Get Familiar with Android and Gradle
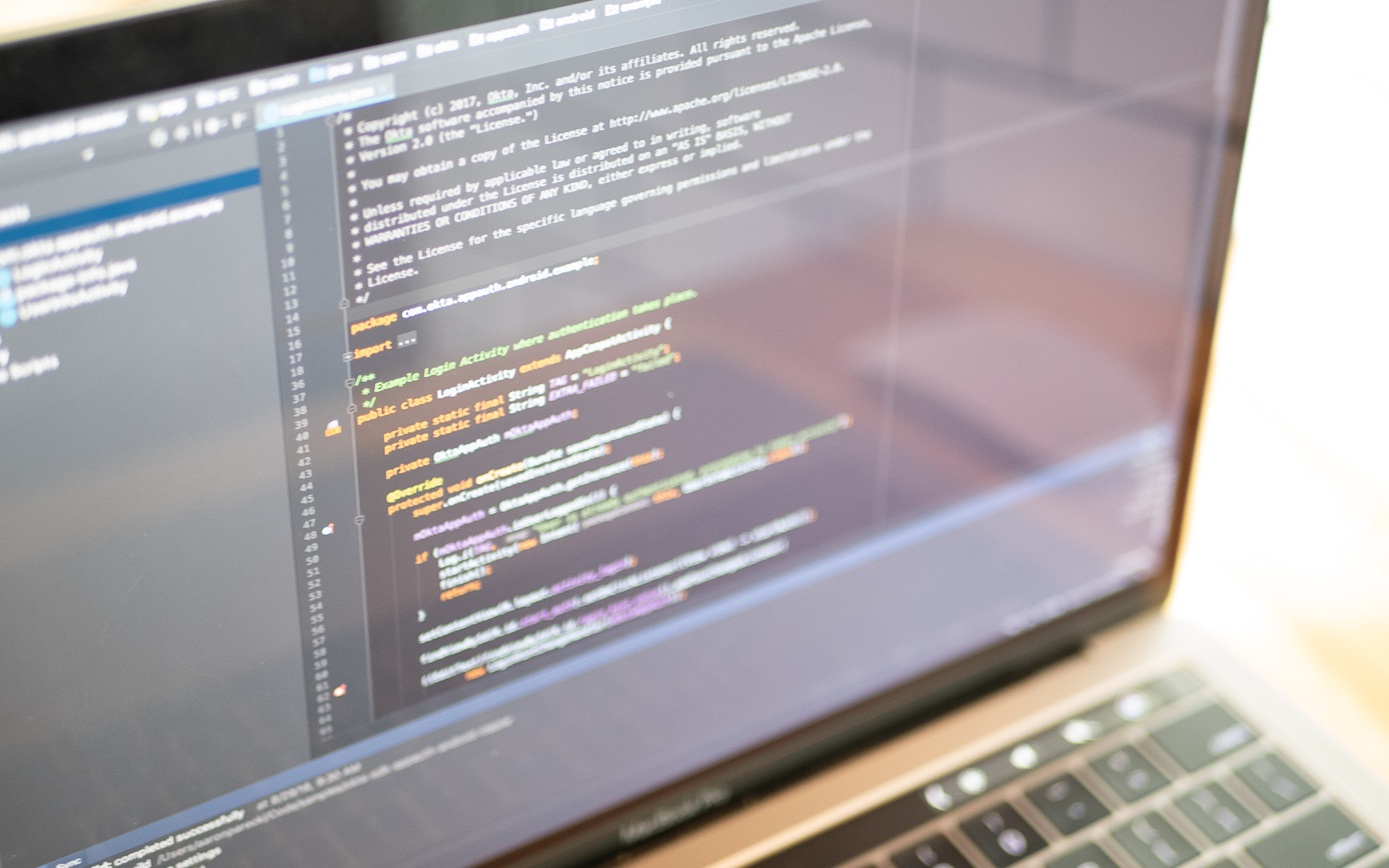
Interested in Android development? Then you should get familiar with Gradle, the only Android development build system that Google officially supports. Gradle manages all aspects of the Android development process, making it easy to: Compile your code Solve dependency trees and conflicts between libraries Merge your code and resources with those of your libraries Cache resources for compilation performance Connect it all to the Android SDK Finally packaging it into the right format with the...
