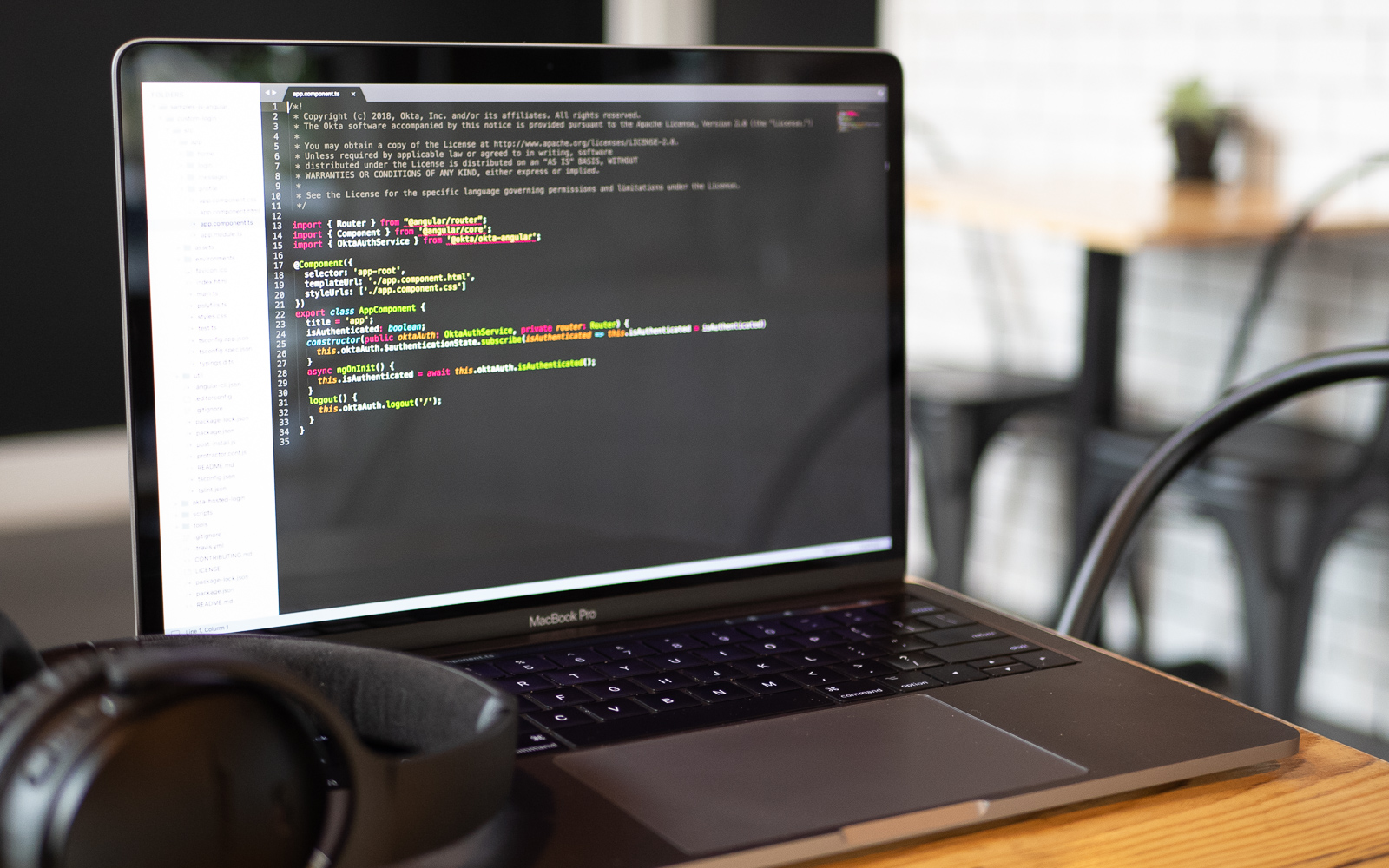
Developers all have their favorite GitHub repositories. They have software projects that they love and watch closely for the latest changes. In this tutorial, you’ll create a simple CRUD application to save and to display your favorite GitHub open source projects. You will use Angular to implement the user interface features and Python for the backend. These days it is not uncommon to have an API that is responsible not only for persisting data to...
Build a Desktop Application with Angular and Electron
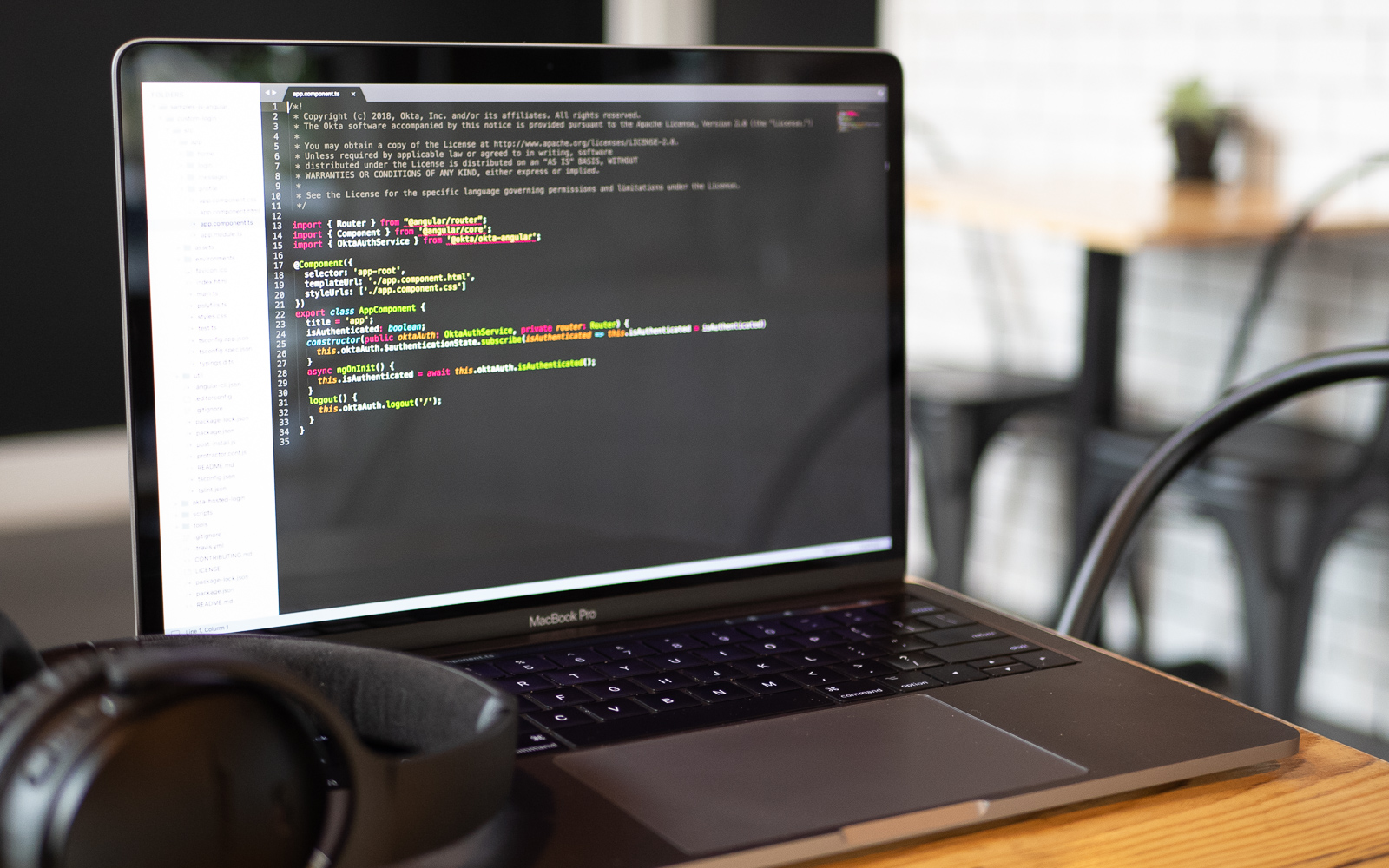
So, you have been learning all about web technologies including JavaScript, HTML, and CSS. The advantage of web technologies is, of course, that the same software can be used on many different platforms. But this advantage comes with a number of problems. Web applications have to be run inside a browser and the interoperability with the operating system is limited. Direct access to features of the operating system is usually the domain for desktop applications....
