Articles tagged spring-security
Get Started with Spring Boot and SAML
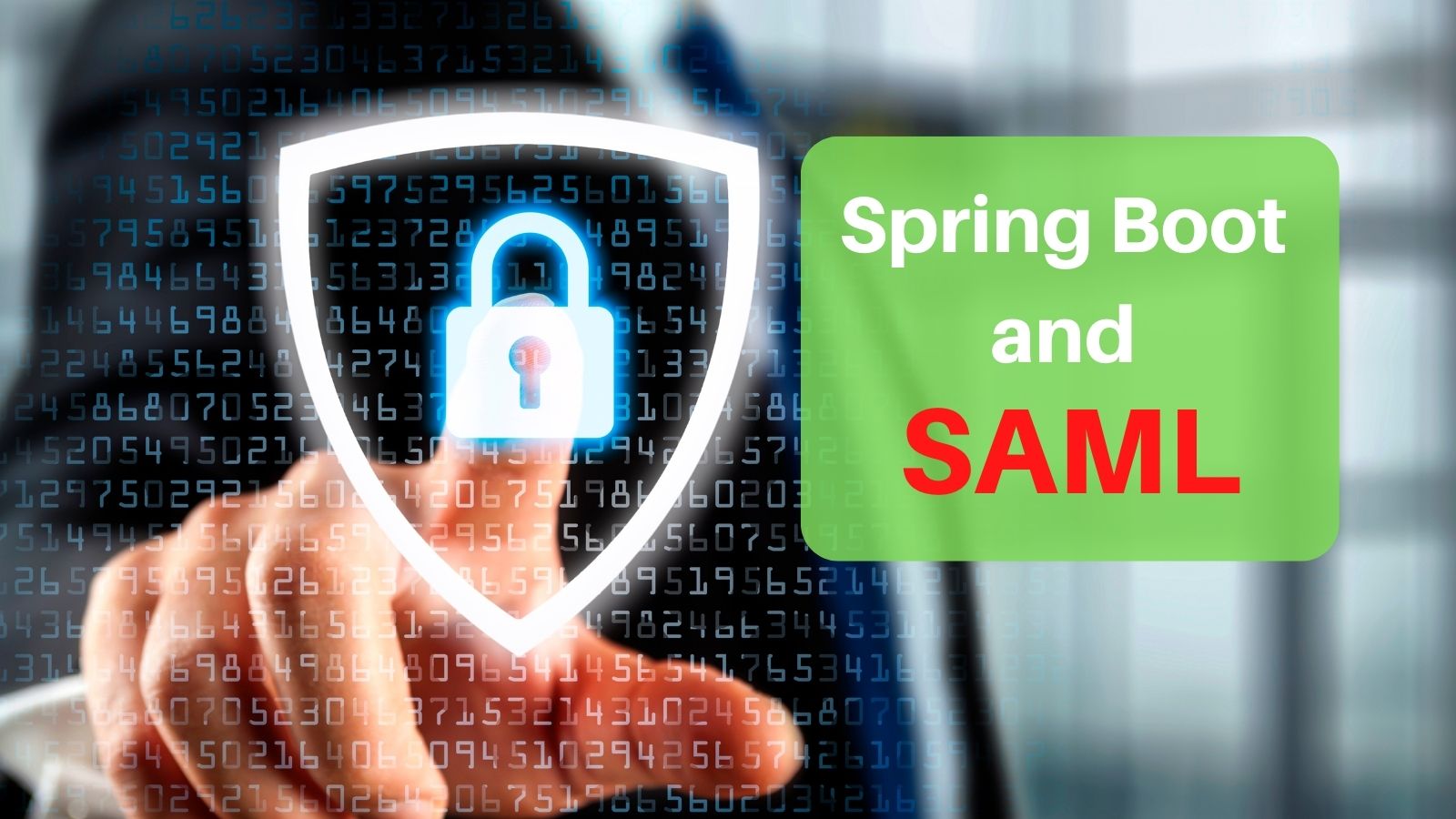
Spring is a long-time friend to enterprise companies throughout the world. When Spring Boot came along in 2014, it greatly simplified configuring a Spring application. This led to widespread adoption and continued investment in related Spring projects. One of my favorite Spring projects is Spring Security. In most cases, it simplifies web security to just a few lines of code. HTTP Basic, JDBC, JWT, OpenID Connect/OAuth 2.0, you name it—Spring Security does it! You might...
Build Secure Ionic Apps with Angular and JHipster
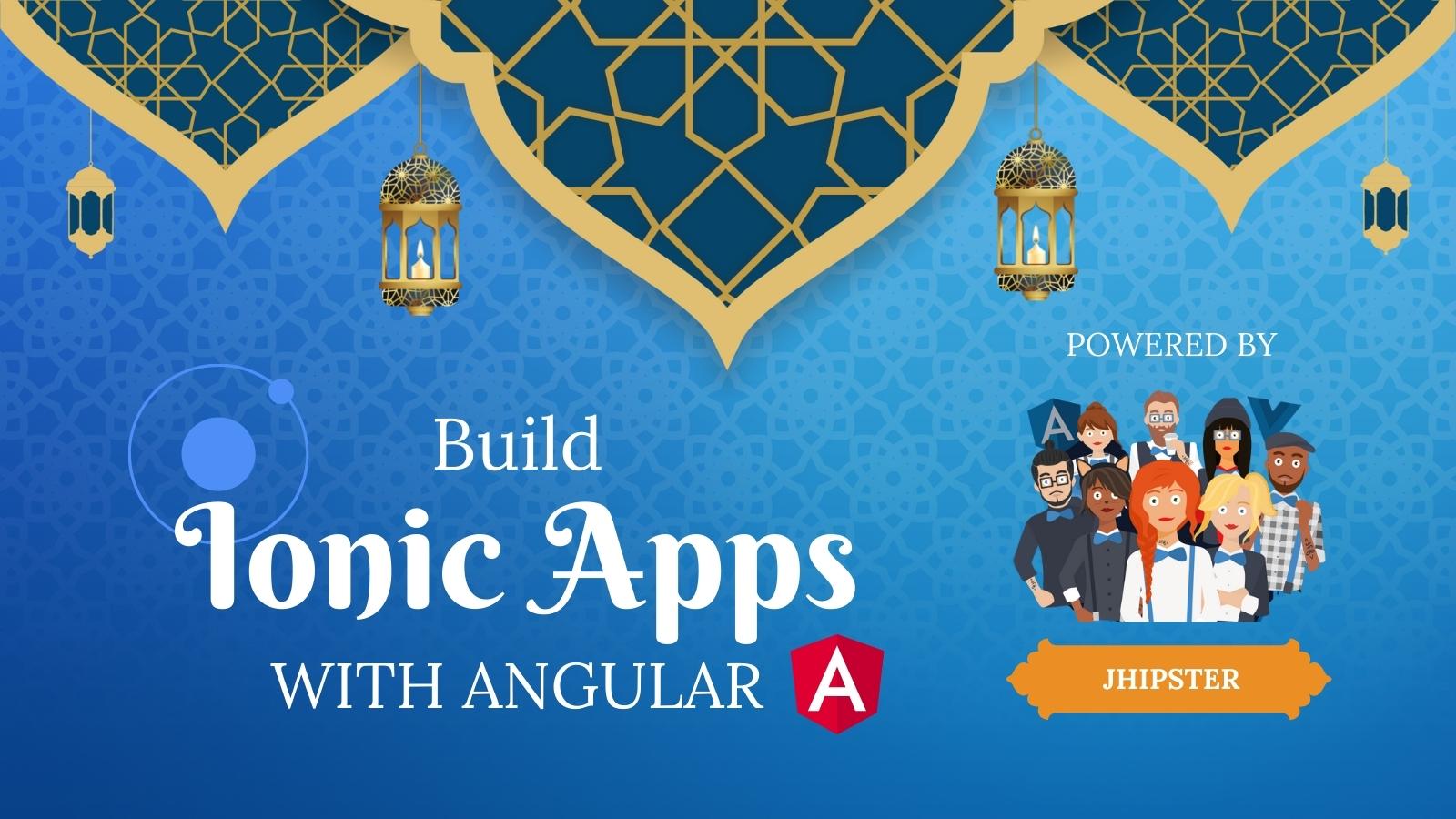
Ionic is a framework for building mobile apps with web technologies that look and act like native apps. Because they’re built with web technologies (HTML, JavaScript, and CSS), you can also deploy your Ionic apps as single-page applications. Or, even better, as progressive web apps (PWAs) that work offline. Ionic supports the big three web frameworks: Angular, React, and Vue. Once you’ve written your app, you can deploy it to a simulator or device with...
Use Thymeleaf Templates with Spring WebFlux to Secure Your Apps
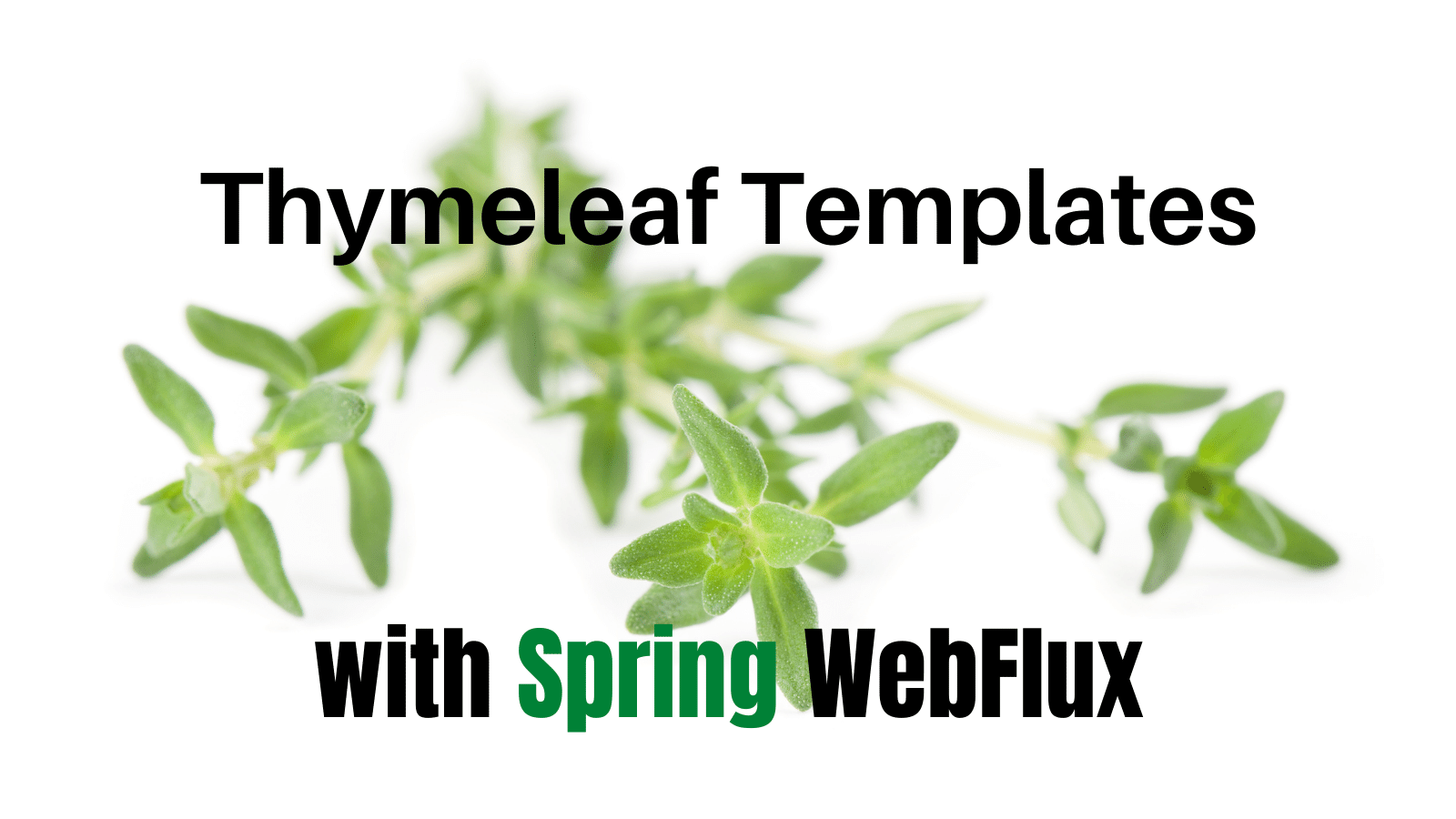
The Thymeleaf library has been around at least for 10 years and it is still actively maintained as of today. It is designed to allow stronger collaboration between design and developer teams for some use cases, as Thymeleaf templates look like HTML and can be displayed in the browser as static prototypes. In this tutorial you will learn how to create a simple Spring WebFlux application with Thymeleaf and Okta OIDC authentication, addressing the security...