The Dangers of Self-Signed Certificates
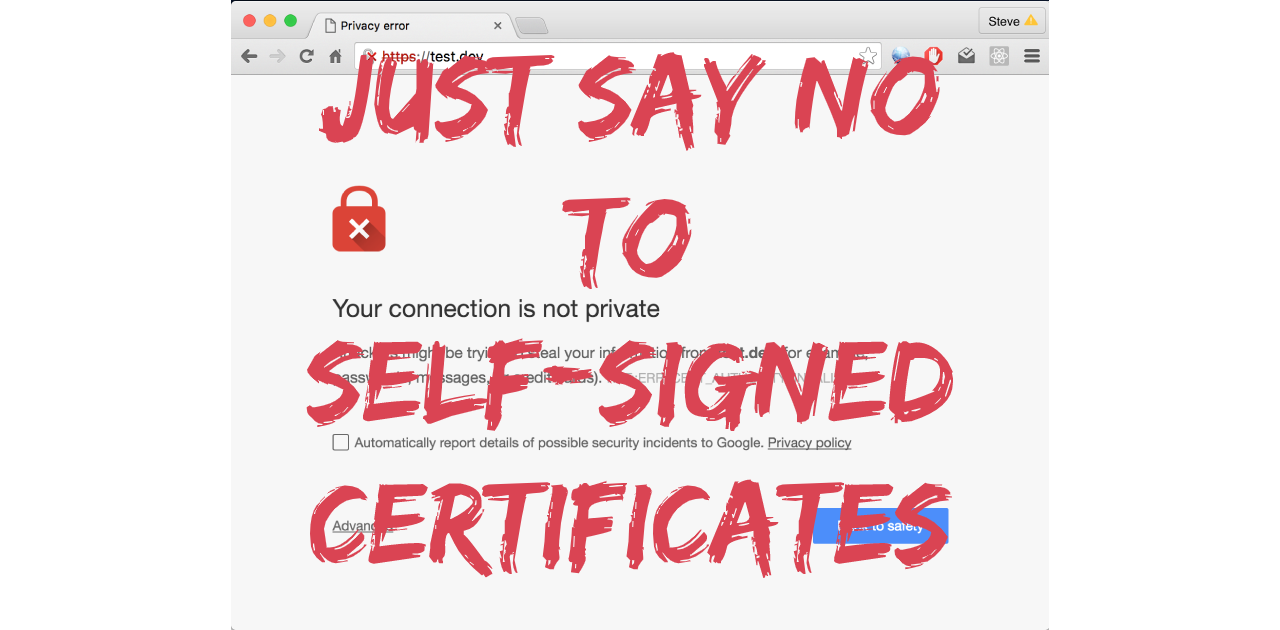
How many times have you started a new job, and the first thing you see on the company intranet is a “Your connection is not private” error message? Maybe you asked around and were directed to a wiki page. Of course, you probably had to click through the security warnings before actually viewing that page. If you are security-minded, this probably bothers you, but because you have a new job to do, you accept the...
Get to Know Entity Framework and PostgreSQL
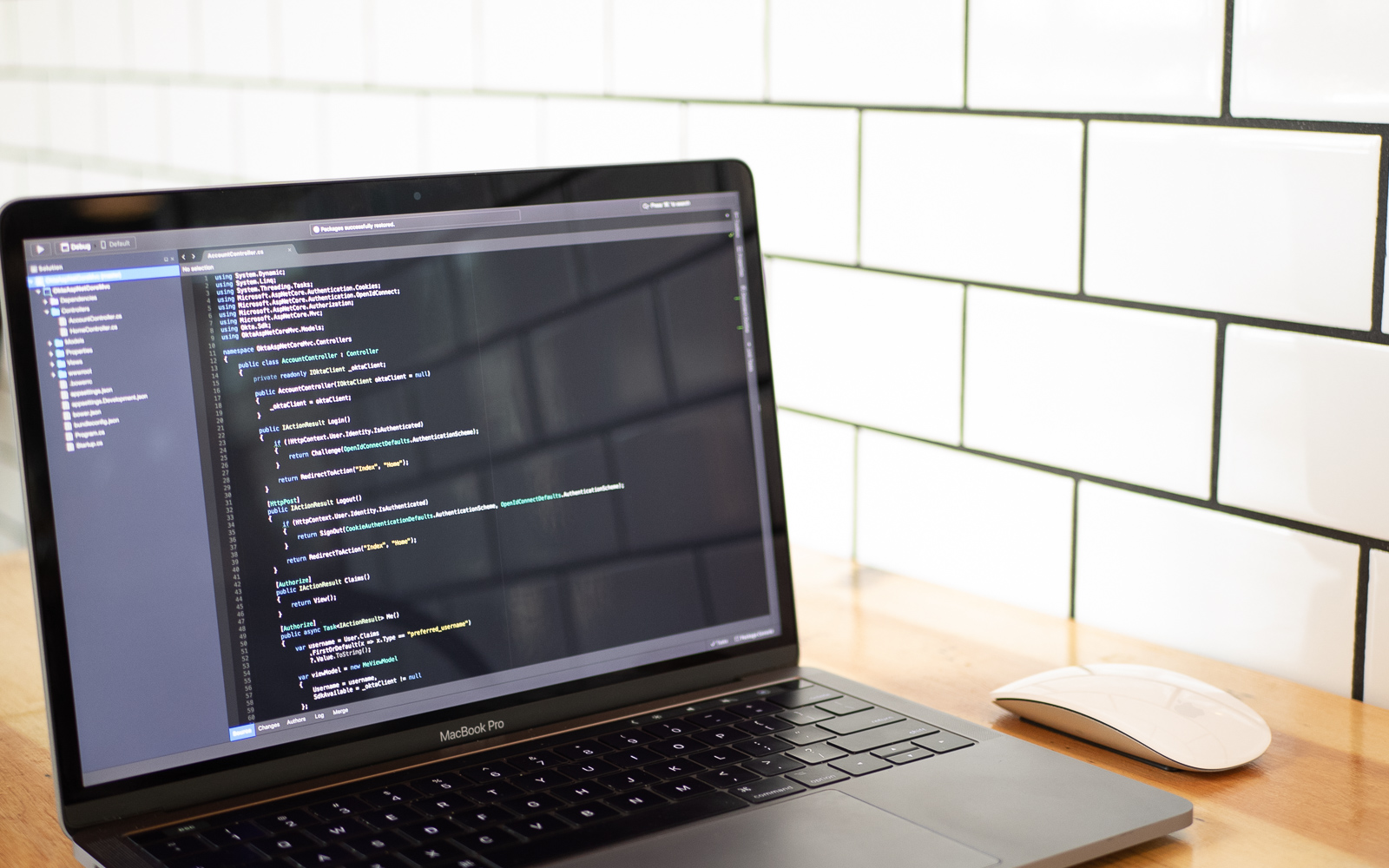
Entity Framework is one of the most pervasive Object-Relational Mappers (ORMs) for ASP.NET. An ORM maps an