Build a CRUD App with Angular and Firebase
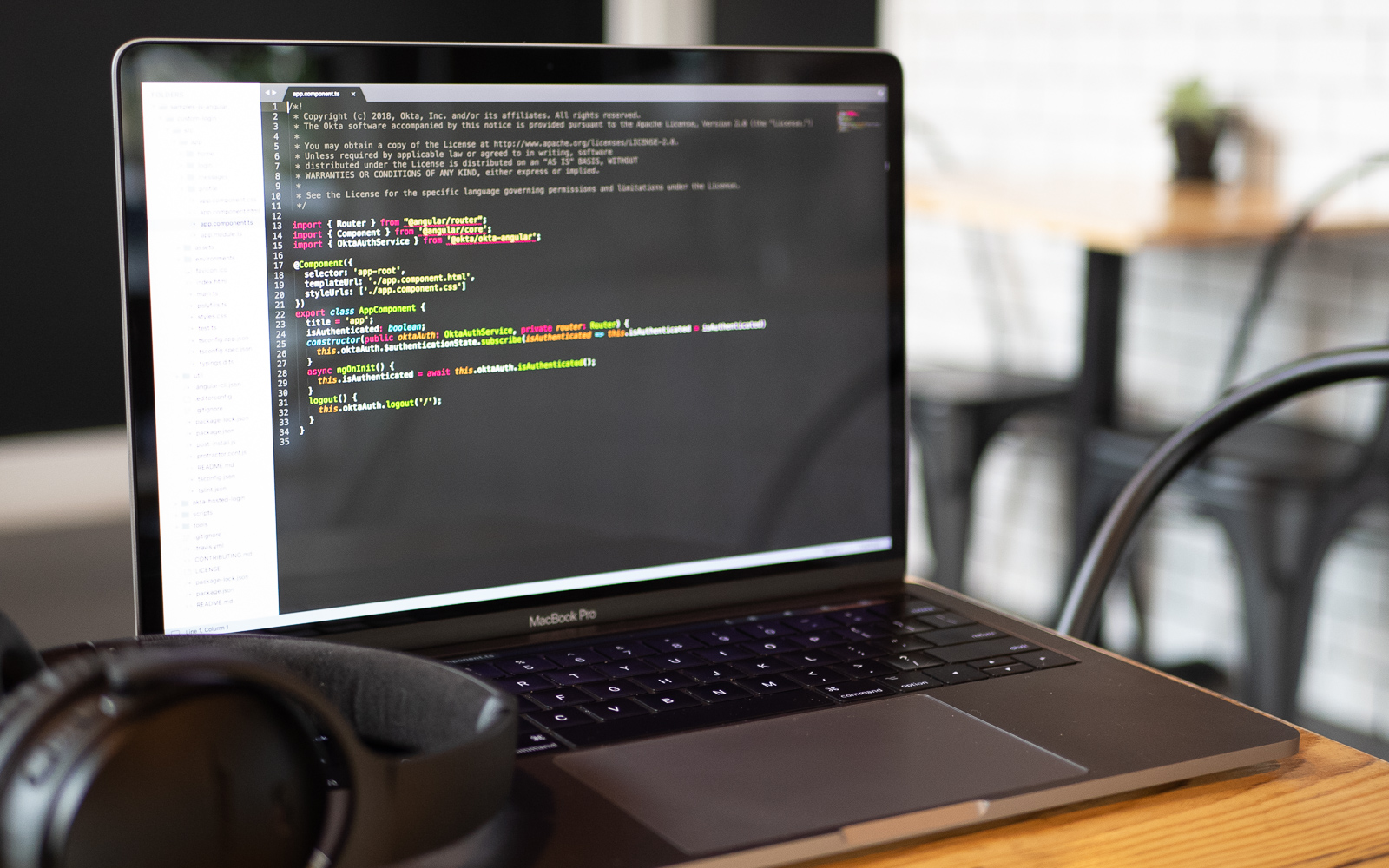
Storage as a Service (SaaS) is becoming ever more popular with many businesses. The advantages are clear. Instead of maintaining your own backend server you can outsource the service to a different provider. This can result in a significant increase in productivity, as well as a reduction in development and maintenance costs. In addition, the worry about server security is offloaded to the storage provider. SaaS is an option whenever the server part of your...
Use Angular Schematics to Simplify Your Life
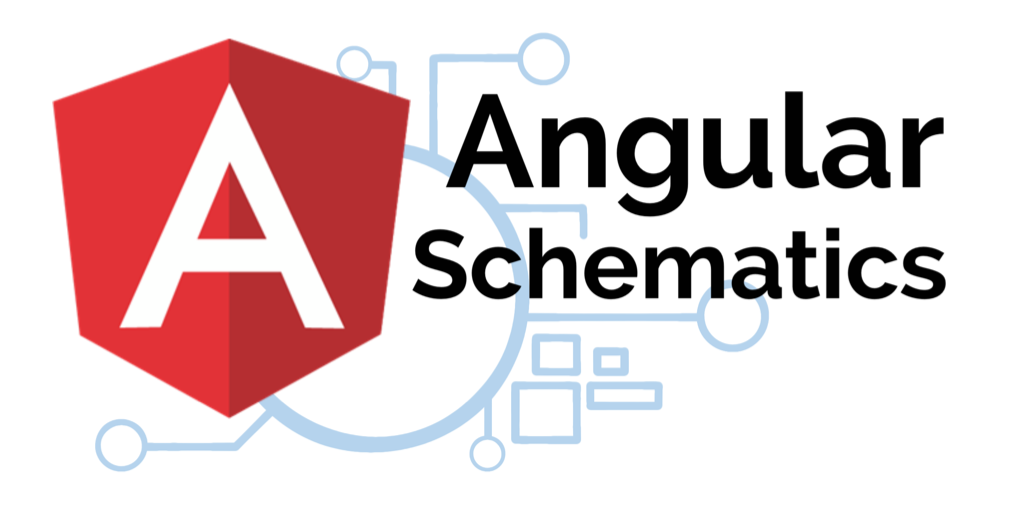
When I first started to learn Angular (it was called Angular 2 at the time), I was appalled by the number of files you needed to create to get a basic "Hello, World" example working. As Angular matured, a lot of this pain went away thanks to Angular CLI. Angular CLI is a command-line tool that generates a basic Angular project for you. In its 7.0 release, it started asking you questions about your application....
Build Secure Login for Your Angular App
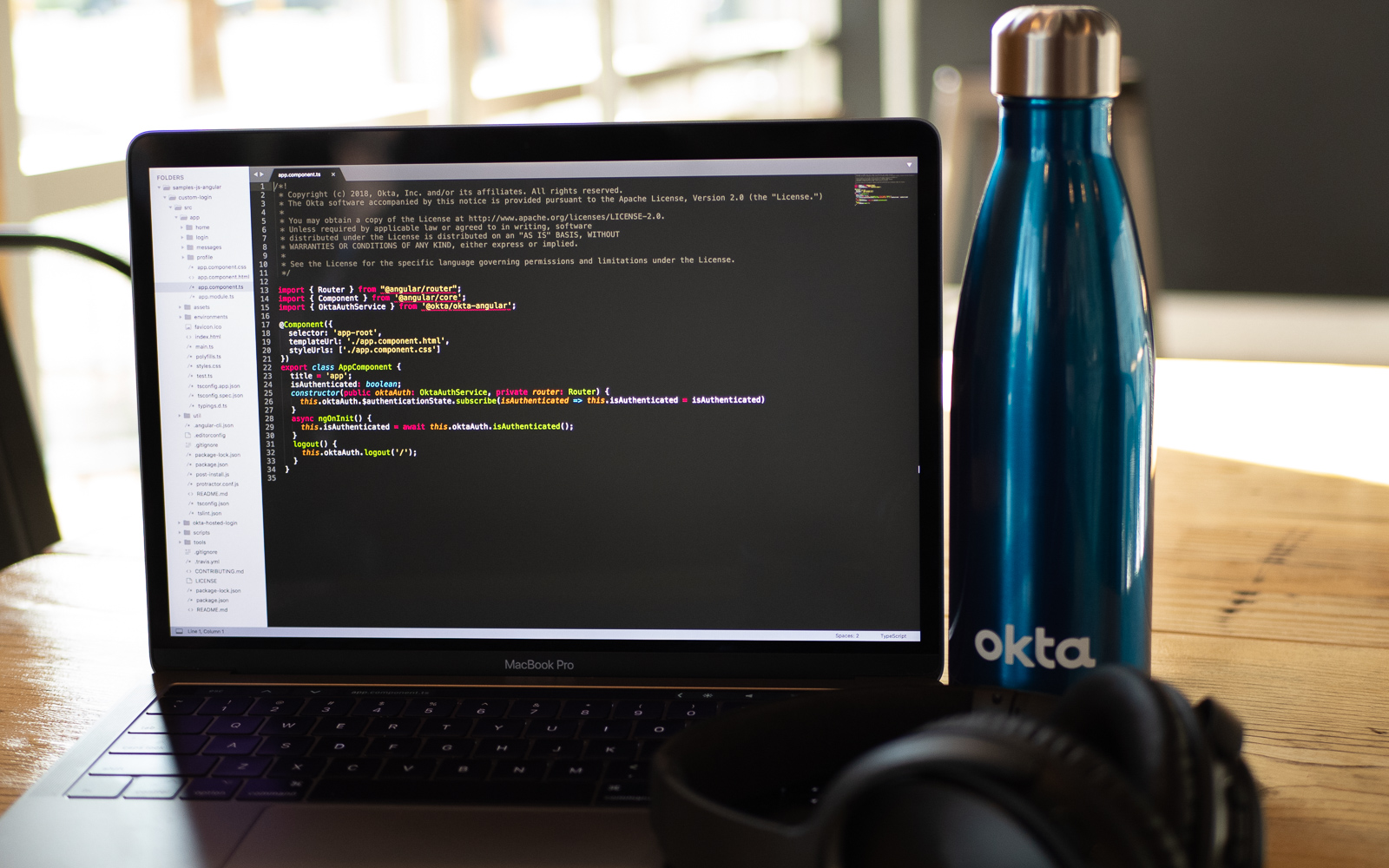
Single page applications (SPAs) are becoming more and more popular. Their appeal is obvious. Fast loading times gives users the feeling of responsiveness even over slow networks. At some point, a developer of a SPA has to think about authentication and authorization. But what do these two terms actually mean? Authentication deals with ensuring that a user truly is who they claim to be. This usually involves a login page in which the user provides...