Better Integration Testing With Spring Cloud Contract
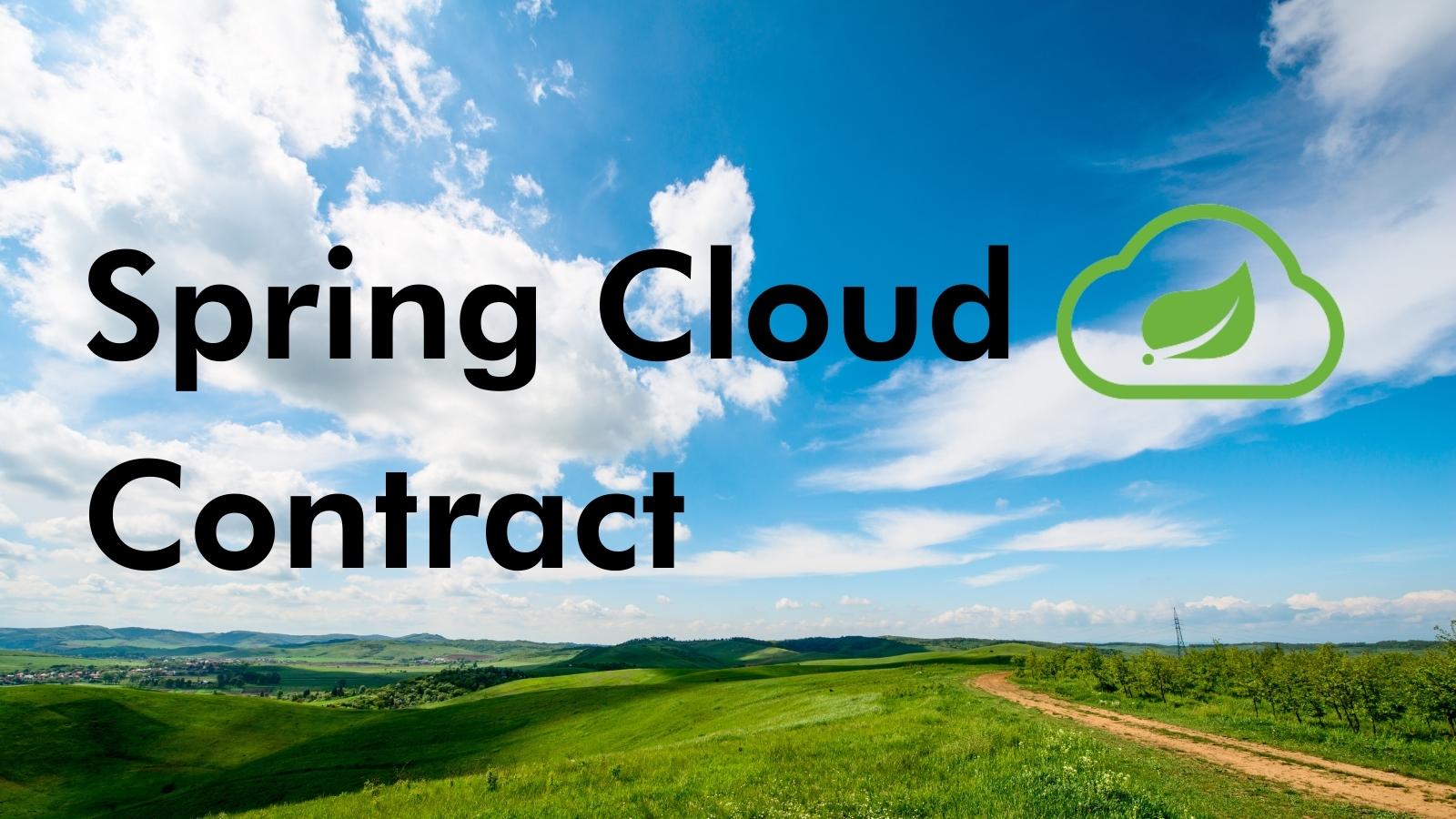
Spring Cloud Contract was created as a way to help test interconnected microservices. Generally speaking, there are two options when testing microservices: 1) you can deploy the entire mesh of services in your integration tests and test against that, or 2) you can mock each service in your integration tests. Both of these options have serious drawbacks. The first, deploying the entire mesh of microservices for testing, has the obvious drawback of being difficult, if...
How to Prevent Reactive Java Applications from Stalling
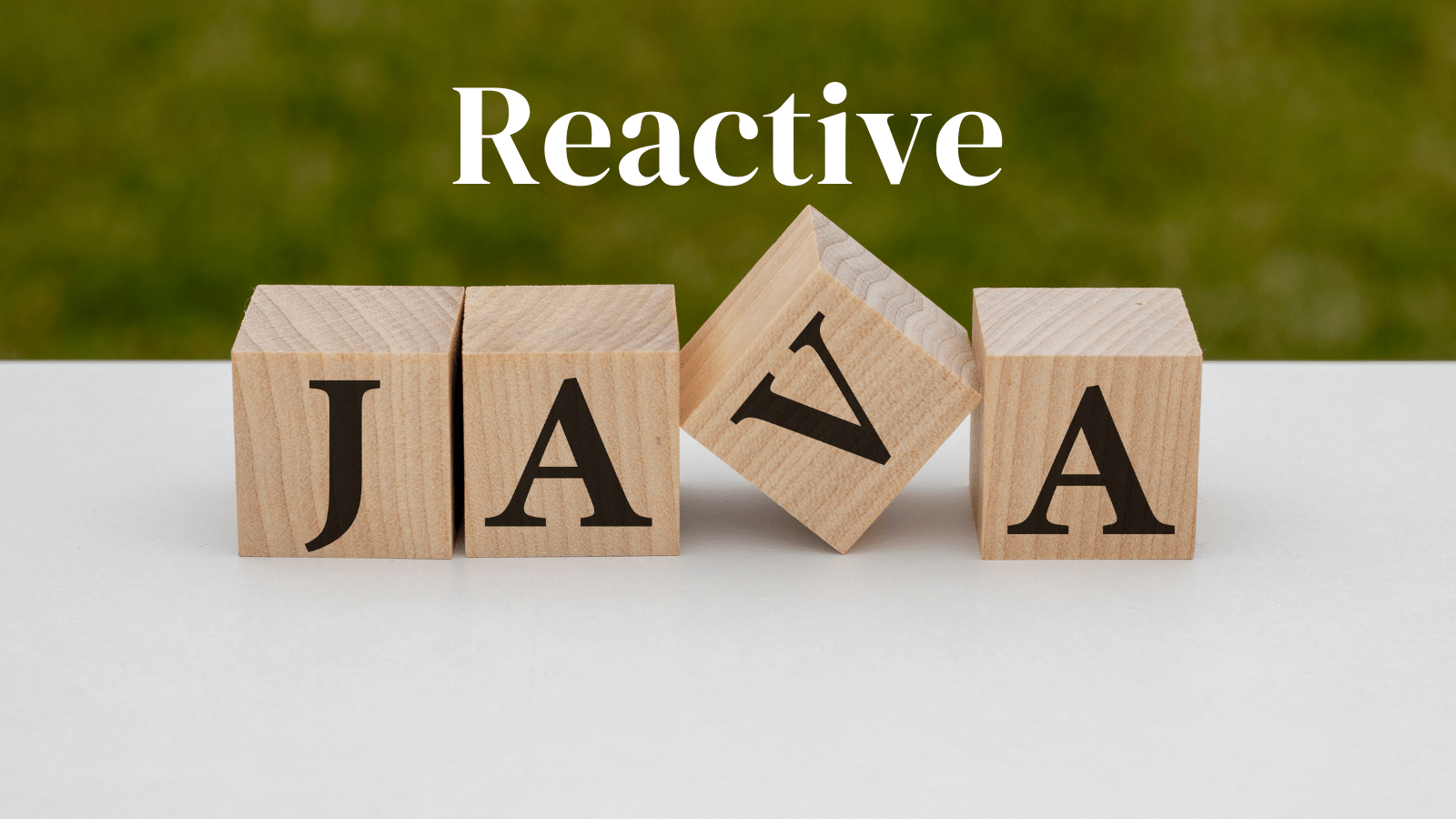
Modern applications must work smoothly on high loads and with a high number of concurrent users. Traditional Java applications run blocking code and a common approach for scaling is to increase the number of available threads. When latency comes into the picture, many of these additional threads sit idle, wasting resources. A different approach increases efficiency by writing asynchronous non-blocking code that lets the execution switch to another task while the asynchronous process completes. Project...
Better Testing with Spring Security Test
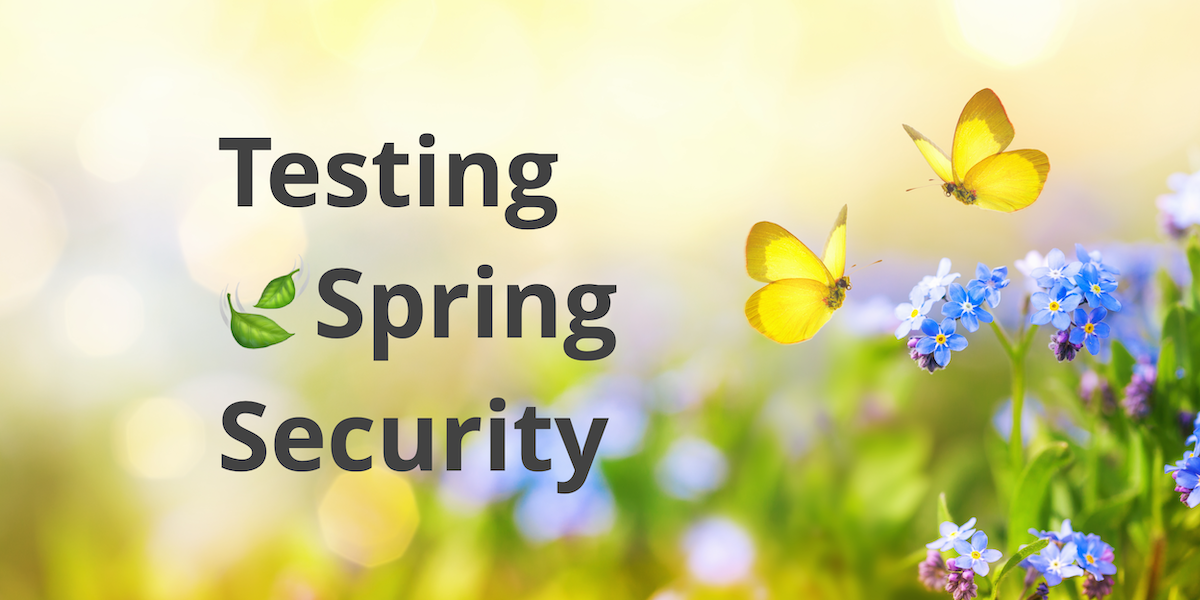
Integration testing in modern Spring Boot microservices has become easier since the release of Spring Framework 5 and Spring Security 5. Spring Framework’s WebTestClient for reactive web, and MockMvc for servlet web, allow for testing controllers in a lightweight fashion without running a server. Both frameworks leverage Spring Test mock implementations of requests and responses, allowing you to verify most of the application functionality using targeted tests. With Spring Security 5, security test support provides...
How to Use Client Credentials Flow with Spring Security
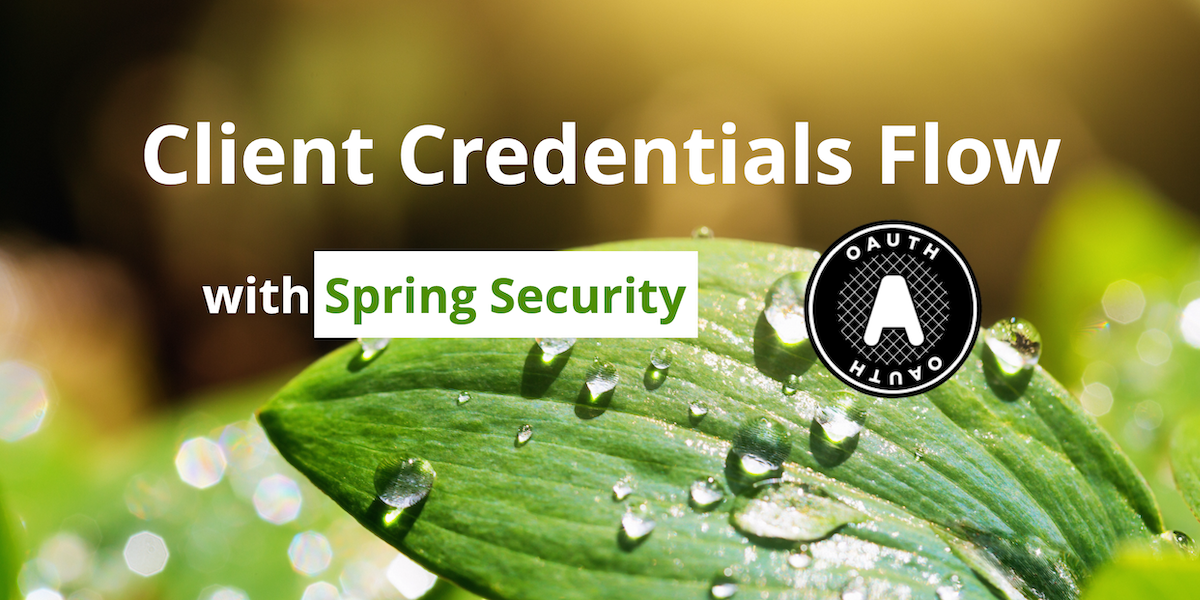
The client credentials grant is used when two servers need to communicate with each other outside the context of a user. This is a very common scenario—and yet, it’s often overlooked by tutorials and documentation online. In contrast, the authorization code grant type is more common, for when an application needs to authenticate a user and retrieve an authorization token, typically a JWT, that represents the user’s identity within the application and defines the resources...
