Articles tagged php
A Developer's Guide to Elasticsearch with Laravel
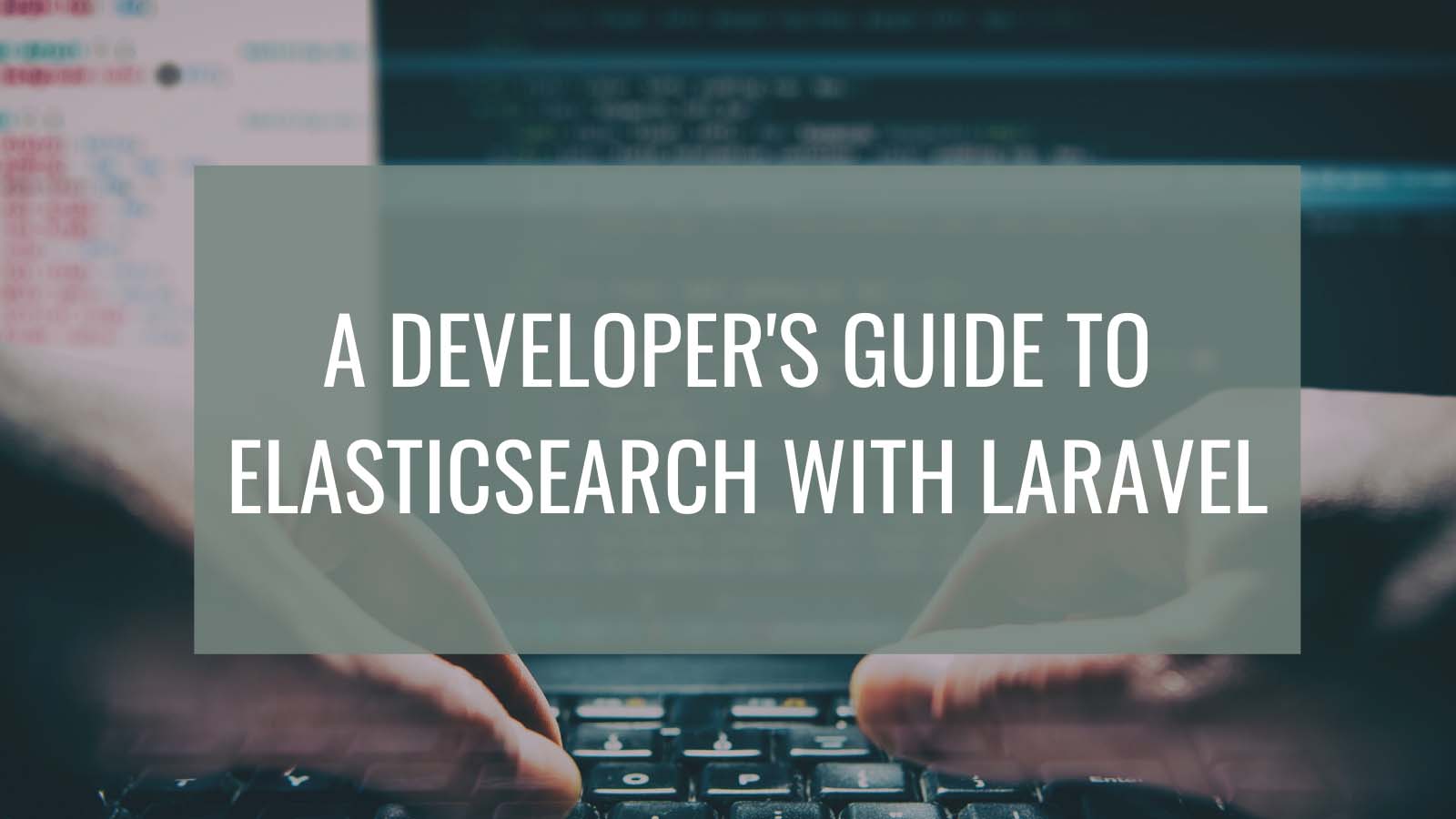
Elasticsearch is a search engine that can be integrated into any of your tech stacks using its REST APIs and its wide selection of libraries. By installing and integrating Elasticsearch into your system, you can add a search engine to your website, add autocomplete functionalities, use its machine learning functionalities to perform analysis on your data and provide better results for your users, and much more. In this tutorial, you’ll learn how to integrate Elasticsearch...
Authentication Patterns for PHP Microservices
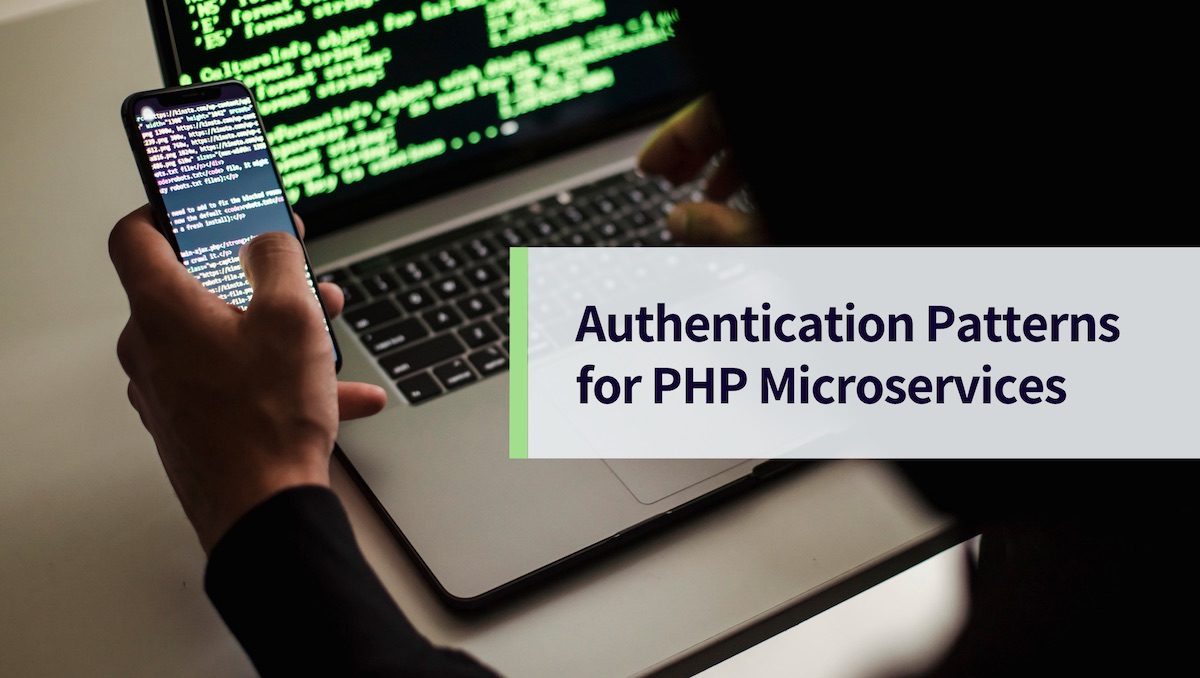
Microservices are an increasingly popular architecture, as they allow you to split application development into smaller, easier-to-manage pieces. However, microservices introduce complexity when it comes to implementing authentication. Generally, you only need to worry about one point of ingress for auth with a traditional monolithic application. Still, there are multiple ways to set things up with microservices and just as many authentication patterns to suit. In this tutorial, you’ll see how to build a small...
Build and Secure GraphQL APIs with Laravel
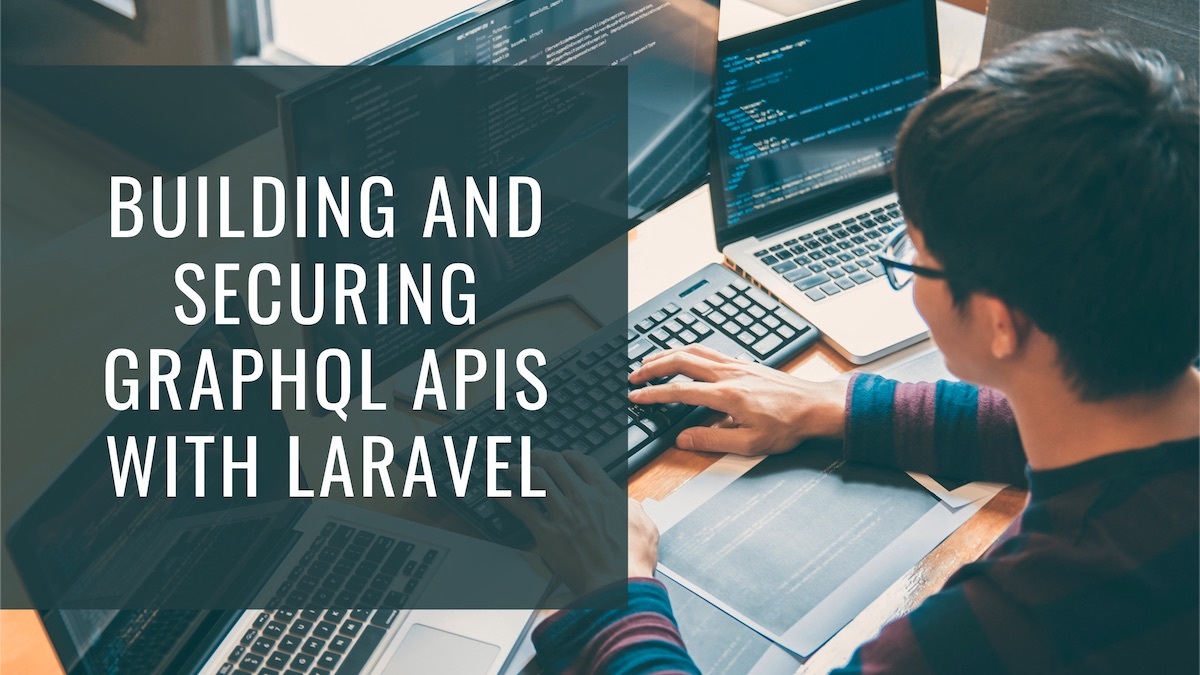
GraphQL’s popularity has grown among frontend and backend developers alike. It allows frontend teams to request only the data they need while preventing an explosion of backend endpoints, since all operations can go through one simple endpoint for all models being worked on. In this tutorial, you will learn how to set up a GraphQL API with Laravel, a free open-source PHP web application framework. You’ll then secure the API so that it’s only accessible...
Comparing Authentication in Laravel Versions 6, 7, and 8
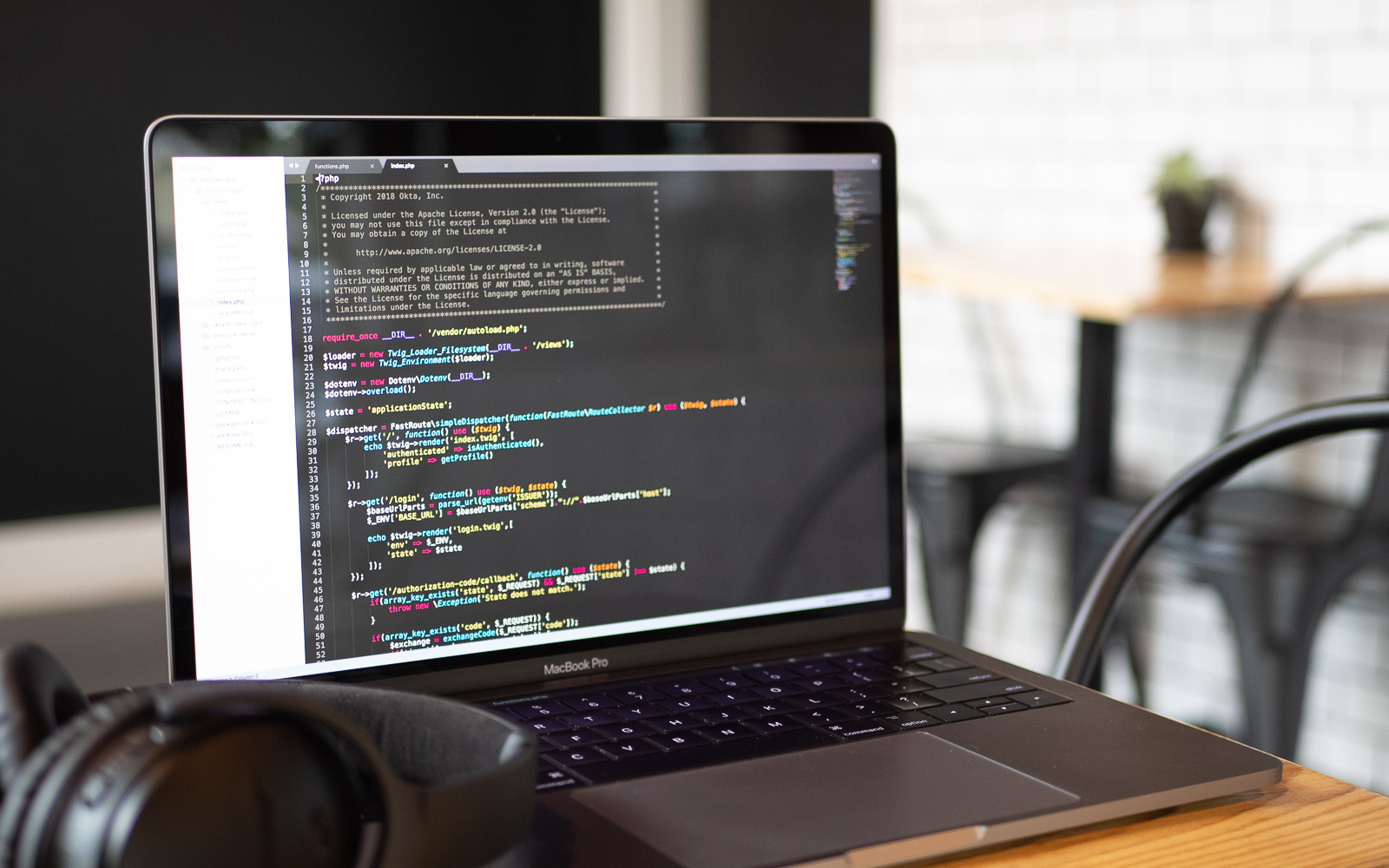
Laravel is one of the most popular PHP frameworks in use today. Version 8 was released in September 2020, and while the bulk of the framework hasn’t changed, each of the three most recent versions have included updates to the authentication pattern. While Laravel 7 and 8 will both stop receiving updates in 2021, Laravel 6 is a long-term support version and will include security fixes until September 2022. So, if you’re upgrading or starting...