Create Login and Registration in Your ASP.NET Core MVC App
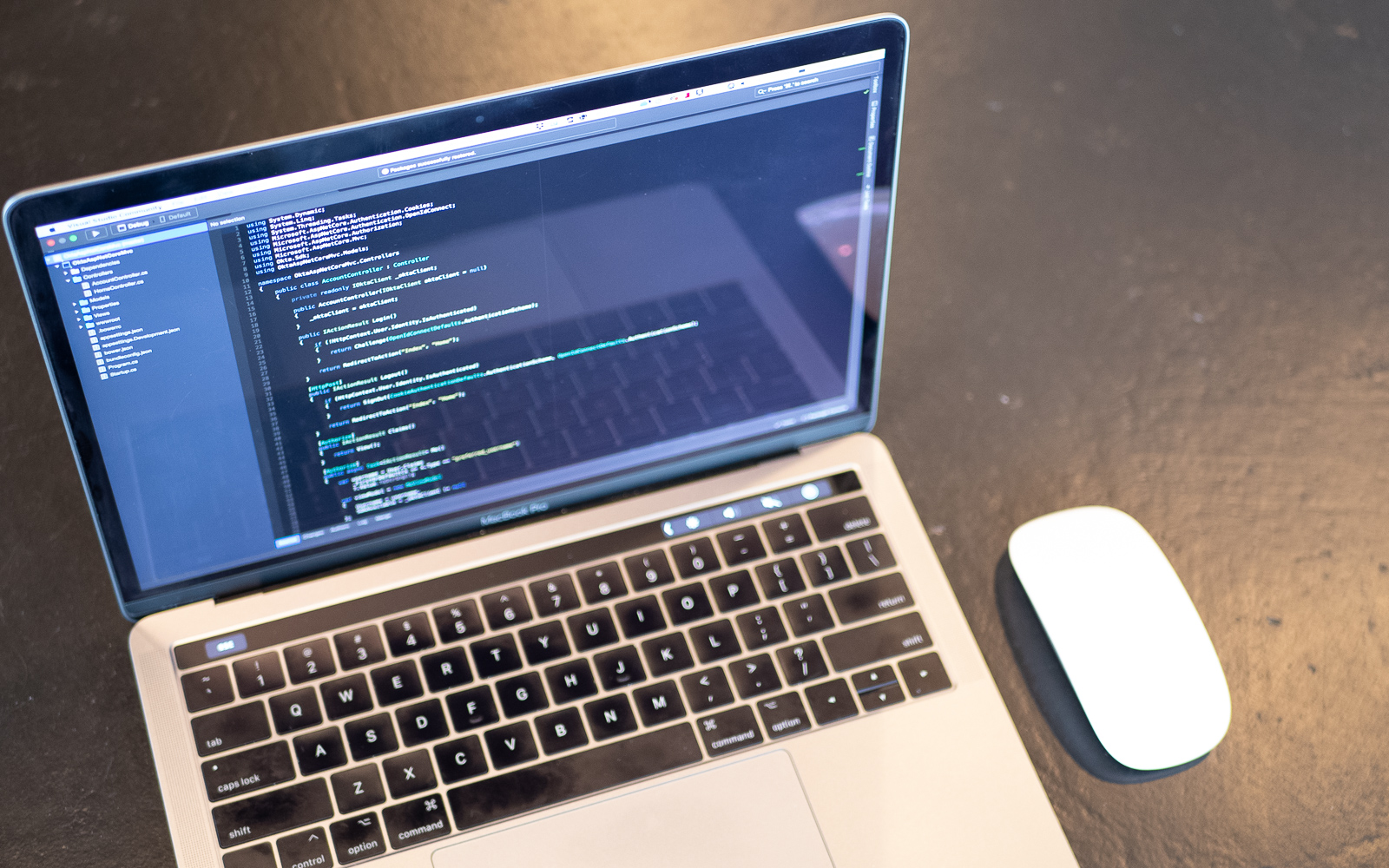
User authentication and authorization are common features in web applications, but building these mechanics has the potential to take a lot of time. Doing so requires setting up persistent storage for user information (in some type of database) and paying keen attention to potential security issues around sensitive operations like hashing passwords, password reset workflows, etc. - weeks of development time begin to add up before we ever get to the functionality that delivers value...
Build Your First PWA with Angular
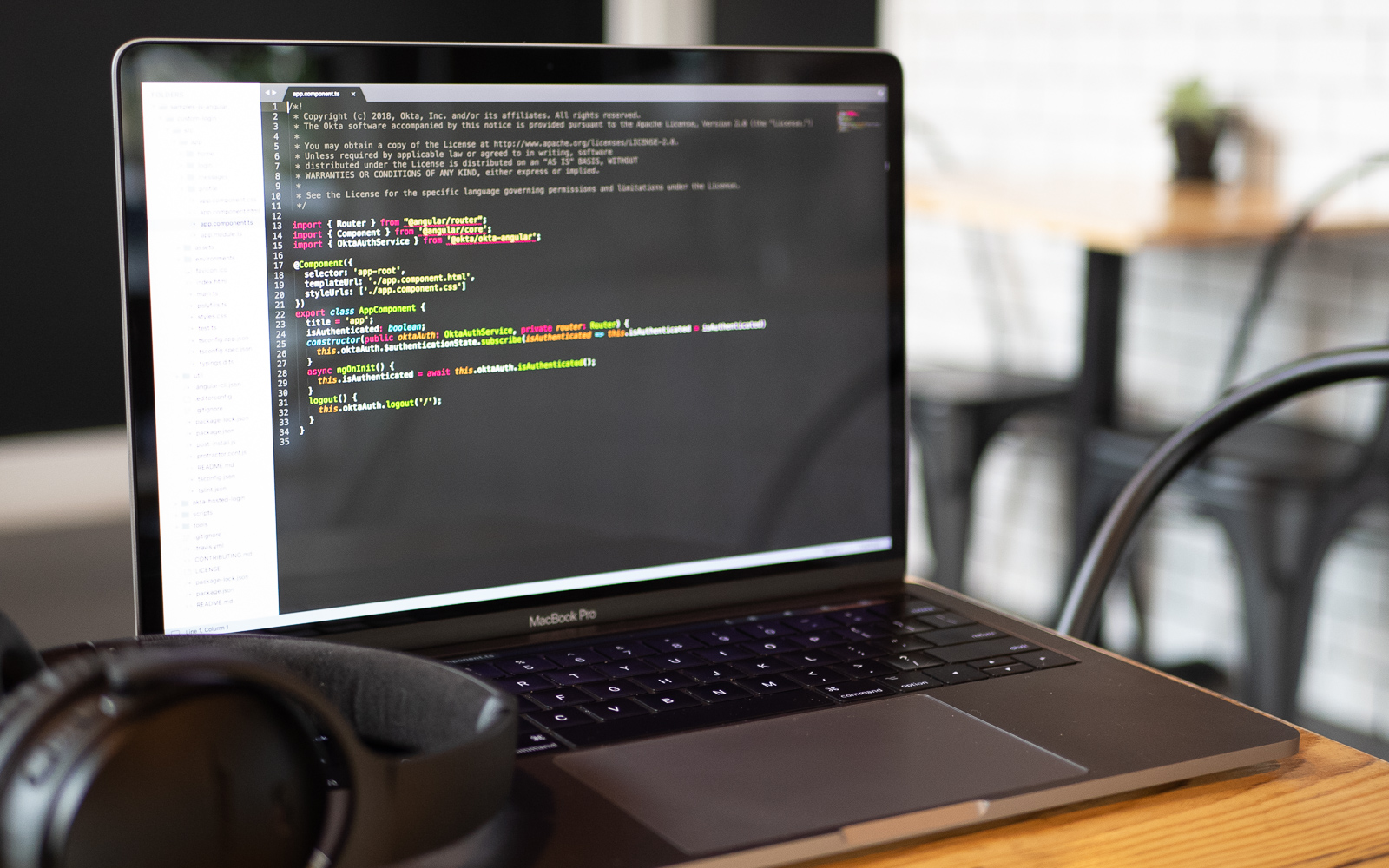
During the last two years, everybody started talking about Progressive Web Applications, or PWAs for short. But what is this new type of application, and how can it make your life as an Angular developer better? To understand what PWAs are all about, and how you can build them in Angular, let’s consider the following scenario. You are out and about in an area with little or no network reception. You are using a cool...