Test in Production with Spring Security and Feature Flags
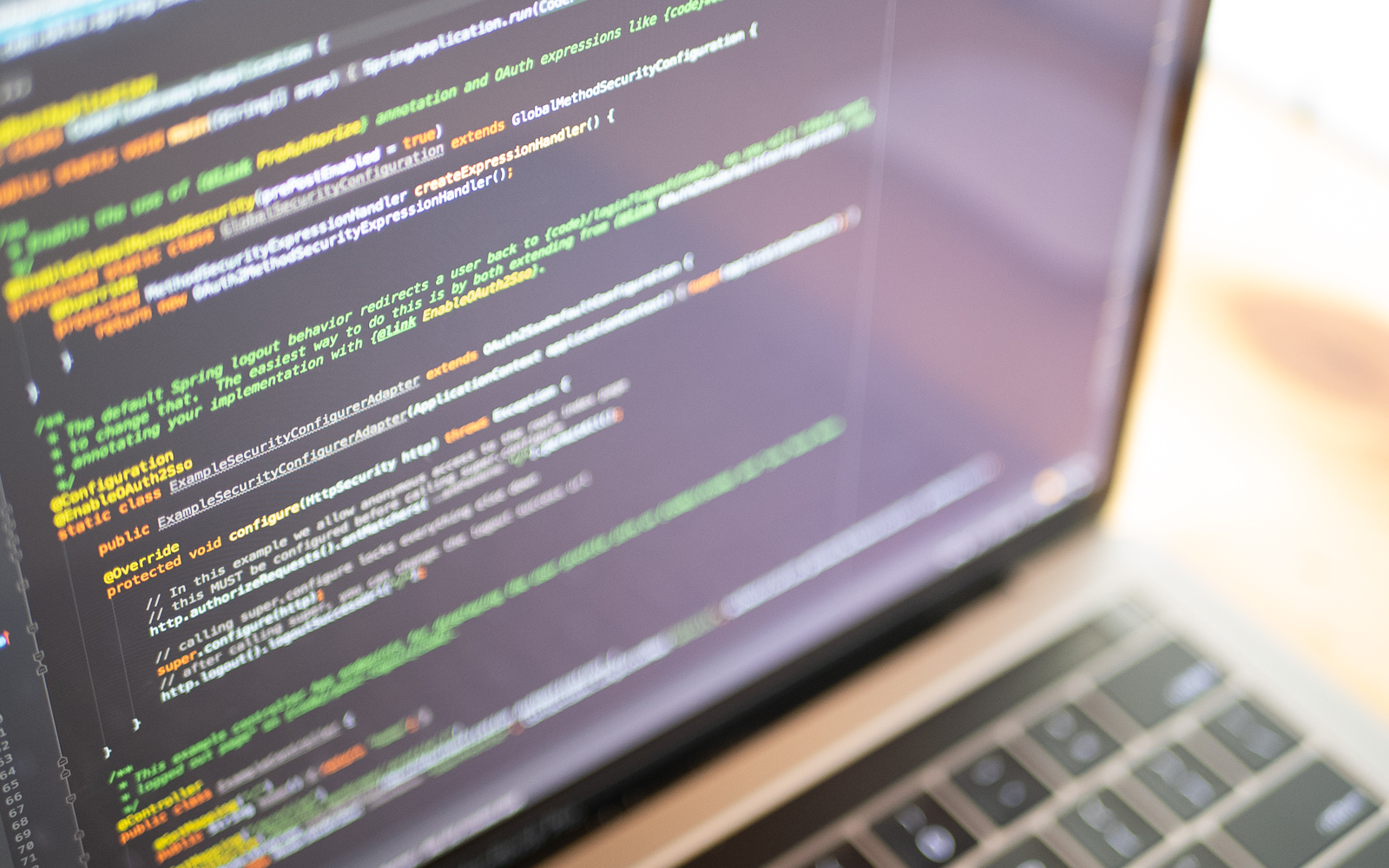
Okta is an Identity and Access Management platform. The TL;DR: you offload the responsibility for secure authentication and authorization to Okta so you can focus on the business logic of the app you’re building. Okta and Spring Boot already go together like peanut butter and chocolate. Add in feature flags care of Split, and you can test new capabilities for your app without having to redeploy. That’s testing in production the smart way! And, you...
Use PKCE with OAuth 2.0 and Spring Boot for Better Security
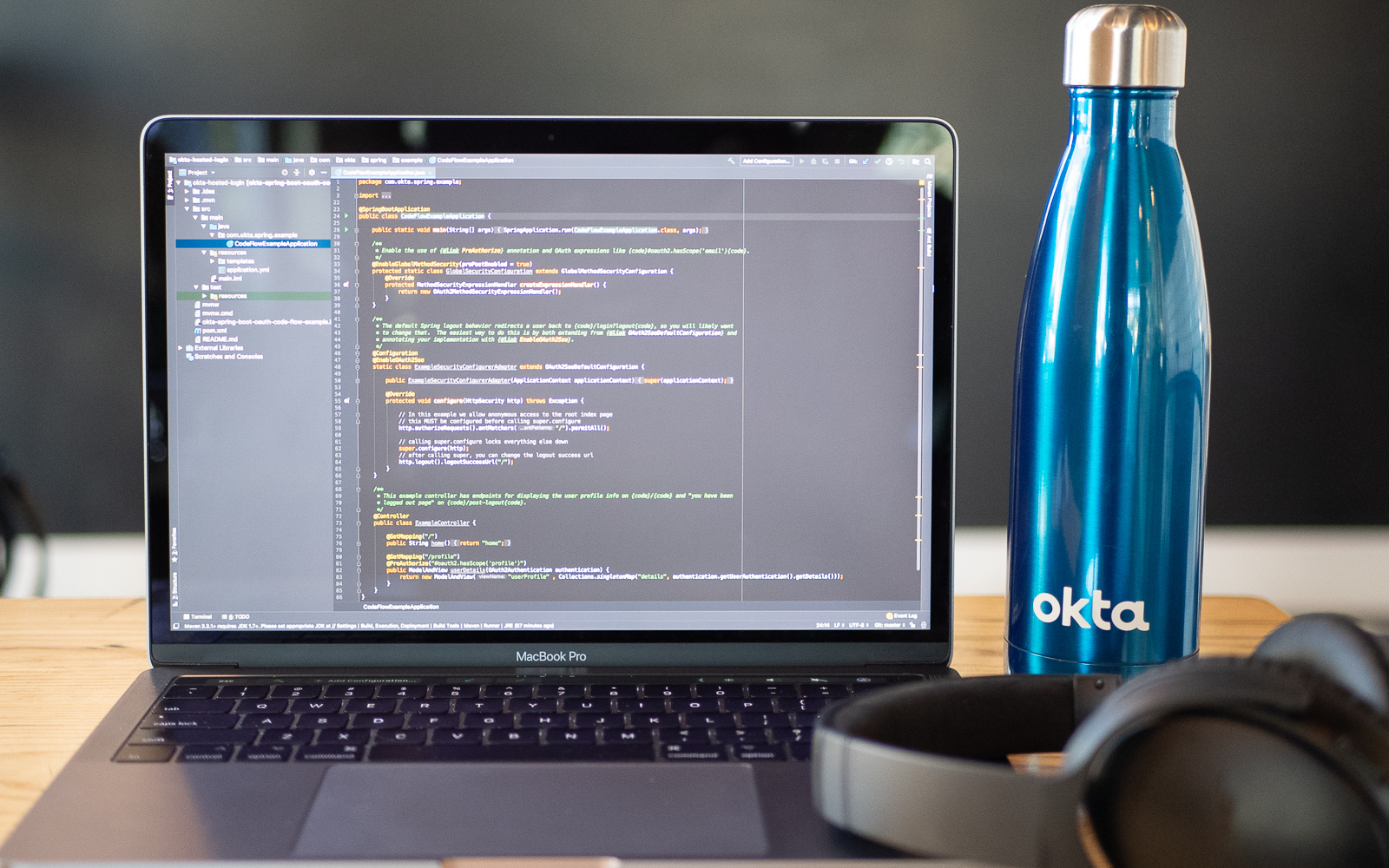
Browser and mobile feature enhancements move fast. Often times, these technologies move faster than security standards designed to protect them can keep up. OAuth 2.0 offers the best and most mature standard for modern applications. However, there hasn’t been an official release of this standard since 2012. Eight years is a very long time in Internet technology years! That doesn’t mean that its contributors have been sitting idly by. There is active work on the...
Use Okta Token Hooks to Supercharge OpenID Connect
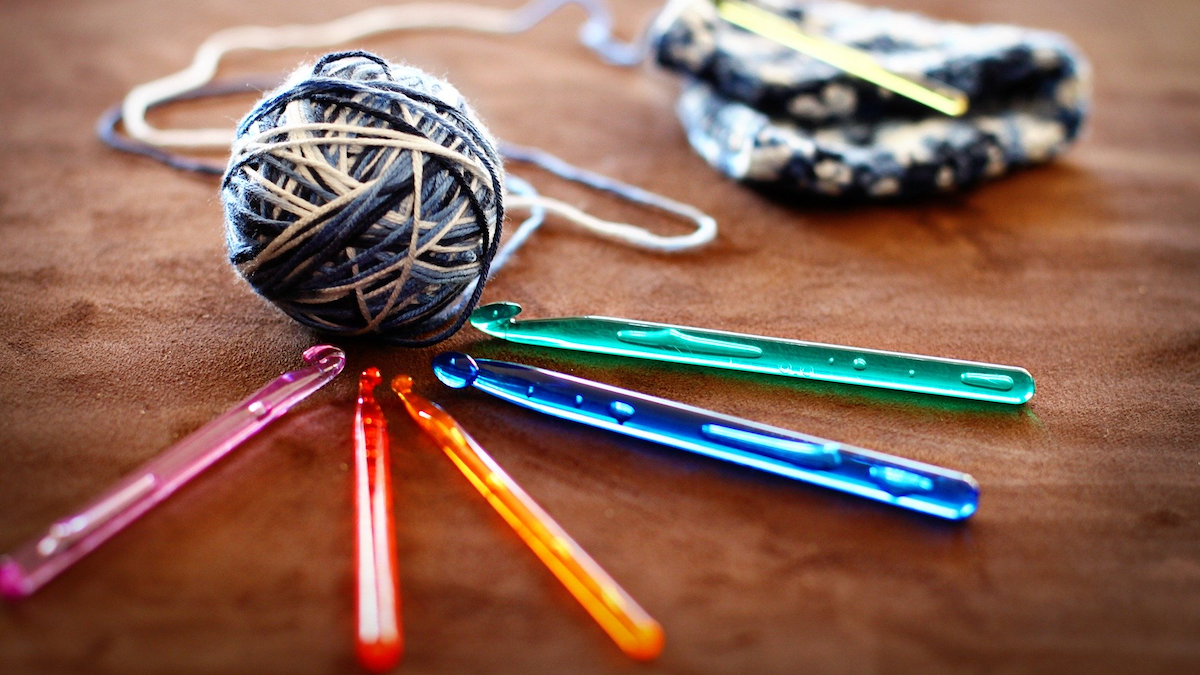
OpenID Connect (OIDC) and OAuth 2.0 are already recognized as powerful tools for incorporating authentication and authorization into modern web applications. Okta has enhanced the capabilities of these standards by introducing our Inline Hooks feature. There are a number of different types of inline hooks that Okta supports. In this post, I focus on hooks that allow you to patch information into the tokens you get back from Okta via OIDC and OAuth. You’ll first...
OAuth 2.0 Java Guide: Secure Your App in 5 Minutes
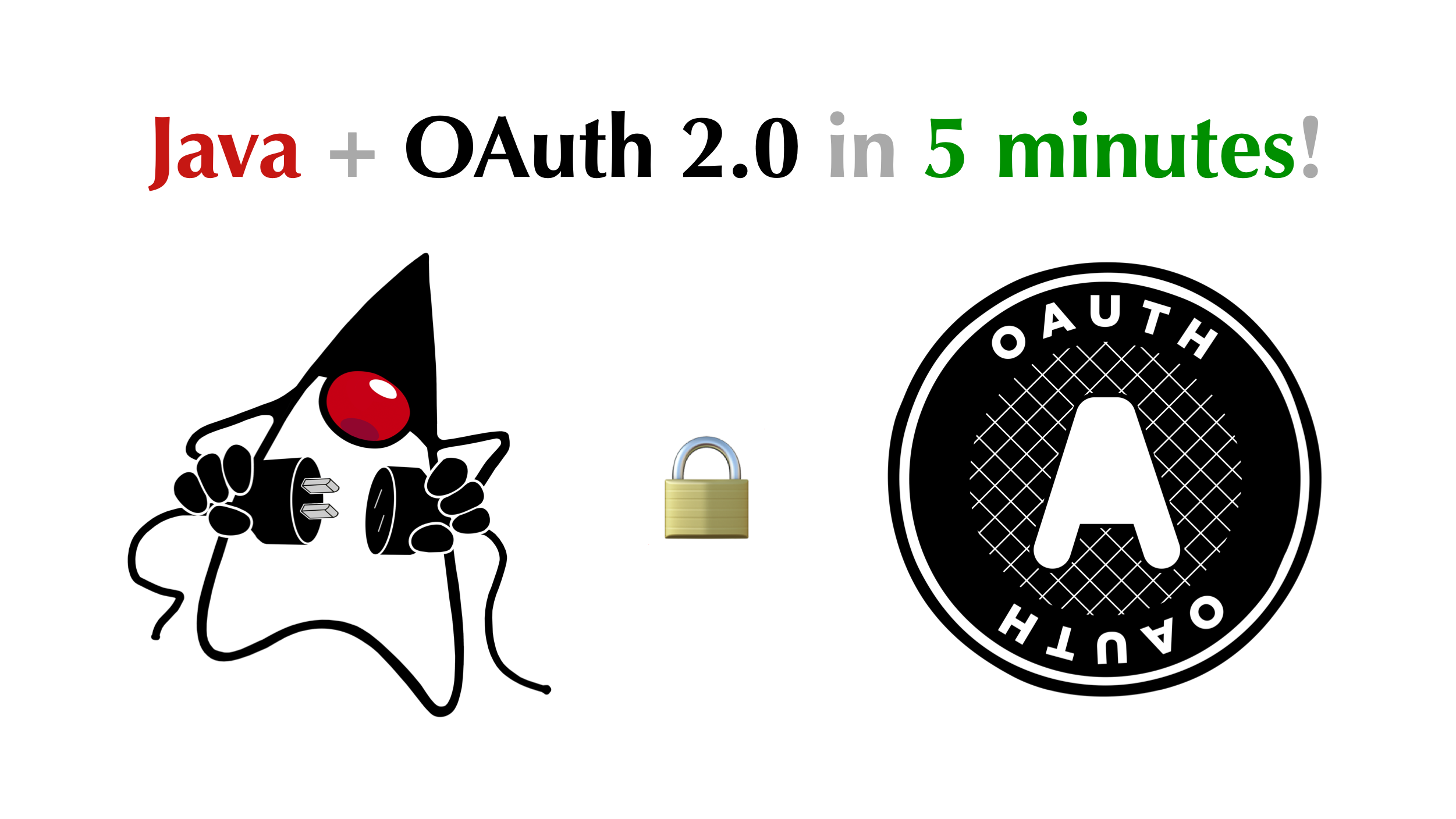
Modern applications rely on user authentication, but it can present Java developers with a difficult challenge, as well as a range of framework-specific options to choose from. We have seen many Spring developers start with a simple, home-grown authentication service they plan to replace “later” with a more robust option… only for that homegrown service to bikeshed its way to a permanent place in the stack. To end this cycle of heartbreak, this post will...