Protecting a Laravel API with JWT
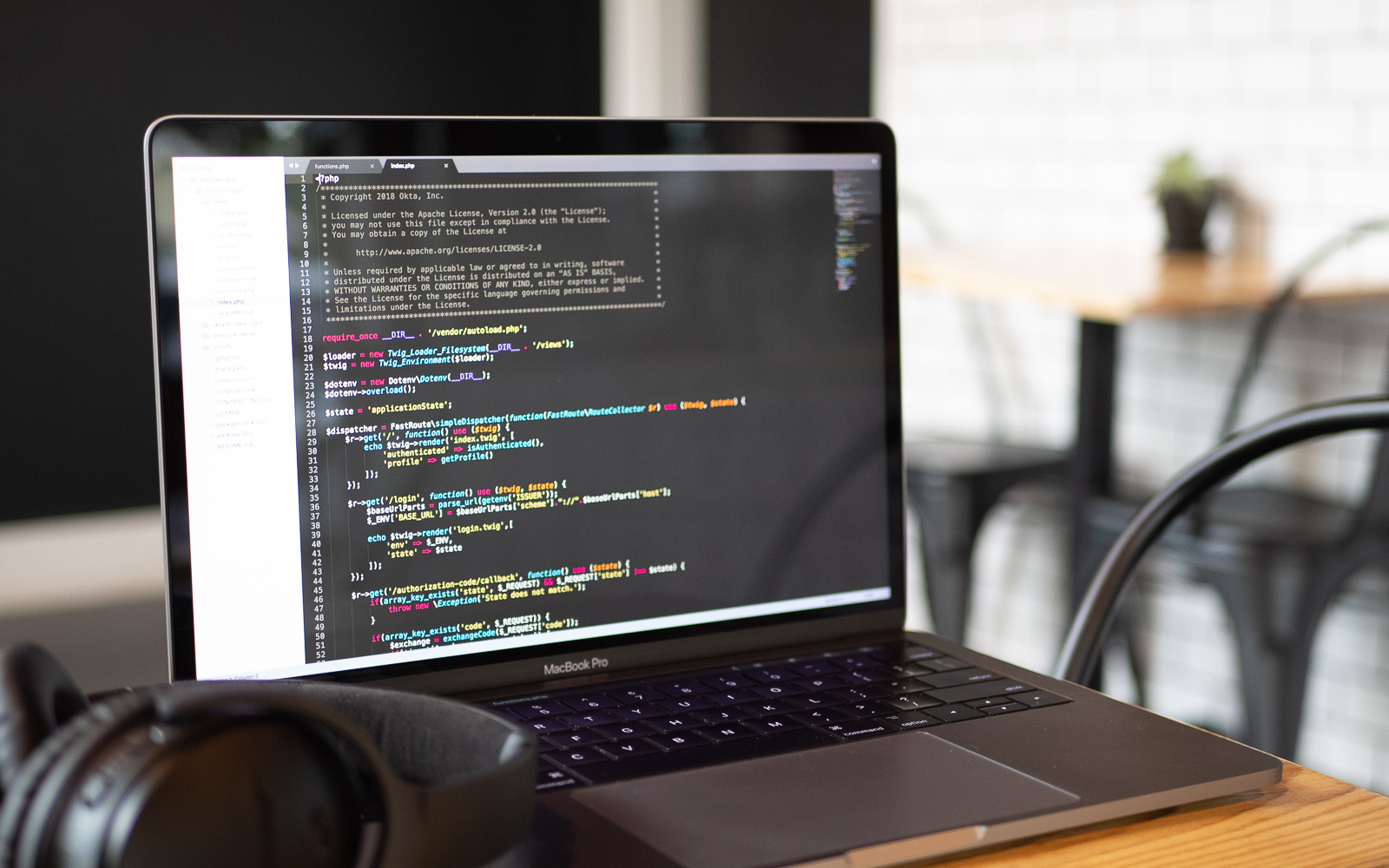
With the increasing popularity of single-page apps and the growing API economy, JSON Web Tokens (JWTs) are becoming a very popular method for authenticating users. Rather than relying on the server to store the user’s state, JWTs encode information in a keyed payload stored on the client. JWTs are not inherently less secure than server-side session storage. But developers should understand the tradeoffs and know what to do if a JWT is compromised. You should...
What's New in Laravel 8
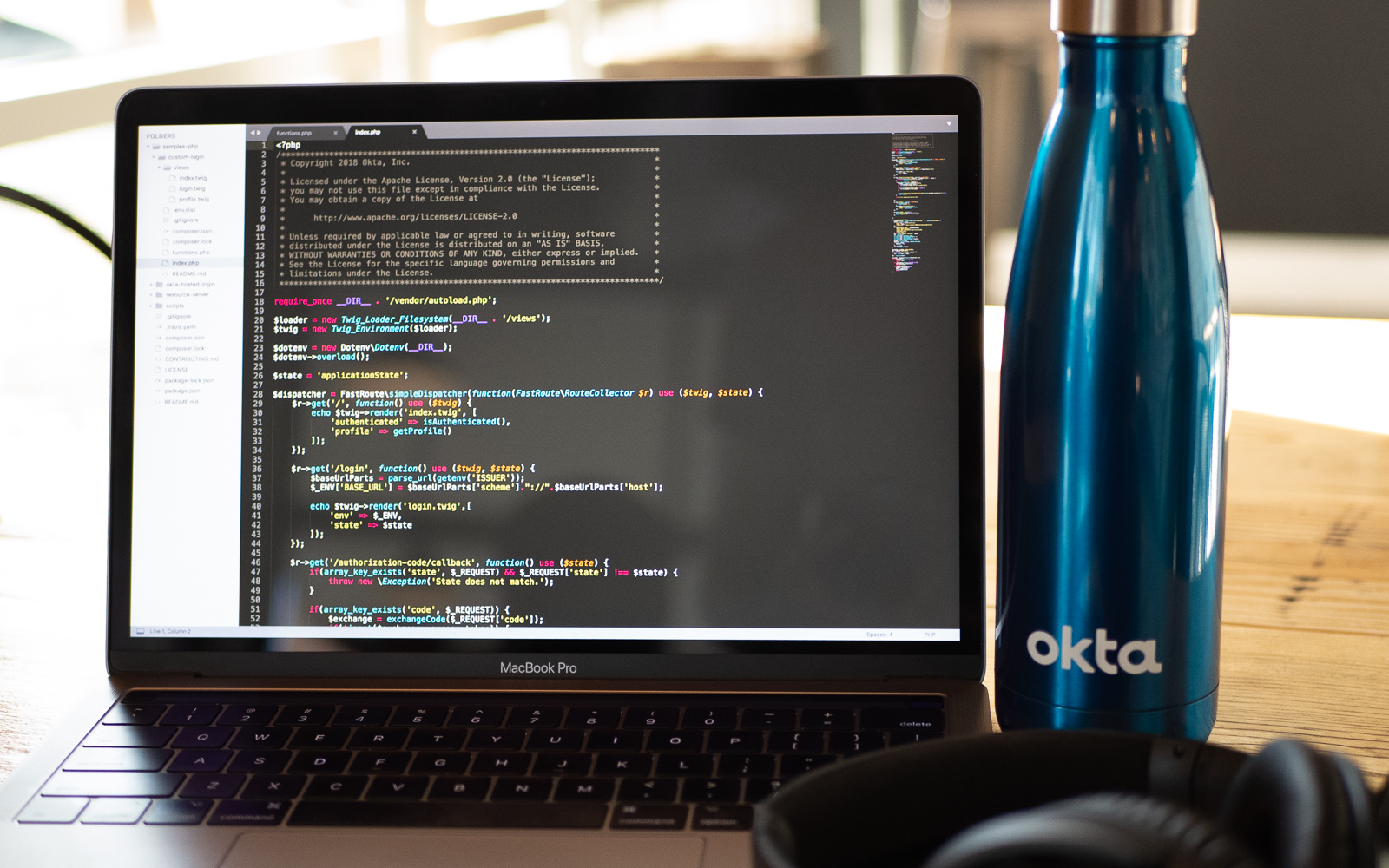
With Laravel 8’s release in September 2020, the popular PHP framework continues to offer new features and improvements. After version 5, Laravel moved to semantic versioning and the more frequent releases have meant smaller changes between each one. That said, there are still several exciting updates in this version of the framework. While Laravel will continue to offer security fixes for version 7 until early 2021, no more bug fixes will be released after October...
Validating Okta Access Tokens in PHP using AWS API Gateway and Lambda Authorizers
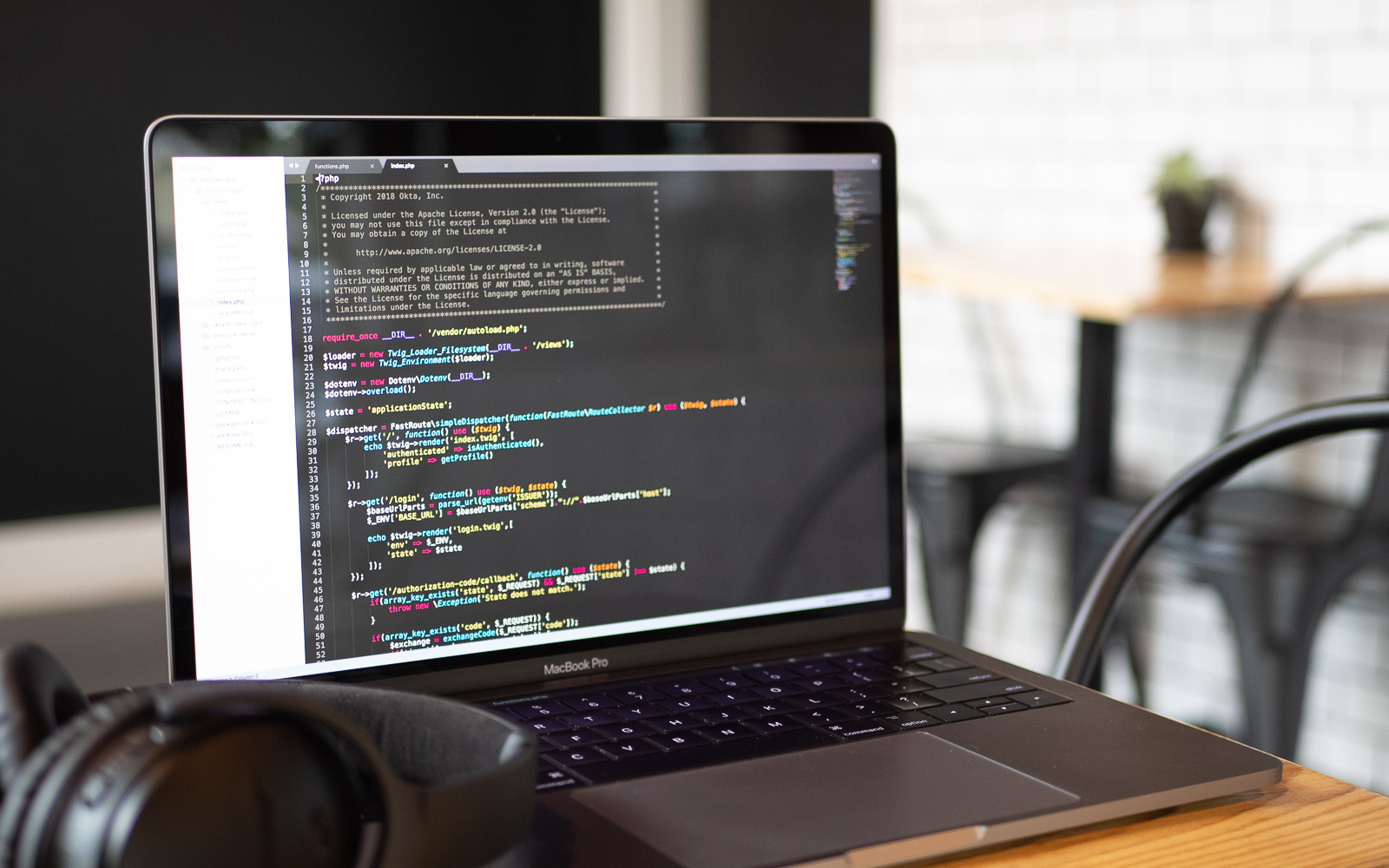
Running REST APIs with AWS Lambda and AWS API Gateway has recently become a very popular option. Although AWS provides its own mechanisms to add an authentication and authorization layer to these APIs, you may want to use your Okta centralized user database and credentials instead. Today we’ll talk about how you can use Okta as the authentication and authorization layer of your REST API hosted in AWS Lambda, validating Okta access tokens using a...