Build a Basic Website with ASP.NET MVC and Angular
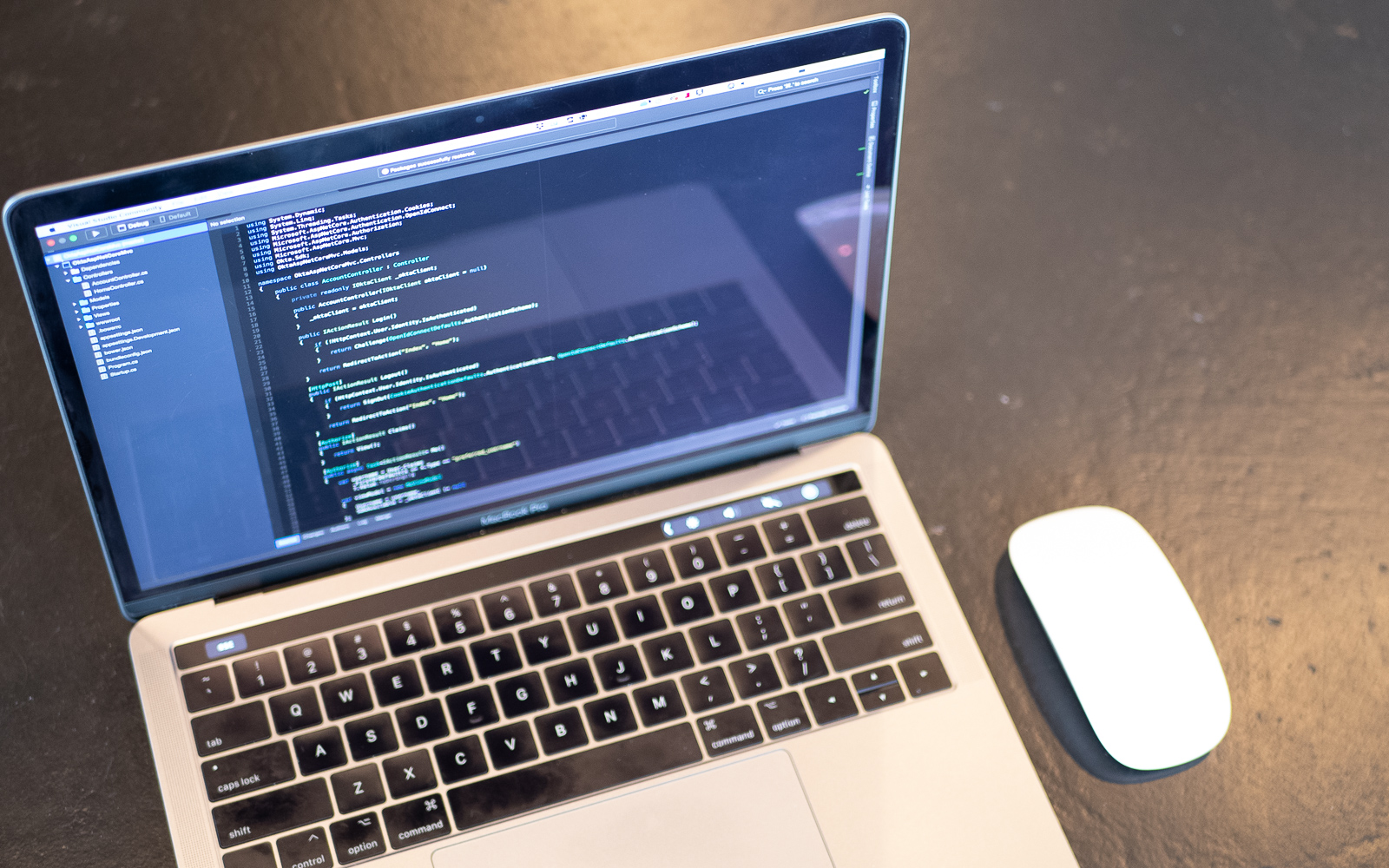
ASP.NET has been around for a long time. When Microsoft introduced ASP.NET MVC, it changed the way many developers approach their codebase. There is an excellent separation of concerns, a TDD friendly framework, and easy integration with JavaScript while maintaining full control over rendered HTML. On the client side, a lot of .NET developers prefer Angular because it comes with TypeScript and it’s a much closer language to C# than plain JavaScript. Angular is an...
Angular 7: What's New and Noteworthy + OIDC Goodness
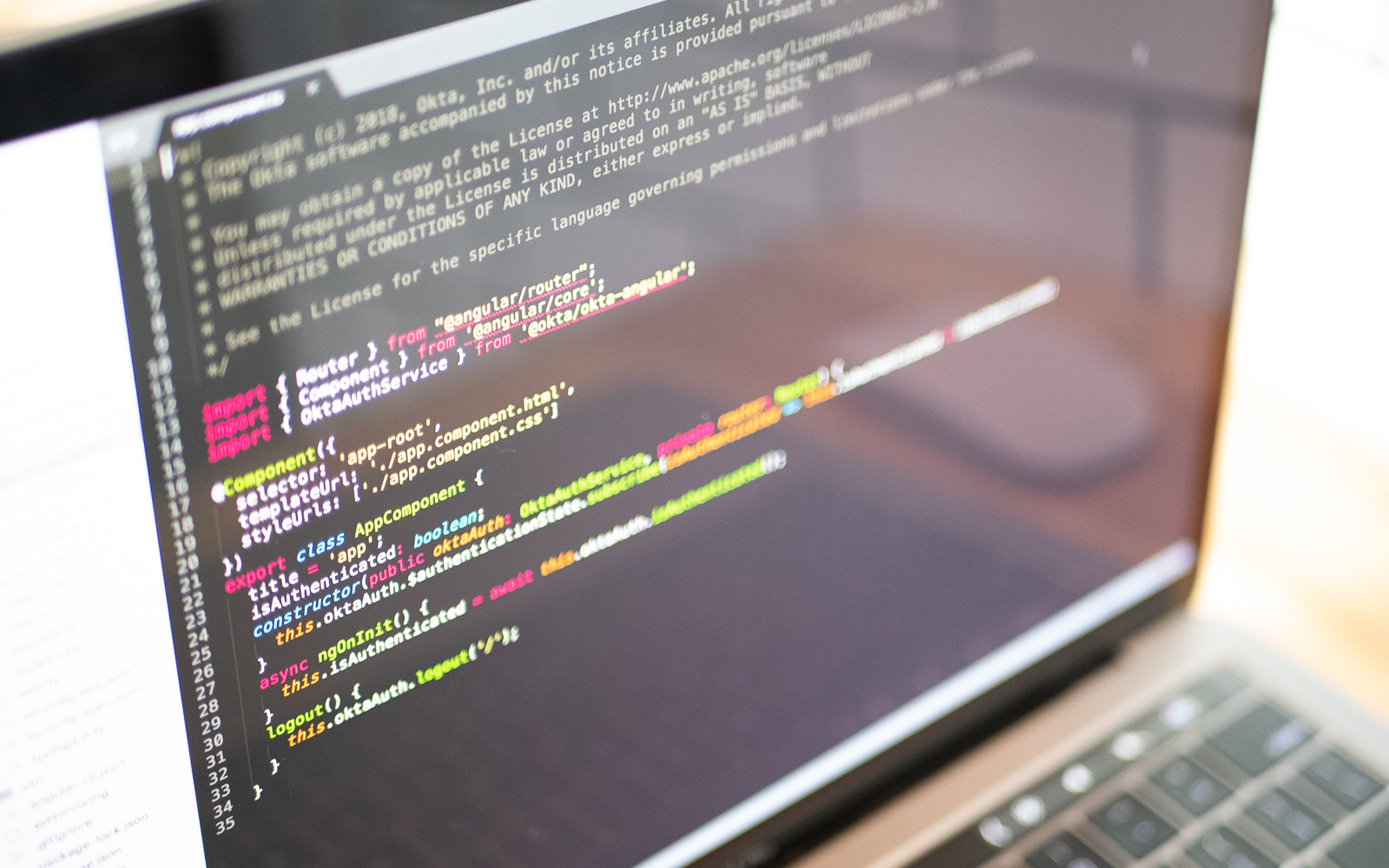
Angular 7 was released earlier this quarter and I’m pumped about a few of its features. If you’ve been following Angular since Angular 2, you know that upgrading can sometimes be a pain. There was no Angular 3, but upgrading to Angular 4 wasn’t too bad, aside from a bunch of changes in Angular’s testing infrastructure. Angular 4 to Angular 5 was painless, and 5 to 6 only required changes to classes that used RxJS....