Easy Spring Boot Deployment with AWS Elastic Beanstalk
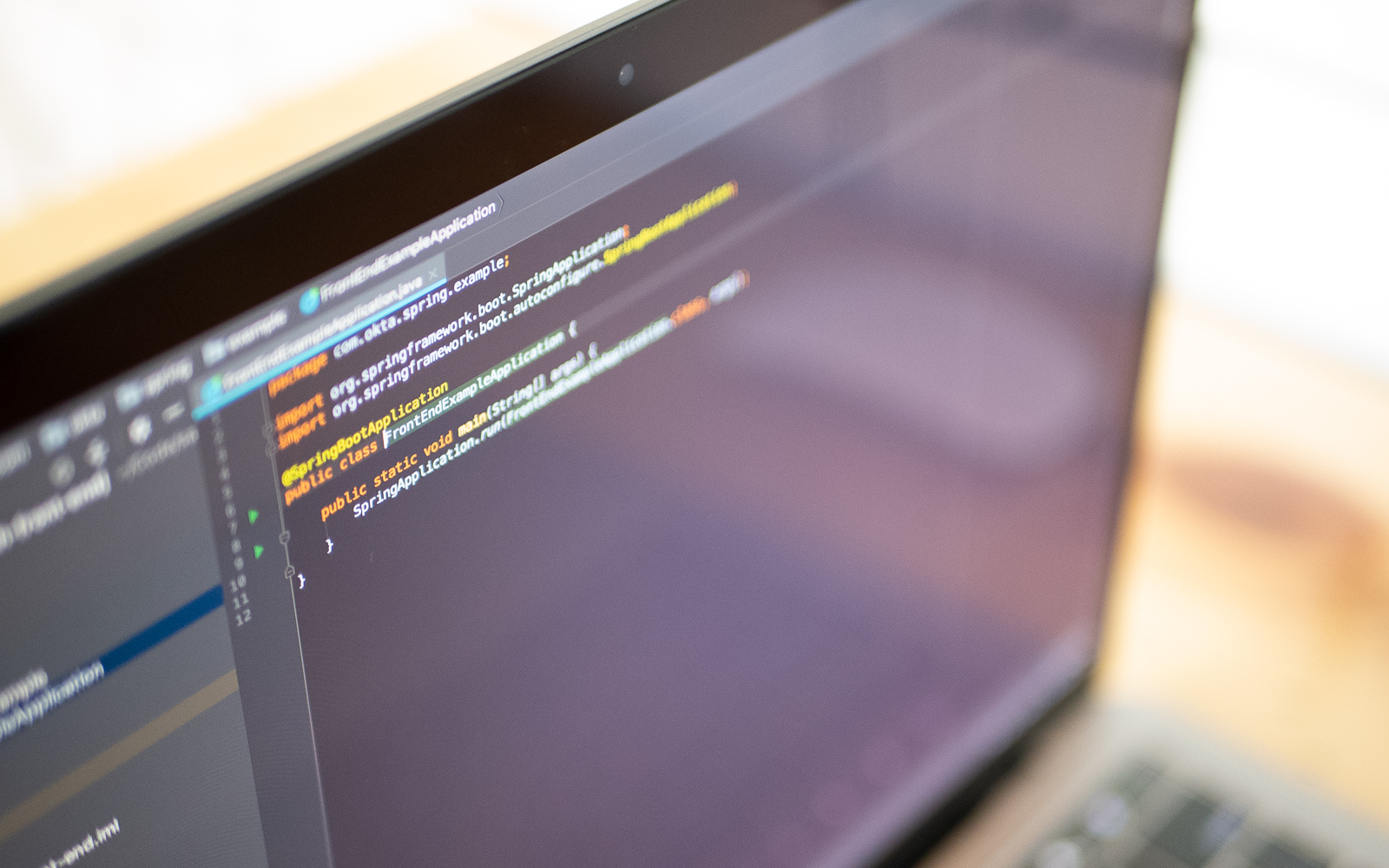
Nearly all applications rely on authentication. Developers, and the companies that employ them, want to confirm who is making the request and are they who they say they are. And, this needs to happen fast enough for a good user experience. Fortunately, there are great tools to help. Spring Boot with Spring Security is a fantastic solution for Java-based web development. With relatively little code, developers can implement, test, update, and expand authentication schemes easily...
Use Spring Boot and MySQL to go Beyond Authentication
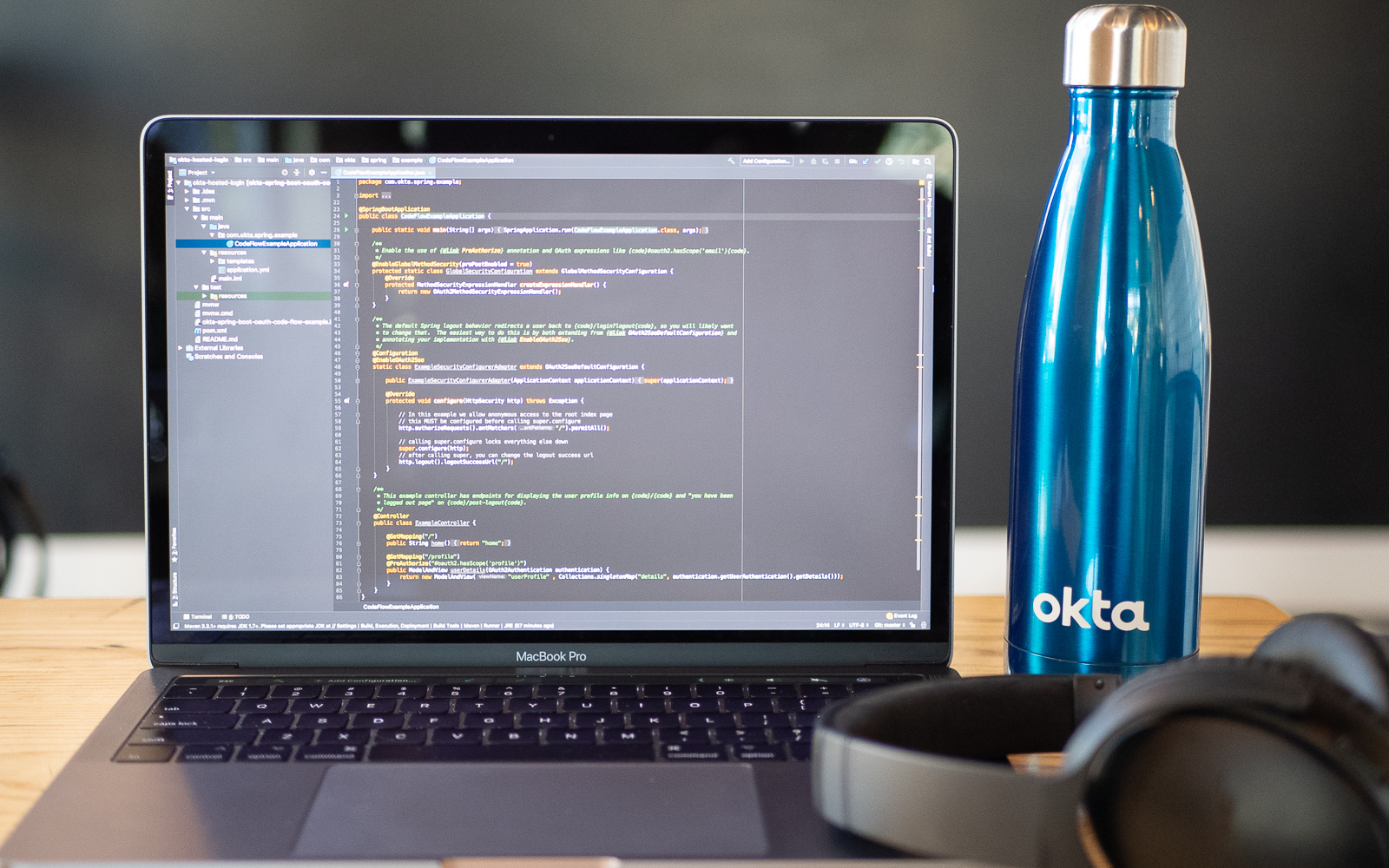
In this post, we will walk through how to build a simple CRUD application using Spring Boot, MySQL, JPA/Hibernate and Okta OpenID Connect (OIDC) Single Sign-On (SSO). The Java Persistence API (JPA) provides a specification for persisting, reading, and managing data from your Java object to relational tables in the database. The default implementation of JPA via Spring Boot is Hibernate. Hibernate saves you a lot of time writing code to persist data to a...
Spring Method Security with PreAuthorize
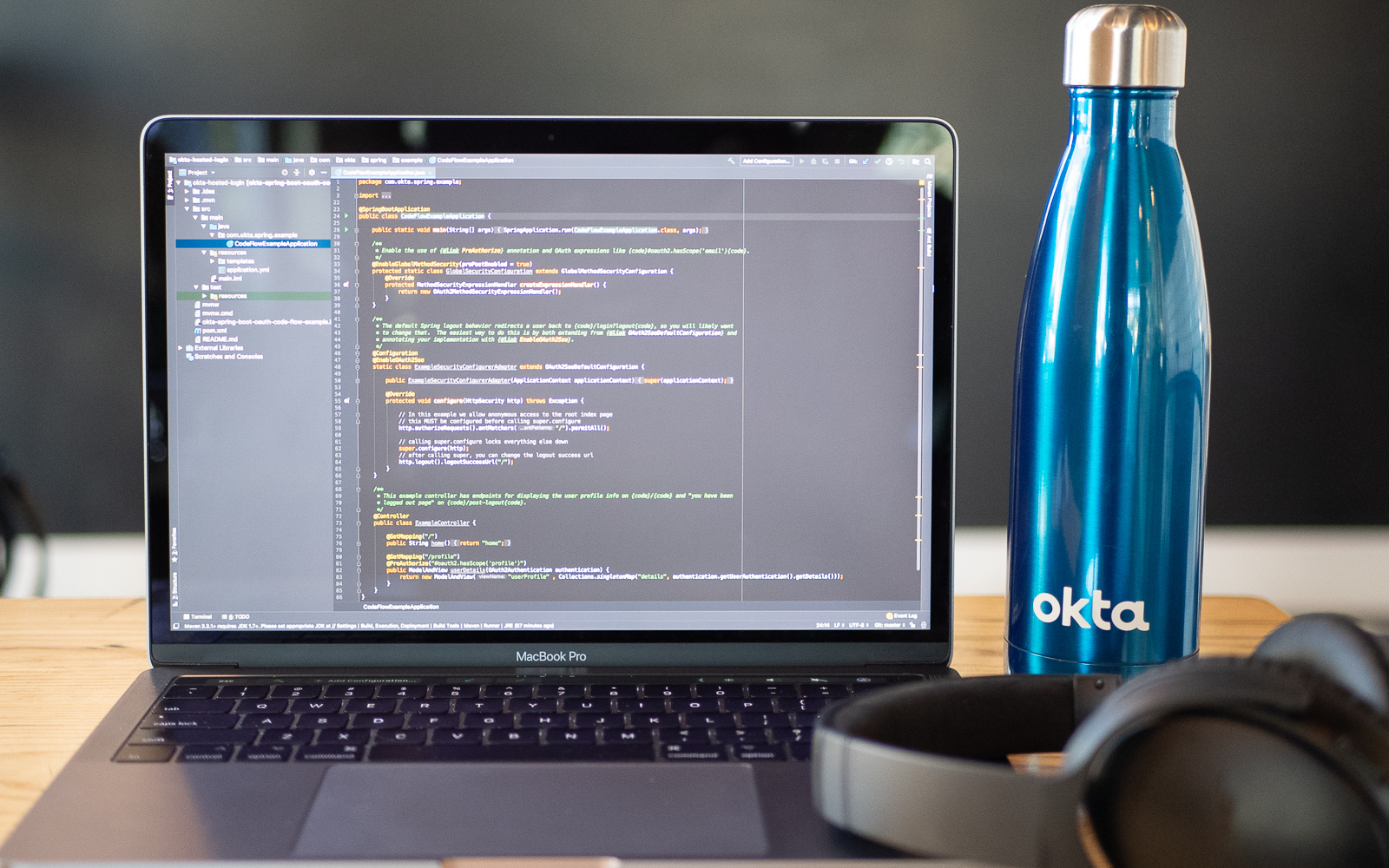
This tutorial will explore two ways to configure authentication and authorization in Spring Boot using Spring Security. One method is to create a WebSecurityConfigurerAdapter and use the fluent API to override the default settings on the HttpSecurity object. Another is to use the @PreAuthorize annotation on controller methods, known as method-level security or expression-based security. The latter will be the main focus of this tutorial. However, I will present some HttpSecurity code and ideas by...