application’s object entities to relational entities in a database, and allows developers to build and edit the database schema from the code. Furthermore, Entity Framework’s design makes it particularly friendly for PostgreSQL developers. Entity Framework (EFCore) Core is a lighter weight and more flexible version that specifically enables .NET objects. It reduces the amount of data access code...
An Illustrated Guide to OAuth and OpenID Connect
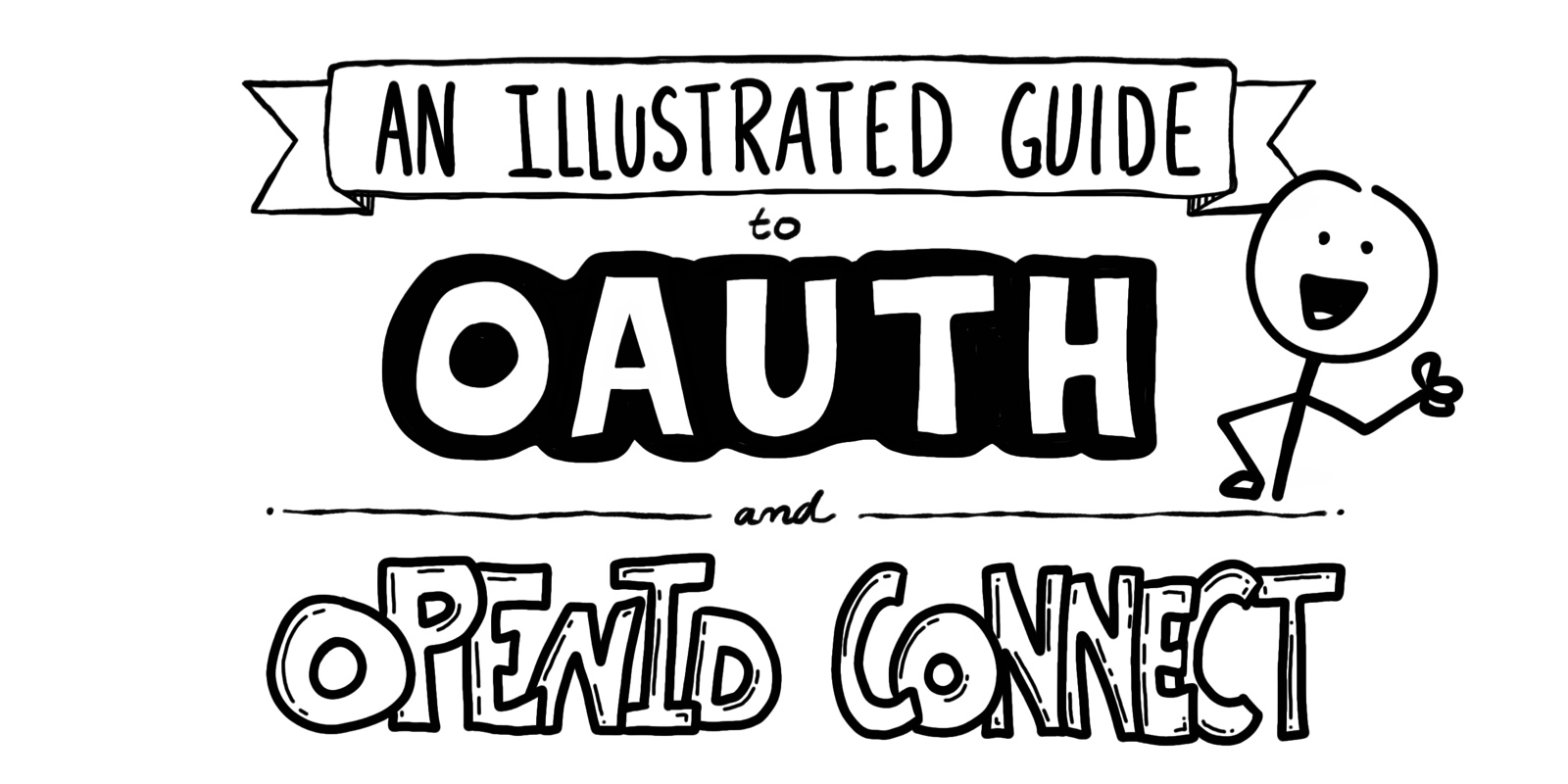
In the “stone age” days of the Internet, sharing information between services was easy. You simply gave your username and password for one service to another so they could login to your account and