SQL Injection in PHP: Practices to Avoid
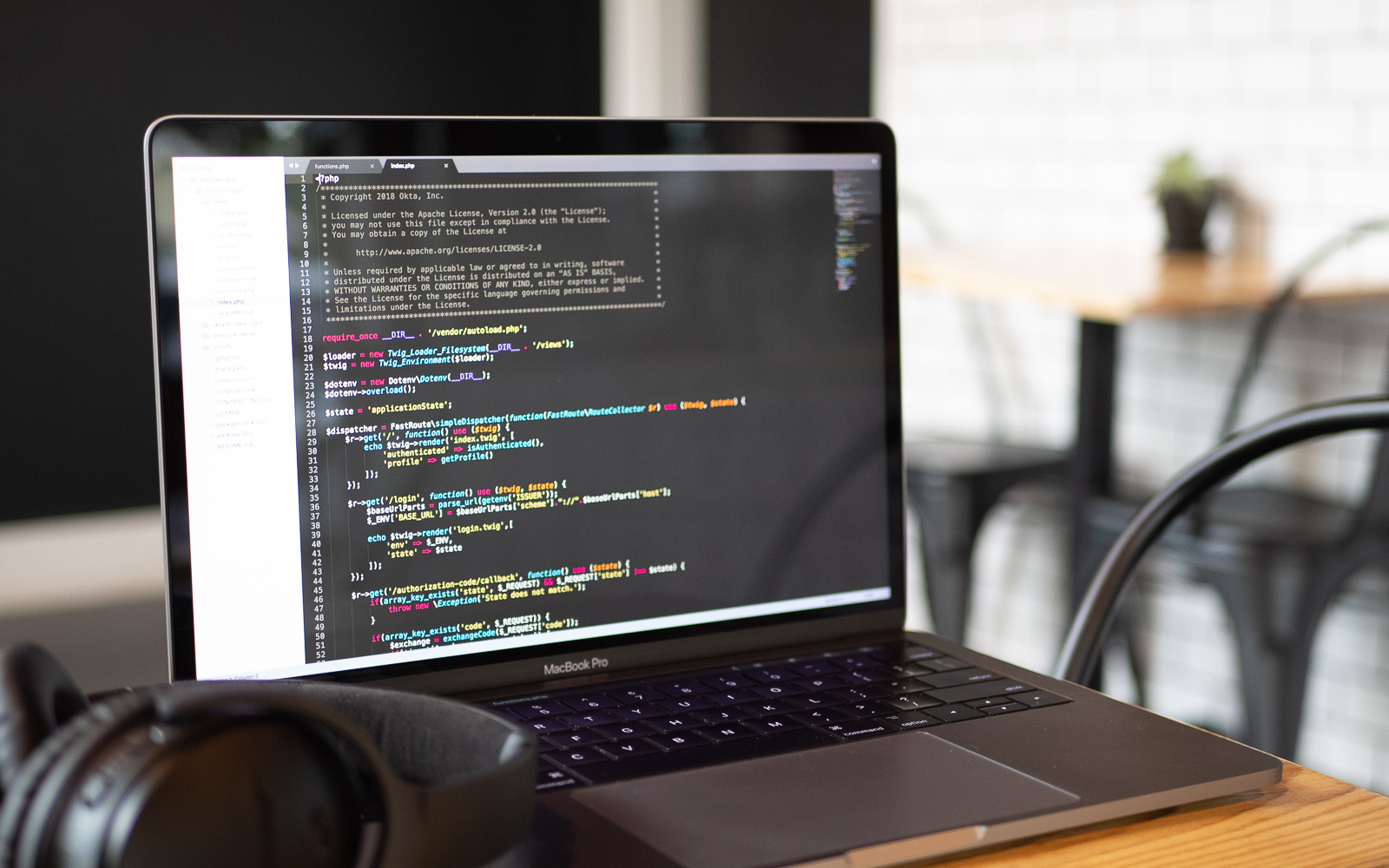
SQL injections are one of the most common vulnerabilities found in web applications. Today, I’m going to explain what a SQL injection attack is and take a look at an example of a simple vulnerable PHP application accessing a SQLite or MySQL database. After that, we’ll look at several methods to prevent this attack, fixing the problem. Prerequisites Make sure you have the following software installed and enabled on your system: PHP 7 Composer PHP...
Stop Writing Server-Based Web Apps
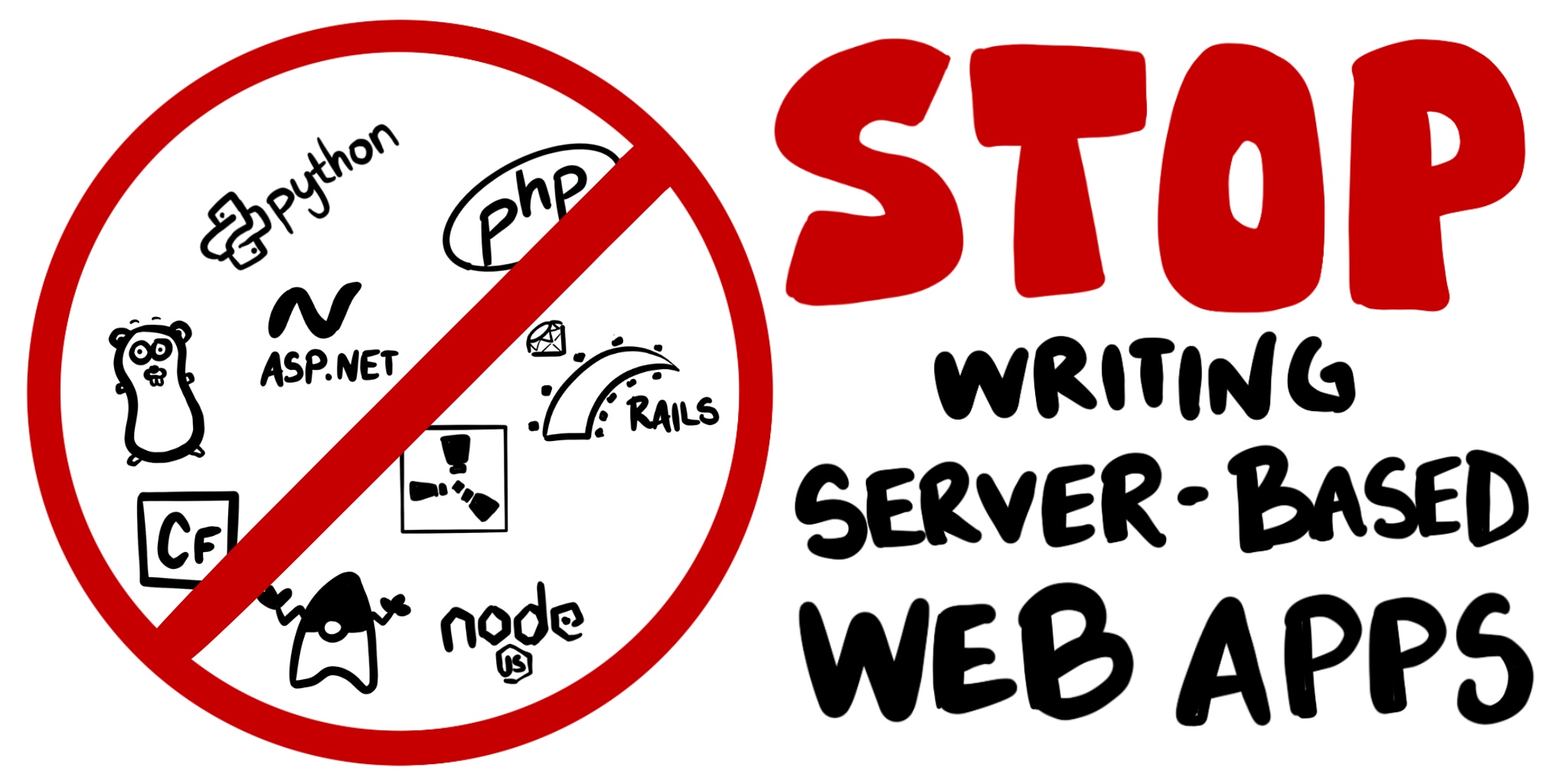
The World-Wide Web, as we know it, started around 1993 by serving static HTML files with links to other HTML files. It didn’t take long for developers to find ways of making websites more “dynamic” using technologies like Common Gateway Interface (CGI), Perl, and Python. Since the ’90s, I have built web applications using a variety of languages, platforms, and frameworks. I’ve written application frameworks, content management systems, a blog engine, and a social media...
Protecting a PHP API Using OAuth
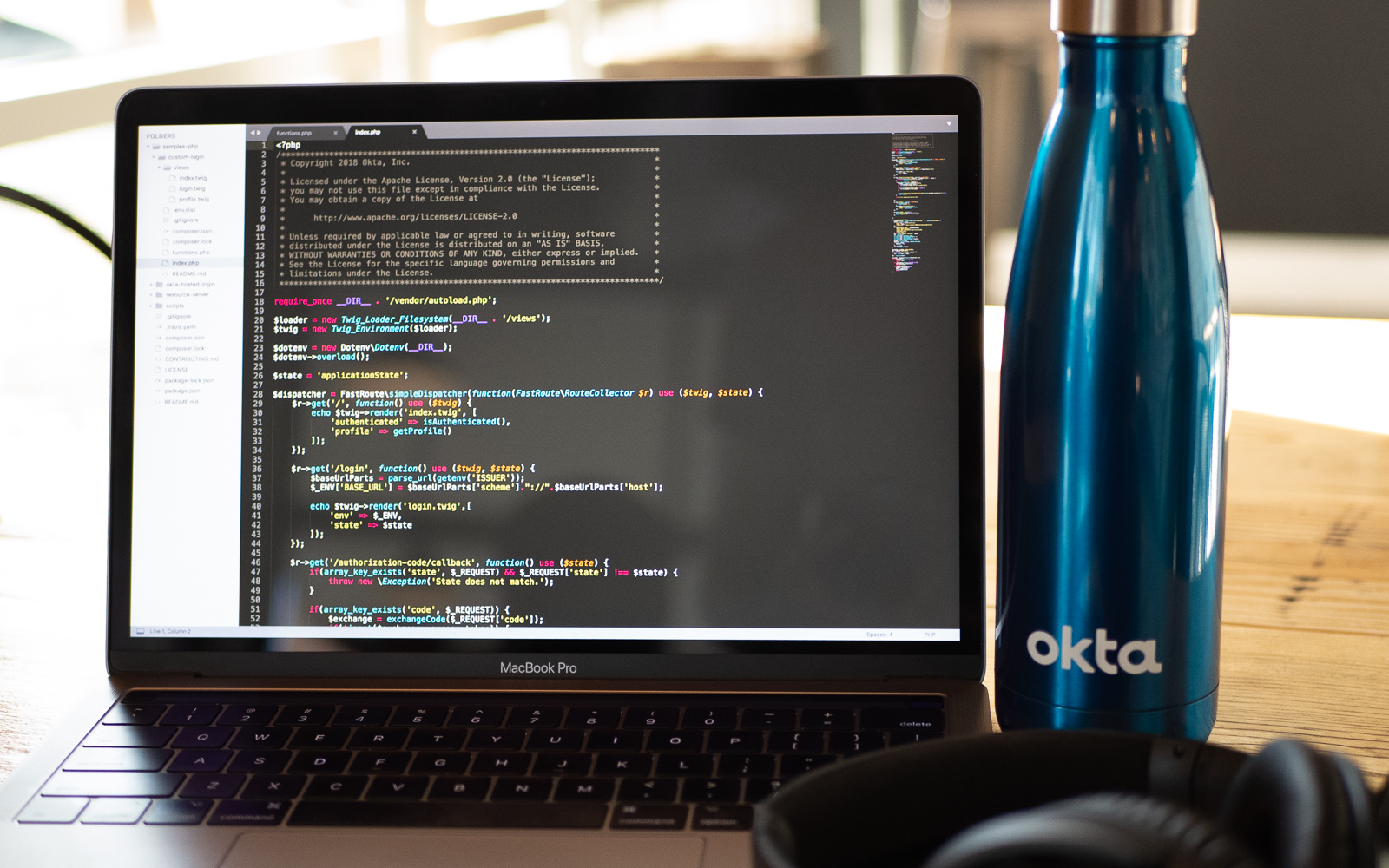
REST APIs are a big part of today’s Internet. Some of the everyday use cases of REST APIs are: driving the backend of single-page Web applications/mobile applications integrating different applications to exchange data and automate workflows providing the communication channel for the different parts of a complex service-oriented architecture connecting IoT devices. REST API security is essential because an API can expose powerful, mission-critical, and outright dangerous functionality over the Internet. For example, a fintech...