Build a Simple Web App with Express, Angular, and GraphQL
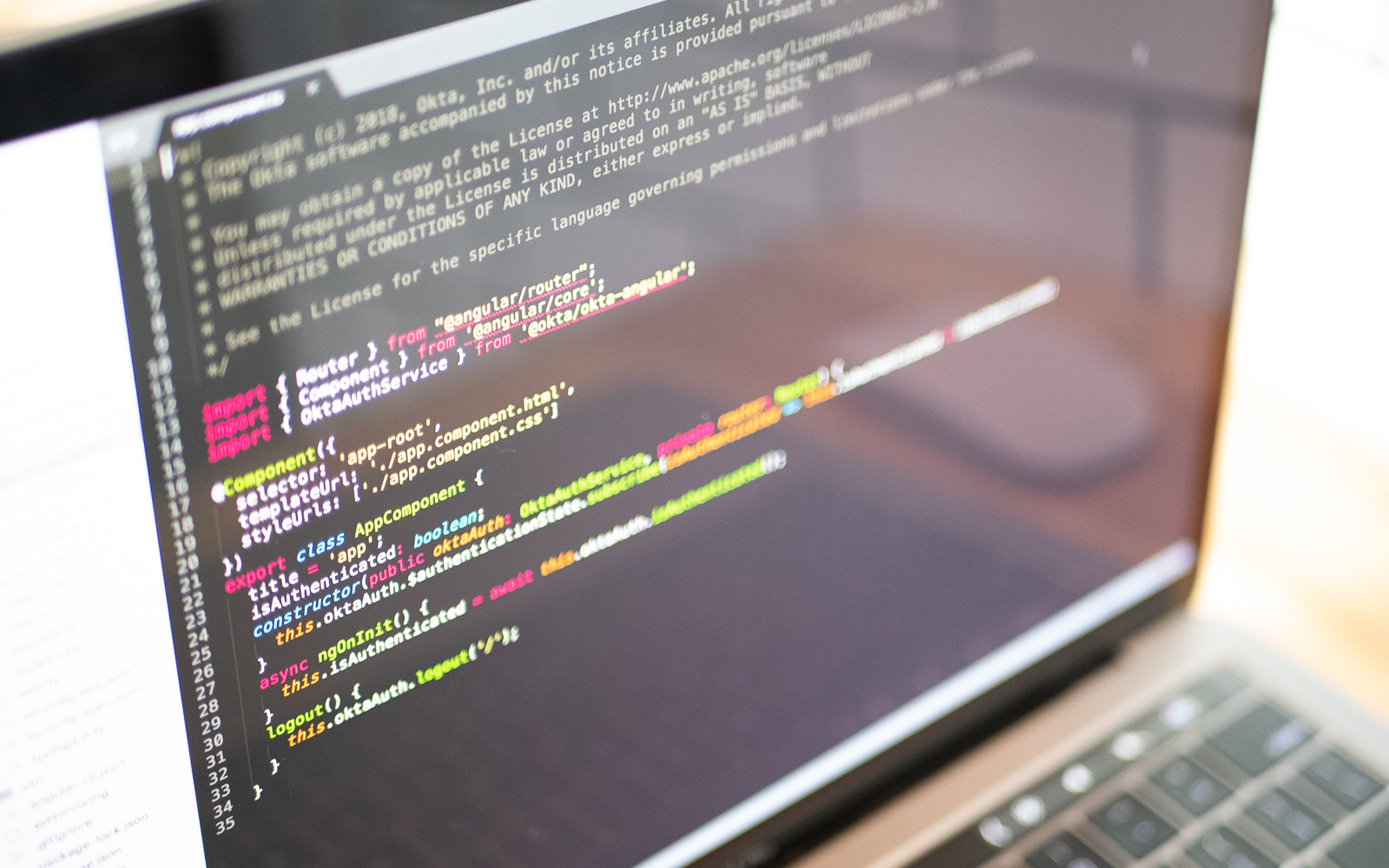
During the past 10 years or so, the concept of REST APIs for web services has become the bread and butter for most web developers. Recently a new concept has emerged, GraphQL. GraphQL is a query language that was invented by Facebook and released to the public in 2015. During the last three years, it has created quite a stir. Some regard it as a new revolutionary way of creating web APIs. The main difference...
Build a Basic CRUD App with Angular and Node
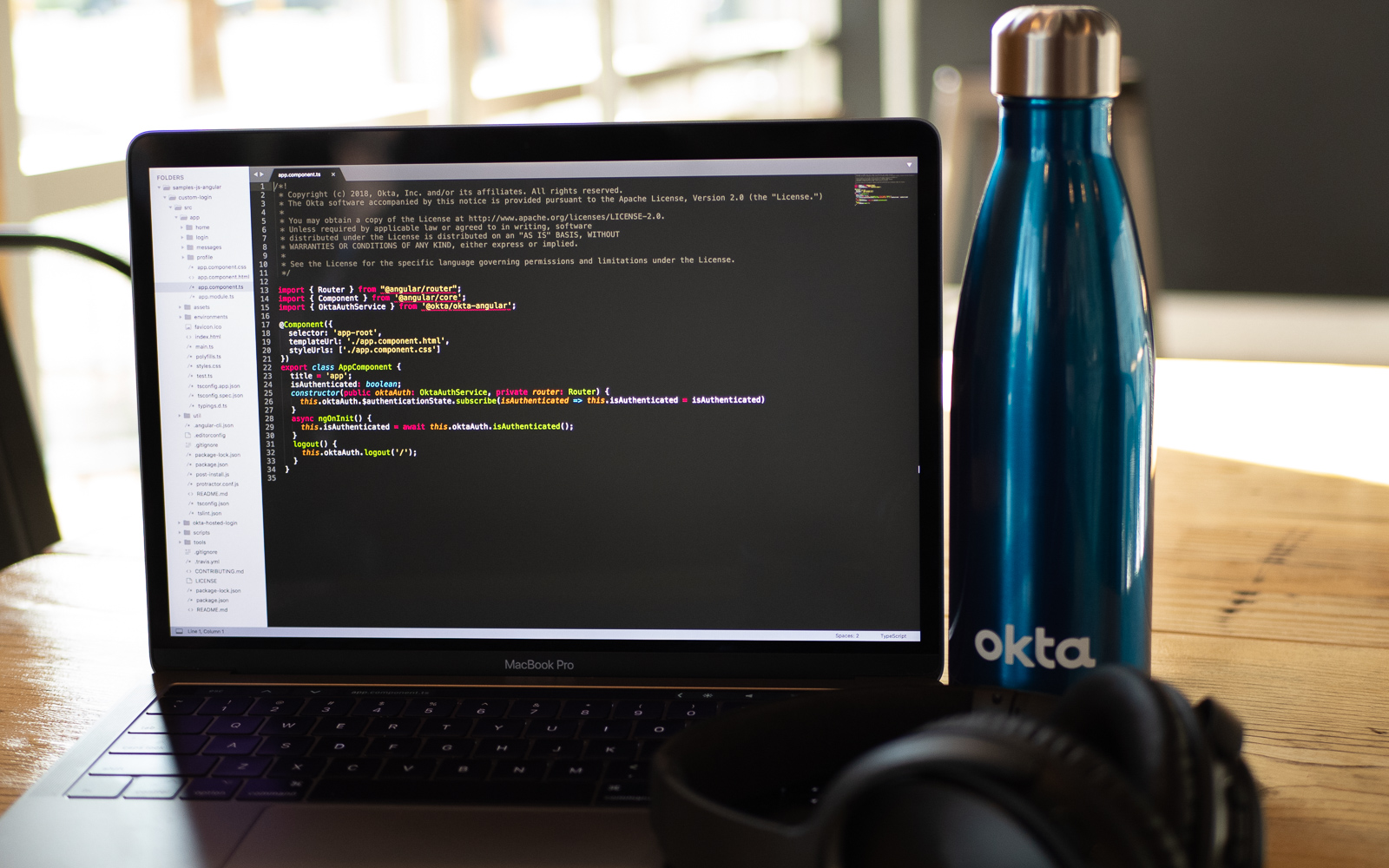
In recent years, single page applications (SPAs) have become more and more popular. A SPA is a website that consists of just one page. That lone page acts as a container for a JavaScript application. The JavaScript is responsible for obtaining the content and rendering it within the container. The content is typically obtained from a web service and RESTful APIs have become the go-to choice in many situations. The part of the application making...