grab whatever information they wanted! Yikes! You should never be required to share your username and password, your credentials, to another service. There’s no guarantee that an organization will keep your credentials safe, or guarantee their service won’t access more of...
A Thorough Introduction to PASETO
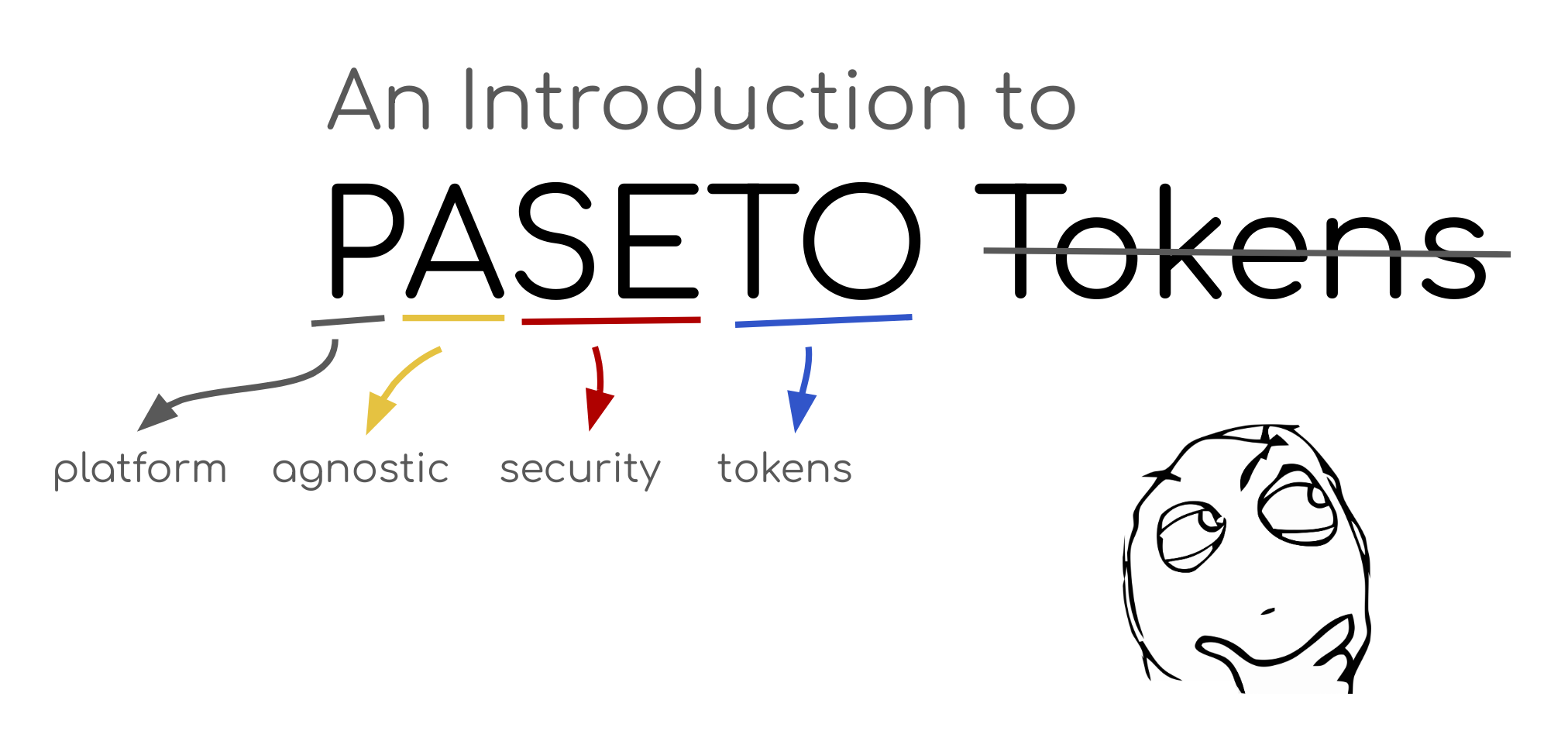
Today I’m going to introduce you to one of my favorite pieces of security technology released in the last several years: PASETO (platform-agnostic security tokens). PASETO is a relatively new protocol, designed by Scott Arciszewski in early 2018 that is quickly gaining adoption in the security community. While PASETO is still a young