Simple Authentication with Spring Security
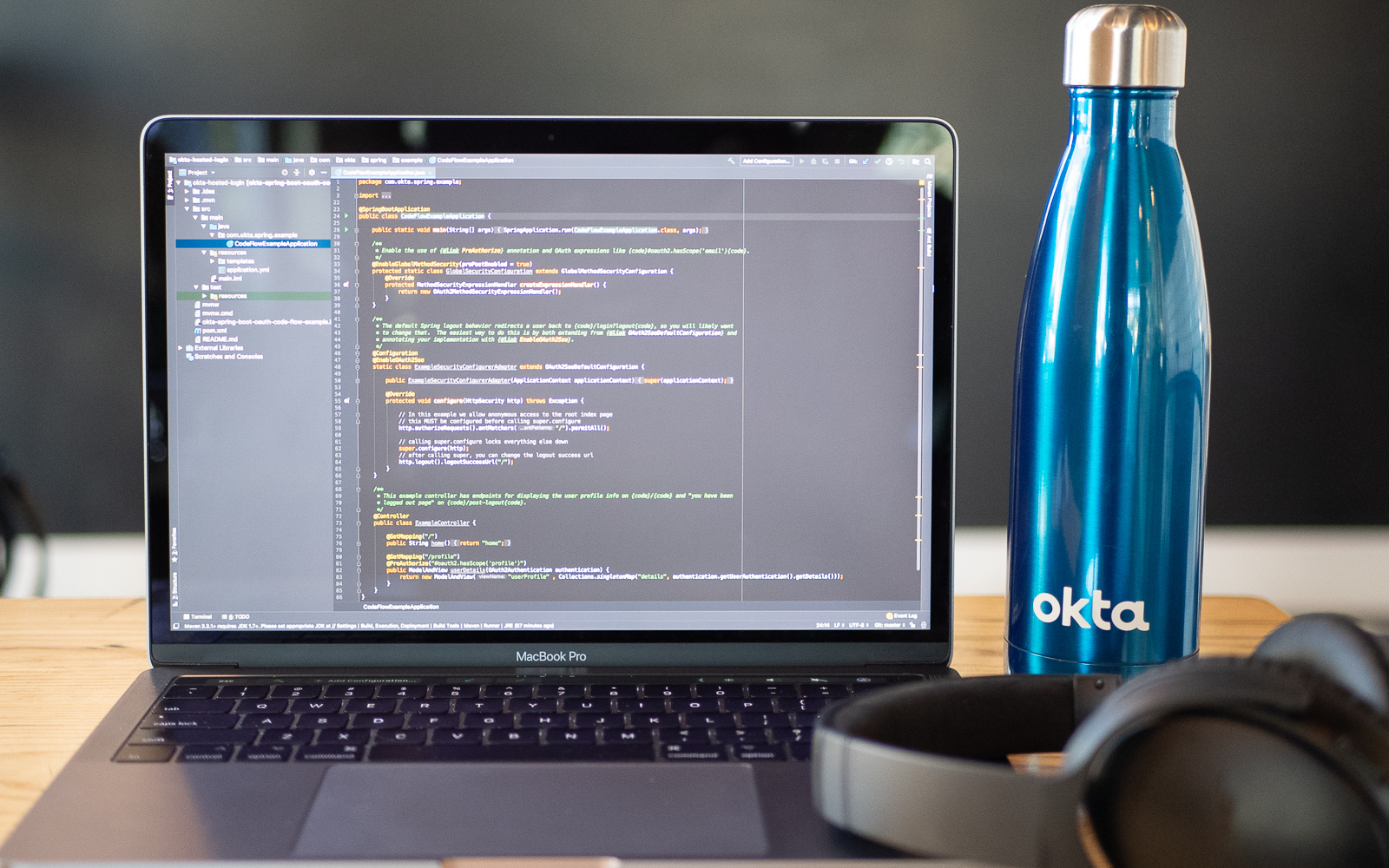
Authentication is vital to all but the most basic web applications. Who is making the request, wanting data, or wanting to update or delete data? Can you be sure that the request is coming from the stated user or agent? Answering this question with certainty is hard in today’s computer security environment. Fortunately, there is absolutely no reason to reinvent the wheel. Spring Boot with Spring Security is a powerful combination for web application development....
Easy Single Sign-On with Spring Boot and OAuth 2.0
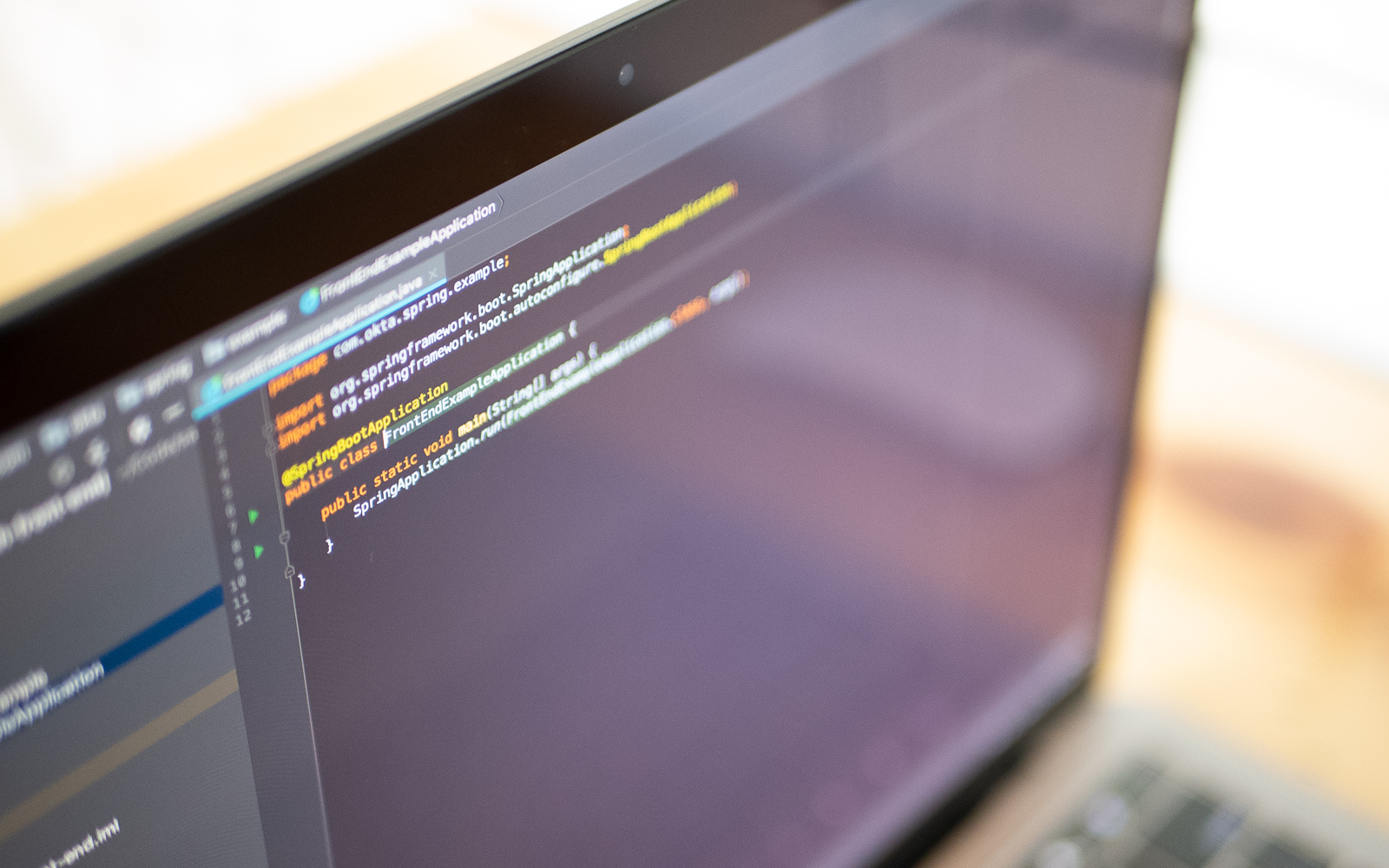
Single sign-on used to be the “Holy Grail” of enterprise size companies and was usually only available companies that could afford it. Nowadays, we take SSO as a matter of course. For instance, you would think it was completely weird (and unpleasant) if you logged into GMail and then had to log in again when you went to Google Docs. But, what about building custom applications for developers? SSO was still in the domain of...
A Quick Guide to OAuth 2.0 with Spring Security
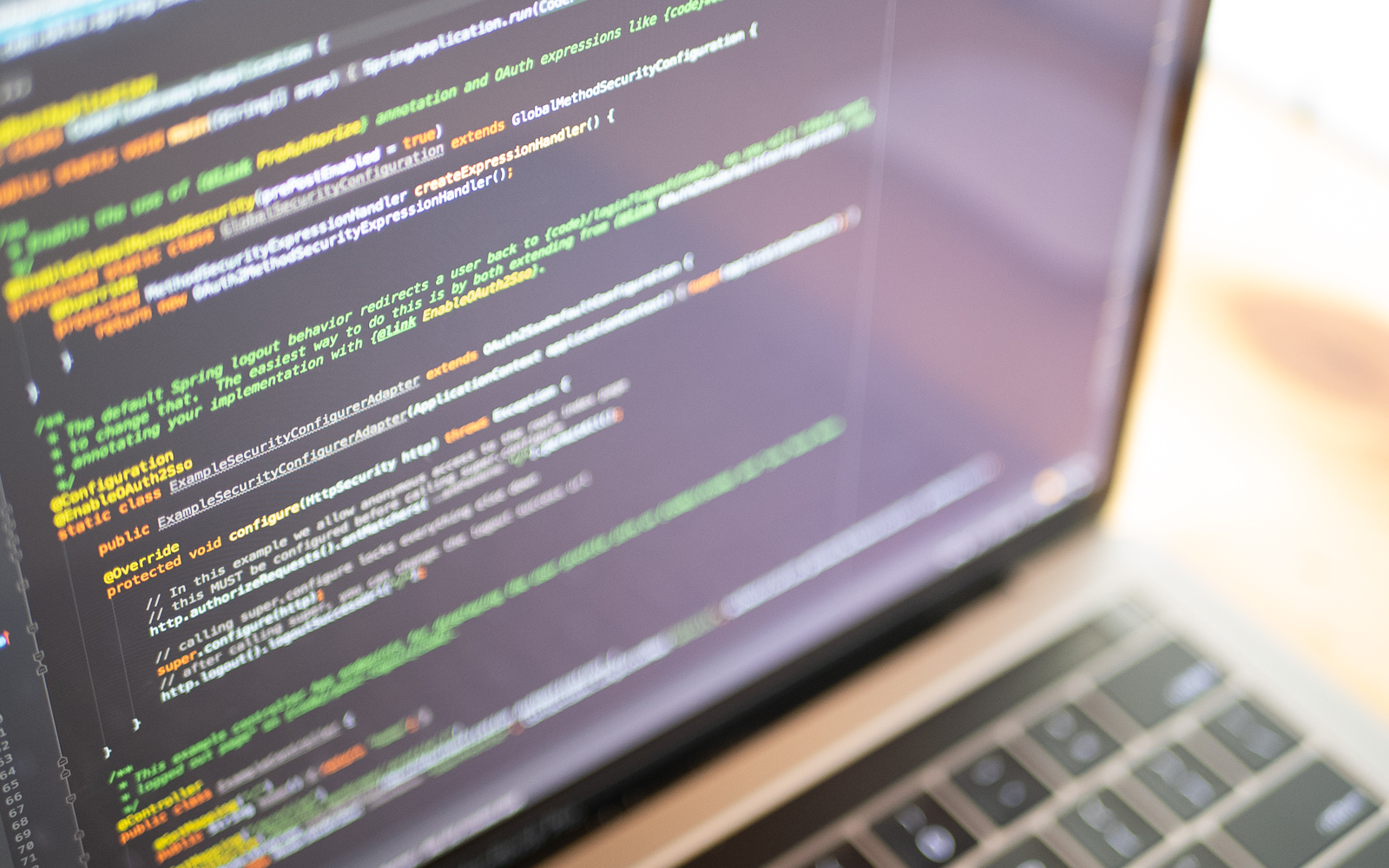
When building a web application, authentication and authorization is a must. Doing it right, however, is not simple. Computer security is a true specialty. Legions of developers work day and night against equally numerous international hackers creating a continual development cycle of finding vulnerabilities, attacking them, and fixing them. Keeping up with all this solo would be painful (if not impossible). Fortunately, there’s no need. Spring Security and Spring Boot have made implementing a web...
Secure Service-to-Service Spring Microservices with HTTPS and OAuth 2.0
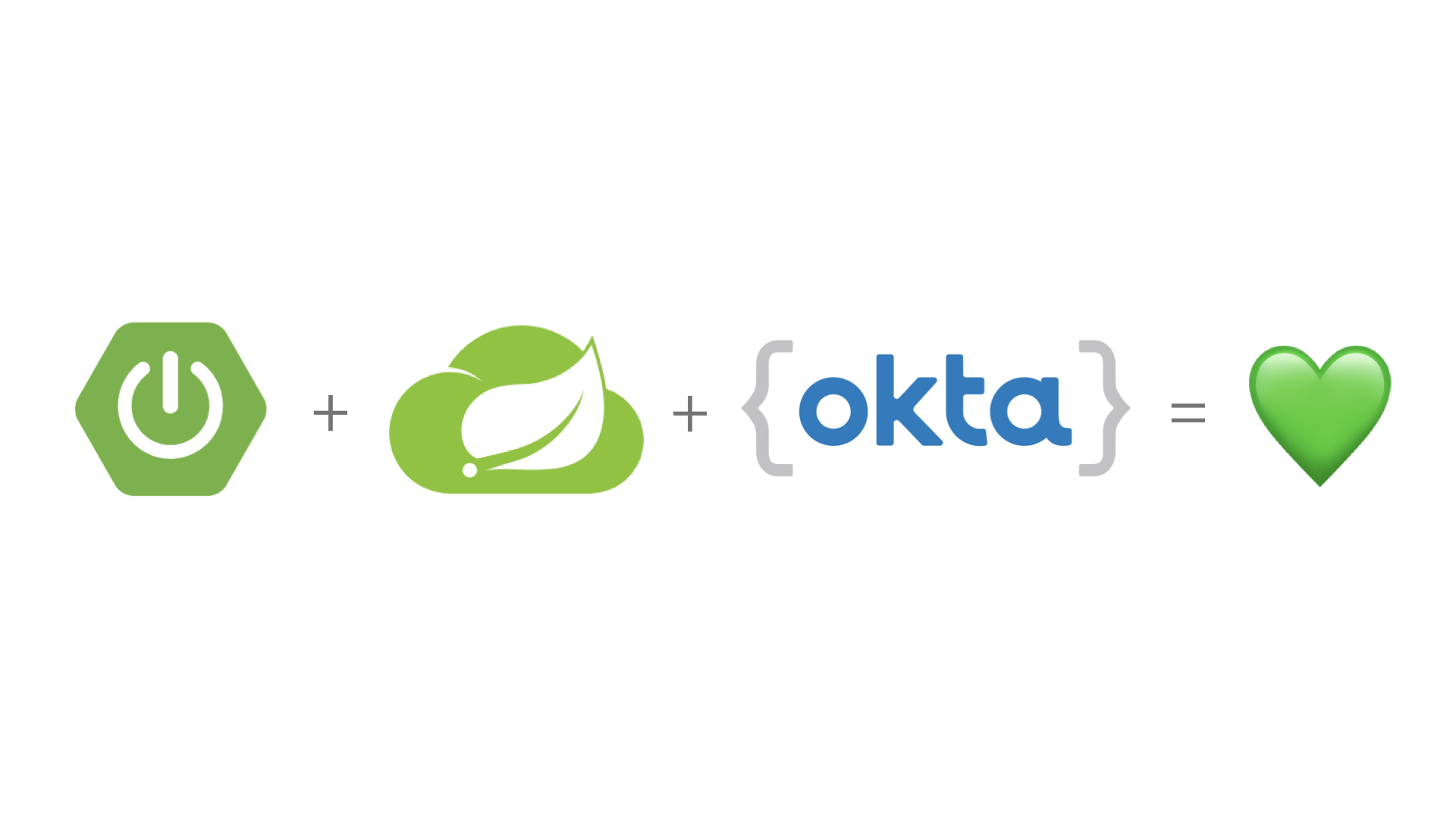
Building a microservices architecture is possible with minimal code if you use Spring Boot, Spring Cloud, and Spring Cloud Config. Package everything up in Docker containers and you can run everything using Docker Compose. If you’re communicating between services, you can ensure your services are somewhat secure by not exposing their ports in your docker-compose.yml file. But what happens if someone accidentally exposes the ports of your microservice apps? Will they still be secure or...