Tutorial: Build a Basic CRUD App with Laravel and Angular
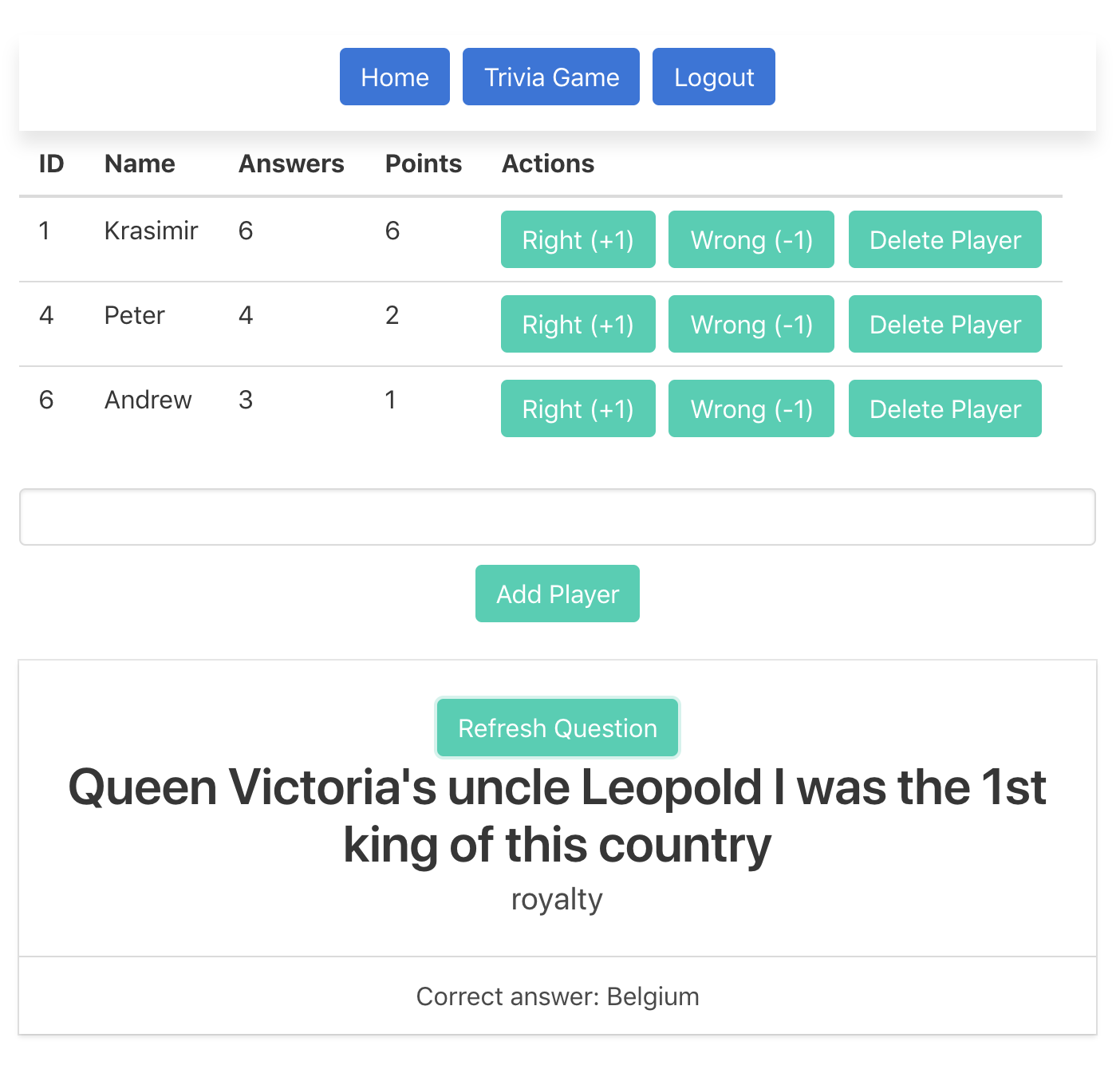
Laravel is a popular PHP framework for Web application development and it’s a pretty good choice if you’re starting a new project today for multiple reasons: Laravel is a well-architectured framework that’s easy to pick up and write elegant code, but it’s powerful as well. It contains many advanced features out-of-the-box: Eloquent ORM, support for unit/feature/browser tests, job queues, and many more. There’s an abundance of great learning resources and it boasts one of the...
Build a Basic CRUD App with Angular 7.0 and Spring Boot 2.1
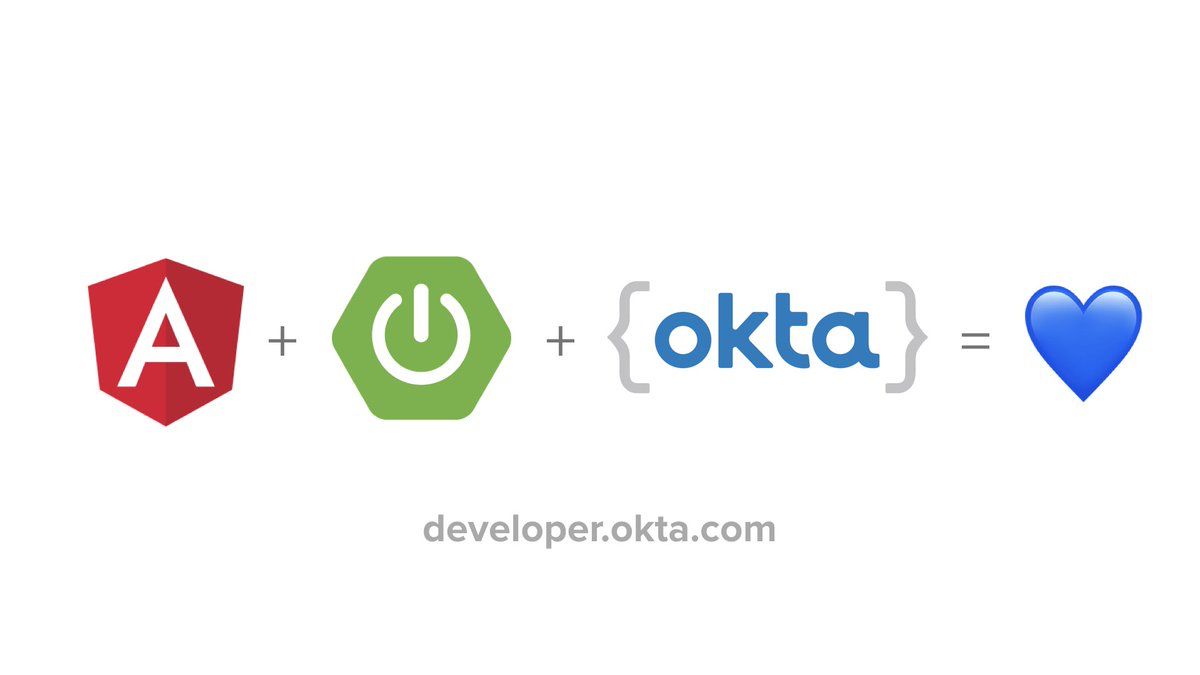
Technology moves fast these days. It can be challenging to keep up with the latest trends as well as new releases of your favorite projects. I’m here to help! Spring Boot and Angular are two of my favorite projects, so I figured I’d write y’all a guide to show you how to build and secure a basic app using their latest and greatest releases. In Spring Boot, the most significant change in 2.0 is its...
Tutorial: Build Your First CRUD App with Symfony and Angular
Building a web application isn’t supposed to be drudgery. No developer has ever said “I’d really like to spend two hours configuring webpack and TypeScript this weekend.” You’d rather build cool stuff NOW and spend time thinking about your applications, not the tools you’re forced to use. In a lot of cases the “cool stuff” is a dynamic, fast, secure single-page app. To achieve that, in this tutorial I’ll show you how to get a...