technology, I thought it’d be interesting to take an in-depth look at it, since it’s both incredibly useful and solves a lot...
Goodbye Javascript! Build an Authenticated Web App in C# with Blazor + ASP.NET Core 3.0
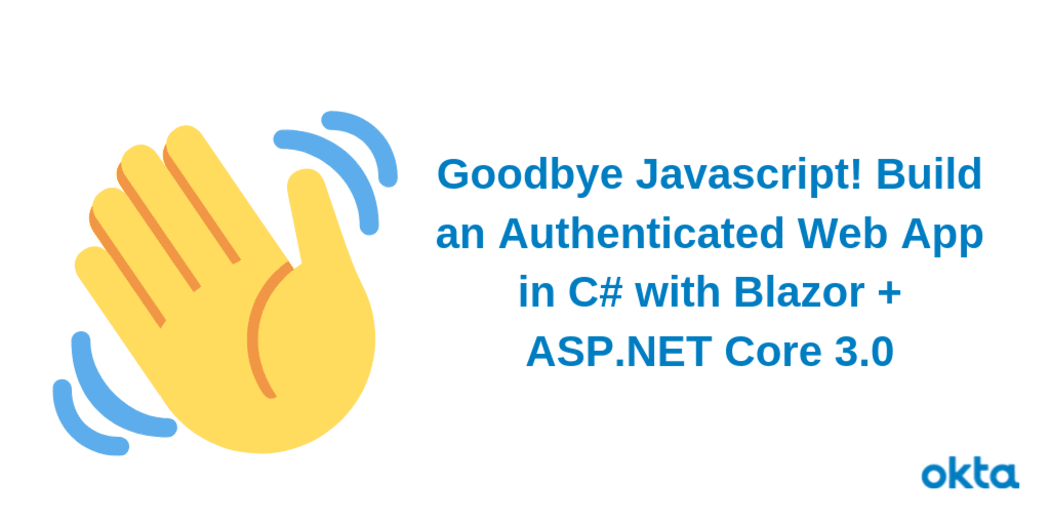
Curious what the experience would be like to trade in Javascript for C# on the front end? You are about to find out! For many years, Javascript (and it’s child frameworks) have had their run of the DOM (Document Object Model) in a browser, and it took having that scripting knowledge to really manipulate client-side UI. About 2 years ago, all of that changed with the introduction of Web Assembly - which allows compiled languages...