Migrate Your Spring Boot App to the Latest and Greatest Spring Security and OAuth 2.0
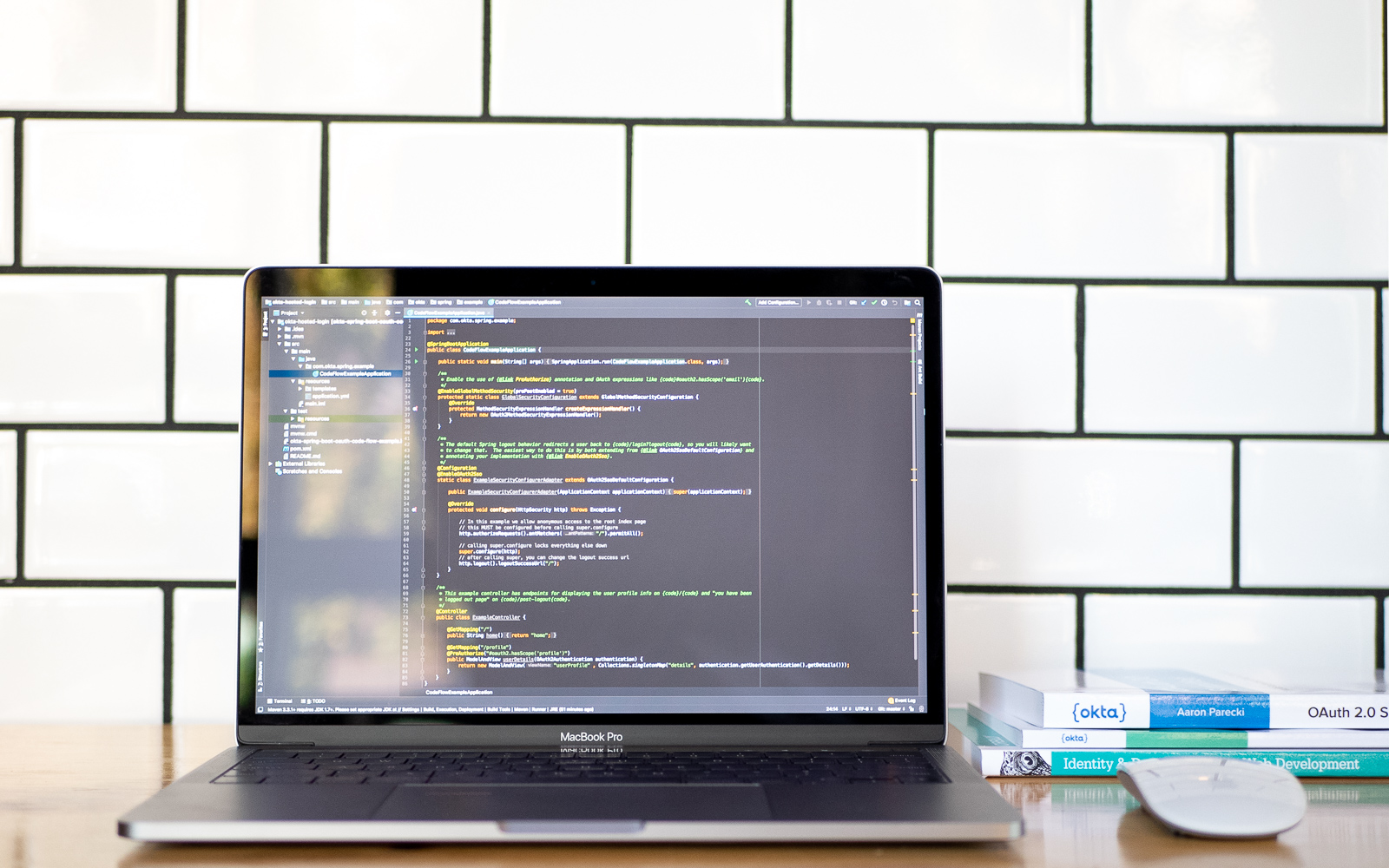
Spring Boot 1.5.x made it easier than ever before to integrate Spring Security with OAuth 2.0 into your application. Spring Boot 2.1.x dials it up to 11 by making OpenID Connect a first class citizen in the stack. In this post, you start with Spring Boot 1.5.19 and Spring Security 4.2.x. You integrate it with Okta’s OAuth service. From there, you move onto Spring Boot 2.1.3 and Spring Security 5.1. You’ll see how integrating with...
Build Spring Microservices and Dockerize Them for Production
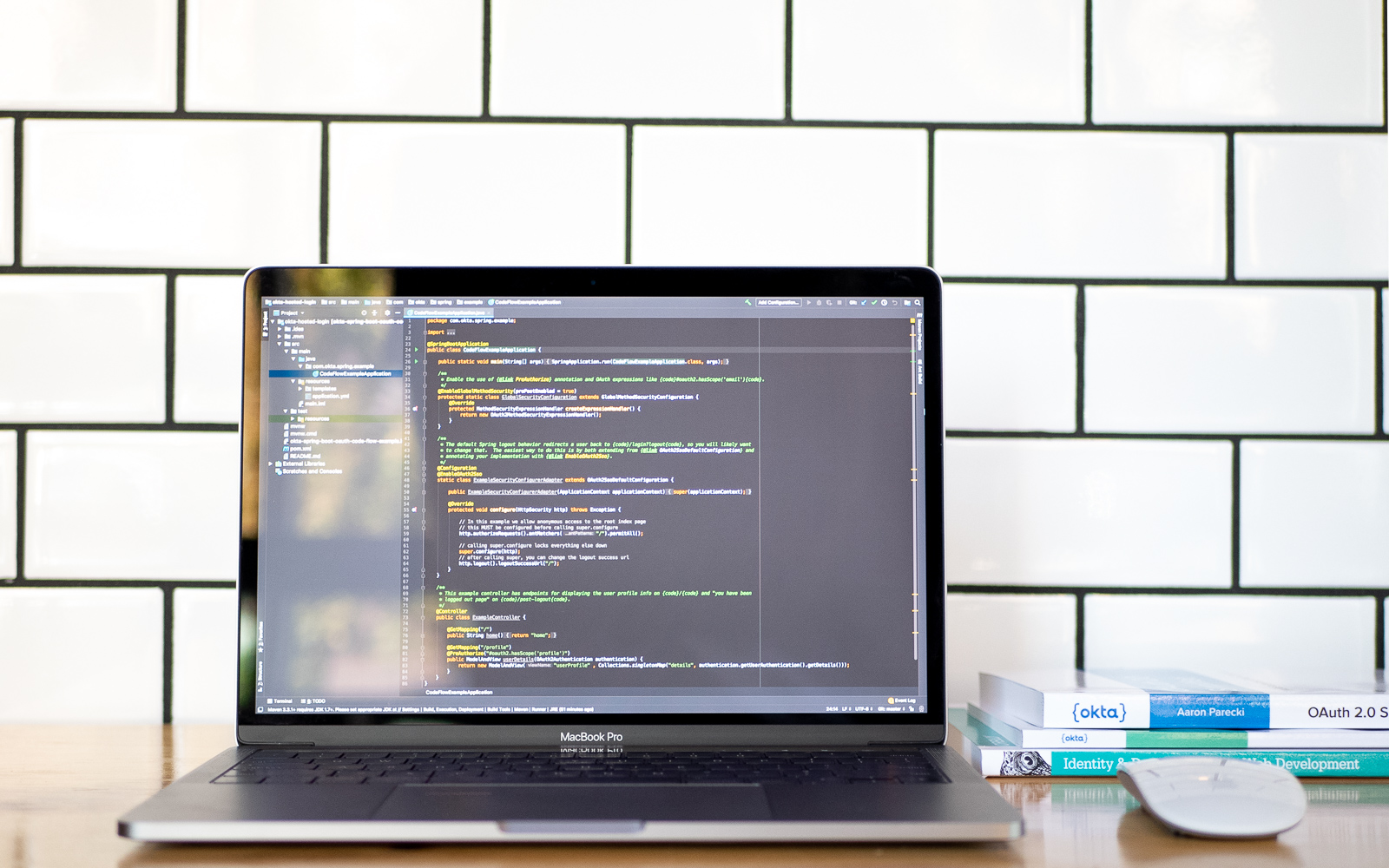
In this post, you’ll learn about microservices architecture and how to implement it using Spring Boot. After creating some projects with the technique, you will deploy the artifacts as Docker containers and will simulate a container orchestrator (such as Kubernetes) using Docker Compose for simplification. The icing on the cake will be authentication integration using Spring Profiles; you will see how to enable it with a production profile. But first, let’s talk about microservices. Understand...
Add Social Login to Your JHipster App
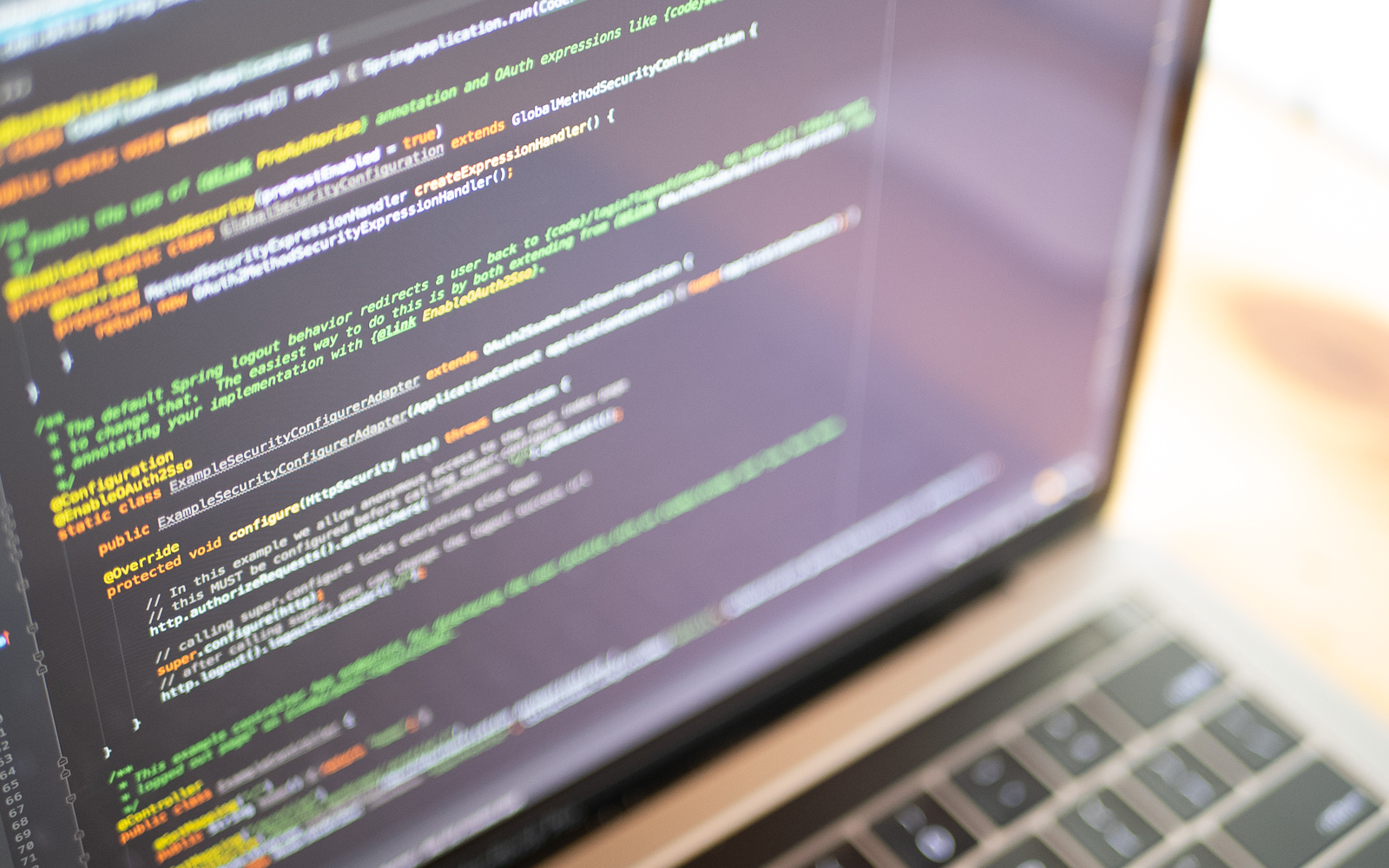
Social login is a great way to offer your customers a simple and secure authentication method. Why force them to create and forget yet another password? The vast majority of your users will have an account with Facebook or Google, so why no go ahead and let them use one of these accounts to log in? In this tutorial, you are going to integrate two social login providers: Google and Facebook. You are also going...