Symfony 4.3 + Vue.js: PHP Apps Made Simple
Today’s Web users are a lot more sophisticated than just a few years ago. They expect applications to be fast, secure, and work equally well on their desktops and phones. Meeting these expectations requires a great deal of work so you can provide a reliable backend and a fully dynamic frontend, while keeping the whole system secure and scalable. However, with the right set of tools, you can get started quickly and become productive in...
Simple, Secure Authentication with CodeIgniter
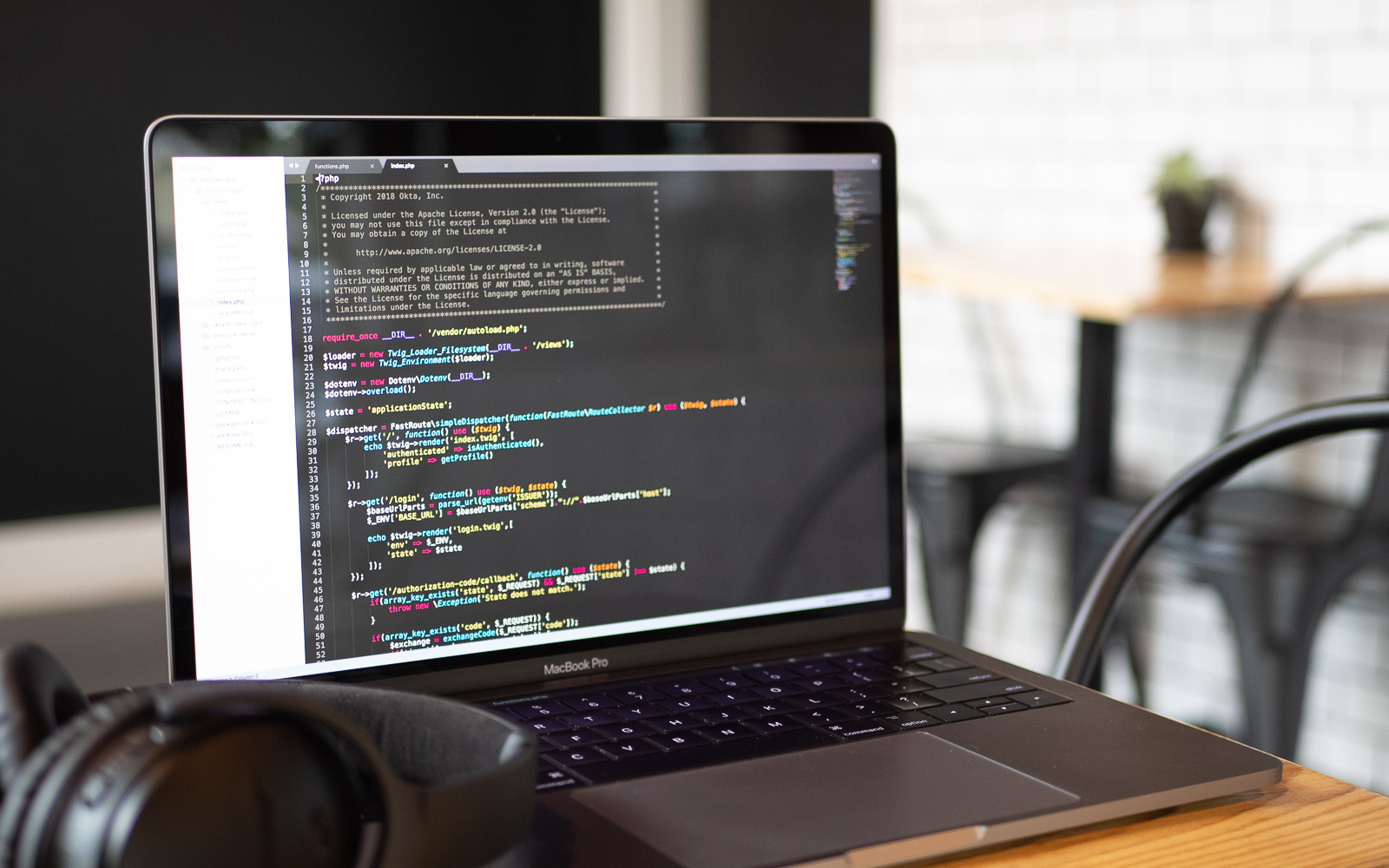
CodeIgniter is a simple, lightweight PHP framework that remains a popular option for many developers. It might lack the sophisticated architecture and advanced features of Symfony or Laravel, but it compensates with a small footprint and a shallow learning curve. In this tutorial, I will show you how to build a simple application for creating/viewing news items. We will extend the standard CodeIgniter tutorial to add user authentication to the application, require a logged-in user...
How to Create a Simple Symfony Application with Authentication
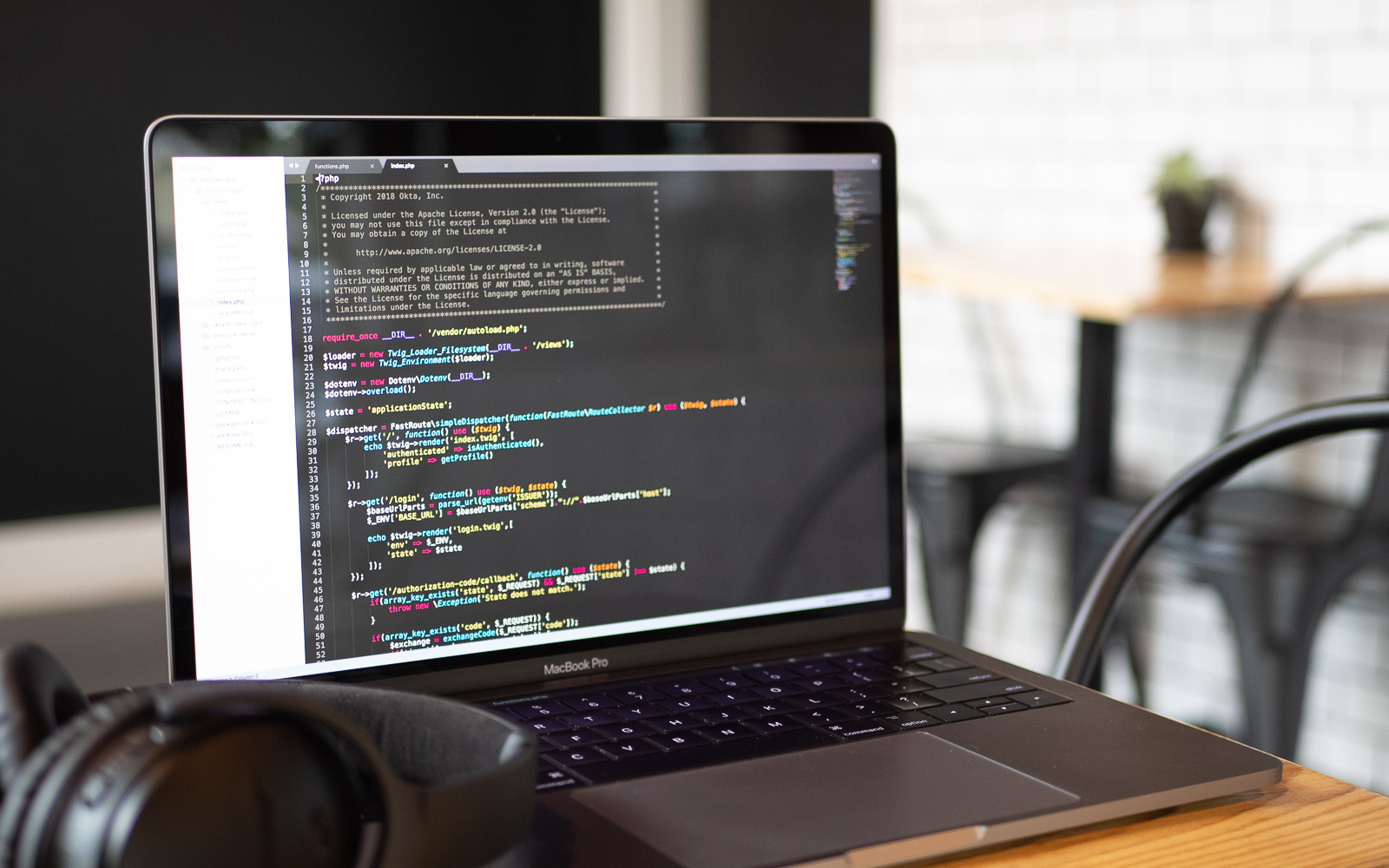
Symfony is not the most popular or loved PHP framework, but it’s arguably the most mature, flexible, and reliable. Since its initial release, Symfony has evolved into a set of loosely-coupled, high-quality components that can be chosen individually or combined to create powerful applications, without the compromise of bloat or huge runtime overhead. These components are also widely used outside the context of the framework, as stand-alone modules or sitting at the bottom of other...
Build a Simple Laravel App with Authentication
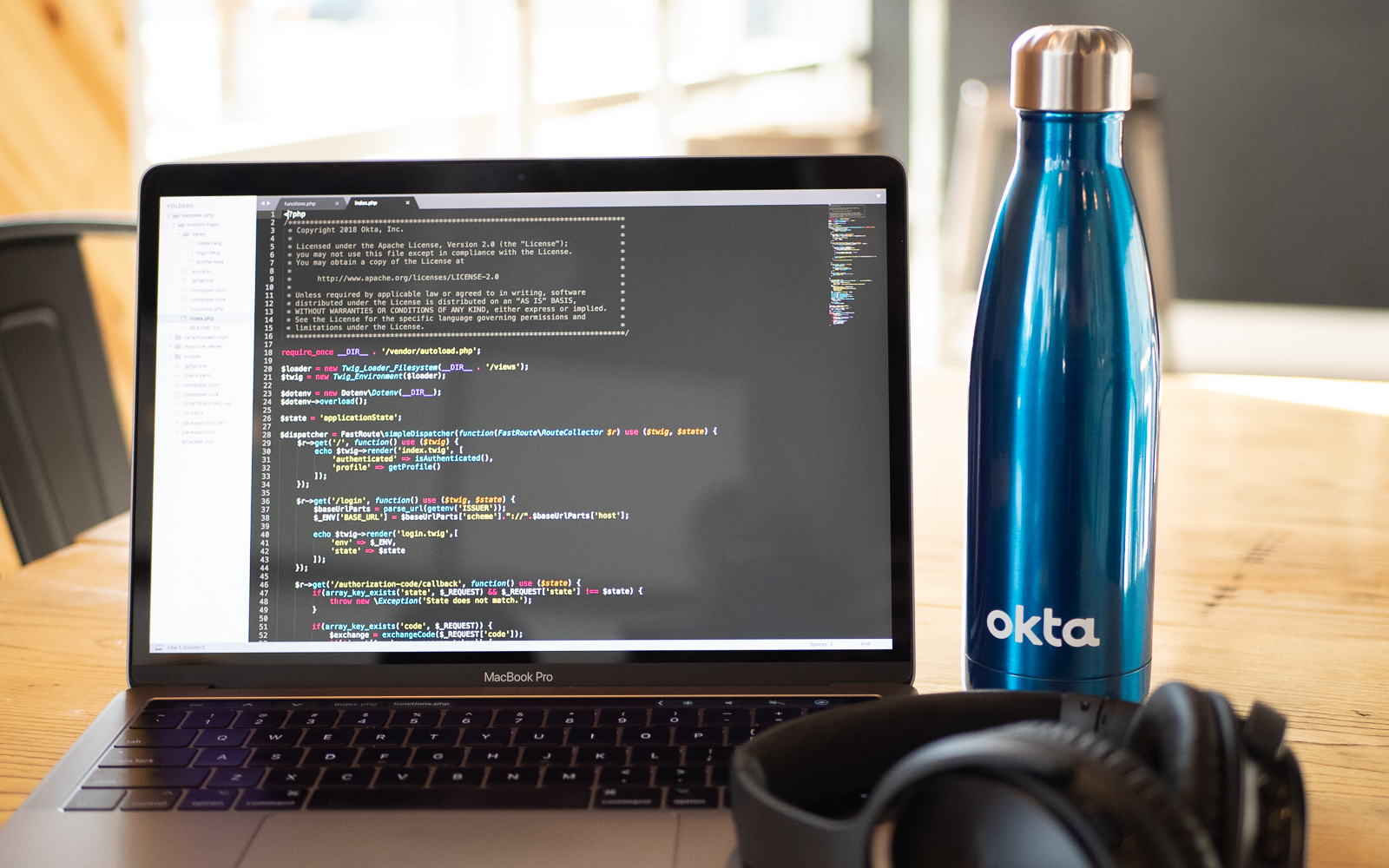
PHP remains the single most popular language choice when creating the backend of a new web application. Within the PHP ecosystem, there are many options when starting a new project: you can use a content management system (CMS) like Wordpress or Drupal, or one of the many frameworks with large user bases and active communities in the PHP world (such as Symfony, CakePHP, CodeIgniter, Yii, Zend Framework). However, if you look at the PHP framework...