Build a SPA with ASP.NET Core 2.1, Stripe, and Angular 6
Buying things on the Internet has become a daily activity and is a feature many new projects require. In this tutorial, I will show you how to build an app to sell tickets using an Angular 6 single page app (SPA) using an ASP.NET Core 2.1 backend API. You’ll build both the Angular and ASP.NET Core applications and run them from within VS Code. Let’s get to it! Note: In May 2025, the Okta Integrator...
Build a CRUD-y SPA with Node and Angular
Even before the release of Angular 6, Angular had gone through some changes over the years. The biggest one was the jump from AngularJS (v1.x) to Angular (v2+), which included a lot of breaking syntax changes and made TypeScript the default language instead of JavaScript. TypeScript is actually a superset of JavaScript, but it allows you to have strongly typed functions and variables, and it will get compiled down to JavaScript so that it can...
Upgrade your ASP.NET Core 2.1 App to Angular 6
With the release of ASP.NET Core, there are several templates in the DotNet CLI. One of those templates is an Angular template that scaffolds a single page application built with Angular and ASP.NET Core. The problem with that template is that it scaffolds an Angular 4.2.5 project and Angular released Angular 6 in May of 2018! In this post, not only will I show you how to build a base CRUD app with ASP.NET Core...
Build a CRUD App with ASP.NET Framework 4.x Web API and Angular
Even with all the hype around ASP.NET Core, many .NET developers continue to develop applications with ASP.NET 4.x. The ASP.NET 4.X framework is still being developed, and will be supported for a long time to come. It’s a battle-tested web framework that has existed for over 15 years and is supported by a mature ecosystem. On the client side, many developers prefer Angular, and it is outstanding for building enterprise-level, feature rich, applications. The application...
Add CI/CD to Your Spring Boot App with Jenkins X and Kubernetes
A lot has happened in the last five years of software development. What it means to build, deploy, and orchestrate software has changed drastically. There’s been a move from hosting software on-premise to public cloud and shift from virtual machines (VMs) to containers. Containers are cheaper to run than VMs because they require fewer resources and run as single processes. Moving to containers has reduced costs, but created the problem of how to run containers...
Deploy Your Secure Spring Boot + Angular PWA as a Single Artifact
I’ve written several posts on this blog that show you how to develop an Angular SPA (single-page application) that talks to a Spring Boot API. In almost all of them, I’ve used OAuth 2.0’s implicit flow and built, tested, and deployed them as separate applications. IMHO, this is the way many applications (outside of the Java world) are built and deployed. What if you could combine the two applications for production, and still get all...
Angular 6: What's New, and Why Upgrade
Angular 6 is now available and it’s not a drop-in replacement for Angular 5. If you’ve been developing with Angular since Angular 2, you likely remember that it wasn’t too difficult to upgrade to Angular 4 or Angular 5. In most projects, you could change the version numbers in your package.json and you were on your way. In fact, the most significant change I remember in the last couple of years was the introduction of...
The Hitchhiker's Guide to Testing Spring Boot APIs and Angular Components with WireMock, Jest, Protractor, and Travis CI
Writing apps with Spring Boot and Ionic (which is built on Angular) can be fun because both frameworks offer a good developer experience (DX). You change a file, save it, and the app automatically reloads with your changes. This feature is often called hot-reload and it’s a blissful way to develop. It’s so nice, it can cause you to forget about automating your tests. I’ll admit, writing tests is difficult to do. Writing the test...
Build a CRUD App with ASP.NET Core and Angular
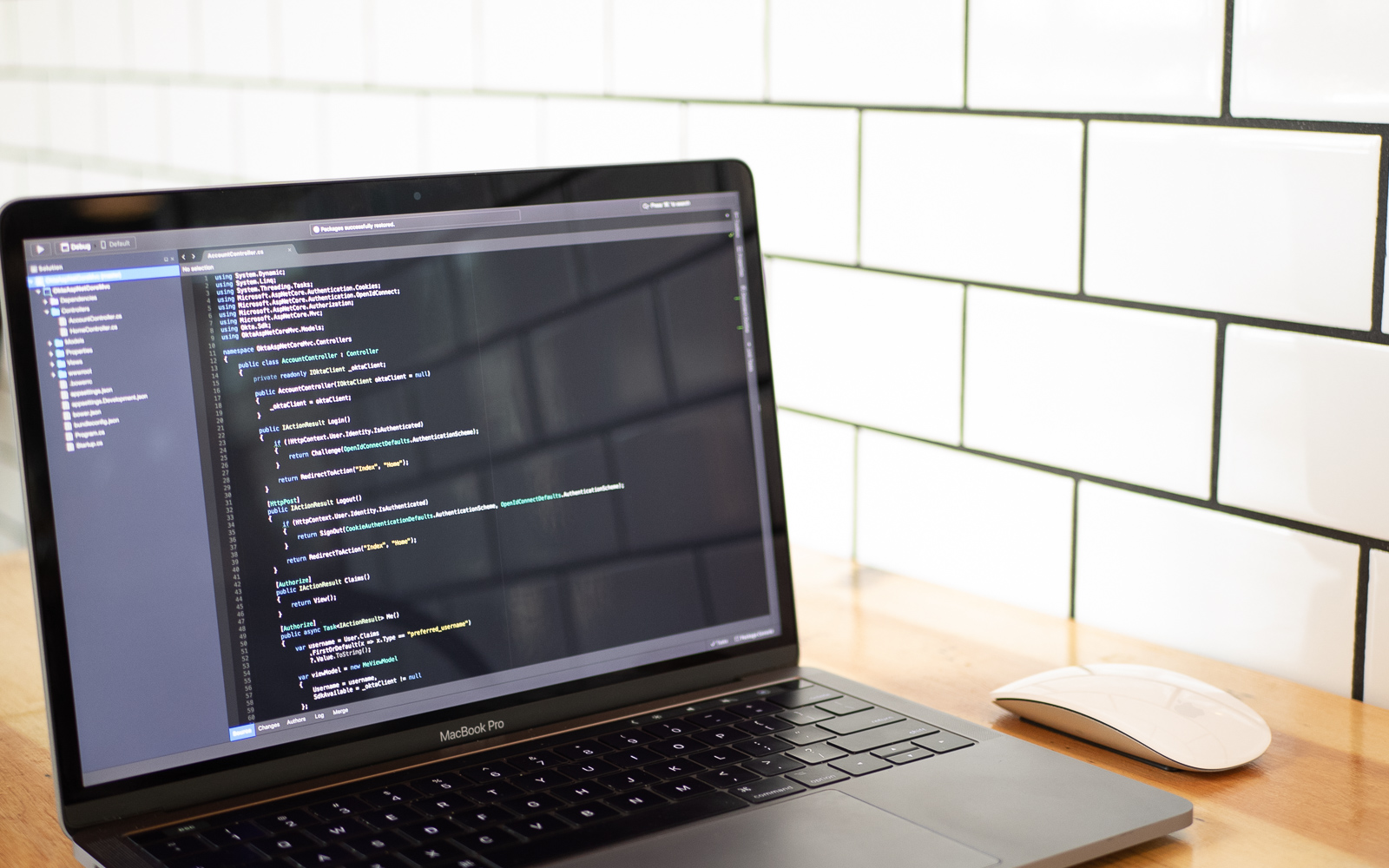
A lot of applications today are built with an API on the backend, and then a single page application on the front end. This is a good approach because it allows you a ton of flexibility. For example, if you get a requirement to build a native mobile client later on: it’s easy, you already have the server side in place. Today you’ll use ASP.NET Core 2.0 on the server side, and Angular 5 on...
Open Source Framework Samples and Quickstarts for Okta's Developer APIs
Developers love sample applications. It’s one thing to see the steps to create an application or feature; but when someone provides a working app you can just build and run it’s simply fantastic. Open source is near and dear to many developers today. Many of the frameworks we use to build applications are open source. It’s a great way to develop widely-used software and get contributions from your users. Okta’s Developer Experience (DevEx) team believes...
Protect Your Cryptocurrency Wealth Tracking PWA with Okta
Cryptocurrencies are all the rage. Over the last year, the value of Bitcoin alone has risen 1,603%, driving more and more people to wonder if they’re missing out on the “next big thing.” Because of the massive influx of money into cryptocurrencies like Bitcoin, Ethereum, Monero, and Ripple — blockchain technology (which is the foundation of all cryptocurrency) has become an area of intense technical study. At its core, blockchain technology does nothing more than...