Performance Testing with Apache Bench
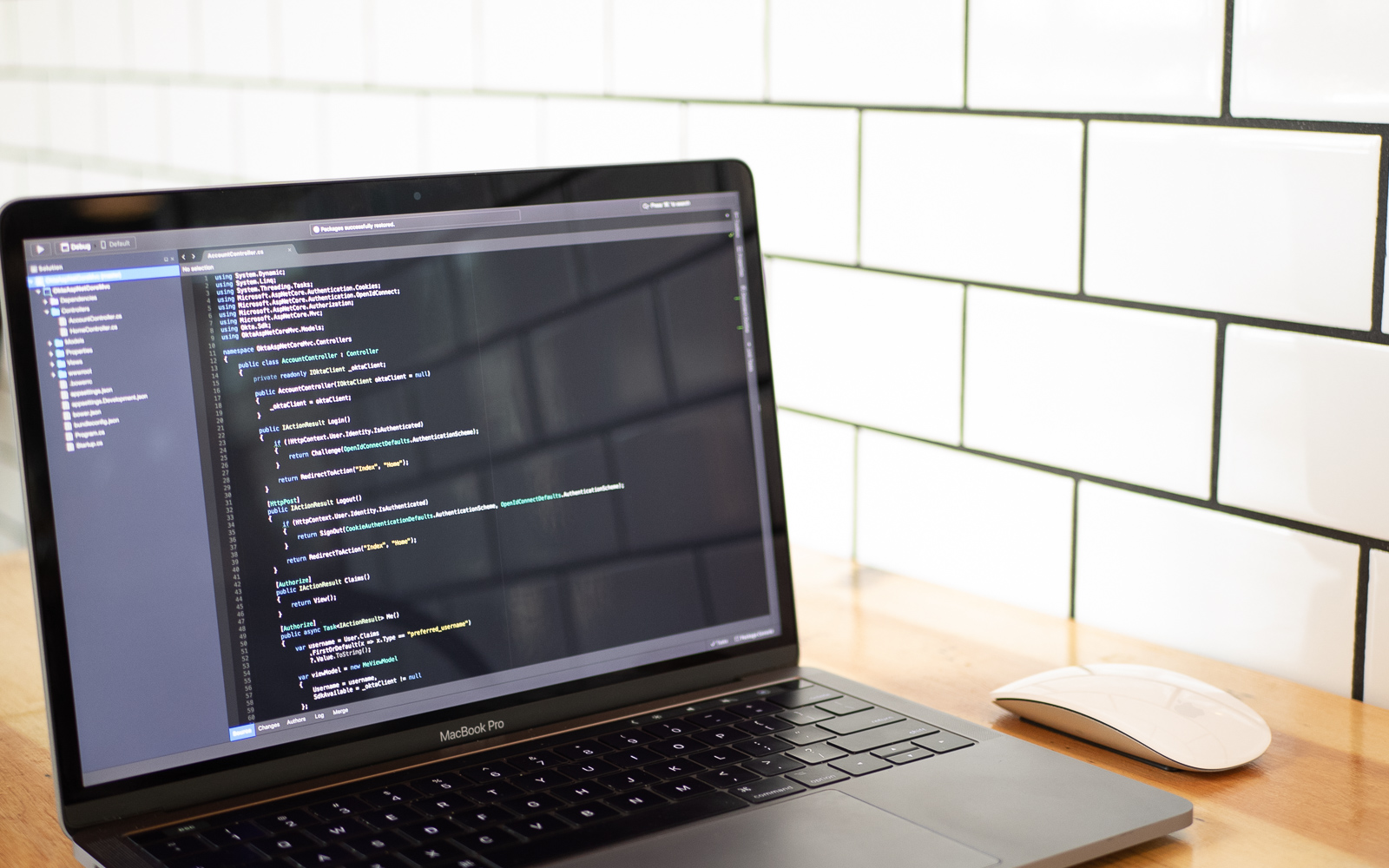
Every web developer I know is concerned with the performance of their web applications. How fast will my app load? Will it handle a lot of users? How well will it lots of data? Will it scale? These are just some of the questions that developers ask themselves but very rarely have any idea how to test. Generally, when performance testing, most developers worry about “load testing”, or how an app will perform under load....