PHP Authorization with OAuth 2.0 and Okta
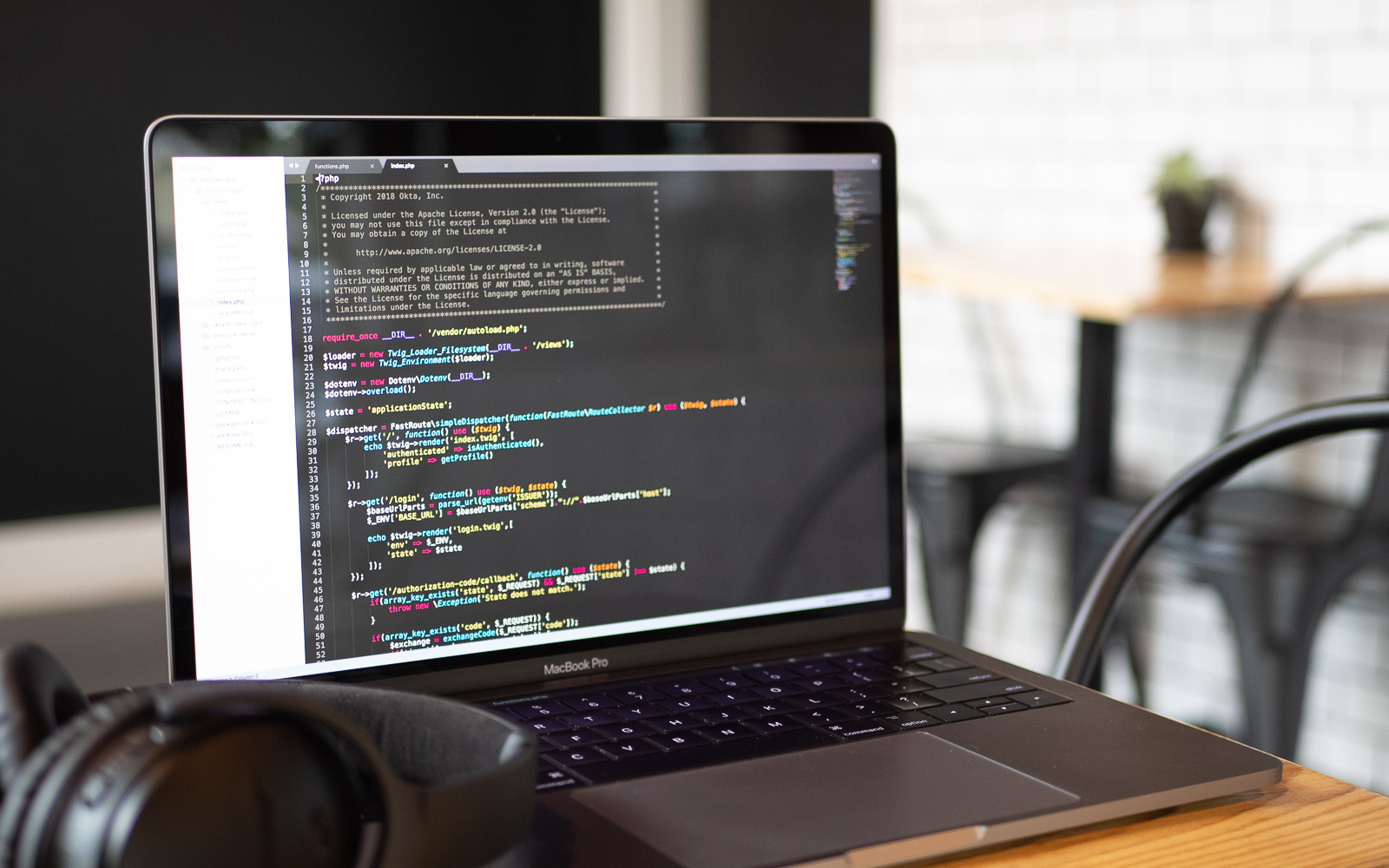
User account management, authorization, and access control can be difficult to implement for many web developers. At the same time, they are critical cornerstones of application security – any mistake can lead to data leaks, financial losses, and legal troubles. Successful web applications inevitably grow to a stage where simple user authorization is no longer sufficient and certain features have to be made available only to certain users. There are a variety of ways to...
Building Scalable Laravel Apps with PostgreSQL
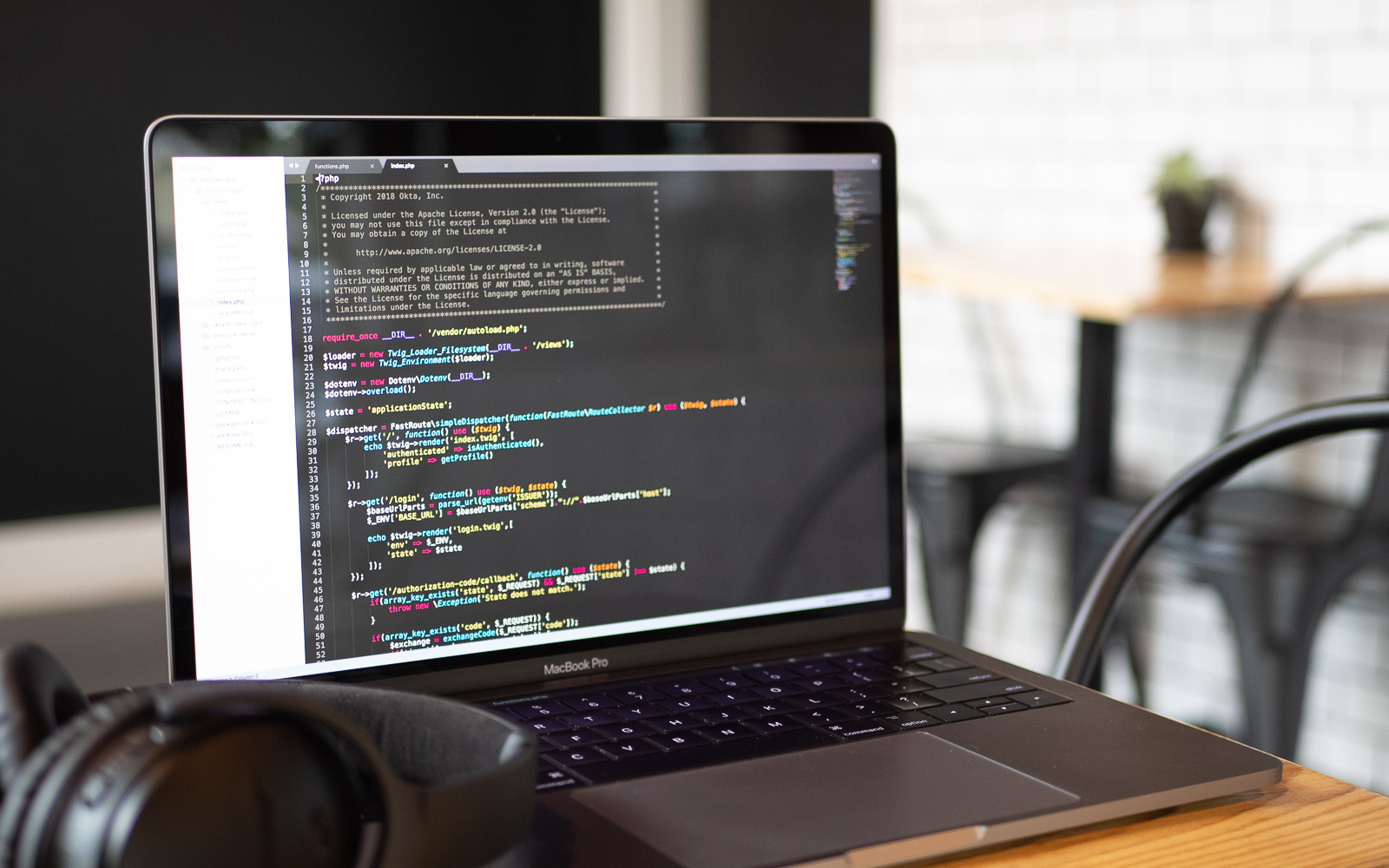
Laravel is one of the hottest frameworks for backend and full-stack development of Web applications today. It boasts a large number of quality features out-of-the-box, but it’s still easy to learn the basics. The community is vast, and there are tons of free resources available on the Internet. This sample application will use Postgres as the backend database system, Okta for user authentication, and Heroku for quick deployment to a scalable platform. Heroku is a...
Build Secure Microservices in PHP
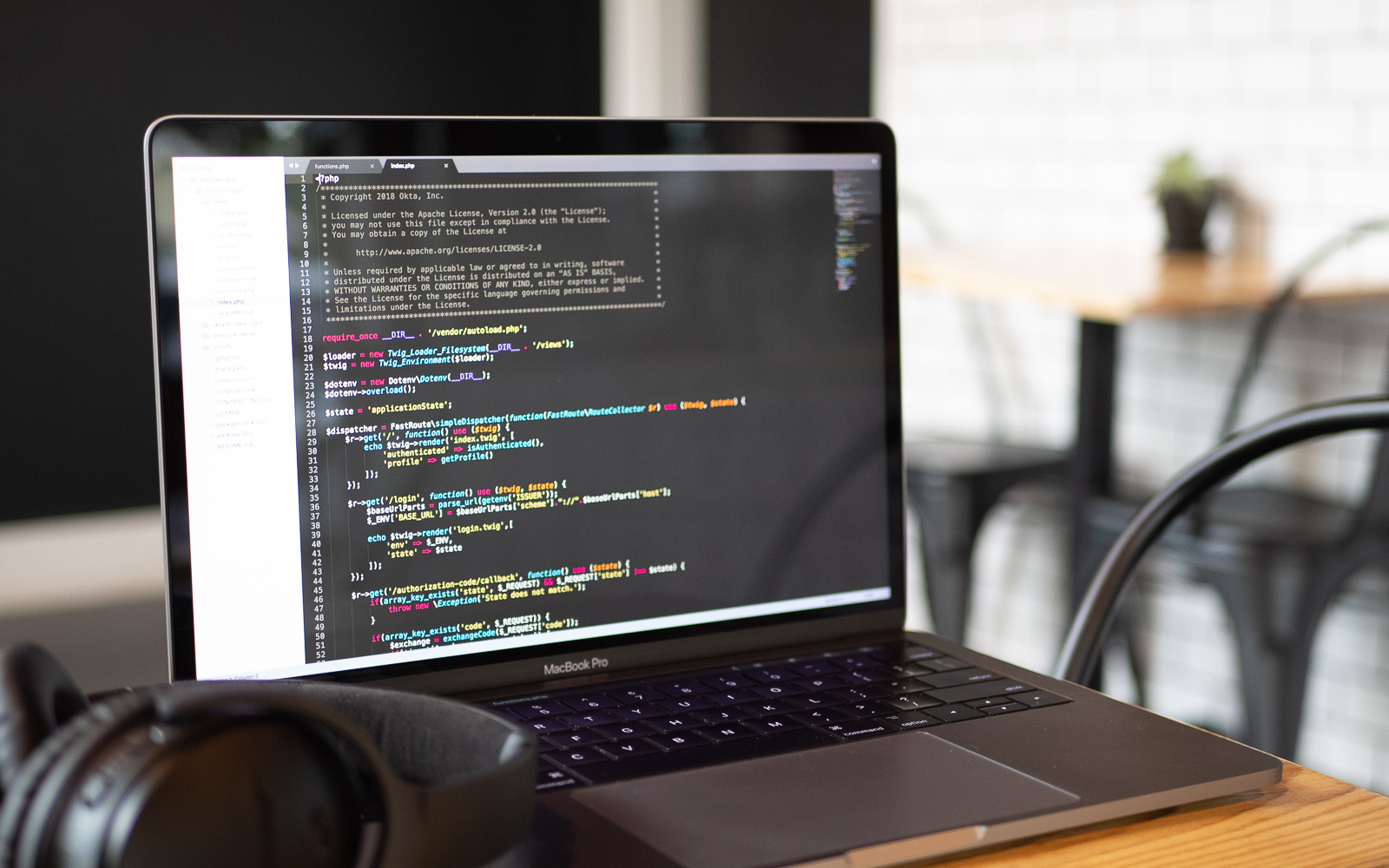
The history of software is a history of improving architectures - from the underlying hardware, OS and virtualization platforms, programming languages and frameworks, to the architecture of the applications we build. The microservice architecture in PHP is a relatively new improvement that’s emerged from the desire of fast-paced companies like Netflix and Amazon to improve their software continually, experiment with different ideas, ship early and ship often. This is difficult to achieve in a traditional...