Build a Basic CRUD App with Angular 5.0 and Spring Boot 2.0
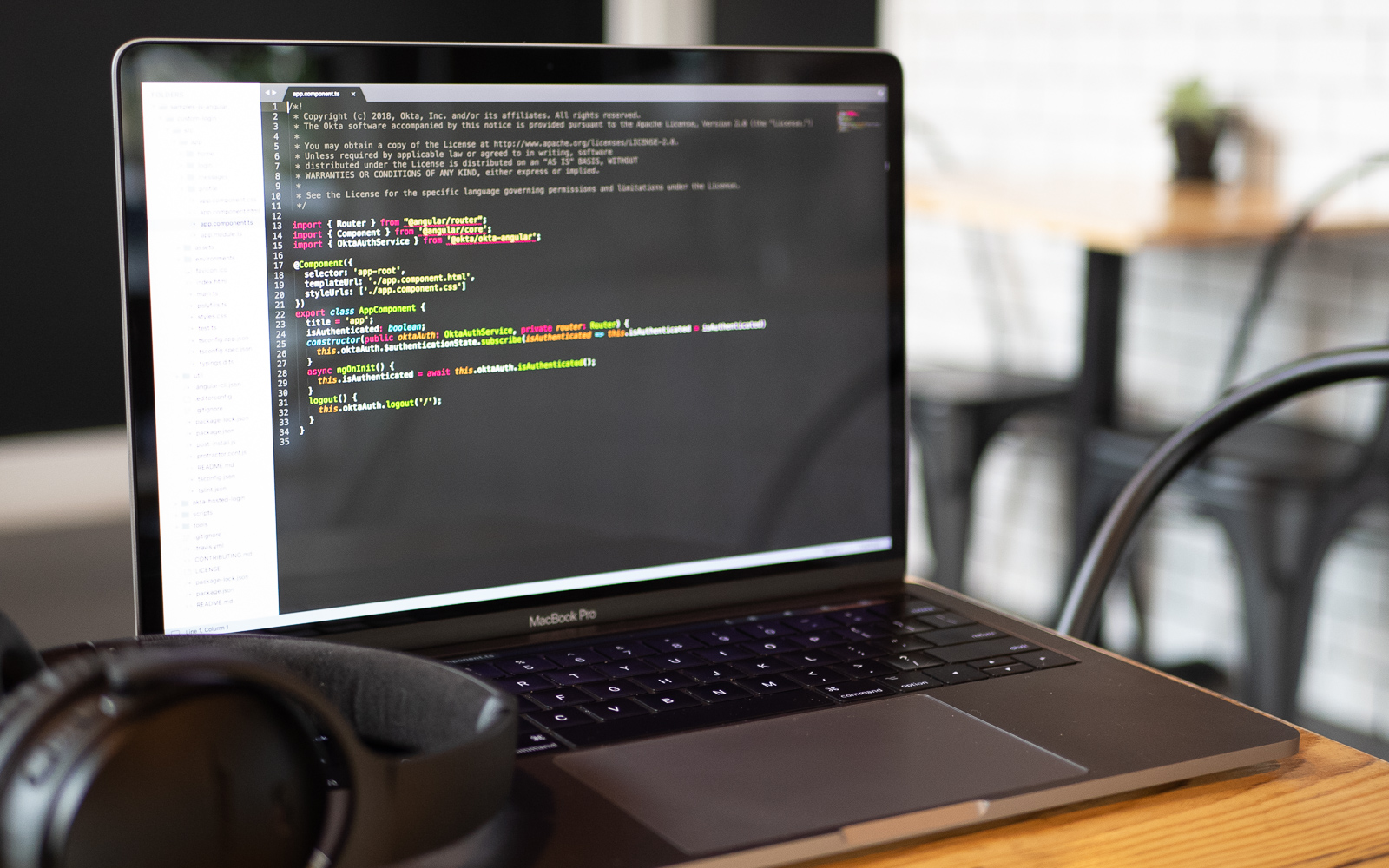
Technology moves fast these days. It can be challenging to keep up with the latest trends as well as new releases of your favorite projects. I’m here to help! Spring Boot and Angular are two of my favorite projects, so I figured I’d write y’all a guide to show you how to build and secure a basic app using their latest and greatest releases. In Spring Boot, the most significant change in 2.0 is its...
Use OpenID Connect Support with JHipster
Single sign-on (SSO) is a feature that most developers don’t care about when building one-off applications for clients or themselves. However, when developing apps for their company, which will be used by employees of their business, they often need to hook into an existing identity provider. It might be Active Directory (AD), LDAP, or a myriad of other systems. Okta provides SSO for many companies around the world and allows them to configure AD and...
The Top 10 JavaOne 2017 Sessions for the Java Hipster
A “hipster” is defined as a person who is exceptionally aware of or interested in the latest trends and tastes. JHipster is an open source project whose name stands for “Java Hipster.” If you’re using JHipster, chances are you’re aware of and using the latest trends and techniques in Java development. Trendy things in server-side Java development include microservices, embedded app servers, deployment with containers, auto-configuration, and monitoring. JHipster supports all of these trends, embracing...
Build a Secure Notes Application with Kotlin, TypeScript, and Okta
I love my job as a developer advocate at Okta. I get to learn a lot, write interesting blog posts and create example apps with cool technologies like Kotlin, TypeScript, Spring Boot, and Angular, which I’m about to demo. When it comes to writing Hello World apps with authentication, I can whip one out in a few minutes. That isn’t because I’m a particularly good programmer, it’s because the languages, frameworks, tools, and platforms available...
Build an Ionic App with User Authentication
With Okta and OpenID Connect (OIDC) you can easily integrate authentication into an Ionic application, and never have to build it yourself again. OIDC allows you to authenticate directly against the Okta API, and this article shows you how to do just that in an Ionic application. I’ll demo how to log in with OIDC redirect, using Okta’s Auth SDK as well as how to use OAuth with Cordova’s in-app browser; user registration is omitted...
The Ultimate Guide to Progressive Web Applications
Progressive Web Apps, aka PWAs, are the best way for developers to make their webapps load faster and more performant. In a nutshell, PWAs are websites that use recent web standards to allow for installation on a user’s computer or device, and deliver an app-like experience to those users. Twitter recently launched mobile.twitter.com as a PWA built with React and Node.js. They’ve had a good experience with PWAs, showing that the technology is finally ready...
Develop and Deploy Microservices with JHipster
JHipster is one of those open-source projects you stumble upon and immediately think, “Of course!” It combines three very successful frameworks in web development: Bootstrap, Angular, and Spring Boot. Bootstrap was one of the first dominant web-component frameworks. Its largest appeal was that it only required a bit of HTML and it worked! Bootstrap showed many in the Java community how to develop components for the web. It leveled the playing field in HTML/CSS development,...
Add Authentication to Your Angular PWA
You’re developing a Progressive Web Application (PWA), and your service worker and web app manifest are working swimmingly. You’ve even taken the time to deploy it to a server with HTTPS, and you’re feeling pretty good about things. But wait, you don’t have any way of knowing who your users are! Don’t you want to provide them with an opportunity to authenticate and tell you who they are? Once you know who they are, you...
Build Your First Progressive Web Application with Angular and Spring Boot
An October 2016 DoubleClick report found 53% of visits are abandoned if a mobile site takes more than 3 seconds to load. That same report said the average mobile sites load in 19 seconds. According to Alex Russell in his recent talk on the state of mobile development, one of the biggest problems in mobile today is that developers use powerful laptops and desktops to develop their mobile applications, rather than using a $200 device...
Bootiful Development with Spring Boot and Angular
To simplify development and deployment, you want everything in the same artifact, so you put your Angular app “inside” your Spring Boot app, right? But what if you could create your Angular app as a standalone app and make cross-origin requests to your API? Hey guess what, you can do both! I believe that most frontend developers are used to having their apps standalone and making cross-origin requests to APIs. The beauty of having a...
Angular Authentication with OpenID Connect and Okta in 20 Minutes
Angular (formerly called Angular 2.0) is quickly becoming one of the most powerful ways to build a modern single-page app. A core strength is Angular’s focus on building reusable components, which help you decouple the various concerns in your application. Take authentication, for example: it can be painful to build, but once you wrap it in a component, the authentication logic can be reused throughout your application. The Angular CLI makes it easy to scaffold...
Build an Angular App with Okta's Sign-In Widget in 15 Minutes
AngularJS reigned as king of JavaScript MVC frameworks for several years. However, when the Angular team announced they would not provide backwards compatibility for their next version, there was a bit of a stir in its community, giving opportunities for frameworks like React and Vue.js to flourish. Fast forward a few years and both Angular 2 and Angular 4 have been released. Many developers are trying its TypeScript and finding the experience a pleasant one....