Spring Boot 2.1: Outstanding OIDC, OAuth 2.0, and Reactive API Support
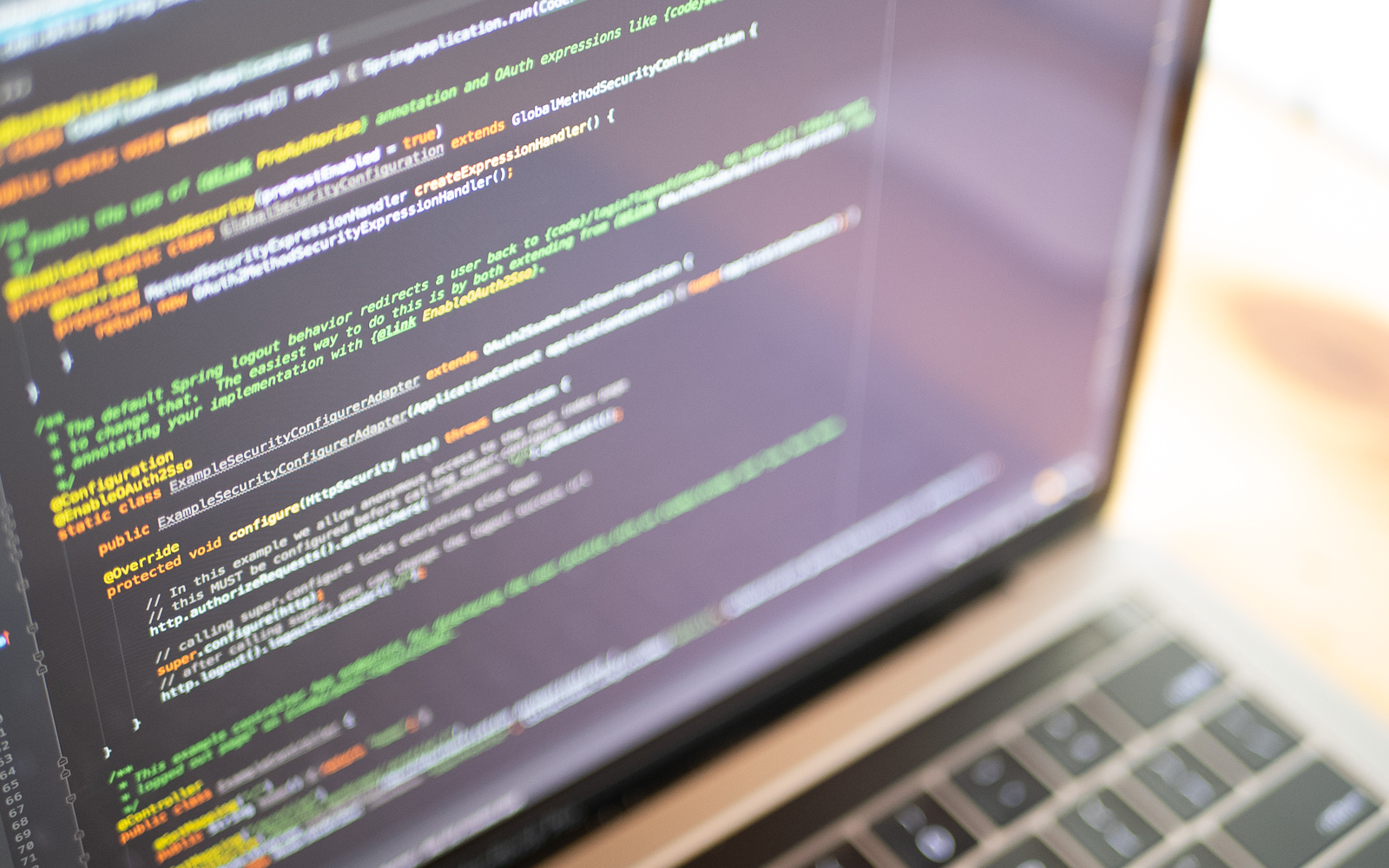
Spring Boot 2.1 was recently released, eight months after the huge launch of Spring Boot 2.0. The reason I’m most excited about Spring Boot 2.1 to me is its improved performance and OpenID Connect (OIDC) support from Spring Security 5.1. The combination of Spring Boot and Spring Security has provided excellent OAuth 2.0 support for years, and making OIDC a first-class citizen simplifies its configuration quite a bit. For those that aren’t aware, OIDC is...
Build Reactive APIs with Spring WebFlux
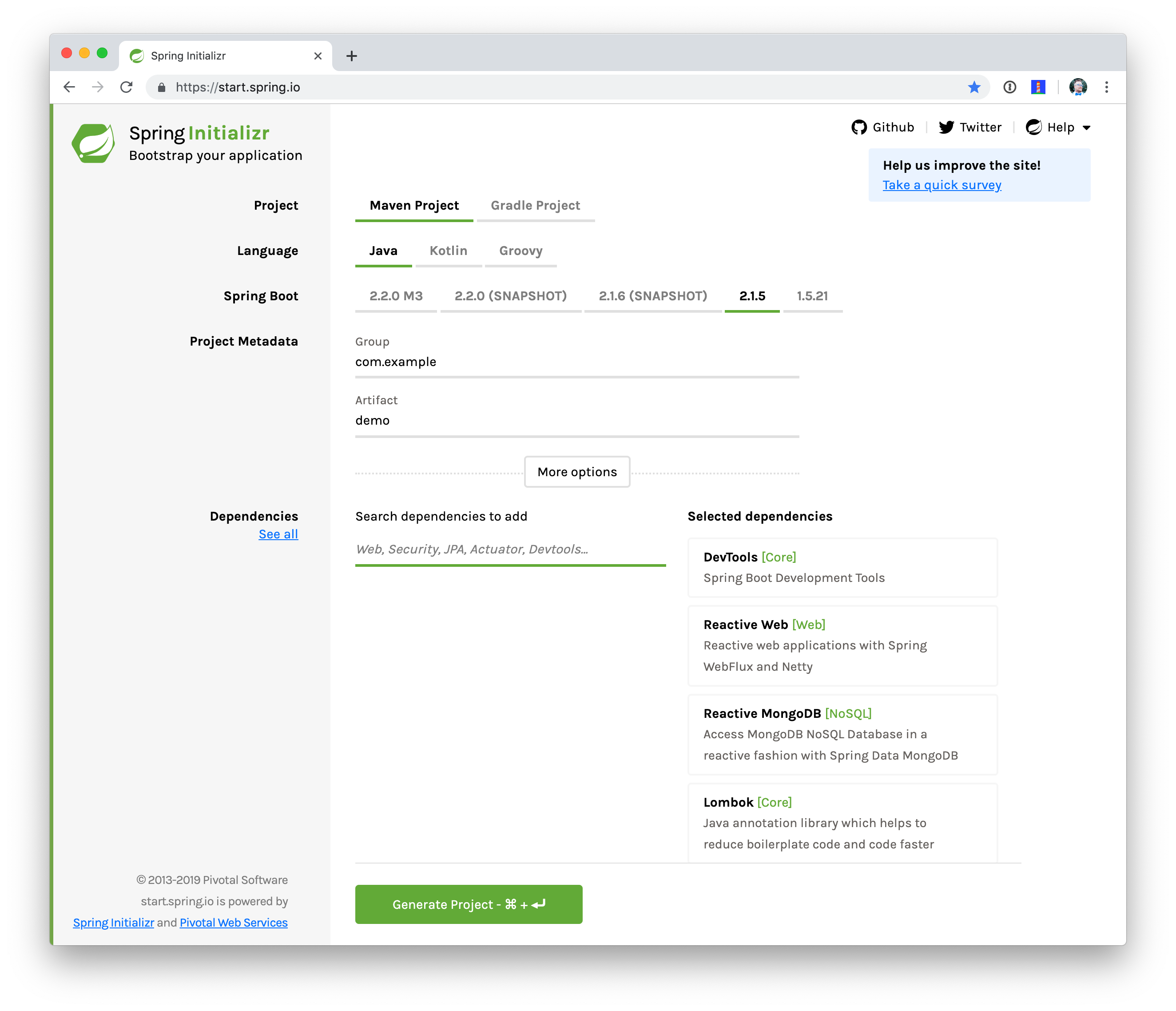
Spring Boot 2.0 was a long-awaited release from the good folks at Pivotal. One of its new features is reactive web programming support with Spring WebFlux. Spring WebFlux is a web framework that’s built on top of Project Reactor, to give you asynchronous I/O, and allow your application to perform better. If you’re familiar with Spring MVC and building REST APIs, you’ll enjoy Spring WebFlux. There’s just a few basic concepts that are different. Once...
Build a Java REST API with Java EE and OIDC
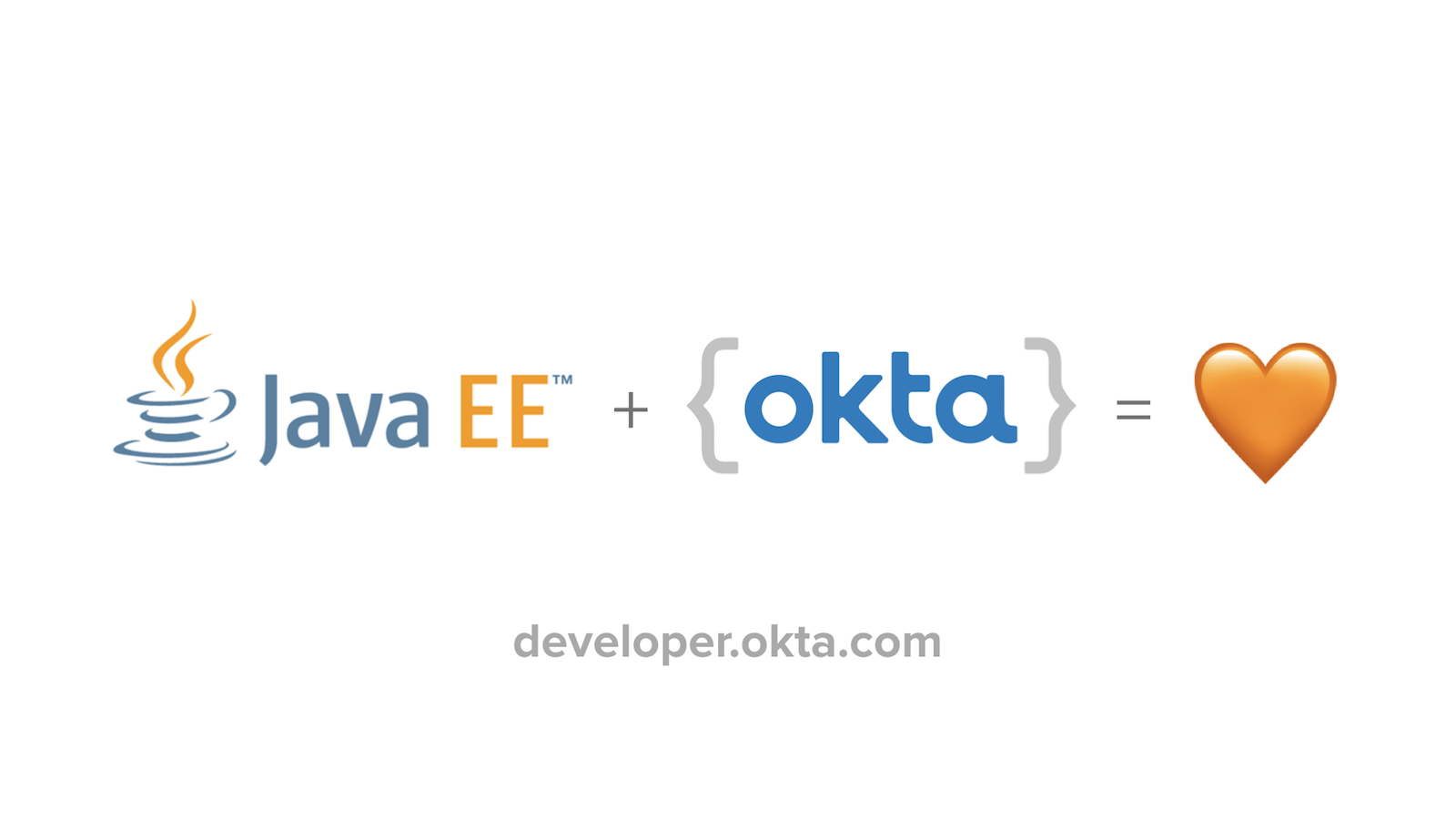
Java EE allows you to build Java REST APIs quickly and easily with JAX-RS and JPA. Java EE is an umbrella standards specification that describes a number of Java technologies, including EJB, JPA, JAX-RS, and many others. It was originally designed to allow portability between Java application servers, and flourished in the early 2000s. Back then, application servers were all the rage and provided by many well-known companies such as IBM, BEA, and Sun. JBoss...