Token Authentication in PHP
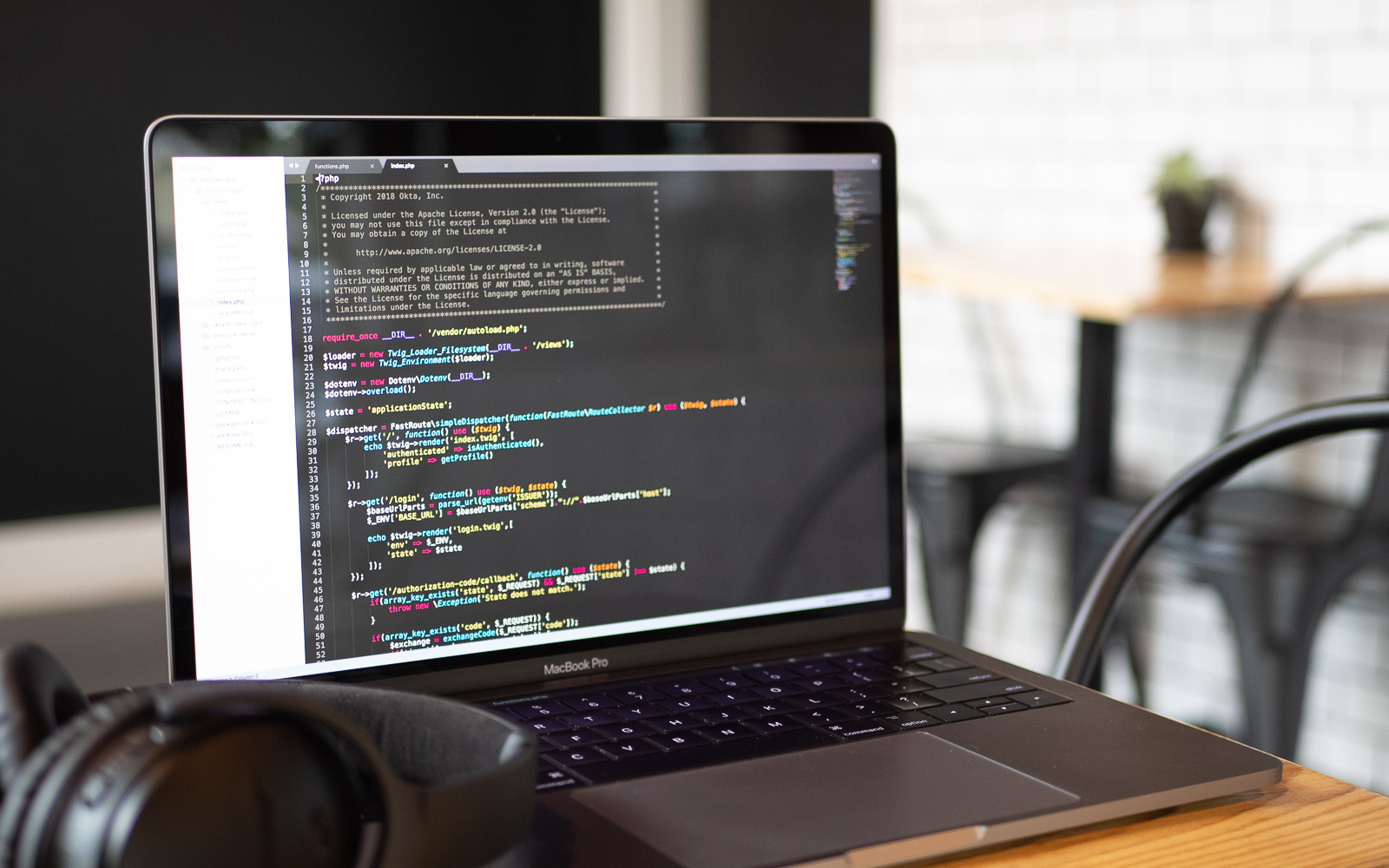
JSON Web Tokens (JWTs) have turned into the de-facto standard for stateless authentication of mobile apps, single-page web applications, and machine-to-machine communication. They have mostly superseded the traditional authentication method (server-side sessions) because of some key benefits: They are decentralized and portable (you can request a token from a dedicated service, and then use it with multiple backends) There is no need for server-side sessions - a JWT can contain all the required information about...
Build a Simple REST API in PHP
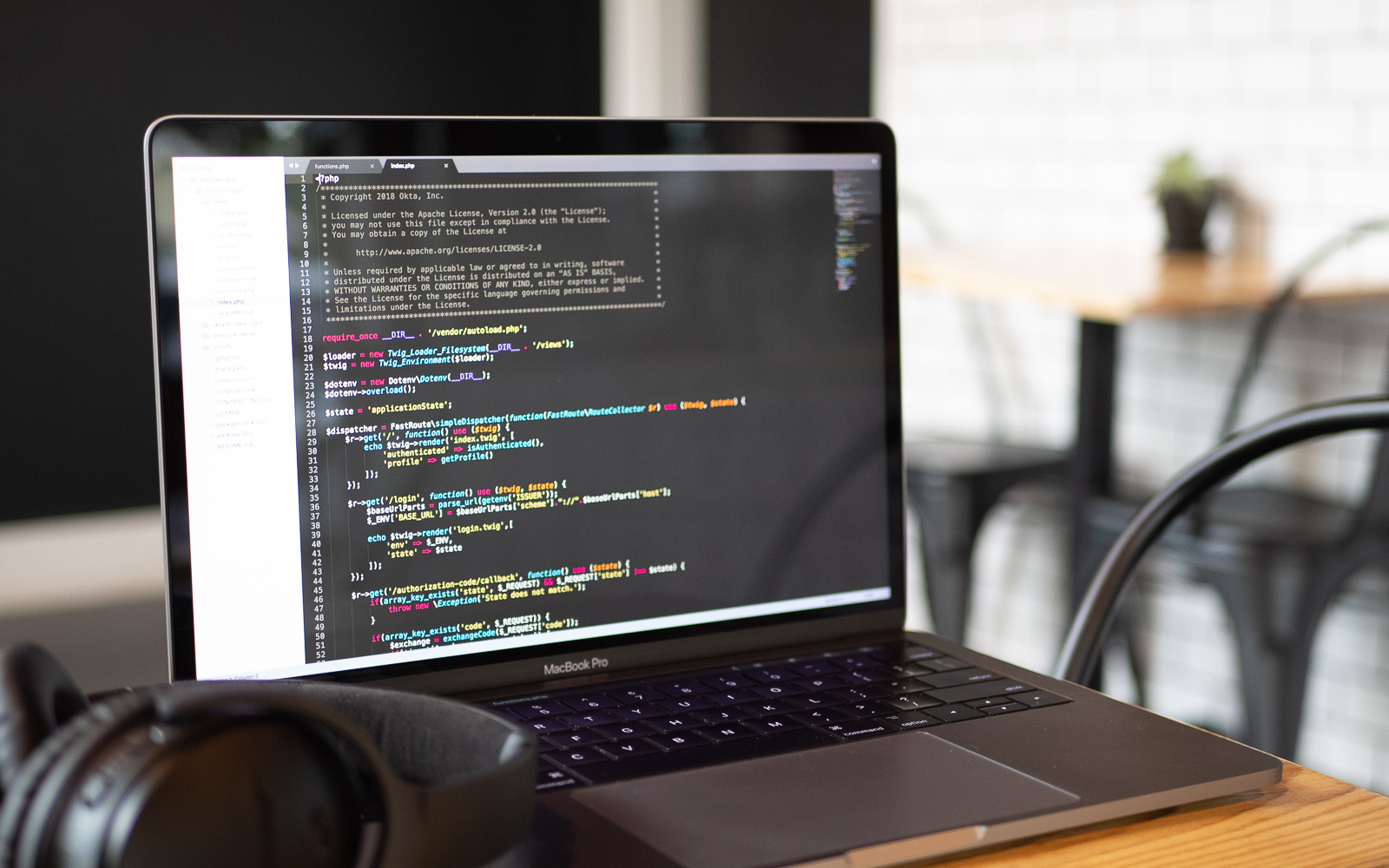
REST APIs are the backbone of modern web development. Most web applications these days are developed as single-page applications on the frontend, connected to backend APIs written in various languages. There are many great frameworks that can help you build REST APIs quickly. Laravel/Lumen and Symfony’s API platform are the most often used examples in the PHP ecosystem. They provide great tools to process requests and generate JSON responses with the correct HTTP status codes....
Add the OAuth 2.0 Device Flow to any OAuth Server
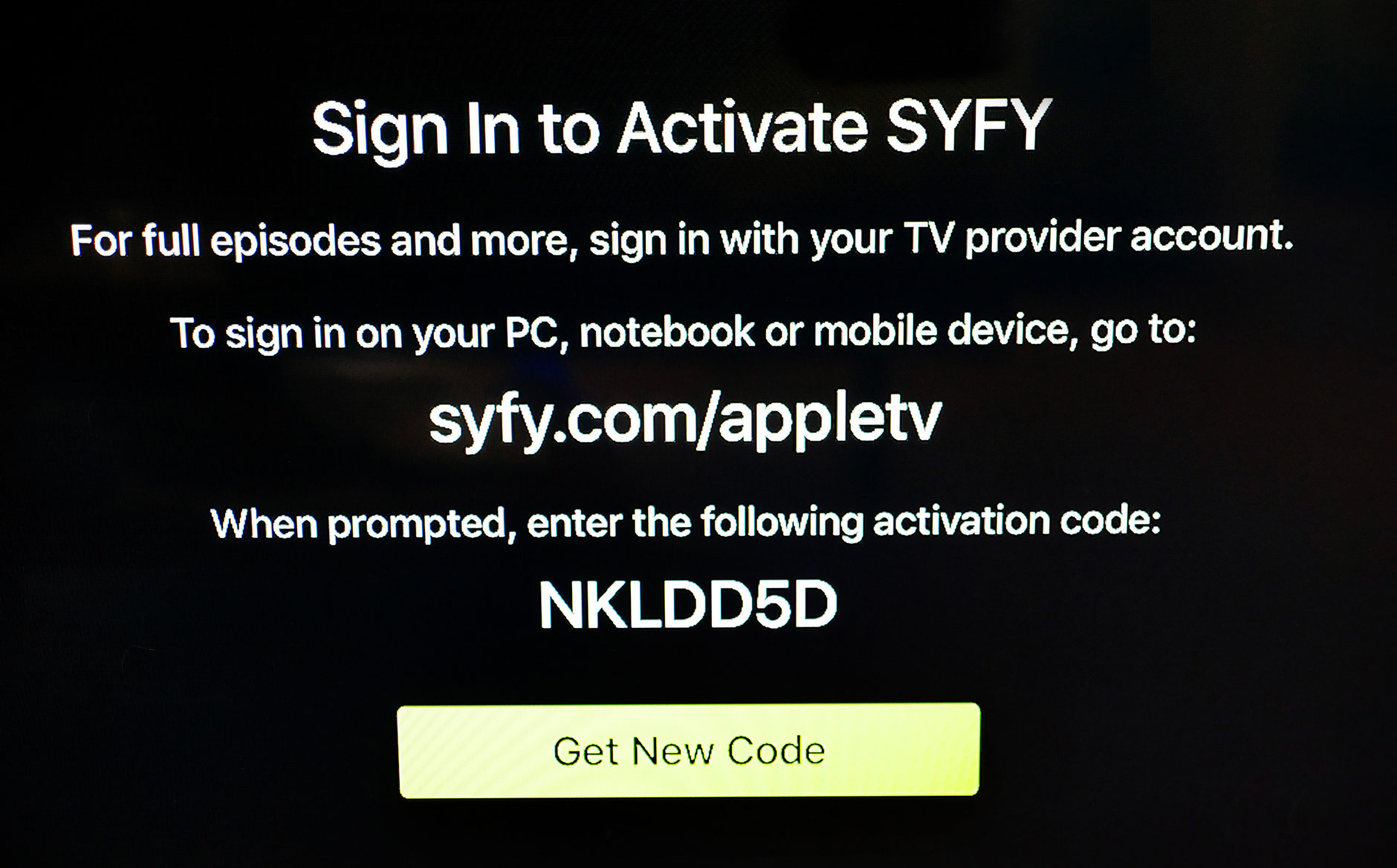
You may not have heard of the Device Flow before, but you’ve probably used it if you have an Apple TV, Roku or Amazon FireTV! The OAuth 2.0 Device Flow is used to log in to a device using OAuth when the device doesn’t have a browser, or also when the device has limited keyboard input ability. The Apple TV is a great device, but it’s missing a browser, which means it can’t do a...