Tutorial: Develop Apps with Secure WebSockets in Java
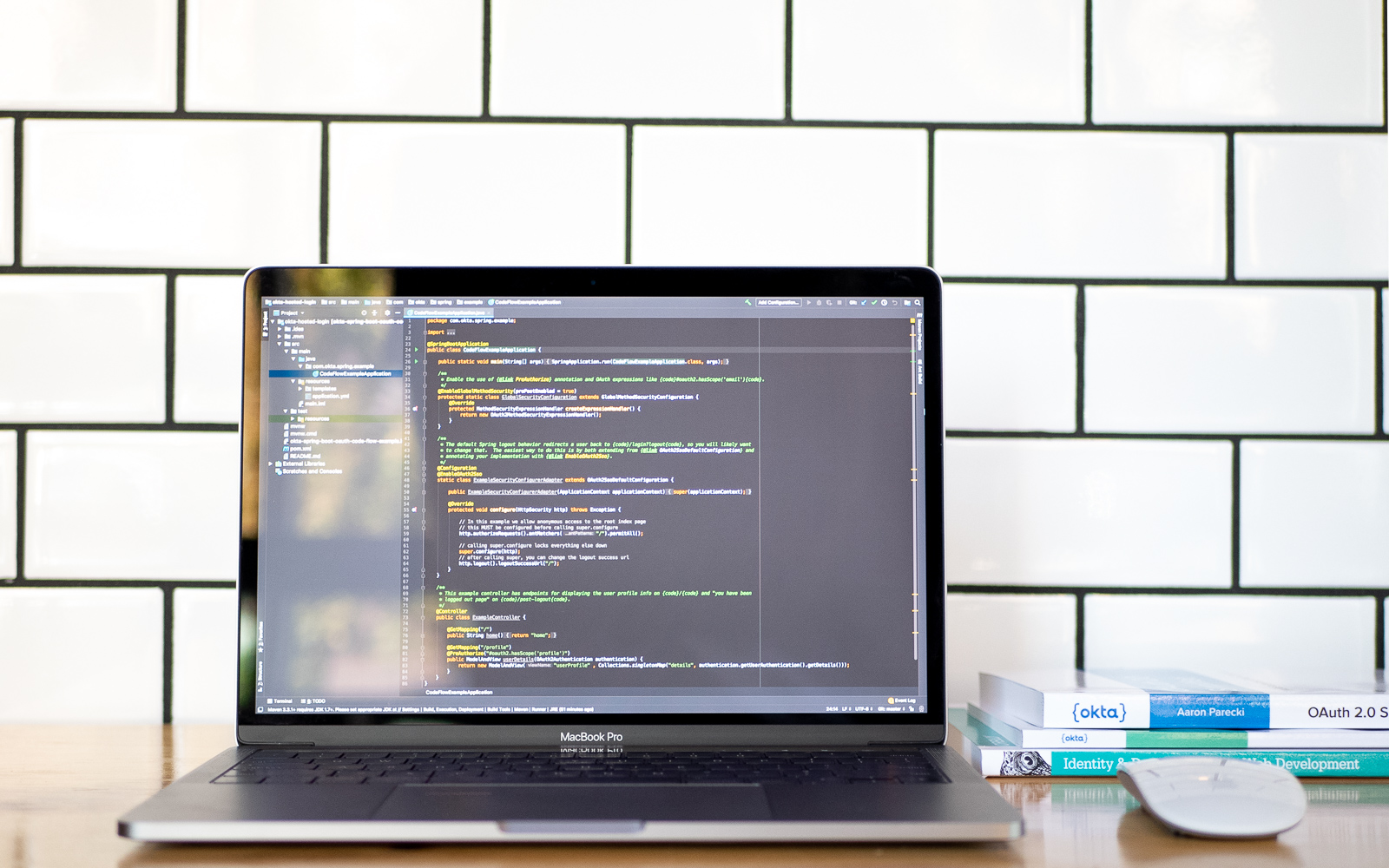
WebSockets is a modern transport layer technology that establishes a two-way communication channel between a client and a server, perfect for low-latency, high-frequency interactions. WebSockets tend to be used in collaborative, real-time or event-driven applications, where traditional client-server request-response architecture or long polling would not satisfy requirements. Use cases include stock trading and shared dashboard applications. In this tutorial, I’ll give you a quick overview of the WebSockets protocol and how it handles messages with...