Build a Secure API with Spring Boot and GraphQL
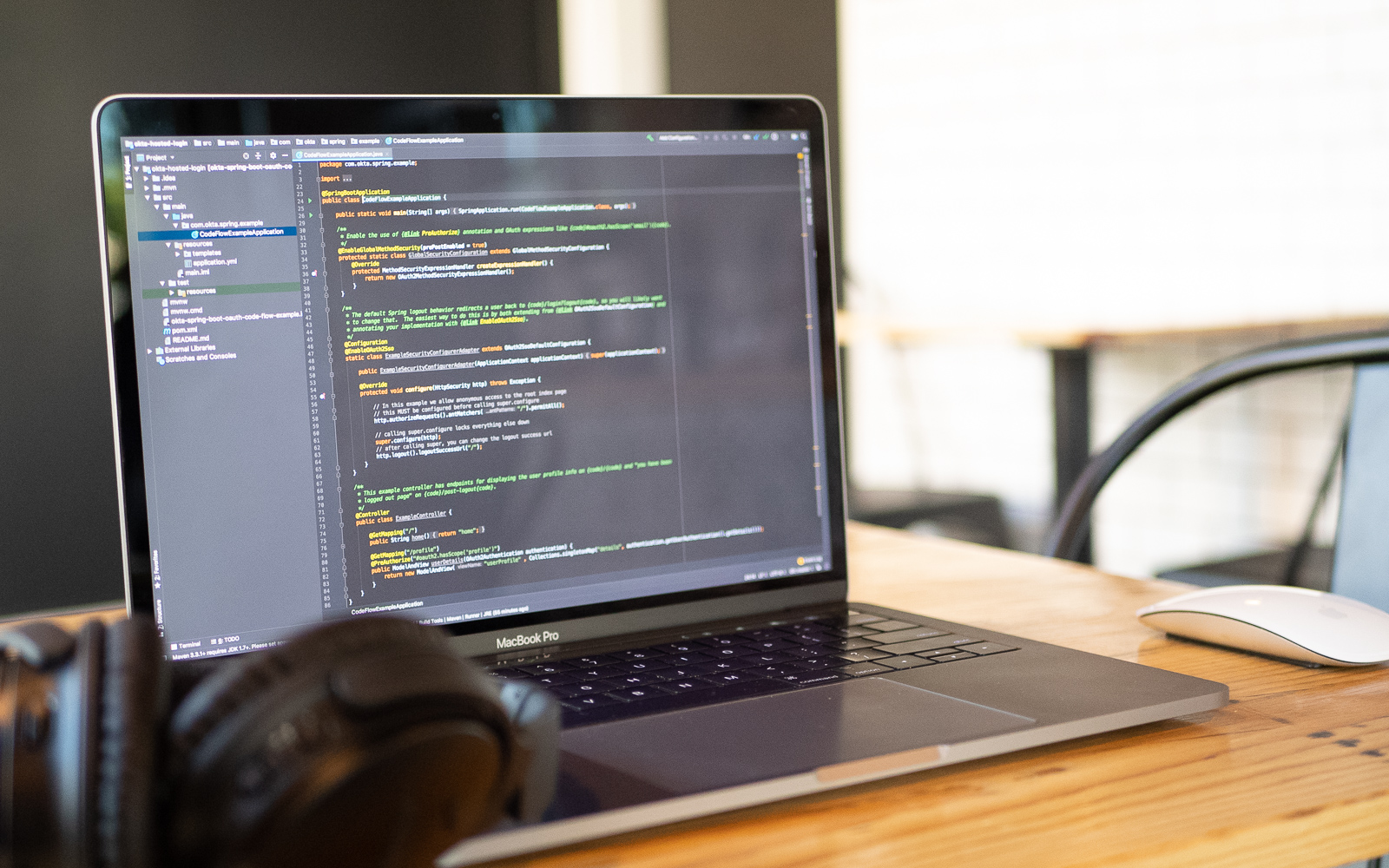
GraphQL is a data query language developed by Facebook in 2012 to solve a shortcoming of REST APIs and traditional database models. All too often, when programmers write REST API data queries, they default to retrieving entire data structures when they need only a part of it. For example, if you want to find out the number of comments on a blog post, a developer might typically retrieve the entire post and all associated fields...
Build and Secure Microservices with Spring Boot 2.0 and OAuth 2.0
Spring Boot has experienced massive adoption over the last several years. For Spring users, it offers a breath of fresh air, where they don’t have to worry about how things are configured if they’re comfortable with defaults. The Spring Boot ecosystem is filled with a wealth of what they call starters. Starters are bundles of dependencies that autoconfigure themselves to work as a developer might expect. Spring Boot allows you to create standalone web apps,...
Develop a Microservices Architecture with OAuth 2.0 and JHipster
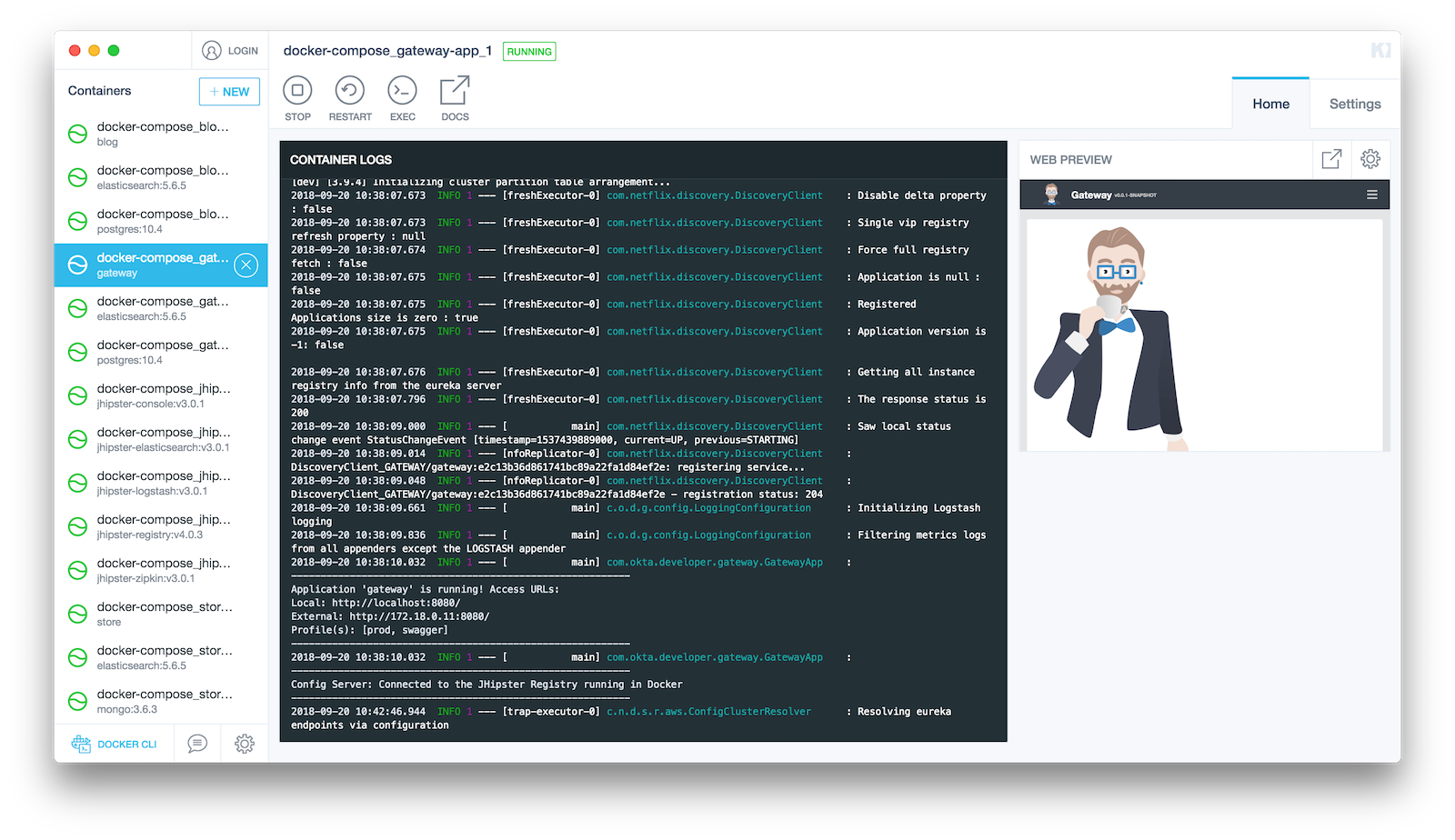
JHipster is a development platform to generate, develop, and deploy Spring Boot + Angular web applications and Spring microservices. It supports using many types of authentication: JWT, session-based, and OAuth 2.0. In its 5.0 release, it added React as a UI option. In addition to having two popular UI frameworks, JHipster also has modules that support generating mobile applications. If you like Ionic, which currently leverages Angular, you can use Ionic for JHipster. If you’re...
Secure a Spring Microservices Architecture with Spring Security and OAuth 2.0
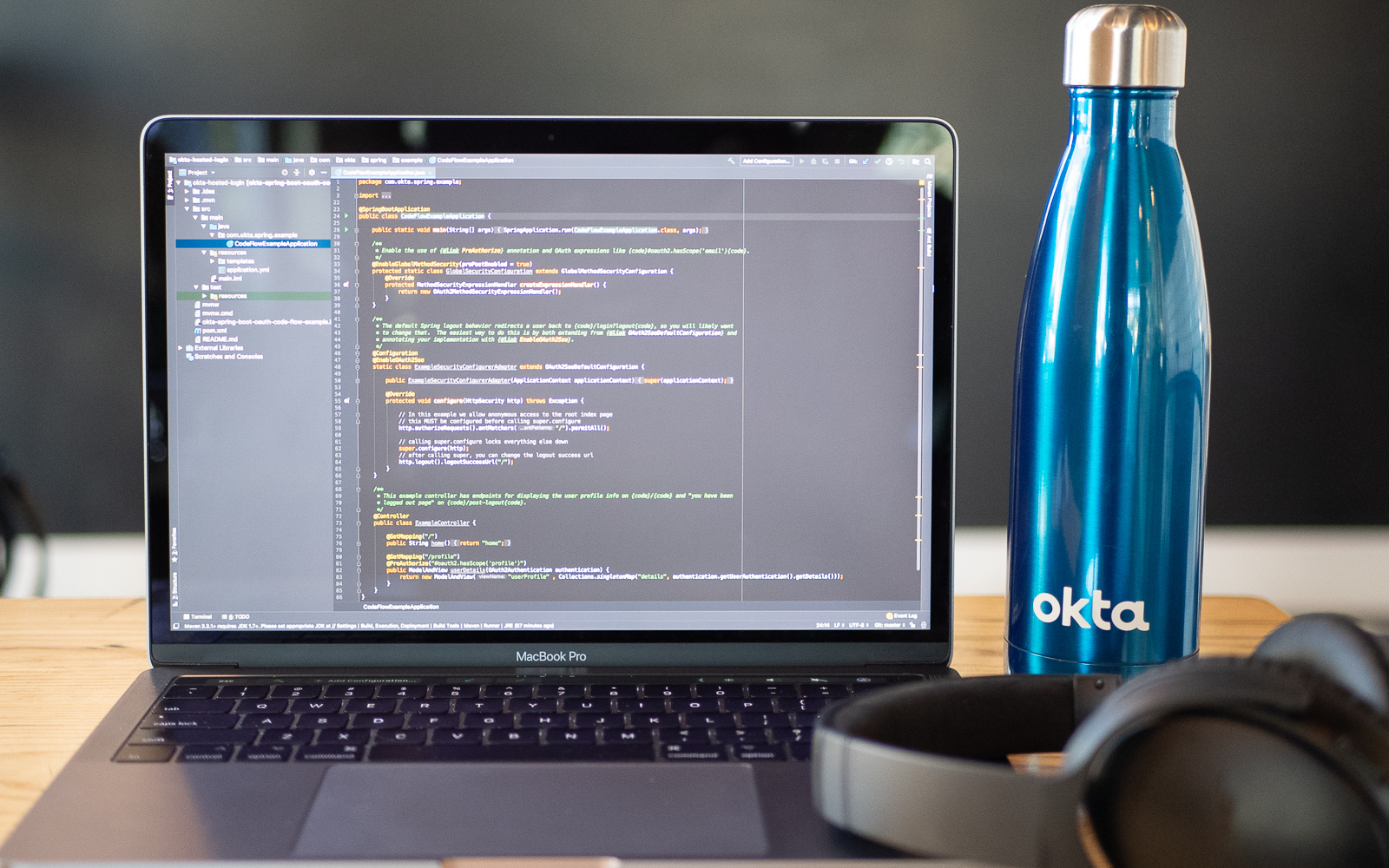
Building a microservices architecture with Spring Boot and Spring Cloud can allow your team to scale and develop software faster. It can add resilience and elasticity to your architecture that will enable it to fail gracefully and scale infinitely. All this is great, but you need continuous deployment and excellent security to ensure your system stays up-to-date, healthy, and safe for years to come. With Spring Security and its OAuth 2.0 support, you have everything...
Get Started with Spring Security 5.0 and OIDC
Spring Security is a powerful and highly customizable authentication and access-control framework. It is the de-facto standard for securing Spring-based applications. I first encountered Spring Security when it was called Acegi Security in 2005. I had implemented standard Java EE in my open source project, AppFuse. Acegi Security offered a lot more, including remember me and password encryption as standard features. I had managed to get “remember me” working with Java EE, but it wasn’t...
Secure your SPA with Spring Boot and OAuth
If you have a JavaScript single-page application (SPA) that needs to securely access resources from a Spring Boot application, you likely want to use the OAuth 2.0 implicit flow! With this flow your client will send a bearer token with each request and your server side application will verify the token with an Identity Provider (IdP). This allows your resource server to trust that your client is authorized to make the request. In OAuth terms...
Play Zork, Learn OAuth
In the early ’80s, some of the best “video” games were text-based adventures. These games would print out descriptive text of your surroundings and you would interact with the game using simple, but natural language commands like: “go north” or “take sword”. Fast forward some 30 years and a specification for an authorization framework called OAuth 2.0 was published. This framework allows an application to receive a token from an external party (like Okta) that...
Add Role-Based Access Control to Your App with Spring Security and Thymeleaf
User management functions are required by a wide variety of apps and APIs, and it’s a common use-case to partition access to parts of an application according to roles assigned to a user. This is the basis of role-based access control (RBAC). Okta manages these roles with groups. Users can belong to one or more groups. With the Okta Spring Security integration, these groups are automatically mapped to roles that can be called out in...
Secure a Spring Microservices Architecture with Spring Security, JWTs, Juiser, and Okta
You’ve built a microservices architecture with Spring Boot and Spring Cloud. You’re happy with the results, and you like how it adds resiliency to your application. You’re also pleased with how it scales and how different teams can deploy microservices independently. But what about security? Are you using Spring Security to lock everything down? Are your microservices locked down too, or are they just behind the firewall? This tutorial shows you how you can use...