Create and Verify JWTs in PHP with OAuth 2.0
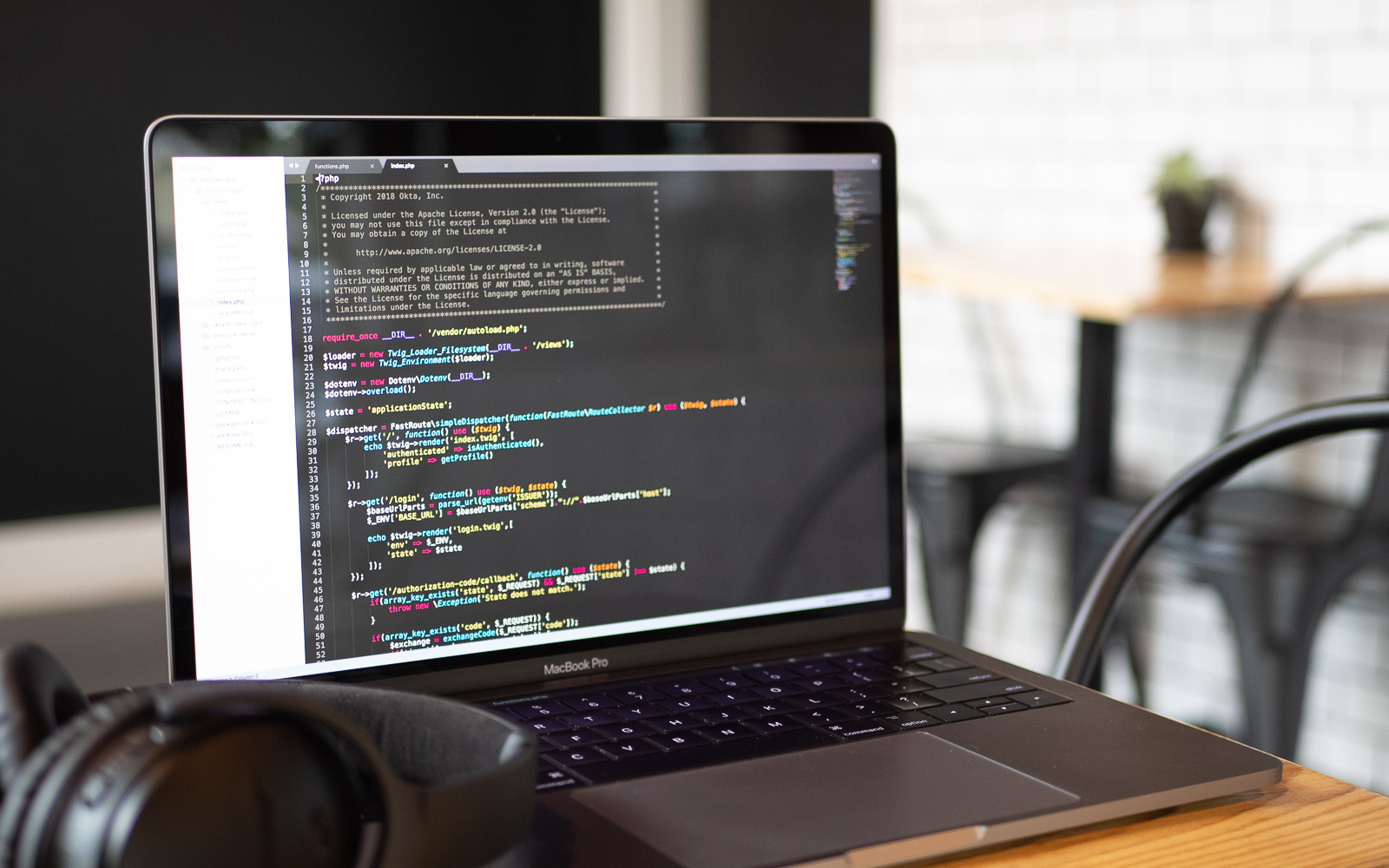
JSON Web Tokens (JWTs) allow you to implement stateless authentication (without the use of server-side sessions). JWTs are digitally signed with a secret key and can contain various information about the user: identity, role, permissions, etc in JSON format. This information is simply encoded and not encrypted. However, because of the digital signature, the payload cannot be modified without access to the secret key. JWTs are a relatively hot topic as they are widely used...
Build a Basic CRUD App with Laravel and Vue
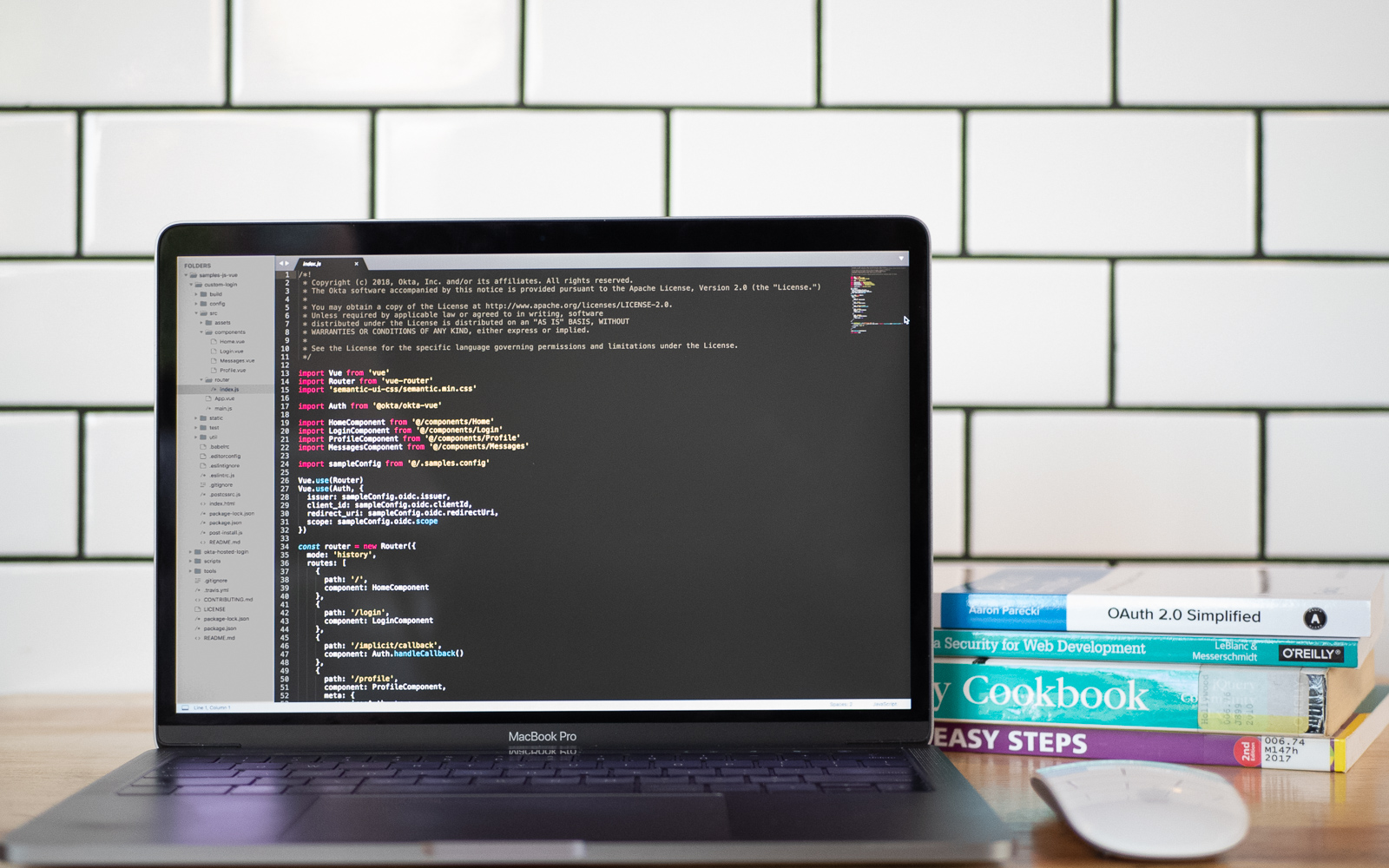
Laravel is one of the most popular web frameworks today because of its elegance, simplicity, and readability. It also boasts one of the largest and most active developer communities. The Laravel community has produced a ton of valuable educational resources, including this one! In this tutorial, you’ll build a trivia game as two separate projects: a Laravel API and a Vue frontend (using vue-cli). This approach offers some important benefits: It allows you to separate...
Build Simple Login in PHP
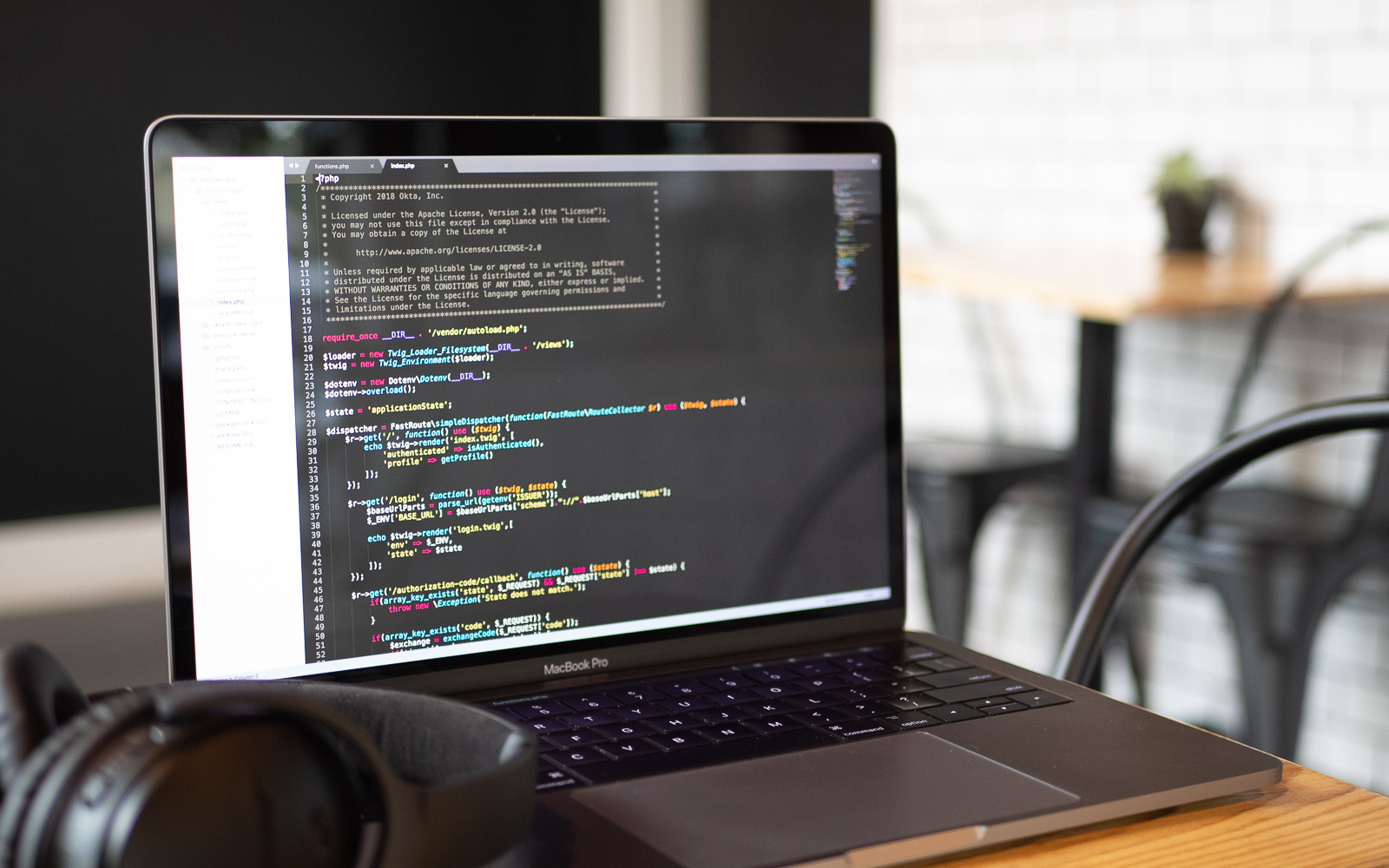
Building a user authentication system for your Web application from scratch can be a deceptively tricky job. It seems easy at first, but there are so many details you have to consider - hashing the passwords properly, securing the user sessions, providing a way to reset forgotten passwords. Most modern frameworks offer boilerplate code for dealing with all of these issues but even if you’re not using a framework, do not despair. In this article,...