Secure and Scalable: An Introduction to JAMStack
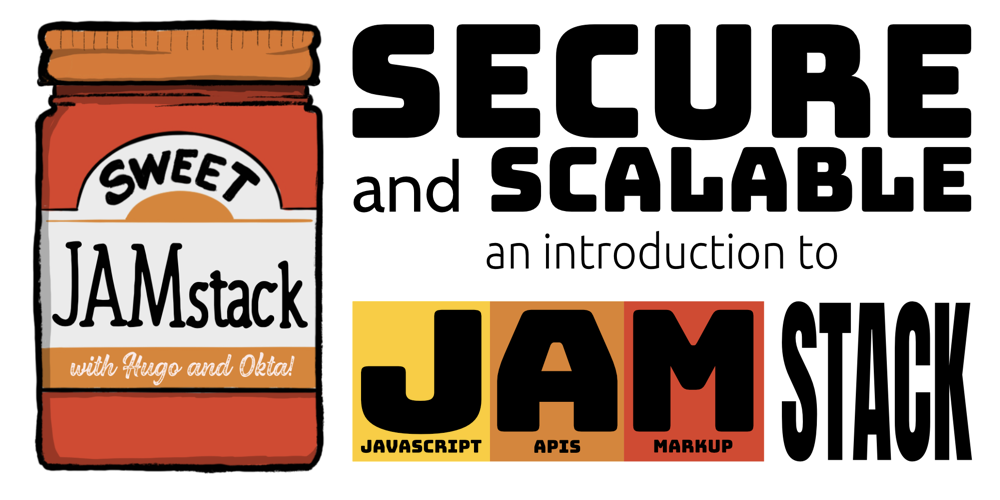
If you’re a web developer, chances are you have heard the term “JAMstack.” Curiously, JAMstack isn’t a solution to prevent clogged printers, something to eat on toast, or a way to make music. However, by the time you finish reading this tutorial, you’ll understand JAMstack and its benefits, and learn one approach to implementing JAMstack for yourself. Let’s get ready to JAM. The “JAM” in JAMstack stands for JavaScript, APIs, and Markup. JAMstack’s pattern of...
Painless Node.js Authentication
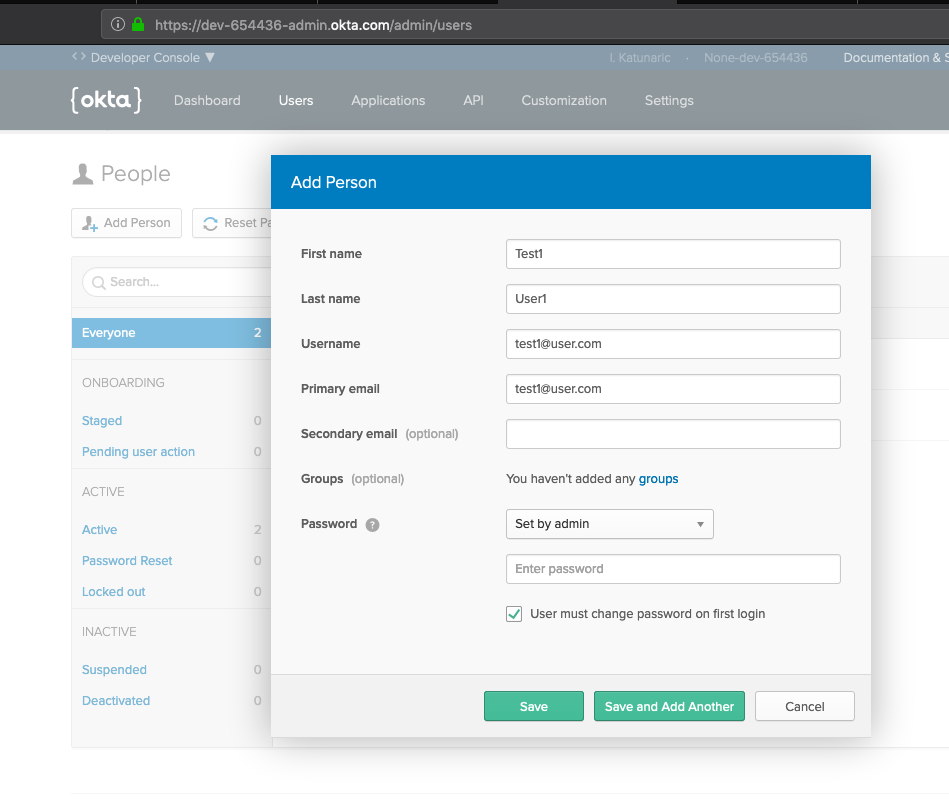
User authentication is a critical component of just about every web application. Unfortunately, while authentication is a core part of all websites, it can still be difficult to get right. Despite the Node.js community being around for a while, there still aren’t a lot of simple, foolproof ways to authenticate users in Node.js applications. In this article I’m going to explain how to build a Node.js application that authenticates users in a best practices way....