Build a Basic CRUD App with Laravel and React
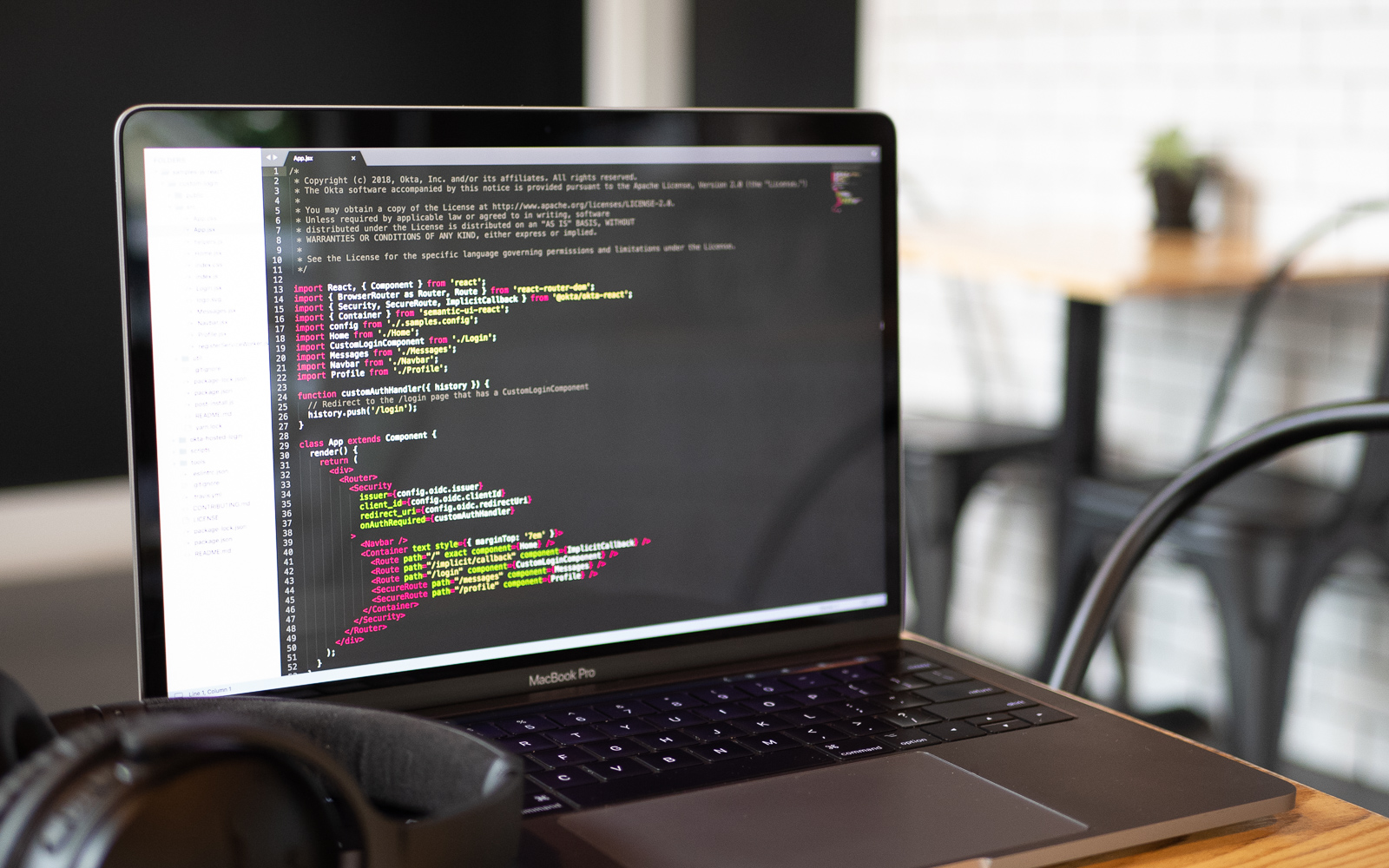
Laravel is an amazing web application framework which regularly tops the lists of best PHP frameworks available today. This is partly because its based on PHP which runs 80% of the web today and the learning curve is relatively small (despite it being packed with advanced features, you can understand the basic concepts easily). However, the real reason for its popularity is its robust ecosystem and abundance of high-quality learning resources available for free (like...
Tutorial: Build a Basic CRUD App with Laravel and Angular
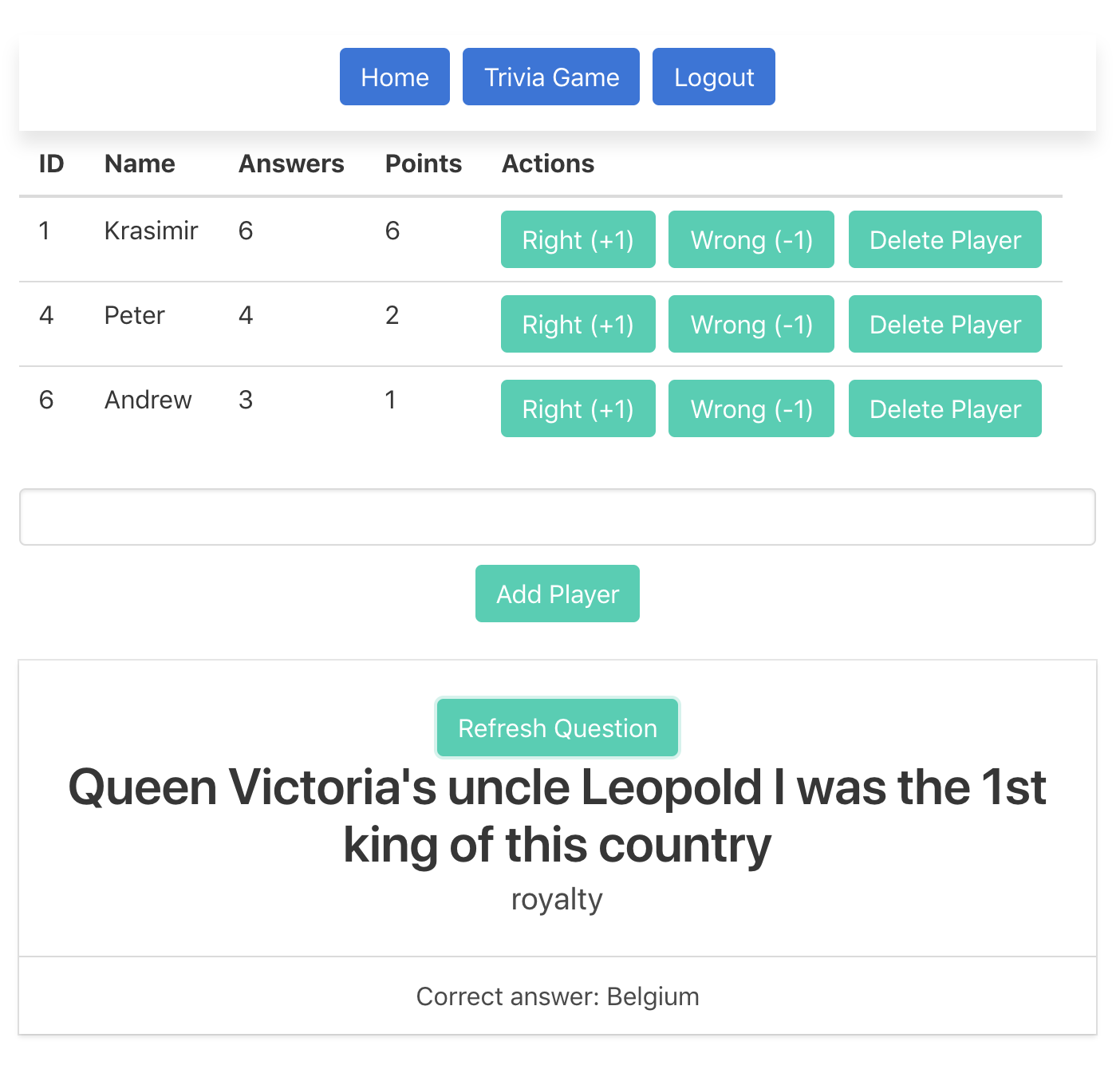
Laravel is a popular PHP framework for Web application development and it’s a pretty good choice if you’re starting a new project today for multiple reasons: Laravel is a well-architectured framework that’s easy to pick up and write elegant code, but it’s powerful as well. It contains many advanced features out-of-the-box: Eloquent ORM, support for unit/feature/browser tests, job queues, and many more. There’s an abundance of great learning resources and it boasts one of the...
Tutorial: Build a Secure CRUD App with Symfony and React
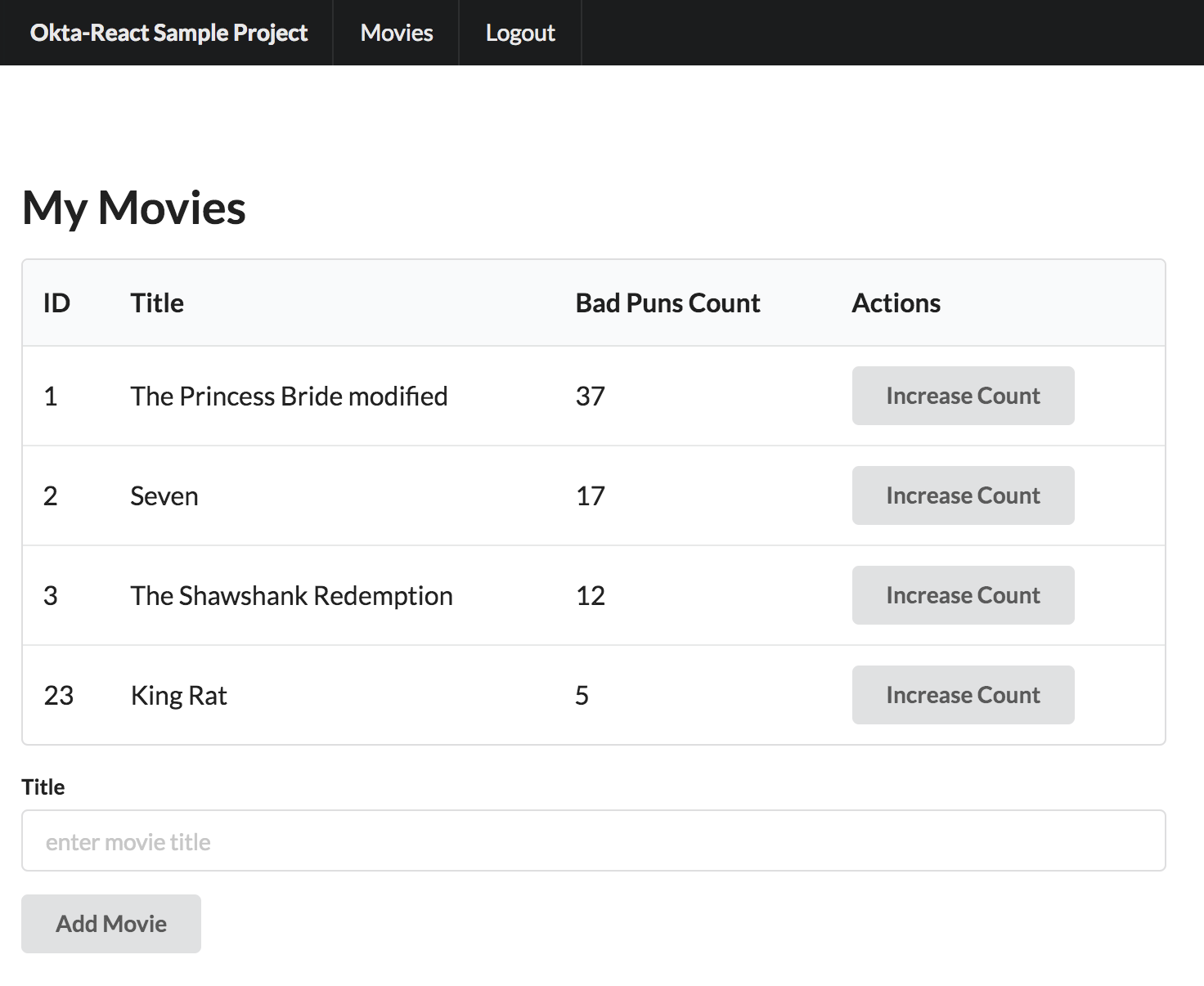
Building a modern single-page application can be a daunting task for a sole developer because of the sheer amount of different components you need to get in place – you need a backend API, a dynamic frontend, a decent user interface, and everything has to be secure and scalable. However, with the right tools in place, you can get started quickly without compromising quality or performance. Today I’ll show you how to create an app...
Tutorial: Build Your First CRUD App with Symfony and Angular
Building a web application isn’t supposed to be drudgery. No developer has ever said “I’d really like to spend two hours configuring webpack and TypeScript this weekend.” You’d rather build cool stuff NOW and spend time thinking about your applications, not the tools you’re forced to use. In a lot of cases the “cool stuff” is a dynamic, fast, secure single-page app. To achieve that, in this tutorial I’ll show you how to get a...
OAuth 2.0 from the Command Line
So you’ve found yourself writing a command line script and needing to talk to an API that uses OAuth 2.0? The typical approaches to getting an OAuth access token from a command line script usually involve copying and pasting the authorization code into the terminal. But we can do better! In this tutorial, I’ll show you how to write a command line script which is able to complete the OAuth exchange all without any copying...
Add Authentication to your PHP App in 5 Minutes
Have you ever found yourself building an app and needing to add authentication, dreading the thought of setting up yet another username and password database? In this post, I’ll show you how easy it is to use Okta to add authentication to a simple PHP app in 5 minutes. By leveraging Okta’s simple OAuth API, we can breeze past most of the challenges involved in authenticating users by letting Okta take care of the hard...
Tutorial: Build a Basic CRUD App with Symfony 4 and Vue
If you’re a web developer in 2018, then you already know that the expectations are high and the tools are many. Users want progressive web applications and seamless experiences across every device. The focus is shifting from monolithic code to APIs built as microservices and consumed by multiple frontends, and finding our way through the ecosystem of ever-changing tools can be a daunting task even for the most experienced of us. If you’re looking for...